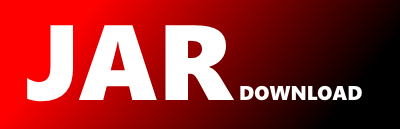
software.amazon.awscdk.services.redshift.CfnScheduledActionProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.redshift;
/**
* Properties for defining a `CfnScheduledAction`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.redshift.*;
* CfnScheduledActionProps cfnScheduledActionProps = CfnScheduledActionProps.builder()
* .scheduledActionName("scheduledActionName")
* // the properties below are optional
* .enable(false)
* .endTime("endTime")
* .iamRole("iamRole")
* .schedule("schedule")
* .scheduledActionDescription("scheduledActionDescription")
* .startTime("startTime")
* .targetAction(ScheduledActionTypeProperty.builder()
* .pauseCluster(PauseClusterMessageProperty.builder()
* .clusterIdentifier("clusterIdentifier")
* .build())
* .resizeCluster(ResizeClusterMessageProperty.builder()
* .clusterIdentifier("clusterIdentifier")
* // the properties below are optional
* .classic(false)
* .clusterType("clusterType")
* .nodeType("nodeType")
* .numberOfNodes(123)
* .build())
* .resumeCluster(ResumeClusterMessageProperty.builder()
* .clusterIdentifier("clusterIdentifier")
* .build())
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-10T17:05:58.316Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.CfnScheduledActionProps")
@software.amazon.jsii.Jsii.Proxy(CfnScheduledActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnScheduledActionProps extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the scheduled action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getScheduledActionName();
/**
* If true, the schedule is enabled.
*
* If false, the scheduled action does not trigger. For more information about state
of the scheduled action, see ScheduledAction
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEnable() {
return null;
}
/**
* The end time in UTC when the schedule is no longer active.
*
* After this time, the scheduled action does not trigger.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getEndTime() {
return null;
}
/**
* The IAM role to assume to run the scheduled action.
*
* This IAM role must have permission to run the Amazon Redshift API operation in the scheduled action. This IAM role must allow the Amazon Redshift scheduler (Principal scheduler.redshift.amazonaws.com) to assume permissions on your behalf. For more information about the IAM role to use with the Amazon Redshift scheduler, see Using Identity-Based Policies for Amazon Redshift in the Amazon Redshift Cluster Management Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getIamRole() {
return null;
}
/**
* The schedule for a one-time (at format) or recurring (cron format) scheduled action.
*
* Schedule invocations must be separated by at least one hour.
*
* Format of at expressions is " at(yyyy-mm-ddThh:mm:ss)
". For example, " at(2016-03-04T17:27:00)
".
*
* Format of cron expressions is " cron(Minutes Hours Day-of-month Month Day-of-week Year)
". For example, " cron(0 10 ? * MON *)
". For more information, see Cron Expressions in the Amazon CloudWatch Events User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSchedule() {
return null;
}
/**
* The description of the scheduled action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getScheduledActionDescription() {
return null;
}
/**
* The start time in UTC when the schedule is active.
*
* Before this time, the scheduled action does not trigger.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getStartTime() {
return null;
}
/**
* A JSON format string of the Amazon Redshift API operation with input parameters.
*
* " {\"ResizeCluster\":{\"NodeType\":\"ds2.8xlarge\",\"ClusterIdentifier\":\"my-test-cluster\",\"NumberOfNodes\":3}}
".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getTargetAction() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnScheduledActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnScheduledActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String scheduledActionName;
java.lang.Object enable;
java.lang.String endTime;
java.lang.String iamRole;
java.lang.String schedule;
java.lang.String scheduledActionDescription;
java.lang.String startTime;
java.lang.Object targetAction;
/**
* Sets the value of {@link CfnScheduledActionProps#getScheduledActionName}
* @param scheduledActionName The name of the scheduled action. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scheduledActionName(java.lang.String scheduledActionName) {
this.scheduledActionName = scheduledActionName;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getEnable}
* @param enable If true, the schedule is enabled.
* If false, the scheduled action does not trigger. For more information about state
of the scheduled action, see ScheduledAction
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enable(java.lang.Boolean enable) {
this.enable = enable;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getEnable}
* @param enable If true, the schedule is enabled.
* If false, the scheduled action does not trigger. For more information about state
of the scheduled action, see ScheduledAction
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enable(software.amazon.awscdk.core.IResolvable enable) {
this.enable = enable;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getEndTime}
* @param endTime The end time in UTC when the schedule is no longer active.
* After this time, the scheduled action does not trigger.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder endTime(java.lang.String endTime) {
this.endTime = endTime;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getIamRole}
* @param iamRole The IAM role to assume to run the scheduled action.
* This IAM role must have permission to run the Amazon Redshift API operation in the scheduled action. This IAM role must allow the Amazon Redshift scheduler (Principal scheduler.redshift.amazonaws.com) to assume permissions on your behalf. For more information about the IAM role to use with the Amazon Redshift scheduler, see Using Identity-Based Policies for Amazon Redshift in the Amazon Redshift Cluster Management Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iamRole(java.lang.String iamRole) {
this.iamRole = iamRole;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getSchedule}
* @param schedule The schedule for a one-time (at format) or recurring (cron format) scheduled action.
* Schedule invocations must be separated by at least one hour.
*
* Format of at expressions is " at(yyyy-mm-ddThh:mm:ss)
". For example, " at(2016-03-04T17:27:00)
".
*
* Format of cron expressions is " cron(Minutes Hours Day-of-month Month Day-of-week Year)
". For example, " cron(0 10 ? * MON *)
". For more information, see Cron Expressions in the Amazon CloudWatch Events User Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder schedule(java.lang.String schedule) {
this.schedule = schedule;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getScheduledActionDescription}
* @param scheduledActionDescription The description of the scheduled action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scheduledActionDescription(java.lang.String scheduledActionDescription) {
this.scheduledActionDescription = scheduledActionDescription;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getStartTime}
* @param startTime The start time in UTC when the schedule is active.
* Before this time, the scheduled action does not trigger.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder startTime(java.lang.String startTime) {
this.startTime = startTime;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getTargetAction}
* @param targetAction A JSON format string of the Amazon Redshift API operation with input parameters.
* " {\"ResizeCluster\":{\"NodeType\":\"ds2.8xlarge\",\"ClusterIdentifier\":\"my-test-cluster\",\"NumberOfNodes\":3}}
".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder targetAction(software.amazon.awscdk.core.IResolvable targetAction) {
this.targetAction = targetAction;
return this;
}
/**
* Sets the value of {@link CfnScheduledActionProps#getTargetAction}
* @param targetAction A JSON format string of the Amazon Redshift API operation with input parameters.
* " {\"ResizeCluster\":{\"NodeType\":\"ds2.8xlarge\",\"ClusterIdentifier\":\"my-test-cluster\",\"NumberOfNodes\":3}}
".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder targetAction(software.amazon.awscdk.services.redshift.CfnScheduledAction.ScheduledActionTypeProperty targetAction) {
this.targetAction = targetAction;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnScheduledActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnScheduledActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnScheduledActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnScheduledActionProps {
private final java.lang.String scheduledActionName;
private final java.lang.Object enable;
private final java.lang.String endTime;
private final java.lang.String iamRole;
private final java.lang.String schedule;
private final java.lang.String scheduledActionDescription;
private final java.lang.String startTime;
private final java.lang.Object targetAction;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.scheduledActionName = software.amazon.jsii.Kernel.get(this, "scheduledActionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.enable = software.amazon.jsii.Kernel.get(this, "enable", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.endTime = software.amazon.jsii.Kernel.get(this, "endTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.iamRole = software.amazon.jsii.Kernel.get(this, "iamRole", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.schedule = software.amazon.jsii.Kernel.get(this, "schedule", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.scheduledActionDescription = software.amazon.jsii.Kernel.get(this, "scheduledActionDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.startTime = software.amazon.jsii.Kernel.get(this, "startTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.targetAction = software.amazon.jsii.Kernel.get(this, "targetAction", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.scheduledActionName = java.util.Objects.requireNonNull(builder.scheduledActionName, "scheduledActionName is required");
this.enable = builder.enable;
this.endTime = builder.endTime;
this.iamRole = builder.iamRole;
this.schedule = builder.schedule;
this.scheduledActionDescription = builder.scheduledActionDescription;
this.startTime = builder.startTime;
this.targetAction = builder.targetAction;
}
@Override
public final java.lang.String getScheduledActionName() {
return this.scheduledActionName;
}
@Override
public final java.lang.Object getEnable() {
return this.enable;
}
@Override
public final java.lang.String getEndTime() {
return this.endTime;
}
@Override
public final java.lang.String getIamRole() {
return this.iamRole;
}
@Override
public final java.lang.String getSchedule() {
return this.schedule;
}
@Override
public final java.lang.String getScheduledActionDescription() {
return this.scheduledActionDescription;
}
@Override
public final java.lang.String getStartTime() {
return this.startTime;
}
@Override
public final java.lang.Object getTargetAction() {
return this.targetAction;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("scheduledActionName", om.valueToTree(this.getScheduledActionName()));
if (this.getEnable() != null) {
data.set("enable", om.valueToTree(this.getEnable()));
}
if (this.getEndTime() != null) {
data.set("endTime", om.valueToTree(this.getEndTime()));
}
if (this.getIamRole() != null) {
data.set("iamRole", om.valueToTree(this.getIamRole()));
}
if (this.getSchedule() != null) {
data.set("schedule", om.valueToTree(this.getSchedule()));
}
if (this.getScheduledActionDescription() != null) {
data.set("scheduledActionDescription", om.valueToTree(this.getScheduledActionDescription()));
}
if (this.getStartTime() != null) {
data.set("startTime", om.valueToTree(this.getStartTime()));
}
if (this.getTargetAction() != null) {
data.set("targetAction", om.valueToTree(this.getTargetAction()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-redshift.CfnScheduledActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnScheduledActionProps.Jsii$Proxy that = (CfnScheduledActionProps.Jsii$Proxy) o;
if (!scheduledActionName.equals(that.scheduledActionName)) return false;
if (this.enable != null ? !this.enable.equals(that.enable) : that.enable != null) return false;
if (this.endTime != null ? !this.endTime.equals(that.endTime) : that.endTime != null) return false;
if (this.iamRole != null ? !this.iamRole.equals(that.iamRole) : that.iamRole != null) return false;
if (this.schedule != null ? !this.schedule.equals(that.schedule) : that.schedule != null) return false;
if (this.scheduledActionDescription != null ? !this.scheduledActionDescription.equals(that.scheduledActionDescription) : that.scheduledActionDescription != null) return false;
if (this.startTime != null ? !this.startTime.equals(that.startTime) : that.startTime != null) return false;
return this.targetAction != null ? this.targetAction.equals(that.targetAction) : that.targetAction == null;
}
@Override
public final int hashCode() {
int result = this.scheduledActionName.hashCode();
result = 31 * result + (this.enable != null ? this.enable.hashCode() : 0);
result = 31 * result + (this.endTime != null ? this.endTime.hashCode() : 0);
result = 31 * result + (this.iamRole != null ? this.iamRole.hashCode() : 0);
result = 31 * result + (this.schedule != null ? this.schedule.hashCode() : 0);
result = 31 * result + (this.scheduledActionDescription != null ? this.scheduledActionDescription.hashCode() : 0);
result = 31 * result + (this.startTime != null ? this.startTime.hashCode() : 0);
result = 31 * result + (this.targetAction != null ? this.targetAction.hashCode() : 0);
return result;
}
}
}