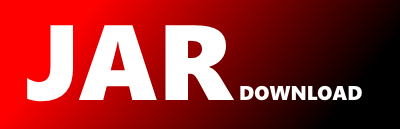
software.amazon.awscdk.services.neptune.alpha.DatabaseCluster Maven / Gradle / Ivy
Show all versions of neptune-alpha Show documentation
package software.amazon.awscdk.services.neptune.alpha;
/**
* (experimental) Create a clustered database with a given number of instances.
*
* Example:
*
*
* DatabaseCluster cluster = DatabaseCluster.Builder.create(this, "ServerlessDatabase")
* .vpc(vpc)
* .instanceType(InstanceType.SERVERLESS)
* .serverlessScalingConfiguration(ServerlessScalingConfiguration.builder()
* .minCapacity(1)
* .maxCapacity(5)
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.89.0 (build 2f74b3e)", date = "2023-10-25T19:57:43.182Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.neptune.alpha.$Module.class, fqn = "@aws-cdk/aws-neptune-alpha.DatabaseCluster")
public class DatabaseCluster extends software.amazon.awscdk.services.neptune.alpha.DatabaseClusterBase implements software.amazon.awscdk.services.neptune.alpha.IDatabaseCluster {
protected DatabaseCluster(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected DatabaseCluster(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
DEFAULT_NUM_INSTANCES = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.neptune.alpha.DatabaseCluster.class, "DEFAULT_NUM_INSTANCES", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public DatabaseCluster(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.neptune.alpha.DatabaseClusterProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) The default number of instances in the Neptune cluster if none are specified.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public final static java.lang.Number DEFAULT_NUM_INSTANCES;
/**
* (experimental) The endpoint to use for read/write operations.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.neptune.alpha.Endpoint getClusterEndpoint() {
return software.amazon.jsii.Kernel.get(this, "clusterEndpoint", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.neptune.alpha.Endpoint.class));
}
/**
* (experimental) Identifier of the cluster.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getClusterIdentifier() {
return software.amazon.jsii.Kernel.get(this, "clusterIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) Endpoint to use for load-balanced read-only operations.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.neptune.alpha.Endpoint getClusterReadEndpoint() {
return software.amazon.jsii.Kernel.get(this, "clusterReadEndpoint", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.neptune.alpha.Endpoint.class));
}
/**
* (experimental) The resource id for the cluster;
*
* for example: cluster-ABCD1234EFGH5678IJKL90MNOP. The cluster ID uniquely
* identifies the cluster and is used in things like IAM authentication policies.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getClusterResourceIdentifier() {
return software.amazon.jsii.Kernel.get(this, "clusterResourceIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The connections object to implement IConnectable.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.Connections getConnections() {
return software.amazon.jsii.Kernel.get(this, "connections", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.Connections.class));
}
/**
* (experimental) Endpoints which address each individual instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.util.List getInstanceEndpoints() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "instanceEndpoints", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.neptune.alpha.Endpoint.class))));
}
/**
* (experimental) Identifiers of the instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.util.List getInstanceIdentifiers() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "instanceIdentifiers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* (experimental) Subnet group used by the DB.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.neptune.alpha.ISubnetGroup getSubnetGroup() {
return software.amazon.jsii.Kernel.get(this, "subnetGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.neptune.alpha.ISubnetGroup.class));
}
/**
* (experimental) The VPC where the DB subnet group is created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.IVpc getVpc() {
return software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IVpc.class));
}
/**
* (experimental) The subnets used by the DB subnet group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected @org.jetbrains.annotations.Nullable java.lang.Boolean getEnableIamAuthentication() {
return software.amazon.jsii.Kernel.get(this, "enableIamAuthentication", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected void setEnableIamAuthentication(final @org.jetbrains.annotations.Nullable java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "enableIamAuthentication", value);
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.neptune.alpha.DatabaseCluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.neptune.alpha.DatabaseClusterProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.neptune.alpha.DatabaseClusterProps.Builder();
}
/**
* (experimental) What type of instance to start for the replicas.
*
* @return {@code this}
* @param instanceType What type of instance to start for the replicas. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder instanceType(final software.amazon.awscdk.services.neptune.alpha.InstanceType instanceType) {
this.props.instanceType(instanceType);
return this;
}
/**
* (experimental) What subnets to run the Neptune instances in.
*
* Must be at least 2 subnets in two different AZs.
*
* @return {@code this}
* @param vpc What subnets to run the Neptune instances in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(final software.amazon.awscdk.services.ec2.IVpc vpc) {
this.props.vpc(vpc);
return this;
}
/**
* (experimental) A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services.
*
* Default: - No role is attached to the cluster.
*
* @return {@code this}
* @param associatedRoles A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder associatedRoles(final java.util.List extends software.amazon.awscdk.services.iam.IRole> associatedRoles) {
this.props.associatedRoles(associatedRoles);
return this;
}
/**
* (experimental) If set to true, Neptune will automatically update the engine of the entire cluster to the latest minor version after a stabilization window of 2 to 3 weeks.
*
* Default: - false
*
* @return {@code this}
* @param autoMinorVersionUpgrade If set to true, Neptune will automatically update the engine of the entire cluster to the latest minor version after a stabilization window of 2 to 3 weeks. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder autoMinorVersionUpgrade(final java.lang.Boolean autoMinorVersionUpgrade) {
this.props.autoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
* (experimental) How many days to retain the backup.
*
* Default: - cdk.Duration.days(1)
*
* @return {@code this}
* @param backupRetention How many days to retain the backup. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder backupRetention(final software.amazon.awscdk.Duration backupRetention) {
this.props.backupRetention(backupRetention);
return this;
}
/**
* (experimental) The list of log types that need to be enabled for exporting to CloudWatch Logs.
*
* Default: - no log exports
*
* @return {@code this}
* @see https://docs.aws.amazon.com/neptune/latest/userguide/auditing.html#auditing-enable
* @param cloudwatchLogsExports The list of log types that need to be enabled for exporting to CloudWatch Logs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder cloudwatchLogsExports(final java.util.List extends software.amazon.awscdk.services.neptune.alpha.LogType> cloudwatchLogsExports) {
this.props.cloudwatchLogsExports(cloudwatchLogsExports);
return this;
}
/**
* (experimental) The number of days log events are kept in CloudWatch Logs.
*
* When updating
* this property, unsetting it doesn't remove the log retention policy. To
* remove the retention policy, set the value to Infinity
.
*
* Default: - logs never expire
*
* @return {@code this}
* @param cloudwatchLogsRetention The number of days log events are kept in CloudWatch Logs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder cloudwatchLogsRetention(final software.amazon.awscdk.services.logs.RetentionDays cloudwatchLogsRetention) {
this.props.cloudwatchLogsRetention(cloudwatchLogsRetention);
return this;
}
/**
* (experimental) The IAM role for the Lambda function associated with the custom resource that sets the retention policy.
*
* Default: - a new role is created.
*
* @return {@code this}
* @param cloudwatchLogsRetentionRole The IAM role for the Lambda function associated with the custom resource that sets the retention policy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder cloudwatchLogsRetentionRole(final software.amazon.awscdk.services.iam.IRole cloudwatchLogsRetentionRole) {
this.props.cloudwatchLogsRetentionRole(cloudwatchLogsRetentionRole);
return this;
}
/**
* (experimental) Additional parameters to pass to the database engine.
*
* Default: - No parameter group.
*
* @return {@code this}
* @param clusterParameterGroup Additional parameters to pass to the database engine. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterParameterGroup(final software.amazon.awscdk.services.neptune.alpha.IClusterParameterGroup clusterParameterGroup) {
this.props.clusterParameterGroup(clusterParameterGroup);
return this;
}
/**
* (experimental) An optional identifier for the cluster.
*
* Default: - A name is automatically generated.
*
* @return {@code this}
* @param dbClusterName An optional identifier for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder dbClusterName(final java.lang.String dbClusterName) {
this.props.dbClusterName(dbClusterName);
return this;
}
/**
* (experimental) Indicates whether the DB cluster should have deletion protection enabled.
*
* Default: - true if ``removalPolicy`` is RETAIN, false otherwise
*
* @return {@code this}
* @param deletionProtection Indicates whether the DB cluster should have deletion protection enabled. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder deletionProtection(final java.lang.Boolean deletionProtection) {
this.props.deletionProtection(deletionProtection);
return this;
}
/**
* (experimental) What version of the database to start.
*
* Default: - The default engine version.
*
* @return {@code this}
* @param engineVersion What version of the database to start. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder engineVersion(final software.amazon.awscdk.services.neptune.alpha.EngineVersion engineVersion) {
this.props.engineVersion(engineVersion);
return this;
}
/**
* (experimental) Map AWS Identity and Access Management (IAM) accounts to database accounts.
*
* Default: - `false`
*
* @return {@code this}
* @param iamAuthentication Map AWS Identity and Access Management (IAM) accounts to database accounts. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder iamAuthentication(final java.lang.Boolean iamAuthentication) {
this.props.iamAuthentication(iamAuthentication);
return this;
}
/**
* (experimental) Base identifier for instances.
*
* Every replica is named by appending the replica number to this string, 1-based.
*
* Default: - `dbClusterName` is used with the word "Instance" appended. If `dbClusterName` is not provided, the
* identifier is automatically generated.
*
* @return {@code this}
* @param instanceIdentifierBase Base identifier for instances. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder instanceIdentifierBase(final java.lang.String instanceIdentifierBase) {
this.props.instanceIdentifierBase(instanceIdentifierBase);
return this;
}
/**
* (experimental) Number of Neptune compute instances.
*
* Default: 1
*
* @return {@code this}
* @param instances Number of Neptune compute instances. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder instances(final java.lang.Number instances) {
this.props.instances(instances);
return this;
}
/**
* (experimental) The KMS key for storage encryption.
*
* Default: - default master key.
*
* @return {@code this}
* @param kmsKey The KMS key for storage encryption. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder kmsKey(final software.amazon.awscdk.services.kms.IKey kmsKey) {
this.props.kmsKey(kmsKey);
return this;
}
/**
* (experimental) The DB parameter group to associate with the instance.
*
* Default: no parameter group
*
* @return {@code this}
* @param parameterGroup The DB parameter group to associate with the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder parameterGroup(final software.amazon.awscdk.services.neptune.alpha.IParameterGroup parameterGroup) {
this.props.parameterGroup(parameterGroup);
return this;
}
/**
* (experimental) A daily time range in 24-hours UTC format in which backups preferably execute.
*
* Must be at least 30 minutes long.
*
* Example: '01:00-02:00'
*
* Default: - a 30-minute window selected at random from an 8-hour block of
* time for each AWS Region. To see the time blocks available, see
*
* @return {@code this}
* @param preferredBackupWindow A daily time range in 24-hours UTC format in which backups preferably execute. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder preferredBackupWindow(final java.lang.String preferredBackupWindow) {
this.props.preferredBackupWindow(preferredBackupWindow);
return this;
}
/**
* (experimental) A weekly time range in which maintenance should preferably execute.
*
* Must be at least 30 minutes long.
*
* Example: 'tue:04:17-tue:04:47'
*
* Default: - 30-minute window selected at random from an 8-hour block of time for
* each AWS Region, occurring on a random day of the week.
*
* @return {@code this}
* @param preferredMaintenanceWindow A weekly time range in which maintenance should preferably execute. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder preferredMaintenanceWindow(final java.lang.String preferredMaintenanceWindow) {
this.props.preferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
* (experimental) The removal policy to apply when the cluster and its instances are removed or replaced during a stack update, or when the stack is deleted.
*
* This
* removal policy also applies to the implicit security group created for the
* cluster if one is not supplied as a parameter.
*
* Default: - Retain cluster.
*
* @return {@code this}
* @param removalPolicy The removal policy to apply when the cluster and its instances are removed or replaced during a stack update, or when the stack is deleted. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder removalPolicy(final software.amazon.awscdk.RemovalPolicy removalPolicy) {
this.props.removalPolicy(removalPolicy);
return this;
}
/**
* (experimental) Security group.
*
* Default: a new security group is created.
*
* @return {@code this}
* @param securityGroups Security group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder securityGroups(final java.util.List extends software.amazon.awscdk.services.ec2.ISecurityGroup> securityGroups) {
this.props.securityGroups(securityGroups);
return this;
}
/**
* (experimental) Specify minimum and maximum NCUs capacity for a serverless cluster.
*
* See https://docs.aws.amazon.com/neptune/latest/userguide/neptune-serverless-capacity-scaling.html
*
* Default: - required if instanceType is db.serverless
*
* @return {@code this}
* @param serverlessScalingConfiguration Specify minimum and maximum NCUs capacity for a serverless cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder serverlessScalingConfiguration(final software.amazon.awscdk.services.neptune.alpha.ServerlessScalingConfiguration serverlessScalingConfiguration) {
this.props.serverlessScalingConfiguration(serverlessScalingConfiguration);
return this;
}
/**
* (experimental) Whether to enable storage encryption.
*
* Default: true
*
* @return {@code this}
* @param storageEncrypted Whether to enable storage encryption. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder storageEncrypted(final java.lang.Boolean storageEncrypted) {
this.props.storageEncrypted(storageEncrypted);
return this;
}
/**
* (experimental) Existing subnet group for the cluster.
*
* Default: - a new subnet group will be created.
*
* @return {@code this}
* @param subnetGroup Existing subnet group for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subnetGroup(final software.amazon.awscdk.services.neptune.alpha.ISubnetGroup subnetGroup) {
this.props.subnetGroup(subnetGroup);
return this;
}
/**
* (experimental) Where to place the instances within the VPC.
*
* Default: private subnets
*
* @return {@code this}
* @param vpcSubnets Where to place the instances within the VPC. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcSubnets(final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.props.vpcSubnets(vpcSubnets);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.neptune.alpha.DatabaseCluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.neptune.alpha.DatabaseCluster build() {
return new software.amazon.awscdk.services.neptune.alpha.DatabaseCluster(
this.scope,
this.id,
this.props.build()
);
}
}
}