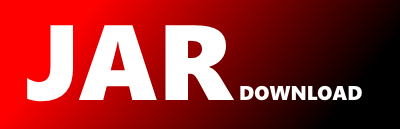
software.amazon.awscdk.services.redshift.Cluster Maven / Gradle / Ivy
package software.amazon.awscdk.services.redshift;
/**
* (experimental) Create a Redshift cluster a given number of nodes.
*
* Example:
*
*
* import software.amazon.awscdk.services.ec2.*;
* Vpc vpc = new Vpc(this, "Vpc");
* Cluster cluster = Cluster.Builder.create(this, "Redshift")
* .masterUser(Login.builder()
* .masterUsername("admin")
* .build())
* .vpc(vpc)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-10T17:05:58.317Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.Cluster")
public class Cluster extends software.amazon.awscdk.core.Resource implements software.amazon.awscdk.services.redshift.ICluster {
protected Cluster(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Cluster(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Cluster(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.ClusterProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Import an existing DatabaseCluster from properties.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param attrs This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.ICluster fromClusterAttributes(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.ClusterAttributes attrs) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.redshift.Cluster.class, "fromClusterAttributes", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.redshift.ICluster.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(attrs, "attrs is required") });
}
/**
* (experimental) Adds the multi user rotation to this cluster.
*
* @param id This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationMultiUser(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.RotationMultiUserOptions options) {
return software.amazon.jsii.Kernel.call(this, "addRotationMultiUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class), new Object[] { java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* (experimental) Adds the single user rotation of the master password to this cluster.
*
* @param automaticallyAfter Specifies the number of days after the previous rotation before Secrets Manager triggers the next automatic rotation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationSingleUser(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration automaticallyAfter) {
return software.amazon.jsii.Kernel.call(this, "addRotationSingleUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class), new Object[] { automaticallyAfter });
}
/**
* (experimental) Adds the single user rotation of the master password to this cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretRotation addRotationSingleUser() {
return software.amazon.jsii.Kernel.call(this, "addRotationSingleUser", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretRotation.class));
}
/**
* (experimental) Renders the secret attachment target specifications.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.secretsmanager.SecretAttachmentTargetProps asSecretAttachmentTarget() {
return software.amazon.jsii.Kernel.call(this, "asSecretAttachmentTarget", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.SecretAttachmentTargetProps.class));
}
/**
* (experimental) The endpoint to use for read/write operations.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.Endpoint getClusterEndpoint() {
return software.amazon.jsii.Kernel.get(this, "clusterEndpoint", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.redshift.Endpoint.class));
}
/**
* (experimental) Identifier of the cluster.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getClusterName() {
return software.amazon.jsii.Kernel.get(this, "clusterName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) Access to the network connections.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.Connections getConnections() {
return software.amazon.jsii.Kernel.get(this, "connections", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.Connections.class));
}
/**
* (experimental) The secret attached to this cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.secretsmanager.ISecret getSecret() {
return software.amazon.jsii.Kernel.get(this, "secret", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.secretsmanager.ISecret.class));
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.redshift.Cluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.redshift.ClusterProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.redshift.ClusterProps.Builder();
}
/**
* (experimental) Username and password for the administrative user.
*
* @return {@code this}
* @param masterUser Username and password for the administrative user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder masterUser(final software.amazon.awscdk.services.redshift.Login masterUser) {
this.props.masterUser(masterUser);
return this;
}
/**
* (experimental) The VPC to place the cluster in.
*
* @return {@code this}
* @param vpc The VPC to place the cluster in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(final software.amazon.awscdk.services.ec2.IVpc vpc) {
this.props.vpc(vpc);
return this;
}
/**
* (experimental) An optional identifier for the cluster.
*
* Default: - A name is automatically generated.
*
* @return {@code this}
* @param clusterName An optional identifier for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterName(final java.lang.String clusterName) {
this.props.clusterName(clusterName);
return this;
}
/**
* (experimental) Settings for the individual instances that are launched.
*
* Default: {@link ClusterType.MULTI_NODE}
*
* @return {@code this}
* @param clusterType Settings for the individual instances that are launched. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterType(final software.amazon.awscdk.services.redshift.ClusterType clusterType) {
this.props.clusterType(clusterType);
return this;
}
/**
* (experimental) Name of a database which is automatically created inside the cluster.
*
* Default: - default_db
*
* @return {@code this}
* @param defaultDatabaseName Name of a database which is automatically created inside the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder defaultDatabaseName(final java.lang.String defaultDatabaseName) {
this.props.defaultDatabaseName(defaultDatabaseName);
return this;
}
/**
* (experimental) Whether to enable encryption of data at rest in the cluster.
*
* Default: true
*
* @return {@code this}
* @param encrypted Whether to enable encryption of data at rest in the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encrypted(final java.lang.Boolean encrypted) {
this.props.encrypted(encrypted);
return this;
}
/**
* (experimental) The KMS key to use for encryption of data at rest.
*
* Default: - AWS-managed key, if encryption at rest is enabled
*
* @return {@code this}
* @param encryptionKey The KMS key to use for encryption of data at rest. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encryptionKey(final software.amazon.awscdk.services.kms.IKey encryptionKey) {
this.props.encryptionKey(encryptionKey);
return this;
}
/**
* (experimental) Bucket to send logs to.
*
* Logging information includes queries and connection attempts, for the specified Amazon Redshift cluster.
*
* Default: - No Logs
*
* @return {@code this}
* @param loggingBucket Bucket to send logs to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder loggingBucket(final software.amazon.awscdk.services.s3.IBucket loggingBucket) {
this.props.loggingBucket(loggingBucket);
return this;
}
/**
* (experimental) Prefix used for logging.
*
* Default: - no prefix
*
* @return {@code this}
* @param loggingKeyPrefix Prefix used for logging. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder loggingKeyPrefix(final java.lang.String loggingKeyPrefix) {
this.props.loggingKeyPrefix(loggingKeyPrefix);
return this;
}
/**
* (experimental) The node type to be provisioned for the cluster.
*
* Default: {@link NodeType.DC2_LARGE}
*
* @return {@code this}
* @param nodeType The node type to be provisioned for the cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder nodeType(final software.amazon.awscdk.services.redshift.NodeType nodeType) {
this.props.nodeType(nodeType);
return this;
}
/**
* (experimental) Number of compute nodes in the cluster. Only specify this property for multi-node clusters.
*
* Value must be at least 2 and no more than 100.
*
* Default: - 2 if `clusterType` is ClusterType.MULTI_NODE, undefined otherwise
*
* @return {@code this}
* @param numberOfNodes Number of compute nodes in the cluster. Only specify this property for multi-node clusters. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder numberOfNodes(final java.lang.Number numberOfNodes) {
this.props.numberOfNodes(numberOfNodes);
return this;
}
/**
* (experimental) Additional parameters to pass to the database engine https://docs.aws.amazon.com/redshift/latest/mgmt/working-with-parameter-groups.html.
*
* Default: - No parameter group.
*
* @return {@code this}
* @param parameterGroup Additional parameters to pass to the database engine https://docs.aws.amazon.com/redshift/latest/mgmt/working-with-parameter-groups.html. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder parameterGroup(final software.amazon.awscdk.services.redshift.IClusterParameterGroup parameterGroup) {
this.props.parameterGroup(parameterGroup);
return this;
}
/**
* (experimental) What port to listen on.
*
* Default: - The default for the engine is used.
*
* @return {@code this}
* @param port What port to listen on. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder port(final java.lang.Number port) {
this.props.port(port);
return this;
}
/**
* (experimental) A preferred maintenance window day/time range. Should be specified as a range ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC).
*
* Example: 'Sun:23:45-Mon:00:15'
*
* Default: - 30-minute window selected at random from an 8-hour block of time for
* each AWS Region, occurring on a random day of the week.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/USER_UpgradeDBInstance.Maintenance.html#Concepts.DBMaintenance
* @param preferredMaintenanceWindow A preferred maintenance window day/time range. Should be specified as a range ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder preferredMaintenanceWindow(final java.lang.String preferredMaintenanceWindow) {
this.props.preferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
* (experimental) Whether to make cluster publicly accessible.
*
* Default: false
*
* @return {@code this}
* @param publiclyAccessible Whether to make cluster publicly accessible. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder publiclyAccessible(final java.lang.Boolean publiclyAccessible) {
this.props.publiclyAccessible(publiclyAccessible);
return this;
}
/**
* (experimental) The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update.
*
* Default: RemovalPolicy.RETAIN
*
* @return {@code this}
* @param removalPolicy The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder removalPolicy(final software.amazon.awscdk.core.RemovalPolicy removalPolicy) {
this.props.removalPolicy(removalPolicy);
return this;
}
/**
* (experimental) A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services.
*
* Specify a maximum of 10 roles.
*
* Default: - No role is attached to the cluster.
*
* @return {@code this}
* @param roles A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder roles(final java.util.List extends software.amazon.awscdk.services.iam.IRole> roles) {
this.props.roles(roles);
return this;
}
/**
* (experimental) Security group.
*
* Default: - a new security group is created.
*
* @return {@code this}
* @param securityGroups Security group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder securityGroups(final java.util.List extends software.amazon.awscdk.services.ec2.ISecurityGroup> securityGroups) {
this.props.securityGroups(securityGroups);
return this;
}
/**
* (experimental) A cluster subnet group to use with this cluster.
*
* Default: - a new subnet group will be created.
*
* @return {@code this}
* @param subnetGroup A cluster subnet group to use with this cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subnetGroup(final software.amazon.awscdk.services.redshift.IClusterSubnetGroup subnetGroup) {
this.props.subnetGroup(subnetGroup);
return this;
}
/**
* (experimental) Where to place the instances within the VPC.
*
* Default: - private subnets
*
* @return {@code this}
* @param vpcSubnets Where to place the instances within the VPC. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcSubnets(final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.props.vpcSubnets(vpcSubnets);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.redshift.Cluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.redshift.Cluster build() {
return new software.amazon.awscdk.services.redshift.Cluster(
this.scope,
this.id,
this.props.build()
);
}
}
}