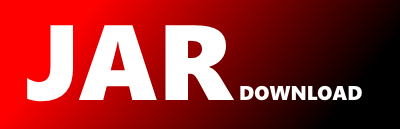
software.amazon.awscdk.services.rds.ServerlessClusterProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.rds;
/**
* Properties for a new Aurora Serverless Cluster.
*
* Example:
*
*
* Vpc vpc;
* Code code;
* ServerlessCluster cluster = ServerlessCluster.Builder.create(this, "AnotherCluster")
* .engine(DatabaseClusterEngine.AURORA_MYSQL)
* .vpc(vpc) // this parameter is optional for serverless Clusters
* .enableDataApi(true)
* .build();
* Function fn = Function.Builder.create(this, "MyFunction")
* .runtime(Runtime.NODEJS_14_X)
* .handler("index.handler")
* .code(code)
* .environment(Map.of(
* "CLUSTER_ARN", cluster.getClusterArn(),
* "SECRET_ARN", cluster.getSecret().getSecretArn()))
* .build();
* cluster.grantDataApiAccess(fn);
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:49.266Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.rds.$Module.class, fqn = "@aws-cdk/aws-rds.ServerlessClusterProps")
@software.amazon.jsii.Jsii.Proxy(ServerlessClusterProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface ServerlessClusterProps extends software.amazon.jsii.JsiiSerializable {
/**
* What kind of database to start.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.rds.IClusterEngine getEngine();
/**
* The number of days during which automatic DB snapshots are retained.
*
* Automatic backup retention cannot be disabled on serverless clusters.
* Must be a value from 1 day to 35 days.
*
* Default: Duration.days(1)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getBackupRetention() {
return null;
}
/**
* An optional identifier for the cluster.
*
* Default: - A name is automatically generated.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getClusterIdentifier() {
return null;
}
/**
* Credentials for the administrative user.
*
* Default: - A username of 'admin' and SecretsManager-generated password
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.Credentials getCredentials() {
return null;
}
/**
* Name of a database which is automatically created inside the cluster.
*
* Default: - Database is not created in cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDefaultDatabaseName() {
return null;
}
/**
* Indicates whether the DB cluster should have deletion protection enabled.
*
* Default: - true if removalPolicy is RETAIN, false otherwise
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getDeletionProtection() {
return null;
}
/**
* Whether to enable the Data API.
*
* Default: false
*
* @see https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/data-api.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getEnableDataApi() {
return null;
}
/**
* Additional parameters to pass to the database engine.
*
* Default: - no parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.IParameterGroup getParameterGroup() {
return null;
}
/**
* The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update.
*
* Default: - RemovalPolicy.SNAPSHOT (remove the cluster and instances, but retain a snapshot of the data)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.RemovalPolicy getRemovalPolicy() {
return null;
}
/**
* Scaling configuration of an Aurora Serverless database cluster.
*
* Default: - Serverless cluster is automatically paused after 5 minutes of being idle.
* minimum capacity: 2 ACU
* maximum capacity: 16 ACU
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.ServerlessScalingOptions getScaling() {
return null;
}
/**
* Security group.
*
* Default: - a new security group is created if `vpc` was provided.
* If the `vpc` property was not provided, no VPC security groups will be associated with the DB cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getSecurityGroups() {
return null;
}
/**
* The KMS key for storage encryption.
*
* Default: - the default master key will be used for storage encryption
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.kms.IKey getStorageEncryptionKey() {
return null;
}
/**
* Existing subnet group for the cluster.
*
* Default: - a new subnet group is created if `vpc` was provided.
* If the `vpc` property was not provided, no subnet group will be associated with the DB cluster
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.rds.ISubnetGroup getSubnetGroup() {
return null;
}
/**
* The VPC that this Aurora Serverless cluster has been created in.
*
* Default: - the default VPC in the account and region will be used
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.IVpc getVpc() {
return null;
}
/**
* Where to place the instances within the VPC.
*
* If provided, the vpc
property must also be specified.
*
* Default: - the VPC default strategy if not specified.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return null;
}
/**
* @return a {@link Builder} of {@link ServerlessClusterProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ServerlessClusterProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.rds.IClusterEngine engine;
software.amazon.awscdk.core.Duration backupRetention;
java.lang.String clusterIdentifier;
software.amazon.awscdk.services.rds.Credentials credentials;
java.lang.String defaultDatabaseName;
java.lang.Boolean deletionProtection;
java.lang.Boolean enableDataApi;
software.amazon.awscdk.services.rds.IParameterGroup parameterGroup;
software.amazon.awscdk.core.RemovalPolicy removalPolicy;
software.amazon.awscdk.services.rds.ServerlessScalingOptions scaling;
java.util.List securityGroups;
software.amazon.awscdk.services.kms.IKey storageEncryptionKey;
software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup;
software.amazon.awscdk.services.ec2.IVpc vpc;
software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Sets the value of {@link ServerlessClusterProps#getEngine}
* @param engine What kind of database to start. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder engine(software.amazon.awscdk.services.rds.IClusterEngine engine) {
this.engine = engine;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getBackupRetention}
* @param backupRetention The number of days during which automatic DB snapshots are retained.
* Automatic backup retention cannot be disabled on serverless clusters.
* Must be a value from 1 day to 35 days.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder backupRetention(software.amazon.awscdk.core.Duration backupRetention) {
this.backupRetention = backupRetention;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getClusterIdentifier}
* @param clusterIdentifier An optional identifier for the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder clusterIdentifier(java.lang.String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getCredentials}
* @param credentials Credentials for the administrative user.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder credentials(software.amazon.awscdk.services.rds.Credentials credentials) {
this.credentials = credentials;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getDefaultDatabaseName}
* @param defaultDatabaseName Name of a database which is automatically created inside the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultDatabaseName(java.lang.String defaultDatabaseName) {
this.defaultDatabaseName = defaultDatabaseName;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getDeletionProtection}
* @param deletionProtection Indicates whether the DB cluster should have deletion protection enabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deletionProtection(java.lang.Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getEnableDataApi}
* @param enableDataApi Whether to enable the Data API.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableDataApi(java.lang.Boolean enableDataApi) {
this.enableDataApi = enableDataApi;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getParameterGroup}
* @param parameterGroup Additional parameters to pass to the database engine.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterGroup(software.amazon.awscdk.services.rds.IParameterGroup parameterGroup) {
this.parameterGroup = parameterGroup;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getRemovalPolicy}
* @param removalPolicy The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder removalPolicy(software.amazon.awscdk.core.RemovalPolicy removalPolicy) {
this.removalPolicy = removalPolicy;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getScaling}
* @param scaling Scaling configuration of an Aurora Serverless database cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scaling(software.amazon.awscdk.services.rds.ServerlessScalingOptions scaling) {
this.scaling = scaling;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getSecurityGroups}
* @param securityGroups Security group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder securityGroups(java.util.List extends software.amazon.awscdk.services.ec2.ISecurityGroup> securityGroups) {
this.securityGroups = (java.util.List)securityGroups;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getStorageEncryptionKey}
* @param storageEncryptionKey The KMS key for storage encryption.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder storageEncryptionKey(software.amazon.awscdk.services.kms.IKey storageEncryptionKey) {
this.storageEncryptionKey = storageEncryptionKey;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getSubnetGroup}
* @param subnetGroup Existing subnet group for the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subnetGroup(software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup) {
this.subnetGroup = subnetGroup;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getVpc}
* @param vpc The VPC that this Aurora Serverless cluster has been created in.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpc(software.amazon.awscdk.services.ec2.IVpc vpc) {
this.vpc = vpc;
return this;
}
/**
* Sets the value of {@link ServerlessClusterProps#getVpcSubnets}
* @param vpcSubnets Where to place the instances within the VPC.
* If provided, the vpc
property must also be specified.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ServerlessClusterProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ServerlessClusterProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ServerlessClusterProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ServerlessClusterProps {
private final software.amazon.awscdk.services.rds.IClusterEngine engine;
private final software.amazon.awscdk.core.Duration backupRetention;
private final java.lang.String clusterIdentifier;
private final software.amazon.awscdk.services.rds.Credentials credentials;
private final java.lang.String defaultDatabaseName;
private final java.lang.Boolean deletionProtection;
private final java.lang.Boolean enableDataApi;
private final software.amazon.awscdk.services.rds.IParameterGroup parameterGroup;
private final software.amazon.awscdk.core.RemovalPolicy removalPolicy;
private final software.amazon.awscdk.services.rds.ServerlessScalingOptions scaling;
private final java.util.List securityGroups;
private final software.amazon.awscdk.services.kms.IKey storageEncryptionKey;
private final software.amazon.awscdk.services.rds.ISubnetGroup subnetGroup;
private final software.amazon.awscdk.services.ec2.IVpc vpc;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.engine = software.amazon.jsii.Kernel.get(this, "engine", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IClusterEngine.class));
this.backupRetention = software.amazon.jsii.Kernel.get(this, "backupRetention", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.clusterIdentifier = software.amazon.jsii.Kernel.get(this, "clusterIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.credentials = software.amazon.jsii.Kernel.get(this, "credentials", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.Credentials.class));
this.defaultDatabaseName = software.amazon.jsii.Kernel.get(this, "defaultDatabaseName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.deletionProtection = software.amazon.jsii.Kernel.get(this, "deletionProtection", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.enableDataApi = software.amazon.jsii.Kernel.get(this, "enableDataApi", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.parameterGroup = software.amazon.jsii.Kernel.get(this, "parameterGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.IParameterGroup.class));
this.removalPolicy = software.amazon.jsii.Kernel.get(this, "removalPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.RemovalPolicy.class));
this.scaling = software.amazon.jsii.Kernel.get(this, "scaling", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.ServerlessScalingOptions.class));
this.securityGroups = software.amazon.jsii.Kernel.get(this, "securityGroups", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.ISecurityGroup.class)));
this.storageEncryptionKey = software.amazon.jsii.Kernel.get(this, "storageEncryptionKey", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.kms.IKey.class));
this.subnetGroup = software.amazon.jsii.Kernel.get(this, "subnetGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.rds.ISubnetGroup.class));
this.vpc = software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IVpc.class));
this.vpcSubnets = software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.engine = java.util.Objects.requireNonNull(builder.engine, "engine is required");
this.backupRetention = builder.backupRetention;
this.clusterIdentifier = builder.clusterIdentifier;
this.credentials = builder.credentials;
this.defaultDatabaseName = builder.defaultDatabaseName;
this.deletionProtection = builder.deletionProtection;
this.enableDataApi = builder.enableDataApi;
this.parameterGroup = builder.parameterGroup;
this.removalPolicy = builder.removalPolicy;
this.scaling = builder.scaling;
this.securityGroups = (java.util.List)builder.securityGroups;
this.storageEncryptionKey = builder.storageEncryptionKey;
this.subnetGroup = builder.subnetGroup;
this.vpc = builder.vpc;
this.vpcSubnets = builder.vpcSubnets;
}
@Override
public final software.amazon.awscdk.services.rds.IClusterEngine getEngine() {
return this.engine;
}
@Override
public final software.amazon.awscdk.core.Duration getBackupRetention() {
return this.backupRetention;
}
@Override
public final java.lang.String getClusterIdentifier() {
return this.clusterIdentifier;
}
@Override
public final software.amazon.awscdk.services.rds.Credentials getCredentials() {
return this.credentials;
}
@Override
public final java.lang.String getDefaultDatabaseName() {
return this.defaultDatabaseName;
}
@Override
public final java.lang.Boolean getDeletionProtection() {
return this.deletionProtection;
}
@Override
public final java.lang.Boolean getEnableDataApi() {
return this.enableDataApi;
}
@Override
public final software.amazon.awscdk.services.rds.IParameterGroup getParameterGroup() {
return this.parameterGroup;
}
@Override
public final software.amazon.awscdk.core.RemovalPolicy getRemovalPolicy() {
return this.removalPolicy;
}
@Override
public final software.amazon.awscdk.services.rds.ServerlessScalingOptions getScaling() {
return this.scaling;
}
@Override
public final java.util.List getSecurityGroups() {
return this.securityGroups;
}
@Override
public final software.amazon.awscdk.services.kms.IKey getStorageEncryptionKey() {
return this.storageEncryptionKey;
}
@Override
public final software.amazon.awscdk.services.rds.ISubnetGroup getSubnetGroup() {
return this.subnetGroup;
}
@Override
public final software.amazon.awscdk.services.ec2.IVpc getVpc() {
return this.vpc;
}
@Override
public final software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("engine", om.valueToTree(this.getEngine()));
if (this.getBackupRetention() != null) {
data.set("backupRetention", om.valueToTree(this.getBackupRetention()));
}
if (this.getClusterIdentifier() != null) {
data.set("clusterIdentifier", om.valueToTree(this.getClusterIdentifier()));
}
if (this.getCredentials() != null) {
data.set("credentials", om.valueToTree(this.getCredentials()));
}
if (this.getDefaultDatabaseName() != null) {
data.set("defaultDatabaseName", om.valueToTree(this.getDefaultDatabaseName()));
}
if (this.getDeletionProtection() != null) {
data.set("deletionProtection", om.valueToTree(this.getDeletionProtection()));
}
if (this.getEnableDataApi() != null) {
data.set("enableDataApi", om.valueToTree(this.getEnableDataApi()));
}
if (this.getParameterGroup() != null) {
data.set("parameterGroup", om.valueToTree(this.getParameterGroup()));
}
if (this.getRemovalPolicy() != null) {
data.set("removalPolicy", om.valueToTree(this.getRemovalPolicy()));
}
if (this.getScaling() != null) {
data.set("scaling", om.valueToTree(this.getScaling()));
}
if (this.getSecurityGroups() != null) {
data.set("securityGroups", om.valueToTree(this.getSecurityGroups()));
}
if (this.getStorageEncryptionKey() != null) {
data.set("storageEncryptionKey", om.valueToTree(this.getStorageEncryptionKey()));
}
if (this.getSubnetGroup() != null) {
data.set("subnetGroup", om.valueToTree(this.getSubnetGroup()));
}
if (this.getVpc() != null) {
data.set("vpc", om.valueToTree(this.getVpc()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-rds.ServerlessClusterProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ServerlessClusterProps.Jsii$Proxy that = (ServerlessClusterProps.Jsii$Proxy) o;
if (!engine.equals(that.engine)) return false;
if (this.backupRetention != null ? !this.backupRetention.equals(that.backupRetention) : that.backupRetention != null) return false;
if (this.clusterIdentifier != null ? !this.clusterIdentifier.equals(that.clusterIdentifier) : that.clusterIdentifier != null) return false;
if (this.credentials != null ? !this.credentials.equals(that.credentials) : that.credentials != null) return false;
if (this.defaultDatabaseName != null ? !this.defaultDatabaseName.equals(that.defaultDatabaseName) : that.defaultDatabaseName != null) return false;
if (this.deletionProtection != null ? !this.deletionProtection.equals(that.deletionProtection) : that.deletionProtection != null) return false;
if (this.enableDataApi != null ? !this.enableDataApi.equals(that.enableDataApi) : that.enableDataApi != null) return false;
if (this.parameterGroup != null ? !this.parameterGroup.equals(that.parameterGroup) : that.parameterGroup != null) return false;
if (this.removalPolicy != null ? !this.removalPolicy.equals(that.removalPolicy) : that.removalPolicy != null) return false;
if (this.scaling != null ? !this.scaling.equals(that.scaling) : that.scaling != null) return false;
if (this.securityGroups != null ? !this.securityGroups.equals(that.securityGroups) : that.securityGroups != null) return false;
if (this.storageEncryptionKey != null ? !this.storageEncryptionKey.equals(that.storageEncryptionKey) : that.storageEncryptionKey != null) return false;
if (this.subnetGroup != null ? !this.subnetGroup.equals(that.subnetGroup) : that.subnetGroup != null) return false;
if (this.vpc != null ? !this.vpc.equals(that.vpc) : that.vpc != null) return false;
return this.vpcSubnets != null ? this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets == null;
}
@Override
public final int hashCode() {
int result = this.engine.hashCode();
result = 31 * result + (this.backupRetention != null ? this.backupRetention.hashCode() : 0);
result = 31 * result + (this.clusterIdentifier != null ? this.clusterIdentifier.hashCode() : 0);
result = 31 * result + (this.credentials != null ? this.credentials.hashCode() : 0);
result = 31 * result + (this.defaultDatabaseName != null ? this.defaultDatabaseName.hashCode() : 0);
result = 31 * result + (this.deletionProtection != null ? this.deletionProtection.hashCode() : 0);
result = 31 * result + (this.enableDataApi != null ? this.enableDataApi.hashCode() : 0);
result = 31 * result + (this.parameterGroup != null ? this.parameterGroup.hashCode() : 0);
result = 31 * result + (this.removalPolicy != null ? this.removalPolicy.hashCode() : 0);
result = 31 * result + (this.scaling != null ? this.scaling.hashCode() : 0);
result = 31 * result + (this.securityGroups != null ? this.securityGroups.hashCode() : 0);
result = 31 * result + (this.storageEncryptionKey != null ? this.storageEncryptionKey.hashCode() : 0);
result = 31 * result + (this.subnetGroup != null ? this.subnetGroup.hashCode() : 0);
result = 31 * result + (this.vpc != null ? this.vpc.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
return result;
}
}
}