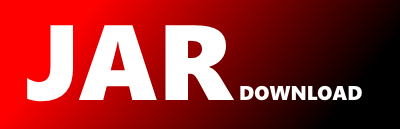
software.amazon.awscdk.services.redshift.CfnEventSubscription Maven / Gradle / Ivy
Show all versions of redshift Show documentation
package software.amazon.awscdk.services.redshift;
/**
* A CloudFormation `AWS::Redshift::EventSubscription`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.redshift.*;
* CfnEventSubscription cfnEventSubscription = CfnEventSubscription.Builder.create(this, "MyCfnEventSubscription")
* .subscriptionName("subscriptionName")
* // the properties below are optional
* .enabled(false)
* .eventCategories(List.of("eventCategories"))
* .severity("severity")
* .snsTopicArn("snsTopicArn")
* .sourceIds(List.of("sourceIds"))
* .sourceType("sourceType")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.58.0 (build f8ba112)", date = "2022-05-11T19:24:16.529Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnEventSubscription")
public class CfnEventSubscription extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnEventSubscription(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnEventSubscription(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.redshift.CfnEventSubscription.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::Redshift::EventSubscription`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnEventSubscription(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.CfnEventSubscriptionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The AWS account associated with the Amazon Redshift event notification subscription.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCustomerAwsId() {
return software.amazon.jsii.Kernel.get(this, "attrCustomerAwsId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the Amazon Redshift event notification subscription.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCustSubscriptionId() {
return software.amazon.jsii.Kernel.get(this, "attrCustSubscriptionId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The list of Amazon Redshift event categories specified in the event notification subscription.
*
* Values: Configuration, Management, Monitoring, Security, Pending
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getAttrEventCategoriesList() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "attrEventCategoriesList", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* A list of the sources that publish events to the Amazon Redshift event notification subscription.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getAttrSourceIdsList() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "attrSourceIdsList", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* The status of the Amazon Redshift event notification subscription.
*
* Constraints:
*
*
* - Can be one of the following: active | no-permission | topic-not-exist
* - The status "no-permission" indicates that Amazon Redshift no longer has permission to post to the Amazon SNS topic. The status "topic-not-exist" indicates that the topic was deleted after the subscription was created.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrStatus() {
return software.amazon.jsii.Kernel.get(this, "attrStatus", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The date and time the Amazon Redshift event notification subscription was created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrSubscriptionCreationTime() {
return software.amazon.jsii.Kernel.get(this, "attrSubscriptionCreationTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* A list of tag instances.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The name of the event subscription to be created.
*
* Constraints:
*
*
* - Cannot be null, empty, or blank.
* - Must contain from 1 to 255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getSubscriptionName() {
return software.amazon.jsii.Kernel.get(this, "subscriptionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the event subscription to be created.
*
* Constraints:
*
*
* - Cannot be null, empty, or blank.
* - Must contain from 1 to 255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSubscriptionName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "subscriptionName", java.util.Objects.requireNonNull(value, "subscriptionName is required"));
}
/**
* A boolean value;
*
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getEnabled() {
return software.amazon.jsii.Kernel.get(this, "enabled", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* A boolean value;
*
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEnabled(final @org.jetbrains.annotations.Nullable java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "enabled", value);
}
/**
* A boolean value;
*
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEnabled(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "enabled", value);
}
/**
* Specifies the Amazon Redshift event categories to be published by the event notification subscription.
*
* Values: configuration, management, monitoring, security, pending
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.util.List getEventCategories() {
return java.util.Optional.ofNullable((java.util.List)(software.amazon.jsii.Kernel.get(this, "eventCategories", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))))).map(java.util.Collections::unmodifiableList).orElse(null);
}
/**
* Specifies the Amazon Redshift event categories to be published by the event notification subscription.
*
* Values: configuration, management, monitoring, security, pending
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEventCategories(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "eventCategories", value);
}
/**
* Specifies the Amazon Redshift event severity to be published by the event notification subscription.
*
* Values: ERROR, INFO
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getSeverity() {
return software.amazon.jsii.Kernel.get(this, "severity", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Specifies the Amazon Redshift event severity to be published by the event notification subscription.
*
* Values: ERROR, INFO
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSeverity(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "severity", value);
}
/**
* The Amazon Resource Name (ARN) of the Amazon SNS topic used to transmit the event notifications.
*
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getSnsTopicArn() {
return software.amazon.jsii.Kernel.get(this, "snsTopicArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Name (ARN) of the Amazon SNS topic used to transmit the event notifications.
*
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSnsTopicArn(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "snsTopicArn", value);
}
/**
* A list of one or more identifiers of Amazon Redshift source objects.
*
* All of the objects must be of the same type as was specified in the source type parameter. The event subscription will return only events generated by the specified objects. If not specified, then events are returned for all objects within the source type specified.
*
* Example: my-cluster-1, my-cluster-2
*
* Example: my-snapshot-20131010
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.util.List getSourceIds() {
return java.util.Optional.ofNullable((java.util.List)(software.amazon.jsii.Kernel.get(this, "sourceIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))))).map(java.util.Collections::unmodifiableList).orElse(null);
}
/**
* A list of one or more identifiers of Amazon Redshift source objects.
*
* All of the objects must be of the same type as was specified in the source type parameter. The event subscription will return only events generated by the specified objects. If not specified, then events are returned for all objects within the source type specified.
*
* Example: my-cluster-1, my-cluster-2
*
* Example: my-snapshot-20131010
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSourceIds(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "sourceIds", value);
}
/**
* The type of source that will be generating the events.
*
* For example, if you want to be notified of events generated by a cluster, you would set this parameter to cluster. If this value is not specified, events are returned for all Amazon Redshift objects in your AWS account . You must specify a source type in order to specify source IDs.
*
* Valid values: cluster, cluster-parameter-group, cluster-security-group, cluster-snapshot, and scheduled-action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getSourceType() {
return software.amazon.jsii.Kernel.get(this, "sourceType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The type of source that will be generating the events.
*
* For example, if you want to be notified of events generated by a cluster, you would set this parameter to cluster. If this value is not specified, events are returned for all Amazon Redshift objects in your AWS account . You must specify a source type in order to specify source IDs.
*
* Valid values: cluster, cluster-parameter-group, cluster-security-group, cluster-snapshot, and scheduled-action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSourceType(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "sourceType", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.redshift.CfnEventSubscription}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.redshift.CfnEventSubscriptionProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.redshift.CfnEventSubscriptionProps.Builder();
}
/**
* The name of the event subscription to be created.
*
* Constraints:
*
*
* - Cannot be null, empty, or blank.
* - Must contain from 1 to 255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* @return {@code this}
* @param subscriptionName The name of the event subscription to be created. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subscriptionName(final java.lang.String subscriptionName) {
this.props.subscriptionName(subscriptionName);
return this;
}
/**
* A boolean value;
*
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
*
* @return {@code this}
* @param enabled A boolean value;. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(final java.lang.Boolean enabled) {
this.props.enabled(enabled);
return this;
}
/**
* A boolean value;
*
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
*
* @return {@code this}
* @param enabled A boolean value;. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(final software.amazon.awscdk.core.IResolvable enabled) {
this.props.enabled(enabled);
return this;
}
/**
* Specifies the Amazon Redshift event categories to be published by the event notification subscription.
*
* Values: configuration, management, monitoring, security, pending
*
* @return {@code this}
* @param eventCategories Specifies the Amazon Redshift event categories to be published by the event notification subscription. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eventCategories(final java.util.List eventCategories) {
this.props.eventCategories(eventCategories);
return this;
}
/**
* Specifies the Amazon Redshift event severity to be published by the event notification subscription.
*
* Values: ERROR, INFO
*
* @return {@code this}
* @param severity Specifies the Amazon Redshift event severity to be published by the event notification subscription. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder severity(final java.lang.String severity) {
this.props.severity(severity);
return this;
}
/**
* The Amazon Resource Name (ARN) of the Amazon SNS topic used to transmit the event notifications.
*
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
*
* @return {@code this}
* @param snsTopicArn The Amazon Resource Name (ARN) of the Amazon SNS topic used to transmit the event notifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder snsTopicArn(final java.lang.String snsTopicArn) {
this.props.snsTopicArn(snsTopicArn);
return this;
}
/**
* A list of one or more identifiers of Amazon Redshift source objects.
*
* All of the objects must be of the same type as was specified in the source type parameter. The event subscription will return only events generated by the specified objects. If not specified, then events are returned for all objects within the source type specified.
*
* Example: my-cluster-1, my-cluster-2
*
* Example: my-snapshot-20131010
*
* @return {@code this}
* @param sourceIds A list of one or more identifiers of Amazon Redshift source objects. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceIds(final java.util.List sourceIds) {
this.props.sourceIds(sourceIds);
return this;
}
/**
* The type of source that will be generating the events.
*
* For example, if you want to be notified of events generated by a cluster, you would set this parameter to cluster. If this value is not specified, events are returned for all Amazon Redshift objects in your AWS account . You must specify a source type in order to specify source IDs.
*
* Valid values: cluster, cluster-parameter-group, cluster-security-group, cluster-snapshot, and scheduled-action.
*
* @return {@code this}
* @param sourceType The type of source that will be generating the events. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceType(final java.lang.String sourceType) {
this.props.sourceType(sourceType);
return this;
}
/**
* A list of tag instances.
*
* @return {@code this}
* @param tags A list of tag instances. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.redshift.CfnEventSubscription}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.redshift.CfnEventSubscription build() {
return new software.amazon.awscdk.services.redshift.CfnEventSubscription(
this.scope,
this.id,
this.props.build()
);
}
}
}