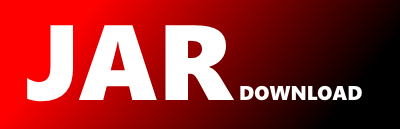
software.amazon.awscdk.services.sagemaker.CfnModelCard Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
package software.amazon.awscdk.services.sagemaker;
/**
* A CloudFormation `AWS::SageMaker::ModelCard`.
*
* Creates an Amazon SageMaker Model Card.
*
* For information about how to use model cards, see Amazon SageMaker Model Card .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* Object value;
* CfnModelCard cfnModelCard = CfnModelCard.Builder.create(this, "MyCfnModelCard")
* .content(ContentProperty.builder()
* .additionalInformation(AdditionalInformationProperty.builder()
* .caveatsAndRecommendations("caveatsAndRecommendations")
* .customDetails(Map.of(
* "customDetailsKey", "customDetails"))
* .ethicalConsiderations("ethicalConsiderations")
* .build())
* .businessDetails(BusinessDetailsProperty.builder()
* .businessProblem("businessProblem")
* .businessStakeholders("businessStakeholders")
* .lineOfBusiness("lineOfBusiness")
* .build())
* .evaluationDetails(List.of(EvaluationDetailProperty.builder()
* .name("name")
* // the properties below are optional
* .datasets(List.of("datasets"))
* .evaluationJobArn("evaluationJobArn")
* .evaluationObservation("evaluationObservation")
* .metadata(Map.of(
* "metadataKey", "metadata"))
* .metricGroups(List.of(MetricGroupProperty.builder()
* .metricData(List.of(MetricDataItemsProperty.builder()
* .name("name")
* .type("type")
* .value(value)
* // the properties below are optional
* .notes("notes")
* .xAxisName(List.of("xAxisName"))
* .yAxisName(List.of("yAxisName"))
* .build()))
* .name("name")
* .build()))
* .build()))
* .intendedUses(IntendedUsesProperty.builder()
* .explanationsForRiskRating("explanationsForRiskRating")
* .factorsAffectingModelEfficiency("factorsAffectingModelEfficiency")
* .intendedUses("intendedUses")
* .purposeOfModel("purposeOfModel")
* .riskRating("riskRating")
* .build())
* .modelOverview(ModelOverviewProperty.builder()
* .algorithmType("algorithmType")
* .inferenceEnvironment(InferenceEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .modelArtifact(List.of("modelArtifact"))
* .modelCreator("modelCreator")
* .modelDescription("modelDescription")
* .modelId("modelId")
* .modelName("modelName")
* .modelOwner("modelOwner")
* .modelVersion(123)
* .problemType("problemType")
* .build())
* .modelPackageDetails(ModelPackageDetailsProperty.builder()
* .approvalDescription("approvalDescription")
* .createdBy(ModelPackageCreatorProperty.builder()
* .userProfileName("userProfileName")
* .build())
* .domain("domain")
* .inferenceSpecification(InferenceSpecificationProperty.builder()
* .containers(List.of(ContainerProperty.builder()
* .image("image")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .nearestModelName("nearestModelName")
* .build()))
* .build())
* .modelApprovalStatus("modelApprovalStatus")
* .modelPackageArn("modelPackageArn")
* .modelPackageDescription("modelPackageDescription")
* .modelPackageGroupName("modelPackageGroupName")
* .modelPackageName("modelPackageName")
* .modelPackageStatus("modelPackageStatus")
* .modelPackageVersion(123)
* .sourceAlgorithms(List.of(SourceAlgorithmProperty.builder()
* .algorithmName("algorithmName")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .build()))
* .task("task")
* .build())
* .trainingDetails(TrainingDetailsProperty.builder()
* .objectiveFunction(ObjectiveFunctionProperty.builder()
* .function(FunctionProperty.builder()
* .condition("condition")
* .facet("facet")
* .function("function")
* .build())
* .notes("notes")
* .build())
* .trainingJobDetails(TrainingJobDetailsProperty.builder()
* .hyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .trainingArn("trainingArn")
* .trainingDatasets(List.of("trainingDatasets"))
* .trainingEnvironment(TrainingEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .trainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .userProvidedHyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .userProvidedTrainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .build())
* .trainingObservations("trainingObservations")
* .build())
* .build())
* .modelCardName("modelCardName")
* .modelCardStatus("modelCardStatus")
* // the properties below are optional
* .createdBy(UserContextProperty.builder()
* .domainId("domainId")
* .userProfileArn("userProfileArn")
* .userProfileName("userProfileName")
* .build())
* .lastModifiedBy(UserContextProperty.builder()
* .domainId("domainId")
* .userProfileArn("userProfileArn")
* .userProfileName("userProfileName")
* .build())
* .securityConfig(SecurityConfigProperty.builder()
* .kmsKeyId("kmsKeyId")
* .build())
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:22.620Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard")
public class CfnModelCard extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnModelCard(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnModelCard(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.sagemaker.CfnModelCard.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::SageMaker::ModelCard`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnModelCard(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.sagemaker.CfnModelCardProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreatedByDomainId() {
return software.amazon.jsii.Kernel.get(this, "attrCreatedByDomainId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreatedByUserProfileArn() {
return software.amazon.jsii.Kernel.get(this, "attrCreatedByUserProfileArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreatedByUserProfileName() {
return software.amazon.jsii.Kernel.get(this, "attrCreatedByUserProfileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreationTime() {
return software.amazon.jsii.Kernel.get(this, "attrCreationTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrLastModifiedByDomainId() {
return software.amazon.jsii.Kernel.get(this, "attrLastModifiedByDomainId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrLastModifiedByUserProfileArn() {
return software.amazon.jsii.Kernel.get(this, "attrLastModifiedByUserProfileArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrLastModifiedByUserProfileName() {
return software.amazon.jsii.Kernel.get(this, "attrLastModifiedByUserProfileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrLastModifiedTime() {
return software.amazon.jsii.Kernel.get(this, "attrLastModifiedTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Number (ARN) of the model card.
*
* For example, arn:aws:sagemaker:us-west-2:012345678901:modelcard/examplemodelcard
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrModelCardArn() {
return software.amazon.jsii.Kernel.get(this, "attrModelCardArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrModelCardProcessingStatus() {
return software.amazon.jsii.Kernel.get(this, "attrModelCardProcessingStatus", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number getAttrModelCardVersion() {
return software.amazon.jsii.Kernel.get(this, "attrModelCardVersion", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* Key-value pairs used to manage metadata for the model card.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The content of the model card.
*
* Content uses the model card JSON schema .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getContent() {
return software.amazon.jsii.Kernel.get(this, "content", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The content of the model card.
*
* Content uses the model card JSON schema .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setContent(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "content", java.util.Objects.requireNonNull(value, "content is required"));
}
/**
* The content of the model card.
*
* Content uses the model card JSON schema .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setContent(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.sagemaker.CfnModelCard.ContentProperty value) {
software.amazon.jsii.Kernel.set(this, "content", java.util.Objects.requireNonNull(value, "content is required"));
}
/**
* The unique name of the model card.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getModelCardName() {
return software.amazon.jsii.Kernel.get(this, "modelCardName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique name of the model card.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setModelCardName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "modelCardName", java.util.Objects.requireNonNull(value, "modelCardName is required"));
}
/**
* The approval status of the model card within your organization.
*
* Different organizations might have different criteria for model card review and approval.
*
*
* Draft
: The model card is a work in progress.
* PendingReview
: The model card is pending review.
* Approved
: The model card is approved.
* Archived
: The model card is archived. No more updates should be made to the model card, but it can still be exported.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getModelCardStatus() {
return software.amazon.jsii.Kernel.get(this, "modelCardStatus", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The approval status of the model card within your organization.
*
* Different organizations might have different criteria for model card review and approval.
*
*
* Draft
: The model card is a work in progress.
* PendingReview
: The model card is pending review.
* Approved
: The model card is approved.
* Archived
: The model card is archived. No more updates should be made to the model card, but it can still be exported.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setModelCardStatus(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "modelCardStatus", java.util.Objects.requireNonNull(value, "modelCardStatus is required"));
}
/**
* Information about the user who created or modified one or more of the following:.
*
*
* - Experiment
* - Trial
* - Trial component
* - Lineage group
* - Project
* - Model Card
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getCreatedBy() {
return software.amazon.jsii.Kernel.get(this, "createdBy", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Information about the user who created or modified one or more of the following:.
*
*
* - Experiment
* - Trial
* - Trial component
* - Lineage group
* - Project
* - Model Card
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCreatedBy(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "createdBy", value);
}
/**
* Information about the user who created or modified one or more of the following:.
*
*
* - Experiment
* - Trial
* - Trial component
* - Lineage group
* - Project
* - Model Card
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCreatedBy(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.sagemaker.CfnModelCard.UserContextProperty value) {
software.amazon.jsii.Kernel.set(this, "createdBy", value);
}
/**
* `AWS::SageMaker::ModelCard.LastModifiedBy`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getLastModifiedBy() {
return software.amazon.jsii.Kernel.get(this, "lastModifiedBy", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::SageMaker::ModelCard.LastModifiedBy`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLastModifiedBy(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "lastModifiedBy", value);
}
/**
* `AWS::SageMaker::ModelCard.LastModifiedBy`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLastModifiedBy(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.sagemaker.CfnModelCard.UserContextProperty value) {
software.amazon.jsii.Kernel.set(this, "lastModifiedBy", value);
}
/**
* The security configuration used to protect model card data.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getSecurityConfig() {
return software.amazon.jsii.Kernel.get(this, "securityConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The security configuration used to protect model card data.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSecurityConfig(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "securityConfig", value);
}
/**
* The security configuration used to protect model card data.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSecurityConfig(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.sagemaker.CfnModelCard.SecurityConfigProperty value) {
software.amazon.jsii.Kernel.set(this, "securityConfig", value);
}
/**
* Additional information about the model.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* AdditionalInformationProperty additionalInformationProperty = AdditionalInformationProperty.builder()
* .caveatsAndRecommendations("caveatsAndRecommendations")
* .customDetails(Map.of(
* "customDetailsKey", "customDetails"))
* .ethicalConsiderations("ethicalConsiderations")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.AdditionalInformationProperty")
@software.amazon.jsii.Jsii.Proxy(AdditionalInformationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface AdditionalInformationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* Caveats and recommendations for those who might use this model in their applications.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCaveatsAndRecommendations() {
return null;
}
/**
* Any additional information to document about the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getCustomDetails() {
return null;
}
/**
* Any ethical considerations documented by the model card author.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getEthicalConsiderations() {
return null;
}
/**
* @return a {@link Builder} of {@link AdditionalInformationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AdditionalInformationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String caveatsAndRecommendations;
java.lang.Object customDetails;
java.lang.String ethicalConsiderations;
/**
* Sets the value of {@link AdditionalInformationProperty#getCaveatsAndRecommendations}
* @param caveatsAndRecommendations Caveats and recommendations for those who might use this model in their applications.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caveatsAndRecommendations(java.lang.String caveatsAndRecommendations) {
this.caveatsAndRecommendations = caveatsAndRecommendations;
return this;
}
/**
* Sets the value of {@link AdditionalInformationProperty#getCustomDetails}
* @param customDetails Any additional information to document about the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder customDetails(software.amazon.awscdk.core.IResolvable customDetails) {
this.customDetails = customDetails;
return this;
}
/**
* Sets the value of {@link AdditionalInformationProperty#getCustomDetails}
* @param customDetails Any additional information to document about the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder customDetails(java.util.Map customDetails) {
this.customDetails = customDetails;
return this;
}
/**
* Sets the value of {@link AdditionalInformationProperty#getEthicalConsiderations}
* @param ethicalConsiderations Any ethical considerations documented by the model card author.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ethicalConsiderations(java.lang.String ethicalConsiderations) {
this.ethicalConsiderations = ethicalConsiderations;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AdditionalInformationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AdditionalInformationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link AdditionalInformationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AdditionalInformationProperty {
private final java.lang.String caveatsAndRecommendations;
private final java.lang.Object customDetails;
private final java.lang.String ethicalConsiderations;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.caveatsAndRecommendations = software.amazon.jsii.Kernel.get(this, "caveatsAndRecommendations", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.customDetails = software.amazon.jsii.Kernel.get(this, "customDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.ethicalConsiderations = software.amazon.jsii.Kernel.get(this, "ethicalConsiderations", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.caveatsAndRecommendations = builder.caveatsAndRecommendations;
this.customDetails = builder.customDetails;
this.ethicalConsiderations = builder.ethicalConsiderations;
}
@Override
public final java.lang.String getCaveatsAndRecommendations() {
return this.caveatsAndRecommendations;
}
@Override
public final java.lang.Object getCustomDetails() {
return this.customDetails;
}
@Override
public final java.lang.String getEthicalConsiderations() {
return this.ethicalConsiderations;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCaveatsAndRecommendations() != null) {
data.set("caveatsAndRecommendations", om.valueToTree(this.getCaveatsAndRecommendations()));
}
if (this.getCustomDetails() != null) {
data.set("customDetails", om.valueToTree(this.getCustomDetails()));
}
if (this.getEthicalConsiderations() != null) {
data.set("ethicalConsiderations", om.valueToTree(this.getEthicalConsiderations()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.AdditionalInformationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AdditionalInformationProperty.Jsii$Proxy that = (AdditionalInformationProperty.Jsii$Proxy) o;
if (this.caveatsAndRecommendations != null ? !this.caveatsAndRecommendations.equals(that.caveatsAndRecommendations) : that.caveatsAndRecommendations != null) return false;
if (this.customDetails != null ? !this.customDetails.equals(that.customDetails) : that.customDetails != null) return false;
return this.ethicalConsiderations != null ? this.ethicalConsiderations.equals(that.ethicalConsiderations) : that.ethicalConsiderations == null;
}
@Override
public final int hashCode() {
int result = this.caveatsAndRecommendations != null ? this.caveatsAndRecommendations.hashCode() : 0;
result = 31 * result + (this.customDetails != null ? this.customDetails.hashCode() : 0);
result = 31 * result + (this.ethicalConsiderations != null ? this.ethicalConsiderations.hashCode() : 0);
return result;
}
}
}
/**
* Information about how the model supports business goals.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* BusinessDetailsProperty businessDetailsProperty = BusinessDetailsProperty.builder()
* .businessProblem("businessProblem")
* .businessStakeholders("businessStakeholders")
* .lineOfBusiness("lineOfBusiness")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.BusinessDetailsProperty")
@software.amazon.jsii.Jsii.Proxy(BusinessDetailsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface BusinessDetailsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The specific business problem that the model is trying to solve.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getBusinessProblem() {
return null;
}
/**
* The relevant stakeholders for the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getBusinessStakeholders() {
return null;
}
/**
* The broader business need that the model is serving.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getLineOfBusiness() {
return null;
}
/**
* @return a {@link Builder} of {@link BusinessDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link BusinessDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String businessProblem;
java.lang.String businessStakeholders;
java.lang.String lineOfBusiness;
/**
* Sets the value of {@link BusinessDetailsProperty#getBusinessProblem}
* @param businessProblem The specific business problem that the model is trying to solve.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder businessProblem(java.lang.String businessProblem) {
this.businessProblem = businessProblem;
return this;
}
/**
* Sets the value of {@link BusinessDetailsProperty#getBusinessStakeholders}
* @param businessStakeholders The relevant stakeholders for the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder businessStakeholders(java.lang.String businessStakeholders) {
this.businessStakeholders = businessStakeholders;
return this;
}
/**
* Sets the value of {@link BusinessDetailsProperty#getLineOfBusiness}
* @param lineOfBusiness The broader business need that the model is serving.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder lineOfBusiness(java.lang.String lineOfBusiness) {
this.lineOfBusiness = lineOfBusiness;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link BusinessDetailsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public BusinessDetailsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link BusinessDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements BusinessDetailsProperty {
private final java.lang.String businessProblem;
private final java.lang.String businessStakeholders;
private final java.lang.String lineOfBusiness;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.businessProblem = software.amazon.jsii.Kernel.get(this, "businessProblem", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.businessStakeholders = software.amazon.jsii.Kernel.get(this, "businessStakeholders", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.lineOfBusiness = software.amazon.jsii.Kernel.get(this, "lineOfBusiness", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.businessProblem = builder.businessProblem;
this.businessStakeholders = builder.businessStakeholders;
this.lineOfBusiness = builder.lineOfBusiness;
}
@Override
public final java.lang.String getBusinessProblem() {
return this.businessProblem;
}
@Override
public final java.lang.String getBusinessStakeholders() {
return this.businessStakeholders;
}
@Override
public final java.lang.String getLineOfBusiness() {
return this.lineOfBusiness;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getBusinessProblem() != null) {
data.set("businessProblem", om.valueToTree(this.getBusinessProblem()));
}
if (this.getBusinessStakeholders() != null) {
data.set("businessStakeholders", om.valueToTree(this.getBusinessStakeholders()));
}
if (this.getLineOfBusiness() != null) {
data.set("lineOfBusiness", om.valueToTree(this.getLineOfBusiness()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.BusinessDetailsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
BusinessDetailsProperty.Jsii$Proxy that = (BusinessDetailsProperty.Jsii$Proxy) o;
if (this.businessProblem != null ? !this.businessProblem.equals(that.businessProblem) : that.businessProblem != null) return false;
if (this.businessStakeholders != null ? !this.businessStakeholders.equals(that.businessStakeholders) : that.businessStakeholders != null) return false;
return this.lineOfBusiness != null ? this.lineOfBusiness.equals(that.lineOfBusiness) : that.lineOfBusiness == null;
}
@Override
public final int hashCode() {
int result = this.businessProblem != null ? this.businessProblem.hashCode() : 0;
result = 31 * result + (this.businessStakeholders != null ? this.businessStakeholders.hashCode() : 0);
result = 31 * result + (this.lineOfBusiness != null ? this.lineOfBusiness.hashCode() : 0);
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* ContainerProperty containerProperty = ContainerProperty.builder()
* .image("image")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .nearestModelName("nearestModelName")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.ContainerProperty")
@software.amazon.jsii.Jsii.Proxy(ContainerProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ContainerProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnModelCard.ContainerProperty.Image`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getImage();
/**
* `CfnModelCard.ContainerProperty.ModelDataUrl`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelDataUrl() {
return null;
}
/**
* `CfnModelCard.ContainerProperty.NearestModelName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getNearestModelName() {
return null;
}
/**
* @return a {@link Builder} of {@link ContainerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ContainerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String image;
java.lang.String modelDataUrl;
java.lang.String nearestModelName;
/**
* Sets the value of {@link ContainerProperty#getImage}
* @param image `CfnModelCard.ContainerProperty.Image`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder image(java.lang.String image) {
this.image = image;
return this;
}
/**
* Sets the value of {@link ContainerProperty#getModelDataUrl}
* @param modelDataUrl `CfnModelCard.ContainerProperty.ModelDataUrl`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelDataUrl(java.lang.String modelDataUrl) {
this.modelDataUrl = modelDataUrl;
return this;
}
/**
* Sets the value of {@link ContainerProperty#getNearestModelName}
* @param nearestModelName `CfnModelCard.ContainerProperty.NearestModelName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder nearestModelName(java.lang.String nearestModelName) {
this.nearestModelName = nearestModelName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ContainerProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ContainerProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ContainerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ContainerProperty {
private final java.lang.String image;
private final java.lang.String modelDataUrl;
private final java.lang.String nearestModelName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.image = software.amazon.jsii.Kernel.get(this, "image", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelDataUrl = software.amazon.jsii.Kernel.get(this, "modelDataUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.nearestModelName = software.amazon.jsii.Kernel.get(this, "nearestModelName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.image = java.util.Objects.requireNonNull(builder.image, "image is required");
this.modelDataUrl = builder.modelDataUrl;
this.nearestModelName = builder.nearestModelName;
}
@Override
public final java.lang.String getImage() {
return this.image;
}
@Override
public final java.lang.String getModelDataUrl() {
return this.modelDataUrl;
}
@Override
public final java.lang.String getNearestModelName() {
return this.nearestModelName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("image", om.valueToTree(this.getImage()));
if (this.getModelDataUrl() != null) {
data.set("modelDataUrl", om.valueToTree(this.getModelDataUrl()));
}
if (this.getNearestModelName() != null) {
data.set("nearestModelName", om.valueToTree(this.getNearestModelName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.ContainerProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ContainerProperty.Jsii$Proxy that = (ContainerProperty.Jsii$Proxy) o;
if (!image.equals(that.image)) return false;
if (this.modelDataUrl != null ? !this.modelDataUrl.equals(that.modelDataUrl) : that.modelDataUrl != null) return false;
return this.nearestModelName != null ? this.nearestModelName.equals(that.nearestModelName) : that.nearestModelName == null;
}
@Override
public final int hashCode() {
int result = this.image.hashCode();
result = 31 * result + (this.modelDataUrl != null ? this.modelDataUrl.hashCode() : 0);
result = 31 * result + (this.nearestModelName != null ? this.nearestModelName.hashCode() : 0);
return result;
}
}
}
/**
* The content of the model card.
*
* It follows the model card json schema .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* Object value;
* ContentProperty contentProperty = ContentProperty.builder()
* .additionalInformation(AdditionalInformationProperty.builder()
* .caveatsAndRecommendations("caveatsAndRecommendations")
* .customDetails(Map.of(
* "customDetailsKey", "customDetails"))
* .ethicalConsiderations("ethicalConsiderations")
* .build())
* .businessDetails(BusinessDetailsProperty.builder()
* .businessProblem("businessProblem")
* .businessStakeholders("businessStakeholders")
* .lineOfBusiness("lineOfBusiness")
* .build())
* .evaluationDetails(List.of(EvaluationDetailProperty.builder()
* .name("name")
* // the properties below are optional
* .datasets(List.of("datasets"))
* .evaluationJobArn("evaluationJobArn")
* .evaluationObservation("evaluationObservation")
* .metadata(Map.of(
* "metadataKey", "metadata"))
* .metricGroups(List.of(MetricGroupProperty.builder()
* .metricData(List.of(MetricDataItemsProperty.builder()
* .name("name")
* .type("type")
* .value(value)
* // the properties below are optional
* .notes("notes")
* .xAxisName(List.of("xAxisName"))
* .yAxisName(List.of("yAxisName"))
* .build()))
* .name("name")
* .build()))
* .build()))
* .intendedUses(IntendedUsesProperty.builder()
* .explanationsForRiskRating("explanationsForRiskRating")
* .factorsAffectingModelEfficiency("factorsAffectingModelEfficiency")
* .intendedUses("intendedUses")
* .purposeOfModel("purposeOfModel")
* .riskRating("riskRating")
* .build())
* .modelOverview(ModelOverviewProperty.builder()
* .algorithmType("algorithmType")
* .inferenceEnvironment(InferenceEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .modelArtifact(List.of("modelArtifact"))
* .modelCreator("modelCreator")
* .modelDescription("modelDescription")
* .modelId("modelId")
* .modelName("modelName")
* .modelOwner("modelOwner")
* .modelVersion(123)
* .problemType("problemType")
* .build())
* .modelPackageDetails(ModelPackageDetailsProperty.builder()
* .approvalDescription("approvalDescription")
* .createdBy(ModelPackageCreatorProperty.builder()
* .userProfileName("userProfileName")
* .build())
* .domain("domain")
* .inferenceSpecification(InferenceSpecificationProperty.builder()
* .containers(List.of(ContainerProperty.builder()
* .image("image")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .nearestModelName("nearestModelName")
* .build()))
* .build())
* .modelApprovalStatus("modelApprovalStatus")
* .modelPackageArn("modelPackageArn")
* .modelPackageDescription("modelPackageDescription")
* .modelPackageGroupName("modelPackageGroupName")
* .modelPackageName("modelPackageName")
* .modelPackageStatus("modelPackageStatus")
* .modelPackageVersion(123)
* .sourceAlgorithms(List.of(SourceAlgorithmProperty.builder()
* .algorithmName("algorithmName")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .build()))
* .task("task")
* .build())
* .trainingDetails(TrainingDetailsProperty.builder()
* .objectiveFunction(ObjectiveFunctionProperty.builder()
* .function(FunctionProperty.builder()
* .condition("condition")
* .facet("facet")
* .function("function")
* .build())
* .notes("notes")
* .build())
* .trainingJobDetails(TrainingJobDetailsProperty.builder()
* .hyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .trainingArn("trainingArn")
* .trainingDatasets(List.of("trainingDatasets"))
* .trainingEnvironment(TrainingEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .trainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .userProvidedHyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .userProvidedTrainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .build())
* .trainingObservations("trainingObservations")
* .build())
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.ContentProperty")
@software.amazon.jsii.Jsii.Proxy(ContentProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ContentProperty extends software.amazon.jsii.JsiiSerializable {
/**
* Additional information about the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getAdditionalInformation() {
return null;
}
/**
* Information about how the model supports business goals.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getBusinessDetails() {
return null;
}
/**
* An overview about the model's evaluation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEvaluationDetails() {
return null;
}
/**
* The intended usage of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getIntendedUses() {
return null;
}
/**
* An overview about the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getModelOverview() {
return null;
}
/**
* `CfnModelCard.ContentProperty.ModelPackageDetails`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getModelPackageDetails() {
return null;
}
/**
* An overview about model training.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getTrainingDetails() {
return null;
}
/**
* @return a {@link Builder} of {@link ContentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ContentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object additionalInformation;
java.lang.Object businessDetails;
java.lang.Object evaluationDetails;
java.lang.Object intendedUses;
java.lang.Object modelOverview;
java.lang.Object modelPackageDetails;
java.lang.Object trainingDetails;
/**
* Sets the value of {@link ContentProperty#getAdditionalInformation}
* @param additionalInformation Additional information about the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder additionalInformation(software.amazon.awscdk.core.IResolvable additionalInformation) {
this.additionalInformation = additionalInformation;
return this;
}
/**
* Sets the value of {@link ContentProperty#getAdditionalInformation}
* @param additionalInformation Additional information about the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder additionalInformation(software.amazon.awscdk.services.sagemaker.CfnModelCard.AdditionalInformationProperty additionalInformation) {
this.additionalInformation = additionalInformation;
return this;
}
/**
* Sets the value of {@link ContentProperty#getBusinessDetails}
* @param businessDetails Information about how the model supports business goals.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder businessDetails(software.amazon.awscdk.core.IResolvable businessDetails) {
this.businessDetails = businessDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getBusinessDetails}
* @param businessDetails Information about how the model supports business goals.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder businessDetails(software.amazon.awscdk.services.sagemaker.CfnModelCard.BusinessDetailsProperty businessDetails) {
this.businessDetails = businessDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getEvaluationDetails}
* @param evaluationDetails An overview about the model's evaluation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder evaluationDetails(software.amazon.awscdk.core.IResolvable evaluationDetails) {
this.evaluationDetails = evaluationDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getEvaluationDetails}
* @param evaluationDetails An overview about the model's evaluation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder evaluationDetails(java.util.List extends java.lang.Object> evaluationDetails) {
this.evaluationDetails = evaluationDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getIntendedUses}
* @param intendedUses The intended usage of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder intendedUses(software.amazon.awscdk.core.IResolvable intendedUses) {
this.intendedUses = intendedUses;
return this;
}
/**
* Sets the value of {@link ContentProperty#getIntendedUses}
* @param intendedUses The intended usage of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder intendedUses(software.amazon.awscdk.services.sagemaker.CfnModelCard.IntendedUsesProperty intendedUses) {
this.intendedUses = intendedUses;
return this;
}
/**
* Sets the value of {@link ContentProperty#getModelOverview}
* @param modelOverview An overview about the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelOverview(software.amazon.awscdk.core.IResolvable modelOverview) {
this.modelOverview = modelOverview;
return this;
}
/**
* Sets the value of {@link ContentProperty#getModelOverview}
* @param modelOverview An overview about the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelOverview(software.amazon.awscdk.services.sagemaker.CfnModelCard.ModelOverviewProperty modelOverview) {
this.modelOverview = modelOverview;
return this;
}
/**
* Sets the value of {@link ContentProperty#getModelPackageDetails}
* @param modelPackageDetails `CfnModelCard.ContentProperty.ModelPackageDetails`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageDetails(software.amazon.awscdk.core.IResolvable modelPackageDetails) {
this.modelPackageDetails = modelPackageDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getModelPackageDetails}
* @param modelPackageDetails `CfnModelCard.ContentProperty.ModelPackageDetails`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageDetails(software.amazon.awscdk.services.sagemaker.CfnModelCard.ModelPackageDetailsProperty modelPackageDetails) {
this.modelPackageDetails = modelPackageDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getTrainingDetails}
* @param trainingDetails An overview about model training.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingDetails(software.amazon.awscdk.core.IResolvable trainingDetails) {
this.trainingDetails = trainingDetails;
return this;
}
/**
* Sets the value of {@link ContentProperty#getTrainingDetails}
* @param trainingDetails An overview about model training.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingDetails(software.amazon.awscdk.services.sagemaker.CfnModelCard.TrainingDetailsProperty trainingDetails) {
this.trainingDetails = trainingDetails;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ContentProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ContentProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ContentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ContentProperty {
private final java.lang.Object additionalInformation;
private final java.lang.Object businessDetails;
private final java.lang.Object evaluationDetails;
private final java.lang.Object intendedUses;
private final java.lang.Object modelOverview;
private final java.lang.Object modelPackageDetails;
private final java.lang.Object trainingDetails;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.additionalInformation = software.amazon.jsii.Kernel.get(this, "additionalInformation", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.businessDetails = software.amazon.jsii.Kernel.get(this, "businessDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.evaluationDetails = software.amazon.jsii.Kernel.get(this, "evaluationDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.intendedUses = software.amazon.jsii.Kernel.get(this, "intendedUses", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.modelOverview = software.amazon.jsii.Kernel.get(this, "modelOverview", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.modelPackageDetails = software.amazon.jsii.Kernel.get(this, "modelPackageDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.trainingDetails = software.amazon.jsii.Kernel.get(this, "trainingDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.additionalInformation = builder.additionalInformation;
this.businessDetails = builder.businessDetails;
this.evaluationDetails = builder.evaluationDetails;
this.intendedUses = builder.intendedUses;
this.modelOverview = builder.modelOverview;
this.modelPackageDetails = builder.modelPackageDetails;
this.trainingDetails = builder.trainingDetails;
}
@Override
public final java.lang.Object getAdditionalInformation() {
return this.additionalInformation;
}
@Override
public final java.lang.Object getBusinessDetails() {
return this.businessDetails;
}
@Override
public final java.lang.Object getEvaluationDetails() {
return this.evaluationDetails;
}
@Override
public final java.lang.Object getIntendedUses() {
return this.intendedUses;
}
@Override
public final java.lang.Object getModelOverview() {
return this.modelOverview;
}
@Override
public final java.lang.Object getModelPackageDetails() {
return this.modelPackageDetails;
}
@Override
public final java.lang.Object getTrainingDetails() {
return this.trainingDetails;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAdditionalInformation() != null) {
data.set("additionalInformation", om.valueToTree(this.getAdditionalInformation()));
}
if (this.getBusinessDetails() != null) {
data.set("businessDetails", om.valueToTree(this.getBusinessDetails()));
}
if (this.getEvaluationDetails() != null) {
data.set("evaluationDetails", om.valueToTree(this.getEvaluationDetails()));
}
if (this.getIntendedUses() != null) {
data.set("intendedUses", om.valueToTree(this.getIntendedUses()));
}
if (this.getModelOverview() != null) {
data.set("modelOverview", om.valueToTree(this.getModelOverview()));
}
if (this.getModelPackageDetails() != null) {
data.set("modelPackageDetails", om.valueToTree(this.getModelPackageDetails()));
}
if (this.getTrainingDetails() != null) {
data.set("trainingDetails", om.valueToTree(this.getTrainingDetails()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.ContentProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ContentProperty.Jsii$Proxy that = (ContentProperty.Jsii$Proxy) o;
if (this.additionalInformation != null ? !this.additionalInformation.equals(that.additionalInformation) : that.additionalInformation != null) return false;
if (this.businessDetails != null ? !this.businessDetails.equals(that.businessDetails) : that.businessDetails != null) return false;
if (this.evaluationDetails != null ? !this.evaluationDetails.equals(that.evaluationDetails) : that.evaluationDetails != null) return false;
if (this.intendedUses != null ? !this.intendedUses.equals(that.intendedUses) : that.intendedUses != null) return false;
if (this.modelOverview != null ? !this.modelOverview.equals(that.modelOverview) : that.modelOverview != null) return false;
if (this.modelPackageDetails != null ? !this.modelPackageDetails.equals(that.modelPackageDetails) : that.modelPackageDetails != null) return false;
return this.trainingDetails != null ? this.trainingDetails.equals(that.trainingDetails) : that.trainingDetails == null;
}
@Override
public final int hashCode() {
int result = this.additionalInformation != null ? this.additionalInformation.hashCode() : 0;
result = 31 * result + (this.businessDetails != null ? this.businessDetails.hashCode() : 0);
result = 31 * result + (this.evaluationDetails != null ? this.evaluationDetails.hashCode() : 0);
result = 31 * result + (this.intendedUses != null ? this.intendedUses.hashCode() : 0);
result = 31 * result + (this.modelOverview != null ? this.modelOverview.hashCode() : 0);
result = 31 * result + (this.modelPackageDetails != null ? this.modelPackageDetails.hashCode() : 0);
result = 31 * result + (this.trainingDetails != null ? this.trainingDetails.hashCode() : 0);
return result;
}
}
}
/**
* The evaluation details of the model.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* Object value;
* EvaluationDetailProperty evaluationDetailProperty = EvaluationDetailProperty.builder()
* .name("name")
* // the properties below are optional
* .datasets(List.of("datasets"))
* .evaluationJobArn("evaluationJobArn")
* .evaluationObservation("evaluationObservation")
* .metadata(Map.of(
* "metadataKey", "metadata"))
* .metricGroups(List.of(MetricGroupProperty.builder()
* .metricData(List.of(MetricDataItemsProperty.builder()
* .name("name")
* .type("type")
* .value(value)
* // the properties below are optional
* .notes("notes")
* .xAxisName(List.of("xAxisName"))
* .yAxisName(List.of("yAxisName"))
* .build()))
* .name("name")
* .build()))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.EvaluationDetailProperty")
@software.amazon.jsii.Jsii.Proxy(EvaluationDetailProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface EvaluationDetailProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The evaluation job name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* The location of the datasets used to evaluate the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getDatasets() {
return null;
}
/**
* The Amazon Resource Name (ARN) of the evaluation job.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getEvaluationJobArn() {
return null;
}
/**
* Any observations made during the model evaluation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getEvaluationObservation() {
return null;
}
/**
* Additional attributes associated with the evaluation results.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getMetadata() {
return null;
}
/**
* An evaluation Metric Group object.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getMetricGroups() {
return null;
}
/**
* @return a {@link Builder} of {@link EvaluationDetailProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link EvaluationDetailProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String name;
java.util.List datasets;
java.lang.String evaluationJobArn;
java.lang.String evaluationObservation;
java.lang.Object metadata;
java.lang.Object metricGroups;
/**
* Sets the value of {@link EvaluationDetailProperty#getName}
* @param name The evaluation job name. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getDatasets}
* @param datasets The location of the datasets used to evaluate the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder datasets(java.util.List datasets) {
this.datasets = datasets;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getEvaluationJobArn}
* @param evaluationJobArn The Amazon Resource Name (ARN) of the evaluation job.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder evaluationJobArn(java.lang.String evaluationJobArn) {
this.evaluationJobArn = evaluationJobArn;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getEvaluationObservation}
* @param evaluationObservation Any observations made during the model evaluation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder evaluationObservation(java.lang.String evaluationObservation) {
this.evaluationObservation = evaluationObservation;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getMetadata}
* @param metadata Additional attributes associated with the evaluation results.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metadata(software.amazon.awscdk.core.IResolvable metadata) {
this.metadata = metadata;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getMetadata}
* @param metadata Additional attributes associated with the evaluation results.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metadata(java.util.Map metadata) {
this.metadata = metadata;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getMetricGroups}
* @param metricGroups An evaluation Metric Group object.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metricGroups(software.amazon.awscdk.core.IResolvable metricGroups) {
this.metricGroups = metricGroups;
return this;
}
/**
* Sets the value of {@link EvaluationDetailProperty#getMetricGroups}
* @param metricGroups An evaluation Metric Group object.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metricGroups(java.util.List extends java.lang.Object> metricGroups) {
this.metricGroups = metricGroups;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link EvaluationDetailProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public EvaluationDetailProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link EvaluationDetailProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements EvaluationDetailProperty {
private final java.lang.String name;
private final java.util.List datasets;
private final java.lang.String evaluationJobArn;
private final java.lang.String evaluationObservation;
private final java.lang.Object metadata;
private final java.lang.Object metricGroups;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.datasets = software.amazon.jsii.Kernel.get(this, "datasets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.evaluationJobArn = software.amazon.jsii.Kernel.get(this, "evaluationJobArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.evaluationObservation = software.amazon.jsii.Kernel.get(this, "evaluationObservation", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.metadata = software.amazon.jsii.Kernel.get(this, "metadata", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.metricGroups = software.amazon.jsii.Kernel.get(this, "metricGroups", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
this.datasets = builder.datasets;
this.evaluationJobArn = builder.evaluationJobArn;
this.evaluationObservation = builder.evaluationObservation;
this.metadata = builder.metadata;
this.metricGroups = builder.metricGroups;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.util.List getDatasets() {
return this.datasets;
}
@Override
public final java.lang.String getEvaluationJobArn() {
return this.evaluationJobArn;
}
@Override
public final java.lang.String getEvaluationObservation() {
return this.evaluationObservation;
}
@Override
public final java.lang.Object getMetadata() {
return this.metadata;
}
@Override
public final java.lang.Object getMetricGroups() {
return this.metricGroups;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("name", om.valueToTree(this.getName()));
if (this.getDatasets() != null) {
data.set("datasets", om.valueToTree(this.getDatasets()));
}
if (this.getEvaluationJobArn() != null) {
data.set("evaluationJobArn", om.valueToTree(this.getEvaluationJobArn()));
}
if (this.getEvaluationObservation() != null) {
data.set("evaluationObservation", om.valueToTree(this.getEvaluationObservation()));
}
if (this.getMetadata() != null) {
data.set("metadata", om.valueToTree(this.getMetadata()));
}
if (this.getMetricGroups() != null) {
data.set("metricGroups", om.valueToTree(this.getMetricGroups()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.EvaluationDetailProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EvaluationDetailProperty.Jsii$Proxy that = (EvaluationDetailProperty.Jsii$Proxy) o;
if (!name.equals(that.name)) return false;
if (this.datasets != null ? !this.datasets.equals(that.datasets) : that.datasets != null) return false;
if (this.evaluationJobArn != null ? !this.evaluationJobArn.equals(that.evaluationJobArn) : that.evaluationJobArn != null) return false;
if (this.evaluationObservation != null ? !this.evaluationObservation.equals(that.evaluationObservation) : that.evaluationObservation != null) return false;
if (this.metadata != null ? !this.metadata.equals(that.metadata) : that.metadata != null) return false;
return this.metricGroups != null ? this.metricGroups.equals(that.metricGroups) : that.metricGroups == null;
}
@Override
public final int hashCode() {
int result = this.name.hashCode();
result = 31 * result + (this.datasets != null ? this.datasets.hashCode() : 0);
result = 31 * result + (this.evaluationJobArn != null ? this.evaluationJobArn.hashCode() : 0);
result = 31 * result + (this.evaluationObservation != null ? this.evaluationObservation.hashCode() : 0);
result = 31 * result + (this.metadata != null ? this.metadata.hashCode() : 0);
result = 31 * result + (this.metricGroups != null ? this.metricGroups.hashCode() : 0);
return result;
}
}
}
/**
* Function details.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* FunctionProperty functionProperty = FunctionProperty.builder()
* .condition("condition")
* .facet("facet")
* .function("function")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.FunctionProperty")
@software.amazon.jsii.Jsii.Proxy(FunctionProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface FunctionProperty extends software.amazon.jsii.JsiiSerializable {
/**
* An optional description of any conditions of your objective function metric.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCondition() {
return null;
}
/**
* The metric of the model's objective function.
*
* For example, loss or rmse . The following list shows examples of the values that you can specify for the metric:
*
*
* ACCURACY
* AUC
* LOSS
* MAE
* RMSE
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getFacet() {
return null;
}
/**
* The optimization direction of the model's objective function. You must specify one of the following values:.
*
*
* Maximize
* Minimize
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getFunction() {
return null;
}
/**
* @return a {@link Builder} of {@link FunctionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link FunctionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String condition;
java.lang.String facet;
java.lang.String function;
/**
* Sets the value of {@link FunctionProperty#getCondition}
* @param condition An optional description of any conditions of your objective function metric.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder condition(java.lang.String condition) {
this.condition = condition;
return this;
}
/**
* Sets the value of {@link FunctionProperty#getFacet}
* @param facet The metric of the model's objective function.
* For example, loss or rmse . The following list shows examples of the values that you can specify for the metric:
*
*
* ACCURACY
* AUC
* LOSS
* MAE
* RMSE
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder facet(java.lang.String facet) {
this.facet = facet;
return this;
}
/**
* Sets the value of {@link FunctionProperty#getFunction}
* @param function The optimization direction of the model's objective function. You must specify one of the following values:.
*
* Maximize
* Minimize
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder function(java.lang.String function) {
this.function = function;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link FunctionProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public FunctionProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link FunctionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements FunctionProperty {
private final java.lang.String condition;
private final java.lang.String facet;
private final java.lang.String function;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.condition = software.amazon.jsii.Kernel.get(this, "condition", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.facet = software.amazon.jsii.Kernel.get(this, "facet", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.function = software.amazon.jsii.Kernel.get(this, "function", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.condition = builder.condition;
this.facet = builder.facet;
this.function = builder.function;
}
@Override
public final java.lang.String getCondition() {
return this.condition;
}
@Override
public final java.lang.String getFacet() {
return this.facet;
}
@Override
public final java.lang.String getFunction() {
return this.function;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCondition() != null) {
data.set("condition", om.valueToTree(this.getCondition()));
}
if (this.getFacet() != null) {
data.set("facet", om.valueToTree(this.getFacet()));
}
if (this.getFunction() != null) {
data.set("function", om.valueToTree(this.getFunction()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.FunctionProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FunctionProperty.Jsii$Proxy that = (FunctionProperty.Jsii$Proxy) o;
if (this.condition != null ? !this.condition.equals(that.condition) : that.condition != null) return false;
if (this.facet != null ? !this.facet.equals(that.facet) : that.facet != null) return false;
return this.function != null ? this.function.equals(that.function) : that.function == null;
}
@Override
public final int hashCode() {
int result = this.condition != null ? this.condition.hashCode() : 0;
result = 31 * result + (this.facet != null ? this.facet.hashCode() : 0);
result = 31 * result + (this.function != null ? this.function.hashCode() : 0);
return result;
}
}
}
/**
* An overview of a model's inference environment.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* InferenceEnvironmentProperty inferenceEnvironmentProperty = InferenceEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.InferenceEnvironmentProperty")
@software.amazon.jsii.Jsii.Proxy(InferenceEnvironmentProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface InferenceEnvironmentProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The container used to run the inference environment.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getContainerImage() {
return null;
}
/**
* @return a {@link Builder} of {@link InferenceEnvironmentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link InferenceEnvironmentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List containerImage;
/**
* Sets the value of {@link InferenceEnvironmentProperty#getContainerImage}
* @param containerImage The container used to run the inference environment.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerImage(java.util.List containerImage) {
this.containerImage = containerImage;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link InferenceEnvironmentProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public InferenceEnvironmentProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link InferenceEnvironmentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements InferenceEnvironmentProperty {
private final java.util.List containerImage;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containerImage = software.amazon.jsii.Kernel.get(this, "containerImage", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containerImage = builder.containerImage;
}
@Override
public final java.util.List getContainerImage() {
return this.containerImage;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getContainerImage() != null) {
data.set("containerImage", om.valueToTree(this.getContainerImage()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.InferenceEnvironmentProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
InferenceEnvironmentProperty.Jsii$Proxy that = (InferenceEnvironmentProperty.Jsii$Proxy) o;
return this.containerImage != null ? this.containerImage.equals(that.containerImage) : that.containerImage == null;
}
@Override
public final int hashCode() {
int result = this.containerImage != null ? this.containerImage.hashCode() : 0;
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* InferenceSpecificationProperty inferenceSpecificationProperty = InferenceSpecificationProperty.builder()
* .containers(List.of(ContainerProperty.builder()
* .image("image")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .nearestModelName("nearestModelName")
* .build()))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.InferenceSpecificationProperty")
@software.amazon.jsii.Jsii.Proxy(InferenceSpecificationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface InferenceSpecificationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnModelCard.InferenceSpecificationProperty.Containers`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getContainers();
/**
* @return a {@link Builder} of {@link InferenceSpecificationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link InferenceSpecificationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object containers;
/**
* Sets the value of {@link InferenceSpecificationProperty#getContainers}
* @param containers `CfnModelCard.InferenceSpecificationProperty.Containers`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containers(software.amazon.awscdk.core.IResolvable containers) {
this.containers = containers;
return this;
}
/**
* Sets the value of {@link InferenceSpecificationProperty#getContainers}
* @param containers `CfnModelCard.InferenceSpecificationProperty.Containers`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containers(java.util.List extends java.lang.Object> containers) {
this.containers = containers;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link InferenceSpecificationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public InferenceSpecificationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link InferenceSpecificationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements InferenceSpecificationProperty {
private final java.lang.Object containers;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containers = software.amazon.jsii.Kernel.get(this, "containers", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containers = java.util.Objects.requireNonNull(builder.containers, "containers is required");
}
@Override
public final java.lang.Object getContainers() {
return this.containers;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("containers", om.valueToTree(this.getContainers()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.InferenceSpecificationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
InferenceSpecificationProperty.Jsii$Proxy that = (InferenceSpecificationProperty.Jsii$Proxy) o;
return this.containers.equals(that.containers);
}
@Override
public final int hashCode() {
int result = this.containers.hashCode();
return result;
}
}
}
/**
* The intended uses of a model.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* IntendedUsesProperty intendedUsesProperty = IntendedUsesProperty.builder()
* .explanationsForRiskRating("explanationsForRiskRating")
* .factorsAffectingModelEfficiency("factorsAffectingModelEfficiency")
* .intendedUses("intendedUses")
* .purposeOfModel("purposeOfModel")
* .riskRating("riskRating")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.IntendedUsesProperty")
@software.amazon.jsii.Jsii.Proxy(IntendedUsesProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface IntendedUsesProperty extends software.amazon.jsii.JsiiSerializable {
/**
* An explanation of why your organization categorizes the model with its risk rating.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getExplanationsForRiskRating() {
return null;
}
/**
* Factors affecting model efficacy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getFactorsAffectingModelEfficiency() {
return null;
}
/**
* The intended use cases for the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getIntendedUses() {
return null;
}
/**
* The general purpose of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getPurposeOfModel() {
return null;
}
/**
* Your organization's risk rating. You can specify one the following values as the risk rating:.
*
*
* - High
* - Medium
* - Low
* - Unknown
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRiskRating() {
return null;
}
/**
* @return a {@link Builder} of {@link IntendedUsesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link IntendedUsesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String explanationsForRiskRating;
java.lang.String factorsAffectingModelEfficiency;
java.lang.String intendedUses;
java.lang.String purposeOfModel;
java.lang.String riskRating;
/**
* Sets the value of {@link IntendedUsesProperty#getExplanationsForRiskRating}
* @param explanationsForRiskRating An explanation of why your organization categorizes the model with its risk rating.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder explanationsForRiskRating(java.lang.String explanationsForRiskRating) {
this.explanationsForRiskRating = explanationsForRiskRating;
return this;
}
/**
* Sets the value of {@link IntendedUsesProperty#getFactorsAffectingModelEfficiency}
* @param factorsAffectingModelEfficiency Factors affecting model efficacy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder factorsAffectingModelEfficiency(java.lang.String factorsAffectingModelEfficiency) {
this.factorsAffectingModelEfficiency = factorsAffectingModelEfficiency;
return this;
}
/**
* Sets the value of {@link IntendedUsesProperty#getIntendedUses}
* @param intendedUses The intended use cases for the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder intendedUses(java.lang.String intendedUses) {
this.intendedUses = intendedUses;
return this;
}
/**
* Sets the value of {@link IntendedUsesProperty#getPurposeOfModel}
* @param purposeOfModel The general purpose of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder purposeOfModel(java.lang.String purposeOfModel) {
this.purposeOfModel = purposeOfModel;
return this;
}
/**
* Sets the value of {@link IntendedUsesProperty#getRiskRating}
* @param riskRating Your organization's risk rating. You can specify one the following values as the risk rating:.
*
* - High
* - Medium
* - Low
* - Unknown
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder riskRating(java.lang.String riskRating) {
this.riskRating = riskRating;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link IntendedUsesProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public IntendedUsesProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link IntendedUsesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements IntendedUsesProperty {
private final java.lang.String explanationsForRiskRating;
private final java.lang.String factorsAffectingModelEfficiency;
private final java.lang.String intendedUses;
private final java.lang.String purposeOfModel;
private final java.lang.String riskRating;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.explanationsForRiskRating = software.amazon.jsii.Kernel.get(this, "explanationsForRiskRating", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.factorsAffectingModelEfficiency = software.amazon.jsii.Kernel.get(this, "factorsAffectingModelEfficiency", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.intendedUses = software.amazon.jsii.Kernel.get(this, "intendedUses", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.purposeOfModel = software.amazon.jsii.Kernel.get(this, "purposeOfModel", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.riskRating = software.amazon.jsii.Kernel.get(this, "riskRating", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.explanationsForRiskRating = builder.explanationsForRiskRating;
this.factorsAffectingModelEfficiency = builder.factorsAffectingModelEfficiency;
this.intendedUses = builder.intendedUses;
this.purposeOfModel = builder.purposeOfModel;
this.riskRating = builder.riskRating;
}
@Override
public final java.lang.String getExplanationsForRiskRating() {
return this.explanationsForRiskRating;
}
@Override
public final java.lang.String getFactorsAffectingModelEfficiency() {
return this.factorsAffectingModelEfficiency;
}
@Override
public final java.lang.String getIntendedUses() {
return this.intendedUses;
}
@Override
public final java.lang.String getPurposeOfModel() {
return this.purposeOfModel;
}
@Override
public final java.lang.String getRiskRating() {
return this.riskRating;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getExplanationsForRiskRating() != null) {
data.set("explanationsForRiskRating", om.valueToTree(this.getExplanationsForRiskRating()));
}
if (this.getFactorsAffectingModelEfficiency() != null) {
data.set("factorsAffectingModelEfficiency", om.valueToTree(this.getFactorsAffectingModelEfficiency()));
}
if (this.getIntendedUses() != null) {
data.set("intendedUses", om.valueToTree(this.getIntendedUses()));
}
if (this.getPurposeOfModel() != null) {
data.set("purposeOfModel", om.valueToTree(this.getPurposeOfModel()));
}
if (this.getRiskRating() != null) {
data.set("riskRating", om.valueToTree(this.getRiskRating()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.IntendedUsesProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
IntendedUsesProperty.Jsii$Proxy that = (IntendedUsesProperty.Jsii$Proxy) o;
if (this.explanationsForRiskRating != null ? !this.explanationsForRiskRating.equals(that.explanationsForRiskRating) : that.explanationsForRiskRating != null) return false;
if (this.factorsAffectingModelEfficiency != null ? !this.factorsAffectingModelEfficiency.equals(that.factorsAffectingModelEfficiency) : that.factorsAffectingModelEfficiency != null) return false;
if (this.intendedUses != null ? !this.intendedUses.equals(that.intendedUses) : that.intendedUses != null) return false;
if (this.purposeOfModel != null ? !this.purposeOfModel.equals(that.purposeOfModel) : that.purposeOfModel != null) return false;
return this.riskRating != null ? this.riskRating.equals(that.riskRating) : that.riskRating == null;
}
@Override
public final int hashCode() {
int result = this.explanationsForRiskRating != null ? this.explanationsForRiskRating.hashCode() : 0;
result = 31 * result + (this.factorsAffectingModelEfficiency != null ? this.factorsAffectingModelEfficiency.hashCode() : 0);
result = 31 * result + (this.intendedUses != null ? this.intendedUses.hashCode() : 0);
result = 31 * result + (this.purposeOfModel != null ? this.purposeOfModel.hashCode() : 0);
result = 31 * result + (this.riskRating != null ? this.riskRating.hashCode() : 0);
return result;
}
}
}
/**
* Metric data.
*
* The type
determines the data types that you specify for value
, XAxisName
and YAxisName
. For information about specifying values for metrics, see model card JSON schema .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* Object value;
* MetricDataItemsProperty metricDataItemsProperty = MetricDataItemsProperty.builder()
* .name("name")
* .type("type")
* .value(value)
* // the properties below are optional
* .notes("notes")
* .xAxisName(List.of("xAxisName"))
* .yAxisName(List.of("yAxisName"))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.MetricDataItemsProperty")
@software.amazon.jsii.Jsii.Proxy(MetricDataItemsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface MetricDataItemsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The names of the metrics.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* You must specify one of the following data types:.
*
*
* - Bar Chart
bar_char
* - Boolean
boolean
* - Linear Graph
linear_graph
* - Matrix
matrix
* - Number
number
* - String
string
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getType();
/**
* The datatype of the metric.
*
* The metric's value must be compatible with the metric's type .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getValue();
/**
* Any notes to add to the metric.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getNotes() {
return null;
}
/**
* The name of the x axis.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getXAxisName() {
return null;
}
/**
* The name of the y axis.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getYAxisName() {
return null;
}
/**
* @return a {@link Builder} of {@link MetricDataItemsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link MetricDataItemsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String name;
java.lang.String type;
java.lang.Object value;
java.lang.String notes;
java.util.List xAxisName;
java.util.List yAxisName;
/**
* Sets the value of {@link MetricDataItemsProperty#getName}
* @param name The names of the metrics. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link MetricDataItemsProperty#getType}
* @param type You must specify one of the following data types:. This parameter is required.
*
* - Bar Chart
bar_char
* - Boolean
boolean
* - Linear Graph
linear_graph
* - Matrix
matrix
* - Number
number
* - String
string
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link MetricDataItemsProperty#getValue}
* @param value The datatype of the metric. This parameter is required.
* The metric's value must be compatible with the metric's type .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.Object value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link MetricDataItemsProperty#getNotes}
* @param notes Any notes to add to the metric.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder notes(java.lang.String notes) {
this.notes = notes;
return this;
}
/**
* Sets the value of {@link MetricDataItemsProperty#getXAxisName}
* @param xAxisName The name of the x axis.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder xAxisName(java.util.List xAxisName) {
this.xAxisName = xAxisName;
return this;
}
/**
* Sets the value of {@link MetricDataItemsProperty#getYAxisName}
* @param yAxisName The name of the y axis.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder yAxisName(java.util.List yAxisName) {
this.yAxisName = yAxisName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link MetricDataItemsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public MetricDataItemsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link MetricDataItemsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements MetricDataItemsProperty {
private final java.lang.String name;
private final java.lang.String type;
private final java.lang.Object value;
private final java.lang.String notes;
private final java.util.List xAxisName;
private final java.util.List yAxisName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.notes = software.amazon.jsii.Kernel.get(this, "notes", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.xAxisName = software.amazon.jsii.Kernel.get(this, "xAxisName", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.yAxisName = software.amazon.jsii.Kernel.get(this, "yAxisName", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
this.type = java.util.Objects.requireNonNull(builder.type, "type is required");
this.value = java.util.Objects.requireNonNull(builder.value, "value is required");
this.notes = builder.notes;
this.xAxisName = builder.xAxisName;
this.yAxisName = builder.yAxisName;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.lang.String getType() {
return this.type;
}
@Override
public final java.lang.Object getValue() {
return this.value;
}
@Override
public final java.lang.String getNotes() {
return this.notes;
}
@Override
public final java.util.List getXAxisName() {
return this.xAxisName;
}
@Override
public final java.util.List getYAxisName() {
return this.yAxisName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("name", om.valueToTree(this.getName()));
data.set("type", om.valueToTree(this.getType()));
data.set("value", om.valueToTree(this.getValue()));
if (this.getNotes() != null) {
data.set("notes", om.valueToTree(this.getNotes()));
}
if (this.getXAxisName() != null) {
data.set("xAxisName", om.valueToTree(this.getXAxisName()));
}
if (this.getYAxisName() != null) {
data.set("yAxisName", om.valueToTree(this.getYAxisName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.MetricDataItemsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MetricDataItemsProperty.Jsii$Proxy that = (MetricDataItemsProperty.Jsii$Proxy) o;
if (!name.equals(that.name)) return false;
if (!type.equals(that.type)) return false;
if (!value.equals(that.value)) return false;
if (this.notes != null ? !this.notes.equals(that.notes) : that.notes != null) return false;
if (this.xAxisName != null ? !this.xAxisName.equals(that.xAxisName) : that.xAxisName != null) return false;
return this.yAxisName != null ? this.yAxisName.equals(that.yAxisName) : that.yAxisName == null;
}
@Override
public final int hashCode() {
int result = this.name.hashCode();
result = 31 * result + (this.type.hashCode());
result = 31 * result + (this.value.hashCode());
result = 31 * result + (this.notes != null ? this.notes.hashCode() : 0);
result = 31 * result + (this.xAxisName != null ? this.xAxisName.hashCode() : 0);
result = 31 * result + (this.yAxisName != null ? this.yAxisName.hashCode() : 0);
return result;
}
}
}
/**
* A group of metric data that you use to initialize a metric group object.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* Object value;
* MetricGroupProperty metricGroupProperty = MetricGroupProperty.builder()
* .metricData(List.of(MetricDataItemsProperty.builder()
* .name("name")
* .type("type")
* .value(value)
* // the properties below are optional
* .notes("notes")
* .xAxisName(List.of("xAxisName"))
* .yAxisName(List.of("yAxisName"))
* .build()))
* .name("name")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.MetricGroupProperty")
@software.amazon.jsii.Jsii.Proxy(MetricGroupProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface MetricGroupProperty extends software.amazon.jsii.JsiiSerializable {
/**
* A list of metric objects. The `MetricDataItems` list can have one of the following values:.
*
*
* bar_chart_metric
* matrix_metric
* simple_metric
* linear_graph_metric
*
*
* For more information about the metric schema, see the definition section of the model card JSON schema .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getMetricData();
/**
* The metric group name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* @return a {@link Builder} of {@link MetricGroupProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link MetricGroupProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object metricData;
java.lang.String name;
/**
* Sets the value of {@link MetricGroupProperty#getMetricData}
* @param metricData A list of metric objects. The `MetricDataItems` list can have one of the following values:. This parameter is required.
*
* bar_chart_metric
* matrix_metric
* simple_metric
* linear_graph_metric
*
*
* For more information about the metric schema, see the definition section of the model card JSON schema .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metricData(software.amazon.awscdk.core.IResolvable metricData) {
this.metricData = metricData;
return this;
}
/**
* Sets the value of {@link MetricGroupProperty#getMetricData}
* @param metricData A list of metric objects. The `MetricDataItems` list can have one of the following values:. This parameter is required.
*
* bar_chart_metric
* matrix_metric
* simple_metric
* linear_graph_metric
*
*
* For more information about the metric schema, see the definition section of the model card JSON schema .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metricData(java.util.List extends java.lang.Object> metricData) {
this.metricData = metricData;
return this;
}
/**
* Sets the value of {@link MetricGroupProperty#getName}
* @param name The metric group name. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link MetricGroupProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public MetricGroupProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link MetricGroupProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements MetricGroupProperty {
private final java.lang.Object metricData;
private final java.lang.String name;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.metricData = software.amazon.jsii.Kernel.get(this, "metricData", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.metricData = java.util.Objects.requireNonNull(builder.metricData, "metricData is required");
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
}
@Override
public final java.lang.Object getMetricData() {
return this.metricData;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("metricData", om.valueToTree(this.getMetricData()));
data.set("name", om.valueToTree(this.getName()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.MetricGroupProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MetricGroupProperty.Jsii$Proxy that = (MetricGroupProperty.Jsii$Proxy) o;
if (!metricData.equals(that.metricData)) return false;
return this.name.equals(that.name);
}
@Override
public final int hashCode() {
int result = this.metricData.hashCode();
result = 31 * result + (this.name.hashCode());
return result;
}
}
}
/**
* An overview about the model.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* ModelOverviewProperty modelOverviewProperty = ModelOverviewProperty.builder()
* .algorithmType("algorithmType")
* .inferenceEnvironment(InferenceEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .modelArtifact(List.of("modelArtifact"))
* .modelCreator("modelCreator")
* .modelDescription("modelDescription")
* .modelId("modelId")
* .modelName("modelName")
* .modelOwner("modelOwner")
* .modelVersion(123)
* .problemType("problemType")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.ModelOverviewProperty")
@software.amazon.jsii.Jsii.Proxy(ModelOverviewProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ModelOverviewProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The algorithm used to solve the problem.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAlgorithmType() {
return null;
}
/**
* An overview about model inference.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getInferenceEnvironment() {
return null;
}
/**
* The location of the model artifact.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getModelArtifact() {
return null;
}
/**
* The creator of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelCreator() {
return null;
}
/**
* A description of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelDescription() {
return null;
}
/**
* The SageMaker Model ARN or non- SageMaker Model ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelId() {
return null;
}
/**
* The name of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelName() {
return null;
}
/**
* The owner of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelOwner() {
return null;
}
/**
* The version of the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getModelVersion() {
return null;
}
/**
* The problem being solved with the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProblemType() {
return null;
}
/**
* @return a {@link Builder} of {@link ModelOverviewProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ModelOverviewProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String algorithmType;
java.lang.Object inferenceEnvironment;
java.util.List modelArtifact;
java.lang.String modelCreator;
java.lang.String modelDescription;
java.lang.String modelId;
java.lang.String modelName;
java.lang.String modelOwner;
java.lang.Number modelVersion;
java.lang.String problemType;
/**
* Sets the value of {@link ModelOverviewProperty#getAlgorithmType}
* @param algorithmType The algorithm used to solve the problem.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder algorithmType(java.lang.String algorithmType) {
this.algorithmType = algorithmType;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getInferenceEnvironment}
* @param inferenceEnvironment An overview about model inference.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inferenceEnvironment(software.amazon.awscdk.core.IResolvable inferenceEnvironment) {
this.inferenceEnvironment = inferenceEnvironment;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getInferenceEnvironment}
* @param inferenceEnvironment An overview about model inference.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inferenceEnvironment(software.amazon.awscdk.services.sagemaker.CfnModelCard.InferenceEnvironmentProperty inferenceEnvironment) {
this.inferenceEnvironment = inferenceEnvironment;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelArtifact}
* @param modelArtifact The location of the model artifact.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelArtifact(java.util.List modelArtifact) {
this.modelArtifact = modelArtifact;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelCreator}
* @param modelCreator The creator of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelCreator(java.lang.String modelCreator) {
this.modelCreator = modelCreator;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelDescription}
* @param modelDescription A description of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelDescription(java.lang.String modelDescription) {
this.modelDescription = modelDescription;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelId}
* @param modelId The SageMaker Model ARN or non- SageMaker Model ID.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelId(java.lang.String modelId) {
this.modelId = modelId;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelName}
* @param modelName The name of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelName(java.lang.String modelName) {
this.modelName = modelName;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelOwner}
* @param modelOwner The owner of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelOwner(java.lang.String modelOwner) {
this.modelOwner = modelOwner;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getModelVersion}
* @param modelVersion The version of the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelVersion(java.lang.Number modelVersion) {
this.modelVersion = modelVersion;
return this;
}
/**
* Sets the value of {@link ModelOverviewProperty#getProblemType}
* @param problemType The problem being solved with the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder problemType(java.lang.String problemType) {
this.problemType = problemType;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ModelOverviewProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ModelOverviewProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ModelOverviewProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ModelOverviewProperty {
private final java.lang.String algorithmType;
private final java.lang.Object inferenceEnvironment;
private final java.util.List modelArtifact;
private final java.lang.String modelCreator;
private final java.lang.String modelDescription;
private final java.lang.String modelId;
private final java.lang.String modelName;
private final java.lang.String modelOwner;
private final java.lang.Number modelVersion;
private final java.lang.String problemType;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.algorithmType = software.amazon.jsii.Kernel.get(this, "algorithmType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.inferenceEnvironment = software.amazon.jsii.Kernel.get(this, "inferenceEnvironment", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.modelArtifact = software.amazon.jsii.Kernel.get(this, "modelArtifact", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.modelCreator = software.amazon.jsii.Kernel.get(this, "modelCreator", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelDescription = software.amazon.jsii.Kernel.get(this, "modelDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelId = software.amazon.jsii.Kernel.get(this, "modelId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelName = software.amazon.jsii.Kernel.get(this, "modelName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelOwner = software.amazon.jsii.Kernel.get(this, "modelOwner", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelVersion = software.amazon.jsii.Kernel.get(this, "modelVersion", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.problemType = software.amazon.jsii.Kernel.get(this, "problemType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.algorithmType = builder.algorithmType;
this.inferenceEnvironment = builder.inferenceEnvironment;
this.modelArtifact = builder.modelArtifact;
this.modelCreator = builder.modelCreator;
this.modelDescription = builder.modelDescription;
this.modelId = builder.modelId;
this.modelName = builder.modelName;
this.modelOwner = builder.modelOwner;
this.modelVersion = builder.modelVersion;
this.problemType = builder.problemType;
}
@Override
public final java.lang.String getAlgorithmType() {
return this.algorithmType;
}
@Override
public final java.lang.Object getInferenceEnvironment() {
return this.inferenceEnvironment;
}
@Override
public final java.util.List getModelArtifact() {
return this.modelArtifact;
}
@Override
public final java.lang.String getModelCreator() {
return this.modelCreator;
}
@Override
public final java.lang.String getModelDescription() {
return this.modelDescription;
}
@Override
public final java.lang.String getModelId() {
return this.modelId;
}
@Override
public final java.lang.String getModelName() {
return this.modelName;
}
@Override
public final java.lang.String getModelOwner() {
return this.modelOwner;
}
@Override
public final java.lang.Number getModelVersion() {
return this.modelVersion;
}
@Override
public final java.lang.String getProblemType() {
return this.problemType;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAlgorithmType() != null) {
data.set("algorithmType", om.valueToTree(this.getAlgorithmType()));
}
if (this.getInferenceEnvironment() != null) {
data.set("inferenceEnvironment", om.valueToTree(this.getInferenceEnvironment()));
}
if (this.getModelArtifact() != null) {
data.set("modelArtifact", om.valueToTree(this.getModelArtifact()));
}
if (this.getModelCreator() != null) {
data.set("modelCreator", om.valueToTree(this.getModelCreator()));
}
if (this.getModelDescription() != null) {
data.set("modelDescription", om.valueToTree(this.getModelDescription()));
}
if (this.getModelId() != null) {
data.set("modelId", om.valueToTree(this.getModelId()));
}
if (this.getModelName() != null) {
data.set("modelName", om.valueToTree(this.getModelName()));
}
if (this.getModelOwner() != null) {
data.set("modelOwner", om.valueToTree(this.getModelOwner()));
}
if (this.getModelVersion() != null) {
data.set("modelVersion", om.valueToTree(this.getModelVersion()));
}
if (this.getProblemType() != null) {
data.set("problemType", om.valueToTree(this.getProblemType()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.ModelOverviewProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ModelOverviewProperty.Jsii$Proxy that = (ModelOverviewProperty.Jsii$Proxy) o;
if (this.algorithmType != null ? !this.algorithmType.equals(that.algorithmType) : that.algorithmType != null) return false;
if (this.inferenceEnvironment != null ? !this.inferenceEnvironment.equals(that.inferenceEnvironment) : that.inferenceEnvironment != null) return false;
if (this.modelArtifact != null ? !this.modelArtifact.equals(that.modelArtifact) : that.modelArtifact != null) return false;
if (this.modelCreator != null ? !this.modelCreator.equals(that.modelCreator) : that.modelCreator != null) return false;
if (this.modelDescription != null ? !this.modelDescription.equals(that.modelDescription) : that.modelDescription != null) return false;
if (this.modelId != null ? !this.modelId.equals(that.modelId) : that.modelId != null) return false;
if (this.modelName != null ? !this.modelName.equals(that.modelName) : that.modelName != null) return false;
if (this.modelOwner != null ? !this.modelOwner.equals(that.modelOwner) : that.modelOwner != null) return false;
if (this.modelVersion != null ? !this.modelVersion.equals(that.modelVersion) : that.modelVersion != null) return false;
return this.problemType != null ? this.problemType.equals(that.problemType) : that.problemType == null;
}
@Override
public final int hashCode() {
int result = this.algorithmType != null ? this.algorithmType.hashCode() : 0;
result = 31 * result + (this.inferenceEnvironment != null ? this.inferenceEnvironment.hashCode() : 0);
result = 31 * result + (this.modelArtifact != null ? this.modelArtifact.hashCode() : 0);
result = 31 * result + (this.modelCreator != null ? this.modelCreator.hashCode() : 0);
result = 31 * result + (this.modelDescription != null ? this.modelDescription.hashCode() : 0);
result = 31 * result + (this.modelId != null ? this.modelId.hashCode() : 0);
result = 31 * result + (this.modelName != null ? this.modelName.hashCode() : 0);
result = 31 * result + (this.modelOwner != null ? this.modelOwner.hashCode() : 0);
result = 31 * result + (this.modelVersion != null ? this.modelVersion.hashCode() : 0);
result = 31 * result + (this.problemType != null ? this.problemType.hashCode() : 0);
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* ModelPackageCreatorProperty modelPackageCreatorProperty = ModelPackageCreatorProperty.builder()
* .userProfileName("userProfileName")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.ModelPackageCreatorProperty")
@software.amazon.jsii.Jsii.Proxy(ModelPackageCreatorProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ModelPackageCreatorProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnModelCard.ModelPackageCreatorProperty.UserProfileName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getUserProfileName() {
return null;
}
/**
* @return a {@link Builder} of {@link ModelPackageCreatorProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ModelPackageCreatorProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String userProfileName;
/**
* Sets the value of {@link ModelPackageCreatorProperty#getUserProfileName}
* @param userProfileName `CfnModelCard.ModelPackageCreatorProperty.UserProfileName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProfileName(java.lang.String userProfileName) {
this.userProfileName = userProfileName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ModelPackageCreatorProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ModelPackageCreatorProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ModelPackageCreatorProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ModelPackageCreatorProperty {
private final java.lang.String userProfileName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.userProfileName = software.amazon.jsii.Kernel.get(this, "userProfileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.userProfileName = builder.userProfileName;
}
@Override
public final java.lang.String getUserProfileName() {
return this.userProfileName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getUserProfileName() != null) {
data.set("userProfileName", om.valueToTree(this.getUserProfileName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.ModelPackageCreatorProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ModelPackageCreatorProperty.Jsii$Proxy that = (ModelPackageCreatorProperty.Jsii$Proxy) o;
return this.userProfileName != null ? this.userProfileName.equals(that.userProfileName) : that.userProfileName == null;
}
@Override
public final int hashCode() {
int result = this.userProfileName != null ? this.userProfileName.hashCode() : 0;
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* ModelPackageDetailsProperty modelPackageDetailsProperty = ModelPackageDetailsProperty.builder()
* .approvalDescription("approvalDescription")
* .createdBy(ModelPackageCreatorProperty.builder()
* .userProfileName("userProfileName")
* .build())
* .domain("domain")
* .inferenceSpecification(InferenceSpecificationProperty.builder()
* .containers(List.of(ContainerProperty.builder()
* .image("image")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .nearestModelName("nearestModelName")
* .build()))
* .build())
* .modelApprovalStatus("modelApprovalStatus")
* .modelPackageArn("modelPackageArn")
* .modelPackageDescription("modelPackageDescription")
* .modelPackageGroupName("modelPackageGroupName")
* .modelPackageName("modelPackageName")
* .modelPackageStatus("modelPackageStatus")
* .modelPackageVersion(123)
* .sourceAlgorithms(List.of(SourceAlgorithmProperty.builder()
* .algorithmName("algorithmName")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .build()))
* .task("task")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.ModelPackageDetailsProperty")
@software.amazon.jsii.Jsii.Proxy(ModelPackageDetailsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ModelPackageDetailsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnModelCard.ModelPackageDetailsProperty.ApprovalDescription`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getApprovalDescription() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.CreatedBy`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getCreatedBy() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.Domain`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDomain() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.InferenceSpecification`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getInferenceSpecification() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelApprovalStatus`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelApprovalStatus() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelPackageArn`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelPackageArn() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelPackageDescription`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelPackageDescription() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelPackageGroupName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelPackageGroupName() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelPackageName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelPackageName() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelPackageStatus`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelPackageStatus() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.ModelPackageVersion`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getModelPackageVersion() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.SourceAlgorithms`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getSourceAlgorithms() {
return null;
}
/**
* `CfnModelCard.ModelPackageDetailsProperty.Task`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTask() {
return null;
}
/**
* @return a {@link Builder} of {@link ModelPackageDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ModelPackageDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String approvalDescription;
java.lang.Object createdBy;
java.lang.String domain;
java.lang.Object inferenceSpecification;
java.lang.String modelApprovalStatus;
java.lang.String modelPackageArn;
java.lang.String modelPackageDescription;
java.lang.String modelPackageGroupName;
java.lang.String modelPackageName;
java.lang.String modelPackageStatus;
java.lang.Number modelPackageVersion;
java.lang.Object sourceAlgorithms;
java.lang.String task;
/**
* Sets the value of {@link ModelPackageDetailsProperty#getApprovalDescription}
* @param approvalDescription `CfnModelCard.ModelPackageDetailsProperty.ApprovalDescription`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder approvalDescription(java.lang.String approvalDescription) {
this.approvalDescription = approvalDescription;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getCreatedBy}
* @param createdBy `CfnModelCard.ModelPackageDetailsProperty.CreatedBy`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder createdBy(software.amazon.awscdk.core.IResolvable createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getCreatedBy}
* @param createdBy `CfnModelCard.ModelPackageDetailsProperty.CreatedBy`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder createdBy(software.amazon.awscdk.services.sagemaker.CfnModelCard.ModelPackageCreatorProperty createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getDomain}
* @param domain `CfnModelCard.ModelPackageDetailsProperty.Domain`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domain(java.lang.String domain) {
this.domain = domain;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getInferenceSpecification}
* @param inferenceSpecification `CfnModelCard.ModelPackageDetailsProperty.InferenceSpecification`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inferenceSpecification(software.amazon.awscdk.core.IResolvable inferenceSpecification) {
this.inferenceSpecification = inferenceSpecification;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getInferenceSpecification}
* @param inferenceSpecification `CfnModelCard.ModelPackageDetailsProperty.InferenceSpecification`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inferenceSpecification(software.amazon.awscdk.services.sagemaker.CfnModelCard.InferenceSpecificationProperty inferenceSpecification) {
this.inferenceSpecification = inferenceSpecification;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelApprovalStatus}
* @param modelApprovalStatus `CfnModelCard.ModelPackageDetailsProperty.ModelApprovalStatus`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelApprovalStatus(java.lang.String modelApprovalStatus) {
this.modelApprovalStatus = modelApprovalStatus;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelPackageArn}
* @param modelPackageArn `CfnModelCard.ModelPackageDetailsProperty.ModelPackageArn`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageArn(java.lang.String modelPackageArn) {
this.modelPackageArn = modelPackageArn;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelPackageDescription}
* @param modelPackageDescription `CfnModelCard.ModelPackageDetailsProperty.ModelPackageDescription`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageDescription(java.lang.String modelPackageDescription) {
this.modelPackageDescription = modelPackageDescription;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelPackageGroupName}
* @param modelPackageGroupName `CfnModelCard.ModelPackageDetailsProperty.ModelPackageGroupName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageGroupName(java.lang.String modelPackageGroupName) {
this.modelPackageGroupName = modelPackageGroupName;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelPackageName}
* @param modelPackageName `CfnModelCard.ModelPackageDetailsProperty.ModelPackageName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageName(java.lang.String modelPackageName) {
this.modelPackageName = modelPackageName;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelPackageStatus}
* @param modelPackageStatus `CfnModelCard.ModelPackageDetailsProperty.ModelPackageStatus`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageStatus(java.lang.String modelPackageStatus) {
this.modelPackageStatus = modelPackageStatus;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getModelPackageVersion}
* @param modelPackageVersion `CfnModelCard.ModelPackageDetailsProperty.ModelPackageVersion`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelPackageVersion(java.lang.Number modelPackageVersion) {
this.modelPackageVersion = modelPackageVersion;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getSourceAlgorithms}
* @param sourceAlgorithms `CfnModelCard.ModelPackageDetailsProperty.SourceAlgorithms`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceAlgorithms(software.amazon.awscdk.core.IResolvable sourceAlgorithms) {
this.sourceAlgorithms = sourceAlgorithms;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getSourceAlgorithms}
* @param sourceAlgorithms `CfnModelCard.ModelPackageDetailsProperty.SourceAlgorithms`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceAlgorithms(java.util.List extends java.lang.Object> sourceAlgorithms) {
this.sourceAlgorithms = sourceAlgorithms;
return this;
}
/**
* Sets the value of {@link ModelPackageDetailsProperty#getTask}
* @param task `CfnModelCard.ModelPackageDetailsProperty.Task`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder task(java.lang.String task) {
this.task = task;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ModelPackageDetailsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ModelPackageDetailsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ModelPackageDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ModelPackageDetailsProperty {
private final java.lang.String approvalDescription;
private final java.lang.Object createdBy;
private final java.lang.String domain;
private final java.lang.Object inferenceSpecification;
private final java.lang.String modelApprovalStatus;
private final java.lang.String modelPackageArn;
private final java.lang.String modelPackageDescription;
private final java.lang.String modelPackageGroupName;
private final java.lang.String modelPackageName;
private final java.lang.String modelPackageStatus;
private final java.lang.Number modelPackageVersion;
private final java.lang.Object sourceAlgorithms;
private final java.lang.String task;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.approvalDescription = software.amazon.jsii.Kernel.get(this, "approvalDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.createdBy = software.amazon.jsii.Kernel.get(this, "createdBy", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.domain = software.amazon.jsii.Kernel.get(this, "domain", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.inferenceSpecification = software.amazon.jsii.Kernel.get(this, "inferenceSpecification", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.modelApprovalStatus = software.amazon.jsii.Kernel.get(this, "modelApprovalStatus", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelPackageArn = software.amazon.jsii.Kernel.get(this, "modelPackageArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelPackageDescription = software.amazon.jsii.Kernel.get(this, "modelPackageDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelPackageGroupName = software.amazon.jsii.Kernel.get(this, "modelPackageGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelPackageName = software.amazon.jsii.Kernel.get(this, "modelPackageName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelPackageStatus = software.amazon.jsii.Kernel.get(this, "modelPackageStatus", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelPackageVersion = software.amazon.jsii.Kernel.get(this, "modelPackageVersion", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.sourceAlgorithms = software.amazon.jsii.Kernel.get(this, "sourceAlgorithms", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.task = software.amazon.jsii.Kernel.get(this, "task", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.approvalDescription = builder.approvalDescription;
this.createdBy = builder.createdBy;
this.domain = builder.domain;
this.inferenceSpecification = builder.inferenceSpecification;
this.modelApprovalStatus = builder.modelApprovalStatus;
this.modelPackageArn = builder.modelPackageArn;
this.modelPackageDescription = builder.modelPackageDescription;
this.modelPackageGroupName = builder.modelPackageGroupName;
this.modelPackageName = builder.modelPackageName;
this.modelPackageStatus = builder.modelPackageStatus;
this.modelPackageVersion = builder.modelPackageVersion;
this.sourceAlgorithms = builder.sourceAlgorithms;
this.task = builder.task;
}
@Override
public final java.lang.String getApprovalDescription() {
return this.approvalDescription;
}
@Override
public final java.lang.Object getCreatedBy() {
return this.createdBy;
}
@Override
public final java.lang.String getDomain() {
return this.domain;
}
@Override
public final java.lang.Object getInferenceSpecification() {
return this.inferenceSpecification;
}
@Override
public final java.lang.String getModelApprovalStatus() {
return this.modelApprovalStatus;
}
@Override
public final java.lang.String getModelPackageArn() {
return this.modelPackageArn;
}
@Override
public final java.lang.String getModelPackageDescription() {
return this.modelPackageDescription;
}
@Override
public final java.lang.String getModelPackageGroupName() {
return this.modelPackageGroupName;
}
@Override
public final java.lang.String getModelPackageName() {
return this.modelPackageName;
}
@Override
public final java.lang.String getModelPackageStatus() {
return this.modelPackageStatus;
}
@Override
public final java.lang.Number getModelPackageVersion() {
return this.modelPackageVersion;
}
@Override
public final java.lang.Object getSourceAlgorithms() {
return this.sourceAlgorithms;
}
@Override
public final java.lang.String getTask() {
return this.task;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getApprovalDescription() != null) {
data.set("approvalDescription", om.valueToTree(this.getApprovalDescription()));
}
if (this.getCreatedBy() != null) {
data.set("createdBy", om.valueToTree(this.getCreatedBy()));
}
if (this.getDomain() != null) {
data.set("domain", om.valueToTree(this.getDomain()));
}
if (this.getInferenceSpecification() != null) {
data.set("inferenceSpecification", om.valueToTree(this.getInferenceSpecification()));
}
if (this.getModelApprovalStatus() != null) {
data.set("modelApprovalStatus", om.valueToTree(this.getModelApprovalStatus()));
}
if (this.getModelPackageArn() != null) {
data.set("modelPackageArn", om.valueToTree(this.getModelPackageArn()));
}
if (this.getModelPackageDescription() != null) {
data.set("modelPackageDescription", om.valueToTree(this.getModelPackageDescription()));
}
if (this.getModelPackageGroupName() != null) {
data.set("modelPackageGroupName", om.valueToTree(this.getModelPackageGroupName()));
}
if (this.getModelPackageName() != null) {
data.set("modelPackageName", om.valueToTree(this.getModelPackageName()));
}
if (this.getModelPackageStatus() != null) {
data.set("modelPackageStatus", om.valueToTree(this.getModelPackageStatus()));
}
if (this.getModelPackageVersion() != null) {
data.set("modelPackageVersion", om.valueToTree(this.getModelPackageVersion()));
}
if (this.getSourceAlgorithms() != null) {
data.set("sourceAlgorithms", om.valueToTree(this.getSourceAlgorithms()));
}
if (this.getTask() != null) {
data.set("task", om.valueToTree(this.getTask()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.ModelPackageDetailsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ModelPackageDetailsProperty.Jsii$Proxy that = (ModelPackageDetailsProperty.Jsii$Proxy) o;
if (this.approvalDescription != null ? !this.approvalDescription.equals(that.approvalDescription) : that.approvalDescription != null) return false;
if (this.createdBy != null ? !this.createdBy.equals(that.createdBy) : that.createdBy != null) return false;
if (this.domain != null ? !this.domain.equals(that.domain) : that.domain != null) return false;
if (this.inferenceSpecification != null ? !this.inferenceSpecification.equals(that.inferenceSpecification) : that.inferenceSpecification != null) return false;
if (this.modelApprovalStatus != null ? !this.modelApprovalStatus.equals(that.modelApprovalStatus) : that.modelApprovalStatus != null) return false;
if (this.modelPackageArn != null ? !this.modelPackageArn.equals(that.modelPackageArn) : that.modelPackageArn != null) return false;
if (this.modelPackageDescription != null ? !this.modelPackageDescription.equals(that.modelPackageDescription) : that.modelPackageDescription != null) return false;
if (this.modelPackageGroupName != null ? !this.modelPackageGroupName.equals(that.modelPackageGroupName) : that.modelPackageGroupName != null) return false;
if (this.modelPackageName != null ? !this.modelPackageName.equals(that.modelPackageName) : that.modelPackageName != null) return false;
if (this.modelPackageStatus != null ? !this.modelPackageStatus.equals(that.modelPackageStatus) : that.modelPackageStatus != null) return false;
if (this.modelPackageVersion != null ? !this.modelPackageVersion.equals(that.modelPackageVersion) : that.modelPackageVersion != null) return false;
if (this.sourceAlgorithms != null ? !this.sourceAlgorithms.equals(that.sourceAlgorithms) : that.sourceAlgorithms != null) return false;
return this.task != null ? this.task.equals(that.task) : that.task == null;
}
@Override
public final int hashCode() {
int result = this.approvalDescription != null ? this.approvalDescription.hashCode() : 0;
result = 31 * result + (this.createdBy != null ? this.createdBy.hashCode() : 0);
result = 31 * result + (this.domain != null ? this.domain.hashCode() : 0);
result = 31 * result + (this.inferenceSpecification != null ? this.inferenceSpecification.hashCode() : 0);
result = 31 * result + (this.modelApprovalStatus != null ? this.modelApprovalStatus.hashCode() : 0);
result = 31 * result + (this.modelPackageArn != null ? this.modelPackageArn.hashCode() : 0);
result = 31 * result + (this.modelPackageDescription != null ? this.modelPackageDescription.hashCode() : 0);
result = 31 * result + (this.modelPackageGroupName != null ? this.modelPackageGroupName.hashCode() : 0);
result = 31 * result + (this.modelPackageName != null ? this.modelPackageName.hashCode() : 0);
result = 31 * result + (this.modelPackageStatus != null ? this.modelPackageStatus.hashCode() : 0);
result = 31 * result + (this.modelPackageVersion != null ? this.modelPackageVersion.hashCode() : 0);
result = 31 * result + (this.sourceAlgorithms != null ? this.sourceAlgorithms.hashCode() : 0);
result = 31 * result + (this.task != null ? this.task.hashCode() : 0);
return result;
}
}
}
/**
* The function that is optimized during model training.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* ObjectiveFunctionProperty objectiveFunctionProperty = ObjectiveFunctionProperty.builder()
* .function(FunctionProperty.builder()
* .condition("condition")
* .facet("facet")
* .function("function")
* .build())
* .notes("notes")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.ObjectiveFunctionProperty")
@software.amazon.jsii.Jsii.Proxy(ObjectiveFunctionProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ObjectiveFunctionProperty extends software.amazon.jsii.JsiiSerializable {
/**
* A function object that details optimization direction, metric, and additional descriptions.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getFunction() {
return null;
}
/**
* Notes about the object function, including other considerations for possible objective functions.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getNotes() {
return null;
}
/**
* @return a {@link Builder} of {@link ObjectiveFunctionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ObjectiveFunctionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object function;
java.lang.String notes;
/**
* Sets the value of {@link ObjectiveFunctionProperty#getFunction}
* @param function A function object that details optimization direction, metric, and additional descriptions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder function(software.amazon.awscdk.core.IResolvable function) {
this.function = function;
return this;
}
/**
* Sets the value of {@link ObjectiveFunctionProperty#getFunction}
* @param function A function object that details optimization direction, metric, and additional descriptions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder function(software.amazon.awscdk.services.sagemaker.CfnModelCard.FunctionProperty function) {
this.function = function;
return this;
}
/**
* Sets the value of {@link ObjectiveFunctionProperty#getNotes}
* @param notes Notes about the object function, including other considerations for possible objective functions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder notes(java.lang.String notes) {
this.notes = notes;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ObjectiveFunctionProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ObjectiveFunctionProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ObjectiveFunctionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ObjectiveFunctionProperty {
private final java.lang.Object function;
private final java.lang.String notes;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.function = software.amazon.jsii.Kernel.get(this, "function", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.notes = software.amazon.jsii.Kernel.get(this, "notes", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.function = builder.function;
this.notes = builder.notes;
}
@Override
public final java.lang.Object getFunction() {
return this.function;
}
@Override
public final java.lang.String getNotes() {
return this.notes;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getFunction() != null) {
data.set("function", om.valueToTree(this.getFunction()));
}
if (this.getNotes() != null) {
data.set("notes", om.valueToTree(this.getNotes()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.ObjectiveFunctionProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ObjectiveFunctionProperty.Jsii$Proxy that = (ObjectiveFunctionProperty.Jsii$Proxy) o;
if (this.function != null ? !this.function.equals(that.function) : that.function != null) return false;
return this.notes != null ? this.notes.equals(that.notes) : that.notes == null;
}
@Override
public final int hashCode() {
int result = this.function != null ? this.function.hashCode() : 0;
result = 31 * result + (this.notes != null ? this.notes.hashCode() : 0);
return result;
}
}
}
/**
* The security configuration used to protect model card data.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* SecurityConfigProperty securityConfigProperty = SecurityConfigProperty.builder()
* .kmsKeyId("kmsKeyId")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.SecurityConfigProperty")
@software.amazon.jsii.Jsii.Proxy(SecurityConfigProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface SecurityConfigProperty extends software.amazon.jsii.JsiiSerializable {
/**
* A AWS Key Management Service [key ID](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#key-id-key-id) used to encrypt a model card.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getKmsKeyId() {
return null;
}
/**
* @return a {@link Builder} of {@link SecurityConfigProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SecurityConfigProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String kmsKeyId;
/**
* Sets the value of {@link SecurityConfigProperty#getKmsKeyId}
* @param kmsKeyId A AWS Key Management Service [key ID](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#key-id-key-id) used to encrypt a model card.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder kmsKeyId(java.lang.String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SecurityConfigProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public SecurityConfigProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link SecurityConfigProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements SecurityConfigProperty {
private final java.lang.String kmsKeyId;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.kmsKeyId = software.amazon.jsii.Kernel.get(this, "kmsKeyId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.kmsKeyId = builder.kmsKeyId;
}
@Override
public final java.lang.String getKmsKeyId() {
return this.kmsKeyId;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getKmsKeyId() != null) {
data.set("kmsKeyId", om.valueToTree(this.getKmsKeyId()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.SecurityConfigProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SecurityConfigProperty.Jsii$Proxy that = (SecurityConfigProperty.Jsii$Proxy) o;
return this.kmsKeyId != null ? this.kmsKeyId.equals(that.kmsKeyId) : that.kmsKeyId == null;
}
@Override
public final int hashCode() {
int result = this.kmsKeyId != null ? this.kmsKeyId.hashCode() : 0;
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* SourceAlgorithmProperty sourceAlgorithmProperty = SourceAlgorithmProperty.builder()
* .algorithmName("algorithmName")
* // the properties below are optional
* .modelDataUrl("modelDataUrl")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.SourceAlgorithmProperty")
@software.amazon.jsii.Jsii.Proxy(SourceAlgorithmProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface SourceAlgorithmProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnModelCard.SourceAlgorithmProperty.AlgorithmName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAlgorithmName();
/**
* `CfnModelCard.SourceAlgorithmProperty.ModelDataUrl`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getModelDataUrl() {
return null;
}
/**
* @return a {@link Builder} of {@link SourceAlgorithmProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SourceAlgorithmProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String algorithmName;
java.lang.String modelDataUrl;
/**
* Sets the value of {@link SourceAlgorithmProperty#getAlgorithmName}
* @param algorithmName `CfnModelCard.SourceAlgorithmProperty.AlgorithmName`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder algorithmName(java.lang.String algorithmName) {
this.algorithmName = algorithmName;
return this;
}
/**
* Sets the value of {@link SourceAlgorithmProperty#getModelDataUrl}
* @param modelDataUrl `CfnModelCard.SourceAlgorithmProperty.ModelDataUrl`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelDataUrl(java.lang.String modelDataUrl) {
this.modelDataUrl = modelDataUrl;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SourceAlgorithmProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public SourceAlgorithmProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link SourceAlgorithmProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements SourceAlgorithmProperty {
private final java.lang.String algorithmName;
private final java.lang.String modelDataUrl;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.algorithmName = software.amazon.jsii.Kernel.get(this, "algorithmName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.modelDataUrl = software.amazon.jsii.Kernel.get(this, "modelDataUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.algorithmName = java.util.Objects.requireNonNull(builder.algorithmName, "algorithmName is required");
this.modelDataUrl = builder.modelDataUrl;
}
@Override
public final java.lang.String getAlgorithmName() {
return this.algorithmName;
}
@Override
public final java.lang.String getModelDataUrl() {
return this.modelDataUrl;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("algorithmName", om.valueToTree(this.getAlgorithmName()));
if (this.getModelDataUrl() != null) {
data.set("modelDataUrl", om.valueToTree(this.getModelDataUrl()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.SourceAlgorithmProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SourceAlgorithmProperty.Jsii$Proxy that = (SourceAlgorithmProperty.Jsii$Proxy) o;
if (!algorithmName.equals(that.algorithmName)) return false;
return this.modelDataUrl != null ? this.modelDataUrl.equals(that.modelDataUrl) : that.modelDataUrl == null;
}
@Override
public final int hashCode() {
int result = this.algorithmName.hashCode();
result = 31 * result + (this.modelDataUrl != null ? this.modelDataUrl.hashCode() : 0);
return result;
}
}
}
/**
* The training details of the model.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* TrainingDetailsProperty trainingDetailsProperty = TrainingDetailsProperty.builder()
* .objectiveFunction(ObjectiveFunctionProperty.builder()
* .function(FunctionProperty.builder()
* .condition("condition")
* .facet("facet")
* .function("function")
* .build())
* .notes("notes")
* .build())
* .trainingJobDetails(TrainingJobDetailsProperty.builder()
* .hyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .trainingArn("trainingArn")
* .trainingDatasets(List.of("trainingDatasets"))
* .trainingEnvironment(TrainingEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .trainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .userProvidedHyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .userProvidedTrainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .build())
* .trainingObservations("trainingObservations")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.TrainingDetailsProperty")
@software.amazon.jsii.Jsii.Proxy(TrainingDetailsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface TrainingDetailsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The function that is optimized during model training.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getObjectiveFunction() {
return null;
}
/**
* Details about any associated training jobs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getTrainingJobDetails() {
return null;
}
/**
* Any observations about training.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTrainingObservations() {
return null;
}
/**
* @return a {@link Builder} of {@link TrainingDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TrainingDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object objectiveFunction;
java.lang.Object trainingJobDetails;
java.lang.String trainingObservations;
/**
* Sets the value of {@link TrainingDetailsProperty#getObjectiveFunction}
* @param objectiveFunction The function that is optimized during model training.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder objectiveFunction(software.amazon.awscdk.core.IResolvable objectiveFunction) {
this.objectiveFunction = objectiveFunction;
return this;
}
/**
* Sets the value of {@link TrainingDetailsProperty#getObjectiveFunction}
* @param objectiveFunction The function that is optimized during model training.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder objectiveFunction(software.amazon.awscdk.services.sagemaker.CfnModelCard.ObjectiveFunctionProperty objectiveFunction) {
this.objectiveFunction = objectiveFunction;
return this;
}
/**
* Sets the value of {@link TrainingDetailsProperty#getTrainingJobDetails}
* @param trainingJobDetails Details about any associated training jobs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingJobDetails(software.amazon.awscdk.core.IResolvable trainingJobDetails) {
this.trainingJobDetails = trainingJobDetails;
return this;
}
/**
* Sets the value of {@link TrainingDetailsProperty#getTrainingJobDetails}
* @param trainingJobDetails Details about any associated training jobs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingJobDetails(software.amazon.awscdk.services.sagemaker.CfnModelCard.TrainingJobDetailsProperty trainingJobDetails) {
this.trainingJobDetails = trainingJobDetails;
return this;
}
/**
* Sets the value of {@link TrainingDetailsProperty#getTrainingObservations}
* @param trainingObservations Any observations about training.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingObservations(java.lang.String trainingObservations) {
this.trainingObservations = trainingObservations;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TrainingDetailsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public TrainingDetailsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link TrainingDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements TrainingDetailsProperty {
private final java.lang.Object objectiveFunction;
private final java.lang.Object trainingJobDetails;
private final java.lang.String trainingObservations;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.objectiveFunction = software.amazon.jsii.Kernel.get(this, "objectiveFunction", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.trainingJobDetails = software.amazon.jsii.Kernel.get(this, "trainingJobDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.trainingObservations = software.amazon.jsii.Kernel.get(this, "trainingObservations", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.objectiveFunction = builder.objectiveFunction;
this.trainingJobDetails = builder.trainingJobDetails;
this.trainingObservations = builder.trainingObservations;
}
@Override
public final java.lang.Object getObjectiveFunction() {
return this.objectiveFunction;
}
@Override
public final java.lang.Object getTrainingJobDetails() {
return this.trainingJobDetails;
}
@Override
public final java.lang.String getTrainingObservations() {
return this.trainingObservations;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getObjectiveFunction() != null) {
data.set("objectiveFunction", om.valueToTree(this.getObjectiveFunction()));
}
if (this.getTrainingJobDetails() != null) {
data.set("trainingJobDetails", om.valueToTree(this.getTrainingJobDetails()));
}
if (this.getTrainingObservations() != null) {
data.set("trainingObservations", om.valueToTree(this.getTrainingObservations()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.TrainingDetailsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TrainingDetailsProperty.Jsii$Proxy that = (TrainingDetailsProperty.Jsii$Proxy) o;
if (this.objectiveFunction != null ? !this.objectiveFunction.equals(that.objectiveFunction) : that.objectiveFunction != null) return false;
if (this.trainingJobDetails != null ? !this.trainingJobDetails.equals(that.trainingJobDetails) : that.trainingJobDetails != null) return false;
return this.trainingObservations != null ? this.trainingObservations.equals(that.trainingObservations) : that.trainingObservations == null;
}
@Override
public final int hashCode() {
int result = this.objectiveFunction != null ? this.objectiveFunction.hashCode() : 0;
result = 31 * result + (this.trainingJobDetails != null ? this.trainingJobDetails.hashCode() : 0);
result = 31 * result + (this.trainingObservations != null ? this.trainingObservations.hashCode() : 0);
return result;
}
}
}
/**
* SageMaker training image.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* TrainingEnvironmentProperty trainingEnvironmentProperty = TrainingEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.TrainingEnvironmentProperty")
@software.amazon.jsii.Jsii.Proxy(TrainingEnvironmentProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface TrainingEnvironmentProperty extends software.amazon.jsii.JsiiSerializable {
/**
* SageMaker inference image URI.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getContainerImage() {
return null;
}
/**
* @return a {@link Builder} of {@link TrainingEnvironmentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TrainingEnvironmentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List containerImage;
/**
* Sets the value of {@link TrainingEnvironmentProperty#getContainerImage}
* @param containerImage SageMaker inference image URI.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerImage(java.util.List containerImage) {
this.containerImage = containerImage;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TrainingEnvironmentProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public TrainingEnvironmentProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link TrainingEnvironmentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements TrainingEnvironmentProperty {
private final java.util.List containerImage;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containerImage = software.amazon.jsii.Kernel.get(this, "containerImage", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containerImage = builder.containerImage;
}
@Override
public final java.util.List getContainerImage() {
return this.containerImage;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getContainerImage() != null) {
data.set("containerImage", om.valueToTree(this.getContainerImage()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.TrainingEnvironmentProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TrainingEnvironmentProperty.Jsii$Proxy that = (TrainingEnvironmentProperty.Jsii$Proxy) o;
return this.containerImage != null ? this.containerImage.equals(that.containerImage) : that.containerImage == null;
}
@Override
public final int hashCode() {
int result = this.containerImage != null ? this.containerImage.hashCode() : 0;
return result;
}
}
}
/**
* A hyper parameter that was configured in training the model.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* TrainingHyperParameterProperty trainingHyperParameterProperty = TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.TrainingHyperParameterProperty")
@software.amazon.jsii.Jsii.Proxy(TrainingHyperParameterProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface TrainingHyperParameterProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the hyper parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* The value specified for the hyper parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getValue();
/**
* @return a {@link Builder} of {@link TrainingHyperParameterProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TrainingHyperParameterProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String name;
java.lang.String value;
/**
* Sets the value of {@link TrainingHyperParameterProperty#getName}
* @param name The name of the hyper parameter. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link TrainingHyperParameterProperty#getValue}
* @param value The value specified for the hyper parameter. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.String value) {
this.value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TrainingHyperParameterProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public TrainingHyperParameterProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link TrainingHyperParameterProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements TrainingHyperParameterProperty {
private final java.lang.String name;
private final java.lang.String value;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
this.value = java.util.Objects.requireNonNull(builder.value, "value is required");
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.lang.String getValue() {
return this.value;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("name", om.valueToTree(this.getName()));
data.set("value", om.valueToTree(this.getValue()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.TrainingHyperParameterProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TrainingHyperParameterProperty.Jsii$Proxy that = (TrainingHyperParameterProperty.Jsii$Proxy) o;
if (!name.equals(that.name)) return false;
return this.value.equals(that.value);
}
@Override
public final int hashCode() {
int result = this.name.hashCode();
result = 31 * result + (this.value.hashCode());
return result;
}
}
}
/**
* The overview of a training job.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* TrainingJobDetailsProperty trainingJobDetailsProperty = TrainingJobDetailsProperty.builder()
* .hyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .trainingArn("trainingArn")
* .trainingDatasets(List.of("trainingDatasets"))
* .trainingEnvironment(TrainingEnvironmentProperty.builder()
* .containerImage(List.of("containerImage"))
* .build())
* .trainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .userProvidedHyperParameters(List.of(TrainingHyperParameterProperty.builder()
* .name("name")
* .value("value")
* .build()))
* .userProvidedTrainingMetrics(List.of(TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build()))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.TrainingJobDetailsProperty")
@software.amazon.jsii.Jsii.Proxy(TrainingJobDetailsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface TrainingJobDetailsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The hyper parameters used in the training job.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getHyperParameters() {
return null;
}
/**
* The SageMaker training job Amazon Resource Name (ARN).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTrainingArn() {
return null;
}
/**
* The location of the datasets used to train the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTrainingDatasets() {
return null;
}
/**
* The SageMaker training job image URI.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getTrainingEnvironment() {
return null;
}
/**
* The SageMaker training job results.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getTrainingMetrics() {
return null;
}
/**
* Additional hyper parameters that you've specified when training the model.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getUserProvidedHyperParameters() {
return null;
}
/**
* Custom training job results.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getUserProvidedTrainingMetrics() {
return null;
}
/**
* @return a {@link Builder} of {@link TrainingJobDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TrainingJobDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object hyperParameters;
java.lang.String trainingArn;
java.util.List trainingDatasets;
java.lang.Object trainingEnvironment;
java.lang.Object trainingMetrics;
java.lang.Object userProvidedHyperParameters;
java.lang.Object userProvidedTrainingMetrics;
/**
* Sets the value of {@link TrainingJobDetailsProperty#getHyperParameters}
* @param hyperParameters The hyper parameters used in the training job.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder hyperParameters(software.amazon.awscdk.core.IResolvable hyperParameters) {
this.hyperParameters = hyperParameters;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getHyperParameters}
* @param hyperParameters The hyper parameters used in the training job.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder hyperParameters(java.util.List extends java.lang.Object> hyperParameters) {
this.hyperParameters = hyperParameters;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getTrainingArn}
* @param trainingArn The SageMaker training job Amazon Resource Name (ARN).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingArn(java.lang.String trainingArn) {
this.trainingArn = trainingArn;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getTrainingDatasets}
* @param trainingDatasets The location of the datasets used to train the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingDatasets(java.util.List trainingDatasets) {
this.trainingDatasets = trainingDatasets;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getTrainingEnvironment}
* @param trainingEnvironment The SageMaker training job image URI.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingEnvironment(software.amazon.awscdk.core.IResolvable trainingEnvironment) {
this.trainingEnvironment = trainingEnvironment;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getTrainingEnvironment}
* @param trainingEnvironment The SageMaker training job image URI.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingEnvironment(software.amazon.awscdk.services.sagemaker.CfnModelCard.TrainingEnvironmentProperty trainingEnvironment) {
this.trainingEnvironment = trainingEnvironment;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getTrainingMetrics}
* @param trainingMetrics The SageMaker training job results.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingMetrics(software.amazon.awscdk.core.IResolvable trainingMetrics) {
this.trainingMetrics = trainingMetrics;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getTrainingMetrics}
* @param trainingMetrics The SageMaker training job results.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trainingMetrics(java.util.List extends java.lang.Object> trainingMetrics) {
this.trainingMetrics = trainingMetrics;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getUserProvidedHyperParameters}
* @param userProvidedHyperParameters Additional hyper parameters that you've specified when training the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProvidedHyperParameters(software.amazon.awscdk.core.IResolvable userProvidedHyperParameters) {
this.userProvidedHyperParameters = userProvidedHyperParameters;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getUserProvidedHyperParameters}
* @param userProvidedHyperParameters Additional hyper parameters that you've specified when training the model.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProvidedHyperParameters(java.util.List extends java.lang.Object> userProvidedHyperParameters) {
this.userProvidedHyperParameters = userProvidedHyperParameters;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getUserProvidedTrainingMetrics}
* @param userProvidedTrainingMetrics Custom training job results.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProvidedTrainingMetrics(software.amazon.awscdk.core.IResolvable userProvidedTrainingMetrics) {
this.userProvidedTrainingMetrics = userProvidedTrainingMetrics;
return this;
}
/**
* Sets the value of {@link TrainingJobDetailsProperty#getUserProvidedTrainingMetrics}
* @param userProvidedTrainingMetrics Custom training job results.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProvidedTrainingMetrics(java.util.List extends java.lang.Object> userProvidedTrainingMetrics) {
this.userProvidedTrainingMetrics = userProvidedTrainingMetrics;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TrainingJobDetailsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public TrainingJobDetailsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link TrainingJobDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements TrainingJobDetailsProperty {
private final java.lang.Object hyperParameters;
private final java.lang.String trainingArn;
private final java.util.List trainingDatasets;
private final java.lang.Object trainingEnvironment;
private final java.lang.Object trainingMetrics;
private final java.lang.Object userProvidedHyperParameters;
private final java.lang.Object userProvidedTrainingMetrics;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.hyperParameters = software.amazon.jsii.Kernel.get(this, "hyperParameters", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.trainingArn = software.amazon.jsii.Kernel.get(this, "trainingArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.trainingDatasets = software.amazon.jsii.Kernel.get(this, "trainingDatasets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.trainingEnvironment = software.amazon.jsii.Kernel.get(this, "trainingEnvironment", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.trainingMetrics = software.amazon.jsii.Kernel.get(this, "trainingMetrics", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.userProvidedHyperParameters = software.amazon.jsii.Kernel.get(this, "userProvidedHyperParameters", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.userProvidedTrainingMetrics = software.amazon.jsii.Kernel.get(this, "userProvidedTrainingMetrics", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.hyperParameters = builder.hyperParameters;
this.trainingArn = builder.trainingArn;
this.trainingDatasets = builder.trainingDatasets;
this.trainingEnvironment = builder.trainingEnvironment;
this.trainingMetrics = builder.trainingMetrics;
this.userProvidedHyperParameters = builder.userProvidedHyperParameters;
this.userProvidedTrainingMetrics = builder.userProvidedTrainingMetrics;
}
@Override
public final java.lang.Object getHyperParameters() {
return this.hyperParameters;
}
@Override
public final java.lang.String getTrainingArn() {
return this.trainingArn;
}
@Override
public final java.util.List getTrainingDatasets() {
return this.trainingDatasets;
}
@Override
public final java.lang.Object getTrainingEnvironment() {
return this.trainingEnvironment;
}
@Override
public final java.lang.Object getTrainingMetrics() {
return this.trainingMetrics;
}
@Override
public final java.lang.Object getUserProvidedHyperParameters() {
return this.userProvidedHyperParameters;
}
@Override
public final java.lang.Object getUserProvidedTrainingMetrics() {
return this.userProvidedTrainingMetrics;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getHyperParameters() != null) {
data.set("hyperParameters", om.valueToTree(this.getHyperParameters()));
}
if (this.getTrainingArn() != null) {
data.set("trainingArn", om.valueToTree(this.getTrainingArn()));
}
if (this.getTrainingDatasets() != null) {
data.set("trainingDatasets", om.valueToTree(this.getTrainingDatasets()));
}
if (this.getTrainingEnvironment() != null) {
data.set("trainingEnvironment", om.valueToTree(this.getTrainingEnvironment()));
}
if (this.getTrainingMetrics() != null) {
data.set("trainingMetrics", om.valueToTree(this.getTrainingMetrics()));
}
if (this.getUserProvidedHyperParameters() != null) {
data.set("userProvidedHyperParameters", om.valueToTree(this.getUserProvidedHyperParameters()));
}
if (this.getUserProvidedTrainingMetrics() != null) {
data.set("userProvidedTrainingMetrics", om.valueToTree(this.getUserProvidedTrainingMetrics()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.TrainingJobDetailsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TrainingJobDetailsProperty.Jsii$Proxy that = (TrainingJobDetailsProperty.Jsii$Proxy) o;
if (this.hyperParameters != null ? !this.hyperParameters.equals(that.hyperParameters) : that.hyperParameters != null) return false;
if (this.trainingArn != null ? !this.trainingArn.equals(that.trainingArn) : that.trainingArn != null) return false;
if (this.trainingDatasets != null ? !this.trainingDatasets.equals(that.trainingDatasets) : that.trainingDatasets != null) return false;
if (this.trainingEnvironment != null ? !this.trainingEnvironment.equals(that.trainingEnvironment) : that.trainingEnvironment != null) return false;
if (this.trainingMetrics != null ? !this.trainingMetrics.equals(that.trainingMetrics) : that.trainingMetrics != null) return false;
if (this.userProvidedHyperParameters != null ? !this.userProvidedHyperParameters.equals(that.userProvidedHyperParameters) : that.userProvidedHyperParameters != null) return false;
return this.userProvidedTrainingMetrics != null ? this.userProvidedTrainingMetrics.equals(that.userProvidedTrainingMetrics) : that.userProvidedTrainingMetrics == null;
}
@Override
public final int hashCode() {
int result = this.hyperParameters != null ? this.hyperParameters.hashCode() : 0;
result = 31 * result + (this.trainingArn != null ? this.trainingArn.hashCode() : 0);
result = 31 * result + (this.trainingDatasets != null ? this.trainingDatasets.hashCode() : 0);
result = 31 * result + (this.trainingEnvironment != null ? this.trainingEnvironment.hashCode() : 0);
result = 31 * result + (this.trainingMetrics != null ? this.trainingMetrics.hashCode() : 0);
result = 31 * result + (this.userProvidedHyperParameters != null ? this.userProvidedHyperParameters.hashCode() : 0);
result = 31 * result + (this.userProvidedTrainingMetrics != null ? this.userProvidedTrainingMetrics.hashCode() : 0);
return result;
}
}
}
/**
* A result from a SageMaker training job.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* TrainingMetricProperty trainingMetricProperty = TrainingMetricProperty.builder()
* .name("name")
* .value(123)
* // the properties below are optional
* .notes("notes")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.TrainingMetricProperty")
@software.amazon.jsii.Jsii.Proxy(TrainingMetricProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface TrainingMetricProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the result from the SageMaker training job.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* The value of a result from the SageMaker training job.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getValue();
/**
* Any additional notes describing the result of the training job.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getNotes() {
return null;
}
/**
* @return a {@link Builder} of {@link TrainingMetricProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TrainingMetricProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String name;
java.lang.Number value;
java.lang.String notes;
/**
* Sets the value of {@link TrainingMetricProperty#getName}
* @param name The name of the result from the SageMaker training job. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link TrainingMetricProperty#getValue}
* @param value The value of a result from the SageMaker training job. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.Number value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link TrainingMetricProperty#getNotes}
* @param notes Any additional notes describing the result of the training job.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder notes(java.lang.String notes) {
this.notes = notes;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TrainingMetricProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public TrainingMetricProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link TrainingMetricProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements TrainingMetricProperty {
private final java.lang.String name;
private final java.lang.Number value;
private final java.lang.String notes;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.notes = software.amazon.jsii.Kernel.get(this, "notes", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
this.value = java.util.Objects.requireNonNull(builder.value, "value is required");
this.notes = builder.notes;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.lang.Number getValue() {
return this.value;
}
@Override
public final java.lang.String getNotes() {
return this.notes;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("name", om.valueToTree(this.getName()));
data.set("value", om.valueToTree(this.getValue()));
if (this.getNotes() != null) {
data.set("notes", om.valueToTree(this.getNotes()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.TrainingMetricProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TrainingMetricProperty.Jsii$Proxy that = (TrainingMetricProperty.Jsii$Proxy) o;
if (!name.equals(that.name)) return false;
if (!value.equals(that.value)) return false;
return this.notes != null ? this.notes.equals(that.notes) : that.notes == null;
}
@Override
public final int hashCode() {
int result = this.name.hashCode();
result = 31 * result + (this.value.hashCode());
result = 31 * result + (this.notes != null ? this.notes.hashCode() : 0);
return result;
}
}
}
/**
* Information about the user who created or modified an experiment, trial, trial component, lineage group, project, or model card.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* UserContextProperty userContextProperty = UserContextProperty.builder()
* .domainId("domainId")
* .userProfileArn("userProfileArn")
* .userProfileName("userProfileName")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnModelCard.UserContextProperty")
@software.amazon.jsii.Jsii.Proxy(UserContextProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface UserContextProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The domain associated with the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDomainId() {
return null;
}
/**
* The Amazon Resource Name (ARN) of the user's profile.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getUserProfileArn() {
return null;
}
/**
* The name of the user's profile.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getUserProfileName() {
return null;
}
/**
* @return a {@link Builder} of {@link UserContextProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link UserContextProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String domainId;
java.lang.String userProfileArn;
java.lang.String userProfileName;
/**
* Sets the value of {@link UserContextProperty#getDomainId}
* @param domainId The domain associated with the user.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainId(java.lang.String domainId) {
this.domainId = domainId;
return this;
}
/**
* Sets the value of {@link UserContextProperty#getUserProfileArn}
* @param userProfileArn The Amazon Resource Name (ARN) of the user's profile.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProfileArn(java.lang.String userProfileArn) {
this.userProfileArn = userProfileArn;
return this;
}
/**
* Sets the value of {@link UserContextProperty#getUserProfileName}
* @param userProfileName The name of the user's profile.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userProfileName(java.lang.String userProfileName) {
this.userProfileName = userProfileName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link UserContextProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public UserContextProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link UserContextProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements UserContextProperty {
private final java.lang.String domainId;
private final java.lang.String userProfileArn;
private final java.lang.String userProfileName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.domainId = software.amazon.jsii.Kernel.get(this, "domainId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.userProfileArn = software.amazon.jsii.Kernel.get(this, "userProfileArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.userProfileName = software.amazon.jsii.Kernel.get(this, "userProfileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.domainId = builder.domainId;
this.userProfileArn = builder.userProfileArn;
this.userProfileName = builder.userProfileName;
}
@Override
public final java.lang.String getDomainId() {
return this.domainId;
}
@Override
public final java.lang.String getUserProfileArn() {
return this.userProfileArn;
}
@Override
public final java.lang.String getUserProfileName() {
return this.userProfileName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDomainId() != null) {
data.set("domainId", om.valueToTree(this.getDomainId()));
}
if (this.getUserProfileArn() != null) {
data.set("userProfileArn", om.valueToTree(this.getUserProfileArn()));
}
if (this.getUserProfileName() != null) {
data.set("userProfileName", om.valueToTree(this.getUserProfileName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnModelCard.UserContextProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
UserContextProperty.Jsii$Proxy that = (UserContextProperty.Jsii$Proxy) o;
if (this.domainId != null ? !this.domainId.equals(that.domainId) : that.domainId != null) return false;
if (this.userProfileArn != null ? !this.userProfileArn.equals(that.userProfileArn) : that.userProfileArn != null) return false;
return this.userProfileName != null ? this.userProfileName.equals(that.userProfileName) : that.userProfileName == null;
}
@Override
public final int hashCode() {
int result = this.domainId != null ? this.domainId.hashCode() : 0;
result = 31 * result + (this.userProfileArn != null ? this.userProfileArn.hashCode() : 0);
result = 31 * result + (this.userProfileName != null ? this.userProfileName.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.sagemaker.CfnModelCard}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.sagemaker.CfnModelCardProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.sagemaker.CfnModelCardProps.Builder();
}
/**
* The content of the model card.
*
* Content uses the model card JSON schema .
*
* @return {@code this}
* @param content The content of the model card. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder content(final software.amazon.awscdk.core.IResolvable content) {
this.props.content(content);
return this;
}
/**
* The content of the model card.
*
* Content uses the model card JSON schema .
*
* @return {@code this}
* @param content The content of the model card. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder content(final software.amazon.awscdk.services.sagemaker.CfnModelCard.ContentProperty content) {
this.props.content(content);
return this;
}
/**
* The unique name of the model card.
*
* @return {@code this}
* @param modelCardName The unique name of the model card. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelCardName(final java.lang.String modelCardName) {
this.props.modelCardName(modelCardName);
return this;
}
/**
* The approval status of the model card within your organization.
*
* Different organizations might have different criteria for model card review and approval.
*
*
* Draft
: The model card is a work in progress.
* PendingReview
: The model card is pending review.
* Approved
: The model card is approved.
* Archived
: The model card is archived. No more updates should be made to the model card, but it can still be exported.
*
*
* @return {@code this}
* @param modelCardStatus The approval status of the model card within your organization. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder modelCardStatus(final java.lang.String modelCardStatus) {
this.props.modelCardStatus(modelCardStatus);
return this;
}
/**
* Information about the user who created or modified one or more of the following:.
*
*
* - Experiment
* - Trial
* - Trial component
* - Lineage group
* - Project
* - Model Card
*
*
* @return {@code this}
* @param createdBy Information about the user who created or modified one or more of the following:. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder createdBy(final software.amazon.awscdk.core.IResolvable createdBy) {
this.props.createdBy(createdBy);
return this;
}
/**
* Information about the user who created or modified one or more of the following:.
*
*
* - Experiment
* - Trial
* - Trial component
* - Lineage group
* - Project
* - Model Card
*
*
* @return {@code this}
* @param createdBy Information about the user who created or modified one or more of the following:. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder createdBy(final software.amazon.awscdk.services.sagemaker.CfnModelCard.UserContextProperty createdBy) {
this.props.createdBy(createdBy);
return this;
}
/**
* `AWS::SageMaker::ModelCard.LastModifiedBy`.
*
* @return {@code this}
* @param lastModifiedBy `AWS::SageMaker::ModelCard.LastModifiedBy`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder lastModifiedBy(final software.amazon.awscdk.core.IResolvable lastModifiedBy) {
this.props.lastModifiedBy(lastModifiedBy);
return this;
}
/**
* `AWS::SageMaker::ModelCard.LastModifiedBy`.
*
* @return {@code this}
* @param lastModifiedBy `AWS::SageMaker::ModelCard.LastModifiedBy`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder lastModifiedBy(final software.amazon.awscdk.services.sagemaker.CfnModelCard.UserContextProperty lastModifiedBy) {
this.props.lastModifiedBy(lastModifiedBy);
return this;
}
/**
* The security configuration used to protect model card data.
*
* @return {@code this}
* @param securityConfig The security configuration used to protect model card data. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityConfig(final software.amazon.awscdk.core.IResolvable securityConfig) {
this.props.securityConfig(securityConfig);
return this;
}
/**
* The security configuration used to protect model card data.
*
* @return {@code this}
* @param securityConfig The security configuration used to protect model card data. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityConfig(final software.amazon.awscdk.services.sagemaker.CfnModelCard.SecurityConfigProperty securityConfig) {
this.props.securityConfig(securityConfig);
return this;
}
/**
* Key-value pairs used to manage metadata for the model card.
*
* @return {@code this}
* @param tags Key-value pairs used to manage metadata for the model card. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.sagemaker.CfnModelCard}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.sagemaker.CfnModelCard build() {
return new software.amazon.awscdk.services.sagemaker.CfnModelCard(
this.scope,
this.id,
this.props.build()
);
}
}
}