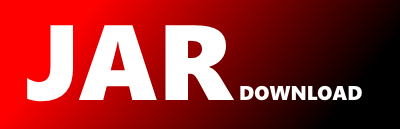
software.amazon.awscdk.services.redshift.ClusterProps Maven / Gradle / Ivy
Show all versions of redshift Show documentation
package software.amazon.awscdk.services.redshift;
/**
* Properties for a new database cluster.
*
* EXPERIMENTAL
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.6.0 (build 248e75b)", date = "2020-06-04T00:02:28.471Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.redshift.$Module.class, fqn = "@aws-cdk/aws-redshift.ClusterProps")
@software.amazon.jsii.Jsii.Proxy(ClusterProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface ClusterProps extends software.amazon.jsii.JsiiSerializable {
/**
* Username and password for the administrative user.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.redshift.Login getMasterUser();
/**
* The VPC to place the cluster in.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.IVpc getVpc();
/**
* An optional identifier for the cluster.
*
* Default: - A name is automatically generated.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getClusterName() {
return null;
}
/**
* Settings for the individual instances that are launched.
*
* Default: {@link ClusterType.MULTI_NODE}
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.redshift.ClusterType getClusterType() {
return null;
}
/**
* Name of a database which is automatically created inside the cluster.
*
* Default: - default_db
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getDefaultDatabaseName() {
return null;
}
/**
* Whether to enable encryption of data at rest in the cluster.
*
* Default: true
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getEncrypted() {
return null;
}
/**
* The KMS key to use for encryption of data at rest.
*
* Default: - AWS-managed key, if encryption at rest is enabled
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.kms.IKey getEncryptionKey() {
return null;
}
/**
* Bucket to send logs to.
*
* Logging information includes queries and connection attempts, for the specified Amazon Redshift cluster.
*
* Default: - No Logs
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.s3.IBucket getLoggingBucket() {
return null;
}
/**
* Prefix used for logging.
*
* Default: - no prefix
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getLoggingKeyPrefix() {
return null;
}
/**
* The node type to be provisioned for the cluster.
*
* Default: {@link NodeType.DC2_LARGE}
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.redshift.NodeType getNodeType() {
return null;
}
/**
* Number of compute nodes in the cluster.
*
* Value must be at least 1 and no more than 100.
*
* Default: 1
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getNumberOfNodes() {
return null;
}
/**
* Additional parameters to pass to the database engine https://docs.aws.amazon.com/redshift/latest/mgmt/working-with-parameter-groups.html.
*
* Default: - No parameter group.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.redshift.IClusterParameterGroup getParameterGroup() {
return null;
}
/**
* What port to listen on.
*
* Default: - The default for the engine is used.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getPort() {
return null;
}
/**
* A preferred maintenance window day/time range. Should be specified as a range ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC).
*
* Example: 'Sun:23:45-Mon:00:15'
*
* Default: - 30-minute window selected at random from an 8-hour block of time for
* each AWS Region, occurring on a random day of the week.
*
* EXPERIMENTAL
*
* @see https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/USER_UpgradeDBInstance.Maintenance.html#Concepts.DBMaintenance
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getPreferredMaintenanceWindow() {
return null;
}
/**
* The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update.
*
* Default: RemovalPolicy.RETAIN
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.RemovalPolicy getRemovalPolicy() {
return null;
}
/**
* A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services.
*
* Specify a maximum of 10 roles.
*
* Default: - No role is attached to the cluster.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.util.List getRoles() {
return null;
}
/**
* Security group.
*
* Default: a new security group is created.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.util.List getSecurityGroups() {
return null;
}
/**
* Where to place the instances within the VPC.
*
* Default: private subnets
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return null;
}
/**
* @return a {@link Builder} of {@link ClusterProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ClusterProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
private software.amazon.awscdk.services.redshift.Login masterUser;
private software.amazon.awscdk.services.ec2.IVpc vpc;
private java.lang.String clusterName;
private software.amazon.awscdk.services.redshift.ClusterType clusterType;
private java.lang.String defaultDatabaseName;
private java.lang.Boolean encrypted;
private software.amazon.awscdk.services.kms.IKey encryptionKey;
private software.amazon.awscdk.services.s3.IBucket loggingBucket;
private java.lang.String loggingKeyPrefix;
private software.amazon.awscdk.services.redshift.NodeType nodeType;
private java.lang.Number numberOfNodes;
private software.amazon.awscdk.services.redshift.IClusterParameterGroup parameterGroup;
private java.lang.Number port;
private java.lang.String preferredMaintenanceWindow;
private software.amazon.awscdk.core.RemovalPolicy removalPolicy;
private java.util.List roles;
private java.util.List securityGroups;
private software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Sets the value of {@link ClusterProps#getMasterUser}
* @param masterUser Username and password for the administrative user. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder masterUser(software.amazon.awscdk.services.redshift.Login masterUser) {
this.masterUser = masterUser;
return this;
}
/**
* Sets the value of {@link ClusterProps#getVpc}
* @param vpc The VPC to place the cluster in. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(software.amazon.awscdk.services.ec2.IVpc vpc) {
this.vpc = vpc;
return this;
}
/**
* Sets the value of {@link ClusterProps#getClusterName}
* @param clusterName An optional identifier for the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Sets the value of {@link ClusterProps#getClusterType}
* @param clusterType Settings for the individual instances that are launched.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clusterType(software.amazon.awscdk.services.redshift.ClusterType clusterType) {
this.clusterType = clusterType;
return this;
}
/**
* Sets the value of {@link ClusterProps#getDefaultDatabaseName}
* @param defaultDatabaseName Name of a database which is automatically created inside the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder defaultDatabaseName(java.lang.String defaultDatabaseName) {
this.defaultDatabaseName = defaultDatabaseName;
return this;
}
/**
* Sets the value of {@link ClusterProps#getEncrypted}
* @param encrypted Whether to enable encryption of data at rest in the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encrypted(java.lang.Boolean encrypted) {
this.encrypted = encrypted;
return this;
}
/**
* Sets the value of {@link ClusterProps#getEncryptionKey}
* @param encryptionKey The KMS key to use for encryption of data at rest.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encryptionKey(software.amazon.awscdk.services.kms.IKey encryptionKey) {
this.encryptionKey = encryptionKey;
return this;
}
/**
* Sets the value of {@link ClusterProps#getLoggingBucket}
* @param loggingBucket Bucket to send logs to.
* Logging information includes queries and connection attempts, for the specified Amazon Redshift cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder loggingBucket(software.amazon.awscdk.services.s3.IBucket loggingBucket) {
this.loggingBucket = loggingBucket;
return this;
}
/**
* Sets the value of {@link ClusterProps#getLoggingKeyPrefix}
* @param loggingKeyPrefix Prefix used for logging.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder loggingKeyPrefix(java.lang.String loggingKeyPrefix) {
this.loggingKeyPrefix = loggingKeyPrefix;
return this;
}
/**
* Sets the value of {@link ClusterProps#getNodeType}
* @param nodeType The node type to be provisioned for the cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder nodeType(software.amazon.awscdk.services.redshift.NodeType nodeType) {
this.nodeType = nodeType;
return this;
}
/**
* Sets the value of {@link ClusterProps#getNumberOfNodes}
* @param numberOfNodes Number of compute nodes in the cluster.
* Value must be at least 1 and no more than 100.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder numberOfNodes(java.lang.Number numberOfNodes) {
this.numberOfNodes = numberOfNodes;
return this;
}
/**
* Sets the value of {@link ClusterProps#getParameterGroup}
* @param parameterGroup Additional parameters to pass to the database engine https://docs.aws.amazon.com/redshift/latest/mgmt/working-with-parameter-groups.html.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder parameterGroup(software.amazon.awscdk.services.redshift.IClusterParameterGroup parameterGroup) {
this.parameterGroup = parameterGroup;
return this;
}
/**
* Sets the value of {@link ClusterProps#getPort}
* @param port What port to listen on.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link ClusterProps#getPreferredMaintenanceWindow}
* @param preferredMaintenanceWindow A preferred maintenance window day/time range. Should be specified as a range ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC).
* Example: 'Sun:23:45-Mon:00:15'
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder preferredMaintenanceWindow(java.lang.String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
/**
* Sets the value of {@link ClusterProps#getRemovalPolicy}
* @param removalPolicy The removal policy to apply when the cluster and its instances are removed from the stack or replaced during an update.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder removalPolicy(software.amazon.awscdk.core.RemovalPolicy removalPolicy) {
this.removalPolicy = removalPolicy;
return this;
}
/**
* Sets the value of {@link ClusterProps#getRoles}
* @param roles A list of AWS Identity and Access Management (IAM) role that can be used by the cluster to access other AWS services.
* Specify a maximum of 10 roles.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder roles(java.util.List roles) {
this.roles = roles;
return this;
}
/**
* Sets the value of {@link ClusterProps#getSecurityGroups}
* @param securityGroups Security group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder securityGroups(java.util.List securityGroups) {
this.securityGroups = securityGroups;
return this;
}
/**
* Sets the value of {@link ClusterProps#getVpcSubnets}
* @param vpcSubnets Where to place the instances within the VPC.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ClusterProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public ClusterProps build() {
return new Jsii$Proxy(masterUser, vpc, clusterName, clusterType, defaultDatabaseName, encrypted, encryptionKey, loggingBucket, loggingKeyPrefix, nodeType, numberOfNodes, parameterGroup, port, preferredMaintenanceWindow, removalPolicy, roles, securityGroups, vpcSubnets);
}
}
/**
* An implementation for {@link ClusterProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ClusterProps {
private final software.amazon.awscdk.services.redshift.Login masterUser;
private final software.amazon.awscdk.services.ec2.IVpc vpc;
private final java.lang.String clusterName;
private final software.amazon.awscdk.services.redshift.ClusterType clusterType;
private final java.lang.String defaultDatabaseName;
private final java.lang.Boolean encrypted;
private final software.amazon.awscdk.services.kms.IKey encryptionKey;
private final software.amazon.awscdk.services.s3.IBucket loggingBucket;
private final java.lang.String loggingKeyPrefix;
private final software.amazon.awscdk.services.redshift.NodeType nodeType;
private final java.lang.Number numberOfNodes;
private final software.amazon.awscdk.services.redshift.IClusterParameterGroup parameterGroup;
private final java.lang.Number port;
private final java.lang.String preferredMaintenanceWindow;
private final software.amazon.awscdk.core.RemovalPolicy removalPolicy;
private final java.util.List roles;
private final java.util.List securityGroups;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.masterUser = this.jsiiGet("masterUser", software.amazon.awscdk.services.redshift.Login.class);
this.vpc = this.jsiiGet("vpc", software.amazon.awscdk.services.ec2.IVpc.class);
this.clusterName = this.jsiiGet("clusterName", java.lang.String.class);
this.clusterType = this.jsiiGet("clusterType", software.amazon.awscdk.services.redshift.ClusterType.class);
this.defaultDatabaseName = this.jsiiGet("defaultDatabaseName", java.lang.String.class);
this.encrypted = this.jsiiGet("encrypted", java.lang.Boolean.class);
this.encryptionKey = this.jsiiGet("encryptionKey", software.amazon.awscdk.services.kms.IKey.class);
this.loggingBucket = this.jsiiGet("loggingBucket", software.amazon.awscdk.services.s3.IBucket.class);
this.loggingKeyPrefix = this.jsiiGet("loggingKeyPrefix", java.lang.String.class);
this.nodeType = this.jsiiGet("nodeType", software.amazon.awscdk.services.redshift.NodeType.class);
this.numberOfNodes = this.jsiiGet("numberOfNodes", java.lang.Number.class);
this.parameterGroup = this.jsiiGet("parameterGroup", software.amazon.awscdk.services.redshift.IClusterParameterGroup.class);
this.port = this.jsiiGet("port", java.lang.Number.class);
this.preferredMaintenanceWindow = this.jsiiGet("preferredMaintenanceWindow", java.lang.String.class);
this.removalPolicy = this.jsiiGet("removalPolicy", software.amazon.awscdk.core.RemovalPolicy.class);
this.roles = this.jsiiGet("roles", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class)));
this.securityGroups = this.jsiiGet("securityGroups", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.ISecurityGroup.class)));
this.vpcSubnets = this.jsiiGet("vpcSubnets", software.amazon.awscdk.services.ec2.SubnetSelection.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final software.amazon.awscdk.services.redshift.Login masterUser, final software.amazon.awscdk.services.ec2.IVpc vpc, final java.lang.String clusterName, final software.amazon.awscdk.services.redshift.ClusterType clusterType, final java.lang.String defaultDatabaseName, final java.lang.Boolean encrypted, final software.amazon.awscdk.services.kms.IKey encryptionKey, final software.amazon.awscdk.services.s3.IBucket loggingBucket, final java.lang.String loggingKeyPrefix, final software.amazon.awscdk.services.redshift.NodeType nodeType, final java.lang.Number numberOfNodes, final software.amazon.awscdk.services.redshift.IClusterParameterGroup parameterGroup, final java.lang.Number port, final java.lang.String preferredMaintenanceWindow, final software.amazon.awscdk.core.RemovalPolicy removalPolicy, final java.util.List roles, final java.util.List securityGroups, final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.masterUser = java.util.Objects.requireNonNull(masterUser, "masterUser is required");
this.vpc = java.util.Objects.requireNonNull(vpc, "vpc is required");
this.clusterName = clusterName;
this.clusterType = clusterType;
this.defaultDatabaseName = defaultDatabaseName;
this.encrypted = encrypted;
this.encryptionKey = encryptionKey;
this.loggingBucket = loggingBucket;
this.loggingKeyPrefix = loggingKeyPrefix;
this.nodeType = nodeType;
this.numberOfNodes = numberOfNodes;
this.parameterGroup = parameterGroup;
this.port = port;
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
this.removalPolicy = removalPolicy;
this.roles = roles;
this.securityGroups = securityGroups;
this.vpcSubnets = vpcSubnets;
}
@Override
public software.amazon.awscdk.services.redshift.Login getMasterUser() {
return this.masterUser;
}
@Override
public software.amazon.awscdk.services.ec2.IVpc getVpc() {
return this.vpc;
}
@Override
public java.lang.String getClusterName() {
return this.clusterName;
}
@Override
public software.amazon.awscdk.services.redshift.ClusterType getClusterType() {
return this.clusterType;
}
@Override
public java.lang.String getDefaultDatabaseName() {
return this.defaultDatabaseName;
}
@Override
public java.lang.Boolean getEncrypted() {
return this.encrypted;
}
@Override
public software.amazon.awscdk.services.kms.IKey getEncryptionKey() {
return this.encryptionKey;
}
@Override
public software.amazon.awscdk.services.s3.IBucket getLoggingBucket() {
return this.loggingBucket;
}
@Override
public java.lang.String getLoggingKeyPrefix() {
return this.loggingKeyPrefix;
}
@Override
public software.amazon.awscdk.services.redshift.NodeType getNodeType() {
return this.nodeType;
}
@Override
public java.lang.Number getNumberOfNodes() {
return this.numberOfNodes;
}
@Override
public software.amazon.awscdk.services.redshift.IClusterParameterGroup getParameterGroup() {
return this.parameterGroup;
}
@Override
public java.lang.Number getPort() {
return this.port;
}
@Override
public java.lang.String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
@Override
public software.amazon.awscdk.core.RemovalPolicy getRemovalPolicy() {
return this.removalPolicy;
}
@Override
public java.util.List getRoles() {
return this.roles;
}
@Override
public java.util.List getSecurityGroups() {
return this.securityGroups;
}
@Override
public software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("masterUser", om.valueToTree(this.getMasterUser()));
data.set("vpc", om.valueToTree(this.getVpc()));
if (this.getClusterName() != null) {
data.set("clusterName", om.valueToTree(this.getClusterName()));
}
if (this.getClusterType() != null) {
data.set("clusterType", om.valueToTree(this.getClusterType()));
}
if (this.getDefaultDatabaseName() != null) {
data.set("defaultDatabaseName", om.valueToTree(this.getDefaultDatabaseName()));
}
if (this.getEncrypted() != null) {
data.set("encrypted", om.valueToTree(this.getEncrypted()));
}
if (this.getEncryptionKey() != null) {
data.set("encryptionKey", om.valueToTree(this.getEncryptionKey()));
}
if (this.getLoggingBucket() != null) {
data.set("loggingBucket", om.valueToTree(this.getLoggingBucket()));
}
if (this.getLoggingKeyPrefix() != null) {
data.set("loggingKeyPrefix", om.valueToTree(this.getLoggingKeyPrefix()));
}
if (this.getNodeType() != null) {
data.set("nodeType", om.valueToTree(this.getNodeType()));
}
if (this.getNumberOfNodes() != null) {
data.set("numberOfNodes", om.valueToTree(this.getNumberOfNodes()));
}
if (this.getParameterGroup() != null) {
data.set("parameterGroup", om.valueToTree(this.getParameterGroup()));
}
if (this.getPort() != null) {
data.set("port", om.valueToTree(this.getPort()));
}
if (this.getPreferredMaintenanceWindow() != null) {
data.set("preferredMaintenanceWindow", om.valueToTree(this.getPreferredMaintenanceWindow()));
}
if (this.getRemovalPolicy() != null) {
data.set("removalPolicy", om.valueToTree(this.getRemovalPolicy()));
}
if (this.getRoles() != null) {
data.set("roles", om.valueToTree(this.getRoles()));
}
if (this.getSecurityGroups() != null) {
data.set("securityGroups", om.valueToTree(this.getSecurityGroups()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-redshift.ClusterProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ClusterProps.Jsii$Proxy that = (ClusterProps.Jsii$Proxy) o;
if (!masterUser.equals(that.masterUser)) return false;
if (!vpc.equals(that.vpc)) return false;
if (this.clusterName != null ? !this.clusterName.equals(that.clusterName) : that.clusterName != null) return false;
if (this.clusterType != null ? !this.clusterType.equals(that.clusterType) : that.clusterType != null) return false;
if (this.defaultDatabaseName != null ? !this.defaultDatabaseName.equals(that.defaultDatabaseName) : that.defaultDatabaseName != null) return false;
if (this.encrypted != null ? !this.encrypted.equals(that.encrypted) : that.encrypted != null) return false;
if (this.encryptionKey != null ? !this.encryptionKey.equals(that.encryptionKey) : that.encryptionKey != null) return false;
if (this.loggingBucket != null ? !this.loggingBucket.equals(that.loggingBucket) : that.loggingBucket != null) return false;
if (this.loggingKeyPrefix != null ? !this.loggingKeyPrefix.equals(that.loggingKeyPrefix) : that.loggingKeyPrefix != null) return false;
if (this.nodeType != null ? !this.nodeType.equals(that.nodeType) : that.nodeType != null) return false;
if (this.numberOfNodes != null ? !this.numberOfNodes.equals(that.numberOfNodes) : that.numberOfNodes != null) return false;
if (this.parameterGroup != null ? !this.parameterGroup.equals(that.parameterGroup) : that.parameterGroup != null) return false;
if (this.port != null ? !this.port.equals(that.port) : that.port != null) return false;
if (this.preferredMaintenanceWindow != null ? !this.preferredMaintenanceWindow.equals(that.preferredMaintenanceWindow) : that.preferredMaintenanceWindow != null) return false;
if (this.removalPolicy != null ? !this.removalPolicy.equals(that.removalPolicy) : that.removalPolicy != null) return false;
if (this.roles != null ? !this.roles.equals(that.roles) : that.roles != null) return false;
if (this.securityGroups != null ? !this.securityGroups.equals(that.securityGroups) : that.securityGroups != null) return false;
return this.vpcSubnets != null ? this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets == null;
}
@Override
public int hashCode() {
int result = this.masterUser.hashCode();
result = 31 * result + (this.vpc.hashCode());
result = 31 * result + (this.clusterName != null ? this.clusterName.hashCode() : 0);
result = 31 * result + (this.clusterType != null ? this.clusterType.hashCode() : 0);
result = 31 * result + (this.defaultDatabaseName != null ? this.defaultDatabaseName.hashCode() : 0);
result = 31 * result + (this.encrypted != null ? this.encrypted.hashCode() : 0);
result = 31 * result + (this.encryptionKey != null ? this.encryptionKey.hashCode() : 0);
result = 31 * result + (this.loggingBucket != null ? this.loggingBucket.hashCode() : 0);
result = 31 * result + (this.loggingKeyPrefix != null ? this.loggingKeyPrefix.hashCode() : 0);
result = 31 * result + (this.nodeType != null ? this.nodeType.hashCode() : 0);
result = 31 * result + (this.numberOfNodes != null ? this.numberOfNodes.hashCode() : 0);
result = 31 * result + (this.parameterGroup != null ? this.parameterGroup.hashCode() : 0);
result = 31 * result + (this.port != null ? this.port.hashCode() : 0);
result = 31 * result + (this.preferredMaintenanceWindow != null ? this.preferredMaintenanceWindow.hashCode() : 0);
result = 31 * result + (this.removalPolicy != null ? this.removalPolicy.hashCode() : 0);
result = 31 * result + (this.roles != null ? this.roles.hashCode() : 0);
result = 31 * result + (this.securityGroups != null ? this.securityGroups.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
return result;
}
}
}