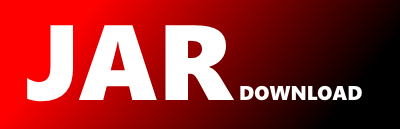
software.amazon.awscdk.services.lookoutmetrics.CfnAnomalyDetectorProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.lookoutmetrics;
/**
* Properties for defining a `CfnAnomalyDetector`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lookoutmetrics.*;
* CfnAnomalyDetectorProps cfnAnomalyDetectorProps = CfnAnomalyDetectorProps.builder()
* .anomalyDetectorConfig(AnomalyDetectorConfigProperty.builder()
* .anomalyDetectorFrequency("anomalyDetectorFrequency")
* .build())
* .metricSetList(List.of(MetricSetProperty.builder()
* .metricList(List.of(MetricProperty.builder()
* .aggregationFunction("aggregationFunction")
* .metricName("metricName")
* // the properties below are optional
* .namespace("namespace")
* .build()))
* .metricSetName("metricSetName")
* .metricSource(MetricSourceProperty.builder()
* .appFlowConfig(AppFlowConfigProperty.builder()
* .flowName("flowName")
* .roleArn("roleArn")
* .build())
* .cloudwatchConfig(CloudwatchConfigProperty.builder()
* .roleArn("roleArn")
* .build())
* .rdsSourceConfig(RDSSourceConfigProperty.builder()
* .databaseHost("databaseHost")
* .databaseName("databaseName")
* .databasePort(123)
* .dbInstanceIdentifier("dbInstanceIdentifier")
* .roleArn("roleArn")
* .secretManagerArn("secretManagerArn")
* .tableName("tableName")
* .vpcConfiguration(VpcConfigurationProperty.builder()
* .securityGroupIdList(List.of("securityGroupIdList"))
* .subnetIdList(List.of("subnetIdList"))
* .build())
* .build())
* .redshiftSourceConfig(RedshiftSourceConfigProperty.builder()
* .clusterIdentifier("clusterIdentifier")
* .databaseHost("databaseHost")
* .databaseName("databaseName")
* .databasePort(123)
* .roleArn("roleArn")
* .secretManagerArn("secretManagerArn")
* .tableName("tableName")
* .vpcConfiguration(VpcConfigurationProperty.builder()
* .securityGroupIdList(List.of("securityGroupIdList"))
* .subnetIdList(List.of("subnetIdList"))
* .build())
* .build())
* .s3SourceConfig(S3SourceConfigProperty.builder()
* .fileFormatDescriptor(FileFormatDescriptorProperty.builder()
* .csvFormatDescriptor(CsvFormatDescriptorProperty.builder()
* .charset("charset")
* .containsHeader(false)
* .delimiter("delimiter")
* .fileCompression("fileCompression")
* .headerList(List.of("headerList"))
* .quoteSymbol("quoteSymbol")
* .build())
* .jsonFormatDescriptor(JsonFormatDescriptorProperty.builder()
* .charset("charset")
* .fileCompression("fileCompression")
* .build())
* .build())
* .roleArn("roleArn")
* // the properties below are optional
* .historicalDataPathList(List.of("historicalDataPathList"))
* .templatedPathList(List.of("templatedPathList"))
* .build())
* .build())
* // the properties below are optional
* .dimensionList(List.of("dimensionList"))
* .metricSetDescription("metricSetDescription")
* .metricSetFrequency("metricSetFrequency")
* .offset(123)
* .timestampColumn(TimestampColumnProperty.builder()
* .columnFormat("columnFormat")
* .columnName("columnName")
* .build())
* .timezone("timezone")
* .build()))
* // the properties below are optional
* .anomalyDetectorDescription("anomalyDetectorDescription")
* .anomalyDetectorName("anomalyDetectorName")
* .kmsKeyArn("kmsKeyArn")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.60.0 (build ebcefe6)", date = "2022-07-06T20:02:47.113Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lookoutmetrics.$Module.class, fqn = "@aws-cdk/aws-lookoutmetrics.CfnAnomalyDetectorProps")
@software.amazon.jsii.Jsii.Proxy(CfnAnomalyDetectorProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnAnomalyDetectorProps extends software.amazon.jsii.JsiiSerializable {
/**
* Contains information about the configuration of the anomaly detector.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getAnomalyDetectorConfig();
/**
* The detector's dataset.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getMetricSetList();
/**
* A description of the detector.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAnomalyDetectorDescription() {
return null;
}
/**
* The name of the detector.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAnomalyDetectorName() {
return null;
}
/**
* The ARN of the KMS key to use to encrypt your data.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getKmsKeyArn() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnAnomalyDetectorProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnAnomalyDetectorProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object anomalyDetectorConfig;
java.lang.Object metricSetList;
java.lang.String anomalyDetectorDescription;
java.lang.String anomalyDetectorName;
java.lang.String kmsKeyArn;
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getAnomalyDetectorConfig}
* @param anomalyDetectorConfig Contains information about the configuration of the anomaly detector. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder anomalyDetectorConfig(software.amazon.awscdk.core.IResolvable anomalyDetectorConfig) {
this.anomalyDetectorConfig = anomalyDetectorConfig;
return this;
}
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getAnomalyDetectorConfig}
* @param anomalyDetectorConfig Contains information about the configuration of the anomaly detector. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder anomalyDetectorConfig(software.amazon.awscdk.services.lookoutmetrics.CfnAnomalyDetector.AnomalyDetectorConfigProperty anomalyDetectorConfig) {
this.anomalyDetectorConfig = anomalyDetectorConfig;
return this;
}
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getMetricSetList}
* @param metricSetList The detector's dataset. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metricSetList(software.amazon.awscdk.core.IResolvable metricSetList) {
this.metricSetList = metricSetList;
return this;
}
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getMetricSetList}
* @param metricSetList The detector's dataset. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metricSetList(java.util.List extends java.lang.Object> metricSetList) {
this.metricSetList = metricSetList;
return this;
}
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getAnomalyDetectorDescription}
* @param anomalyDetectorDescription A description of the detector.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder anomalyDetectorDescription(java.lang.String anomalyDetectorDescription) {
this.anomalyDetectorDescription = anomalyDetectorDescription;
return this;
}
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getAnomalyDetectorName}
* @param anomalyDetectorName The name of the detector.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder anomalyDetectorName(java.lang.String anomalyDetectorName) {
this.anomalyDetectorName = anomalyDetectorName;
return this;
}
/**
* Sets the value of {@link CfnAnomalyDetectorProps#getKmsKeyArn}
* @param kmsKeyArn The ARN of the KMS key to use to encrypt your data.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder kmsKeyArn(java.lang.String kmsKeyArn) {
this.kmsKeyArn = kmsKeyArn;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnAnomalyDetectorProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnAnomalyDetectorProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnAnomalyDetectorProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnAnomalyDetectorProps {
private final java.lang.Object anomalyDetectorConfig;
private final java.lang.Object metricSetList;
private final java.lang.String anomalyDetectorDescription;
private final java.lang.String anomalyDetectorName;
private final java.lang.String kmsKeyArn;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.anomalyDetectorConfig = software.amazon.jsii.Kernel.get(this, "anomalyDetectorConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.metricSetList = software.amazon.jsii.Kernel.get(this, "metricSetList", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.anomalyDetectorDescription = software.amazon.jsii.Kernel.get(this, "anomalyDetectorDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.anomalyDetectorName = software.amazon.jsii.Kernel.get(this, "anomalyDetectorName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.kmsKeyArn = software.amazon.jsii.Kernel.get(this, "kmsKeyArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.anomalyDetectorConfig = java.util.Objects.requireNonNull(builder.anomalyDetectorConfig, "anomalyDetectorConfig is required");
this.metricSetList = java.util.Objects.requireNonNull(builder.metricSetList, "metricSetList is required");
this.anomalyDetectorDescription = builder.anomalyDetectorDescription;
this.anomalyDetectorName = builder.anomalyDetectorName;
this.kmsKeyArn = builder.kmsKeyArn;
}
@Override
public final java.lang.Object getAnomalyDetectorConfig() {
return this.anomalyDetectorConfig;
}
@Override
public final java.lang.Object getMetricSetList() {
return this.metricSetList;
}
@Override
public final java.lang.String getAnomalyDetectorDescription() {
return this.anomalyDetectorDescription;
}
@Override
public final java.lang.String getAnomalyDetectorName() {
return this.anomalyDetectorName;
}
@Override
public final java.lang.String getKmsKeyArn() {
return this.kmsKeyArn;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("anomalyDetectorConfig", om.valueToTree(this.getAnomalyDetectorConfig()));
data.set("metricSetList", om.valueToTree(this.getMetricSetList()));
if (this.getAnomalyDetectorDescription() != null) {
data.set("anomalyDetectorDescription", om.valueToTree(this.getAnomalyDetectorDescription()));
}
if (this.getAnomalyDetectorName() != null) {
data.set("anomalyDetectorName", om.valueToTree(this.getAnomalyDetectorName()));
}
if (this.getKmsKeyArn() != null) {
data.set("kmsKeyArn", om.valueToTree(this.getKmsKeyArn()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lookoutmetrics.CfnAnomalyDetectorProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnAnomalyDetectorProps.Jsii$Proxy that = (CfnAnomalyDetectorProps.Jsii$Proxy) o;
if (!anomalyDetectorConfig.equals(that.anomalyDetectorConfig)) return false;
if (!metricSetList.equals(that.metricSetList)) return false;
if (this.anomalyDetectorDescription != null ? !this.anomalyDetectorDescription.equals(that.anomalyDetectorDescription) : that.anomalyDetectorDescription != null) return false;
if (this.anomalyDetectorName != null ? !this.anomalyDetectorName.equals(that.anomalyDetectorName) : that.anomalyDetectorName != null) return false;
return this.kmsKeyArn != null ? this.kmsKeyArn.equals(that.kmsKeyArn) : that.kmsKeyArn == null;
}
@Override
public final int hashCode() {
int result = this.anomalyDetectorConfig.hashCode();
result = 31 * result + (this.metricSetList.hashCode());
result = 31 * result + (this.anomalyDetectorDescription != null ? this.anomalyDetectorDescription.hashCode() : 0);
result = 31 * result + (this.anomalyDetectorName != null ? this.anomalyDetectorName.hashCode() : 0);
result = 31 * result + (this.kmsKeyArn != null ? this.kmsKeyArn.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy