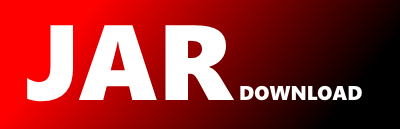
software.amazon.awscdk.services.rds.DatabaseInstanceSourceProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.rds;
/**
* Construction properties for a DatabaseInstanceSource.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.ec2.*;
* import software.amazon.awscdk.services.iam.*;
* import software.amazon.awscdk.services.kms.*;
* import software.amazon.awscdk.services.logs.*;
* import software.amazon.awscdk.services.rds.*;
* import software.amazon.awscdk.services.s3.*;
* import software.amazon.awscdk.core.*;
* Bucket bucket;
* IInstanceEngine instanceEngine;
* InstanceType instanceType;
* Key key;
* OptionGroup optionGroup;
* ParameterGroup parameterGroup;
* Role role;
* SecurityGroup securityGroup;
* Subnet subnet;
* SubnetFilter subnetFilter;
* SubnetGroup subnetGroup;
* Vpc vpc;
* DatabaseInstanceSourceProps databaseInstanceSourceProps = DatabaseInstanceSourceProps.builder()
* .engine(instanceEngine)
* .vpc(vpc)
* // the properties below are optional
* .allocatedStorage(123)
* .allowMajorVersionUpgrade(false)
* .autoMinorVersionUpgrade(false)
* .availabilityZone("availabilityZone")
* .backupRetention(Duration.minutes(30))
* .cloudwatchLogsExports(List.of("cloudwatchLogsExports"))
* .cloudwatchLogsRetention(RetentionDays.ONE_DAY)
* .cloudwatchLogsRetentionRole(role)
* .copyTagsToSnapshot(false)
* .databaseName("databaseName")
* .deleteAutomatedBackups(false)
* .deletionProtection(false)
* .domain("domain")
* .domainRole(role)
* .enablePerformanceInsights(false)
* .iamAuthentication(false)
* .instanceIdentifier("instanceIdentifier")
* .instanceType(instanceType)
* .iops(123)
* .licenseModel(LicenseModel.LICENSE_INCLUDED)
* .maxAllocatedStorage(123)
* .monitoringInterval(Duration.minutes(30))
* .monitoringRole(role)
* .multiAz(false)
* .optionGroup(optionGroup)
* .parameterGroup(parameterGroup)
* .parameters(Map.of(
* "parametersKey", "parameters"))
* .performanceInsightEncryptionKey(key)
* .performanceInsightRetention(PerformanceInsightRetention.DEFAULT)
* .port(123)
* .preferredBackupWindow("preferredBackupWindow")
* .preferredMaintenanceWindow("preferredMaintenanceWindow")
* .processorFeatures(ProcessorFeatures.builder()
* .coreCount(123)
* .threadsPerCore(123)
* .build())
* .publiclyAccessible(false)
* .removalPolicy(RemovalPolicy.DESTROY)
* .s3ExportBuckets(List.of(bucket))
* .s3ExportRole(role)
* .s3ImportBuckets(List.of(bucket))
* .s3ImportRole(role)
* .securityGroups(List.of(securityGroup))
* .storageType(StorageType.STANDARD)
* .subnetGroup(subnetGroup)
* .timezone("timezone")
* .vpcPlacement(SubnetSelection.builder()
* .availabilityZones(List.of("availabilityZones"))
* .onePerAz(false)
* .subnetFilters(List.of(subnetFilter))
* .subnetGroupName("subnetGroupName")
* .subnetName("subnetName")
* .subnets(List.of(subnet))
* .subnetType(SubnetType.ISOLATED)
* .build())
* .vpcSubnets(SubnetSelection.builder()
* .availabilityZones(List.of("availabilityZones"))
* .onePerAz(false)
* .subnetFilters(List.of(subnetFilter))
* .subnetGroupName("subnetGroupName")
* .subnetName("subnetName")
* .subnets(List.of(subnet))
* .subnetType(SubnetType.ISOLATED)
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.70.0 (build 03c2f6f)", date = "2022-11-01T13:16:39.621Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.DatabaseInstanceSourceProps")
@software.amazon.jsii.Jsii.Proxy(DatabaseInstanceSourceProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface DatabaseInstanceSourceProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.rds.DatabaseInstanceNewProps {
/**
* The database engine.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.IInstanceEngine getEngine();
/**
* The allocated storage size, specified in gigabytes (GB).
*
* Default: 100
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getAllocatedStorage() {
return null;
}
/**
* Whether to allow major version upgrades.
*
* Default: false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getAllowMajorVersionUpgrade() {
return null;
}
/**
* The name of the database.
*
* Default: - no name
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDatabaseName() {
return null;
}
/**
* The name of the compute and memory capacity for the instance.
*
* Default: - m5.large (or, more specifically, db.m5.large)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.InstanceType getInstanceType() {
return null;
}
/**
* The license model.
*
* Default: - RDS default license model
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.LicenseModel getLicenseModel() {
return null;
}
/**
* The parameters in the DBParameterGroup to create automatically.
*
* You can only specify parameterGroup or parameters but not both.
* You need to use a versioned engine to auto-generate a DBParameterGroup.
*
* Default: - None
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getParameters() {
return null;
}
/**
* The time zone of the instance.
*
* This is currently supported only by Microsoft Sql Server.
*
* Default: - RDS default timezone
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTimezone() {
return null;
}
/**
* @return a {@link Builder} of {@link DatabaseInstanceSourceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DatabaseInstanceSourceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.rds.IInstanceEngine engine;
java.lang.Number allocatedStorage;
java.lang.Boolean allowMajorVersionUpgrade;
java.lang.String databaseName;
software.amazon.awscdk.services.ec2.InstanceType instanceType;
software.amazon.awscdk.services.rds.LicenseModel licenseModel;
java.util.Map parameters;
java.lang.String timezone;
software.amazon.awscdk.services.ec2.IVpc vpc;
java.lang.Boolean autoMinorVersionUpgrade;
java.lang.String availabilityZone;
software.amazon.awscdk.core.Duration backupRetention;
java.util.List cloudwatchLogsExports;
software.amazon.awscdk.services.logs.RetentionDays cloudwatchLogsRetention;
software.amazon.awscdk.services.iam.IRole cloudwatchLogsRetentionRole;
java.lang.Boolean copyTagsToSnapshot;
java.lang.Boolean deleteAutomatedBackups;
java.lang.Boolean deletionProtection;
java.lang.String domain;
software.amazon.awscdk.services.iam.IRole domainRole;
java.lang.Boolean enablePerformanceInsights;
java.lang.Boolean iamAuthentication;
java.lang.String instanceIdentifier;
java.lang.Number iops;
java.lang.Number maxAllocatedStorage;
software.amazon.awscdk.core.Duration monitoringInterval;
software.amazon.awscdk.services.iam.IRole monitoringRole;
java.lang.Boolean multiAz;
software.amazon.awscdk.services.rds.IOptionGroup optionGroup;
software.amazon.awscdk.services.rds.IParameterGroup parameterGroup;
software.amazon.awscdk.services.kms.IKey performanceInsightEncryptionKey;
software.amazon.awscdk.services.rds.PerformanceInsightRetention performanceInsightRetention;
java.lang.Number port;
java.lang.String preferredBackupWindow;
java.lang.String preferredMaintenanceWindow;
software.amazon.awscdk.services.rds.ProcessorFeatures processorFeatures;
java.lang.Boolean publiclyAccessible;
software.amazon.awscdk.core.RemovalPolicy removalPolicy;
java.util.List s3ExportBuckets;
software.amazon.awscdk.services.iam.IRole s3ExportRole;
java.util.List s3ImportBuckets;
software.amazon.awscdk.services.iam.IRole s3ImportRole;
java.util.List securityGroups;
software.amazon.awscdk.services.rds.StorageType storageType;
software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup;
software.amazon.awscdk.services.ec2.SubnetSelection vpcPlacement;
software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getEngine}
* @param engine The database engine. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder engine(software.amazon.awscdk.services.rds.IInstanceEngine engine) {
this.engine = engine;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getAllocatedStorage}
* @param allocatedStorage The allocated storage size, specified in gigabytes (GB).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder allocatedStorage(java.lang.Number allocatedStorage) {
this.allocatedStorage = allocatedStorage;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getAllowMajorVersionUpgrade}
* @param allowMajorVersionUpgrade Whether to allow major version upgrades.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder allowMajorVersionUpgrade(java.lang.Boolean allowMajorVersionUpgrade) {
this.allowMajorVersionUpgrade = allowMajorVersionUpgrade;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getDatabaseName}
* @param databaseName The name of the database.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder databaseName(java.lang.String databaseName) {
this.databaseName = databaseName;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getInstanceType}
* @param instanceType The name of the compute and memory capacity for the instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceType(software.amazon.awscdk.services.ec2.InstanceType instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getLicenseModel}
* @param licenseModel The license model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder licenseModel(software.amazon.awscdk.services.rds.LicenseModel licenseModel) {
this.licenseModel = licenseModel;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getParameters}
* @param parameters The parameters in the DBParameterGroup to create automatically.
* You can only specify parameterGroup or parameters but not both.
* You need to use a versioned engine to auto-generate a DBParameterGroup.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(java.util.Map parameters) {
this.parameters = parameters;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getTimezone}
* @param timezone The time zone of the instance.
* This is currently supported only by Microsoft Sql Server.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timezone(java.lang.String timezone) {
this.timezone = timezone;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getVpc}
* @param vpc The VPC network where the DB subnet group should be created. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpc(software.amazon.awscdk.services.ec2.IVpc vpc) {
this.vpc = vpc;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getAutoMinorVersionUpgrade}
* @param autoMinorVersionUpgrade Indicates that minor engine upgrades are applied automatically to the DB instance during the maintenance window.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder autoMinorVersionUpgrade(java.lang.Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getAvailabilityZone}
* @param availabilityZone The name of the Availability Zone where the DB instance will be located.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder availabilityZone(java.lang.String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getBackupRetention}
* @param backupRetention The number of days during which automatic DB snapshots are retained.
* Set to zero to disable backups.
* When creating a read replica, you must enable automatic backups on the source
* database instance by setting the backup retention to a value other than zero.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder backupRetention(software.amazon.awscdk.core.Duration backupRetention) {
this.backupRetention = backupRetention;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getCloudwatchLogsExports}
* @param cloudwatchLogsExports The list of log types that need to be enabled for exporting to CloudWatch Logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudwatchLogsExports(java.util.List cloudwatchLogsExports) {
this.cloudwatchLogsExports = cloudwatchLogsExports;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getCloudwatchLogsRetention}
* @param cloudwatchLogsRetention The number of days log events are kept in CloudWatch Logs.
* When updating
* this property, unsetting it doesn't remove the log retention policy. To
* remove the retention policy, set the value to Infinity
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudwatchLogsRetention(software.amazon.awscdk.services.logs.RetentionDays cloudwatchLogsRetention) {
this.cloudwatchLogsRetention = cloudwatchLogsRetention;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getCloudwatchLogsRetentionRole}
* @param cloudwatchLogsRetentionRole The IAM role for the Lambda function associated with the custom resource that sets the retention policy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudwatchLogsRetentionRole(software.amazon.awscdk.services.iam.IRole cloudwatchLogsRetentionRole) {
this.cloudwatchLogsRetentionRole = cloudwatchLogsRetentionRole;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getCopyTagsToSnapshot}
* @param copyTagsToSnapshot Indicates whether to copy all of the user-defined tags from the DB instance to snapshots of the DB instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder copyTagsToSnapshot(java.lang.Boolean copyTagsToSnapshot) {
this.copyTagsToSnapshot = copyTagsToSnapshot;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getDeleteAutomatedBackups}
* @param deleteAutomatedBackups Indicates whether automated backups should be deleted or retained when you delete a DB instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deleteAutomatedBackups(java.lang.Boolean deleteAutomatedBackups) {
this.deleteAutomatedBackups = deleteAutomatedBackups;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getDeletionProtection}
* @param deletionProtection Indicates whether the DB instance should have deletion protection enabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deletionProtection(java.lang.Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getDomain}
* @param domain The Active Directory directory ID to create the DB instance in.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domain(java.lang.String domain) {
this.domain = domain;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getDomainRole}
* @param domainRole The IAM role to be used when making API calls to the Directory Service.
* The role needs the AWS-managed policy
* AmazonRDSDirectoryServiceAccess or equivalent.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainRole(software.amazon.awscdk.services.iam.IRole domainRole) {
this.domainRole = domainRole;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getEnablePerformanceInsights}
* @param enablePerformanceInsights Whether to enable Performance Insights for the DB instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enablePerformanceInsights(java.lang.Boolean enablePerformanceInsights) {
this.enablePerformanceInsights = enablePerformanceInsights;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getIamAuthentication}
* @param iamAuthentication Whether to enable mapping of AWS Identity and Access Management (IAM) accounts to database accounts.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iamAuthentication(java.lang.Boolean iamAuthentication) {
this.iamAuthentication = iamAuthentication;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getInstanceIdentifier}
* @param instanceIdentifier A name for the DB instance.
* If you specify a name, AWS CloudFormation
* converts it to lowercase.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceIdentifier(java.lang.String instanceIdentifier) {
this.instanceIdentifier = instanceIdentifier;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getIops}
* @param iops The number of I/O operations per second (IOPS) that the database provisions.
* The value must be equal to or greater than 1000.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iops(java.lang.Number iops) {
this.iops = iops;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getMaxAllocatedStorage}
* @param maxAllocatedStorage Upper limit to which RDS can scale the storage in GiB(Gibibyte).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxAllocatedStorage(java.lang.Number maxAllocatedStorage) {
this.maxAllocatedStorage = maxAllocatedStorage;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getMonitoringInterval}
* @param monitoringInterval The interval, in seconds, between points when Amazon RDS collects enhanced monitoring metrics for the DB instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder monitoringInterval(software.amazon.awscdk.core.Duration monitoringInterval) {
this.monitoringInterval = monitoringInterval;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getMonitoringRole}
* @param monitoringRole Role that will be used to manage DB instance monitoring.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder monitoringRole(software.amazon.awscdk.services.iam.IRole monitoringRole) {
this.monitoringRole = monitoringRole;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getMultiAz}
* @param multiAz Specifies if the database instance is a multiple Availability Zone deployment.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder multiAz(java.lang.Boolean multiAz) {
this.multiAz = multiAz;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getOptionGroup}
* @param optionGroup The option group to associate with the instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder optionGroup(software.amazon.awscdk.services.rds.IOptionGroup optionGroup) {
this.optionGroup = optionGroup;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getParameterGroup}
* @param parameterGroup The DB parameter group to associate with the instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterGroup(software.amazon.awscdk.services.rds.IParameterGroup parameterGroup) {
this.parameterGroup = parameterGroup;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getPerformanceInsightEncryptionKey}
* @param performanceInsightEncryptionKey The AWS KMS key for encryption of Performance Insights data.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder performanceInsightEncryptionKey(software.amazon.awscdk.services.kms.IKey performanceInsightEncryptionKey) {
this.performanceInsightEncryptionKey = performanceInsightEncryptionKey;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getPerformanceInsightRetention}
* @param performanceInsightRetention The amount of time, in days, to retain Performance Insights data.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder performanceInsightRetention(software.amazon.awscdk.services.rds.PerformanceInsightRetention performanceInsightRetention) {
this.performanceInsightRetention = performanceInsightRetention;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getPort}
* @param port The port for the instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getPreferredBackupWindow}
* @param preferredBackupWindow The daily time range during which automated backups are performed.
* Constraints:
*
*
* - Must be in the format
hh24:mi-hh24:mi
.
* - Must be in Universal Coordinated Time (UTC).
* - Must not conflict with the preferred maintenance window.
* - Must be at least 30 minutes.
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder preferredBackupWindow(java.lang.String preferredBackupWindow) {
this.preferredBackupWindow = preferredBackupWindow;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getPreferredMaintenanceWindow}
* @param preferredMaintenanceWindow The weekly time range (in UTC) during which system maintenance can occur.
* Format: ddd:hh24:mi-ddd:hh24:mi
* Constraint: Minimum 30-minute window
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder preferredMaintenanceWindow(java.lang.String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getProcessorFeatures}
* @param processorFeatures The number of CPU cores and the number of threads per core.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder processorFeatures(software.amazon.awscdk.services.rds.ProcessorFeatures processorFeatures) {
this.processorFeatures = processorFeatures;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getPubliclyAccessible}
* @param publiclyAccessible Indicates whether the DB instance is an internet-facing instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder publiclyAccessible(java.lang.Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getRemovalPolicy}
* @param removalPolicy The CloudFormation policy to apply when the instance is removed from the stack or replaced during an update.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder removalPolicy(software.amazon.awscdk.core.RemovalPolicy removalPolicy) {
this.removalPolicy = removalPolicy;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getS3ExportBuckets}
* @param s3ExportBuckets S3 buckets that you want to load data into.
* This property must not be used if s3ExportRole
is used.
*
* For Microsoft SQL Server:
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder s3ExportBuckets(java.util.List extends software.amazon.awscdk.services.s3.IBucket> s3ExportBuckets) {
this.s3ExportBuckets = (java.util.List)s3ExportBuckets;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getS3ExportRole}
* @param s3ExportRole Role that will be associated with this DB instance to enable S3 export.
* This property must not be used if s3ExportBuckets
is used.
*
* For Microsoft SQL Server:
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3ExportRole(software.amazon.awscdk.services.iam.IRole s3ExportRole) {
this.s3ExportRole = s3ExportRole;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getS3ImportBuckets}
* @param s3ImportBuckets S3 buckets that you want to load data from.
* This feature is only supported by the Microsoft SQL Server, Oracle, and PostgreSQL engines.
*
* This property must not be used if s3ImportRole
is used.
*
* For Microsoft SQL Server:
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder s3ImportBuckets(java.util.List extends software.amazon.awscdk.services.s3.IBucket> s3ImportBuckets) {
this.s3ImportBuckets = (java.util.List)s3ImportBuckets;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getS3ImportRole}
* @param s3ImportRole Role that will be associated with this DB instance to enable S3 import.
* This feature is only supported by the Microsoft SQL Server, Oracle, and PostgreSQL engines.
*
* This property must not be used if s3ImportBuckets
is used.
*
* For Microsoft SQL Server:
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3ImportRole(software.amazon.awscdk.services.iam.IRole s3ImportRole) {
this.s3ImportRole = s3ImportRole;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getSecurityGroups}
* @param securityGroups The security groups to assign to the DB instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder securityGroups(java.util.List extends software.amazon.awscdk.services.ec2.ISecurityGroup> securityGroups) {
this.securityGroups = (java.util.List)securityGroups;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getStorageType}
* @param storageType The storage type.
* Storage types supported are gp2, io1, standard.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder storageType(software.amazon.awscdk.services.rds.StorageType storageType) {
this.storageType = storageType;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getSubnetGroup}
* @param subnetGroup Existing subnet group for the instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subnetGroup(software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup) {
this.subnetGroup = subnetGroup;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getVpcPlacement}
* @param vpcPlacement The type of subnets to add to the created DB subnet group.
* @return {@code this}
* @deprecated use `vpcSubnets`
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder vpcPlacement(software.amazon.awscdk.services.ec2.SubnetSelection vpcPlacement) {
this.vpcPlacement = vpcPlacement;
return this;
}
/**
* Sets the value of {@link DatabaseInstanceSourceProps#getVpcSubnets}
* @param vpcSubnets The type of subnets to add to the created DB subnet group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DatabaseInstanceSourceProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DatabaseInstanceSourceProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DatabaseInstanceSourceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DatabaseInstanceSourceProps {
private final software.amazon.awscdk.services.rds.IInstanceEngine engine;
private final java.lang.Number allocatedStorage;
private final java.lang.Boolean allowMajorVersionUpgrade;
private final java.lang.String databaseName;
private final software.amazon.awscdk.services.ec2.InstanceType instanceType;
private final software.amazon.awscdk.services.rds.LicenseModel licenseModel;
private final java.util.Map parameters;
private final java.lang.String timezone;
private final software.amazon.awscdk.services.ec2.IVpc vpc;
private final java.lang.Boolean autoMinorVersionUpgrade;
private final java.lang.String availabilityZone;
private final software.amazon.awscdk.core.Duration backupRetention;
private final java.util.List cloudwatchLogsExports;
private final software.amazon.awscdk.services.logs.RetentionDays cloudwatchLogsRetention;
private final software.amazon.awscdk.services.iam.IRole cloudwatchLogsRetentionRole;
private final java.lang.Boolean copyTagsToSnapshot;
private final java.lang.Boolean deleteAutomatedBackups;
private final java.lang.Boolean deletionProtection;
private final java.lang.String domain;
private final software.amazon.awscdk.services.iam.IRole domainRole;
private final java.lang.Boolean enablePerformanceInsights;
private final java.lang.Boolean iamAuthentication;
private final java.lang.String instanceIdentifier;
private final java.lang.Number iops;
private final java.lang.Number maxAllocatedStorage;
private final software.amazon.awscdk.core.Duration monitoringInterval;
private final software.amazon.awscdk.services.iam.IRole monitoringRole;
private final java.lang.Boolean multiAz;
private final software.amazon.awscdk.services.rds.IOptionGroup optionGroup;
private final software.amazon.awscdk.services.rds.IParameterGroup parameterGroup;
private final software.amazon.awscdk.services.kms.IKey performanceInsightEncryptionKey;
private final software.amazon.awscdk.services.rds.PerformanceInsightRetention performanceInsightRetention;
private final java.lang.Number port;
private final java.lang.String preferredBackupWindow;
private final java.lang.String preferredMaintenanceWindow;
private final software.amazon.awscdk.services.rds.ProcessorFeatures processorFeatures;
private final java.lang.Boolean publiclyAccessible;
private final software.amazon.awscdk.core.RemovalPolicy removalPolicy;
private final java.util.List s3ExportBuckets;
private final software.amazon.awscdk.services.iam.IRole s3ExportRole;
private final java.util.List s3ImportBuckets;
private final software.amazon.awscdk.services.iam.IRole s3ImportRole;
private final java.util.List securityGroups;
private final software.amazon.awscdk.services.rds.StorageType storageType;
private final software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcPlacement;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.engine = software.amazon.jsii.Kernel.get(this, "engine", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IInstanceEngine.class));
this.allocatedStorage = software.amazon.jsii.Kernel.get(this, "allocatedStorage", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.allowMajorVersionUpgrade = software.amazon.jsii.Kernel.get(this, "allowMajorVersionUpgrade", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.databaseName = software.amazon.jsii.Kernel.get(this, "databaseName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.instanceType = software.amazon.jsii.Kernel.get(this, "instanceType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.InstanceType.class));
this.licenseModel = software.amazon.jsii.Kernel.get(this, "licenseModel", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.LicenseModel.class));
this.parameters = software.amazon.jsii.Kernel.get(this, "parameters", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.timezone = software.amazon.jsii.Kernel.get(this, "timezone", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpc = software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IVpc.class));
this.autoMinorVersionUpgrade = software.amazon.jsii.Kernel.get(this, "autoMinorVersionUpgrade", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.availabilityZone = software.amazon.jsii.Kernel.get(this, "availabilityZone", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.backupRetention = software.amazon.jsii.Kernel.get(this, "backupRetention", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.cloudwatchLogsExports = software.amazon.jsii.Kernel.get(this, "cloudwatchLogsExports", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.cloudwatchLogsRetention = software.amazon.jsii.Kernel.get(this, "cloudwatchLogsRetention", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.logs.RetentionDays.class));
this.cloudwatchLogsRetentionRole = software.amazon.jsii.Kernel.get(this, "cloudwatchLogsRetentionRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.copyTagsToSnapshot = software.amazon.jsii.Kernel.get(this, "copyTagsToSnapshot", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.deleteAutomatedBackups = software.amazon.jsii.Kernel.get(this, "deleteAutomatedBackups", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.deletionProtection = software.amazon.jsii.Kernel.get(this, "deletionProtection", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.domain = software.amazon.jsii.Kernel.get(this, "domain", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.domainRole = software.amazon.jsii.Kernel.get(this, "domainRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.enablePerformanceInsights = software.amazon.jsii.Kernel.get(this, "enablePerformanceInsights", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.iamAuthentication = software.amazon.jsii.Kernel.get(this, "iamAuthentication", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.instanceIdentifier = software.amazon.jsii.Kernel.get(this, "instanceIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.iops = software.amazon.jsii.Kernel.get(this, "iops", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.maxAllocatedStorage = software.amazon.jsii.Kernel.get(this, "maxAllocatedStorage", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.monitoringInterval = software.amazon.jsii.Kernel.get(this, "monitoringInterval", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.monitoringRole = software.amazon.jsii.Kernel.get(this, "monitoringRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.multiAz = software.amazon.jsii.Kernel.get(this, "multiAz", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.optionGroup = software.amazon.jsii.Kernel.get(this, "optionGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IOptionGroup.class));
this.parameterGroup = software.amazon.jsii.Kernel.get(this, "parameterGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IParameterGroup.class));
this.performanceInsightEncryptionKey = software.amazon.jsii.Kernel.get(this, "performanceInsightEncryptionKey", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.kms.IKey.class));
this.performanceInsightRetention = software.amazon.jsii.Kernel.get(this, "performanceInsightRetention", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.PerformanceInsightRetention.class));
this.port = software.amazon.jsii.Kernel.get(this, "port", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.preferredBackupWindow = software.amazon.jsii.Kernel.get(this, "preferredBackupWindow", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.preferredMaintenanceWindow = software.amazon.jsii.Kernel.get(this, "preferredMaintenanceWindow", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.processorFeatures = software.amazon.jsii.Kernel.get(this, "processorFeatures", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.ProcessorFeatures.class));
this.publiclyAccessible = software.amazon.jsii.Kernel.get(this, "publiclyAccessible", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.removalPolicy = software.amazon.jsii.Kernel.get(this, "removalPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.RemovalPolicy.class));
this.s3ExportBuckets = software.amazon.jsii.Kernel.get(this, "s3ExportBuckets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.s3.IBucket.class)));
this.s3ExportRole = software.amazon.jsii.Kernel.get(this, "s3ExportRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.s3ImportBuckets = software.amazon.jsii.Kernel.get(this, "s3ImportBuckets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.s3.IBucket.class)));
this.s3ImportRole = software.amazon.jsii.Kernel.get(this, "s3ImportRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.securityGroups = software.amazon.jsii.Kernel.get(this, "securityGroups", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.ISecurityGroup.class)));
this.storageType = software.amazon.jsii.Kernel.get(this, "storageType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.StorageType.class));
this.subnetGroup = software.amazon.jsii.Kernel.get(this, "subnetGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.ISubnetGroup.class));
this.vpcPlacement = software.amazon.jsii.Kernel.get(this, "vpcPlacement", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
this.vpcSubnets = software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.engine = java.util.Objects.requireNonNull(builder.engine, "engine is required");
this.allocatedStorage = builder.allocatedStorage;
this.allowMajorVersionUpgrade = builder.allowMajorVersionUpgrade;
this.databaseName = builder.databaseName;
this.instanceType = builder.instanceType;
this.licenseModel = builder.licenseModel;
this.parameters = builder.parameters;
this.timezone = builder.timezone;
this.vpc = java.util.Objects.requireNonNull(builder.vpc, "vpc is required");
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.availabilityZone = builder.availabilityZone;
this.backupRetention = builder.backupRetention;
this.cloudwatchLogsExports = builder.cloudwatchLogsExports;
this.cloudwatchLogsRetention = builder.cloudwatchLogsRetention;
this.cloudwatchLogsRetentionRole = builder.cloudwatchLogsRetentionRole;
this.copyTagsToSnapshot = builder.copyTagsToSnapshot;
this.deleteAutomatedBackups = builder.deleteAutomatedBackups;
this.deletionProtection = builder.deletionProtection;
this.domain = builder.domain;
this.domainRole = builder.domainRole;
this.enablePerformanceInsights = builder.enablePerformanceInsights;
this.iamAuthentication = builder.iamAuthentication;
this.instanceIdentifier = builder.instanceIdentifier;
this.iops = builder.iops;
this.maxAllocatedStorage = builder.maxAllocatedStorage;
this.monitoringInterval = builder.monitoringInterval;
this.monitoringRole = builder.monitoringRole;
this.multiAz = builder.multiAz;
this.optionGroup = builder.optionGroup;
this.parameterGroup = builder.parameterGroup;
this.performanceInsightEncryptionKey = builder.performanceInsightEncryptionKey;
this.performanceInsightRetention = builder.performanceInsightRetention;
this.port = builder.port;
this.preferredBackupWindow = builder.preferredBackupWindow;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.processorFeatures = builder.processorFeatures;
this.publiclyAccessible = builder.publiclyAccessible;
this.removalPolicy = builder.removalPolicy;
this.s3ExportBuckets = (java.util.List)builder.s3ExportBuckets;
this.s3ExportRole = builder.s3ExportRole;
this.s3ImportBuckets = (java.util.List)builder.s3ImportBuckets;
this.s3ImportRole = builder.s3ImportRole;
this.securityGroups = (java.util.List)builder.securityGroups;
this.storageType = builder.storageType;
this.subnetGroup = builder.subnetGroup;
this.vpcPlacement = builder.vpcPlacement;
this.vpcSubnets = builder.vpcSubnets;
}
@Override
public final software.amazon.awscdk.services.rds.IInstanceEngine getEngine() {
return this.engine;
}
@Override
public final java.lang.Number getAllocatedStorage() {
return this.allocatedStorage;
}
@Override
public final java.lang.Boolean getAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
@Override
public final java.lang.String getDatabaseName() {
return this.databaseName;
}
@Override
public final software.amazon.awscdk.services.ec2.InstanceType getInstanceType() {
return this.instanceType;
}
@Override
public final software.amazon.awscdk.services.rds.LicenseModel getLicenseModel() {
return this.licenseModel;
}
@Override
public final java.util.Map getParameters() {
return this.parameters;
}
@Override
public final java.lang.String getTimezone() {
return this.timezone;
}
@Override
public final software.amazon.awscdk.services.ec2.IVpc getVpc() {
return this.vpc;
}
@Override
public final java.lang.Boolean getAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
@Override
public final java.lang.String getAvailabilityZone() {
return this.availabilityZone;
}
@Override
public final software.amazon.awscdk.core.Duration getBackupRetention() {
return this.backupRetention;
}
@Override
public final java.util.List getCloudwatchLogsExports() {
return this.cloudwatchLogsExports;
}
@Override
public final software.amazon.awscdk.services.logs.RetentionDays getCloudwatchLogsRetention() {
return this.cloudwatchLogsRetention;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getCloudwatchLogsRetentionRole() {
return this.cloudwatchLogsRetentionRole;
}
@Override
public final java.lang.Boolean getCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
@Override
public final java.lang.Boolean getDeleteAutomatedBackups() {
return this.deleteAutomatedBackups;
}
@Override
public final java.lang.Boolean getDeletionProtection() {
return this.deletionProtection;
}
@Override
public final java.lang.String getDomain() {
return this.domain;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getDomainRole() {
return this.domainRole;
}
@Override
public final java.lang.Boolean getEnablePerformanceInsights() {
return this.enablePerformanceInsights;
}
@Override
public final java.lang.Boolean getIamAuthentication() {
return this.iamAuthentication;
}
@Override
public final java.lang.String getInstanceIdentifier() {
return this.instanceIdentifier;
}
@Override
public final java.lang.Number getIops() {
return this.iops;
}
@Override
public final java.lang.Number getMaxAllocatedStorage() {
return this.maxAllocatedStorage;
}
@Override
public final software.amazon.awscdk.core.Duration getMonitoringInterval() {
return this.monitoringInterval;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getMonitoringRole() {
return this.monitoringRole;
}
@Override
public final java.lang.Boolean getMultiAz() {
return this.multiAz;
}
@Override
public final software.amazon.awscdk.services.rds.IOptionGroup getOptionGroup() {
return this.optionGroup;
}
@Override
public final software.amazon.awscdk.services.rds.IParameterGroup getParameterGroup() {
return this.parameterGroup;
}
@Override
public final software.amazon.awscdk.services.kms.IKey getPerformanceInsightEncryptionKey() {
return this.performanceInsightEncryptionKey;
}
@Override
public final software.amazon.awscdk.services.rds.PerformanceInsightRetention getPerformanceInsightRetention() {
return this.performanceInsightRetention;
}
@Override
public final java.lang.Number getPort() {
return this.port;
}
@Override
public final java.lang.String getPreferredBackupWindow() {
return this.preferredBackupWindow;
}
@Override
public final java.lang.String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
@Override
public final software.amazon.awscdk.services.rds.ProcessorFeatures getProcessorFeatures() {
return this.processorFeatures;
}
@Override
public final java.lang.Boolean getPubliclyAccessible() {
return this.publiclyAccessible;
}
@Override
public final software.amazon.awscdk.core.RemovalPolicy getRemovalPolicy() {
return this.removalPolicy;
}
@Override
public final java.util.List getS3ExportBuckets() {
return this.s3ExportBuckets;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getS3ExportRole() {
return this.s3ExportRole;
}
@Override
public final java.util.List getS3ImportBuckets() {
return this.s3ImportBuckets;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getS3ImportRole() {
return this.s3ImportRole;
}
@Override
public final java.util.List getSecurityGroups() {
return this.securityGroups;
}
@Override
public final software.amazon.awscdk.services.rds.StorageType getStorageType() {
return this.storageType;
}
@Override
public final software.amazon.awscdk.services.rds.ISubnetGroup getSubnetGroup() {
return this.subnetGroup;
}
@Override
public final software.amazon.awscdk.services.ec2.SubnetSelection getVpcPlacement() {
return this.vpcPlacement;
}
@Override
public final software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("engine", om.valueToTree(this.getEngine()));
if (this.getAllocatedStorage() != null) {
data.set("allocatedStorage", om.valueToTree(this.getAllocatedStorage()));
}
if (this.getAllowMajorVersionUpgrade() != null) {
data.set("allowMajorVersionUpgrade", om.valueToTree(this.getAllowMajorVersionUpgrade()));
}
if (this.getDatabaseName() != null) {
data.set("databaseName", om.valueToTree(this.getDatabaseName()));
}
if (this.getInstanceType() != null) {
data.set("instanceType", om.valueToTree(this.getInstanceType()));
}
if (this.getLicenseModel() != null) {
data.set("licenseModel", om.valueToTree(this.getLicenseModel()));
}
if (this.getParameters() != null) {
data.set("parameters", om.valueToTree(this.getParameters()));
}
if (this.getTimezone() != null) {
data.set("timezone", om.valueToTree(this.getTimezone()));
}
data.set("vpc", om.valueToTree(this.getVpc()));
if (this.getAutoMinorVersionUpgrade() != null) {
data.set("autoMinorVersionUpgrade", om.valueToTree(this.getAutoMinorVersionUpgrade()));
}
if (this.getAvailabilityZone() != null) {
data.set("availabilityZone", om.valueToTree(this.getAvailabilityZone()));
}
if (this.getBackupRetention() != null) {
data.set("backupRetention", om.valueToTree(this.getBackupRetention()));
}
if (this.getCloudwatchLogsExports() != null) {
data.set("cloudwatchLogsExports", om.valueToTree(this.getCloudwatchLogsExports()));
}
if (this.getCloudwatchLogsRetention() != null) {
data.set("cloudwatchLogsRetention", om.valueToTree(this.getCloudwatchLogsRetention()));
}
if (this.getCloudwatchLogsRetentionRole() != null) {
data.set("cloudwatchLogsRetentionRole", om.valueToTree(this.getCloudwatchLogsRetentionRole()));
}
if (this.getCopyTagsToSnapshot() != null) {
data.set("copyTagsToSnapshot", om.valueToTree(this.getCopyTagsToSnapshot()));
}
if (this.getDeleteAutomatedBackups() != null) {
data.set("deleteAutomatedBackups", om.valueToTree(this.getDeleteAutomatedBackups()));
}
if (this.getDeletionProtection() != null) {
data.set("deletionProtection", om.valueToTree(this.getDeletionProtection()));
}
if (this.getDomain() != null) {
data.set("domain", om.valueToTree(this.getDomain()));
}
if (this.getDomainRole() != null) {
data.set("domainRole", om.valueToTree(this.getDomainRole()));
}
if (this.getEnablePerformanceInsights() != null) {
data.set("enablePerformanceInsights", om.valueToTree(this.getEnablePerformanceInsights()));
}
if (this.getIamAuthentication() != null) {
data.set("iamAuthentication", om.valueToTree(this.getIamAuthentication()));
}
if (this.getInstanceIdentifier() != null) {
data.set("instanceIdentifier", om.valueToTree(this.getInstanceIdentifier()));
}
if (this.getIops() != null) {
data.set("iops", om.valueToTree(this.getIops()));
}
if (this.getMaxAllocatedStorage() != null) {
data.set("maxAllocatedStorage", om.valueToTree(this.getMaxAllocatedStorage()));
}
if (this.getMonitoringInterval() != null) {
data.set("monitoringInterval", om.valueToTree(this.getMonitoringInterval()));
}
if (this.getMonitoringRole() != null) {
data.set("monitoringRole", om.valueToTree(this.getMonitoringRole()));
}
if (this.getMultiAz() != null) {
data.set("multiAz", om.valueToTree(this.getMultiAz()));
}
if (this.getOptionGroup() != null) {
data.set("optionGroup", om.valueToTree(this.getOptionGroup()));
}
if (this.getParameterGroup() != null) {
data.set("parameterGroup", om.valueToTree(this.getParameterGroup()));
}
if (this.getPerformanceInsightEncryptionKey() != null) {
data.set("performanceInsightEncryptionKey", om.valueToTree(this.getPerformanceInsightEncryptionKey()));
}
if (this.getPerformanceInsightRetention() != null) {
data.set("performanceInsightRetention", om.valueToTree(this.getPerformanceInsightRetention()));
}
if (this.getPort() != null) {
data.set("port", om.valueToTree(this.getPort()));
}
if (this.getPreferredBackupWindow() != null) {
data.set("preferredBackupWindow", om.valueToTree(this.getPreferredBackupWindow()));
}
if (this.getPreferredMaintenanceWindow() != null) {
data.set("preferredMaintenanceWindow", om.valueToTree(this.getPreferredMaintenanceWindow()));
}
if (this.getProcessorFeatures() != null) {
data.set("processorFeatures", om.valueToTree(this.getProcessorFeatures()));
}
if (this.getPubliclyAccessible() != null) {
data.set("publiclyAccessible", om.valueToTree(this.getPubliclyAccessible()));
}
if (this.getRemovalPolicy() != null) {
data.set("removalPolicy", om.valueToTree(this.getRemovalPolicy()));
}
if (this.getS3ExportBuckets() != null) {
data.set("s3ExportBuckets", om.valueToTree(this.getS3ExportBuckets()));
}
if (this.getS3ExportRole() != null) {
data.set("s3ExportRole", om.valueToTree(this.getS3ExportRole()));
}
if (this.getS3ImportBuckets() != null) {
data.set("s3ImportBuckets", om.valueToTree(this.getS3ImportBuckets()));
}
if (this.getS3ImportRole() != null) {
data.set("s3ImportRole", om.valueToTree(this.getS3ImportRole()));
}
if (this.getSecurityGroups() != null) {
data.set("securityGroups", om.valueToTree(this.getSecurityGroups()));
}
if (this.getStorageType() != null) {
data.set("storageType", om.valueToTree(this.getStorageType()));
}
if (this.getSubnetGroup() != null) {
data.set("subnetGroup", om.valueToTree(this.getSubnetGroup()));
}
if (this.getVpcPlacement() != null) {
data.set("vpcPlacement", om.valueToTree(this.getVpcPlacement()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.DatabaseInstanceSourceProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DatabaseInstanceSourceProps.Jsii$Proxy that = (DatabaseInstanceSourceProps.Jsii$Proxy) o;
if (!engine.equals(that.engine)) return false;
if (this.allocatedStorage != null ? !this.allocatedStorage.equals(that.allocatedStorage) : that.allocatedStorage != null) return false;
if (this.allowMajorVersionUpgrade != null ? !this.allowMajorVersionUpgrade.equals(that.allowMajorVersionUpgrade) : that.allowMajorVersionUpgrade != null) return false;
if (this.databaseName != null ? !this.databaseName.equals(that.databaseName) : that.databaseName != null) return false;
if (this.instanceType != null ? !this.instanceType.equals(that.instanceType) : that.instanceType != null) return false;
if (this.licenseModel != null ? !this.licenseModel.equals(that.licenseModel) : that.licenseModel != null) return false;
if (this.parameters != null ? !this.parameters.equals(that.parameters) : that.parameters != null) return false;
if (this.timezone != null ? !this.timezone.equals(that.timezone) : that.timezone != null) return false;
if (!vpc.equals(that.vpc)) return false;
if (this.autoMinorVersionUpgrade != null ? !this.autoMinorVersionUpgrade.equals(that.autoMinorVersionUpgrade) : that.autoMinorVersionUpgrade != null) return false;
if (this.availabilityZone != null ? !this.availabilityZone.equals(that.availabilityZone) : that.availabilityZone != null) return false;
if (this.backupRetention != null ? !this.backupRetention.equals(that.backupRetention) : that.backupRetention != null) return false;
if (this.cloudwatchLogsExports != null ? !this.cloudwatchLogsExports.equals(that.cloudwatchLogsExports) : that.cloudwatchLogsExports != null) return false;
if (this.cloudwatchLogsRetention != null ? !this.cloudwatchLogsRetention.equals(that.cloudwatchLogsRetention) : that.cloudwatchLogsRetention != null) return false;
if (this.cloudwatchLogsRetentionRole != null ? !this.cloudwatchLogsRetentionRole.equals(that.cloudwatchLogsRetentionRole) : that.cloudwatchLogsRetentionRole != null) return false;
if (this.copyTagsToSnapshot != null ? !this.copyTagsToSnapshot.equals(that.copyTagsToSnapshot) : that.copyTagsToSnapshot != null) return false;
if (this.deleteAutomatedBackups != null ? !this.deleteAutomatedBackups.equals(that.deleteAutomatedBackups) : that.deleteAutomatedBackups != null) return false;
if (this.deletionProtection != null ? !this.deletionProtection.equals(that.deletionProtection) : that.deletionProtection != null) return false;
if (this.domain != null ? !this.domain.equals(that.domain) : that.domain != null) return false;
if (this.domainRole != null ? !this.domainRole.equals(that.domainRole) : that.domainRole != null) return false;
if (this.enablePerformanceInsights != null ? !this.enablePerformanceInsights.equals(that.enablePerformanceInsights) : that.enablePerformanceInsights != null) return false;
if (this.iamAuthentication != null ? !this.iamAuthentication.equals(that.iamAuthentication) : that.iamAuthentication != null) return false;
if (this.instanceIdentifier != null ? !this.instanceIdentifier.equals(that.instanceIdentifier) : that.instanceIdentifier != null) return false;
if (this.iops != null ? !this.iops.equals(that.iops) : that.iops != null) return false;
if (this.maxAllocatedStorage != null ? !this.maxAllocatedStorage.equals(that.maxAllocatedStorage) : that.maxAllocatedStorage != null) return false;
if (this.monitoringInterval != null ? !this.monitoringInterval.equals(that.monitoringInterval) : that.monitoringInterval != null) return false;
if (this.monitoringRole != null ? !this.monitoringRole.equals(that.monitoringRole) : that.monitoringRole != null) return false;
if (this.multiAz != null ? !this.multiAz.equals(that.multiAz) : that.multiAz != null) return false;
if (this.optionGroup != null ? !this.optionGroup.equals(that.optionGroup) : that.optionGroup != null) return false;
if (this.parameterGroup != null ? !this.parameterGroup.equals(that.parameterGroup) : that.parameterGroup != null) return false;
if (this.performanceInsightEncryptionKey != null ? !this.performanceInsightEncryptionKey.equals(that.performanceInsightEncryptionKey) : that.performanceInsightEncryptionKey != null) return false;
if (this.performanceInsightRetention != null ? !this.performanceInsightRetention.equals(that.performanceInsightRetention) : that.performanceInsightRetention != null) return false;
if (this.port != null ? !this.port.equals(that.port) : that.port != null) return false;
if (this.preferredBackupWindow != null ? !this.preferredBackupWindow.equals(that.preferredBackupWindow) : that.preferredBackupWindow != null) return false;
if (this.preferredMaintenanceWindow != null ? !this.preferredMaintenanceWindow.equals(that.preferredMaintenanceWindow) : that.preferredMaintenanceWindow != null) return false;
if (this.processorFeatures != null ? !this.processorFeatures.equals(that.processorFeatures) : that.processorFeatures != null) return false;
if (this.publiclyAccessible != null ? !this.publiclyAccessible.equals(that.publiclyAccessible) : that.publiclyAccessible != null) return false;
if (this.removalPolicy != null ? !this.removalPolicy.equals(that.removalPolicy) : that.removalPolicy != null) return false;
if (this.s3ExportBuckets != null ? !this.s3ExportBuckets.equals(that.s3ExportBuckets) : that.s3ExportBuckets != null) return false;
if (this.s3ExportRole != null ? !this.s3ExportRole.equals(that.s3ExportRole) : that.s3ExportRole != null) return false;
if (this.s3ImportBuckets != null ? !this.s3ImportBuckets.equals(that.s3ImportBuckets) : that.s3ImportBuckets != null) return false;
if (this.s3ImportRole != null ? !this.s3ImportRole.equals(that.s3ImportRole) : that.s3ImportRole != null) return false;
if (this.securityGroups != null ? !this.securityGroups.equals(that.securityGroups) : that.securityGroups != null) return false;
if (this.storageType != null ? !this.storageType.equals(that.storageType) : that.storageType != null) return false;
if (this.subnetGroup != null ? !this.subnetGroup.equals(that.subnetGroup) : that.subnetGroup != null) return false;
if (this.vpcPlacement != null ? !this.vpcPlacement.equals(that.vpcPlacement) : that.vpcPlacement != null) return false;
return this.vpcSubnets != null ? this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets == null;
}
@Override
public final int hashCode() {
int result = this.engine.hashCode();
result = 31 * result + (this.allocatedStorage != null ? this.allocatedStorage.hashCode() : 0);
result = 31 * result + (this.allowMajorVersionUpgrade != null ? this.allowMajorVersionUpgrade.hashCode() : 0);
result = 31 * result + (this.databaseName != null ? this.databaseName.hashCode() : 0);
result = 31 * result + (this.instanceType != null ? this.instanceType.hashCode() : 0);
result = 31 * result + (this.licenseModel != null ? this.licenseModel.hashCode() : 0);
result = 31 * result + (this.parameters != null ? this.parameters.hashCode() : 0);
result = 31 * result + (this.timezone != null ? this.timezone.hashCode() : 0);
result = 31 * result + (this.vpc.hashCode());
result = 31 * result + (this.autoMinorVersionUpgrade != null ? this.autoMinorVersionUpgrade.hashCode() : 0);
result = 31 * result + (this.availabilityZone != null ? this.availabilityZone.hashCode() : 0);
result = 31 * result + (this.backupRetention != null ? this.backupRetention.hashCode() : 0);
result = 31 * result + (this.cloudwatchLogsExports != null ? this.cloudwatchLogsExports.hashCode() : 0);
result = 31 * result + (this.cloudwatchLogsRetention != null ? this.cloudwatchLogsRetention.hashCode() : 0);
result = 31 * result + (this.cloudwatchLogsRetentionRole != null ? this.cloudwatchLogsRetentionRole.hashCode() : 0);
result = 31 * result + (this.copyTagsToSnapshot != null ? this.copyTagsToSnapshot.hashCode() : 0);
result = 31 * result + (this.deleteAutomatedBackups != null ? this.deleteAutomatedBackups.hashCode() : 0);
result = 31 * result + (this.deletionProtection != null ? this.deletionProtection.hashCode() : 0);
result = 31 * result + (this.domain != null ? this.domain.hashCode() : 0);
result = 31 * result + (this.domainRole != null ? this.domainRole.hashCode() : 0);
result = 31 * result + (this.enablePerformanceInsights != null ? this.enablePerformanceInsights.hashCode() : 0);
result = 31 * result + (this.iamAuthentication != null ? this.iamAuthentication.hashCode() : 0);
result = 31 * result + (this.instanceIdentifier != null ? this.instanceIdentifier.hashCode() : 0);
result = 31 * result + (this.iops != null ? this.iops.hashCode() : 0);
result = 31 * result + (this.maxAllocatedStorage != null ? this.maxAllocatedStorage.hashCode() : 0);
result = 31 * result + (this.monitoringInterval != null ? this.monitoringInterval.hashCode() : 0);
result = 31 * result + (this.monitoringRole != null ? this.monitoringRole.hashCode() : 0);
result = 31 * result + (this.multiAz != null ? this.multiAz.hashCode() : 0);
result = 31 * result + (this.optionGroup != null ? this.optionGroup.hashCode() : 0);
result = 31 * result + (this.parameterGroup != null ? this.parameterGroup.hashCode() : 0);
result = 31 * result + (this.performanceInsightEncryptionKey != null ? this.performanceInsightEncryptionKey.hashCode() : 0);
result = 31 * result + (this.performanceInsightRetention != null ? this.performanceInsightRetention.hashCode() : 0);
result = 31 * result + (this.port != null ? this.port.hashCode() : 0);
result = 31 * result + (this.preferredBackupWindow != null ? this.preferredBackupWindow.hashCode() : 0);
result = 31 * result + (this.preferredMaintenanceWindow != null ? this.preferredMaintenanceWindow.hashCode() : 0);
result = 31 * result + (this.processorFeatures != null ? this.processorFeatures.hashCode() : 0);
result = 31 * result + (this.publiclyAccessible != null ? this.publiclyAccessible.hashCode() : 0);
result = 31 * result + (this.removalPolicy != null ? this.removalPolicy.hashCode() : 0);
result = 31 * result + (this.s3ExportBuckets != null ? this.s3ExportBuckets.hashCode() : 0);
result = 31 * result + (this.s3ExportRole != null ? this.s3ExportRole.hashCode() : 0);
result = 31 * result + (this.s3ImportBuckets != null ? this.s3ImportBuckets.hashCode() : 0);
result = 31 * result + (this.s3ImportRole != null ? this.s3ImportRole.hashCode() : 0);
result = 31 * result + (this.securityGroups != null ? this.securityGroups.hashCode() : 0);
result = 31 * result + (this.storageType != null ? this.storageType.hashCode() : 0);
result = 31 * result + (this.subnetGroup != null ? this.subnetGroup.hashCode() : 0);
result = 31 * result + (this.vpcPlacement != null ? this.vpcPlacement.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
return result;
}
}
}