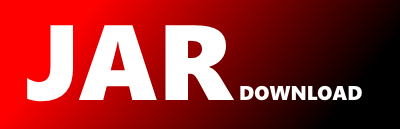
software.amazon.awscdk.services.redshift.CfnCluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redshift Show documentation
Show all versions of redshift Show documentation
The CDK Construct Library for AWS::Redshift
package software.amazon.awscdk.services.redshift;
/**
* A CloudFormation `AWS::Redshift::Cluster`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:17:47.939Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnCluster")
public class CfnCluster extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnCluster(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnCluster(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.redshift.CfnCluster.class, "CFN_RESOURCE_TYPE_NAME", java.lang.String.class);
}
/**
* Create a new `AWS::Redshift::Cluster`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public CfnCluster(final software.amazon.awscdk.core.Construct scope, final java.lang.String id, final software.amazon.awscdk.services.redshift.CfnClusterProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* EXPERIMENTAL
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public void inspect(final software.amazon.awscdk.core.TreeInspector inspector) {
this.jsiiCall("inspect", Void.class, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
@Override
protected java.util.Map renderProperties(final java.util.Map props) {
return java.util.Collections.unmodifiableMap(this.jsiiCall("renderProperties", java.util.Map.class, new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getAttrEndpointAddress() {
return this.jsiiGet("attrEndpointAddress", java.lang.String.class);
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getAttrEndpointPort() {
return this.jsiiGet("attrEndpointPort", java.lang.String.class);
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
protected java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(this.jsiiGet("cfnProperties", java.util.Map.class));
}
/**
* `AWS::Redshift::Cluster.Tags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-tags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public software.amazon.awscdk.core.TagManager getTags() {
return this.jsiiGet("tags", software.amazon.awscdk.core.TagManager.class);
}
/**
* `AWS::Redshift::Cluster.ClusterType`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clustertype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getClusterType() {
return this.jsiiGet("clusterType", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.ClusterType`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clustertype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setClusterType(final java.lang.String value) {
this.jsiiSet("clusterType", java.util.Objects.requireNonNull(value, "clusterType is required"));
}
/**
* `AWS::Redshift::Cluster.DBName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-dbname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getDbName() {
return this.jsiiGet("dbName", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.DBName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-dbname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setDbName(final java.lang.String value) {
this.jsiiSet("dbName", java.util.Objects.requireNonNull(value, "dbName is required"));
}
/**
* `AWS::Redshift::Cluster.MasterUsername`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-masterusername
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getMasterUsername() {
return this.jsiiGet("masterUsername", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.MasterUsername`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-masterusername
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setMasterUsername(final java.lang.String value) {
this.jsiiSet("masterUsername", java.util.Objects.requireNonNull(value, "masterUsername is required"));
}
/**
* `AWS::Redshift::Cluster.MasterUserPassword`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-masteruserpassword
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getMasterUserPassword() {
return this.jsiiGet("masterUserPassword", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.MasterUserPassword`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-masteruserpassword
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setMasterUserPassword(final java.lang.String value) {
this.jsiiSet("masterUserPassword", java.util.Objects.requireNonNull(value, "masterUserPassword is required"));
}
/**
* `AWS::Redshift::Cluster.NodeType`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-nodetype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getNodeType() {
return this.jsiiGet("nodeType", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.NodeType`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-nodetype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setNodeType(final java.lang.String value) {
this.jsiiSet("nodeType", java.util.Objects.requireNonNull(value, "nodeType is required"));
}
/**
* `AWS::Redshift::Cluster.AllowVersionUpgrade`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-allowversionupgrade
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Object getAllowVersionUpgrade() {
return this.jsiiGet("allowVersionUpgrade", java.lang.Object.class);
}
/**
* `AWS::Redshift::Cluster.AllowVersionUpgrade`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-allowversionupgrade
