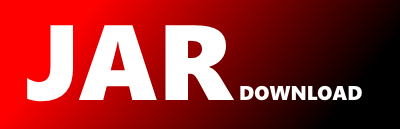
software.amazon.awscdk.services.lambda.CfnAlias Maven / Gradle / Ivy
Show all versions of lambda Show documentation
package software.amazon.awscdk.services.lambda;
/**
* A CloudFormation `AWS::Lambda::Alias`.
*
* The AWS::Lambda::Alias
resource creates an alias for a Lambda function version. Use aliases to provide clients with a function identifier that you can update to invoke a different version.
*
* You can also map an alias to split invocation requests between two versions. Use the RoutingConfig
parameter to specify a second version and the percentage of invocation requests that it receives.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lambda.*;
* CfnAlias cfnAlias = CfnAlias.Builder.create(this, "MyCfnAlias")
* .functionName("functionName")
* .functionVersion("functionVersion")
* .name("name")
* // the properties below are optional
* .description("description")
* .provisionedConcurrencyConfig(ProvisionedConcurrencyConfigurationProperty.builder()
* .provisionedConcurrentExecutions(123)
* .build())
* .routingConfig(AliasRoutingConfigurationProperty.builder()
* .additionalVersionWeights(List.of(VersionWeightProperty.builder()
* .functionVersion("functionVersion")
* .functionWeight(123)
* .build()))
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-22T19:35:44.020Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.CfnAlias")
public class CfnAlias extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnAlias(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnAlias(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.lambda.CfnAlias.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::Lambda::Alias`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnAlias(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.lambda.CfnAliasProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The name of the Lambda function.
*
* Name formats - Function name - MyFunction
.
*
*
* - Function ARN -
arn:aws:lambda:us-west-2:123456789012:function:MyFunction
.
* - Partial ARN -
123456789012:function:MyFunction
.
*
*
* The length constraint applies only to the full ARN. If you specify only the function name, it is limited to 64 characters in length.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getFunctionName() {
return software.amazon.jsii.Kernel.get(this, "functionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the Lambda function.
*
* Name formats - Function name - MyFunction
.
*
*
* - Function ARN -
arn:aws:lambda:us-west-2:123456789012:function:MyFunction
.
* - Partial ARN -
123456789012:function:MyFunction
.
*
*
* The length constraint applies only to the full ARN. If you specify only the function name, it is limited to 64 characters in length.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setFunctionName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "functionName", java.util.Objects.requireNonNull(value, "functionName is required"));
}
/**
* The function version that the alias invokes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getFunctionVersion() {
return software.amazon.jsii.Kernel.get(this, "functionVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The function version that the alias invokes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setFunctionVersion(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "functionVersion", java.util.Objects.requireNonNull(value, "functionVersion is required"));
}
/**
* The name of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getName() {
return software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "name", java.util.Objects.requireNonNull(value, "name is required"));
}
/**
* A description of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* A description of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
* Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getProvisionedConcurrencyConfig() {
return software.amazon.jsii.Kernel.get(this, "provisionedConcurrencyConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setProvisionedConcurrencyConfig(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.lambda.CfnAlias.ProvisionedConcurrencyConfigurationProperty value) {
software.amazon.jsii.Kernel.set(this, "provisionedConcurrencyConfig", value);
}
/**
* Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setProvisionedConcurrencyConfig(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "provisionedConcurrencyConfig", value);
}
/**
* The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getRoutingConfig() {
return software.amazon.jsii.Kernel.get(this, "routingConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setRoutingConfig(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "routingConfig", value);
}
/**
* The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setRoutingConfig(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.lambda.CfnAlias.AliasRoutingConfigurationProperty value) {
software.amazon.jsii.Kernel.set(this, "routingConfig", value);
}
/**
* The [traffic-shifting](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) configuration of a Lambda function alias.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lambda.*;
* AliasRoutingConfigurationProperty aliasRoutingConfigurationProperty = AliasRoutingConfigurationProperty.builder()
* .additionalVersionWeights(List.of(VersionWeightProperty.builder()
* .functionVersion("functionVersion")
* .functionWeight(123)
* .build()))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.CfnAlias.AliasRoutingConfigurationProperty")
@software.amazon.jsii.Jsii.Proxy(AliasRoutingConfigurationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface AliasRoutingConfigurationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The second version, and the percentage of traffic that's routed to it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getAdditionalVersionWeights();
/**
* @return a {@link Builder} of {@link AliasRoutingConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AliasRoutingConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object additionalVersionWeights;
/**
* Sets the value of {@link AliasRoutingConfigurationProperty#getAdditionalVersionWeights}
* @param additionalVersionWeights The second version, and the percentage of traffic that's routed to it. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder additionalVersionWeights(software.amazon.awscdk.core.IResolvable additionalVersionWeights) {
this.additionalVersionWeights = additionalVersionWeights;
return this;
}
/**
* Sets the value of {@link AliasRoutingConfigurationProperty#getAdditionalVersionWeights}
* @param additionalVersionWeights The second version, and the percentage of traffic that's routed to it. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder additionalVersionWeights(java.util.List extends java.lang.Object> additionalVersionWeights) {
this.additionalVersionWeights = additionalVersionWeights;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AliasRoutingConfigurationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AliasRoutingConfigurationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link AliasRoutingConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AliasRoutingConfigurationProperty {
private final java.lang.Object additionalVersionWeights;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.additionalVersionWeights = software.amazon.jsii.Kernel.get(this, "additionalVersionWeights", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.additionalVersionWeights = java.util.Objects.requireNonNull(builder.additionalVersionWeights, "additionalVersionWeights is required");
}
@Override
public final java.lang.Object getAdditionalVersionWeights() {
return this.additionalVersionWeights;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("additionalVersionWeights", om.valueToTree(this.getAdditionalVersionWeights()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.CfnAlias.AliasRoutingConfigurationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AliasRoutingConfigurationProperty.Jsii$Proxy that = (AliasRoutingConfigurationProperty.Jsii$Proxy) o;
return this.additionalVersionWeights.equals(that.additionalVersionWeights);
}
@Override
public final int hashCode() {
int result = this.additionalVersionWeights.hashCode();
return result;
}
}
}
/**
* A provisioned concurrency configuration for a function's alias.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lambda.*;
* ProvisionedConcurrencyConfigurationProperty provisionedConcurrencyConfigurationProperty = ProvisionedConcurrencyConfigurationProperty.builder()
* .provisionedConcurrentExecutions(123)
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.CfnAlias.ProvisionedConcurrencyConfigurationProperty")
@software.amazon.jsii.Jsii.Proxy(ProvisionedConcurrencyConfigurationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ProvisionedConcurrencyConfigurationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The amount of provisioned concurrency to allocate for the alias.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getProvisionedConcurrentExecutions();
/**
* @return a {@link Builder} of {@link ProvisionedConcurrencyConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ProvisionedConcurrencyConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Number provisionedConcurrentExecutions;
/**
* Sets the value of {@link ProvisionedConcurrencyConfigurationProperty#getProvisionedConcurrentExecutions}
* @param provisionedConcurrentExecutions The amount of provisioned concurrency to allocate for the alias. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder provisionedConcurrentExecutions(java.lang.Number provisionedConcurrentExecutions) {
this.provisionedConcurrentExecutions = provisionedConcurrentExecutions;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ProvisionedConcurrencyConfigurationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ProvisionedConcurrencyConfigurationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ProvisionedConcurrencyConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ProvisionedConcurrencyConfigurationProperty {
private final java.lang.Number provisionedConcurrentExecutions;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.provisionedConcurrentExecutions = software.amazon.jsii.Kernel.get(this, "provisionedConcurrentExecutions", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.provisionedConcurrentExecutions = java.util.Objects.requireNonNull(builder.provisionedConcurrentExecutions, "provisionedConcurrentExecutions is required");
}
@Override
public final java.lang.Number getProvisionedConcurrentExecutions() {
return this.provisionedConcurrentExecutions;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("provisionedConcurrentExecutions", om.valueToTree(this.getProvisionedConcurrentExecutions()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.CfnAlias.ProvisionedConcurrencyConfigurationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ProvisionedConcurrencyConfigurationProperty.Jsii$Proxy that = (ProvisionedConcurrencyConfigurationProperty.Jsii$Proxy) o;
return this.provisionedConcurrentExecutions.equals(that.provisionedConcurrentExecutions);
}
@Override
public final int hashCode() {
int result = this.provisionedConcurrentExecutions.hashCode();
return result;
}
}
}
/**
* The [traffic-shifting](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) configuration of a Lambda function alias.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lambda.*;
* VersionWeightProperty versionWeightProperty = VersionWeightProperty.builder()
* .functionVersion("functionVersion")
* .functionWeight(123)
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.CfnAlias.VersionWeightProperty")
@software.amazon.jsii.Jsii.Proxy(VersionWeightProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface VersionWeightProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The qualifier of the second version.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getFunctionVersion();
/**
* The percentage of traffic that the alias routes to the second version.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getFunctionWeight();
/**
* @return a {@link Builder} of {@link VersionWeightProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link VersionWeightProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String functionVersion;
java.lang.Number functionWeight;
/**
* Sets the value of {@link VersionWeightProperty#getFunctionVersion}
* @param functionVersion The qualifier of the second version. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionVersion(java.lang.String functionVersion) {
this.functionVersion = functionVersion;
return this;
}
/**
* Sets the value of {@link VersionWeightProperty#getFunctionWeight}
* @param functionWeight The percentage of traffic that the alias routes to the second version. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionWeight(java.lang.Number functionWeight) {
this.functionWeight = functionWeight;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link VersionWeightProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public VersionWeightProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link VersionWeightProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements VersionWeightProperty {
private final java.lang.String functionVersion;
private final java.lang.Number functionWeight;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.functionVersion = software.amazon.jsii.Kernel.get(this, "functionVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.functionWeight = software.amazon.jsii.Kernel.get(this, "functionWeight", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.functionVersion = java.util.Objects.requireNonNull(builder.functionVersion, "functionVersion is required");
this.functionWeight = java.util.Objects.requireNonNull(builder.functionWeight, "functionWeight is required");
}
@Override
public final java.lang.String getFunctionVersion() {
return this.functionVersion;
}
@Override
public final java.lang.Number getFunctionWeight() {
return this.functionWeight;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("functionVersion", om.valueToTree(this.getFunctionVersion()));
data.set("functionWeight", om.valueToTree(this.getFunctionWeight()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.CfnAlias.VersionWeightProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
VersionWeightProperty.Jsii$Proxy that = (VersionWeightProperty.Jsii$Proxy) o;
if (!functionVersion.equals(that.functionVersion)) return false;
return this.functionWeight.equals(that.functionWeight);
}
@Override
public final int hashCode() {
int result = this.functionVersion.hashCode();
result = 31 * result + (this.functionWeight.hashCode());
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.lambda.CfnAlias}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.lambda.CfnAliasProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.lambda.CfnAliasProps.Builder();
}
/**
* The name of the Lambda function.
*
* Name formats - Function name - MyFunction
.
*
*
* - Function ARN -
arn:aws:lambda:us-west-2:123456789012:function:MyFunction
.
* - Partial ARN -
123456789012:function:MyFunction
.
*
*
* The length constraint applies only to the full ARN. If you specify only the function name, it is limited to 64 characters in length.
*
* @return {@code this}
* @param functionName The name of the Lambda function. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionName(final java.lang.String functionName) {
this.props.functionName(functionName);
return this;
}
/**
* The function version that the alias invokes.
*
* @return {@code this}
* @param functionVersion The function version that the alias invokes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionVersion(final java.lang.String functionVersion) {
this.props.functionVersion(functionVersion);
return this;
}
/**
* The name of the alias.
*
* @return {@code this}
* @param name The name of the alias. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(final java.lang.String name) {
this.props.name(name);
return this;
}
/**
* A description of the alias.
*
* @return {@code this}
* @param description A description of the alias. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias.
*
* @return {@code this}
* @param provisionedConcurrencyConfig Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder provisionedConcurrencyConfig(final software.amazon.awscdk.services.lambda.CfnAlias.ProvisionedConcurrencyConfigurationProperty provisionedConcurrencyConfig) {
this.props.provisionedConcurrencyConfig(provisionedConcurrencyConfig);
return this;
}
/**
* Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias.
*
* @return {@code this}
* @param provisionedConcurrencyConfig Specifies a [provisioned concurrency](https://docs.aws.amazon.com/lambda/latest/dg/configuration-concurrency.html) configuration for a function's alias. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder provisionedConcurrencyConfig(final software.amazon.awscdk.core.IResolvable provisionedConcurrencyConfig) {
this.props.provisionedConcurrencyConfig(provisionedConcurrencyConfig);
return this;
}
/**
* The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias.
*
* @return {@code this}
* @param routingConfig The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder routingConfig(final software.amazon.awscdk.core.IResolvable routingConfig) {
this.props.routingConfig(routingConfig);
return this;
}
/**
* The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias.
*
* @return {@code this}
* @param routingConfig The [routing configuration](https://docs.aws.amazon.com/lambda/latest/dg/lambda-traffic-shifting-using-aliases.html) of the alias. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder routingConfig(final software.amazon.awscdk.services.lambda.CfnAlias.AliasRoutingConfigurationProperty routingConfig) {
this.props.routingConfig(routingConfig);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.lambda.CfnAlias}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.lambda.CfnAlias build() {
return new software.amazon.awscdk.services.lambda.CfnAlias(
this.scope,
this.id,
this.props.build()
);
}
}
}