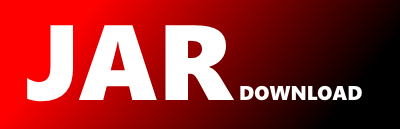
software.amazon.awscdk.services.sam.CfnFunctionProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sam Show documentation
Show all versions of sam Show documentation
The CDK Construct Library for the AWS Serverless Application Model (SAM) resources
package software.amazon.awscdk.services.sam;
/**
* Properties for defining a `CfnFunction`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.sam.*;
* Object assumeRolePolicyDocument;
* CfnFunctionProps cfnFunctionProps = CfnFunctionProps.builder()
* .architectures(List.of("architectures"))
* .assumeRolePolicyDocument(assumeRolePolicyDocument)
* .autoPublishAlias("autoPublishAlias")
* .autoPublishCodeSha256("autoPublishCodeSha256")
* .codeSigningConfigArn("codeSigningConfigArn")
* .codeUri("codeUri")
* .deadLetterQueue(DeadLetterQueueProperty.builder()
* .targetArn("targetArn")
* .type("type")
* .build())
* .deploymentPreference(DeploymentPreferenceProperty.builder()
* .enabled(false)
* .type("type")
* // the properties below are optional
* .alarms(List.of("alarms"))
* .hooks(HooksProperty.builder()
* .postTraffic("postTraffic")
* .preTraffic("preTraffic")
* .build())
* .build())
* .description("description")
* .environment(FunctionEnvironmentProperty.builder()
* .variables(Map.of(
* "variablesKey", "variables"))
* .build())
* .eventInvokeConfig(EventInvokeConfigProperty.builder()
* .destinationConfig(EventInvokeDestinationConfigProperty.builder()
* .onFailure(DestinationProperty.builder()
* .destination("destination")
* // the properties below are optional
* .type("type")
* .build())
* .onSuccess(DestinationProperty.builder()
* .destination("destination")
* // the properties below are optional
* .type("type")
* .build())
* .build())
* .maximumEventAgeInSeconds(123)
* .maximumRetryAttempts(123)
* .build())
* .events(Map.of(
* "eventsKey", EventSourceProperty.builder()
* .properties(S3EventProperty.builder()
* .variables(Map.of(
* "variablesKey", "variables"))
* .build())
* .type("type")
* .build()))
* .fileSystemConfigs(List.of(FileSystemConfigProperty.builder()
* .arn("arn")
* .localMountPath("localMountPath")
* .build()))
* .functionName("functionName")
* .handler("handler")
* .imageConfig(ImageConfigProperty.builder()
* .command(List.of("command"))
* .entryPoint(List.of("entryPoint"))
* .workingDirectory("workingDirectory")
* .build())
* .imageUri("imageUri")
* .inlineCode("inlineCode")
* .kmsKeyArn("kmsKeyArn")
* .layers(List.of("layers"))
* .memorySize(123)
* .packageType("packageType")
* .permissionsBoundary("permissionsBoundary")
* .policies("policies")
* .provisionedConcurrencyConfig(ProvisionedConcurrencyConfigProperty.builder()
* .provisionedConcurrentExecutions("provisionedConcurrentExecutions")
* .build())
* .reservedConcurrentExecutions(123)
* .role("role")
* .runtime("runtime")
* .tags(Map.of(
* "tagsKey", "tags"))
* .timeout(123)
* .tracing("tracing")
* .versionDescription("versionDescription")
* .vpcConfig(VpcConfigProperty.builder()
* .securityGroupIds(List.of("securityGroupIds"))
* .subnetIds(List.of("subnetIds"))
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.60.0 (build ebcefe6)", date = "2022-07-09T01:30:39.347Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.sam.$Module.class, fqn = "@aws-cdk/aws-sam.CfnFunctionProps")
@software.amazon.jsii.Jsii.Proxy(CfnFunctionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnFunctionProps extends software.amazon.jsii.JsiiSerializable {
/**
* `AWS::Serverless::Function.Architectures`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getArchitectures() {
return null;
}
/**
* `AWS::Serverless::Function.AssumeRolePolicyDocument`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getAssumeRolePolicyDocument() {
return null;
}
/**
* `AWS::Serverless::Function.AutoPublishAlias`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAutoPublishAlias() {
return null;
}
/**
* `AWS::Serverless::Function.AutoPublishCodeSha256`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAutoPublishCodeSha256() {
return null;
}
/**
* `AWS::Serverless::Function.CodeSigningConfigArn`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCodeSigningConfigArn() {
return null;
}
/**
* `AWS::Serverless::Function.CodeUri`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getCodeUri() {
return null;
}
/**
* `AWS::Serverless::Function.DeadLetterQueue`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDeadLetterQueue() {
return null;
}
/**
* `AWS::Serverless::Function.DeploymentPreference`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDeploymentPreference() {
return null;
}
/**
* `AWS::Serverless::Function.Description`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* `AWS::Serverless::Function.Environment`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEnvironment() {
return null;
}
/**
* `AWS::Serverless::Function.EventInvokeConfig`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEventInvokeConfig() {
return null;
}
/**
* `AWS::Serverless::Function.Events`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEvents() {
return null;
}
/**
* `AWS::Serverless::Function.FileSystemConfigs`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getFileSystemConfigs() {
return null;
}
/**
* `AWS::Serverless::Function.FunctionName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getFunctionName() {
return null;
}
/**
* `AWS::Serverless::Function.Handler`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getHandler() {
return null;
}
/**
* `AWS::Serverless::Function.ImageConfig`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getImageConfig() {
return null;
}
/**
* `AWS::Serverless::Function.ImageUri`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getImageUri() {
return null;
}
/**
* `AWS::Serverless::Function.InlineCode`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getInlineCode() {
return null;
}
/**
* `AWS::Serverless::Function.KmsKeyArn`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getKmsKeyArn() {
return null;
}
/**
* `AWS::Serverless::Function.Layers`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getLayers() {
return null;
}
/**
* `AWS::Serverless::Function.MemorySize`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getMemorySize() {
return null;
}
/**
* `AWS::Serverless::Function.PackageType`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getPackageType() {
return null;
}
/**
* `AWS::Serverless::Function.PermissionsBoundary`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getPermissionsBoundary() {
return null;
}
/**
* `AWS::Serverless::Function.Policies`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getPolicies() {
return null;
}
/**
* `AWS::Serverless::Function.ProvisionedConcurrencyConfig`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getProvisionedConcurrencyConfig() {
return null;
}
/**
* `AWS::Serverless::Function.ReservedConcurrentExecutions`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getReservedConcurrentExecutions() {
return null;
}
/**
* `AWS::Serverless::Function.Role`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRole() {
return null;
}
/**
* `AWS::Serverless::Function.Runtime`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRuntime() {
return null;
}
/**
* `AWS::Serverless::Function.Tags`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getTags() {
return null;
}
/**
* `AWS::Serverless::Function.Timeout`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getTimeout() {
return null;
}
/**
* `AWS::Serverless::Function.Tracing`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTracing() {
return null;
}
/**
* `AWS::Serverless::Function.VersionDescription`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getVersionDescription() {
return null;
}
/**
* `AWS::Serverless::Function.VpcConfig`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getVpcConfig() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnFunctionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnFunctionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List architectures;
java.lang.Object assumeRolePolicyDocument;
java.lang.String autoPublishAlias;
java.lang.String autoPublishCodeSha256;
java.lang.String codeSigningConfigArn;
java.lang.Object codeUri;
java.lang.Object deadLetterQueue;
java.lang.Object deploymentPreference;
java.lang.String description;
java.lang.Object environment;
java.lang.Object eventInvokeConfig;
java.lang.Object events;
java.lang.Object fileSystemConfigs;
java.lang.String functionName;
java.lang.String handler;
java.lang.Object imageConfig;
java.lang.String imageUri;
java.lang.String inlineCode;
java.lang.String kmsKeyArn;
java.util.List layers;
java.lang.Number memorySize;
java.lang.String packageType;
java.lang.String permissionsBoundary;
java.lang.Object policies;
java.lang.Object provisionedConcurrencyConfig;
java.lang.Number reservedConcurrentExecutions;
java.lang.String role;
java.lang.String runtime;
java.util.Map tags;
java.lang.Number timeout;
java.lang.String tracing;
java.lang.String versionDescription;
java.lang.Object vpcConfig;
/**
* Sets the value of {@link CfnFunctionProps#getArchitectures}
* @param architectures `AWS::Serverless::Function.Architectures`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder architectures(java.util.List architectures) {
this.architectures = architectures;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getAssumeRolePolicyDocument}
* @param assumeRolePolicyDocument `AWS::Serverless::Function.AssumeRolePolicyDocument`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assumeRolePolicyDocument(java.lang.Object assumeRolePolicyDocument) {
this.assumeRolePolicyDocument = assumeRolePolicyDocument;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getAutoPublishAlias}
* @param autoPublishAlias `AWS::Serverless::Function.AutoPublishAlias`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder autoPublishAlias(java.lang.String autoPublishAlias) {
this.autoPublishAlias = autoPublishAlias;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getAutoPublishCodeSha256}
* @param autoPublishCodeSha256 `AWS::Serverless::Function.AutoPublishCodeSha256`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder autoPublishCodeSha256(java.lang.String autoPublishCodeSha256) {
this.autoPublishCodeSha256 = autoPublishCodeSha256;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getCodeSigningConfigArn}
* @param codeSigningConfigArn `AWS::Serverless::Function.CodeSigningConfigArn`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder codeSigningConfigArn(java.lang.String codeSigningConfigArn) {
this.codeSigningConfigArn = codeSigningConfigArn;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getCodeUri}
* @param codeUri `AWS::Serverless::Function.CodeUri`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder codeUri(java.lang.String codeUri) {
this.codeUri = codeUri;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getCodeUri}
* @param codeUri `AWS::Serverless::Function.CodeUri`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder codeUri(software.amazon.awscdk.core.IResolvable codeUri) {
this.codeUri = codeUri;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getCodeUri}
* @param codeUri `AWS::Serverless::Function.CodeUri`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder codeUri(software.amazon.awscdk.services.sam.CfnFunction.S3LocationProperty codeUri) {
this.codeUri = codeUri;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getDeadLetterQueue}
* @param deadLetterQueue `AWS::Serverless::Function.DeadLetterQueue`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deadLetterQueue(software.amazon.awscdk.core.IResolvable deadLetterQueue) {
this.deadLetterQueue = deadLetterQueue;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getDeadLetterQueue}
* @param deadLetterQueue `AWS::Serverless::Function.DeadLetterQueue`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deadLetterQueue(software.amazon.awscdk.services.sam.CfnFunction.DeadLetterQueueProperty deadLetterQueue) {
this.deadLetterQueue = deadLetterQueue;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getDeploymentPreference}
* @param deploymentPreference `AWS::Serverless::Function.DeploymentPreference`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentPreference(software.amazon.awscdk.core.IResolvable deploymentPreference) {
this.deploymentPreference = deploymentPreference;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getDeploymentPreference}
* @param deploymentPreference `AWS::Serverless::Function.DeploymentPreference`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentPreference(software.amazon.awscdk.services.sam.CfnFunction.DeploymentPreferenceProperty deploymentPreference) {
this.deploymentPreference = deploymentPreference;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getDescription}
* @param description `AWS::Serverless::Function.Description`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getEnvironment}
* @param environment `AWS::Serverless::Function.Environment`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environment(software.amazon.awscdk.core.IResolvable environment) {
this.environment = environment;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getEnvironment}
* @param environment `AWS::Serverless::Function.Environment`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environment(software.amazon.awscdk.services.sam.CfnFunction.FunctionEnvironmentProperty environment) {
this.environment = environment;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getEventInvokeConfig}
* @param eventInvokeConfig `AWS::Serverless::Function.EventInvokeConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eventInvokeConfig(software.amazon.awscdk.core.IResolvable eventInvokeConfig) {
this.eventInvokeConfig = eventInvokeConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getEventInvokeConfig}
* @param eventInvokeConfig `AWS::Serverless::Function.EventInvokeConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eventInvokeConfig(software.amazon.awscdk.services.sam.CfnFunction.EventInvokeConfigProperty eventInvokeConfig) {
this.eventInvokeConfig = eventInvokeConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getEvents}
* @param events `AWS::Serverless::Function.Events`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder events(software.amazon.awscdk.core.IResolvable events) {
this.events = events;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getEvents}
* @param events `AWS::Serverless::Function.Events`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder events(java.util.Map events) {
this.events = events;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getFileSystemConfigs}
* @param fileSystemConfigs `AWS::Serverless::Function.FileSystemConfigs`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder fileSystemConfigs(software.amazon.awscdk.core.IResolvable fileSystemConfigs) {
this.fileSystemConfigs = fileSystemConfigs;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getFileSystemConfigs}
* @param fileSystemConfigs `AWS::Serverless::Function.FileSystemConfigs`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder fileSystemConfigs(java.util.List extends java.lang.Object> fileSystemConfigs) {
this.fileSystemConfigs = fileSystemConfigs;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getFunctionName}
* @param functionName `AWS::Serverless::Function.FunctionName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionName(java.lang.String functionName) {
this.functionName = functionName;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getHandler}
* @param handler `AWS::Serverless::Function.Handler`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder handler(java.lang.String handler) {
this.handler = handler;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getImageConfig}
* @param imageConfig `AWS::Serverless::Function.ImageConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageConfig(software.amazon.awscdk.core.IResolvable imageConfig) {
this.imageConfig = imageConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getImageConfig}
* @param imageConfig `AWS::Serverless::Function.ImageConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageConfig(software.amazon.awscdk.services.sam.CfnFunction.ImageConfigProperty imageConfig) {
this.imageConfig = imageConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getImageUri}
* @param imageUri `AWS::Serverless::Function.ImageUri`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageUri(java.lang.String imageUri) {
this.imageUri = imageUri;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getInlineCode}
* @param inlineCode `AWS::Serverless::Function.InlineCode`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inlineCode(java.lang.String inlineCode) {
this.inlineCode = inlineCode;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getKmsKeyArn}
* @param kmsKeyArn `AWS::Serverless::Function.KmsKeyArn`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder kmsKeyArn(java.lang.String kmsKeyArn) {
this.kmsKeyArn = kmsKeyArn;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getLayers}
* @param layers `AWS::Serverless::Function.Layers`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder layers(java.util.List layers) {
this.layers = layers;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getMemorySize}
* @param memorySize `AWS::Serverless::Function.MemorySize`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memorySize(java.lang.Number memorySize) {
this.memorySize = memorySize;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getPackageType}
* @param packageType `AWS::Serverless::Function.PackageType`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder packageType(java.lang.String packageType) {
this.packageType = packageType;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getPermissionsBoundary}
* @param permissionsBoundary `AWS::Serverless::Function.PermissionsBoundary`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder permissionsBoundary(java.lang.String permissionsBoundary) {
this.permissionsBoundary = permissionsBoundary;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getPolicies}
* @param policies `AWS::Serverless::Function.Policies`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder policies(java.lang.String policies) {
this.policies = policies;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getPolicies}
* @param policies `AWS::Serverless::Function.Policies`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder policies(software.amazon.awscdk.core.IResolvable policies) {
this.policies = policies;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getPolicies}
* @param policies `AWS::Serverless::Function.Policies`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder policies(software.amazon.awscdk.services.sam.CfnFunction.IAMPolicyDocumentProperty policies) {
this.policies = policies;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getPolicies}
* @param policies `AWS::Serverless::Function.Policies`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder policies(java.util.List extends java.lang.Object> policies) {
this.policies = policies;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getProvisionedConcurrencyConfig}
* @param provisionedConcurrencyConfig `AWS::Serverless::Function.ProvisionedConcurrencyConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder provisionedConcurrencyConfig(software.amazon.awscdk.core.IResolvable provisionedConcurrencyConfig) {
this.provisionedConcurrencyConfig = provisionedConcurrencyConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getProvisionedConcurrencyConfig}
* @param provisionedConcurrencyConfig `AWS::Serverless::Function.ProvisionedConcurrencyConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder provisionedConcurrencyConfig(software.amazon.awscdk.services.sam.CfnFunction.ProvisionedConcurrencyConfigProperty provisionedConcurrencyConfig) {
this.provisionedConcurrencyConfig = provisionedConcurrencyConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getReservedConcurrentExecutions}
* @param reservedConcurrentExecutions `AWS::Serverless::Function.ReservedConcurrentExecutions`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder reservedConcurrentExecutions(java.lang.Number reservedConcurrentExecutions) {
this.reservedConcurrentExecutions = reservedConcurrentExecutions;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getRole}
* @param role `AWS::Serverless::Function.Role`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(java.lang.String role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getRuntime}
* @param runtime `AWS::Serverless::Function.Runtime`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runtime(java.lang.String runtime) {
this.runtime = runtime;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getTags}
* @param tags `AWS::Serverless::Function.Tags`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(java.util.Map tags) {
this.tags = tags;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getTimeout}
* @param timeout `AWS::Serverless::Function.Timeout`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timeout(java.lang.Number timeout) {
this.timeout = timeout;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getTracing}
* @param tracing `AWS::Serverless::Function.Tracing`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tracing(java.lang.String tracing) {
this.tracing = tracing;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getVersionDescription}
* @param versionDescription `AWS::Serverless::Function.VersionDescription`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder versionDescription(java.lang.String versionDescription) {
this.versionDescription = versionDescription;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getVpcConfig}
* @param vpcConfig `AWS::Serverless::Function.VpcConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcConfig(software.amazon.awscdk.core.IResolvable vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Sets the value of {@link CfnFunctionProps#getVpcConfig}
* @param vpcConfig `AWS::Serverless::Function.VpcConfig`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcConfig(software.amazon.awscdk.services.sam.CfnFunction.VpcConfigProperty vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnFunctionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnFunctionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnFunctionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnFunctionProps {
private final java.util.List architectures;
private final java.lang.Object assumeRolePolicyDocument;
private final java.lang.String autoPublishAlias;
private final java.lang.String autoPublishCodeSha256;
private final java.lang.String codeSigningConfigArn;
private final java.lang.Object codeUri;
private final java.lang.Object deadLetterQueue;
private final java.lang.Object deploymentPreference;
private final java.lang.String description;
private final java.lang.Object environment;
private final java.lang.Object eventInvokeConfig;
private final java.lang.Object events;
private final java.lang.Object fileSystemConfigs;
private final java.lang.String functionName;
private final java.lang.String handler;
private final java.lang.Object imageConfig;
private final java.lang.String imageUri;
private final java.lang.String inlineCode;
private final java.lang.String kmsKeyArn;
private final java.util.List layers;
private final java.lang.Number memorySize;
private final java.lang.String packageType;
private final java.lang.String permissionsBoundary;
private final java.lang.Object policies;
private final java.lang.Object provisionedConcurrencyConfig;
private final java.lang.Number reservedConcurrentExecutions;
private final java.lang.String role;
private final java.lang.String runtime;
private final java.util.Map tags;
private final java.lang.Number timeout;
private final java.lang.String tracing;
private final java.lang.String versionDescription;
private final java.lang.Object vpcConfig;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.architectures = software.amazon.jsii.Kernel.get(this, "architectures", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.assumeRolePolicyDocument = software.amazon.jsii.Kernel.get(this, "assumeRolePolicyDocument", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.autoPublishAlias = software.amazon.jsii.Kernel.get(this, "autoPublishAlias", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.autoPublishCodeSha256 = software.amazon.jsii.Kernel.get(this, "autoPublishCodeSha256", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.codeSigningConfigArn = software.amazon.jsii.Kernel.get(this, "codeSigningConfigArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.codeUri = software.amazon.jsii.Kernel.get(this, "codeUri", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.deadLetterQueue = software.amazon.jsii.Kernel.get(this, "deadLetterQueue", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.deploymentPreference = software.amazon.jsii.Kernel.get(this, "deploymentPreference", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.environment = software.amazon.jsii.Kernel.get(this, "environment", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.eventInvokeConfig = software.amazon.jsii.Kernel.get(this, "eventInvokeConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.events = software.amazon.jsii.Kernel.get(this, "events", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.fileSystemConfigs = software.amazon.jsii.Kernel.get(this, "fileSystemConfigs", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.functionName = software.amazon.jsii.Kernel.get(this, "functionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.handler = software.amazon.jsii.Kernel.get(this, "handler", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.imageConfig = software.amazon.jsii.Kernel.get(this, "imageConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.imageUri = software.amazon.jsii.Kernel.get(this, "imageUri", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.inlineCode = software.amazon.jsii.Kernel.get(this, "inlineCode", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.kmsKeyArn = software.amazon.jsii.Kernel.get(this, "kmsKeyArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.layers = software.amazon.jsii.Kernel.get(this, "layers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.memorySize = software.amazon.jsii.Kernel.get(this, "memorySize", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.packageType = software.amazon.jsii.Kernel.get(this, "packageType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.permissionsBoundary = software.amazon.jsii.Kernel.get(this, "permissionsBoundary", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.policies = software.amazon.jsii.Kernel.get(this, "policies", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.provisionedConcurrencyConfig = software.amazon.jsii.Kernel.get(this, "provisionedConcurrencyConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.reservedConcurrentExecutions = software.amazon.jsii.Kernel.get(this, "reservedConcurrentExecutions", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runtime = software.amazon.jsii.Kernel.get(this, "runtime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.timeout = software.amazon.jsii.Kernel.get(this, "timeout", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.tracing = software.amazon.jsii.Kernel.get(this, "tracing", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.versionDescription = software.amazon.jsii.Kernel.get(this, "versionDescription", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpcConfig = software.amazon.jsii.Kernel.get(this, "vpcConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.architectures = builder.architectures;
this.assumeRolePolicyDocument = builder.assumeRolePolicyDocument;
this.autoPublishAlias = builder.autoPublishAlias;
this.autoPublishCodeSha256 = builder.autoPublishCodeSha256;
this.codeSigningConfigArn = builder.codeSigningConfigArn;
this.codeUri = builder.codeUri;
this.deadLetterQueue = builder.deadLetterQueue;
this.deploymentPreference = builder.deploymentPreference;
this.description = builder.description;
this.environment = builder.environment;
this.eventInvokeConfig = builder.eventInvokeConfig;
this.events = builder.events;
this.fileSystemConfigs = builder.fileSystemConfigs;
this.functionName = builder.functionName;
this.handler = builder.handler;
this.imageConfig = builder.imageConfig;
this.imageUri = builder.imageUri;
this.inlineCode = builder.inlineCode;
this.kmsKeyArn = builder.kmsKeyArn;
this.layers = builder.layers;
this.memorySize = builder.memorySize;
this.packageType = builder.packageType;
this.permissionsBoundary = builder.permissionsBoundary;
this.policies = builder.policies;
this.provisionedConcurrencyConfig = builder.provisionedConcurrencyConfig;
this.reservedConcurrentExecutions = builder.reservedConcurrentExecutions;
this.role = builder.role;
this.runtime = builder.runtime;
this.tags = builder.tags;
this.timeout = builder.timeout;
this.tracing = builder.tracing;
this.versionDescription = builder.versionDescription;
this.vpcConfig = builder.vpcConfig;
}
@Override
public final java.util.List getArchitectures() {
return this.architectures;
}
@Override
public final java.lang.Object getAssumeRolePolicyDocument() {
return this.assumeRolePolicyDocument;
}
@Override
public final java.lang.String getAutoPublishAlias() {
return this.autoPublishAlias;
}
@Override
public final java.lang.String getAutoPublishCodeSha256() {
return this.autoPublishCodeSha256;
}
@Override
public final java.lang.String getCodeSigningConfigArn() {
return this.codeSigningConfigArn;
}
@Override
public final java.lang.Object getCodeUri() {
return this.codeUri;
}
@Override
public final java.lang.Object getDeadLetterQueue() {
return this.deadLetterQueue;
}
@Override
public final java.lang.Object getDeploymentPreference() {
return this.deploymentPreference;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.Object getEnvironment() {
return this.environment;
}
@Override
public final java.lang.Object getEventInvokeConfig() {
return this.eventInvokeConfig;
}
@Override
public final java.lang.Object getEvents() {
return this.events;
}
@Override
public final java.lang.Object getFileSystemConfigs() {
return this.fileSystemConfigs;
}
@Override
public final java.lang.String getFunctionName() {
return this.functionName;
}
@Override
public final java.lang.String getHandler() {
return this.handler;
}
@Override
public final java.lang.Object getImageConfig() {
return this.imageConfig;
}
@Override
public final java.lang.String getImageUri() {
return this.imageUri;
}
@Override
public final java.lang.String getInlineCode() {
return this.inlineCode;
}
@Override
public final java.lang.String getKmsKeyArn() {
return this.kmsKeyArn;
}
@Override
public final java.util.List getLayers() {
return this.layers;
}
@Override
public final java.lang.Number getMemorySize() {
return this.memorySize;
}
@Override
public final java.lang.String getPackageType() {
return this.packageType;
}
@Override
public final java.lang.String getPermissionsBoundary() {
return this.permissionsBoundary;
}
@Override
public final java.lang.Object getPolicies() {
return this.policies;
}
@Override
public final java.lang.Object getProvisionedConcurrencyConfig() {
return this.provisionedConcurrencyConfig;
}
@Override
public final java.lang.Number getReservedConcurrentExecutions() {
return this.reservedConcurrentExecutions;
}
@Override
public final java.lang.String getRole() {
return this.role;
}
@Override
public final java.lang.String getRuntime() {
return this.runtime;
}
@Override
public final java.util.Map getTags() {
return this.tags;
}
@Override
public final java.lang.Number getTimeout() {
return this.timeout;
}
@Override
public final java.lang.String getTracing() {
return this.tracing;
}
@Override
public final java.lang.String getVersionDescription() {
return this.versionDescription;
}
@Override
public final java.lang.Object getVpcConfig() {
return this.vpcConfig;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getArchitectures() != null) {
data.set("architectures", om.valueToTree(this.getArchitectures()));
}
if (this.getAssumeRolePolicyDocument() != null) {
data.set("assumeRolePolicyDocument", om.valueToTree(this.getAssumeRolePolicyDocument()));
}
if (this.getAutoPublishAlias() != null) {
data.set("autoPublishAlias", om.valueToTree(this.getAutoPublishAlias()));
}
if (this.getAutoPublishCodeSha256() != null) {
data.set("autoPublishCodeSha256", om.valueToTree(this.getAutoPublishCodeSha256()));
}
if (this.getCodeSigningConfigArn() != null) {
data.set("codeSigningConfigArn", om.valueToTree(this.getCodeSigningConfigArn()));
}
if (this.getCodeUri() != null) {
data.set("codeUri", om.valueToTree(this.getCodeUri()));
}
if (this.getDeadLetterQueue() != null) {
data.set("deadLetterQueue", om.valueToTree(this.getDeadLetterQueue()));
}
if (this.getDeploymentPreference() != null) {
data.set("deploymentPreference", om.valueToTree(this.getDeploymentPreference()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getEnvironment() != null) {
data.set("environment", om.valueToTree(this.getEnvironment()));
}
if (this.getEventInvokeConfig() != null) {
data.set("eventInvokeConfig", om.valueToTree(this.getEventInvokeConfig()));
}
if (this.getEvents() != null) {
data.set("events", om.valueToTree(this.getEvents()));
}
if (this.getFileSystemConfigs() != null) {
data.set("fileSystemConfigs", om.valueToTree(this.getFileSystemConfigs()));
}
if (this.getFunctionName() != null) {
data.set("functionName", om.valueToTree(this.getFunctionName()));
}
if (this.getHandler() != null) {
data.set("handler", om.valueToTree(this.getHandler()));
}
if (this.getImageConfig() != null) {
data.set("imageConfig", om.valueToTree(this.getImageConfig()));
}
if (this.getImageUri() != null) {
data.set("imageUri", om.valueToTree(this.getImageUri()));
}
if (this.getInlineCode() != null) {
data.set("inlineCode", om.valueToTree(this.getInlineCode()));
}
if (this.getKmsKeyArn() != null) {
data.set("kmsKeyArn", om.valueToTree(this.getKmsKeyArn()));
}
if (this.getLayers() != null) {
data.set("layers", om.valueToTree(this.getLayers()));
}
if (this.getMemorySize() != null) {
data.set("memorySize", om.valueToTree(this.getMemorySize()));
}
if (this.getPackageType() != null) {
data.set("packageType", om.valueToTree(this.getPackageType()));
}
if (this.getPermissionsBoundary() != null) {
data.set("permissionsBoundary", om.valueToTree(this.getPermissionsBoundary()));
}
if (this.getPolicies() != null) {
data.set("policies", om.valueToTree(this.getPolicies()));
}
if (this.getProvisionedConcurrencyConfig() != null) {
data.set("provisionedConcurrencyConfig", om.valueToTree(this.getProvisionedConcurrencyConfig()));
}
if (this.getReservedConcurrentExecutions() != null) {
data.set("reservedConcurrentExecutions", om.valueToTree(this.getReservedConcurrentExecutions()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
if (this.getRuntime() != null) {
data.set("runtime", om.valueToTree(this.getRuntime()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getTimeout() != null) {
data.set("timeout", om.valueToTree(this.getTimeout()));
}
if (this.getTracing() != null) {
data.set("tracing", om.valueToTree(this.getTracing()));
}
if (this.getVersionDescription() != null) {
data.set("versionDescription", om.valueToTree(this.getVersionDescription()));
}
if (this.getVpcConfig() != null) {
data.set("vpcConfig", om.valueToTree(this.getVpcConfig()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-sam.CfnFunctionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnFunctionProps.Jsii$Proxy that = (CfnFunctionProps.Jsii$Proxy) o;
if (this.architectures != null ? !this.architectures.equals(that.architectures) : that.architectures != null) return false;
if (this.assumeRolePolicyDocument != null ? !this.assumeRolePolicyDocument.equals(that.assumeRolePolicyDocument) : that.assumeRolePolicyDocument != null) return false;
if (this.autoPublishAlias != null ? !this.autoPublishAlias.equals(that.autoPublishAlias) : that.autoPublishAlias != null) return false;
if (this.autoPublishCodeSha256 != null ? !this.autoPublishCodeSha256.equals(that.autoPublishCodeSha256) : that.autoPublishCodeSha256 != null) return false;
if (this.codeSigningConfigArn != null ? !this.codeSigningConfigArn.equals(that.codeSigningConfigArn) : that.codeSigningConfigArn != null) return false;
if (this.codeUri != null ? !this.codeUri.equals(that.codeUri) : that.codeUri != null) return false;
if (this.deadLetterQueue != null ? !this.deadLetterQueue.equals(that.deadLetterQueue) : that.deadLetterQueue != null) return false;
if (this.deploymentPreference != null ? !this.deploymentPreference.equals(that.deploymentPreference) : that.deploymentPreference != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.environment != null ? !this.environment.equals(that.environment) : that.environment != null) return false;
if (this.eventInvokeConfig != null ? !this.eventInvokeConfig.equals(that.eventInvokeConfig) : that.eventInvokeConfig != null) return false;
if (this.events != null ? !this.events.equals(that.events) : that.events != null) return false;
if (this.fileSystemConfigs != null ? !this.fileSystemConfigs.equals(that.fileSystemConfigs) : that.fileSystemConfigs != null) return false;
if (this.functionName != null ? !this.functionName.equals(that.functionName) : that.functionName != null) return false;
if (this.handler != null ? !this.handler.equals(that.handler) : that.handler != null) return false;
if (this.imageConfig != null ? !this.imageConfig.equals(that.imageConfig) : that.imageConfig != null) return false;
if (this.imageUri != null ? !this.imageUri.equals(that.imageUri) : that.imageUri != null) return false;
if (this.inlineCode != null ? !this.inlineCode.equals(that.inlineCode) : that.inlineCode != null) return false;
if (this.kmsKeyArn != null ? !this.kmsKeyArn.equals(that.kmsKeyArn) : that.kmsKeyArn != null) return false;
if (this.layers != null ? !this.layers.equals(that.layers) : that.layers != null) return false;
if (this.memorySize != null ? !this.memorySize.equals(that.memorySize) : that.memorySize != null) return false;
if (this.packageType != null ? !this.packageType.equals(that.packageType) : that.packageType != null) return false;
if (this.permissionsBoundary != null ? !this.permissionsBoundary.equals(that.permissionsBoundary) : that.permissionsBoundary != null) return false;
if (this.policies != null ? !this.policies.equals(that.policies) : that.policies != null) return false;
if (this.provisionedConcurrencyConfig != null ? !this.provisionedConcurrencyConfig.equals(that.provisionedConcurrencyConfig) : that.provisionedConcurrencyConfig != null) return false;
if (this.reservedConcurrentExecutions != null ? !this.reservedConcurrentExecutions.equals(that.reservedConcurrentExecutions) : that.reservedConcurrentExecutions != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (this.runtime != null ? !this.runtime.equals(that.runtime) : that.runtime != null) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
if (this.timeout != null ? !this.timeout.equals(that.timeout) : that.timeout != null) return false;
if (this.tracing != null ? !this.tracing.equals(that.tracing) : that.tracing != null) return false;
if (this.versionDescription != null ? !this.versionDescription.equals(that.versionDescription) : that.versionDescription != null) return false;
return this.vpcConfig != null ? this.vpcConfig.equals(that.vpcConfig) : that.vpcConfig == null;
}
@Override
public final int hashCode() {
int result = this.architectures != null ? this.architectures.hashCode() : 0;
result = 31 * result + (this.assumeRolePolicyDocument != null ? this.assumeRolePolicyDocument.hashCode() : 0);
result = 31 * result + (this.autoPublishAlias != null ? this.autoPublishAlias.hashCode() : 0);
result = 31 * result + (this.autoPublishCodeSha256 != null ? this.autoPublishCodeSha256.hashCode() : 0);
result = 31 * result + (this.codeSigningConfigArn != null ? this.codeSigningConfigArn.hashCode() : 0);
result = 31 * result + (this.codeUri != null ? this.codeUri.hashCode() : 0);
result = 31 * result + (this.deadLetterQueue != null ? this.deadLetterQueue.hashCode() : 0);
result = 31 * result + (this.deploymentPreference != null ? this.deploymentPreference.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.environment != null ? this.environment.hashCode() : 0);
result = 31 * result + (this.eventInvokeConfig != null ? this.eventInvokeConfig.hashCode() : 0);
result = 31 * result + (this.events != null ? this.events.hashCode() : 0);
result = 31 * result + (this.fileSystemConfigs != null ? this.fileSystemConfigs.hashCode() : 0);
result = 31 * result + (this.functionName != null ? this.functionName.hashCode() : 0);
result = 31 * result + (this.handler != null ? this.handler.hashCode() : 0);
result = 31 * result + (this.imageConfig != null ? this.imageConfig.hashCode() : 0);
result = 31 * result + (this.imageUri != null ? this.imageUri.hashCode() : 0);
result = 31 * result + (this.inlineCode != null ? this.inlineCode.hashCode() : 0);
result = 31 * result + (this.kmsKeyArn != null ? this.kmsKeyArn.hashCode() : 0);
result = 31 * result + (this.layers != null ? this.layers.hashCode() : 0);
result = 31 * result + (this.memorySize != null ? this.memorySize.hashCode() : 0);
result = 31 * result + (this.packageType != null ? this.packageType.hashCode() : 0);
result = 31 * result + (this.permissionsBoundary != null ? this.permissionsBoundary.hashCode() : 0);
result = 31 * result + (this.policies != null ? this.policies.hashCode() : 0);
result = 31 * result + (this.provisionedConcurrencyConfig != null ? this.provisionedConcurrencyConfig.hashCode() : 0);
result = 31 * result + (this.reservedConcurrentExecutions != null ? this.reservedConcurrentExecutions.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.runtime != null ? this.runtime.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.timeout != null ? this.timeout.hashCode() : 0);
result = 31 * result + (this.tracing != null ? this.tracing.hashCode() : 0);
result = 31 * result + (this.versionDescription != null ? this.versionDescription.hashCode() : 0);
result = 31 * result + (this.vpcConfig != null ? this.vpcConfig.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy