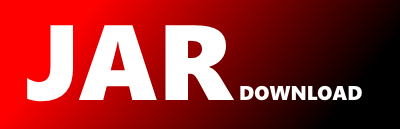
software.amazon.awscdk.services.networkmanager.CfnDeviceProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of networkmanager Show documentation
Show all versions of networkmanager Show documentation
The CDK Construct Library for AWS::NetworkManager
package software.amazon.awscdk.services.networkmanager;
/**
* Properties for defining a `AWS::NetworkManager::Device`.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.29.0 (build 41df200)", date = "2021-05-14T22:39:42.145Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.networkmanager.$Module.class, fqn = "@aws-cdk/aws-networkmanager.CfnDeviceProps")
@software.amazon.jsii.Jsii.Proxy(CfnDeviceProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnDeviceProps extends software.amazon.jsii.JsiiSerializable {
/**
* `AWS::NetworkManager::Device.GlobalNetworkId`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getGlobalNetworkId();
/**
* `AWS::NetworkManager::Device.Description`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* `AWS::NetworkManager::Device.Location`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getLocation() {
return null;
}
/**
* `AWS::NetworkManager::Device.Model`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModel() {
return null;
}
/**
* `AWS::NetworkManager::Device.SerialNumber`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSerialNumber() {
return null;
}
/**
* `AWS::NetworkManager::Device.SiteId`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSiteId() {
return null;
}
/**
* `AWS::NetworkManager::Device.Tags`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* `AWS::NetworkManager::Device.Type`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getType() {
return null;
}
/**
* `AWS::NetworkManager::Device.Vendor`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getVendor() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnDeviceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnDeviceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String globalNetworkId;
private java.lang.String description;
private java.lang.Object location;
private java.lang.String model;
private java.lang.String serialNumber;
private java.lang.String siteId;
private java.util.List tags;
private java.lang.String type;
private java.lang.String vendor;
/**
* Sets the value of {@link CfnDeviceProps#getGlobalNetworkId}
* @param globalNetworkId `AWS::NetworkManager::Device.GlobalNetworkId`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder globalNetworkId(java.lang.String globalNetworkId) {
this.globalNetworkId = globalNetworkId;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getDescription}
* @param description `AWS::NetworkManager::Device.Description`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getLocation}
* @param location `AWS::NetworkManager::Device.Location`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder location(software.amazon.awscdk.core.IResolvable location) {
this.location = location;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getLocation}
* @param location `AWS::NetworkManager::Device.Location`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder location(software.amazon.awscdk.services.networkmanager.CfnDevice.LocationProperty location) {
this.location = location;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getModel}
* @param model `AWS::NetworkManager::Device.Model`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder model(java.lang.String model) {
this.model = model;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getSerialNumber}
* @param serialNumber `AWS::NetworkManager::Device.SerialNumber`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serialNumber(java.lang.String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getSiteId}
* @param siteId `AWS::NetworkManager::Device.SiteId`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder siteId(java.lang.String siteId) {
this.siteId = siteId;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getTags}
* @param tags `AWS::NetworkManager::Device.Tags`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getType}
* @param type `AWS::NetworkManager::Device.Type`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link CfnDeviceProps#getVendor}
* @param vendor `AWS::NetworkManager::Device.Vendor`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vendor(java.lang.String vendor) {
this.vendor = vendor;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnDeviceProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnDeviceProps build() {
return new Jsii$Proxy(globalNetworkId, description, location, model, serialNumber, siteId, tags, type, vendor);
}
}
/**
* An implementation for {@link CfnDeviceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnDeviceProps {
private final java.lang.String globalNetworkId;
private final java.lang.String description;
private final java.lang.Object location;
private final java.lang.String model;
private final java.lang.String serialNumber;
private final java.lang.String siteId;
private final java.util.List tags;
private final java.lang.String type;
private final java.lang.String vendor;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.globalNetworkId = software.amazon.jsii.Kernel.get(this, "globalNetworkId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.location = software.amazon.jsii.Kernel.get(this, "location", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.model = software.amazon.jsii.Kernel.get(this, "model", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.serialNumber = software.amazon.jsii.Kernel.get(this, "serialNumber", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.siteId = software.amazon.jsii.Kernel.get(this, "siteId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vendor = software.amazon.jsii.Kernel.get(this, "vendor", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final java.lang.String globalNetworkId, final java.lang.String description, final java.lang.Object location, final java.lang.String model, final java.lang.String serialNumber, final java.lang.String siteId, final java.util.List extends software.amazon.awscdk.core.CfnTag> tags, final java.lang.String type, final java.lang.String vendor) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.globalNetworkId = java.util.Objects.requireNonNull(globalNetworkId, "globalNetworkId is required");
this.description = description;
this.location = location;
this.model = model;
this.serialNumber = serialNumber;
this.siteId = siteId;
this.tags = (java.util.List)tags;
this.type = type;
this.vendor = vendor;
}
@Override
public final java.lang.String getGlobalNetworkId() {
return this.globalNetworkId;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.Object getLocation() {
return this.location;
}
@Override
public final java.lang.String getModel() {
return this.model;
}
@Override
public final java.lang.String getSerialNumber() {
return this.serialNumber;
}
@Override
public final java.lang.String getSiteId() {
return this.siteId;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
public final java.lang.String getType() {
return this.type;
}
@Override
public final java.lang.String getVendor() {
return this.vendor;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("globalNetworkId", om.valueToTree(this.getGlobalNetworkId()));
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getLocation() != null) {
data.set("location", om.valueToTree(this.getLocation()));
}
if (this.getModel() != null) {
data.set("model", om.valueToTree(this.getModel()));
}
if (this.getSerialNumber() != null) {
data.set("serialNumber", om.valueToTree(this.getSerialNumber()));
}
if (this.getSiteId() != null) {
data.set("siteId", om.valueToTree(this.getSiteId()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getType() != null) {
data.set("type", om.valueToTree(this.getType()));
}
if (this.getVendor() != null) {
data.set("vendor", om.valueToTree(this.getVendor()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-networkmanager.CfnDeviceProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnDeviceProps.Jsii$Proxy that = (CfnDeviceProps.Jsii$Proxy) o;
if (!globalNetworkId.equals(that.globalNetworkId)) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.location != null ? !this.location.equals(that.location) : that.location != null) return false;
if (this.model != null ? !this.model.equals(that.model) : that.model != null) return false;
if (this.serialNumber != null ? !this.serialNumber.equals(that.serialNumber) : that.serialNumber != null) return false;
if (this.siteId != null ? !this.siteId.equals(that.siteId) : that.siteId != null) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
if (this.type != null ? !this.type.equals(that.type) : that.type != null) return false;
return this.vendor != null ? this.vendor.equals(that.vendor) : that.vendor == null;
}
@Override
public final int hashCode() {
int result = this.globalNetworkId.hashCode();
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.location != null ? this.location.hashCode() : 0);
result = 31 * result + (this.model != null ? this.model.hashCode() : 0);
result = 31 * result + (this.serialNumber != null ? this.serialNumber.hashCode() : 0);
result = 31 * result + (this.siteId != null ? this.siteId.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.type != null ? this.type.hashCode() : 0);
result = 31 * result + (this.vendor != null ? this.vendor.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy