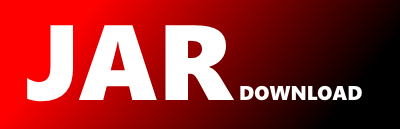
software.amazon.awscdk.services.rds.ParameterGroupProps Maven / Gradle / Ivy
Show all versions of rds Show documentation
package software.amazon.awscdk.services.rds;
/**
* Properties for a parameter group.
*
* Example:
*
*
* // Set open cursors with parameter group
* ParameterGroup parameterGroup = ParameterGroup.Builder.create(this, "ParameterGroup")
* .engine(DatabaseInstanceEngine.oracleSe2(OracleSe2InstanceEngineProps.builder().version(OracleEngineVersion.VER_19_0_0_0_2020_04_R1).build()))
* .parameters(Map.of(
* "open_cursors", "2500"))
* .build();
* OptionGroup optionGroup = OptionGroup.Builder.create(this, "OptionGroup")
* .engine(DatabaseInstanceEngine.oracleSe2(OracleSe2InstanceEngineProps.builder().version(OracleEngineVersion.VER_19_0_0_0_2020_04_R1).build()))
* .configurations(List.of(OptionConfiguration.builder()
* .name("LOCATOR")
* .build(), OptionConfiguration.builder()
* .name("OEM")
* .port(1158)
* .vpc(vpc)
* .build()))
* .build();
* // Allow connections to OEM
* optionGroup.optionConnections.OEM.connections.allowDefaultPortFromAnyIpv4();
* // Database instance with production values
* DatabaseInstance instance = DatabaseInstance.Builder.create(this, "Instance")
* .engine(DatabaseInstanceEngine.oracleSe2(OracleSe2InstanceEngineProps.builder().version(OracleEngineVersion.VER_19_0_0_0_2020_04_R1).build()))
* .licenseModel(LicenseModel.BRING_YOUR_OWN_LICENSE)
* .instanceType(InstanceType.of(InstanceClass.BURSTABLE3, InstanceSize.MEDIUM))
* .multiAz(true)
* .storageType(StorageType.IO1)
* .credentials(Credentials.fromUsername("syscdk"))
* .vpc(vpc)
* .databaseName("ORCL")
* .storageEncrypted(true)
* .backupRetention(Duration.days(7))
* .monitoringInterval(Duration.seconds(60))
* .enablePerformanceInsights(true)
* .cloudwatchLogsExports(List.of("trace", "audit", "alert", "listener"))
* .cloudwatchLogsRetention(RetentionDays.ONE_MONTH)
* .autoMinorVersionUpgrade(true) // required to be true if LOCATOR is used in the option group
* .optionGroup(optionGroup)
* .parameterGroup(parameterGroup)
* .removalPolicy(RemovalPolicy.DESTROY)
* .build();
* // Allow connections on default port from any IPV4
* instance.connections.allowDefaultPortFromAnyIpv4();
* // Rotate the master user password every 30 days
* instance.addRotationSingleUser();
* // Add alarm for high CPU
* // Add alarm for high CPU
* Alarm.Builder.create(this, "HighCPU")
* .metric(instance.metricCPUUtilization())
* .threshold(90)
* .evaluationPeriods(1)
* .build();
* // Trigger Lambda function on instance availability events
* Function fn = Function.Builder.create(this, "Function")
* .code(Code.fromInline("exports.handler = (event) => console.log(event);"))
* .handler("index.handler")
* .runtime(Runtime.NODEJS_14_X)
* .build();
* Rule availabilityRule = instance.onEvent("Availability", OnEventOptions.builder().target(new LambdaFunction(fn)).build());
* availabilityRule.addEventPattern(EventPattern.builder()
* .detail(Map.of(
* "EventCategories", List.of("availability")))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:49.233Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.ParameterGroupProps")
@software.amazon.jsii.Jsii.Proxy(ParameterGroupProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface ParameterGroupProps extends software.amazon.jsii.JsiiSerializable {
/**
* The database engine for this parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.IEngine getEngine();
/**
* Description for this parameter group.
*
* Default: a CDK generated description
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* The parameters in this parameter group.
*
* Default: - None
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getParameters() {
return null;
}
/**
* @return a {@link Builder} of {@link ParameterGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ParameterGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.rds.IEngine engine;
java.lang.String description;
java.util.Map parameters;
/**
* Sets the value of {@link ParameterGroupProps#getEngine}
* @param engine The database engine for this parameter group. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder engine(software.amazon.awscdk.services.rds.IEngine engine) {
this.engine = engine;
return this;
}
/**
* Sets the value of {@link ParameterGroupProps#getDescription}
* @param description Description for this parameter group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link ParameterGroupProps#getParameters}
* @param parameters The parameters in this parameter group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(java.util.Map parameters) {
this.parameters = parameters;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ParameterGroupProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ParameterGroupProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ParameterGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ParameterGroupProps {
private final software.amazon.awscdk.services.rds.IEngine engine;
private final java.lang.String description;
private final java.util.Map parameters;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.engine = software.amazon.jsii.Kernel.get(this, "engine", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IEngine.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.parameters = software.amazon.jsii.Kernel.get(this, "parameters", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.engine = java.util.Objects.requireNonNull(builder.engine, "engine is required");
this.description = builder.description;
this.parameters = builder.parameters;
}
@Override
public final software.amazon.awscdk.services.rds.IEngine getEngine() {
return this.engine;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.util.Map getParameters() {
return this.parameters;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("engine", om.valueToTree(this.getEngine()));
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getParameters() != null) {
data.set("parameters", om.valueToTree(this.getParameters()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.ParameterGroupProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ParameterGroupProps.Jsii$Proxy that = (ParameterGroupProps.Jsii$Proxy) o;
if (!engine.equals(that.engine)) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
return this.parameters != null ? this.parameters.equals(that.parameters) : that.parameters == null;
}
@Override
public final int hashCode() {
int result = this.engine.hashCode();
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.parameters != null ? this.parameters.hashCode() : 0);
return result;
}
}
}