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setAllowVersionUpgrade(final java.lang.Boolean value) {
this.jsiiSet("allowVersionUpgrade", value);
}
/**
* `AWS::Redshift::Cluster.AllowVersionUpgrade`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-allowversionupgrade
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setAllowVersionUpgrade(final software.amazon.awscdk.core.IResolvable value) {
this.jsiiSet("allowVersionUpgrade", value);
}
/**
* `AWS::Redshift::Cluster.AutomatedSnapshotRetentionPeriod`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-automatedsnapshotretentionperiod
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Number getAutomatedSnapshotRetentionPeriod() {
return this.jsiiGet("automatedSnapshotRetentionPeriod", java.lang.Number.class);
}
/**
* `AWS::Redshift::Cluster.AutomatedSnapshotRetentionPeriod`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-automatedsnapshotretentionperiod
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setAutomatedSnapshotRetentionPeriod(final java.lang.Number value) {
this.jsiiSet("automatedSnapshotRetentionPeriod", value);
}
/**
* `AWS::Redshift::Cluster.AvailabilityZone`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-availabilityzone
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getAvailabilityZone() {
return this.jsiiGet("availabilityZone", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.AvailabilityZone`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-availabilityzone
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setAvailabilityZone(final java.lang.String value) {
this.jsiiSet("availabilityZone", value);
}
/**
* `AWS::Redshift::Cluster.ClusterIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clusteridentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getClusterIdentifier() {
return this.jsiiGet("clusterIdentifier", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.ClusterIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clusteridentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setClusterIdentifier(final java.lang.String value) {
this.jsiiSet("clusterIdentifier", value);
}
/**
* `AWS::Redshift::Cluster.ClusterParameterGroupName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clusterparametergroupname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getClusterParameterGroupName() {
return this.jsiiGet("clusterParameterGroupName", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.ClusterParameterGroupName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clusterparametergroupname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setClusterParameterGroupName(final java.lang.String value) {
this.jsiiSet("clusterParameterGroupName", value);
}
/**
* `AWS::Redshift::Cluster.ClusterSecurityGroups`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clustersecuritygroups
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.util.List getClusterSecurityGroups() {
return java.util.Collections.unmodifiableList(this.jsiiGet("clusterSecurityGroups", java.util.List.class));
}
/**
* `AWS::Redshift::Cluster.ClusterSecurityGroups`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clustersecuritygroups
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setClusterSecurityGroups(final java.util.List value) {
this.jsiiSet("clusterSecurityGroups", value);
}
/**
* `AWS::Redshift::Cluster.ClusterSubnetGroupName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clustersubnetgroupname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getClusterSubnetGroupName() {
return this.jsiiGet("clusterSubnetGroupName", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.ClusterSubnetGroupName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clustersubnetgroupname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setClusterSubnetGroupName(final java.lang.String value) {
this.jsiiSet("clusterSubnetGroupName", value);
}
/**
* `AWS::Redshift::Cluster.ClusterVersion`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clusterversion
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getClusterVersion() {
return this.jsiiGet("clusterVersion", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.ClusterVersion`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-clusterversion
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setClusterVersion(final java.lang.String value) {
this.jsiiSet("clusterVersion", value);
}
/**
* `AWS::Redshift::Cluster.ElasticIp`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-elasticip
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getElasticIp() {
return this.jsiiGet("elasticIp", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.ElasticIp`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-elasticip
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setElasticIp(final java.lang.String value) {
this.jsiiSet("elasticIp", value);
}
/**
* `AWS::Redshift::Cluster.Encrypted`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-encrypted
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Object getEncrypted() {
return this.jsiiGet("encrypted", java.lang.Object.class);
}
/**
* `AWS::Redshift::Cluster.Encrypted`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-encrypted
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setEncrypted(final java.lang.Boolean value) {
this.jsiiSet("encrypted", value);
}
/**
* `AWS::Redshift::Cluster.Encrypted`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-encrypted
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setEncrypted(final software.amazon.awscdk.core.IResolvable value) {
this.jsiiSet("encrypted", value);
}
/**
* `AWS::Redshift::Cluster.HsmClientCertificateIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-hsmclientcertidentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getHsmClientCertificateIdentifier() {
return this.jsiiGet("hsmClientCertificateIdentifier", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.HsmClientCertificateIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-hsmclientcertidentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setHsmClientCertificateIdentifier(final java.lang.String value) {
this.jsiiSet("hsmClientCertificateIdentifier", value);
}
/**
* `AWS::Redshift::Cluster.HsmConfigurationIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-HsmConfigurationIdentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getHsmConfigurationIdentifier() {
return this.jsiiGet("hsmConfigurationIdentifier", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.HsmConfigurationIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-HsmConfigurationIdentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setHsmConfigurationIdentifier(final java.lang.String value) {
this.jsiiSet("hsmConfigurationIdentifier", value);
}
/**
* `AWS::Redshift::Cluster.IamRoles`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-iamroles
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.util.List getIamRoles() {
return java.util.Collections.unmodifiableList(this.jsiiGet("iamRoles", java.util.List.class));
}
/**
* `AWS::Redshift::Cluster.IamRoles`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-iamroles
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setIamRoles(final java.util.List value) {
this.jsiiSet("iamRoles", value);
}
/**
* `AWS::Redshift::Cluster.KmsKeyId`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-kmskeyid
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getKmsKeyId() {
return this.jsiiGet("kmsKeyId", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.KmsKeyId`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-kmskeyid
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setKmsKeyId(final java.lang.String value) {
this.jsiiSet("kmsKeyId", value);
}
/**
* `AWS::Redshift::Cluster.LoggingProperties`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-loggingproperties
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Object getLoggingProperties() {
return this.jsiiGet("loggingProperties", java.lang.Object.class);
}
/**
* `AWS::Redshift::Cluster.LoggingProperties`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-loggingproperties
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setLoggingProperties(final software.amazon.awscdk.core.IResolvable value) {
this.jsiiSet("loggingProperties", value);
}
/**
* `AWS::Redshift::Cluster.LoggingProperties`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-loggingproperties
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setLoggingProperties(final software.amazon.awscdk.services.redshift.CfnCluster.LoggingPropertiesProperty value) {
this.jsiiSet("loggingProperties", value);
}
/**
* `AWS::Redshift::Cluster.NumberOfNodes`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-nodetype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Number getNumberOfNodes() {
return this.jsiiGet("numberOfNodes", java.lang.Number.class);
}
/**
* `AWS::Redshift::Cluster.NumberOfNodes`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-nodetype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setNumberOfNodes(final java.lang.Number value) {
this.jsiiSet("numberOfNodes", value);
}
/**
* `AWS::Redshift::Cluster.OwnerAccount`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-owneraccount
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getOwnerAccount() {
return this.jsiiGet("ownerAccount", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.OwnerAccount`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-owneraccount
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setOwnerAccount(final java.lang.String value) {
this.jsiiSet("ownerAccount", value);
}
/**
* `AWS::Redshift::Cluster.Port`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-port
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Number getPort() {
return this.jsiiGet("port", java.lang.Number.class);
}
/**
* `AWS::Redshift::Cluster.Port`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-port
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setPort(final java.lang.Number value) {
this.jsiiSet("port", value);
}
/**
* `AWS::Redshift::Cluster.PreferredMaintenanceWindow`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-preferredmaintenancewindow
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getPreferredMaintenanceWindow() {
return this.jsiiGet("preferredMaintenanceWindow", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.PreferredMaintenanceWindow`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-preferredmaintenancewindow
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setPreferredMaintenanceWindow(final java.lang.String value) {
this.jsiiSet("preferredMaintenanceWindow", value);
}
/**
* `AWS::Redshift::Cluster.PubliclyAccessible`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-publiclyaccessible
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.Object getPubliclyAccessible() {
return this.jsiiGet("publiclyAccessible", java.lang.Object.class);
}
/**
* `AWS::Redshift::Cluster.PubliclyAccessible`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-publiclyaccessible
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setPubliclyAccessible(final java.lang.Boolean value) {
this.jsiiSet("publiclyAccessible", value);
}
/**
* `AWS::Redshift::Cluster.PubliclyAccessible`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-publiclyaccessible
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setPubliclyAccessible(final software.amazon.awscdk.core.IResolvable value) {
this.jsiiSet("publiclyAccessible", value);
}
/**
* `AWS::Redshift::Cluster.SnapshotClusterIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-snapshotclusteridentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getSnapshotClusterIdentifier() {
return this.jsiiGet("snapshotClusterIdentifier", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.SnapshotClusterIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-snapshotclusteridentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setSnapshotClusterIdentifier(final java.lang.String value) {
this.jsiiSet("snapshotClusterIdentifier", value);
}
/**
* `AWS::Redshift::Cluster.SnapshotIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-snapshotidentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.lang.String getSnapshotIdentifier() {
return this.jsiiGet("snapshotIdentifier", java.lang.String.class);
}
/**
* `AWS::Redshift::Cluster.SnapshotIdentifier`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-snapshotidentifier
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setSnapshotIdentifier(final java.lang.String value) {
this.jsiiSet("snapshotIdentifier", value);
}
/**
* `AWS::Redshift::Cluster.VpcSecurityGroupIds`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-vpcsecuritygroupids
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public java.util.List getVpcSecurityGroupIds() {
return java.util.Collections.unmodifiableList(this.jsiiGet("vpcSecurityGroupIds", java.util.List.class));
}
/**
* `AWS::Redshift::Cluster.VpcSecurityGroupIds`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-cluster.html#cfn-redshift-cluster-vpcsecuritygroupids
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public void setVpcSecurityGroupIds(final java.util.List value) {
this.jsiiSet("vpcSecurityGroupIds", value);
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-redshift-cluster-loggingproperties.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnCluster.LoggingPropertiesProperty")
@software.amazon.jsii.Jsii.Proxy(LoggingPropertiesProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public static interface LoggingPropertiesProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnCluster.LoggingPropertiesProperty.BucketName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-redshift-cluster-loggingproperties.html#cfn-redshift-cluster-loggingproperties-bucketname
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
java.lang.String getBucketName();
/**
* `CfnCluster.LoggingPropertiesProperty.S3KeyPrefix`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-redshift-cluster-loggingproperties.html#cfn-redshift-cluster-loggingproperties-s3keyprefix
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
default java.lang.String getS3KeyPrefix() {
return null;
}
/**
* @return a {@link Builder} of {@link LoggingPropertiesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link LoggingPropertiesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public static final class Builder {
private java.lang.String bucketName;
private java.lang.String s3KeyPrefix;
/**
* Sets the value of BucketName
* @param bucketName `CfnCluster.LoggingPropertiesProperty.BucketName`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder bucketName(java.lang.String bucketName) {
this.bucketName = bucketName;
return this;
}
/**
* Sets the value of S3KeyPrefix
* @param s3KeyPrefix `CfnCluster.LoggingPropertiesProperty.S3KeyPrefix`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder s3KeyPrefix(java.lang.String s3KeyPrefix) {
this.s3KeyPrefix = s3KeyPrefix;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link LoggingPropertiesProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public LoggingPropertiesProperty build() {
return new Jsii$Proxy(bucketName, s3KeyPrefix);
}
}
/**
* An implementation for {@link LoggingPropertiesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements LoggingPropertiesProperty {
private final java.lang.String bucketName;
private final java.lang.String s3KeyPrefix;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.bucketName = this.jsiiGet("bucketName", java.lang.String.class);
this.s3KeyPrefix = this.jsiiGet("s3KeyPrefix", java.lang.String.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.lang.String bucketName, final java.lang.String s3KeyPrefix) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.bucketName = java.util.Objects.requireNonNull(bucketName, "bucketName is required");
this.s3KeyPrefix = s3KeyPrefix;
}
@Override
public java.lang.String getBucketName() {
return this.bucketName;
}
@Override
public java.lang.String getS3KeyPrefix() {
return this.s3KeyPrefix;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("bucketName", om.valueToTree(this.getBucketName()));
if (this.getS3KeyPrefix() != null) {
data.set("s3KeyPrefix", om.valueToTree(this.getS3KeyPrefix()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-redshift.CfnCluster.LoggingPropertiesProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LoggingPropertiesProperty.Jsii$Proxy that = (LoggingPropertiesProperty.Jsii$Proxy) o;
if (!bucketName.equals(that.bucketName)) return false;
return this.s3KeyPrefix != null ? this.s3KeyPrefix.equals(that.s3KeyPrefix) : that.s3KeyPrefix == null;
}
@Override
public int hashCode() {
int result = this.bucketName.hashCode();
result = 31 * result + (this.s3KeyPrefix != null ? this.s3KeyPrefix.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.redshift.CfnCluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public static final class Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.redshift.CfnClusterProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.redshift.CfnClusterProps.Builder();
}
/**
* @return {@code this}
* @param clusterType `AWS::Redshift::Cluster.ClusterType`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder clusterType(final java.lang.String clusterType) {
this.props.clusterType(clusterType);
return this;
}
/**
* @return {@code this}
* @param dbName `AWS::Redshift::Cluster.DBName`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder dbName(final java.lang.String dbName) {
this.props.dbName(dbName);
return this;
}
/**
* @return {@code this}
* @param masterUsername `AWS::Redshift::Cluster.MasterUsername`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder masterUsername(final java.lang.String masterUsername) {
this.props.masterUsername(masterUsername);
return this;
}
/**
* @return {@code this}
* @param masterUserPassword `AWS::Redshift::Cluster.MasterUserPassword`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder masterUserPassword(final java.lang.String masterUserPassword) {
this.props.masterUserPassword(masterUserPassword);
return this;
}
/**
* @return {@code this}
* @param nodeType `AWS::Redshift::Cluster.NodeType`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder nodeType(final java.lang.String nodeType) {
this.props.nodeType(nodeType);
return this;
}
/**
* @return {@code this}
* @param allowVersionUpgrade `AWS::Redshift::Cluster.AllowVersionUpgrade`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder allowVersionUpgrade(final java.lang.Boolean allowVersionUpgrade) {
this.props.allowVersionUpgrade(allowVersionUpgrade);
return this;
}
/**
* @return {@code this}
* @param allowVersionUpgrade `AWS::Redshift::Cluster.AllowVersionUpgrade`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder allowVersionUpgrade(final software.amazon.awscdk.core.IResolvable allowVersionUpgrade) {
this.props.allowVersionUpgrade(allowVersionUpgrade);
return this;
}
/**
* @return {@code this}
* @param automatedSnapshotRetentionPeriod `AWS::Redshift::Cluster.AutomatedSnapshotRetentionPeriod`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder automatedSnapshotRetentionPeriod(final java.lang.Number automatedSnapshotRetentionPeriod) {
this.props.automatedSnapshotRetentionPeriod(automatedSnapshotRetentionPeriod);
return this;
}
/**
* @return {@code this}
* @param availabilityZone `AWS::Redshift::Cluster.AvailabilityZone`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder availabilityZone(final java.lang.String availabilityZone) {
this.props.availabilityZone(availabilityZone);
return this;
}
/**
* @return {@code this}
* @param clusterIdentifier `AWS::Redshift::Cluster.ClusterIdentifier`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder clusterIdentifier(final java.lang.String clusterIdentifier) {
this.props.clusterIdentifier(clusterIdentifier);
return this;
}
/**
* @return {@code this}
* @param clusterParameterGroupName `AWS::Redshift::Cluster.ClusterParameterGroupName`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder clusterParameterGroupName(final java.lang.String clusterParameterGroupName) {
this.props.clusterParameterGroupName(clusterParameterGroupName);
return this;
}
/**
* @return {@code this}
* @param clusterSecurityGroups `AWS::Redshift::Cluster.ClusterSecurityGroups`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder clusterSecurityGroups(final java.util.List clusterSecurityGroups) {
this.props.clusterSecurityGroups(clusterSecurityGroups);
return this;
}
/**
* @return {@code this}
* @param clusterSubnetGroupName `AWS::Redshift::Cluster.ClusterSubnetGroupName`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder clusterSubnetGroupName(final java.lang.String clusterSubnetGroupName) {
this.props.clusterSubnetGroupName(clusterSubnetGroupName);
return this;
}
/**
* @return {@code this}
* @param clusterVersion `AWS::Redshift::Cluster.ClusterVersion`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder clusterVersion(final java.lang.String clusterVersion) {
this.props.clusterVersion(clusterVersion);
return this;
}
/**
* @return {@code this}
* @param elasticIp `AWS::Redshift::Cluster.ElasticIp`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder elasticIp(final java.lang.String elasticIp) {
this.props.elasticIp(elasticIp);
return this;
}
/**
* @return {@code this}
* @param encrypted `AWS::Redshift::Cluster.Encrypted`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder encrypted(final java.lang.Boolean encrypted) {
this.props.encrypted(encrypted);
return this;
}
/**
* @return {@code this}
* @param encrypted `AWS::Redshift::Cluster.Encrypted`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder encrypted(final software.amazon.awscdk.core.IResolvable encrypted) {
this.props.encrypted(encrypted);
return this;
}
/**
* @return {@code this}
* @param hsmClientCertificateIdentifier `AWS::Redshift::Cluster.HsmClientCertificateIdentifier`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder hsmClientCertificateIdentifier(final java.lang.String hsmClientCertificateIdentifier) {
this.props.hsmClientCertificateIdentifier(hsmClientCertificateIdentifier);
return this;
}
/**
* @return {@code this}
* @param hsmConfigurationIdentifier `AWS::Redshift::Cluster.HsmConfigurationIdentifier`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder hsmConfigurationIdentifier(final java.lang.String hsmConfigurationIdentifier) {
this.props.hsmConfigurationIdentifier(hsmConfigurationIdentifier);
return this;
}
/**
* @return {@code this}
* @param iamRoles `AWS::Redshift::Cluster.IamRoles`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder iamRoles(final java.util.List iamRoles) {
this.props.iamRoles(iamRoles);
return this;
}
/**
* @return {@code this}
* @param kmsKeyId `AWS::Redshift::Cluster.KmsKeyId`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder kmsKeyId(final java.lang.String kmsKeyId) {
this.props.kmsKeyId(kmsKeyId);
return this;
}
/**
* @return {@code this}
* @param loggingProperties `AWS::Redshift::Cluster.LoggingProperties`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder loggingProperties(final software.amazon.awscdk.core.IResolvable loggingProperties) {
this.props.loggingProperties(loggingProperties);
return this;
}
/**
* @return {@code this}
* @param loggingProperties `AWS::Redshift::Cluster.LoggingProperties`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder loggingProperties(final software.amazon.awscdk.services.redshift.CfnCluster.LoggingPropertiesProperty loggingProperties) {
this.props.loggingProperties(loggingProperties);
return this;
}
/**
* @return {@code this}
* @param numberOfNodes `AWS::Redshift::Cluster.NumberOfNodes`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder numberOfNodes(final java.lang.Number numberOfNodes) {
this.props.numberOfNodes(numberOfNodes);
return this;
}
/**
* @return {@code this}
* @param ownerAccount `AWS::Redshift::Cluster.OwnerAccount`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder ownerAccount(final java.lang.String ownerAccount) {
this.props.ownerAccount(ownerAccount);
return this;
}
/**
* @return {@code this}
* @param port `AWS::Redshift::Cluster.Port`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder port(final java.lang.Number port) {
this.props.port(port);
return this;
}
/**
* @return {@code this}
* @param preferredMaintenanceWindow `AWS::Redshift::Cluster.PreferredMaintenanceWindow`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder preferredMaintenanceWindow(final java.lang.String preferredMaintenanceWindow) {
this.props.preferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
* @return {@code this}
* @param publiclyAccessible `AWS::Redshift::Cluster.PubliclyAccessible`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder publiclyAccessible(final java.lang.Boolean publiclyAccessible) {
this.props.publiclyAccessible(publiclyAccessible);
return this;
}
/**
* @return {@code this}
* @param publiclyAccessible `AWS::Redshift::Cluster.PubliclyAccessible`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder publiclyAccessible(final software.amazon.awscdk.core.IResolvable publiclyAccessible) {
this.props.publiclyAccessible(publiclyAccessible);
return this;
}
/**
* @return {@code this}
* @param snapshotClusterIdentifier `AWS::Redshift::Cluster.SnapshotClusterIdentifier`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder snapshotClusterIdentifier(final java.lang.String snapshotClusterIdentifier) {
this.props.snapshotClusterIdentifier(snapshotClusterIdentifier);
return this;
}
/**
* @return {@code this}
* @param snapshotIdentifier `AWS::Redshift::Cluster.SnapshotIdentifier`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder snapshotIdentifier(final java.lang.String snapshotIdentifier) {
this.props.snapshotIdentifier(snapshotIdentifier);
return this;
}
/**
* @return {@code this}
* @param tags `AWS::Redshift::Cluster.Tags`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder tags(final java.util.List tags) {
this.props.tags(tags);
return this;
}
/**
* @return {@code this}
* @param vpcSecurityGroupIds `AWS::Redshift::Cluster.VpcSecurityGroupIds`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder vpcSecurityGroupIds(final java.util.List vpcSecurityGroupIds) {
this.props.vpcSecurityGroupIds(vpcSecurityGroupIds);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.redshift.CfnCluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public software.amazon.awscdk.services.redshift.CfnCluster build() {
return new software.amazon.awscdk.services.redshift.CfnCluster(
this.scope,
this.id,
this.props.build()
);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy