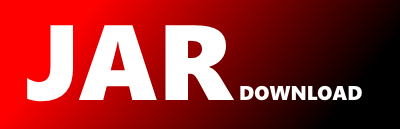
software.amazon.awscdk.services.lambda.LayerVersionProps Maven / Gradle / Ivy
Show all versions of lambda Show documentation
package software.amazon.awscdk.services.lambda;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.21.1 (build 9ff44cb)", date = "2020-01-16T18:34:06.087Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.LayerVersionProps")
@software.amazon.jsii.Jsii.Proxy(LayerVersionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface LayerVersionProps extends software.amazon.jsii.JsiiSerializable {
/**
* The content of this Layer.
*
* Using Code.fromInline
is not supported.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
software.amazon.awscdk.services.lambda.Code getCode();
/**
* The runtimes compatible with this Layer.
*
* Default: - All runtimes are supported.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.util.List getCompatibleRuntimes() {
return null;
}
/**
* The description the this Lambda Layer.
*
* Default: - No description.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.String getDescription() {
return null;
}
/**
* The name of the layer.
*
* Default: - A name will be generated.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.String getLayerVersionName() {
return null;
}
/**
* The SPDX licence identifier or URL to the license file for this layer.
*
* Default: - No license information will be recorded.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.String getLicense() {
return null;
}
/**
* @return a {@link Builder} of {@link LayerVersionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link LayerVersionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private software.amazon.awscdk.services.lambda.Code code;
private java.util.List compatibleRuntimes;
private java.lang.String description;
private java.lang.String layerVersionName;
private java.lang.String license;
/**
* Sets the value of {@link LayerVersionProps#getCode}
* @param code The content of this Layer. This parameter is required.
* Using Code.fromInline
is not supported.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder code(software.amazon.awscdk.services.lambda.Code code) {
this.code = code;
return this;
}
/**
* Sets the value of {@link LayerVersionProps#getCompatibleRuntimes}
* @param compatibleRuntimes The runtimes compatible with this Layer.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder compatibleRuntimes(java.util.List compatibleRuntimes) {
this.compatibleRuntimes = compatibleRuntimes;
return this;
}
/**
* Sets the value of {@link LayerVersionProps#getDescription}
* @param description The description the this Lambda Layer.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link LayerVersionProps#getLayerVersionName}
* @param layerVersionName The name of the layer.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder layerVersionName(java.lang.String layerVersionName) {
this.layerVersionName = layerVersionName;
return this;
}
/**
* Sets the value of {@link LayerVersionProps#getLicense}
* @param license The SPDX licence identifier or URL to the license file for this layer.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder license(java.lang.String license) {
this.license = license;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link LayerVersionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public LayerVersionProps build() {
return new Jsii$Proxy(code, compatibleRuntimes, description, layerVersionName, license);
}
}
/**
* An implementation for {@link LayerVersionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements LayerVersionProps {
private final software.amazon.awscdk.services.lambda.Code code;
private final java.util.List compatibleRuntimes;
private final java.lang.String description;
private final java.lang.String layerVersionName;
private final java.lang.String license;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.code = this.jsiiGet("code", software.amazon.awscdk.services.lambda.Code.class);
this.compatibleRuntimes = this.jsiiGet("compatibleRuntimes", java.util.List.class);
this.description = this.jsiiGet("description", java.lang.String.class);
this.layerVersionName = this.jsiiGet("layerVersionName", java.lang.String.class);
this.license = this.jsiiGet("license", java.lang.String.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final software.amazon.awscdk.services.lambda.Code code, final java.util.List compatibleRuntimes, final java.lang.String description, final java.lang.String layerVersionName, final java.lang.String license) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.code = java.util.Objects.requireNonNull(code, "code is required");
this.compatibleRuntimes = compatibleRuntimes;
this.description = description;
this.layerVersionName = layerVersionName;
this.license = license;
}
@Override
public software.amazon.awscdk.services.lambda.Code getCode() {
return this.code;
}
@Override
public java.util.List getCompatibleRuntimes() {
return this.compatibleRuntimes;
}
@Override
public java.lang.String getDescription() {
return this.description;
}
@Override
public java.lang.String getLayerVersionName() {
return this.layerVersionName;
}
@Override
public java.lang.String getLicense() {
return this.license;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("code", om.valueToTree(this.getCode()));
if (this.getCompatibleRuntimes() != null) {
data.set("compatibleRuntimes", om.valueToTree(this.getCompatibleRuntimes()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getLayerVersionName() != null) {
data.set("layerVersionName", om.valueToTree(this.getLayerVersionName()));
}
if (this.getLicense() != null) {
data.set("license", om.valueToTree(this.getLicense()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.LayerVersionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LayerVersionProps.Jsii$Proxy that = (LayerVersionProps.Jsii$Proxy) o;
if (!code.equals(that.code)) return false;
if (this.compatibleRuntimes != null ? !this.compatibleRuntimes.equals(that.compatibleRuntimes) : that.compatibleRuntimes != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.layerVersionName != null ? !this.layerVersionName.equals(that.layerVersionName) : that.layerVersionName != null) return false;
return this.license != null ? this.license.equals(that.license) : that.license == null;
}
@Override
public int hashCode() {
int result = this.code.hashCode();
result = 31 * result + (this.compatibleRuntimes != null ? this.compatibleRuntimes.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.layerVersionName != null ? this.layerVersionName.hashCode() : 0);
result = 31 * result + (this.license != null ? this.license.hashCode() : 0);
return result;
}
}
}