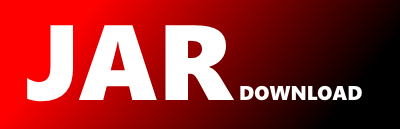
software.amazon.awscdk.services.lambda.SingletonFunctionProps Maven / Gradle / Ivy
Show all versions of lambda Show documentation
package software.amazon.awscdk.services.lambda;
/**
* Properties for a newly created singleton Lambda.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.21.1 (build 9ff44cb)", date = "2020-01-16T18:34:06.091Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.SingletonFunctionProps")
@software.amazon.jsii.Jsii.Proxy(SingletonFunctionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface SingletonFunctionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.lambda.FunctionProps {
/**
* A unique identifier to identify this lambda.
*
* The identifier should be unique across all custom resource providers.
* We recommend generating a UUID per provider.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getUuid();
/**
* A descriptive name for the purpose of this Lambda.
*
* If the Lambda does not have a physical name, this string will be
* reflected its generated name. The combination of lambdaPurpose
* and uuid must be unique.
*
* Default: SingletonLambda
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.String getLambdaPurpose() {
return null;
}
/**
* @return a {@link Builder} of {@link SingletonFunctionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SingletonFunctionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.lang.String uuid;
private java.lang.String lambdaPurpose;
private software.amazon.awscdk.services.lambda.Code code;
private java.lang.String handler;
private software.amazon.awscdk.services.lambda.Runtime runtime;
private java.lang.Boolean allowAllOutbound;
private software.amazon.awscdk.services.sqs.IQueue deadLetterQueue;
private java.lang.Boolean deadLetterQueueEnabled;
private java.lang.String description;
private java.util.Map environment;
private java.util.List events;
private java.lang.String functionName;
private java.util.List initialPolicy;
private java.util.List layers;
private software.amazon.awscdk.services.logs.RetentionDays logRetention;
private software.amazon.awscdk.services.iam.IRole logRetentionRole;
private java.lang.Number memorySize;
private java.lang.Number reservedConcurrentExecutions;
private software.amazon.awscdk.services.iam.IRole role;
private software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
private java.util.List securityGroups;
private software.amazon.awscdk.core.Duration timeout;
private software.amazon.awscdk.services.lambda.Tracing tracing;
private software.amazon.awscdk.services.ec2.IVpc vpc;
private software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
private software.amazon.awscdk.core.Duration maxEventAge;
private software.amazon.awscdk.services.lambda.IDestination onFailure;
private software.amazon.awscdk.services.lambda.IDestination onSuccess;
private java.lang.Number retryAttempts;
/**
* Sets the value of {@link SingletonFunctionProps#getUuid}
* @param uuid A unique identifier to identify this lambda. This parameter is required.
* The identifier should be unique across all custom resource providers.
* We recommend generating a UUID per provider.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder uuid(java.lang.String uuid) {
this.uuid = uuid;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getLambdaPurpose}
* @param lambdaPurpose A descriptive name for the purpose of this Lambda.
* If the Lambda does not have a physical name, this string will be
* reflected its generated name. The combination of lambdaPurpose
* and uuid must be unique.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder lambdaPurpose(java.lang.String lambdaPurpose) {
this.lambdaPurpose = lambdaPurpose;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getCode}
* @param code The source code of your Lambda function. This parameter is required.
* You can point to a file in an
* Amazon Simple Storage Service (Amazon S3) bucket or specify your source
* code as inline text.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder code(software.amazon.awscdk.services.lambda.Code code) {
this.code = code;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getHandler}
* @param handler The name of the method within your code that Lambda calls to execute your function. This parameter is required.
* The format includes the file name. It can also include
* namespaces and other qualifiers, depending on the runtime.
* For more information, see https://docs.aws.amazon.com/lambda/latest/dg/gettingstarted-features.html#gettingstarted-features-programmingmodel.
*
* NOTE: If you specify your source code as inline text by specifying the
* ZipFile property within the Code property, specify index.function_name as
* the handler.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder handler(java.lang.String handler) {
this.handler = handler;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getRuntime}
* @param runtime The runtime environment for the Lambda function that you are uploading. This parameter is required.
* For valid values, see the Runtime property in the AWS Lambda Developer
* Guide.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runtime(software.amazon.awscdk.services.lambda.Runtime runtime) {
this.runtime = runtime;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getAllowAllOutbound}
* @param allowAllOutbound Whether to allow the Lambda to send all network traffic.
* If set to false, you must individually add traffic rules to allow the
* Lambda to connect to network targets.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder allowAllOutbound(java.lang.Boolean allowAllOutbound) {
this.allowAllOutbound = allowAllOutbound;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getDeadLetterQueue}
* @param deadLetterQueue The SQS queue to use if DLQ is enabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deadLetterQueue(software.amazon.awscdk.services.sqs.IQueue deadLetterQueue) {
this.deadLetterQueue = deadLetterQueue;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getDeadLetterQueueEnabled}
* @param deadLetterQueueEnabled Enabled DLQ.
* If deadLetterQueue
is undefined,
* an SQS queue with default options will be defined for your Function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deadLetterQueueEnabled(java.lang.Boolean deadLetterQueueEnabled) {
this.deadLetterQueueEnabled = deadLetterQueueEnabled;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getDescription}
* @param description A description of the function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getEnvironment}
* @param environment Key-value pairs that Lambda caches and makes available for your Lambda functions.
* Use environment variables to apply configuration changes, such
* as test and production environment configurations, without changing your
* Lambda function source code.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environment(java.util.Map environment) {
this.environment = environment;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getEvents}
* @param events Event sources for this function.
* You can also add event sources using addEventSource
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder events(java.util.List events) {
this.events = events;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getFunctionName}
* @param functionName A name for the function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionName(java.lang.String functionName) {
this.functionName = functionName;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getInitialPolicy}
* @param initialPolicy Initial policy statements to add to the created Lambda Role.
* You can call addToRolePolicy
to the created lambda to add statements post creation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder initialPolicy(java.util.List initialPolicy) {
this.initialPolicy = initialPolicy;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getLayers}
* @param layers A list of layers to add to the function's execution environment.
* You can configure your Lambda function to pull in
* additional code during initialization in the form of layers. Layers are packages of libraries or other dependencies
* that can be used by mulitple functions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder layers(java.util.List layers) {
this.layers = layers;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getLogRetention}
* @param logRetention The number of days log events are kept in CloudWatch Logs.
* When updating
* this property, unsetting it doesn't remove the log retention policy. To
* remove the retention policy, set the value to Infinity
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder logRetention(software.amazon.awscdk.services.logs.RetentionDays logRetention) {
this.logRetention = logRetention;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getLogRetentionRole}
* @param logRetentionRole The IAM role for the Lambda function associated with the custom resource that sets the retention policy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder logRetentionRole(software.amazon.awscdk.services.iam.IRole logRetentionRole) {
this.logRetentionRole = logRetentionRole;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getMemorySize}
* @param memorySize The amount of memory, in MB, that is allocated to your Lambda function.
* Lambda uses this value to proportionally allocate the amount of CPU
* power. For more information, see Resource Model in the AWS Lambda
* Developer Guide.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memorySize(java.lang.Number memorySize) {
this.memorySize = memorySize;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getReservedConcurrentExecutions}
* @param reservedConcurrentExecutions The maximum of concurrent executions you want to reserve for the function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder reservedConcurrentExecutions(java.lang.Number reservedConcurrentExecutions) {
this.reservedConcurrentExecutions = reservedConcurrentExecutions;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getRole}
* @param role Lambda execution role.
* This is the role that will be assumed by the function upon execution.
* It controls the permissions that the function will have. The Role must
* be assumable by the 'lambda.amazonaws.com' service principal.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getSecurityGroup}
* @param securityGroup What security group to associate with the Lambda's network interfaces. This property is being deprecated, consider using securityGroups instead.
* Only used if 'vpc' is supplied.
*
* Use securityGroups property instead.
* Function constructor will throw an error if both are specified.
* @return {@code this}
* @deprecated - This property is deprecated, use securityGroups instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder securityGroup(software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup) {
this.securityGroup = securityGroup;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getSecurityGroups}
* @param securityGroups The list of security groups to associate with the Lambda's network interfaces.
* Only used if 'vpc' is supplied.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityGroups(java.util.List securityGroups) {
this.securityGroups = securityGroups;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getTimeout}
* @param timeout The function execution time (in seconds) after which Lambda terminates the function.
* Because the execution time affects cost, set this value
* based on the function's expected execution time.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timeout(software.amazon.awscdk.core.Duration timeout) {
this.timeout = timeout;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getTracing}
* @param tracing Enable AWS X-Ray Tracing for Lambda Function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tracing(software.amazon.awscdk.services.lambda.Tracing tracing) {
this.tracing = tracing;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getVpc}
* @param vpc VPC network to place Lambda network interfaces.
* Specify this if the Lambda function needs to access resources in a VPC.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpc(software.amazon.awscdk.services.ec2.IVpc vpc) {
this.vpc = vpc;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getVpcSubnets}
* @param vpcSubnets Where to place the network interfaces within the VPC.
* Only used if 'vpc' is supplied. Note: internet access for Lambdas
* requires a NAT gateway, so picking Public subnets is not allowed.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getMaxEventAge}
* @param maxEventAge The maximum age of a request that Lambda sends to a function for processing.
* Minimum: 60 seconds
* Maximum: 6 hours
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxEventAge(software.amazon.awscdk.core.Duration maxEventAge) {
this.maxEventAge = maxEventAge;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getOnFailure}
* @param onFailure The destination for failed invocations.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onFailure(software.amazon.awscdk.services.lambda.IDestination onFailure) {
this.onFailure = onFailure;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getOnSuccess}
* @param onSuccess The destination for successful invocations.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onSuccess(software.amazon.awscdk.services.lambda.IDestination onSuccess) {
this.onSuccess = onSuccess;
return this;
}
/**
* Sets the value of {@link SingletonFunctionProps#getRetryAttempts}
* @param retryAttempts The maximum number of times to retry when the function returns an error.
* Minimum: 0
* Maximum: 2
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder retryAttempts(java.lang.Number retryAttempts) {
this.retryAttempts = retryAttempts;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SingletonFunctionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public SingletonFunctionProps build() {
return new Jsii$Proxy(uuid, lambdaPurpose, code, handler, runtime, allowAllOutbound, deadLetterQueue, deadLetterQueueEnabled, description, environment, events, functionName, initialPolicy, layers, logRetention, logRetentionRole, memorySize, reservedConcurrentExecutions, role, securityGroup, securityGroups, timeout, tracing, vpc, vpcSubnets, maxEventAge, onFailure, onSuccess, retryAttempts);
}
}
/**
* An implementation for {@link SingletonFunctionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements SingletonFunctionProps {
private final java.lang.String uuid;
private final java.lang.String lambdaPurpose;
private final software.amazon.awscdk.services.lambda.Code code;
private final java.lang.String handler;
private final software.amazon.awscdk.services.lambda.Runtime runtime;
private final java.lang.Boolean allowAllOutbound;
private final software.amazon.awscdk.services.sqs.IQueue deadLetterQueue;
private final java.lang.Boolean deadLetterQueueEnabled;
private final java.lang.String description;
private final java.util.Map environment;
private final java.util.List events;
private final java.lang.String functionName;
private final java.util.List initialPolicy;
private final java.util.List layers;
private final software.amazon.awscdk.services.logs.RetentionDays logRetention;
private final software.amazon.awscdk.services.iam.IRole logRetentionRole;
private final java.lang.Number memorySize;
private final java.lang.Number reservedConcurrentExecutions;
private final software.amazon.awscdk.services.iam.IRole role;
private final software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
private final java.util.List securityGroups;
private final software.amazon.awscdk.core.Duration timeout;
private final software.amazon.awscdk.services.lambda.Tracing tracing;
private final software.amazon.awscdk.services.ec2.IVpc vpc;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
private final software.amazon.awscdk.core.Duration maxEventAge;
private final software.amazon.awscdk.services.lambda.IDestination onFailure;
private final software.amazon.awscdk.services.lambda.IDestination onSuccess;
private final java.lang.Number retryAttempts;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.uuid = this.jsiiGet("uuid", java.lang.String.class);
this.lambdaPurpose = this.jsiiGet("lambdaPurpose", java.lang.String.class);
this.code = this.jsiiGet("code", software.amazon.awscdk.services.lambda.Code.class);
this.handler = this.jsiiGet("handler", java.lang.String.class);
this.runtime = this.jsiiGet("runtime", software.amazon.awscdk.services.lambda.Runtime.class);
this.allowAllOutbound = this.jsiiGet("allowAllOutbound", java.lang.Boolean.class);
this.deadLetterQueue = this.jsiiGet("deadLetterQueue", software.amazon.awscdk.services.sqs.IQueue.class);
this.deadLetterQueueEnabled = this.jsiiGet("deadLetterQueueEnabled", java.lang.Boolean.class);
this.description = this.jsiiGet("description", java.lang.String.class);
this.environment = this.jsiiGet("environment", java.util.Map.class);
this.events = this.jsiiGet("events", java.util.List.class);
this.functionName = this.jsiiGet("functionName", java.lang.String.class);
this.initialPolicy = this.jsiiGet("initialPolicy", java.util.List.class);
this.layers = this.jsiiGet("layers", java.util.List.class);
this.logRetention = this.jsiiGet("logRetention", software.amazon.awscdk.services.logs.RetentionDays.class);
this.logRetentionRole = this.jsiiGet("logRetentionRole", software.amazon.awscdk.services.iam.IRole.class);
this.memorySize = this.jsiiGet("memorySize", java.lang.Number.class);
this.reservedConcurrentExecutions = this.jsiiGet("reservedConcurrentExecutions", java.lang.Number.class);
this.role = this.jsiiGet("role", software.amazon.awscdk.services.iam.IRole.class);
this.securityGroup = this.jsiiGet("securityGroup", software.amazon.awscdk.services.ec2.ISecurityGroup.class);
this.securityGroups = this.jsiiGet("securityGroups", java.util.List.class);
this.timeout = this.jsiiGet("timeout", software.amazon.awscdk.core.Duration.class);
this.tracing = this.jsiiGet("tracing", software.amazon.awscdk.services.lambda.Tracing.class);
this.vpc = this.jsiiGet("vpc", software.amazon.awscdk.services.ec2.IVpc.class);
this.vpcSubnets = this.jsiiGet("vpcSubnets", software.amazon.awscdk.services.ec2.SubnetSelection.class);
this.maxEventAge = this.jsiiGet("maxEventAge", software.amazon.awscdk.core.Duration.class);
this.onFailure = this.jsiiGet("onFailure", software.amazon.awscdk.services.lambda.IDestination.class);
this.onSuccess = this.jsiiGet("onSuccess", software.amazon.awscdk.services.lambda.IDestination.class);
this.retryAttempts = this.jsiiGet("retryAttempts", java.lang.Number.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.lang.String uuid, final java.lang.String lambdaPurpose, final software.amazon.awscdk.services.lambda.Code code, final java.lang.String handler, final software.amazon.awscdk.services.lambda.Runtime runtime, final java.lang.Boolean allowAllOutbound, final software.amazon.awscdk.services.sqs.IQueue deadLetterQueue, final java.lang.Boolean deadLetterQueueEnabled, final java.lang.String description, final java.util.Map environment, final java.util.List events, final java.lang.String functionName, final java.util.List initialPolicy, final java.util.List layers, final software.amazon.awscdk.services.logs.RetentionDays logRetention, final software.amazon.awscdk.services.iam.IRole logRetentionRole, final java.lang.Number memorySize, final java.lang.Number reservedConcurrentExecutions, final software.amazon.awscdk.services.iam.IRole role, final software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup, final java.util.List securityGroups, final software.amazon.awscdk.core.Duration timeout, final software.amazon.awscdk.services.lambda.Tracing tracing, final software.amazon.awscdk.services.ec2.IVpc vpc, final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets, final software.amazon.awscdk.core.Duration maxEventAge, final software.amazon.awscdk.services.lambda.IDestination onFailure, final software.amazon.awscdk.services.lambda.IDestination onSuccess, final java.lang.Number retryAttempts) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.uuid = java.util.Objects.requireNonNull(uuid, "uuid is required");
this.lambdaPurpose = lambdaPurpose;
this.code = java.util.Objects.requireNonNull(code, "code is required");
this.handler = java.util.Objects.requireNonNull(handler, "handler is required");
this.runtime = java.util.Objects.requireNonNull(runtime, "runtime is required");
this.allowAllOutbound = allowAllOutbound;
this.deadLetterQueue = deadLetterQueue;
this.deadLetterQueueEnabled = deadLetterQueueEnabled;
this.description = description;
this.environment = environment;
this.events = events;
this.functionName = functionName;
this.initialPolicy = initialPolicy;
this.layers = layers;
this.logRetention = logRetention;
this.logRetentionRole = logRetentionRole;
this.memorySize = memorySize;
this.reservedConcurrentExecutions = reservedConcurrentExecutions;
this.role = role;
this.securityGroup = securityGroup;
this.securityGroups = securityGroups;
this.timeout = timeout;
this.tracing = tracing;
this.vpc = vpc;
this.vpcSubnets = vpcSubnets;
this.maxEventAge = maxEventAge;
this.onFailure = onFailure;
this.onSuccess = onSuccess;
this.retryAttempts = retryAttempts;
}
@Override
public java.lang.String getUuid() {
return this.uuid;
}
@Override
public java.lang.String getLambdaPurpose() {
return this.lambdaPurpose;
}
@Override
public software.amazon.awscdk.services.lambda.Code getCode() {
return this.code;
}
@Override
public java.lang.String getHandler() {
return this.handler;
}
@Override
public software.amazon.awscdk.services.lambda.Runtime getRuntime() {
return this.runtime;
}
@Override
public java.lang.Boolean getAllowAllOutbound() {
return this.allowAllOutbound;
}
@Override
public software.amazon.awscdk.services.sqs.IQueue getDeadLetterQueue() {
return this.deadLetterQueue;
}
@Override
public java.lang.Boolean getDeadLetterQueueEnabled() {
return this.deadLetterQueueEnabled;
}
@Override
public java.lang.String getDescription() {
return this.description;
}
@Override
public java.util.Map getEnvironment() {
return this.environment;
}
@Override
public java.util.List getEvents() {
return this.events;
}
@Override
public java.lang.String getFunctionName() {
return this.functionName;
}
@Override
public java.util.List getInitialPolicy() {
return this.initialPolicy;
}
@Override
public java.util.List getLayers() {
return this.layers;
}
@Override
public software.amazon.awscdk.services.logs.RetentionDays getLogRetention() {
return this.logRetention;
}
@Override
public software.amazon.awscdk.services.iam.IRole getLogRetentionRole() {
return this.logRetentionRole;
}
@Override
public java.lang.Number getMemorySize() {
return this.memorySize;
}
@Override
public java.lang.Number getReservedConcurrentExecutions() {
return this.reservedConcurrentExecutions;
}
@Override
public software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup() {
return this.securityGroup;
}
@Override
public java.util.List getSecurityGroups() {
return this.securityGroups;
}
@Override
public software.amazon.awscdk.core.Duration getTimeout() {
return this.timeout;
}
@Override
public software.amazon.awscdk.services.lambda.Tracing getTracing() {
return this.tracing;
}
@Override
public software.amazon.awscdk.services.ec2.IVpc getVpc() {
return this.vpc;
}
@Override
public software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
public software.amazon.awscdk.core.Duration getMaxEventAge() {
return this.maxEventAge;
}
@Override
public software.amazon.awscdk.services.lambda.IDestination getOnFailure() {
return this.onFailure;
}
@Override
public software.amazon.awscdk.services.lambda.IDestination getOnSuccess() {
return this.onSuccess;
}
@Override
public java.lang.Number getRetryAttempts() {
return this.retryAttempts;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("uuid", om.valueToTree(this.getUuid()));
if (this.getLambdaPurpose() != null) {
data.set("lambdaPurpose", om.valueToTree(this.getLambdaPurpose()));
}
data.set("code", om.valueToTree(this.getCode()));
data.set("handler", om.valueToTree(this.getHandler()));
data.set("runtime", om.valueToTree(this.getRuntime()));
if (this.getAllowAllOutbound() != null) {
data.set("allowAllOutbound", om.valueToTree(this.getAllowAllOutbound()));
}
if (this.getDeadLetterQueue() != null) {
data.set("deadLetterQueue", om.valueToTree(this.getDeadLetterQueue()));
}
if (this.getDeadLetterQueueEnabled() != null) {
data.set("deadLetterQueueEnabled", om.valueToTree(this.getDeadLetterQueueEnabled()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getEnvironment() != null) {
data.set("environment", om.valueToTree(this.getEnvironment()));
}
if (this.getEvents() != null) {
data.set("events", om.valueToTree(this.getEvents()));
}
if (this.getFunctionName() != null) {
data.set("functionName", om.valueToTree(this.getFunctionName()));
}
if (this.getInitialPolicy() != null) {
data.set("initialPolicy", om.valueToTree(this.getInitialPolicy()));
}
if (this.getLayers() != null) {
data.set("layers", om.valueToTree(this.getLayers()));
}
if (this.getLogRetention() != null) {
data.set("logRetention", om.valueToTree(this.getLogRetention()));
}
if (this.getLogRetentionRole() != null) {
data.set("logRetentionRole", om.valueToTree(this.getLogRetentionRole()));
}
if (this.getMemorySize() != null) {
data.set("memorySize", om.valueToTree(this.getMemorySize()));
}
if (this.getReservedConcurrentExecutions() != null) {
data.set("reservedConcurrentExecutions", om.valueToTree(this.getReservedConcurrentExecutions()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
if (this.getSecurityGroup() != null) {
data.set("securityGroup", om.valueToTree(this.getSecurityGroup()));
}
if (this.getSecurityGroups() != null) {
data.set("securityGroups", om.valueToTree(this.getSecurityGroups()));
}
if (this.getTimeout() != null) {
data.set("timeout", om.valueToTree(this.getTimeout()));
}
if (this.getTracing() != null) {
data.set("tracing", om.valueToTree(this.getTracing()));
}
if (this.getVpc() != null) {
data.set("vpc", om.valueToTree(this.getVpc()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
if (this.getMaxEventAge() != null) {
data.set("maxEventAge", om.valueToTree(this.getMaxEventAge()));
}
if (this.getOnFailure() != null) {
data.set("onFailure", om.valueToTree(this.getOnFailure()));
}
if (this.getOnSuccess() != null) {
data.set("onSuccess", om.valueToTree(this.getOnSuccess()));
}
if (this.getRetryAttempts() != null) {
data.set("retryAttempts", om.valueToTree(this.getRetryAttempts()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.SingletonFunctionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SingletonFunctionProps.Jsii$Proxy that = (SingletonFunctionProps.Jsii$Proxy) o;
if (!uuid.equals(that.uuid)) return false;
if (this.lambdaPurpose != null ? !this.lambdaPurpose.equals(that.lambdaPurpose) : that.lambdaPurpose != null) return false;
if (!code.equals(that.code)) return false;
if (!handler.equals(that.handler)) return false;
if (!runtime.equals(that.runtime)) return false;
if (this.allowAllOutbound != null ? !this.allowAllOutbound.equals(that.allowAllOutbound) : that.allowAllOutbound != null) return false;
if (this.deadLetterQueue != null ? !this.deadLetterQueue.equals(that.deadLetterQueue) : that.deadLetterQueue != null) return false;
if (this.deadLetterQueueEnabled != null ? !this.deadLetterQueueEnabled.equals(that.deadLetterQueueEnabled) : that.deadLetterQueueEnabled != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.environment != null ? !this.environment.equals(that.environment) : that.environment != null) return false;
if (this.events != null ? !this.events.equals(that.events) : that.events != null) return false;
if (this.functionName != null ? !this.functionName.equals(that.functionName) : that.functionName != null) return false;
if (this.initialPolicy != null ? !this.initialPolicy.equals(that.initialPolicy) : that.initialPolicy != null) return false;
if (this.layers != null ? !this.layers.equals(that.layers) : that.layers != null) return false;
if (this.logRetention != null ? !this.logRetention.equals(that.logRetention) : that.logRetention != null) return false;
if (this.logRetentionRole != null ? !this.logRetentionRole.equals(that.logRetentionRole) : that.logRetentionRole != null) return false;
if (this.memorySize != null ? !this.memorySize.equals(that.memorySize) : that.memorySize != null) return false;
if (this.reservedConcurrentExecutions != null ? !this.reservedConcurrentExecutions.equals(that.reservedConcurrentExecutions) : that.reservedConcurrentExecutions != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (this.securityGroup != null ? !this.securityGroup.equals(that.securityGroup) : that.securityGroup != null) return false;
if (this.securityGroups != null ? !this.securityGroups.equals(that.securityGroups) : that.securityGroups != null) return false;
if (this.timeout != null ? !this.timeout.equals(that.timeout) : that.timeout != null) return false;
if (this.tracing != null ? !this.tracing.equals(that.tracing) : that.tracing != null) return false;
if (this.vpc != null ? !this.vpc.equals(that.vpc) : that.vpc != null) return false;
if (this.vpcSubnets != null ? !this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets != null) return false;
if (this.maxEventAge != null ? !this.maxEventAge.equals(that.maxEventAge) : that.maxEventAge != null) return false;
if (this.onFailure != null ? !this.onFailure.equals(that.onFailure) : that.onFailure != null) return false;
if (this.onSuccess != null ? !this.onSuccess.equals(that.onSuccess) : that.onSuccess != null) return false;
return this.retryAttempts != null ? this.retryAttempts.equals(that.retryAttempts) : that.retryAttempts == null;
}
@Override
public int hashCode() {
int result = this.uuid.hashCode();
result = 31 * result + (this.lambdaPurpose != null ? this.lambdaPurpose.hashCode() : 0);
result = 31 * result + (this.code.hashCode());
result = 31 * result + (this.handler.hashCode());
result = 31 * result + (this.runtime.hashCode());
result = 31 * result + (this.allowAllOutbound != null ? this.allowAllOutbound.hashCode() : 0);
result = 31 * result + (this.deadLetterQueue != null ? this.deadLetterQueue.hashCode() : 0);
result = 31 * result + (this.deadLetterQueueEnabled != null ? this.deadLetterQueueEnabled.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.environment != null ? this.environment.hashCode() : 0);
result = 31 * result + (this.events != null ? this.events.hashCode() : 0);
result = 31 * result + (this.functionName != null ? this.functionName.hashCode() : 0);
result = 31 * result + (this.initialPolicy != null ? this.initialPolicy.hashCode() : 0);
result = 31 * result + (this.layers != null ? this.layers.hashCode() : 0);
result = 31 * result + (this.logRetention != null ? this.logRetention.hashCode() : 0);
result = 31 * result + (this.logRetentionRole != null ? this.logRetentionRole.hashCode() : 0);
result = 31 * result + (this.memorySize != null ? this.memorySize.hashCode() : 0);
result = 31 * result + (this.reservedConcurrentExecutions != null ? this.reservedConcurrentExecutions.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.securityGroup != null ? this.securityGroup.hashCode() : 0);
result = 31 * result + (this.securityGroups != null ? this.securityGroups.hashCode() : 0);
result = 31 * result + (this.timeout != null ? this.timeout.hashCode() : 0);
result = 31 * result + (this.tracing != null ? this.tracing.hashCode() : 0);
result = 31 * result + (this.vpc != null ? this.vpc.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
result = 31 * result + (this.maxEventAge != null ? this.maxEventAge.hashCode() : 0);
result = 31 * result + (this.onFailure != null ? this.onFailure.hashCode() : 0);
result = 31 * result + (this.onSuccess != null ? this.onSuccess.hashCode() : 0);
result = 31 * result + (this.retryAttempts != null ? this.retryAttempts.hashCode() : 0);
return result;
}
}
}