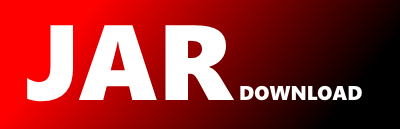
software.amazon.awscdk.services.neptune.alpha.DatabaseClusterAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-alpha Show documentation
Show all versions of neptune-alpha Show documentation
The CDK Construct Library for AWS::Neptune
package software.amazon.awscdk.services.neptune.alpha;
/**
* (experimental) Properties that describe an existing cluster instance.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.neptune.alpha.*;
* import software.amazon.awscdk.services.ec2.*;
* SecurityGroup securityGroup;
* DatabaseClusterAttributes databaseClusterAttributes = DatabaseClusterAttributes.builder()
* .clusterEndpointAddress("clusterEndpointAddress")
* .clusterIdentifier("clusterIdentifier")
* .clusterResourceIdentifier("clusterResourceIdentifier")
* .port(123)
* .readerEndpointAddress("readerEndpointAddress")
* .securityGroup(securityGroup)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.54.0 (build b1b977a)", date = "2022-02-25T01:49:27.814Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.neptune.alpha.$Module.class, fqn = "@aws-cdk/aws-neptune-alpha.DatabaseClusterAttributes")
@software.amazon.jsii.Jsii.Proxy(DatabaseClusterAttributes.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface DatabaseClusterAttributes extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) Cluster endpoint address.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getClusterEndpointAddress();
/**
* (experimental) Identifier for the cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getClusterIdentifier();
/**
* (experimental) Resource Identifier for the cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getClusterResourceIdentifier();
/**
* (experimental) The database port.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.Number getPort();
/**
* (experimental) Reader endpoint address.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getReaderEndpointAddress();
/**
* (experimental) The security group of the database cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup();
/**
* @return a {@link Builder} of {@link DatabaseClusterAttributes}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DatabaseClusterAttributes}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String clusterEndpointAddress;
java.lang.String clusterIdentifier;
java.lang.String clusterResourceIdentifier;
java.lang.Number port;
java.lang.String readerEndpointAddress;
software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
/**
* Sets the value of {@link DatabaseClusterAttributes#getClusterEndpointAddress}
* @param clusterEndpointAddress Cluster endpoint address. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterEndpointAddress(java.lang.String clusterEndpointAddress) {
this.clusterEndpointAddress = clusterEndpointAddress;
return this;
}
/**
* Sets the value of {@link DatabaseClusterAttributes#getClusterIdentifier}
* @param clusterIdentifier Identifier for the cluster. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterIdentifier(java.lang.String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
return this;
}
/**
* Sets the value of {@link DatabaseClusterAttributes#getClusterResourceIdentifier}
* @param clusterResourceIdentifier Resource Identifier for the cluster. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterResourceIdentifier(java.lang.String clusterResourceIdentifier) {
this.clusterResourceIdentifier = clusterResourceIdentifier;
return this;
}
/**
* Sets the value of {@link DatabaseClusterAttributes#getPort}
* @param port The database port. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link DatabaseClusterAttributes#getReaderEndpointAddress}
* @param readerEndpointAddress Reader endpoint address. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder readerEndpointAddress(java.lang.String readerEndpointAddress) {
this.readerEndpointAddress = readerEndpointAddress;
return this;
}
/**
* Sets the value of {@link DatabaseClusterAttributes#getSecurityGroup}
* @param securityGroup The security group of the database cluster. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder securityGroup(software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup) {
this.securityGroup = securityGroup;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DatabaseClusterAttributes}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public DatabaseClusterAttributes build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DatabaseClusterAttributes}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DatabaseClusterAttributes {
private final java.lang.String clusterEndpointAddress;
private final java.lang.String clusterIdentifier;
private final java.lang.String clusterResourceIdentifier;
private final java.lang.Number port;
private final java.lang.String readerEndpointAddress;
private final software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.clusterEndpointAddress = software.amazon.jsii.Kernel.get(this, "clusterEndpointAddress", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clusterIdentifier = software.amazon.jsii.Kernel.get(this, "clusterIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clusterResourceIdentifier = software.amazon.jsii.Kernel.get(this, "clusterResourceIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.port = software.amazon.jsii.Kernel.get(this, "port", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.readerEndpointAddress = software.amazon.jsii.Kernel.get(this, "readerEndpointAddress", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.securityGroup = software.amazon.jsii.Kernel.get(this, "securityGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.ISecurityGroup.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.clusterEndpointAddress = java.util.Objects.requireNonNull(builder.clusterEndpointAddress, "clusterEndpointAddress is required");
this.clusterIdentifier = java.util.Objects.requireNonNull(builder.clusterIdentifier, "clusterIdentifier is required");
this.clusterResourceIdentifier = java.util.Objects.requireNonNull(builder.clusterResourceIdentifier, "clusterResourceIdentifier is required");
this.port = java.util.Objects.requireNonNull(builder.port, "port is required");
this.readerEndpointAddress = java.util.Objects.requireNonNull(builder.readerEndpointAddress, "readerEndpointAddress is required");
this.securityGroup = java.util.Objects.requireNonNull(builder.securityGroup, "securityGroup is required");
}
@Override
public final java.lang.String getClusterEndpointAddress() {
return this.clusterEndpointAddress;
}
@Override
public final java.lang.String getClusterIdentifier() {
return this.clusterIdentifier;
}
@Override
public final java.lang.String getClusterResourceIdentifier() {
return this.clusterResourceIdentifier;
}
@Override
public final java.lang.Number getPort() {
return this.port;
}
@Override
public final java.lang.String getReaderEndpointAddress() {
return this.readerEndpointAddress;
}
@Override
public final software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup() {
return this.securityGroup;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("clusterEndpointAddress", om.valueToTree(this.getClusterEndpointAddress()));
data.set("clusterIdentifier", om.valueToTree(this.getClusterIdentifier()));
data.set("clusterResourceIdentifier", om.valueToTree(this.getClusterResourceIdentifier()));
data.set("port", om.valueToTree(this.getPort()));
data.set("readerEndpointAddress", om.valueToTree(this.getReaderEndpointAddress()));
data.set("securityGroup", om.valueToTree(this.getSecurityGroup()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-neptune-alpha.DatabaseClusterAttributes"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DatabaseClusterAttributes.Jsii$Proxy that = (DatabaseClusterAttributes.Jsii$Proxy) o;
if (!clusterEndpointAddress.equals(that.clusterEndpointAddress)) return false;
if (!clusterIdentifier.equals(that.clusterIdentifier)) return false;
if (!clusterResourceIdentifier.equals(that.clusterResourceIdentifier)) return false;
if (!port.equals(that.port)) return false;
if (!readerEndpointAddress.equals(that.readerEndpointAddress)) return false;
return this.securityGroup.equals(that.securityGroup);
}
@Override
public final int hashCode() {
int result = this.clusterEndpointAddress.hashCode();
result = 31 * result + (this.clusterIdentifier.hashCode());
result = 31 * result + (this.clusterResourceIdentifier.hashCode());
result = 31 * result + (this.port.hashCode());
result = 31 * result + (this.readerEndpointAddress.hashCode());
result = 31 * result + (this.securityGroup.hashCode());
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy