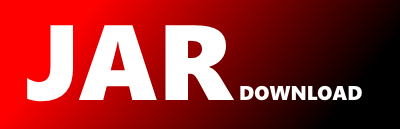
software.amazon.awscdk.services.mediapackage.CfnChannelProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
The CDK Construct Library for AWS::MediaPackage
package software.amazon.awscdk.services.mediapackage;
/**
* Properties for defining a `CfnChannel`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.mediapackage.*;
* CfnChannelProps cfnChannelProps = CfnChannelProps.builder()
* .id("id")
* // the properties below are optional
* .description("description")
* .egressAccessLogs(LogConfigurationProperty.builder()
* .logGroupName("logGroupName")
* .build())
* .ingressAccessLogs(LogConfigurationProperty.builder()
* .logGroupName("logGroupName")
* .build())
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.60.0 (build ebcefe6)", date = "2022-07-06T20:02:47.415Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.mediapackage.$Module.class, fqn = "@aws-cdk/aws-mediapackage.CfnChannelProps")
@software.amazon.jsii.Jsii.Proxy(CfnChannelProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnChannelProps extends software.amazon.jsii.JsiiSerializable {
/**
* Unique identifier that you assign to the channel.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getId();
/**
* Any descriptive information that you want to add to the channel for future identification purposes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* Configures egress access logs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEgressAccessLogs() {
return null;
}
/**
* Configures ingress access logs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getIngressAccessLogs() {
return null;
}
/**
* The tags to assign to the channel.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnChannelProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnChannelProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String id;
java.lang.String description;
java.lang.Object egressAccessLogs;
java.lang.Object ingressAccessLogs;
java.util.List tags;
/**
* Sets the value of {@link CfnChannelProps#getId}
* @param id Unique identifier that you assign to the channel. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder id(java.lang.String id) {
this.id = id;
return this;
}
/**
* Sets the value of {@link CfnChannelProps#getDescription}
* @param description Any descriptive information that you want to add to the channel for future identification purposes.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnChannelProps#getEgressAccessLogs}
* @param egressAccessLogs Configures egress access logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder egressAccessLogs(software.amazon.awscdk.core.IResolvable egressAccessLogs) {
this.egressAccessLogs = egressAccessLogs;
return this;
}
/**
* Sets the value of {@link CfnChannelProps#getEgressAccessLogs}
* @param egressAccessLogs Configures egress access logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder egressAccessLogs(software.amazon.awscdk.services.mediapackage.CfnChannel.LogConfigurationProperty egressAccessLogs) {
this.egressAccessLogs = egressAccessLogs;
return this;
}
/**
* Sets the value of {@link CfnChannelProps#getIngressAccessLogs}
* @param ingressAccessLogs Configures ingress access logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ingressAccessLogs(software.amazon.awscdk.core.IResolvable ingressAccessLogs) {
this.ingressAccessLogs = ingressAccessLogs;
return this;
}
/**
* Sets the value of {@link CfnChannelProps#getIngressAccessLogs}
* @param ingressAccessLogs Configures ingress access logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ingressAccessLogs(software.amazon.awscdk.services.mediapackage.CfnChannel.LogConfigurationProperty ingressAccessLogs) {
this.ingressAccessLogs = ingressAccessLogs;
return this;
}
/**
* Sets the value of {@link CfnChannelProps#getTags}
* @param tags The tags to assign to the channel.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnChannelProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnChannelProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnChannelProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnChannelProps {
private final java.lang.String id;
private final java.lang.String description;
private final java.lang.Object egressAccessLogs;
private final java.lang.Object ingressAccessLogs;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.id = software.amazon.jsii.Kernel.get(this, "id", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.egressAccessLogs = software.amazon.jsii.Kernel.get(this, "egressAccessLogs", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.ingressAccessLogs = software.amazon.jsii.Kernel.get(this, "ingressAccessLogs", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.id = java.util.Objects.requireNonNull(builder.id, "id is required");
this.description = builder.description;
this.egressAccessLogs = builder.egressAccessLogs;
this.ingressAccessLogs = builder.ingressAccessLogs;
this.tags = (java.util.List)builder.tags;
}
@Override
public final java.lang.String getId() {
return this.id;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.Object getEgressAccessLogs() {
return this.egressAccessLogs;
}
@Override
public final java.lang.Object getIngressAccessLogs() {
return this.ingressAccessLogs;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("id", om.valueToTree(this.getId()));
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getEgressAccessLogs() != null) {
data.set("egressAccessLogs", om.valueToTree(this.getEgressAccessLogs()));
}
if (this.getIngressAccessLogs() != null) {
data.set("ingressAccessLogs", om.valueToTree(this.getIngressAccessLogs()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-mediapackage.CfnChannelProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnChannelProps.Jsii$Proxy that = (CfnChannelProps.Jsii$Proxy) o;
if (!id.equals(that.id)) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.egressAccessLogs != null ? !this.egressAccessLogs.equals(that.egressAccessLogs) : that.egressAccessLogs != null) return false;
if (this.ingressAccessLogs != null ? !this.ingressAccessLogs.equals(that.ingressAccessLogs) : that.ingressAccessLogs != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public final int hashCode() {
int result = this.id.hashCode();
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.egressAccessLogs != null ? this.egressAccessLogs.hashCode() : 0);
result = 31 * result + (this.ingressAccessLogs != null ? this.ingressAccessLogs.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy