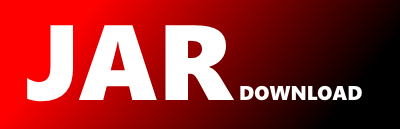
software.amazon.awscdk.services.redshift.CfnClusterParameterGroup Maven / Gradle / Ivy
Show all versions of redshift Show documentation
package software.amazon.awscdk.services.redshift;
/**
* A CloudFormation `AWS::Redshift::ClusterParameterGroup`.
*
* Describes a parameter group.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.redshift.*;
* CfnClusterParameterGroup cfnClusterParameterGroup = CfnClusterParameterGroup.Builder.create(this, "MyCfnClusterParameterGroup")
* .description("description")
* .parameterGroupFamily("parameterGroupFamily")
* // the properties below are optional
* .parameters(List.of(ParameterProperty.builder()
* .parameterName("parameterName")
* .parameterValue("parameterValue")
* .build()))
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.73.0 (build 6faeda3)", date = "2023-01-31T18:36:56.271Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnClusterParameterGroup")
public class CfnClusterParameterGroup extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnClusterParameterGroup(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnClusterParameterGroup(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.redshift.CfnClusterParameterGroup.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::Redshift::ClusterParameterGroup`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnClusterParameterGroup(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.CfnClusterParameterGroupProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The name of the parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrParameterGroupName() {
return software.amazon.jsii.Kernel.get(this, "attrParameterGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The list of tags for the cluster parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The description of the parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the parameter group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", java.util.Objects.requireNonNull(value, "description is required"));
}
/**
* The name of the cluster parameter group family that this cluster parameter group is compatible with.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getParameterGroupFamily() {
return software.amazon.jsii.Kernel.get(this, "parameterGroupFamily", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the cluster parameter group family that this cluster parameter group is compatible with.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setParameterGroupFamily(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "parameterGroupFamily", java.util.Objects.requireNonNull(value, "parameterGroupFamily is required"));
}
/**
* An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request.
*
* For each parameter to be modified, you must supply at least the parameter name and parameter value; other name-value pairs of the parameter are optional.
*
* For the workload management (WLM) configuration, you must supply all the name-value pairs in the wlm_json_configuration parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getParameters() {
return software.amazon.jsii.Kernel.get(this, "parameters", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request.
*
* For each parameter to be modified, you must supply at least the parameter name and parameter value; other name-value pairs of the parameter are optional.
*
* For the workload management (WLM) configuration, you must supply all the name-value pairs in the wlm_json_configuration parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setParameters(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "parameters", value);
}
/**
* An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request.
*
* For each parameter to be modified, you must supply at least the parameter name and parameter value; other name-value pairs of the parameter are optional.
*
* For the workload management (WLM) configuration, you must supply all the name-value pairs in the wlm_json_configuration parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setParameters(final @org.jetbrains.annotations.Nullable java.util.List value) {
if (software.amazon.jsii.Configuration.getRuntimeTypeChecking()) {
for (int __idx_ac66f0 = 0; __idx_ac66f0 < value.size(); __idx_ac66f0++) {
final java.lang.Object __val_ac66f0 = value.get(__idx_ac66f0);
if (
!(__val_ac66f0 instanceof software.amazon.awscdk.core.IResolvable)
&& !(__val_ac66f0 instanceof software.amazon.awscdk.services.redshift.CfnClusterParameterGroup.ParameterProperty)
&& !(__val_ac66f0.getClass().equals(software.amazon.jsii.JsiiObject.class))
) {
throw new IllegalArgumentException(
new java.lang.StringBuilder("Expected ")
.append("value").append(".get(").append(__idx_ac66f0).append(")")
.append(" to be one of: software.amazon.awscdk.core.IResolvable, software.amazon.awscdk.services.redshift.CfnClusterParameterGroup.ParameterProperty; received ")
.append(__val_ac66f0.getClass()).toString());
}
}
}
software.amazon.jsii.Kernel.set(this, "parameters", value);
}
/**
* Describes a parameter in a cluster parameter group.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.redshift.*;
* ParameterProperty parameterProperty = ParameterProperty.builder()
* .parameterName("parameterName")
* .parameterValue("parameterValue")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnClusterParameterGroup.ParameterProperty")
@software.amazon.jsii.Jsii.Proxy(ParameterProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ParameterProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getParameterName();
/**
* The value of the parameter.
*
* If ParameterName
is wlm_json_configuration
, then the maximum size of ParameterValue
is 8000 characters.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getParameterValue();
/**
* @return a {@link Builder} of {@link ParameterProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ParameterProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String parameterName;
java.lang.String parameterValue;
/**
* Sets the value of {@link ParameterProperty#getParameterName}
* @param parameterName The name of the parameter. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterName(java.lang.String parameterName) {
this.parameterName = parameterName;
return this;
}
/**
* Sets the value of {@link ParameterProperty#getParameterValue}
* @param parameterValue The value of the parameter. This parameter is required.
* If ParameterName
is wlm_json_configuration
, then the maximum size of ParameterValue
is 8000 characters.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterValue(java.lang.String parameterValue) {
this.parameterValue = parameterValue;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ParameterProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ParameterProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ParameterProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ParameterProperty {
private final java.lang.String parameterName;
private final java.lang.String parameterValue;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.parameterName = software.amazon.jsii.Kernel.get(this, "parameterName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.parameterValue = software.amazon.jsii.Kernel.get(this, "parameterValue", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.parameterName = java.util.Objects.requireNonNull(builder.parameterName, "parameterName is required");
this.parameterValue = java.util.Objects.requireNonNull(builder.parameterValue, "parameterValue is required");
}
@Override
public final java.lang.String getParameterName() {
return this.parameterName;
}
@Override
public final java.lang.String getParameterValue() {
return this.parameterValue;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("parameterName", om.valueToTree(this.getParameterName()));
data.set("parameterValue", om.valueToTree(this.getParameterValue()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-redshift.CfnClusterParameterGroup.ParameterProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ParameterProperty.Jsii$Proxy that = (ParameterProperty.Jsii$Proxy) o;
if (!parameterName.equals(that.parameterName)) return false;
return this.parameterValue.equals(that.parameterValue);
}
@Override
public final int hashCode() {
int result = this.parameterName.hashCode();
result = 31 * result + (this.parameterValue.hashCode());
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.redshift.CfnClusterParameterGroup}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.redshift.CfnClusterParameterGroupProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.redshift.CfnClusterParameterGroupProps.Builder();
}
/**
* The description of the parameter group.
*
* @return {@code this}
* @param description The description of the parameter group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* The name of the cluster parameter group family that this cluster parameter group is compatible with.
*
* @return {@code this}
* @param parameterGroupFamily The name of the cluster parameter group family that this cluster parameter group is compatible with. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterGroupFamily(final java.lang.String parameterGroupFamily) {
this.props.parameterGroupFamily(parameterGroupFamily);
return this;
}
/**
* An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request.
*
* For each parameter to be modified, you must supply at least the parameter name and parameter value; other name-value pairs of the parameter are optional.
*
* For the workload management (WLM) configuration, you must supply all the name-value pairs in the wlm_json_configuration parameter.
*
* @return {@code this}
* @param parameters An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(final software.amazon.awscdk.core.IResolvable parameters) {
this.props.parameters(parameters);
return this;
}
/**
* An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request.
*
* For each parameter to be modified, you must supply at least the parameter name and parameter value; other name-value pairs of the parameter are optional.
*
* For the workload management (WLM) configuration, you must supply all the name-value pairs in the wlm_json_configuration parameter.
*
* @return {@code this}
* @param parameters An array of parameters to be modified. A maximum of 20 parameters can be modified in a single request. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(final java.util.List extends java.lang.Object> parameters) {
this.props.parameters(parameters);
return this;
}
/**
* The list of tags for the cluster parameter group.
*
* @return {@code this}
* @param tags The list of tags for the cluster parameter group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.redshift.CfnClusterParameterGroup}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.redshift.CfnClusterParameterGroup build() {
return new software.amazon.awscdk.services.redshift.CfnClusterParameterGroup(
this.scope,
this.id,
this.props.build()
);
}
}
}