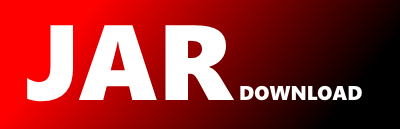
software.amazon.awscdk.services.redshift.CfnClusterParameterGroupProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redshift Show documentation
Show all versions of redshift Show documentation
The CDK Construct Library for AWS::Redshift
package software.amazon.awscdk.services.redshift;
/**
* Properties for defining a `AWS::Redshift::ClusterParameterGroup`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-clusterparametergroup.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:17:47.941Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnClusterParameterGroupProps")
@software.amazon.jsii.Jsii.Proxy(CfnClusterParameterGroupProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public interface CfnClusterParameterGroupProps extends software.amazon.jsii.JsiiSerializable {
/**
* `AWS::Redshift::ClusterParameterGroup.Description`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-clusterparametergroup.html#cfn-redshift-clusterparametergroup-description
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
java.lang.String getDescription();
/**
* `AWS::Redshift::ClusterParameterGroup.ParameterGroupFamily`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-clusterparametergroup.html#cfn-redshift-clusterparametergroup-parametergroupfamily
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
java.lang.String getParameterGroupFamily();
/**
* `AWS::Redshift::ClusterParameterGroup.Parameters`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-clusterparametergroup.html#cfn-redshift-clusterparametergroup-parameters
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
default java.lang.Object getParameters() {
return null;
}
/**
* `AWS::Redshift::ClusterParameterGroup.Tags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-redshift-clusterparametergroup.html#cfn-redshift-clusterparametergroup-tags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
default java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnClusterParameterGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnClusterParameterGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public static final class Builder {
private java.lang.String description;
private java.lang.String parameterGroupFamily;
private java.lang.Object parameters;
private java.util.List tags;
/**
* Sets the value of Description
* @param description `AWS::Redshift::ClusterParameterGroup.Description`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of ParameterGroupFamily
* @param parameterGroupFamily `AWS::Redshift::ClusterParameterGroup.ParameterGroupFamily`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder parameterGroupFamily(java.lang.String parameterGroupFamily) {
this.parameterGroupFamily = parameterGroupFamily;
return this;
}
/**
* Sets the value of Parameters
* @param parameters `AWS::Redshift::ClusterParameterGroup.Parameters`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder parameters(software.amazon.awscdk.core.IResolvable parameters) {
this.parameters = parameters;
return this;
}
/**
* Sets the value of Parameters
* @param parameters `AWS::Redshift::ClusterParameterGroup.Parameters`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder parameters(java.util.List parameters) {
this.parameters = parameters;
return this;
}
/**
* Sets the value of Tags
* @param tags `AWS::Redshift::ClusterParameterGroup.Tags`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public Builder tags(java.util.List tags) {
this.tags = tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnClusterParameterGroupProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
public CfnClusterParameterGroupProps build() {
return new Jsii$Proxy(description, parameterGroupFamily, parameters, tags);
}
}
/**
* An implementation for {@link CfnClusterParameterGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.External)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnClusterParameterGroupProps {
private final java.lang.String description;
private final java.lang.String parameterGroupFamily;
private final java.lang.Object parameters;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.description = this.jsiiGet("description", java.lang.String.class);
this.parameterGroupFamily = this.jsiiGet("parameterGroupFamily", java.lang.String.class);
this.parameters = this.jsiiGet("parameters", java.lang.Object.class);
this.tags = this.jsiiGet("tags", java.util.List.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.lang.String description, final java.lang.String parameterGroupFamily, final java.lang.Object parameters, final java.util.List tags) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.description = java.util.Objects.requireNonNull(description, "description is required");
this.parameterGroupFamily = java.util.Objects.requireNonNull(parameterGroupFamily, "parameterGroupFamily is required");
this.parameters = parameters;
this.tags = tags;
}
@Override
public java.lang.String getDescription() {
return this.description;
}
@Override
public java.lang.String getParameterGroupFamily() {
return this.parameterGroupFamily;
}
@Override
public java.lang.Object getParameters() {
return this.parameters;
}
@Override
public java.util.List getTags() {
return this.tags;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("description", om.valueToTree(this.getDescription()));
data.set("parameterGroupFamily", om.valueToTree(this.getParameterGroupFamily()));
if (this.getParameters() != null) {
data.set("parameters", om.valueToTree(this.getParameters()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-redshift.CfnClusterParameterGroupProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnClusterParameterGroupProps.Jsii$Proxy that = (CfnClusterParameterGroupProps.Jsii$Proxy) o;
if (!description.equals(that.description)) return false;
if (!parameterGroupFamily.equals(that.parameterGroupFamily)) return false;
if (this.parameters != null ? !this.parameters.equals(that.parameters) : that.parameters != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public int hashCode() {
int result = this.description.hashCode();
result = 31 * result + (this.parameterGroupFamily.hashCode());
result = 31 * result + (this.parameters != null ? this.parameters.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy