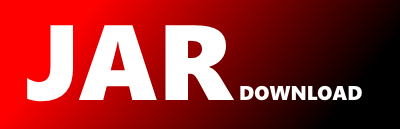
software.amazon.awscdk.services.rds.CfnDBProxyProps Maven / Gradle / Ivy
Show all versions of rds Show documentation
package software.amazon.awscdk.services.rds;
/**
* Properties for defining a CfnDBProxy
.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.rds.*;
* CfnDBProxyProps cfnDBProxyProps = CfnDBProxyProps.builder()
* .auth(List.of(AuthFormatProperty.builder()
* .authScheme("authScheme")
* .clientPasswordAuthType("clientPasswordAuthType")
* .description("description")
* .iamAuth("iamAuth")
* .secretArn("secretArn")
* .build()))
* .dbProxyName("dbProxyName")
* .engineFamily("engineFamily")
* .roleArn("roleArn")
* .vpcSubnetIds(List.of("vpcSubnetIds"))
* // the properties below are optional
* .debugLogging(false)
* .idleClientTimeout(123)
* .requireTls(false)
* .tags(List.of(TagFormatProperty.builder()
* .key("key")
* .value("value")
* .build()))
* .vpcSecurityGroupIds(List.of("vpcSecurityGroupIds"))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:49.045Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.CfnDBProxyProps")
@software.amazon.jsii.Jsii.Proxy(CfnDBProxyProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnDBProxyProps extends software.amazon.jsii.JsiiSerializable {
/**
* The authorization mechanism that the proxy uses.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getAuth();
/**
* The identifier for the proxy.
*
* This name must be unique for all proxies owned by your AWS account in the specified AWS Region . An identifier must begin with a letter and must contain only ASCII letters, digits, and hyphens; it can't end with a hyphen or contain two consecutive hyphens.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getDbProxyName();
/**
* The kinds of databases that the proxy can connect to.
*
* This value determines which database network protocol the proxy recognizes when it interprets network traffic to and from the database. For Aurora MySQL, RDS for MariaDB, and RDS for MySQL databases, specify MYSQL
. For Aurora PostgreSQL and RDS for PostgreSQL databases, specify POSTGRESQL
. For RDS for Microsoft SQL Server, specify SQLSERVER
.
*
* Valid values : MYSQL
| POSTGRESQL
| SQLSERVER
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getEngineFamily();
/**
* The Amazon Resource Name (ARN) of the IAM role that the proxy uses to access secrets in AWS Secrets Manager.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRoleArn();
/**
* One or more VPC subnet IDs to associate with the new proxy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getVpcSubnetIds();
/**
* Whether the proxy includes detailed information about SQL statements in its logs.
*
* This information helps you to debug issues involving SQL behavior or the performance and scalability of the proxy connections. The debug information includes the text of SQL statements that you submit through the proxy. Thus, only enable this setting when needed for debugging, and only when you have security measures in place to safeguard any sensitive information that appears in the logs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDebugLogging() {
return null;
}
/**
* The number of seconds that a connection to the proxy can be inactive before the proxy disconnects it.
*
* You can set this value higher or lower than the connection timeout limit for the associated database.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getIdleClientTimeout() {
return null;
}
/**
* A Boolean parameter that specifies whether Transport Layer Security (TLS) encryption is required for connections to the proxy.
*
* By enabling this setting, you can enforce encrypted TLS connections to the proxy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getRequireTls() {
return null;
}
/**
* An optional set of key-value pairs to associate arbitrary data of your choosing with the proxy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* One or more VPC security group IDs to associate with the new proxy.
*
* If you plan to update the resource, don't specify VPC security groups in a shared VPC.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getVpcSecurityGroupIds() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnDBProxyProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnDBProxyProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object auth;
java.lang.String dbProxyName;
java.lang.String engineFamily;
java.lang.String roleArn;
java.util.List vpcSubnetIds;
java.lang.Object debugLogging;
java.lang.Number idleClientTimeout;
java.lang.Object requireTls;
java.util.List tags;
java.util.List vpcSecurityGroupIds;
/**
* Sets the value of {@link CfnDBProxyProps#getAuth}
* @param auth The authorization mechanism that the proxy uses. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder auth(software.amazon.awscdk.core.IResolvable auth) {
this.auth = auth;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getAuth}
* @param auth The authorization mechanism that the proxy uses. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder auth(java.util.List extends java.lang.Object> auth) {
this.auth = auth;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getDbProxyName}
* @param dbProxyName The identifier for the proxy. This parameter is required.
* This name must be unique for all proxies owned by your AWS account in the specified AWS Region . An identifier must begin with a letter and must contain only ASCII letters, digits, and hyphens; it can't end with a hyphen or contain two consecutive hyphens.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dbProxyName(java.lang.String dbProxyName) {
this.dbProxyName = dbProxyName;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getEngineFamily}
* @param engineFamily The kinds of databases that the proxy can connect to. This parameter is required.
* This value determines which database network protocol the proxy recognizes when it interprets network traffic to and from the database. For Aurora MySQL, RDS for MariaDB, and RDS for MySQL databases, specify MYSQL
. For Aurora PostgreSQL and RDS for PostgreSQL databases, specify POSTGRESQL
. For RDS for Microsoft SQL Server, specify SQLSERVER
.
*
* Valid values : MYSQL
| POSTGRESQL
| SQLSERVER
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder engineFamily(java.lang.String engineFamily) {
this.engineFamily = engineFamily;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getRoleArn}
* @param roleArn The Amazon Resource Name (ARN) of the IAM role that the proxy uses to access secrets in AWS Secrets Manager. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder roleArn(java.lang.String roleArn) {
this.roleArn = roleArn;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getVpcSubnetIds}
* @param vpcSubnetIds One or more VPC subnet IDs to associate with the new proxy. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnetIds(java.util.List vpcSubnetIds) {
this.vpcSubnetIds = vpcSubnetIds;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getDebugLogging}
* @param debugLogging Whether the proxy includes detailed information about SQL statements in its logs.
* This information helps you to debug issues involving SQL behavior or the performance and scalability of the proxy connections. The debug information includes the text of SQL statements that you submit through the proxy. Thus, only enable this setting when needed for debugging, and only when you have security measures in place to safeguard any sensitive information that appears in the logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder debugLogging(java.lang.Boolean debugLogging) {
this.debugLogging = debugLogging;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getDebugLogging}
* @param debugLogging Whether the proxy includes detailed information about SQL statements in its logs.
* This information helps you to debug issues involving SQL behavior or the performance and scalability of the proxy connections. The debug information includes the text of SQL statements that you submit through the proxy. Thus, only enable this setting when needed for debugging, and only when you have security measures in place to safeguard any sensitive information that appears in the logs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder debugLogging(software.amazon.awscdk.core.IResolvable debugLogging) {
this.debugLogging = debugLogging;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getIdleClientTimeout}
* @param idleClientTimeout The number of seconds that a connection to the proxy can be inactive before the proxy disconnects it.
* You can set this value higher or lower than the connection timeout limit for the associated database.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder idleClientTimeout(java.lang.Number idleClientTimeout) {
this.idleClientTimeout = idleClientTimeout;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getRequireTls}
* @param requireTls A Boolean parameter that specifies whether Transport Layer Security (TLS) encryption is required for connections to the proxy.
* By enabling this setting, you can enforce encrypted TLS connections to the proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder requireTls(java.lang.Boolean requireTls) {
this.requireTls = requireTls;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getRequireTls}
* @param requireTls A Boolean parameter that specifies whether Transport Layer Security (TLS) encryption is required for connections to the proxy.
* By enabling this setting, you can enforce encrypted TLS connections to the proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder requireTls(software.amazon.awscdk.core.IResolvable requireTls) {
this.requireTls = requireTls;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getTags}
* @param tags An optional set of key-value pairs to associate arbitrary data of your choosing with the proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.services.rds.CfnDBProxy.TagFormatProperty> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Sets the value of {@link CfnDBProxyProps#getVpcSecurityGroupIds}
* @param vpcSecurityGroupIds One or more VPC security group IDs to associate with the new proxy.
* If you plan to update the resource, don't specify VPC security groups in a shared VPC.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSecurityGroupIds(java.util.List vpcSecurityGroupIds) {
this.vpcSecurityGroupIds = vpcSecurityGroupIds;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnDBProxyProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnDBProxyProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnDBProxyProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnDBProxyProps {
private final java.lang.Object auth;
private final java.lang.String dbProxyName;
private final java.lang.String engineFamily;
private final java.lang.String roleArn;
private final java.util.List vpcSubnetIds;
private final java.lang.Object debugLogging;
private final java.lang.Number idleClientTimeout;
private final java.lang.Object requireTls;
private final java.util.List tags;
private final java.util.List vpcSecurityGroupIds;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.auth = software.amazon.jsii.Kernel.get(this, "auth", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.dbProxyName = software.amazon.jsii.Kernel.get(this, "dbProxyName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.engineFamily = software.amazon.jsii.Kernel.get(this, "engineFamily", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.roleArn = software.amazon.jsii.Kernel.get(this, "roleArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpcSubnetIds = software.amazon.jsii.Kernel.get(this, "vpcSubnetIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.debugLogging = software.amazon.jsii.Kernel.get(this, "debugLogging", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.idleClientTimeout = software.amazon.jsii.Kernel.get(this, "idleClientTimeout", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.requireTls = software.amazon.jsii.Kernel.get(this, "requireTls", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.CfnDBProxy.TagFormatProperty.class)));
this.vpcSecurityGroupIds = software.amazon.jsii.Kernel.get(this, "vpcSecurityGroupIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.auth = java.util.Objects.requireNonNull(builder.auth, "auth is required");
this.dbProxyName = java.util.Objects.requireNonNull(builder.dbProxyName, "dbProxyName is required");
this.engineFamily = java.util.Objects.requireNonNull(builder.engineFamily, "engineFamily is required");
this.roleArn = java.util.Objects.requireNonNull(builder.roleArn, "roleArn is required");
this.vpcSubnetIds = java.util.Objects.requireNonNull(builder.vpcSubnetIds, "vpcSubnetIds is required");
this.debugLogging = builder.debugLogging;
this.idleClientTimeout = builder.idleClientTimeout;
this.requireTls = builder.requireTls;
this.tags = (java.util.List)builder.tags;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
}
@Override
public final java.lang.Object getAuth() {
return this.auth;
}
@Override
public final java.lang.String getDbProxyName() {
return this.dbProxyName;
}
@Override
public final java.lang.String getEngineFamily() {
return this.engineFamily;
}
@Override
public final java.lang.String getRoleArn() {
return this.roleArn;
}
@Override
public final java.util.List getVpcSubnetIds() {
return this.vpcSubnetIds;
}
@Override
public final java.lang.Object getDebugLogging() {
return this.debugLogging;
}
@Override
public final java.lang.Number getIdleClientTimeout() {
return this.idleClientTimeout;
}
@Override
public final java.lang.Object getRequireTls() {
return this.requireTls;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
public final java.util.List getVpcSecurityGroupIds() {
return this.vpcSecurityGroupIds;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("auth", om.valueToTree(this.getAuth()));
data.set("dbProxyName", om.valueToTree(this.getDbProxyName()));
data.set("engineFamily", om.valueToTree(this.getEngineFamily()));
data.set("roleArn", om.valueToTree(this.getRoleArn()));
data.set("vpcSubnetIds", om.valueToTree(this.getVpcSubnetIds()));
if (this.getDebugLogging() != null) {
data.set("debugLogging", om.valueToTree(this.getDebugLogging()));
}
if (this.getIdleClientTimeout() != null) {
data.set("idleClientTimeout", om.valueToTree(this.getIdleClientTimeout()));
}
if (this.getRequireTls() != null) {
data.set("requireTls", om.valueToTree(this.getRequireTls()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getVpcSecurityGroupIds() != null) {
data.set("vpcSecurityGroupIds", om.valueToTree(this.getVpcSecurityGroupIds()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.CfnDBProxyProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnDBProxyProps.Jsii$Proxy that = (CfnDBProxyProps.Jsii$Proxy) o;
if (!auth.equals(that.auth)) return false;
if (!dbProxyName.equals(that.dbProxyName)) return false;
if (!engineFamily.equals(that.engineFamily)) return false;
if (!roleArn.equals(that.roleArn)) return false;
if (!vpcSubnetIds.equals(that.vpcSubnetIds)) return false;
if (this.debugLogging != null ? !this.debugLogging.equals(that.debugLogging) : that.debugLogging != null) return false;
if (this.idleClientTimeout != null ? !this.idleClientTimeout.equals(that.idleClientTimeout) : that.idleClientTimeout != null) return false;
if (this.requireTls != null ? !this.requireTls.equals(that.requireTls) : that.requireTls != null) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
return this.vpcSecurityGroupIds != null ? this.vpcSecurityGroupIds.equals(that.vpcSecurityGroupIds) : that.vpcSecurityGroupIds == null;
}
@Override
public final int hashCode() {
int result = this.auth.hashCode();
result = 31 * result + (this.dbProxyName.hashCode());
result = 31 * result + (this.engineFamily.hashCode());
result = 31 * result + (this.roleArn.hashCode());
result = 31 * result + (this.vpcSubnetIds.hashCode());
result = 31 * result + (this.debugLogging != null ? this.debugLogging.hashCode() : 0);
result = 31 * result + (this.idleClientTimeout != null ? this.idleClientTimeout.hashCode() : 0);
result = 31 * result + (this.requireTls != null ? this.requireTls.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.vpcSecurityGroupIds != null ? this.vpcSecurityGroupIds.hashCode() : 0);
return result;
}
}
}