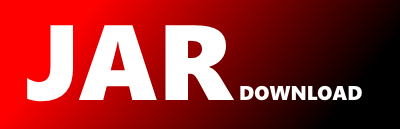
software.amazon.awscdk.services.sagemaker.CfnImage Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
package software.amazon.awscdk.services.sagemaker;
/**
* A CloudFormation `AWS::SageMaker::Image`.
*
* Creates a custom SageMaker image. A SageMaker image is a set of image versions. Each image version represents a container image stored in Amazon Elastic Container Registry (ECR). For more information, see Bring your own SageMaker image .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* CfnImage cfnImage = CfnImage.Builder.create(this, "MyCfnImage")
* .imageName("imageName")
* .imageRoleArn("imageRoleArn")
* // the properties below are optional
* .imageDescription("imageDescription")
* .imageDisplayName("imageDisplayName")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.70.0 (build 03c2f6f)", date = "2022-11-01T13:16:28.506Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnImage")
public class CfnImage extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnImage(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnImage(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.sagemaker.CfnImage.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::SageMaker::Image`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnImage(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.sagemaker.CfnImageProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The Amazon Resource Name (ARN) of the image.
*
* Type : String
*
* Length Constraints : Maximum length of 256.
*
* Pattern : ^arn:aws(-[\w]+)*:sagemaker:.+:[0-9]{12}:image/[a-z0-9]([-.]?[a-z0-9])*$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrImageArn() {
return software.amazon.jsii.Kernel.get(this, "attrImageArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* A list of key-value pairs to apply to this resource.
*
* Array Members : Minimum number of 0 items. Maximum number of 50 items.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The name of the Image. Must be unique by region in your account.
*
* Length Constraints : Minimum length of 1. Maximum length of 63.
*
* Pattern : ^[a-zA-Z0-9]([-.]?[a-zA-Z0-9]){0,62}$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getImageName() {
return software.amazon.jsii.Kernel.get(this, "imageName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the Image. Must be unique by region in your account.
*
* Length Constraints : Minimum length of 1. Maximum length of 63.
*
* Pattern : ^[a-zA-Z0-9]([-.]?[a-zA-Z0-9]){0,62}$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setImageName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "imageName", java.util.Objects.requireNonNull(value, "imageName is required"));
}
/**
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
* Length Constraints : Minimum length of 20. Maximum length of 2048.
*
* Pattern : ^arn:aws[a-z\-]*:iam::\d{12}:role/?[a-zA-Z_0-9+=,.@\-_/]+$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getImageRoleArn() {
return software.amazon.jsii.Kernel.get(this, "imageRoleArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
* Length Constraints : Minimum length of 20. Maximum length of 2048.
*
* Pattern : ^arn:aws[a-z\-]*:iam::\d{12}:role/?[a-zA-Z_0-9+=,.@\-_/]+$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setImageRoleArn(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "imageRoleArn", java.util.Objects.requireNonNull(value, "imageRoleArn is required"));
}
/**
* The description of the image.
*
* Length Constraints : Minimum length of 1. Maximum length of 512.
*
* Pattern : .*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getImageDescription() {
return software.amazon.jsii.Kernel.get(this, "imageDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the image.
*
* Length Constraints : Minimum length of 1. Maximum length of 512.
*
* Pattern : .*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setImageDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "imageDescription", value);
}
/**
* The display name of the image.
*
* Length Constraints : Minimum length of 1. Maximum length of 128.
*
* Pattern : ^\S(.*\S)?$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getImageDisplayName() {
return software.amazon.jsii.Kernel.get(this, "imageDisplayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The display name of the image.
*
* Length Constraints : Minimum length of 1. Maximum length of 128.
*
* Pattern : ^\S(.*\S)?$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setImageDisplayName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "imageDisplayName", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.sagemaker.CfnImage}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.sagemaker.CfnImageProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.sagemaker.CfnImageProps.Builder();
}
/**
* The name of the Image. Must be unique by region in your account.
*
* Length Constraints : Minimum length of 1. Maximum length of 63.
*
* Pattern : ^[a-zA-Z0-9]([-.]?[a-zA-Z0-9]){0,62}$
*
* @return {@code this}
* @param imageName The name of the Image. Must be unique by region in your account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageName(final java.lang.String imageName) {
this.props.imageName(imageName);
return this;
}
/**
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
* Length Constraints : Minimum length of 20. Maximum length of 2048.
*
* Pattern : ^arn:aws[a-z\-]*:iam::\d{12}:role/?[a-zA-Z_0-9+=,.@\-_/]+$
*
* @return {@code this}
* @param imageRoleArn The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageRoleArn(final java.lang.String imageRoleArn) {
this.props.imageRoleArn(imageRoleArn);
return this;
}
/**
* The description of the image.
*
* Length Constraints : Minimum length of 1. Maximum length of 512.
*
* Pattern : .*
*
* @return {@code this}
* @param imageDescription The description of the image. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageDescription(final java.lang.String imageDescription) {
this.props.imageDescription(imageDescription);
return this;
}
/**
* The display name of the image.
*
* Length Constraints : Minimum length of 1. Maximum length of 128.
*
* Pattern : ^\S(.*\S)?$
*
* @return {@code this}
* @param imageDisplayName The display name of the image. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageDisplayName(final java.lang.String imageDisplayName) {
this.props.imageDisplayName(imageDisplayName);
return this;
}
/**
* A list of key-value pairs to apply to this resource.
*
* Array Members : Minimum number of 0 items. Maximum number of 50 items.
*
* @return {@code this}
* @param tags A list of key-value pairs to apply to this resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.sagemaker.CfnImage}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.sagemaker.CfnImage build() {
return new software.amazon.awscdk.services.sagemaker.CfnImage(
this.scope,
this.id,
this.props.build()
);
}
}
}