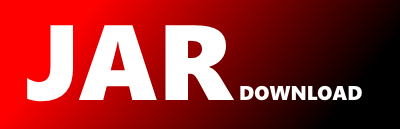
software.amazon.awscdk.services.s3.assets.Asset Maven / Gradle / Ivy
Show all versions of s3-assets Show documentation
package software.amazon.awscdk.services.s3.assets;
/**
* An asset represents a local file or directory, which is automatically uploaded to S3 and then can be referenced within a CDK application.
*
* Example:
*
*
* import software.amazon.awscdk.services.s3.assets.*;
* Cluster cluster;
* Asset chartAsset = Asset.Builder.create(this, "ChartAsset")
* .path("/path/to/asset")
* .build();
* cluster.addHelmChart("test-chart", HelmChartOptions.builder()
* .chartAsset(chartAsset)
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.63.2 (build a8a8833)", date = "2022-08-09T19:16:36.505Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.s3.assets.$Module.class, fqn = "@aws-cdk/aws-s3-assets.Asset")
public class Asset extends software.amazon.awscdk.core.Construct implements software.amazon.awscdk.core.IAsset {
protected Asset(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Asset(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Asset(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.s3.assets.AssetProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Adds CloudFormation template metadata to the specified resource with information that indicates which resource property is mapped to this local asset.
*
* This can be used by tools such as SAM CLI to provide local
* experience such as local invocation and debugging of Lambda functions.
*
* Asset metadata will only be included if the stack is synthesized with the
* "aws:cdk:enable-asset-metadata" context key defined, which is the default
* behavior when synthesizing via the CDK Toolkit.
*
* @see https://github.com/aws/aws-cdk/issues/1432
* @param resource The CloudFormation resource which is using this asset [disable-awslint:ref-via-interface]. This parameter is required.
* @param resourceProperty The property name where this asset is referenced (e.g. "Code" for AWS::Lambda::Function). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addResourceMetadata(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.CfnResource resource, final @org.jetbrains.annotations.NotNull java.lang.String resourceProperty) {
software.amazon.jsii.Kernel.call(this, "addResourceMetadata", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(resource, "resource is required"), java.util.Objects.requireNonNull(resourceProperty, "resourceProperty is required") });
}
/**
* Grants read permissions to the principal on the assets bucket.
*
* @param grantee This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void grantRead(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee) {
software.amazon.jsii.Kernel.call(this, "grantRead", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(grantee, "grantee is required") });
}
/**
* A hash of this asset, which is available at construction time.
*
* As this is a plain string, it
* can be used in construct IDs in order to enforce creation of a new resource when the content
* hash has changed.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAssetHash() {
return software.amazon.jsii.Kernel.get(this, "assetHash", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The path to the asset, relative to the current Cloud Assembly.
*
* If asset staging is disabled, this will just be the original path.
* If asset staging is enabled it will be the staged path.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAssetPath() {
return software.amazon.jsii.Kernel.get(this, "assetPath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The S3 bucket in which this asset resides.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.s3.IBucket getBucket() {
return software.amazon.jsii.Kernel.get(this, "bucket", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.s3.IBucket.class));
}
/**
* Attribute which represents the S3 HTTP URL of this asset.
*
* For example, https://s3.us-west-1.amazonaws.com/bucket/key
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getHttpUrl() {
return software.amazon.jsii.Kernel.get(this, "httpUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Indicates if this asset is a single file.
*
* Allows constructs to ensure that the
* correct file type was used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Boolean getIsFile() {
return software.amazon.jsii.Kernel.get(this, "isFile", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
* Indicates if this asset is a zip archive.
*
* Allows constructs to ensure that the
* correct file type was used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Boolean getIsZipArchive() {
return software.amazon.jsii.Kernel.get(this, "isZipArchive", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
* Attribute that represents the name of the bucket this asset exists in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getS3BucketName() {
return software.amazon.jsii.Kernel.get(this, "s3BucketName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Attribute which represents the S3 object key of this asset.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getS3ObjectKey() {
return software.amazon.jsii.Kernel.get(this, "s3ObjectKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Attribute which represents the S3 URL of this asset.
*
* For example, s3://bucket/key
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getS3ObjectUrl() {
return software.amazon.jsii.Kernel.get(this, "s3ObjectUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (deprecated) Attribute which represents the S3 URL of this asset.
*
* @deprecated use `httpUrl`
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public @org.jetbrains.annotations.NotNull java.lang.String getS3Url() {
return software.amazon.jsii.Kernel.get(this, "s3Url", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (deprecated) A cryptographic hash of the asset.
*
* @deprecated see `assetHash`
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public @org.jetbrains.annotations.NotNull java.lang.String getSourceHash() {
return software.amazon.jsii.Kernel.get(this, "sourceHash", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.s3.assets.Asset}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.s3.assets.AssetProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.s3.assets.AssetProps.Builder();
}
/**
* Glob patterns to exclude from the copy.
*
* Default: - nothing is excluded
*
* @return {@code this}
* @param exclude Glob patterns to exclude from the copy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder exclude(final java.util.List exclude) {
this.props.exclude(exclude);
return this;
}
/**
* (deprecated) A strategy for how to handle symlinks.
*
* Default: Never
*
* @return {@code this}
* @deprecated use `followSymlinks` instead
* @param follow A strategy for how to handle symlinks. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder follow(final software.amazon.awscdk.assets.FollowMode follow) {
this.props.follow(follow);
return this;
}
/**
* The ignore behavior to use for exclude patterns.
*
* Default: IgnoreMode.GLOB
*
* @return {@code this}
* @param ignoreMode The ignore behavior to use for exclude patterns. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ignoreMode(final software.amazon.awscdk.core.IgnoreMode ignoreMode) {
this.props.ignoreMode(ignoreMode);
return this;
}
/**
* A strategy for how to handle symlinks.
*
* Default: SymlinkFollowMode.NEVER
*
* @return {@code this}
* @param followSymlinks A strategy for how to handle symlinks. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder followSymlinks(final software.amazon.awscdk.core.SymlinkFollowMode followSymlinks) {
this.props.followSymlinks(followSymlinks);
return this;
}
/**
* Specify a custom hash for this asset.
*
* If assetHashType
is set it must
* be set to AssetHashType.CUSTOM
. For consistency, this custom hash will
* be SHA256 hashed and encoded as hex. The resulting hash will be the asset
* hash.
*
* NOTE: the hash is used in order to identify a specific revision of the asset, and
* used for optimizing and caching deployment activities related to this asset such as
* packaging, uploading to Amazon S3, etc. If you chose to customize the hash, you will
* need to make sure it is updated every time the asset changes, or otherwise it is
* possible that some deployments will not be invalidated.
*
* Default: - based on `assetHashType`
*
* @return {@code this}
* @param assetHash Specify a custom hash for this asset. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assetHash(final java.lang.String assetHash) {
this.props.assetHash(assetHash);
return this;
}
/**
* Specifies the type of hash to calculate for this asset.
*
* If assetHash
is configured, this option must be undefined
or
* AssetHashType.CUSTOM
.
*
* Default: - the default is `AssetHashType.SOURCE`, but if `assetHash` is
* explicitly specified this value defaults to `AssetHashType.CUSTOM`.
*
* @return {@code this}
* @param assetHashType Specifies the type of hash to calculate for this asset. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assetHashType(final software.amazon.awscdk.core.AssetHashType assetHashType) {
this.props.assetHashType(assetHashType);
return this;
}
/**
* Bundle the asset by executing a command in a Docker container or a custom bundling provider.
*
* The asset path will be mounted at /asset-input
. The Docker
* container is responsible for putting content at /asset-output
.
* The content at /asset-output
will be zipped and used as the
* final asset.
*
* Default: - uploaded as-is to S3 if the asset is a regular file or a .zip file,
* archived into a .zip file and uploaded to S3 otherwise
*
* @return {@code this}
* @param bundling Bundle the asset by executing a command in a Docker container or a custom bundling provider. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bundling(final software.amazon.awscdk.core.BundlingOptions bundling) {
this.props.bundling(bundling);
return this;
}
/**
* A list of principals that should be able to read this asset from S3.
*
* You can use asset.grantRead(principal)
to grant read permissions later.
*
* Default: - No principals that can read file asset.
*
* @return {@code this}
* @param readers A list of principals that should be able to read this asset from S3. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder readers(final java.util.List extends software.amazon.awscdk.services.iam.IGrantable> readers) {
this.props.readers(readers);
return this;
}
/**
* (deprecated) Custom hash to use when identifying the specific version of the asset.
*
* For consistency,
* this custom hash will be SHA256 hashed and encoded as hex. The resulting hash will be
* the asset hash.
*
* NOTE: the source hash is used in order to identify a specific revision of the asset,
* and used for optimizing and caching deployment activities related to this asset such as
* packaging, uploading to Amazon S3, etc. If you chose to customize the source hash,
* you will need to make sure it is updated every time the source changes, or otherwise
* it is possible that some deployments will not be invalidated.
*
* Default: - automatically calculate source hash based on the contents
* of the source file or directory.
*
* @return {@code this}
* @deprecated see `assetHash` and `assetHashType`
* @param sourceHash Custom hash to use when identifying the specific version of the asset. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder sourceHash(final java.lang.String sourceHash) {
this.props.sourceHash(sourceHash);
return this;
}
/**
* The disk location of the asset.
*
* The path should refer to one of the following:
*
*
* - A regular file or a .zip file, in which case the file will be uploaded as-is to S3.
* - A directory, in which case it will be archived into a .zip file and uploaded to S3.
*
*
* @return {@code this}
* @param path The disk location of the asset. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder path(final java.lang.String path) {
this.props.path(path);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.s3.assets.Asset}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.s3.assets.Asset build() {
return new software.amazon.awscdk.services.s3.assets.Asset(
this.scope,
this.id,
this.props.build()
);
}
}
}