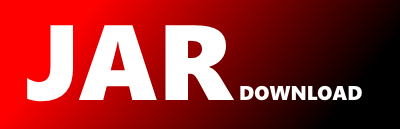
software.amazon.awscdk.services.lookoutmetrics.CfnAlertProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lookoutmetrics Show documentation
Show all versions of lookoutmetrics Show documentation
The CDK Construct Library for AWS::LookoutMetrics
package software.amazon.awscdk.services.lookoutmetrics;
/**
* Properties for defining a `CfnAlert`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lookoutmetrics.*;
* CfnAlertProps cfnAlertProps = CfnAlertProps.builder()
* .action(ActionProperty.builder()
* .lambdaConfiguration(LambdaConfigurationProperty.builder()
* .lambdaArn("lambdaArn")
* .roleArn("roleArn")
* .build())
* .snsConfiguration(SNSConfigurationProperty.builder()
* .roleArn("roleArn")
* .snsTopicArn("snsTopicArn")
* .build())
* .build())
* .alertSensitivityThreshold(123)
* .anomalyDetectorArn("anomalyDetectorArn")
* // the properties below are optional
* .alertDescription("alertDescription")
* .alertName("alertName")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.60.0 (build ebcefe6)", date = "2022-07-09T01:30:38.115Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lookoutmetrics.$Module.class, fqn = "@aws-cdk/aws-lookoutmetrics.CfnAlertProps")
@software.amazon.jsii.Jsii.Proxy(CfnAlertProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnAlertProps extends software.amazon.jsii.JsiiSerializable {
/**
* Action that will be triggered when there is an alert.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getAction();
/**
* An integer from 0 to 100 specifying the alert sensitivity threshold.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getAlertSensitivityThreshold();
/**
* The ARN of the detector to which the alert is attached.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAnomalyDetectorArn();
/**
* A description of the alert.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAlertDescription() {
return null;
}
/**
* The name of the alert.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAlertName() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnAlertProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnAlertProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object action;
java.lang.Number alertSensitivityThreshold;
java.lang.String anomalyDetectorArn;
java.lang.String alertDescription;
java.lang.String alertName;
/**
* Sets the value of {@link CfnAlertProps#getAction}
* @param action Action that will be triggered when there is an alert. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder action(software.amazon.awscdk.services.lookoutmetrics.CfnAlert.ActionProperty action) {
this.action = action;
return this;
}
/**
* Sets the value of {@link CfnAlertProps#getAction}
* @param action Action that will be triggered when there is an alert. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder action(software.amazon.awscdk.core.IResolvable action) {
this.action = action;
return this;
}
/**
* Sets the value of {@link CfnAlertProps#getAlertSensitivityThreshold}
* @param alertSensitivityThreshold An integer from 0 to 100 specifying the alert sensitivity threshold. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder alertSensitivityThreshold(java.lang.Number alertSensitivityThreshold) {
this.alertSensitivityThreshold = alertSensitivityThreshold;
return this;
}
/**
* Sets the value of {@link CfnAlertProps#getAnomalyDetectorArn}
* @param anomalyDetectorArn The ARN of the detector to which the alert is attached. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder anomalyDetectorArn(java.lang.String anomalyDetectorArn) {
this.anomalyDetectorArn = anomalyDetectorArn;
return this;
}
/**
* Sets the value of {@link CfnAlertProps#getAlertDescription}
* @param alertDescription A description of the alert.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder alertDescription(java.lang.String alertDescription) {
this.alertDescription = alertDescription;
return this;
}
/**
* Sets the value of {@link CfnAlertProps#getAlertName}
* @param alertName The name of the alert.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder alertName(java.lang.String alertName) {
this.alertName = alertName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnAlertProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnAlertProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnAlertProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnAlertProps {
private final java.lang.Object action;
private final java.lang.Number alertSensitivityThreshold;
private final java.lang.String anomalyDetectorArn;
private final java.lang.String alertDescription;
private final java.lang.String alertName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.action = software.amazon.jsii.Kernel.get(this, "action", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.alertSensitivityThreshold = software.amazon.jsii.Kernel.get(this, "alertSensitivityThreshold", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.anomalyDetectorArn = software.amazon.jsii.Kernel.get(this, "anomalyDetectorArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.alertDescription = software.amazon.jsii.Kernel.get(this, "alertDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.alertName = software.amazon.jsii.Kernel.get(this, "alertName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.action = java.util.Objects.requireNonNull(builder.action, "action is required");
this.alertSensitivityThreshold = java.util.Objects.requireNonNull(builder.alertSensitivityThreshold, "alertSensitivityThreshold is required");
this.anomalyDetectorArn = java.util.Objects.requireNonNull(builder.anomalyDetectorArn, "anomalyDetectorArn is required");
this.alertDescription = builder.alertDescription;
this.alertName = builder.alertName;
}
@Override
public final java.lang.Object getAction() {
return this.action;
}
@Override
public final java.lang.Number getAlertSensitivityThreshold() {
return this.alertSensitivityThreshold;
}
@Override
public final java.lang.String getAnomalyDetectorArn() {
return this.anomalyDetectorArn;
}
@Override
public final java.lang.String getAlertDescription() {
return this.alertDescription;
}
@Override
public final java.lang.String getAlertName() {
return this.alertName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("action", om.valueToTree(this.getAction()));
data.set("alertSensitivityThreshold", om.valueToTree(this.getAlertSensitivityThreshold()));
data.set("anomalyDetectorArn", om.valueToTree(this.getAnomalyDetectorArn()));
if (this.getAlertDescription() != null) {
data.set("alertDescription", om.valueToTree(this.getAlertDescription()));
}
if (this.getAlertName() != null) {
data.set("alertName", om.valueToTree(this.getAlertName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lookoutmetrics.CfnAlertProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnAlertProps.Jsii$Proxy that = (CfnAlertProps.Jsii$Proxy) o;
if (!action.equals(that.action)) return false;
if (!alertSensitivityThreshold.equals(that.alertSensitivityThreshold)) return false;
if (!anomalyDetectorArn.equals(that.anomalyDetectorArn)) return false;
if (this.alertDescription != null ? !this.alertDescription.equals(that.alertDescription) : that.alertDescription != null) return false;
return this.alertName != null ? this.alertName.equals(that.alertName) : that.alertName == null;
}
@Override
public final int hashCode() {
int result = this.action.hashCode();
result = 31 * result + (this.alertSensitivityThreshold.hashCode());
result = 31 * result + (this.anomalyDetectorArn.hashCode());
result = 31 * result + (this.alertDescription != null ? this.alertDescription.hashCode() : 0);
result = 31 * result + (this.alertName != null ? this.alertName.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy