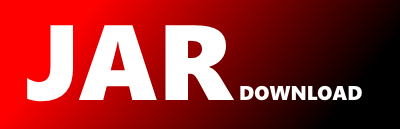
software.amazon.awscdk.services.rds.RotationMultiUserOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rds Show documentation
Show all versions of rds Show documentation
CDK Constructs for AWS RDS
The newest version!
package software.amazon.awscdk.services.rds;
/**
* Options to add the multi user rotation.
*
* Example:
*
*
* DatabaseInstance instance;
* DatabaseSecret myImportedSecret;
* instance.addRotationMultiUser("MyUser", RotationMultiUserOptions.builder()
* .secret(myImportedSecret)
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:49.256Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.RotationMultiUserOptions")
@software.amazon.jsii.Jsii.Proxy(RotationMultiUserOptions.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface RotationMultiUserOptions extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.rds.CommonRotationUserOptions {
/**
* The secret to rotate.
*
* It must be a JSON string with the following format:
*
*
* {
* "engine": <required: database engine>,
* "host": <required: instance host name>,
* "username": <required: username>,
* "password": <required: password>,
* "dbname": <optional: database name>,
* "port": <optional: if not specified, default port will be used>,
* "masterarn": <required: the arn of the master secret which will be used to create users/change passwords>
* }
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.ISecret getSecret();
/**
* @return a {@link Builder} of {@link RotationMultiUserOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link RotationMultiUserOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.secretsmanager.ISecret secret;
software.amazon.awscdk.core.Duration automaticallyAfter;
software.amazon.awscdk.services.ec2.IInterfaceVpcEndpoint endpoint;
java.lang.String excludeCharacters;
software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Sets the value of {@link RotationMultiUserOptions#getSecret}
* @param secret The secret to rotate. This parameter is required.
* It must be a JSON string with the following format:
*
*
* {
* "engine": <required: database engine>,
* "host": <required: instance host name>,
* "username": <required: username>,
* "password": <required: password>,
* "dbname": <optional: database name>,
* "port": <optional: if not specified, default port will be used>,
* "masterarn": <required: the arn of the master secret which will be used to create users/change passwords>
* }
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secret(software.amazon.awscdk.services.secretsmanager.ISecret secret) {
this.secret = secret;
return this;
}
/**
* Sets the value of {@link RotationMultiUserOptions#getAutomaticallyAfter}
* @param automaticallyAfter Specifies the number of days after the previous rotation before Secrets Manager triggers the next automatic rotation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder automaticallyAfter(software.amazon.awscdk.core.Duration automaticallyAfter) {
this.automaticallyAfter = automaticallyAfter;
return this;
}
/**
* Sets the value of {@link RotationMultiUserOptions#getEndpoint}
* @param endpoint The VPC interface endpoint to use for the Secrets Manager API.
* If you enable private DNS hostnames for your VPC private endpoint (the default), you don't
* need to specify an endpoint. The standard Secrets Manager DNS hostname the Secrets Manager
* CLI and SDKs use by default (https://secretsmanager..amazonaws.com) automatically
* resolves to your VPC endpoint.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder endpoint(software.amazon.awscdk.services.ec2.IInterfaceVpcEndpoint endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* Sets the value of {@link RotationMultiUserOptions#getExcludeCharacters}
* @param excludeCharacters Specifies characters to not include in generated passwords.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder excludeCharacters(java.lang.String excludeCharacters) {
this.excludeCharacters = excludeCharacters;
return this;
}
/**
* Sets the value of {@link RotationMultiUserOptions#getVpcSubnets}
* @param vpcSubnets Where to place the rotation Lambda function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link RotationMultiUserOptions}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public RotationMultiUserOptions build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link RotationMultiUserOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements RotationMultiUserOptions {
private final software.amazon.awscdk.services.secretsmanager.ISecret secret;
private final software.amazon.awscdk.core.Duration automaticallyAfter;
private final software.amazon.awscdk.services.ec2.IInterfaceVpcEndpoint endpoint;
private final java.lang.String excludeCharacters;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.secret = software.amazon.jsii.Kernel.get(this, "secret", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.ISecret.class));
this.automaticallyAfter = software.amazon.jsii.Kernel.get(this, "automaticallyAfter", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.endpoint = software.amazon.jsii.Kernel.get(this, "endpoint", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IInterfaceVpcEndpoint.class));
this.excludeCharacters = software.amazon.jsii.Kernel.get(this, "excludeCharacters", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpcSubnets = software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.secret = java.util.Objects.requireNonNull(builder.secret, "secret is required");
this.automaticallyAfter = builder.automaticallyAfter;
this.endpoint = builder.endpoint;
this.excludeCharacters = builder.excludeCharacters;
this.vpcSubnets = builder.vpcSubnets;
}
@Override
public final software.amazon.awscdk.services.secretsmanager.ISecret getSecret() {
return this.secret;
}
@Override
public final software.amazon.awscdk.core.Duration getAutomaticallyAfter() {
return this.automaticallyAfter;
}
@Override
public final software.amazon.awscdk.services.ec2.IInterfaceVpcEndpoint getEndpoint() {
return this.endpoint;
}
@Override
public final java.lang.String getExcludeCharacters() {
return this.excludeCharacters;
}
@Override
public final software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("secret", om.valueToTree(this.getSecret()));
if (this.getAutomaticallyAfter() != null) {
data.set("automaticallyAfter", om.valueToTree(this.getAutomaticallyAfter()));
}
if (this.getEndpoint() != null) {
data.set("endpoint", om.valueToTree(this.getEndpoint()));
}
if (this.getExcludeCharacters() != null) {
data.set("excludeCharacters", om.valueToTree(this.getExcludeCharacters()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.RotationMultiUserOptions"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RotationMultiUserOptions.Jsii$Proxy that = (RotationMultiUserOptions.Jsii$Proxy) o;
if (!secret.equals(that.secret)) return false;
if (this.automaticallyAfter != null ? !this.automaticallyAfter.equals(that.automaticallyAfter) : that.automaticallyAfter != null) return false;
if (this.endpoint != null ? !this.endpoint.equals(that.endpoint) : that.endpoint != null) return false;
if (this.excludeCharacters != null ? !this.excludeCharacters.equals(that.excludeCharacters) : that.excludeCharacters != null) return false;
return this.vpcSubnets != null ? this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets == null;
}
@Override
public final int hashCode() {
int result = this.secret.hashCode();
result = 31 * result + (this.automaticallyAfter != null ? this.automaticallyAfter.hashCode() : 0);
result = 31 * result + (this.endpoint != null ? this.endpoint.hashCode() : 0);
result = 31 * result + (this.excludeCharacters != null ? this.excludeCharacters.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy