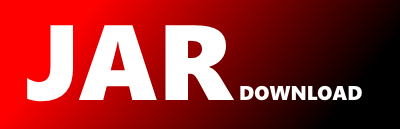
software.amazon.awscdk.services.redshift.alpha.Cluster Maven / Gradle / Ivy
package software.amazon.awscdk.services.redshift.alpha;
/**
* (experimental) Create a Redshift cluster a given number of nodes.
*
* Example:
*
*
* import software.amazon.awscdk.services.ec2.*;
* import software.amazon.awscdk.*;
* Vpc vpc;
* Cluster cluster = Cluster.Builder.create(this, "Cluster")
* .masterUser(Login.builder()
* .masterUsername("admin")
* .masterPassword(SecretValue.unsafePlainText("tooshort"))
* .build())
* .vpc(vpc)
* .build();
* cluster.addToParameterGroup("enable_user_activity_logging", "true");
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-11-15T10:25:09.574Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.alpha.$Module.class, fqn = "@aws-cdk/aws-redshift-alpha.Cluster")
public class Cluster extends software.amazon.awscdk.Resource implements software.amazon.awscdk.services.redshift.alpha.ICluster {
protected Cluster(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Cluster(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Cluster(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.alpha.ClusterProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Import an existing DatabaseCluster from properties.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param attrs This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.alpha.ICluster fromClusterAttributes(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.alpha.ClusterAttributes attrs) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.redshift.alpha.Cluster.class, "fromClusterAttributes", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.redshift.alpha.ICluster.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(attrs, "attrs is required") });
}
/**
* (experimental) Adds default IAM role to cluster.
*
* The default IAM role must be already associated to the cluster to be added as the default role.
*
* @param defaultIamRole the IAM role to be set as the default role. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addDefaultIamRole(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IRole defaultIamRole) {
software.amazon.jsii.Kernel.call(this, "addDefaultIamRole", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(defaultIamRole, "defaultIamRole is required") });
}
/**
* (experimental) Adds a role to the cluster.
*
* @param role the role to add. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addIamRole(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IRole role) {
software.amazon.jsii.Kernel.call(this, "addIamRole", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(role, "role is required") });
}
/**
* (experimental) Adds the multi user rotation to this cluster.
*
* @param id This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationMultiUser(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.alpha.RotationMultiUserOptions options) {
return software.amazon.jsii.Kernel.call(this, "addRotationMultiUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class), new Object[] { java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* (experimental) Adds the single user rotation of the master password to this cluster.
*
* @param automaticallyAfter Specifies the number of days after the previous rotation before Secrets Manager triggers the next automatic rotation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationSingleUser(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.Duration automaticallyAfter) {
return software.amazon.jsii.Kernel.call(this, "addRotationSingleUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class), new Object[] { automaticallyAfter });
}
/**
* (experimental) Adds the single user rotation of the master password to this cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationSingleUser() {
return software.amazon.jsii.Kernel.call(this, "addRotationSingleUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class));
}
/**
* (experimental) Adds a parameter to the Clusters' parameter group.
*
* @param name the parameter name. This parameter is required.
* @param value the parameter name. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addToParameterGroup(final @org.jetbrains.annotations.NotNull java.lang.String name, final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.call(this, "addToParameterGroup", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(name, "name is required"), java.util.Objects.requireNonNull(value, "value is required") });
}
/**
* (experimental) Renders the secret attachment target specifications.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretAttachmentTargetProps asSecretAttachmentTarget() {
return software.amazon.jsii.Kernel.call(this, "asSecretAttachmentTarget", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretAttachmentTargetProps.class));
}
/**
* (experimental) Enables automatic cluster rebooting when changes to the cluster's parameter group require a restart to apply.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void enableRebootForParameterChanges() {
software.amazon.jsii.Kernel.call(this, "enableRebootForParameterChanges", software.amazon.jsii.NativeType.VOID);
}
/**
* (experimental) The endpoint to use for read/write operations.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.alpha.Endpoint getClusterEndpoint() {
return software.amazon.jsii.Kernel.get(this, "clusterEndpoint", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.redshift.alpha.Endpoint.class));
}
/**
* (experimental) Identifier of the cluster.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getClusterName() {
return software.amazon.jsii.Kernel.get(this, "clusterName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) Access to the network connections.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.Connections getConnections() {
return software.amazon.jsii.Kernel.get(this, "connections", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.Connections.class));
}
/**
* (experimental) The secret attached to this cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.secretsmanager.ISecret getSecret() {
return software.amazon.jsii.Kernel.get(this, "secret", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.ISecret.class));
}
/**
* (experimental) The cluster's parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.redshift.alpha.IClusterParameterGroup getParameterGroup() {
return software.amazon.jsii.Kernel.get(this, "parameterGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.redshift.alpha.IClusterParameterGroup.class));
}
/**
* (experimental) The cluster's parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected void setParameterGroup(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.redshift.alpha.IClusterParameterGroup value) {
software.amazon.jsii.Kernel.set(this, "parameterGroup", value);
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.redshift.alpha.Cluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.redshift.alpha.ClusterProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.redshift.alpha.ClusterProps.Builder();
}
/**
* (experimental) Username and password for the administrative user.
*
* @return {@code this}
* @param masterUser Username and password for the administrative user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder masterUser(final software.amazon.awscdk.services.redshift.alpha.Login masterUser) {
this.props.masterUser(masterUser);
return this;
}
/**
* (experimental) The VPC to place the cluster in.
*
* @return {@code this}
* @param vpc The VPC to place the cluster in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(final software.amazon.awscdk.services.ec2.IVpc vpc) {
this.props.vpc(vpc);
return this;
}
/**
* (experimental) Whether to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster is created.
*
* Default: - false
*
* @return {@code this}
* @see https://docs.aws.amazon.com/redshift/latest/mgmt/managing-cluster-recovery.html
* @param availabilityZoneRelocation Whether to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster is created. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder availabilityZoneRelocation(final java.lang.Boolean availabilityZoneRelocation) {
this.props.availabilityZoneRelocation(availabilityZoneRelocation);
return this;
}
/**
* (experimental) If this flag is set, the cluster resizing type will be set to classic.
*
* When resizing a cluster, classic resizing will always provision a new cluster and transfer the data there.
*
* Classic resize takes more time to complete, but it can be useful in cases where the change in node count or
* the node type to migrate to doesn't fall within the bounds for elastic resize.
*
* Default: - Elastic resize type
*
* @return {@code this}
* @see https://docs.aws.amazon.com/redshift/latest/mgmt/managing-cluster-operations.html#elastic-resize
* @param classicResizing If this flag is set, the cluster resizing type will be set to classic. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder classicResizing(final java.lang.Boolean classicResizing) {
this.props.classicResizing(classicResizing);
return this;
}
/**
* (experimental) An optional identifier for the cluster.
*
* Default: - A name is automatically generated.
*
* @return {@code this}
* @param clusterName An optional identifier for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterName(final java.lang.String clusterName) {
this.props.clusterName(clusterName);
return this;
}
/**
* (experimental) Settings for the individual instances that are launched.
*
* Default: `ClusterType.MULTI_NODE`
*
* @return {@code this}
* @param clusterType Settings for the individual instances that are launched. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterType(final software.amazon.awscdk.services.redshift.alpha.ClusterType clusterType) {
this.props.clusterType(clusterType);
return this;
}
/**
* (experimental) Name of a database which is automatically created inside the cluster.
*
* Default: - default_db
*
* @return {@code this}
* @param defaultDatabaseName Name of a database which is automatically created inside the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder defaultDatabaseName(final java.lang.String defaultDatabaseName) {
this.props.defaultDatabaseName(defaultDatabaseName);
return this;
}
/**
* (experimental) A single AWS Identity and Access Management (IAM) role to be used as the default role for the cluster.
