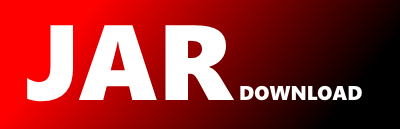
software.amazon.awscdk.services.rds.CfnDBProxyEndpointProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.rds;
/**
* Properties for defining a CfnDBProxyEndpoint
.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.rds.*;
* CfnDBProxyEndpointProps cfnDBProxyEndpointProps = CfnDBProxyEndpointProps.builder()
* .dbProxyEndpointName("dbProxyEndpointName")
* .dbProxyName("dbProxyName")
* .vpcSubnetIds(List.of("vpcSubnetIds"))
* // the properties below are optional
* .tags(List.of(TagFormatProperty.builder()
* .key("key")
* .value("value")
* .build()))
* .targetRole("targetRole")
* .vpcSecurityGroupIds(List.of("vpcSecurityGroupIds"))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:49.032Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.CfnDBProxyEndpointProps")
@software.amazon.jsii.Jsii.Proxy(CfnDBProxyEndpointProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnDBProxyEndpointProps extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the DB proxy endpoint to create.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getDbProxyEndpointName();
/**
* The name of the DB proxy associated with the DB proxy endpoint that you create.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getDbProxyName();
/**
* The VPC subnet IDs for the DB proxy endpoint that you create.
*
* You can specify a different set of subnet IDs than for the original DB proxy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getVpcSubnetIds();
/**
* An optional set of key-value pairs to associate arbitrary data of your choosing with the proxy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* A value that indicates whether the DB proxy endpoint can be used for read/write or read-only operations.
*
* Valid Values: READ_WRITE | READ_ONLY
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTargetRole() {
return null;
}
/**
* The VPC security group IDs for the DB proxy endpoint that you create.
*
* You can specify a different set of security group IDs than for the original DB proxy. The default is the default security group for the VPC.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getVpcSecurityGroupIds() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnDBProxyEndpointProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnDBProxyEndpointProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String dbProxyEndpointName;
java.lang.String dbProxyName;
java.util.List vpcSubnetIds;
java.util.List tags;
java.lang.String targetRole;
java.util.List vpcSecurityGroupIds;
/**
* Sets the value of {@link CfnDBProxyEndpointProps#getDbProxyEndpointName}
* @param dbProxyEndpointName The name of the DB proxy endpoint to create. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dbProxyEndpointName(java.lang.String dbProxyEndpointName) {
this.dbProxyEndpointName = dbProxyEndpointName;
return this;
}
/**
* Sets the value of {@link CfnDBProxyEndpointProps#getDbProxyName}
* @param dbProxyName The name of the DB proxy associated with the DB proxy endpoint that you create. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dbProxyName(java.lang.String dbProxyName) {
this.dbProxyName = dbProxyName;
return this;
}
/**
* Sets the value of {@link CfnDBProxyEndpointProps#getVpcSubnetIds}
* @param vpcSubnetIds The VPC subnet IDs for the DB proxy endpoint that you create. This parameter is required.
* You can specify a different set of subnet IDs than for the original DB proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnetIds(java.util.List vpcSubnetIds) {
this.vpcSubnetIds = vpcSubnetIds;
return this;
}
/**
* Sets the value of {@link CfnDBProxyEndpointProps#getTags}
* @param tags An optional set of key-value pairs to associate arbitrary data of your choosing with the proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.services.rds.CfnDBProxyEndpoint.TagFormatProperty> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Sets the value of {@link CfnDBProxyEndpointProps#getTargetRole}
* @param targetRole A value that indicates whether the DB proxy endpoint can be used for read/write or read-only operations.
* Valid Values: READ_WRITE | READ_ONLY
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder targetRole(java.lang.String targetRole) {
this.targetRole = targetRole;
return this;
}
/**
* Sets the value of {@link CfnDBProxyEndpointProps#getVpcSecurityGroupIds}
* @param vpcSecurityGroupIds The VPC security group IDs for the DB proxy endpoint that you create.
* You can specify a different set of security group IDs than for the original DB proxy. The default is the default security group for the VPC.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSecurityGroupIds(java.util.List vpcSecurityGroupIds) {
this.vpcSecurityGroupIds = vpcSecurityGroupIds;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnDBProxyEndpointProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnDBProxyEndpointProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnDBProxyEndpointProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnDBProxyEndpointProps {
private final java.lang.String dbProxyEndpointName;
private final java.lang.String dbProxyName;
private final java.util.List vpcSubnetIds;
private final java.util.List tags;
private final java.lang.String targetRole;
private final java.util.List vpcSecurityGroupIds;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.dbProxyEndpointName = software.amazon.jsii.Kernel.get(this, "dbProxyEndpointName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.dbProxyName = software.amazon.jsii.Kernel.get(this, "dbProxyName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpcSubnetIds = software.amazon.jsii.Kernel.get(this, "vpcSubnetIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.CfnDBProxyEndpoint.TagFormatProperty.class)));
this.targetRole = software.amazon.jsii.Kernel.get(this, "targetRole", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpcSecurityGroupIds = software.amazon.jsii.Kernel.get(this, "vpcSecurityGroupIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.dbProxyEndpointName = java.util.Objects.requireNonNull(builder.dbProxyEndpointName, "dbProxyEndpointName is required");
this.dbProxyName = java.util.Objects.requireNonNull(builder.dbProxyName, "dbProxyName is required");
this.vpcSubnetIds = java.util.Objects.requireNonNull(builder.vpcSubnetIds, "vpcSubnetIds is required");
this.tags = (java.util.List)builder.tags;
this.targetRole = builder.targetRole;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
}
@Override
public final java.lang.String getDbProxyEndpointName() {
return this.dbProxyEndpointName;
}
@Override
public final java.lang.String getDbProxyName() {
return this.dbProxyName;
}
@Override
public final java.util.List getVpcSubnetIds() {
return this.vpcSubnetIds;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
public final java.lang.String getTargetRole() {
return this.targetRole;
}
@Override
public final java.util.List getVpcSecurityGroupIds() {
return this.vpcSecurityGroupIds;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("dbProxyEndpointName", om.valueToTree(this.getDbProxyEndpointName()));
data.set("dbProxyName", om.valueToTree(this.getDbProxyName()));
data.set("vpcSubnetIds", om.valueToTree(this.getVpcSubnetIds()));
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getTargetRole() != null) {
data.set("targetRole", om.valueToTree(this.getTargetRole()));
}
if (this.getVpcSecurityGroupIds() != null) {
data.set("vpcSecurityGroupIds", om.valueToTree(this.getVpcSecurityGroupIds()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.CfnDBProxyEndpointProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnDBProxyEndpointProps.Jsii$Proxy that = (CfnDBProxyEndpointProps.Jsii$Proxy) o;
if (!dbProxyEndpointName.equals(that.dbProxyEndpointName)) return false;
if (!dbProxyName.equals(that.dbProxyName)) return false;
if (!vpcSubnetIds.equals(that.vpcSubnetIds)) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
if (this.targetRole != null ? !this.targetRole.equals(that.targetRole) : that.targetRole != null) return false;
return this.vpcSecurityGroupIds != null ? this.vpcSecurityGroupIds.equals(that.vpcSecurityGroupIds) : that.vpcSecurityGroupIds == null;
}
@Override
public final int hashCode() {
int result = this.dbProxyEndpointName.hashCode();
result = 31 * result + (this.dbProxyName.hashCode());
result = 31 * result + (this.vpcSubnetIds.hashCode());
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.targetRole != null ? this.targetRole.hashCode() : 0);
result = 31 * result + (this.vpcSecurityGroupIds != null ? this.vpcSecurityGroupIds.hashCode() : 0);
return result;
}
}
}