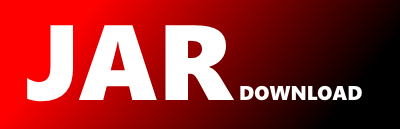
software.amazon.awscdk.services.ses.actions.S3 Maven / Gradle / Ivy
Show all versions of ses-actions Show documentation
package software.amazon.awscdk.services.ses.actions;
/**
* Saves the received message to an Amazon S3 bucket and, optionally, publishes a notification to Amazon SNS.
*
* Example:
*
*
* import software.amazon.awscdk.services.s3.*;
* import software.amazon.awscdk.services.ses.actions.*;
* Bucket bucket = new Bucket(this, "Bucket");
* Topic topic = new Topic(this, "Topic");
* ReceiptRuleSet.Builder.create(this, "RuleSet")
* .rules(List.of(ReceiptRuleOptions.builder()
* .recipients(List.of("hello@aws.com"))
* .actions(List.of(
* AddHeader.Builder.create()
* .name("X-Special-Header")
* .value("aws")
* .build(),
* S3.Builder.create()
* .bucket(bucket)
* .objectKeyPrefix("emails/")
* .topic(topic)
* .build()))
* .build(), ReceiptRuleOptions.builder()
* .recipients(List.of("aws.com"))
* .actions(List.of(
* Sns.Builder.create()
* .topic(topic)
* .build()))
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-02-21T19:24:07.415Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ses.actions.$Module.class, fqn = "@aws-cdk/aws-ses-actions.S3")
public class S3 extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.ses.IReceiptRuleAction {
protected S3(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected S3(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public S3(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ses.actions.S3Props props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Returns the receipt rule action specification.
*
* @param rule This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ses.ReceiptRuleActionConfig bind(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ses.IReceiptRule rule) {
return software.amazon.jsii.Kernel.call(this, "bind", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ses.ReceiptRuleActionConfig.class), new Object[] { java.util.Objects.requireNonNull(rule, "rule is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ses.actions.S3}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.ses.actions.S3Props.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.ses.actions.S3Props.Builder();
}
/**
* The S3 bucket that incoming email will be saved to.
*
* @return {@code this}
* @param bucket The S3 bucket that incoming email will be saved to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bucket(final software.amazon.awscdk.services.s3.IBucket bucket) {
this.props.bucket(bucket);
return this;
}
/**
* The master key that SES should use to encrypt your emails before saving them to the S3 bucket.
*
* Default: no encryption
*
* @return {@code this}
* @param kmsKey The master key that SES should use to encrypt your emails before saving them to the S3 bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder kmsKey(final software.amazon.awscdk.services.kms.IKey kmsKey) {
this.props.kmsKey(kmsKey);
return this;
}
/**
* The key prefix of the S3 bucket.
*
* Default: no prefix
*
* @return {@code this}
* @param objectKeyPrefix The key prefix of the S3 bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder objectKeyPrefix(final java.lang.String objectKeyPrefix) {
this.props.objectKeyPrefix(objectKeyPrefix);
return this;
}
/**
* The SNS topic to notify when the S3 action is taken.
*
* Default: no notification
*
* @return {@code this}
* @param topic The SNS topic to notify when the S3 action is taken. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder topic(final software.amazon.awscdk.services.sns.ITopic topic) {
this.props.topic(topic);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ses.actions.S3}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ses.actions.S3 build() {
return new software.amazon.awscdk.services.ses.actions.S3(
this.props.build()
);
}
}
}