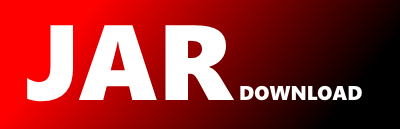
software.amazon.awscdk.services.sagemaker.CfnAppProps Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
package software.amazon.awscdk.services.sagemaker;
/**
* Properties for defining a `CfnApp`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* CfnAppProps cfnAppProps = CfnAppProps.builder()
* .appName("appName")
* .appType("appType")
* .domainId("domainId")
* .userProfileName("userProfileName")
* // the properties below are optional
* .resourceSpec(ResourceSpecProperty.builder()
* .instanceType("instanceType")
* .sageMakerImageArn("sageMakerImageArn")
* .sageMakerImageVersionArn("sageMakerImageVersionArn")
* .build())
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:22.438Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnAppProps")
@software.amazon.jsii.Jsii.Proxy(CfnAppProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnAppProps extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the app.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAppName();
/**
* The type of app.
*
* Allowed Values : JupyterServer | KernelGateway | RSessionGateway | RStudioServerPro | TensorBoard | Canvas
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAppType();
/**
* The domain ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getDomainId();
/**
* The user profile name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getUserProfileName();
/**
* Specifies the ARNs of a SageMaker image and SageMaker image version, and the instance type that the version runs on.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getResourceSpec() {
return null;
}
/**
* An array of key-value pairs to apply to this resource.
*
* For more information, see Tag .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnAppProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnAppProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String appName;
java.lang.String appType;
java.lang.String domainId;
java.lang.String userProfileName;
java.lang.Object resourceSpec;
java.util.List tags;
/**
* Sets the value of {@link CfnAppProps#getAppName}
* @param appName The name of the app. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appName(java.lang.String appName) {
this.appName = appName;
return this;
}
/**
* Sets the value of {@link CfnAppProps#getAppType}
* @param appType The type of app. This parameter is required.
* Allowed Values : JupyterServer | KernelGateway | RSessionGateway | RStudioServerPro | TensorBoard | Canvas
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appType(java.lang.String appType) {
this.appType = appType;
return this;
}
/**
* Sets the value of {@link CfnAppProps#getDomainId}
* @param domainId The domain ID. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainId(java.lang.String domainId) {
this.domainId = domainId;
return this;
}
/**
* Sets the value of {@link CfnAppProps#getUserProfileName}
* @param userProfileName The user profile name. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProfileName(java.lang.String userProfileName) {
this.userProfileName = userProfileName;
return this;
}
/**
* Sets the value of {@link CfnAppProps#getResourceSpec}
* @param resourceSpec Specifies the ARNs of a SageMaker image and SageMaker image version, and the instance type that the version runs on.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder resourceSpec(software.amazon.awscdk.services.sagemaker.CfnApp.ResourceSpecProperty resourceSpec) {
this.resourceSpec = resourceSpec;
return this;
}
/**
* Sets the value of {@link CfnAppProps#getResourceSpec}
* @param resourceSpec Specifies the ARNs of a SageMaker image and SageMaker image version, and the instance type that the version runs on.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder resourceSpec(software.amazon.awscdk.core.IResolvable resourceSpec) {
this.resourceSpec = resourceSpec;
return this;
}
/**
* Sets the value of {@link CfnAppProps#getTags}
* @param tags An array of key-value pairs to apply to this resource.
* For more information, see Tag .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnAppProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnAppProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnAppProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnAppProps {
private final java.lang.String appName;
private final java.lang.String appType;
private final java.lang.String domainId;
private final java.lang.String userProfileName;
private final java.lang.Object resourceSpec;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.appName = software.amazon.jsii.Kernel.get(this, "appName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.appType = software.amazon.jsii.Kernel.get(this, "appType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.domainId = software.amazon.jsii.Kernel.get(this, "domainId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.userProfileName = software.amazon.jsii.Kernel.get(this, "userProfileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.resourceSpec = software.amazon.jsii.Kernel.get(this, "resourceSpec", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.appName = java.util.Objects.requireNonNull(builder.appName, "appName is required");
this.appType = java.util.Objects.requireNonNull(builder.appType, "appType is required");
this.domainId = java.util.Objects.requireNonNull(builder.domainId, "domainId is required");
this.userProfileName = java.util.Objects.requireNonNull(builder.userProfileName, "userProfileName is required");
this.resourceSpec = builder.resourceSpec;
this.tags = (java.util.List)builder.tags;
}
@Override
public final java.lang.String getAppName() {
return this.appName;
}
@Override
public final java.lang.String getAppType() {
return this.appType;
}
@Override
public final java.lang.String getDomainId() {
return this.domainId;
}
@Override
public final java.lang.String getUserProfileName() {
return this.userProfileName;
}
@Override
public final java.lang.Object getResourceSpec() {
return this.resourceSpec;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("appName", om.valueToTree(this.getAppName()));
data.set("appType", om.valueToTree(this.getAppType()));
data.set("domainId", om.valueToTree(this.getDomainId()));
data.set("userProfileName", om.valueToTree(this.getUserProfileName()));
if (this.getResourceSpec() != null) {
data.set("resourceSpec", om.valueToTree(this.getResourceSpec()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnAppProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnAppProps.Jsii$Proxy that = (CfnAppProps.Jsii$Proxy) o;
if (!appName.equals(that.appName)) return false;
if (!appType.equals(that.appType)) return false;
if (!domainId.equals(that.domainId)) return false;
if (!userProfileName.equals(that.userProfileName)) return false;
if (this.resourceSpec != null ? !this.resourceSpec.equals(that.resourceSpec) : that.resourceSpec != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public final int hashCode() {
int result = this.appName.hashCode();
result = 31 * result + (this.appType.hashCode());
result = 31 * result + (this.domainId.hashCode());
result = 31 * result + (this.userProfileName.hashCode());
result = 31 * result + (this.resourceSpec != null ? this.resourceSpec.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}