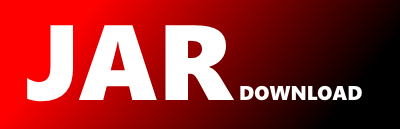
software.amazon.awscdk.services.s3.assets.AssetProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3-assets Show documentation
Show all versions of s3-assets Show documentation
Deploy local files and directories to S3
package software.amazon.awscdk.services.s3.assets;
/**
* EXPERIMENTAL
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.14.2 (build e881f16)", date = "2019-07-25T16:39:20.416Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface AssetProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.assets.CopyOptions {
/**
* The disk location of the asset.
*
* The path should refer to one of the following:
* - A regular file or a .zip file, in which case the file will be uploaded as-is to S3.
* - A directory, in which case it will be archived into a .zip file and uploaded to S3.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
java.lang.String getPath();
/**
* A list of principals that should be able to read this asset from S3. You can use `asset.grantRead(principal)` to grant read permissions later.
*
* Default: - No principals that can read file asset.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
java.util.List getReaders();
/**
* @return a {@link Builder} of {@link AssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
final class Builder {
private java.lang.String _path;
@javax.annotation.Nullable
private java.util.List _readers;
@javax.annotation.Nullable
private java.util.List _exclude;
@javax.annotation.Nullable
private software.amazon.awscdk.assets.FollowMode _follow;
/**
* Sets the value of Path
* @param value The disk location of the asset.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withPath(final java.lang.String value) {
this._path = java.util.Objects.requireNonNull(value, "path is required");
return this;
}
/**
* Sets the value of Readers
* @param value A list of principals that should be able to read this asset from S3. You can use `asset.grantRead(principal)` to grant read permissions later.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withReaders(@javax.annotation.Nullable final java.util.List value) {
this._readers = value;
return this;
}
/**
* Sets the value of Exclude
* @param value Glob patterns to exclude from the copy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withExclude(@javax.annotation.Nullable final java.util.List value) {
this._exclude = value;
return this;
}
/**
* Sets the value of Follow
* @param value A strategy for how to handle symlinks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withFollow(@javax.annotation.Nullable final software.amazon.awscdk.assets.FollowMode value) {
this._follow = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AssetProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public AssetProps build() {
return new AssetProps() {
private final java.lang.String $path = java.util.Objects.requireNonNull(_path, "path is required");
@javax.annotation.Nullable
private final java.util.List $readers = _readers;
@javax.annotation.Nullable
private final java.util.List $exclude = _exclude;
@javax.annotation.Nullable
private final software.amazon.awscdk.assets.FollowMode $follow = _follow;
@Override
public java.lang.String getPath() {
return this.$path;
}
@Override
public java.util.List getReaders() {
return this.$readers;
}
@Override
public java.util.List getExclude() {
return this.$exclude;
}
@Override
public software.amazon.awscdk.assets.FollowMode getFollow() {
return this.$follow;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("path", om.valueToTree(this.getPath()));
if (this.getReaders() != null) {
obj.set("readers", om.valueToTree(this.getReaders()));
}
if (this.getExclude() != null) {
obj.set("exclude", om.valueToTree(this.getExclude()));
}
if (this.getFollow() != null) {
obj.set("follow", om.valueToTree(this.getFollow()));
}
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.s3.assets.AssetProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The disk location of the asset.
*
* The path should refer to one of the following:
* - A regular file or a .zip file, in which case the file will be uploaded as-is to S3.
* - A directory, in which case it will be archived into a .zip file and uploaded to S3.
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public java.lang.String getPath() {
return this.jsiiGet("path", java.lang.String.class);
}
/**
* A list of principals that should be able to read this asset from S3. You can use `asset.grantRead(principal)` to grant read permissions later.
*
* Default: - No principals that can read file asset.
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public java.util.List getReaders() {
return this.jsiiGet("readers", java.util.List.class);
}
/**
* Glob patterns to exclude from the copy.
*
* Default: nothing is excluded
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public java.util.List getExclude() {
return this.jsiiGet("exclude", java.util.List.class);
}
/**
* A strategy for how to handle symlinks.
*
* Default: Never
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public software.amazon.awscdk.assets.FollowMode getFollow() {
return this.jsiiGet("follow", software.amazon.awscdk.assets.FollowMode.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy