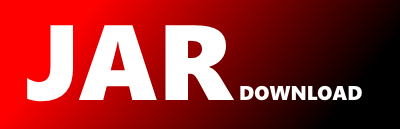
software.amazon.awscdk.services.s3.assets.AssetOptions Maven / Gradle / Ivy
Show all versions of s3-assets Show documentation
package software.amazon.awscdk.services.s3.assets;
/**
* EXPERIMENTAL
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.22.0 (build 14afdde)", date = "2020-03-18T10:30:21.713Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.s3.assets.$Module.class, fqn = "@aws-cdk/aws-s3-assets.AssetOptions")
@software.amazon.jsii.Jsii.Proxy(AssetOptions.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface AssetOptions extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.assets.CopyOptions {
/**
* A list of principals that should be able to read this asset from S3.
*
* You can use asset.grantRead(principal)
to grant read permissions later.
*
* Default: - No principals that can read file asset.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default java.util.List getReaders() {
return null;
}
/**
* Custom source hash to use when identifying the specific version of the asset.
*
* NOTE: the source hash is used in order to identify a specific revision of the asset,
* and used for optimizing and caching deployment activities related to this asset such as
* packaging, uploading to Amazon S3, etc. If you chose to customize the source hash,
* you will need to make sure it is updated every time the source changes, or otherwise
* it is possible that some deployments will not be invalidated.
*
* Default: - automatically calculate source hash based on the contents
* of the source file or directory.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default java.lang.String getSourceHash() {
return null;
}
/**
* @return a {@link Builder} of {@link AssetOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AssetOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder {
private java.util.List readers;
private java.lang.String sourceHash;
private java.util.List exclude;
private software.amazon.awscdk.assets.FollowMode follow;
/**
* Sets the value of {@link AssetOptions#getReaders}
* @param readers A list of principals that should be able to read this asset from S3.
* You can use asset.grantRead(principal)
to grant read permissions later.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder readers(java.util.List readers) {
this.readers = readers;
return this;
}
/**
* Sets the value of {@link AssetOptions#getSourceHash}
* @param sourceHash Custom source hash to use when identifying the specific version of the asset.
* NOTE: the source hash is used in order to identify a specific revision of the asset,
* and used for optimizing and caching deployment activities related to this asset such as
* packaging, uploading to Amazon S3, etc. If you chose to customize the source hash,
* you will need to make sure it is updated every time the source changes, or otherwise
* it is possible that some deployments will not be invalidated.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder sourceHash(java.lang.String sourceHash) {
this.sourceHash = sourceHash;
return this;
}
/**
* Sets the value of {@link AssetOptions#getExclude}
* @param exclude Glob patterns to exclude from the copy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder exclude(java.util.List exclude) {
this.exclude = exclude;
return this;
}
/**
* Sets the value of {@link AssetOptions#getFollow}
* @param follow A strategy for how to handle symlinks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder follow(software.amazon.awscdk.assets.FollowMode follow) {
this.follow = follow;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AssetOptions}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public AssetOptions build() {
return new Jsii$Proxy(readers, sourceHash, exclude, follow);
}
}
/**
* An implementation for {@link AssetOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AssetOptions {
private final java.util.List readers;
private final java.lang.String sourceHash;
private final java.util.List exclude;
private final software.amazon.awscdk.assets.FollowMode follow;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.readers = this.jsiiGet("readers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IGrantable.class)));
this.sourceHash = this.jsiiGet("sourceHash", java.lang.String.class);
this.exclude = this.jsiiGet("exclude", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.follow = this.jsiiGet("follow", software.amazon.awscdk.assets.FollowMode.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.util.List readers, final java.lang.String sourceHash, final java.util.List exclude, final software.amazon.awscdk.assets.FollowMode follow) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.readers = readers;
this.sourceHash = sourceHash;
this.exclude = exclude;
this.follow = follow;
}
@Override
public java.util.List getReaders() {
return this.readers;
}
@Override
public java.lang.String getSourceHash() {
return this.sourceHash;
}
@Override
public java.util.List getExclude() {
return this.exclude;
}
@Override
public software.amazon.awscdk.assets.FollowMode getFollow() {
return this.follow;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getReaders() != null) {
data.set("readers", om.valueToTree(this.getReaders()));
}
if (this.getSourceHash() != null) {
data.set("sourceHash", om.valueToTree(this.getSourceHash()));
}
if (this.getExclude() != null) {
data.set("exclude", om.valueToTree(this.getExclude()));
}
if (this.getFollow() != null) {
data.set("follow", om.valueToTree(this.getFollow()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-s3-assets.AssetOptions"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AssetOptions.Jsii$Proxy that = (AssetOptions.Jsii$Proxy) o;
if (this.readers != null ? !this.readers.equals(that.readers) : that.readers != null) return false;
if (this.sourceHash != null ? !this.sourceHash.equals(that.sourceHash) : that.sourceHash != null) return false;
if (this.exclude != null ? !this.exclude.equals(that.exclude) : that.exclude != null) return false;
return this.follow != null ? this.follow.equals(that.follow) : that.follow == null;
}
@Override
public int hashCode() {
int result = this.readers != null ? this.readers.hashCode() : 0;
result = 31 * result + (this.sourceHash != null ? this.sourceHash.hashCode() : 0);
result = 31 * result + (this.exclude != null ? this.exclude.hashCode() : 0);
result = 31 * result + (this.follow != null ? this.follow.hashCode() : 0);
return result;
}
}
}