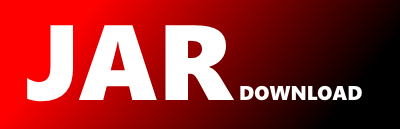
software.amazon.awscdk.services.redshift.CfnEventSubscriptionProps Maven / Gradle / Ivy
Show all versions of redshift Show documentation
package software.amazon.awscdk.services.redshift;
/**
* Properties for defining a `CfnEventSubscription`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.redshift.*;
* CfnEventSubscriptionProps cfnEventSubscriptionProps = CfnEventSubscriptionProps.builder()
* .subscriptionName("subscriptionName")
* // the properties below are optional
* .enabled(false)
* .eventCategories(List.of("eventCategories"))
* .severity("severity")
* .snsTopicArn("snsTopicArn")
* .sourceIds(List.of("sourceIds"))
* .sourceType("sourceType")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.73.0 (build 6faeda3)", date = "2023-01-31T18:36:56.300Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnEventSubscriptionProps")
@software.amazon.jsii.Jsii.Proxy(CfnEventSubscriptionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnEventSubscriptionProps extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the event subscription to be created.
*
* Constraints:
*
*
* - Cannot be null, empty, or blank.
* - Must contain from 1 to 255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getSubscriptionName();
/**
* A boolean value;
*
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEnabled() {
return null;
}
/**
* Specifies the Amazon Redshift event categories to be published by the event notification subscription.
*
* Values: configuration, management, monitoring, security, pending
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getEventCategories() {
return null;
}
/**
* Specifies the Amazon Redshift event severity to be published by the event notification subscription.
*
* Values: ERROR, INFO
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSeverity() {
return null;
}
/**
* The Amazon Resource Name (ARN) of the Amazon SNS topic used to transmit the event notifications.
*
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSnsTopicArn() {
return null;
}
/**
* A list of one or more identifiers of Amazon Redshift source objects.
*
* All of the objects must be of the same type as was specified in the source type parameter. The event subscription will return only events generated by the specified objects. If not specified, then events are returned for all objects within the source type specified.
*
* Example: my-cluster-1, my-cluster-2
*
* Example: my-snapshot-20131010
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getSourceIds() {
return null;
}
/**
* The type of source that will be generating the events.
*
* For example, if you want to be notified of events generated by a cluster, you would set this parameter to cluster. If this value is not specified, events are returned for all Amazon Redshift objects in your AWS account . You must specify a source type in order to specify source IDs.
*
* Valid values: cluster, cluster-parameter-group, cluster-security-group, cluster-snapshot, and scheduled-action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSourceType() {
return null;
}
/**
* A list of tag instances.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnEventSubscriptionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnEventSubscriptionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String subscriptionName;
java.lang.Object enabled;
java.util.List eventCategories;
java.lang.String severity;
java.lang.String snsTopicArn;
java.util.List sourceIds;
java.lang.String sourceType;
java.util.List tags;
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSubscriptionName}
* @param subscriptionName The name of the event subscription to be created. This parameter is required.
* Constraints:
*
*
* - Cannot be null, empty, or blank.
* - Must contain from 1 to 255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subscriptionName(java.lang.String subscriptionName) {
this.subscriptionName = subscriptionName;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getEnabled}
* @param enabled A boolean value;.
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getEnabled}
* @param enabled A boolean value;.
* set to true
to activate the subscription, and set to false
to create the subscription but not activate it.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(software.amazon.awscdk.core.IResolvable enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getEventCategories}
* @param eventCategories Specifies the Amazon Redshift event categories to be published by the event notification subscription.
* Values: configuration, management, monitoring, security, pending
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eventCategories(java.util.List eventCategories) {
this.eventCategories = eventCategories;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSeverity}
* @param severity Specifies the Amazon Redshift event severity to be published by the event notification subscription.
* Values: ERROR, INFO
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder severity(java.lang.String severity) {
this.severity = severity;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSnsTopicArn}
* @param snsTopicArn The Amazon Resource Name (ARN) of the Amazon SNS topic used to transmit the event notifications.
* The ARN is created by Amazon SNS when you create a topic and subscribe to it.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder snsTopicArn(java.lang.String snsTopicArn) {
this.snsTopicArn = snsTopicArn;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSourceIds}
* @param sourceIds A list of one or more identifiers of Amazon Redshift source objects.
* All of the objects must be of the same type as was specified in the source type parameter. The event subscription will return only events generated by the specified objects. If not specified, then events are returned for all objects within the source type specified.
*
* Example: my-cluster-1, my-cluster-2
*
* Example: my-snapshot-20131010
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceIds(java.util.List sourceIds) {
this.sourceIds = sourceIds;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getSourceType}
* @param sourceType The type of source that will be generating the events.
* For example, if you want to be notified of events generated by a cluster, you would set this parameter to cluster. If this value is not specified, events are returned for all Amazon Redshift objects in your AWS account . You must specify a source type in order to specify source IDs.
*
* Valid values: cluster, cluster-parameter-group, cluster-security-group, cluster-snapshot, and scheduled-action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceType(java.lang.String sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Sets the value of {@link CfnEventSubscriptionProps#getTags}
* @param tags A list of tag instances.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnEventSubscriptionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnEventSubscriptionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnEventSubscriptionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnEventSubscriptionProps {
private final java.lang.String subscriptionName;
private final java.lang.Object enabled;
private final java.util.List eventCategories;
private final java.lang.String severity;
private final java.lang.String snsTopicArn;
private final java.util.List sourceIds;
private final java.lang.String sourceType;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.subscriptionName = software.amazon.jsii.Kernel.get(this, "subscriptionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.enabled = software.amazon.jsii.Kernel.get(this, "enabled", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.eventCategories = software.amazon.jsii.Kernel.get(this, "eventCategories", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.severity = software.amazon.jsii.Kernel.get(this, "severity", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.snsTopicArn = software.amazon.jsii.Kernel.get(this, "snsTopicArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.sourceIds = software.amazon.jsii.Kernel.get(this, "sourceIds", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.sourceType = software.amazon.jsii.Kernel.get(this, "sourceType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.subscriptionName = java.util.Objects.requireNonNull(builder.subscriptionName, "subscriptionName is required");
this.enabled = builder.enabled;
this.eventCategories = builder.eventCategories;
this.severity = builder.severity;
this.snsTopicArn = builder.snsTopicArn;
this.sourceIds = builder.sourceIds;
this.sourceType = builder.sourceType;
this.tags = (java.util.List)builder.tags;
}
@Override
public final java.lang.String getSubscriptionName() {
return this.subscriptionName;
}
@Override
public final java.lang.Object getEnabled() {
return this.enabled;
}
@Override
public final java.util.List getEventCategories() {
return this.eventCategories;
}
@Override
public final java.lang.String getSeverity() {
return this.severity;
}
@Override
public final java.lang.String getSnsTopicArn() {
return this.snsTopicArn;
}
@Override
public final java.util.List getSourceIds() {
return this.sourceIds;
}
@Override
public final java.lang.String getSourceType() {
return this.sourceType;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("subscriptionName", om.valueToTree(this.getSubscriptionName()));
if (this.getEnabled() != null) {
data.set("enabled", om.valueToTree(this.getEnabled()));
}
if (this.getEventCategories() != null) {
data.set("eventCategories", om.valueToTree(this.getEventCategories()));
}
if (this.getSeverity() != null) {
data.set("severity", om.valueToTree(this.getSeverity()));
}
if (this.getSnsTopicArn() != null) {
data.set("snsTopicArn", om.valueToTree(this.getSnsTopicArn()));
}
if (this.getSourceIds() != null) {
data.set("sourceIds", om.valueToTree(this.getSourceIds()));
}
if (this.getSourceType() != null) {
data.set("sourceType", om.valueToTree(this.getSourceType()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-redshift.CfnEventSubscriptionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnEventSubscriptionProps.Jsii$Proxy that = (CfnEventSubscriptionProps.Jsii$Proxy) o;
if (!subscriptionName.equals(that.subscriptionName)) return false;
if (this.enabled != null ? !this.enabled.equals(that.enabled) : that.enabled != null) return false;
if (this.eventCategories != null ? !this.eventCategories.equals(that.eventCategories) : that.eventCategories != null) return false;
if (this.severity != null ? !this.severity.equals(that.severity) : that.severity != null) return false;
if (this.snsTopicArn != null ? !this.snsTopicArn.equals(that.snsTopicArn) : that.snsTopicArn != null) return false;
if (this.sourceIds != null ? !this.sourceIds.equals(that.sourceIds) : that.sourceIds != null) return false;
if (this.sourceType != null ? !this.sourceType.equals(that.sourceType) : that.sourceType != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public final int hashCode() {
int result = this.subscriptionName.hashCode();
result = 31 * result + (this.enabled != null ? this.enabled.hashCode() : 0);
result = 31 * result + (this.eventCategories != null ? this.eventCategories.hashCode() : 0);
result = 31 * result + (this.severity != null ? this.severity.hashCode() : 0);
result = 31 * result + (this.snsTopicArn != null ? this.snsTopicArn.hashCode() : 0);
result = 31 * result + (this.sourceIds != null ? this.sourceIds.hashCode() : 0);
result = 31 * result + (this.sourceType != null ? this.sourceType.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}