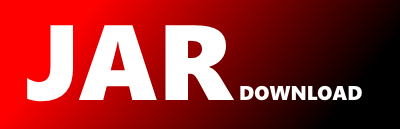
software.amazon.awscdk.services.rds.CfnEventSubscriptionProps Maven / Gradle / Ivy
Show all versions of rds Show documentation
package software.amazon.awscdk.services.rds;
/**
* Properties for defining a CfnEventSubscription
.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.rds.*;
* CfnEventSubscriptionProps cfnEventSubscriptionProps = CfnEventSubscriptionProps.builder()
* .snsTopicArn("snsTopicArn")
* // the properties below are optional
* .enabled(false)
* .eventCategories(List.of("eventCategories"))
* .sourceIds(List.of("sourceIds"))
* .sourceType("sourceType")
* .subscriptionName("subscriptionName")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:49.066Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.CfnEventSubscriptionProps")
@software.amazon.jsii.Jsii.Proxy(CfnEventSubscriptionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnEventSubscriptionProps extends software.amazon.jsii.JsiiSerializable {
/**
* The Amazon Resource Name (ARN) of the SNS topic created for event notification.
*
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getSnsTopicArn();
/**
* A value that indicates whether to activate the subscription.
*
* If the event notification subscription isn't activated, the subscription is created but not active.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEnabled() {
return null;
}
/**
* A list of event categories for a particular source type ( SourceType
) that you want to subscribe to.
*
* You can see a list of the categories for a given source type in the "Amazon RDS event categories and event messages" section of the Amazon RDS User Guide or the Amazon Aurora User Guide . You can also see this list by using the DescribeEventCategories
operation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getEventCategories() {
return null;
}
/**
* The list of identifiers of the event sources for which events are returned.
*
* If not specified, then all sources are included in the response. An identifier must begin with a letter and must contain only ASCII letters, digits, and hyphens. It can't end with a hyphen or contain two consecutive hyphens.
*
* Constraints:
*
*
* - If a
SourceIds
value is supplied, SourceType
must also be provided.
* - If the source type is a DB instance, a
DBInstanceIdentifier
value must be supplied.
* - If the source type is a DB cluster, a
DBClusterIdentifier
value must be supplied.
* - If the source type is a DB parameter group, a
DBParameterGroupName
value must be supplied.
* - If the source type is a DB security group, a
DBSecurityGroupName
value must be supplied.
* - If the source type is a DB snapshot, a
DBSnapshotIdentifier
value must be supplied.
* - If the source type is a DB cluster snapshot, a
DBClusterSnapshotIdentifier
value must be supplied.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getSourceIds() {
return null;
}
/**
* The type of source that is generating the events.
*
* For example, if you want to be notified of events generated by a DB instance, set this parameter to db-instance
. If this value isn't specified, all events are returned.
*
* Valid values: db-instance
| db-cluster
| db-parameter-group
| db-security-group
| db-snapshot
| db-cluster-snapshot
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSourceType() {
return null;
}
/**
* The name of the subscription.
*
* Constraints: The name must be less than 255 characters.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSubscriptionName() {
return null;
}
/**
* An optional array of key-value pairs to apply to this subscription.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnEventSubscriptionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnEventSubscriptionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String snsTopicArn;
java.lang.Object enabled;
java.util.List eventCategories;
java.util.List sourceIds;
java.lang.String sourceType;
java.lang.String subscriptionName;
java.util.List tags;
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSnsTopicArn}
* @param snsTopicArn The Amazon Resource Name (ARN) of the SNS topic created for event notification. This parameter is required.
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder snsTopicArn(java.lang.String snsTopicArn) {
this.snsTopicArn = snsTopicArn;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getEnabled}
* @param enabled A value that indicates whether to activate the subscription.
* If the event notification subscription isn't activated, the subscription is created but not active.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getEnabled}
* @param enabled A value that indicates whether to activate the subscription.
* If the event notification subscription isn't activated, the subscription is created but not active.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(software.amazon.awscdk.core.IResolvable enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getEventCategories}
* @param eventCategories A list of event categories for a particular source type ( SourceType
) that you want to subscribe to.
* You can see a list of the categories for a given source type in the "Amazon RDS event categories and event messages" section of the Amazon RDS User Guide or the Amazon Aurora User Guide . You can also see this list by using the DescribeEventCategories
operation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eventCategories(java.util.List eventCategories) {
this.eventCategories = eventCategories;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSourceIds}
* @param sourceIds The list of identifiers of the event sources for which events are returned.
* If not specified, then all sources are included in the response. An identifier must begin with a letter and must contain only ASCII letters, digits, and hyphens. It can't end with a hyphen or contain two consecutive hyphens.
*
* Constraints:
*
*
* - If a
SourceIds
value is supplied, SourceType
must also be provided.
* - If the source type is a DB instance, a
DBInstanceIdentifier
value must be supplied.
* - If the source type is a DB cluster, a
DBClusterIdentifier
value must be supplied.
* - If the source type is a DB parameter group, a
DBParameterGroupName
value must be supplied.
* - If the source type is a DB security group, a
DBSecurityGroupName
value must be supplied.
* - If the source type is a DB snapshot, a
DBSnapshotIdentifier
value must be supplied.
* - If the source type is a DB cluster snapshot, a
DBClusterSnapshotIdentifier
value must be supplied.
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceIds(java.util.List sourceIds) {
this.sourceIds = sourceIds;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSourceType}
* @param sourceType The type of source that is generating the events.
* For example, if you want to be notified of events generated by a DB instance, set this parameter to db-instance
. If this value isn't specified, all events are returned.
*
* Valid values: db-instance
| db-cluster
| db-parameter-group
| db-security-group
| db-snapshot
| db-cluster-snapshot
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceType(java.lang.String sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSubscriptionName}
* @param subscriptionName The name of the subscription.
* Constraints: The name must be less than 255 characters.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subscriptionName(java.lang.String subscriptionName) {
this.subscriptionName = subscriptionName;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getTags}
* @param tags An optional array of key-value pairs to apply to this subscription.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnEventSubscriptionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnEventSubscriptionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnEventSubscriptionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnEventSubscriptionProps {
private final java.lang.String snsTopicArn;
private final java.lang.Object enabled;
private final java.util.List eventCategories;
private final java.util.List sourceIds;
private final java.lang.String sourceType;
private final java.lang.String subscriptionName;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.snsTopicArn = software.amazon.jsii.Kernel.get(this, "snsTopicArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.enabled = software.amazon.jsii.Kernel.get(this, "enabled", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.eventCategories = software.amazon.jsii.Kernel.get(this, "eventCategories", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.sourceIds = software.amazon.jsii.Kernel.get(this, "sourceIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.sourceType = software.amazon.jsii.Kernel.get(this, "sourceType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.subscriptionName = software.amazon.jsii.Kernel.get(this, "subscriptionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.snsTopicArn = java.util.Objects.requireNonNull(builder.snsTopicArn, "snsTopicArn is required");
this.enabled = builder.enabled;
this.eventCategories = builder.eventCategories;
this.sourceIds = builder.sourceIds;
this.sourceType = builder.sourceType;
this.subscriptionName = builder.subscriptionName;
this.tags = (java.util.List)builder.tags;
}
@Override
public final java.lang.String getSnsTopicArn() {
return this.snsTopicArn;
}
@Override
public final java.lang.Object getEnabled() {
return this.enabled;
}
@Override
public final java.util.List getEventCategories() {
return this.eventCategories;
}
@Override
public final java.util.List getSourceIds() {
return this.sourceIds;
}
@Override
public final java.lang.String getSourceType() {
return this.sourceType;
}
@Override
public final java.lang.String getSubscriptionName() {
return this.subscriptionName;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("snsTopicArn", om.valueToTree(this.getSnsTopicArn()));
if (this.getEnabled() != null) {
data.set("enabled", om.valueToTree(this.getEnabled()));
}
if (this.getEventCategories() != null) {
data.set("eventCategories", om.valueToTree(this.getEventCategories()));
}
if (this.getSourceIds() != null) {
data.set("sourceIds", om.valueToTree(this.getSourceIds()));
}
if (this.getSourceType() != null) {
data.set("sourceType", om.valueToTree(this.getSourceType()));
}
if (this.getSubscriptionName() != null) {
data.set("subscriptionName", om.valueToTree(this.getSubscriptionName()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.CfnEventSubscriptionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnEventSubscriptionProps.Jsii$Proxy that = (CfnEventSubscriptionProps.Jsii$Proxy) o;
if (!snsTopicArn.equals(that.snsTopicArn)) return false;
if (this.enabled != null ? !this.enabled.equals(that.enabled) : that.enabled != null) return false;
if (this.eventCategories != null ? !this.eventCategories.equals(that.eventCategories) : that.eventCategories != null) return false;
if (this.sourceIds != null ? !this.sourceIds.equals(that.sourceIds) : that.sourceIds != null) return false;
if (this.sourceType != null ? !this.sourceType.equals(that.sourceType) : that.sourceType != null) return false;
if (this.subscriptionName != null ? !this.subscriptionName.equals(that.subscriptionName) : that.subscriptionName != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public final int hashCode() {
int result = this.snsTopicArn.hashCode();
result = 31 * result + (this.enabled != null ? this.enabled.hashCode() : 0);
result = 31 * result + (this.eventCategories != null ? this.eventCategories.hashCode() : 0);
result = 31 * result + (this.sourceIds != null ? this.sourceIds.hashCode() : 0);
result = 31 * result + (this.sourceType != null ? this.sourceType.hashCode() : 0);
result = 31 * result + (this.subscriptionName != null ? this.subscriptionName.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}