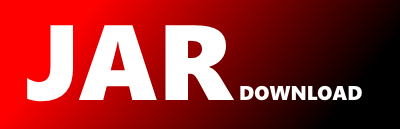
software.amazon.awscdk.services.s3.assets.AssetProps Maven / Gradle / Ivy
Show all versions of s3-assets Show documentation
package software.amazon.awscdk.services.s3.assets;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2020-12-30T23:05:54.339Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.s3.assets.$Module.class, fqn = "@aws-cdk/aws-s3-assets.AssetProps")
@software.amazon.jsii.Jsii.Proxy(AssetProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface AssetProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.s3.assets.AssetOptions {
/**
* (experimental) The disk location of the asset.
*
* The path should refer to one of the following:
*
*
* - A regular file or a .zip file, in which case the file will be uploaded as-is to S3.
* - A directory, in which case it will be archived into a .zip file and uploaded to S3.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getPath();
/**
* @return a {@link Builder} of {@link AssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String path;
private java.util.List readers;
private java.lang.String sourceHash;
private java.util.List exclude;
private software.amazon.awscdk.assets.FollowMode follow;
private software.amazon.awscdk.core.IgnoreMode ignoreMode;
private java.lang.String assetHash;
private software.amazon.awscdk.core.AssetHashType assetHashType;
private software.amazon.awscdk.core.BundlingOptions bundling;
/**
* Sets the value of {@link AssetProps#getPath}
* @param path The disk location of the asset. This parameter is required.
* The path should refer to one of the following:
*
*
* - A regular file or a .zip file, in which case the file will be uploaded as-is to S3.
* - A directory, in which case it will be archived into a .zip file and uploaded to S3.
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder path(java.lang.String path) {
this.path = path;
return this;
}
/**
* Sets the value of {@link AssetProps#getReaders}
* @param readers A list of principals that should be able to read this asset from S3.
* You can use asset.grantRead(principal)
to grant read permissions later.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder readers(java.util.List extends software.amazon.awscdk.services.iam.IGrantable> readers) {
this.readers = (java.util.List)readers;
return this;
}
/**
* Sets the value of {@link AssetProps#getSourceHash}
* @param sourceHash Custom hash to use when identifying the specific version of the asset.
* For consistency,
* this custom hash will be SHA256 hashed and encoded as hex. The resulting hash will be
* the asset hash.
*
* NOTE: the source hash is used in order to identify a specific revision of the asset,
* and used for optimizing and caching deployment activities related to this asset such as
* packaging, uploading to Amazon S3, etc. If you chose to customize the source hash,
* you will need to make sure it is updated every time the source changes, or otherwise
* it is possible that some deployments will not be invalidated.
* @return {@code this}
* @deprecated see `assetHash` and `assetHashType`
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder sourceHash(java.lang.String sourceHash) {
this.sourceHash = sourceHash;
return this;
}
/**
* Sets the value of {@link AssetProps#getExclude}
* @param exclude Glob patterns to exclude from the copy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder exclude(java.util.List exclude) {
this.exclude = exclude;
return this;
}
/**
* Sets the value of {@link AssetProps#getFollow}
* @param follow A strategy for how to handle symlinks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder follow(software.amazon.awscdk.assets.FollowMode follow) {
this.follow = follow;
return this;
}
/**
* Sets the value of {@link AssetProps#getIgnoreMode}
* @param ignoreMode The ignore behavior to use for exclude patterns.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder ignoreMode(software.amazon.awscdk.core.IgnoreMode ignoreMode) {
this.ignoreMode = ignoreMode;
return this;
}
/**
* Sets the value of {@link AssetProps#getAssetHash}
* @param assetHash Specify a custom hash for this asset.
* If assetHashType
is set it must
* be set to AssetHashType.CUSTOM
. For consistency, this custom hash will
* be SHA256 hashed and encoded as hex. The resulting hash will be the asset
* hash.
*
* NOTE: the hash is used in order to identify a specific revision of the asset, and
* used for optimizing and caching deployment activities related to this asset such as
* packaging, uploading to Amazon S3, etc. If you chose to customize the hash, you will
* need to make sure it is updated every time the asset changes, or otherwise it is
* possible that some deployments will not be invalidated.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assetHash(java.lang.String assetHash) {
this.assetHash = assetHash;
return this;
}
/**
* Sets the value of {@link AssetProps#getAssetHashType}
* @param assetHashType Specifies the type of hash to calculate for this asset.
* If assetHash
is configured, this option must be undefined
or
* AssetHashType.CUSTOM
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assetHashType(software.amazon.awscdk.core.AssetHashType assetHashType) {
this.assetHashType = assetHashType;
return this;
}
/**
* Sets the value of {@link AssetProps#getBundling}
* @param bundling Bundle the asset by executing a command in a Docker container.
* The asset path will be mounted at /asset-input
. The Docker
* container is responsible for putting content at /asset-output
.
* The content at /asset-output
will be zipped and used as the
* final asset.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder bundling(software.amazon.awscdk.core.BundlingOptions bundling) {
this.bundling = bundling;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AssetProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public AssetProps build() {
return new Jsii$Proxy(path, readers, sourceHash, exclude, follow, ignoreMode, assetHash, assetHashType, bundling);
}
}
/**
* An implementation for {@link AssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AssetProps {
private final java.lang.String path;
private final java.util.List readers;
private final java.lang.String sourceHash;
private final java.util.List exclude;
private final software.amazon.awscdk.assets.FollowMode follow;
private final software.amazon.awscdk.core.IgnoreMode ignoreMode;
private final java.lang.String assetHash;
private final software.amazon.awscdk.core.AssetHashType assetHashType;
private final software.amazon.awscdk.core.BundlingOptions bundling;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.path = software.amazon.jsii.Kernel.get(this, "path", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.readers = software.amazon.jsii.Kernel.get(this, "readers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IGrantable.class)));
this.sourceHash = software.amazon.jsii.Kernel.get(this, "sourceHash", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.exclude = software.amazon.jsii.Kernel.get(this, "exclude", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.follow = software.amazon.jsii.Kernel.get(this, "follow", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.assets.FollowMode.class));
this.ignoreMode = software.amazon.jsii.Kernel.get(this, "ignoreMode", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.IgnoreMode.class));
this.assetHash = software.amazon.jsii.Kernel.get(this, "assetHash", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.assetHashType = software.amazon.jsii.Kernel.get(this, "assetHashType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.AssetHashType.class));
this.bundling = software.amazon.jsii.Kernel.get(this, "bundling", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.BundlingOptions.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final java.lang.String path, final java.util.List extends software.amazon.awscdk.services.iam.IGrantable> readers, final java.lang.String sourceHash, final java.util.List exclude, final software.amazon.awscdk.assets.FollowMode follow, final software.amazon.awscdk.core.IgnoreMode ignoreMode, final java.lang.String assetHash, final software.amazon.awscdk.core.AssetHashType assetHashType, final software.amazon.awscdk.core.BundlingOptions bundling) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.path = java.util.Objects.requireNonNull(path, "path is required");
this.readers = (java.util.List)readers;
this.sourceHash = sourceHash;
this.exclude = exclude;
this.follow = follow;
this.ignoreMode = ignoreMode;
this.assetHash = assetHash;
this.assetHashType = assetHashType;
this.bundling = bundling;
}
@Override
public final java.lang.String getPath() {
return this.path;
}
@Override
public final java.util.List getReaders() {
return this.readers;
}
@Override
public final java.lang.String getSourceHash() {
return this.sourceHash;
}
@Override
public final java.util.List getExclude() {
return this.exclude;
}
@Override
public final software.amazon.awscdk.assets.FollowMode getFollow() {
return this.follow;
}
@Override
public final software.amazon.awscdk.core.IgnoreMode getIgnoreMode() {
return this.ignoreMode;
}
@Override
public final java.lang.String getAssetHash() {
return this.assetHash;
}
@Override
public final software.amazon.awscdk.core.AssetHashType getAssetHashType() {
return this.assetHashType;
}
@Override
public final software.amazon.awscdk.core.BundlingOptions getBundling() {
return this.bundling;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("path", om.valueToTree(this.getPath()));
if (this.getReaders() != null) {
data.set("readers", om.valueToTree(this.getReaders()));
}
if (this.getSourceHash() != null) {
data.set("sourceHash", om.valueToTree(this.getSourceHash()));
}
if (this.getExclude() != null) {
data.set("exclude", om.valueToTree(this.getExclude()));
}
if (this.getFollow() != null) {
data.set("follow", om.valueToTree(this.getFollow()));
}
if (this.getIgnoreMode() != null) {
data.set("ignoreMode", om.valueToTree(this.getIgnoreMode()));
}
if (this.getAssetHash() != null) {
data.set("assetHash", om.valueToTree(this.getAssetHash()));
}
if (this.getAssetHashType() != null) {
data.set("assetHashType", om.valueToTree(this.getAssetHashType()));
}
if (this.getBundling() != null) {
data.set("bundling", om.valueToTree(this.getBundling()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-s3-assets.AssetProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AssetProps.Jsii$Proxy that = (AssetProps.Jsii$Proxy) o;
if (!path.equals(that.path)) return false;
if (this.readers != null ? !this.readers.equals(that.readers) : that.readers != null) return false;
if (this.sourceHash != null ? !this.sourceHash.equals(that.sourceHash) : that.sourceHash != null) return false;
if (this.exclude != null ? !this.exclude.equals(that.exclude) : that.exclude != null) return false;
if (this.follow != null ? !this.follow.equals(that.follow) : that.follow != null) return false;
if (this.ignoreMode != null ? !this.ignoreMode.equals(that.ignoreMode) : that.ignoreMode != null) return false;
if (this.assetHash != null ? !this.assetHash.equals(that.assetHash) : that.assetHash != null) return false;
if (this.assetHashType != null ? !this.assetHashType.equals(that.assetHashType) : that.assetHashType != null) return false;
return this.bundling != null ? this.bundling.equals(that.bundling) : that.bundling == null;
}
@Override
public final int hashCode() {
int result = this.path.hashCode();
result = 31 * result + (this.readers != null ? this.readers.hashCode() : 0);
result = 31 * result + (this.sourceHash != null ? this.sourceHash.hashCode() : 0);
result = 31 * result + (this.exclude != null ? this.exclude.hashCode() : 0);
result = 31 * result + (this.follow != null ? this.follow.hashCode() : 0);
result = 31 * result + (this.ignoreMode != null ? this.ignoreMode.hashCode() : 0);
result = 31 * result + (this.assetHash != null ? this.assetHash.hashCode() : 0);
result = 31 * result + (this.assetHashType != null ? this.assetHashType.hashCode() : 0);
result = 31 * result + (this.bundling != null ? this.bundling.hashCode() : 0);
return result;
}
}
}