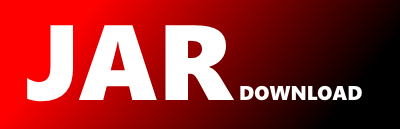
software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshot Maven / Gradle / Ivy
package software.amazon.awscdk.services.rds;
/**
* A database instance restored from a snapshot.
*
* Example:
*
*
* Vpc vpc;
* DatabaseInstance sourceInstance;
* DatabaseInstanceFromSnapshot.Builder.create(this, "Instance")
* .snapshotIdentifier("my-snapshot")
* .engine(DatabaseInstanceEngine.postgres(PostgresInstanceEngineProps.builder().version(PostgresEngineVersion.VER_12_3).build()))
* // optional, defaults to m5.large
* .instanceType(InstanceType.of(InstanceClass.BURSTABLE2, InstanceSize.LARGE))
* .vpc(vpc)
* .build();
* DatabaseInstanceReadReplica.Builder.create(this, "ReadReplica")
* .sourceDatabaseInstance(sourceInstance)
* .instanceType(InstanceType.of(InstanceClass.BURSTABLE2, InstanceSize.LARGE))
* .vpc(vpc)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.70.0 (build 03c2f6f)", date = "2022-11-01T13:16:39.604Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.DatabaseInstanceFromSnapshot")
public class DatabaseInstanceFromSnapshot extends software.amazon.awscdk.services.rds.DatabaseInstanceBase implements software.amazon.awscdk.services.rds.IDatabaseInstance {
protected DatabaseInstanceFromSnapshot(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected DatabaseInstanceFromSnapshot(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public DatabaseInstanceFromSnapshot(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshotProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Adds the multi user rotation to this instance.
*
* @param id This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationMultiUser(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.RotationMultiUserOptions options) {
return software.amazon.jsii.Kernel.call(this, "addRotationMultiUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class), new Object[] { java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* Adds the single user rotation of the master password to this instance.
*
* @param options the options for the rotation, if you want to override the defaults.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationSingleUser(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.RotationSingleUserOptions options) {
return software.amazon.jsii.Kernel.call(this, "addRotationSingleUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class), new Object[] { options });
}
/**
* Adds the single user rotation of the master password to this instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationSingleUser() {
return software.amazon.jsii.Kernel.call(this, "addRotationSingleUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected void setLogRetention() {
software.amazon.jsii.Kernel.call(this, "setLogRetention", software.amazon.jsii.NativeType.VOID);
}
/**
* Access to network connections.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.Connections getConnections() {
return software.amazon.jsii.Kernel.get(this, "connections", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.Connections.class));
}
/**
* The instance endpoint address.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getDbInstanceEndpointAddress() {
return software.amazon.jsii.Kernel.get(this, "dbInstanceEndpointAddress", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The instance endpoint port.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getDbInstanceEndpointPort() {
return software.amazon.jsii.Kernel.get(this, "dbInstanceEndpointPort", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The instance endpoint.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.Endpoint getInstanceEndpoint() {
return software.amazon.jsii.Kernel.get(this, "instanceEndpoint", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.Endpoint.class));
}
/**
* The instance identifier.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getInstanceIdentifier() {
return software.amazon.jsii.Kernel.get(this, "instanceIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.InstanceType getInstanceType() {
return software.amazon.jsii.Kernel.get(this, "instanceType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.InstanceType.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.CfnDBInstanceProps getNewCfnProps() {
return software.amazon.jsii.Kernel.get(this, "newCfnProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.CfnDBInstanceProps.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.CfnDBInstanceProps getSourceCfnProps() {
return software.amazon.jsii.Kernel.get(this, "sourceCfnProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.CfnDBInstanceProps.class));
}
/**
* The VPC where this database instance is deployed.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.IVpc getVpc() {
return software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IVpc.class));
}
/**
* The engine of this database Instance.
*
* May be not known for imported Instances if it wasn't provided explicitly,
* or for read replicas.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.IInstanceEngine getEngine() {
return software.amazon.jsii.Kernel.get(this, "engine", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IInstanceEngine.class));
}
/**
* The AWS Secrets Manager secret attached to the instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.secretsmanager.ISecret getSecret() {
return software.amazon.jsii.Kernel.get(this, "secret", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.ISecret.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.SubnetSelection getVpcPlacement() {
return software.amazon.jsii.Kernel.get(this, "vpcPlacement", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.Nullable java.lang.Boolean getEnableIamAuthentication() {
return software.amazon.jsii.Kernel.get(this, "enableIamAuthentication", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected void setEnableIamAuthentication(final @org.jetbrains.annotations.Nullable java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "enableIamAuthentication", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshot}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshotProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshotProps.Builder();
}
/**
* The VPC network where the DB subnet group should be created.
*
* @return {@code this}
* @param vpc The VPC network where the DB subnet group should be created. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpc(final software.amazon.awscdk.services.ec2.IVpc vpc) {
this.props.vpc(vpc);
return this;
}
/**
* Indicates that minor engine upgrades are applied automatically to the DB instance during the maintenance window.
*
* Default: true
*
* @return {@code this}
* @param autoMinorVersionUpgrade Indicates that minor engine upgrades are applied automatically to the DB instance during the maintenance window. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder autoMinorVersionUpgrade(final java.lang.Boolean autoMinorVersionUpgrade) {
this.props.autoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
* The name of the Availability Zone where the DB instance will be located.
*
* Default: - no preference
*
* @return {@code this}
* @param availabilityZone The name of the Availability Zone where the DB instance will be located. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder availabilityZone(final java.lang.String availabilityZone) {
this.props.availabilityZone(availabilityZone);
return this;
}
/**
* The number of days during which automatic DB snapshots are retained.
*
* Set to zero to disable backups.
* When creating a read replica, you must enable automatic backups on the source
* database instance by setting the backup retention to a value other than zero.
*
* Default: - Duration.days(1) for source instances, disabled for read replicas
*
* @return {@code this}
* @param backupRetention The number of days during which automatic DB snapshots are retained. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder backupRetention(final software.amazon.awscdk.core.Duration backupRetention) {
this.props.backupRetention(backupRetention);
return this;
}
/**
* The list of log types that need to be enabled for exporting to CloudWatch Logs.
*
* Default: - no log exports
*
* @return {@code this}
* @param cloudwatchLogsExports The list of log types that need to be enabled for exporting to CloudWatch Logs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudwatchLogsExports(final java.util.List cloudwatchLogsExports) {
this.props.cloudwatchLogsExports(cloudwatchLogsExports);
return this;
}
/**
* The number of days log events are kept in CloudWatch Logs.
*
* When updating
* this property, unsetting it doesn't remove the log retention policy. To
* remove the retention policy, set the value to Infinity
.
*
* Default: - logs never expire
*
* @return {@code this}
* @param cloudwatchLogsRetention The number of days log events are kept in CloudWatch Logs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudwatchLogsRetention(final software.amazon.awscdk.services.logs.RetentionDays cloudwatchLogsRetention) {
this.props.cloudwatchLogsRetention(cloudwatchLogsRetention);
return this;
}
/**
* The IAM role for the Lambda function associated with the custom resource that sets the retention policy.
*
* Default: - a new role is created.
*
* @return {@code this}
* @param cloudwatchLogsRetentionRole The IAM role for the Lambda function associated with the custom resource that sets the retention policy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudwatchLogsRetentionRole(final software.amazon.awscdk.services.iam.IRole cloudwatchLogsRetentionRole) {
this.props.cloudwatchLogsRetentionRole(cloudwatchLogsRetentionRole);
return this;
}
/**
* Indicates whether to copy all of the user-defined tags from the DB instance to snapshots of the DB instance.
*
* Default: true
*
* @return {@code this}
* @param copyTagsToSnapshot Indicates whether to copy all of the user-defined tags from the DB instance to snapshots of the DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder copyTagsToSnapshot(final java.lang.Boolean copyTagsToSnapshot) {
this.props.copyTagsToSnapshot(copyTagsToSnapshot);
return this;
}
/**
* Indicates whether automated backups should be deleted or retained when you delete a DB instance.
*
* Default: false
*
* @return {@code this}
* @param deleteAutomatedBackups Indicates whether automated backups should be deleted or retained when you delete a DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deleteAutomatedBackups(final java.lang.Boolean deleteAutomatedBackups) {
this.props.deleteAutomatedBackups(deleteAutomatedBackups);
return this;
}
/**
* Indicates whether the DB instance should have deletion protection enabled.
*
* Default: - true if ``removalPolicy`` is RETAIN, false otherwise
*
* @return {@code this}
* @param deletionProtection Indicates whether the DB instance should have deletion protection enabled. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deletionProtection(final java.lang.Boolean deletionProtection) {
this.props.deletionProtection(deletionProtection);
return this;
}
/**
* The Active Directory directory ID to create the DB instance in.
*
* Default: - Do not join domain
*
* @return {@code this}
* @param domain The Active Directory directory ID to create the DB instance in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domain(final java.lang.String domain) {
this.props.domain(domain);
return this;
}
/**
* The IAM role to be used when making API calls to the Directory Service.
*
* The role needs the AWS-managed policy
* AmazonRDSDirectoryServiceAccess or equivalent.
*
* Default: - The role will be created for you if {@link DatabaseInstanceNewProps#domain} is specified
*
* @return {@code this}
* @param domainRole The IAM role to be used when making API calls to the Directory Service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainRole(final software.amazon.awscdk.services.iam.IRole domainRole) {
this.props.domainRole(domainRole);
return this;
}
/**
* Whether to enable Performance Insights for the DB instance.
*
* Default: - false, unless ``performanceInsightRentention`` or ``performanceInsightEncryptionKey`` is set.
*
* @return {@code this}
* @param enablePerformanceInsights Whether to enable Performance Insights for the DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enablePerformanceInsights(final java.lang.Boolean enablePerformanceInsights) {
this.props.enablePerformanceInsights(enablePerformanceInsights);
return this;
}
/**
* Whether to enable mapping of AWS Identity and Access Management (IAM) accounts to database accounts.
*
* Default: false
*
* @return {@code this}
* @param iamAuthentication Whether to enable mapping of AWS Identity and Access Management (IAM) accounts to database accounts. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iamAuthentication(final java.lang.Boolean iamAuthentication) {
this.props.iamAuthentication(iamAuthentication);
return this;
}
/**
* A name for the DB instance.
*
* If you specify a name, AWS CloudFormation
* converts it to lowercase.
*
* Default: - a CloudFormation generated name
*
* @return {@code this}
* @param instanceIdentifier A name for the DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceIdentifier(final java.lang.String instanceIdentifier) {
this.props.instanceIdentifier(instanceIdentifier);
return this;
}
/**
* The number of I/O operations per second (IOPS) that the database provisions.
*
* The value must be equal to or greater than 1000.
*
* Default: - no provisioned iops
*
* @return {@code this}
* @param iops The number of I/O operations per second (IOPS) that the database provisions. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iops(final java.lang.Number iops) {
this.props.iops(iops);
return this;
}
/**
* Upper limit to which RDS can scale the storage in GiB(Gibibyte).
*
* Default: - No autoscaling of RDS instance
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_PIOPS.StorageTypes.html#USER_PIOPS.Autoscaling
* @param maxAllocatedStorage Upper limit to which RDS can scale the storage in GiB(Gibibyte). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxAllocatedStorage(final java.lang.Number maxAllocatedStorage) {
this.props.maxAllocatedStorage(maxAllocatedStorage);
return this;
}
/**
* The interval, in seconds, between points when Amazon RDS collects enhanced monitoring metrics for the DB instance.
*
* Default: - no enhanced monitoring
*
* @return {@code this}
* @param monitoringInterval The interval, in seconds, between points when Amazon RDS collects enhanced monitoring metrics for the DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder monitoringInterval(final software.amazon.awscdk.core.Duration monitoringInterval) {
this.props.monitoringInterval(monitoringInterval);
return this;
}
/**
* Role that will be used to manage DB instance monitoring.
*
* Default: - A role is automatically created for you
*
* @return {@code this}
* @param monitoringRole Role that will be used to manage DB instance monitoring. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder monitoringRole(final software.amazon.awscdk.services.iam.IRole monitoringRole) {
this.props.monitoringRole(monitoringRole);
return this;
}
/**
* Specifies if the database instance is a multiple Availability Zone deployment.
*
* Default: false
*
* @return {@code this}
* @param multiAz Specifies if the database instance is a multiple Availability Zone deployment. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder multiAz(final java.lang.Boolean multiAz) {
this.props.multiAz(multiAz);
return this;
}
/**
* The option group to associate with the instance.
*
* Default: - no option group
*
* @return {@code this}
* @param optionGroup The option group to associate with the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder optionGroup(final software.amazon.awscdk.services.rds.IOptionGroup optionGroup) {
this.props.optionGroup(optionGroup);
return this;
}
/**
* The DB parameter group to associate with the instance.
*
* Default: - no parameter group
*
* @return {@code this}
* @param parameterGroup The DB parameter group to associate with the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterGroup(final software.amazon.awscdk.services.rds.IParameterGroup parameterGroup) {
this.props.parameterGroup(parameterGroup);
return this;
}
/**
* The AWS KMS key for encryption of Performance Insights data.
*
* Default: - default master key
*
* @return {@code this}
* @param performanceInsightEncryptionKey The AWS KMS key for encryption of Performance Insights data. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder performanceInsightEncryptionKey(final software.amazon.awscdk.services.kms.IKey performanceInsightEncryptionKey) {
this.props.performanceInsightEncryptionKey(performanceInsightEncryptionKey);
return this;
}
/**
* The amount of time, in days, to retain Performance Insights data.
*
* Default: 7
*
* @return {@code this}
* @param performanceInsightRetention The amount of time, in days, to retain Performance Insights data. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder performanceInsightRetention(final software.amazon.awscdk.services.rds.PerformanceInsightRetention performanceInsightRetention) {
this.props.performanceInsightRetention(performanceInsightRetention);
return this;
}
/**
* The port for the instance.
*
* Default: - the default port for the chosen engine.
*
* @return {@code this}
* @param port The port for the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(final java.lang.Number port) {
this.props.port(port);
return this;
}
/**
* The daily time range during which automated backups are performed.
*
* Constraints:
*
*
* - Must be in the format
hh24:mi-hh24:mi
.
* - Must be in Universal Coordinated Time (UTC).
* - Must not conflict with the preferred maintenance window.
* - Must be at least 30 minutes.
*
*
* Default: - a 30-minute window selected at random from an 8-hour block of
* time for each AWS Region. To see the time blocks available, see
* https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_WorkingWithAutomatedBackups.html#USER_WorkingWithAutomatedBackups.BackupWindow
*
* @return {@code this}
* @param preferredBackupWindow The daily time range during which automated backups are performed. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder preferredBackupWindow(final java.lang.String preferredBackupWindow) {
this.props.preferredBackupWindow(preferredBackupWindow);
return this;
}
/**
* The weekly time range (in UTC) during which system maintenance can occur.
*
* Format: ddd:hh24:mi-ddd:hh24:mi
* Constraint: Minimum 30-minute window
*
* Default: - a 30-minute window selected at random from an 8-hour block of
* time for each AWS Region, occurring on a random day of the week. To see
* the time blocks available, see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_UpgradeDBInstance.Maintenance.html#Concepts.DBMaintenance
*
* @return {@code this}
* @param preferredMaintenanceWindow The weekly time range (in UTC) during which system maintenance can occur. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder preferredMaintenanceWindow(final java.lang.String preferredMaintenanceWindow) {
this.props.preferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
* The number of CPU cores and the number of threads per core.
*
* Default: - the default number of CPU cores and threads per core for the
* chosen instance class.
* See https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Concepts.DBInstanceClass.html#USER_ConfigureProcessor
*
* @return {@code this}
* @param processorFeatures The number of CPU cores and the number of threads per core. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder processorFeatures(final software.amazon.awscdk.services.rds.ProcessorFeatures processorFeatures) {
this.props.processorFeatures(processorFeatures);
return this;
}
/**
* Indicates whether the DB instance is an internet-facing instance.
*
* Default: - `true` if `vpcSubnets` is `subnetType: SubnetType.PUBLIC`, `false` otherwise
*
* @return {@code this}
* @param publiclyAccessible Indicates whether the DB instance is an internet-facing instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder publiclyAccessible(final java.lang.Boolean publiclyAccessible) {
this.props.publiclyAccessible(publiclyAccessible);
return this;
}
/**
* The CloudFormation policy to apply when the instance is removed from the stack or replaced during an update.
*
* Default: - RemovalPolicy.SNAPSHOT (remove the resource, but retain a snapshot of the data)
*
* @return {@code this}
* @param removalPolicy The CloudFormation policy to apply when the instance is removed from the stack or replaced during an update. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder removalPolicy(final software.amazon.awscdk.core.RemovalPolicy removalPolicy) {
this.props.removalPolicy(removalPolicy);
return this;
}
/**
* S3 buckets that you want to load data into.
*
* This property must not be used if s3ExportRole
is used.
*
* For Microsoft SQL Server:
*
* Default: - None
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/oracle-s3-integration.html
* @param s3ExportBuckets S3 buckets that you want to load data into. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3ExportBuckets(final java.util.List extends software.amazon.awscdk.services.s3.IBucket> s3ExportBuckets) {
this.props.s3ExportBuckets(s3ExportBuckets);
return this;
}
/**
* Role that will be associated with this DB instance to enable S3 export.
*
* This property must not be used if s3ExportBuckets
is used.
*
* For Microsoft SQL Server:
*
* Default: - New role is created if `s3ExportBuckets` is set, no role is defined otherwise
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/oracle-s3-integration.html
* @param s3ExportRole Role that will be associated with this DB instance to enable S3 export. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3ExportRole(final software.amazon.awscdk.services.iam.IRole s3ExportRole) {
this.props.s3ExportRole(s3ExportRole);
return this;
}
/**
* S3 buckets that you want to load data from.
*
* This feature is only supported by the Microsoft SQL Server, Oracle, and PostgreSQL engines.
*
* This property must not be used if s3ImportRole
is used.
*
* For Microsoft SQL Server:
*
* Default: - None
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/PostgreSQL.Procedural.Importing.html
* @param s3ImportBuckets S3 buckets that you want to load data from. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3ImportBuckets(final java.util.List extends software.amazon.awscdk.services.s3.IBucket> s3ImportBuckets) {
this.props.s3ImportBuckets(s3ImportBuckets);
return this;
}
/**
* Role that will be associated with this DB instance to enable S3 import.
*
* This feature is only supported by the Microsoft SQL Server, Oracle, and PostgreSQL engines.
*
* This property must not be used if s3ImportBuckets
is used.
*
* For Microsoft SQL Server:
*
* Default: - New role is created if `s3ImportBuckets` is set, no role is defined otherwise
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/PostgreSQL.Procedural.Importing.html
* @param s3ImportRole Role that will be associated with this DB instance to enable S3 import. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3ImportRole(final software.amazon.awscdk.services.iam.IRole s3ImportRole) {
this.props.s3ImportRole(s3ImportRole);
return this;
}
/**
* The security groups to assign to the DB instance.
*
* Default: - a new security group is created
*
* @return {@code this}
* @param securityGroups The security groups to assign to the DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityGroups(final java.util.List extends software.amazon.awscdk.services.ec2.ISecurityGroup> securityGroups) {
this.props.securityGroups(securityGroups);
return this;
}
/**
* The storage type.
*
* Storage types supported are gp2, io1, standard.
*
* Default: GP2
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Storage.html#Concepts.Storage.GeneralSSD
* @param storageType The storage type. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder storageType(final software.amazon.awscdk.services.rds.StorageType storageType) {
this.props.storageType(storageType);
return this;
}
/**
* Existing subnet group for the instance.
*
* Default: - a new subnet group will be created.
*
* @return {@code this}
* @param subnetGroup Existing subnet group for the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subnetGroup(final software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup) {
this.props.subnetGroup(subnetGroup);
return this;
}
/**
* (deprecated) The type of subnets to add to the created DB subnet group.
*
* Default: - private subnets
*
* @return {@code this}
* @deprecated use `vpcSubnets`
* @param vpcPlacement The type of subnets to add to the created DB subnet group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder vpcPlacement(final software.amazon.awscdk.services.ec2.SubnetSelection vpcPlacement) {
this.props.vpcPlacement(vpcPlacement);
return this;
}
/**
* The type of subnets to add to the created DB subnet group.
*
* Default: - private subnets
*
* @return {@code this}
* @param vpcSubnets The type of subnets to add to the created DB subnet group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.props.vpcSubnets(vpcSubnets);
return this;
}
/**
* The database engine.
*
* @return {@code this}
* @param engine The database engine. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder engine(final software.amazon.awscdk.services.rds.IInstanceEngine engine) {
this.props.engine(engine);
return this;
}
/**
* The allocated storage size, specified in gigabytes (GB).
*
* Default: 100
*
* @return {@code this}
* @param allocatedStorage The allocated storage size, specified in gigabytes (GB). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder allocatedStorage(final java.lang.Number allocatedStorage) {
this.props.allocatedStorage(allocatedStorage);
return this;
}
/**
* Whether to allow major version upgrades.
*
* Default: false
*
* @return {@code this}
* @param allowMajorVersionUpgrade Whether to allow major version upgrades. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder allowMajorVersionUpgrade(final java.lang.Boolean allowMajorVersionUpgrade) {
this.props.allowMajorVersionUpgrade(allowMajorVersionUpgrade);
return this;
}
/**
* The name of the database.
*
* Default: - no name
*
* @return {@code this}
* @param databaseName The name of the database. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder databaseName(final java.lang.String databaseName) {
this.props.databaseName(databaseName);
return this;
}
/**
* The name of the compute and memory capacity for the instance.
*
* Default: - m5.large (or, more specifically, db.m5.large)
*
* @return {@code this}
* @param instanceType The name of the compute and memory capacity for the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceType(final software.amazon.awscdk.services.ec2.InstanceType instanceType) {
this.props.instanceType(instanceType);
return this;
}
/**
* The license model.
*
* Default: - RDS default license model
*
* @return {@code this}
* @param licenseModel The license model. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder licenseModel(final software.amazon.awscdk.services.rds.LicenseModel licenseModel) {
this.props.licenseModel(licenseModel);
return this;
}
/**
* The parameters in the DBParameterGroup to create automatically.
*
* You can only specify parameterGroup or parameters but not both.
* You need to use a versioned engine to auto-generate a DBParameterGroup.
*
* Default: - None
*
* @return {@code this}
* @param parameters The parameters in the DBParameterGroup to create automatically. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(final java.util.Map parameters) {
this.props.parameters(parameters);
return this;
}
/**
* The time zone of the instance.
*
* This is currently supported only by Microsoft Sql Server.
*
* Default: - RDS default timezone
*
* @return {@code this}
* @param timezone The time zone of the instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timezone(final java.lang.String timezone) {
this.props.timezone(timezone);
return this;
}
/**
* The name or Amazon Resource Name (ARN) of the DB snapshot that's used to restore the DB instance.
*
* If you're restoring from a shared manual DB
* snapshot, you must specify the ARN of the snapshot.
*
* @return {@code this}
* @param snapshotIdentifier The name or Amazon Resource Name (ARN) of the DB snapshot that's used to restore the DB instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder snapshotIdentifier(final java.lang.String snapshotIdentifier) {
this.props.snapshotIdentifier(snapshotIdentifier);
return this;
}
/**
* Master user credentials.
*
* Note - It is not possible to change the master username for a snapshot;
* however, it is possible to provide (or generate) a new password.
*
* Default: - The existing username and password from the snapshot will be used.
*
* @return {@code this}
* @param credentials Master user credentials. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder credentials(final software.amazon.awscdk.services.rds.SnapshotCredentials credentials) {
this.props.credentials(credentials);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshot}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshot build() {
return new software.amazon.awscdk.services.rds.DatabaseInstanceFromSnapshot(
this.scope,
this.id,
this.props.build()
);
}
}
}