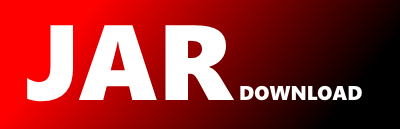
software.amazon.awscdk.services.sagemaker.CfnPipeline Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
package software.amazon.awscdk.services.sagemaker;
/**
* A CloudFormation `AWS::SageMaker::Pipeline`.
*
* The AWS::SageMaker::Pipeline
resource creates shell scripts that run when you create and/or start a SageMaker Pipeline. For information about SageMaker Pipelines, see SageMaker Pipelines in the Amazon SageMaker Developer Guide .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* Object parallelismConfiguration;
* Object pipelineDefinition;
* CfnPipeline cfnPipeline = CfnPipeline.Builder.create(this, "MyCfnPipeline")
* .pipelineDefinition(pipelineDefinition)
* .pipelineName("pipelineName")
* .roleArn("roleArn")
* // the properties below are optional
* .parallelismConfiguration(parallelismConfiguration)
* .pipelineDescription("pipelineDescription")
* .pipelineDisplayName("pipelineDisplayName")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:22.807Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnPipeline")
public class CfnPipeline extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnPipeline(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnPipeline(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.sagemaker.CfnPipeline.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::SageMaker::Pipeline`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnPipeline(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.sagemaker.CfnPipelineProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The tags of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* `AWS::SageMaker::Pipeline.ParallelismConfiguration`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getParallelismConfiguration() {
return software.amazon.jsii.Kernel.get(this, "parallelismConfiguration", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::SageMaker::Pipeline.ParallelismConfiguration`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setParallelismConfiguration(final @org.jetbrains.annotations.NotNull java.lang.Object value) {
software.amazon.jsii.Kernel.set(this, "parallelismConfiguration", java.util.Objects.requireNonNull(value, "parallelismConfiguration is required"));
}
/**
* The definition of the pipeline.
*
* This can be either a JSON string or an Amazon S3 location.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getPipelineDefinition() {
return software.amazon.jsii.Kernel.get(this, "pipelineDefinition", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The definition of the pipeline.
*
* This can be either a JSON string or an Amazon S3 location.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPipelineDefinition(final @org.jetbrains.annotations.NotNull java.lang.Object value) {
software.amazon.jsii.Kernel.set(this, "pipelineDefinition", java.util.Objects.requireNonNull(value, "pipelineDefinition is required"));
}
/**
* The name of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getPipelineName() {
return software.amazon.jsii.Kernel.get(this, "pipelineName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPipelineName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "pipelineName", java.util.Objects.requireNonNull(value, "pipelineName is required"));
}
/**
* The Amazon Resource Name (ARN) of the IAM role used to execute the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getRoleArn() {
return software.amazon.jsii.Kernel.get(this, "roleArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Name (ARN) of the IAM role used to execute the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setRoleArn(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "roleArn", java.util.Objects.requireNonNull(value, "roleArn is required"));
}
/**
* The description of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getPipelineDescription() {
return software.amazon.jsii.Kernel.get(this, "pipelineDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPipelineDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "pipelineDescription", value);
}
/**
* The display name of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getPipelineDisplayName() {
return software.amazon.jsii.Kernel.get(this, "pipelineDisplayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The display name of the pipeline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPipelineDisplayName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "pipelineDisplayName", value);
}
/**
* Configuration that controls the parallelism of the pipeline.
*
* By default, the parallelism configuration specified applies to all executions of the pipeline unless overridden.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* ParallelismConfigurationProperty parallelismConfigurationProperty = ParallelismConfigurationProperty.builder()
* .maxParallelExecutionSteps(123)
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnPipeline.ParallelismConfigurationProperty")
@software.amazon.jsii.Jsii.Proxy(ParallelismConfigurationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ParallelismConfigurationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The max number of steps that can be executed in parallel.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getMaxParallelExecutionSteps();
/**
* @return a {@link Builder} of {@link ParallelismConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ParallelismConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Number maxParallelExecutionSteps;
/**
* Sets the value of {@link ParallelismConfigurationProperty#getMaxParallelExecutionSteps}
* @param maxParallelExecutionSteps The max number of steps that can be executed in parallel. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxParallelExecutionSteps(java.lang.Number maxParallelExecutionSteps) {
this.maxParallelExecutionSteps = maxParallelExecutionSteps;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ParallelismConfigurationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ParallelismConfigurationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ParallelismConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ParallelismConfigurationProperty {
private final java.lang.Number maxParallelExecutionSteps;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.maxParallelExecutionSteps = software.amazon.jsii.Kernel.get(this, "maxParallelExecutionSteps", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.maxParallelExecutionSteps = java.util.Objects.requireNonNull(builder.maxParallelExecutionSteps, "maxParallelExecutionSteps is required");
}
@Override
public final java.lang.Number getMaxParallelExecutionSteps() {
return this.maxParallelExecutionSteps;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("maxParallelExecutionSteps", om.valueToTree(this.getMaxParallelExecutionSteps()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnPipeline.ParallelismConfigurationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ParallelismConfigurationProperty.Jsii$Proxy that = (ParallelismConfigurationProperty.Jsii$Proxy) o;
return this.maxParallelExecutionSteps.equals(that.maxParallelExecutionSteps);
}
@Override
public final int hashCode() {
int result = this.maxParallelExecutionSteps.hashCode();
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* PipelineDefinitionProperty pipelineDefinitionProperty = PipelineDefinitionProperty.builder()
* .pipelineDefinitionBody("pipelineDefinitionBody")
* .pipelineDefinitionS3Location(S3LocationProperty.builder()
* .bucket("bucket")
* .key("key")
* // the properties below are optional
* .eTag("eTag")
* .version("version")
* .build())
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnPipeline.PipelineDefinitionProperty")
@software.amazon.jsii.Jsii.Proxy(PipelineDefinitionProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface PipelineDefinitionProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnPipeline.PipelineDefinitionProperty.PipelineDefinitionBody`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getPipelineDefinitionBody() {
return null;
}
/**
* `CfnPipeline.PipelineDefinitionProperty.PipelineDefinitionS3Location`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getPipelineDefinitionS3Location() {
return null;
}
/**
* @return a {@link Builder} of {@link PipelineDefinitionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PipelineDefinitionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String pipelineDefinitionBody;
java.lang.Object pipelineDefinitionS3Location;
/**
* Sets the value of {@link PipelineDefinitionProperty#getPipelineDefinitionBody}
* @param pipelineDefinitionBody `CfnPipeline.PipelineDefinitionProperty.PipelineDefinitionBody`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineDefinitionBody(java.lang.String pipelineDefinitionBody) {
this.pipelineDefinitionBody = pipelineDefinitionBody;
return this;
}
/**
* Sets the value of {@link PipelineDefinitionProperty#getPipelineDefinitionS3Location}
* @param pipelineDefinitionS3Location `CfnPipeline.PipelineDefinitionProperty.PipelineDefinitionS3Location`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineDefinitionS3Location(software.amazon.awscdk.core.IResolvable pipelineDefinitionS3Location) {
this.pipelineDefinitionS3Location = pipelineDefinitionS3Location;
return this;
}
/**
* Sets the value of {@link PipelineDefinitionProperty#getPipelineDefinitionS3Location}
* @param pipelineDefinitionS3Location `CfnPipeline.PipelineDefinitionProperty.PipelineDefinitionS3Location`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineDefinitionS3Location(software.amazon.awscdk.services.sagemaker.CfnPipeline.S3LocationProperty pipelineDefinitionS3Location) {
this.pipelineDefinitionS3Location = pipelineDefinitionS3Location;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PipelineDefinitionProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public PipelineDefinitionProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link PipelineDefinitionProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PipelineDefinitionProperty {
private final java.lang.String pipelineDefinitionBody;
private final java.lang.Object pipelineDefinitionS3Location;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.pipelineDefinitionBody = software.amazon.jsii.Kernel.get(this, "pipelineDefinitionBody", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.pipelineDefinitionS3Location = software.amazon.jsii.Kernel.get(this, "pipelineDefinitionS3Location", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.pipelineDefinitionBody = builder.pipelineDefinitionBody;
this.pipelineDefinitionS3Location = builder.pipelineDefinitionS3Location;
}
@Override
public final java.lang.String getPipelineDefinitionBody() {
return this.pipelineDefinitionBody;
}
@Override
public final java.lang.Object getPipelineDefinitionS3Location() {
return this.pipelineDefinitionS3Location;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getPipelineDefinitionBody() != null) {
data.set("pipelineDefinitionBody", om.valueToTree(this.getPipelineDefinitionBody()));
}
if (this.getPipelineDefinitionS3Location() != null) {
data.set("pipelineDefinitionS3Location", om.valueToTree(this.getPipelineDefinitionS3Location()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnPipeline.PipelineDefinitionProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PipelineDefinitionProperty.Jsii$Proxy that = (PipelineDefinitionProperty.Jsii$Proxy) o;
if (this.pipelineDefinitionBody != null ? !this.pipelineDefinitionBody.equals(that.pipelineDefinitionBody) : that.pipelineDefinitionBody != null) return false;
return this.pipelineDefinitionS3Location != null ? this.pipelineDefinitionS3Location.equals(that.pipelineDefinitionS3Location) : that.pipelineDefinitionS3Location == null;
}
@Override
public final int hashCode() {
int result = this.pipelineDefinitionBody != null ? this.pipelineDefinitionBody.hashCode() : 0;
result = 31 * result + (this.pipelineDefinitionS3Location != null ? this.pipelineDefinitionS3Location.hashCode() : 0);
return result;
}
}
}
/**
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sagemaker.*;
* S3LocationProperty s3LocationProperty = S3LocationProperty.builder()
* .bucket("bucket")
* .key("key")
* // the properties below are optional
* .eTag("eTag")
* .version("version")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sagemaker.$Module.class, fqn = "@aws-cdk/aws-sagemaker.CfnPipeline.S3LocationProperty")
@software.amazon.jsii.Jsii.Proxy(S3LocationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface S3LocationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnPipeline.S3LocationProperty.Bucket`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getBucket();
/**
* `CfnPipeline.S3LocationProperty.Key`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getKey();
/**
* `CfnPipeline.S3LocationProperty.ETag`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getETag() {
return null;
}
/**
* `CfnPipeline.S3LocationProperty.Version`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getVersion() {
return null;
}
/**
* @return a {@link Builder} of {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String bucket;
java.lang.String key;
java.lang.String eTag;
java.lang.String version;
/**
* Sets the value of {@link S3LocationProperty#getBucket}
* @param bucket `CfnPipeline.S3LocationProperty.Bucket`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bucket(java.lang.String bucket) {
this.bucket = bucket;
return this;
}
/**
* Sets the value of {@link S3LocationProperty#getKey}
* @param key `CfnPipeline.S3LocationProperty.Key`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder key(java.lang.String key) {
this.key = key;
return this;
}
/**
* Sets the value of {@link S3LocationProperty#getETag}
* @param eTag `CfnPipeline.S3LocationProperty.ETag`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eTag(java.lang.String eTag) {
this.eTag = eTag;
return this;
}
/**
* Sets the value of {@link S3LocationProperty#getVersion}
* @param version `CfnPipeline.S3LocationProperty.Version`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder version(java.lang.String version) {
this.version = version;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link S3LocationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public S3LocationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements S3LocationProperty {
private final java.lang.String bucket;
private final java.lang.String key;
private final java.lang.String eTag;
private final java.lang.String version;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.bucket = software.amazon.jsii.Kernel.get(this, "bucket", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.key = software.amazon.jsii.Kernel.get(this, "key", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.eTag = software.amazon.jsii.Kernel.get(this, "eTag", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.version = software.amazon.jsii.Kernel.get(this, "version", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.bucket = java.util.Objects.requireNonNull(builder.bucket, "bucket is required");
this.key = java.util.Objects.requireNonNull(builder.key, "key is required");
this.eTag = builder.eTag;
this.version = builder.version;
}
@Override
public final java.lang.String getBucket() {
return this.bucket;
}
@Override
public final java.lang.String getKey() {
return this.key;
}
@Override
public final java.lang.String getETag() {
return this.eTag;
}
@Override
public final java.lang.String getVersion() {
return this.version;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("bucket", om.valueToTree(this.getBucket()));
data.set("key", om.valueToTree(this.getKey()));
if (this.getETag() != null) {
data.set("eTag", om.valueToTree(this.getETag()));
}
if (this.getVersion() != null) {
data.set("version", om.valueToTree(this.getVersion()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sagemaker.CfnPipeline.S3LocationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
S3LocationProperty.Jsii$Proxy that = (S3LocationProperty.Jsii$Proxy) o;
if (!bucket.equals(that.bucket)) return false;
if (!key.equals(that.key)) return false;
if (this.eTag != null ? !this.eTag.equals(that.eTag) : that.eTag != null) return false;
return this.version != null ? this.version.equals(that.version) : that.version == null;
}
@Override
public final int hashCode() {
int result = this.bucket.hashCode();
result = 31 * result + (this.key.hashCode());
result = 31 * result + (this.eTag != null ? this.eTag.hashCode() : 0);
result = 31 * result + (this.version != null ? this.version.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.sagemaker.CfnPipeline}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.sagemaker.CfnPipelineProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.sagemaker.CfnPipelineProps.Builder();
}
/**
* The definition of the pipeline.
*
* This can be either a JSON string or an Amazon S3 location.
*
* @return {@code this}
* @param pipelineDefinition The definition of the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineDefinition(final java.lang.Object pipelineDefinition) {
this.props.pipelineDefinition(pipelineDefinition);
return this;
}
/**
* The name of the pipeline.
*
* @return {@code this}
* @param pipelineName The name of the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineName(final java.lang.String pipelineName) {
this.props.pipelineName(pipelineName);
return this;
}
/**
* The Amazon Resource Name (ARN) of the IAM role used to execute the pipeline.
*
* @return {@code this}
* @param roleArn The Amazon Resource Name (ARN) of the IAM role used to execute the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder roleArn(final java.lang.String roleArn) {
this.props.roleArn(roleArn);
return this;
}
/**
* `AWS::SageMaker::Pipeline.ParallelismConfiguration`.
*
* @return {@code this}
* @param parallelismConfiguration `AWS::SageMaker::Pipeline.ParallelismConfiguration`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parallelismConfiguration(final java.lang.Object parallelismConfiguration) {
this.props.parallelismConfiguration(parallelismConfiguration);
return this;
}
/**
* The description of the pipeline.
*
* @return {@code this}
* @param pipelineDescription The description of the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineDescription(final java.lang.String pipelineDescription) {
this.props.pipelineDescription(pipelineDescription);
return this;
}
/**
* The display name of the pipeline.
*
* @return {@code this}
* @param pipelineDisplayName The display name of the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pipelineDisplayName(final java.lang.String pipelineDisplayName) {
this.props.pipelineDisplayName(pipelineDisplayName);
return this;
}
/**
* The tags of the pipeline.
*
* @return {@code this}
* @param tags The tags of the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.sagemaker.CfnPipeline}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.sagemaker.CfnPipeline build() {
return new software.amazon.awscdk.services.sagemaker.CfnPipeline(
this.scope,
this.id,
this.props.build()
);
}
}
}