*
* The default role must be included in the roles list.
*
* Default: - No default role is specified for the cluster.
*
* @return {@code this}
* @param defaultRole A single AWS Identity and Access Management (IAM) role to be used as the default role for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder defaultRole(final software.amazon.awscdk.services.iam.IRole defaultRole) {
this.props.defaultRole(defaultRole);
return this;
}
/**
* (experimental) The Elastic IP (EIP) address for the cluster.
*
* Default: - No Elastic IP
*
* @return {@code this}
* @see https://docs.aws.amazon.com/redshift/latest/mgmt/managing-clusters-vpc.html
* @param elasticIp The Elastic IP (EIP) address for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder elasticIp(final java.lang.String elasticIp) {
this.props.elasticIp(elasticIp);
return this;
}
/**
* (experimental) Whether to enable encryption of data at rest in the cluster.
*
* Default: true
*
* @return {@code this}
* @param encrypted Whether to enable encryption of data at rest in the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encrypted(final java.lang.Boolean encrypted) {
this.props.encrypted(encrypted);
return this;
}
/**
* (experimental) The KMS key to use for encryption of data at rest.
*
* Default: - AWS-managed key, if encryption at rest is enabled
*
* @return {@code this}
* @param encryptionKey The KMS key to use for encryption of data at rest. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encryptionKey(final software.amazon.awscdk.services.kms.IKey encryptionKey) {
this.props.encryptionKey(encryptionKey);
return this;
}
/**
* (experimental) If this flag is set, Amazon Redshift forces all COPY and UNLOAD traffic between your cluster and your data repositories through your virtual private cloud (VPC).
*
* Default: - false
*
* @return {@code this}
* @see https://docs.aws.amazon.com/redshift/latest/mgmt/enhanced-vpc-routing.html
* @param enhancedVpcRouting If this flag is set, Amazon Redshift forces all COPY and UNLOAD traffic between your cluster and your data repositories through your virtual private cloud (VPC). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder enhancedVpcRouting(final java.lang.Boolean enhancedVpcRouting) {
this.props.enhancedVpcRouting(enhancedVpcRouting);
return this;
}
/**
* (experimental) Bucket details for log files to be sent to, including prefix.
*
* Default: - No logging bucket is used
*
* @return {@code this}
* @param loggingProperties Bucket details for log files to be sent to, including prefix. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder loggingProperties(final software.amazon.awscdk.services.redshift.alpha.LoggingProperties loggingProperties) {
this.props.loggingProperties(loggingProperties);
return this;
}
/**
* (experimental) Indicating whether Amazon Redshift should deploy the cluster in two Availability Zones.
*
* Default: - false
*
* @return {@code this}
* @param multiAz Indicating whether Amazon Redshift should deploy the cluster in two Availability Zones. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder multiAz(final java.lang.Boolean multiAz) {
this.props.multiAz(multiAz);
return this;
}
/**
* (experimental) The node type to be provisioned for the cluster.
*
* Default: `NodeType.DC2_LARGE`
*
* @return {@code this}
* @param nodeType The node type to be provisioned for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder nodeType(final software.amazon.awscdk.services.redshift.alpha.NodeType nodeType) {
this.props.nodeType(nodeType);
return this;
}
/**
* (experimental) Number of compute nodes in the cluster. Only specify this property for multi-node clusters.
*
* Value must be at least 2 and no more than 100.
*
* Default: - 2 if `clusterType` is ClusterType.MULTI_NODE, undefined otherwise
*
* @return {@code this}
* @param numberOfNodes Number of compute nodes in the cluster. Only specify this property for multi-node clusters. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder numberOfNodes(final java.lang.Number numberOfNodes) {
this.props.numberOfNodes(numberOfNodes);
return this;
}
/**
* (experimental) Additional parameters to pass to the database engine https://docs.aws.amazon.com/redshift/latest/mgmt/working-with-parameter-groups.html.
*
* Default: - No parameter group.
*
* @return {@code this}
* @param parameterGroup Additional parameters to pass to the database engine https://docs.aws.amazon.com/redshift/latest/mgmt/working-with-parameter-groups.html. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder parameterGroup(final software.amazon.awscdk.services.redshift.alpha.IClusterParameterGroup parameterGroup) {
this.props.parameterGroup(parameterGroup);
return this;
}
/**
* (experimental) What port to listen on.
*
* Default: - The default for the engine is used.
*
* @return {@code this}
* @param port What port to listen on. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder port(final java.lang.Number port) {
this.props.port(port);
return this;
}
/**
* (experimental) A preferred maintenance window day/time range. Should be specified as a range ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC).
*
* Example: 'Sun:23:45-Mon:00:15'
*
* Default: - 30-minute window selected at random from an 8-hour block of time for
* each AWS Region, occurring on a random day of the week.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/USER_UpgradeDBInstance.Maintenance.html#Concepts.DBMaintenance
* @param preferredMaintenanceWindow A preferred maintenance window day/time range. Should be specified as a range ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder preferredMaintenanceWindow(final java.lang.String preferredMaintenanceWindow) {
this.props.preferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
* (experimental) Whether to make cluster publicly accessible.
*
* Default: false
*
* @return {@code this}
* @param publiclyAccessible Whether to make cluster publicly accessible. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder publiclyAccessible(final java.lang.Boolean publiclyAccessible) {
this.props.publiclyAccessible(publiclyAccessible);
return this;
}
/**
* (experimental) If this flag is set, the cluster will be rebooted when changes to the cluster's parameter group that require a restart to apply.
*
* Default: false
*
* @return {@code this}
* @param rebootForParameterChanges If this flag is set, the cluster will be rebooted when changes to the cluster's parameter group that require a restart to apply. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder rebootForParameterChanges(final java.lang.Boolean rebootForParameterChanges) {
this.props.rebootForParameterChanges(rebootForParameterChanges);
return this;
}
/**
* (experimental) The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update.
*
* Default: RemovalPolicy.RETAIN
*
* @return {@code this}
* @param removalPolicy The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder removalPolicy(final software.amazon.awscdk.RemovalPolicy removalPolicy) {
this.props.removalPolicy(removalPolicy);
return this;
}
/**
* (experimental) A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services.
*
* The maximum number of roles to attach to a cluster is subject to a quota.
*
* Default: - No role is attached to the cluster.
*
* @return {@code this}
* @param roles A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder roles(final java.util.List extends software.amazon.awscdk.services.iam.IRole> roles) {
this.props.roles(roles);
return this;
}
/**
* (experimental) Security group.
*
* Default: - a new security group is created.
*
* @return {@code this}
* @param securityGroups Security group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder securityGroups(final java.util.List extends software.amazon.awscdk.services.ec2.ISecurityGroup> securityGroups) {
this.props.securityGroups(securityGroups);
return this;
}
/**
* (experimental) A cluster subnet group to use with this cluster.
*
* Default: - a new subnet group will be created.
*
* @return {@code this}
* @param subnetGroup A cluster subnet group to use with this cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subnetGroup(final software.amazon.awscdk.services.redshift.alpha.IClusterSubnetGroup subnetGroup) {
this.props.subnetGroup(subnetGroup);
return this;
}
/**
* (experimental) Where to place the instances within the VPC.
*
* Default: - private subnets
*
* @return {@code this}
* @param vpcSubnets Where to place the instances within the VPC. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcSubnets(final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.props.vpcSubnets(vpcSubnets);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.redshift.alpha.Cluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.redshift.alpha.Cluster build() {
return new software.amazon.awscdk.services.redshift.alpha.Cluster(
this.scope,
this.id,
this.props.build()
);
}
}
}