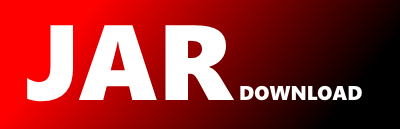
software.amazon.awscdk.services.transfer.CfnCertificateProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transfer Show documentation
Show all versions of transfer Show documentation
The CDK Construct Library for AWS::Transfer
package software.amazon.awscdk.services.transfer;
/**
* Properties for defining a `CfnCertificate`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.transfer.*;
* CfnCertificateProps cfnCertificateProps = CfnCertificateProps.builder()
* .certificate("certificate")
* .usage("usage")
* // the properties below are optional
* .activeDate("activeDate")
* .certificateChain("certificateChain")
* .description("description")
* .inactiveDate("inactiveDate")
* .privateKey("privateKey")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-08T16:00:23.921Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.transfer.$Module.class, fqn = "@aws-cdk/aws-transfer.CfnCertificateProps")
@software.amazon.jsii.Jsii.Proxy(CfnCertificateProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnCertificateProps extends software.amazon.jsii.JsiiSerializable {
/**
* The file name for the certificate.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getCertificate();
/**
* Specifies whether this certificate is used for signing or encryption.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getUsage();
/**
* An optional date that specifies when the certificate becomes active.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getActiveDate() {
return null;
}
/**
* The list of certificates that make up the chain for the certificate.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCertificateChain() {
return null;
}
/**
* The name or description that's used to identity the certificate.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* An optional date that specifies when the certificate becomes inactive.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getInactiveDate() {
return null;
}
/**
* The file that contains the private key for the certificate that's being imported.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getPrivateKey() {
return null;
}
/**
* Key-value pairs that can be used to group and search for certificates.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnCertificateProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnCertificateProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String certificate;
java.lang.String usage;
java.lang.String activeDate;
java.lang.String certificateChain;
java.lang.String description;
java.lang.String inactiveDate;
java.lang.String privateKey;
java.util.List tags;
/**
* Sets the value of {@link CfnCertificateProps#getCertificate}
* @param certificate The file name for the certificate. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder certificate(java.lang.String certificate) {
this.certificate = certificate;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getUsage}
* @param usage Specifies whether this certificate is used for signing or encryption. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder usage(java.lang.String usage) {
this.usage = usage;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getActiveDate}
* @param activeDate An optional date that specifies when the certificate becomes active.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder activeDate(java.lang.String activeDate) {
this.activeDate = activeDate;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getCertificateChain}
* @param certificateChain The list of certificates that make up the chain for the certificate.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder certificateChain(java.lang.String certificateChain) {
this.certificateChain = certificateChain;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getDescription}
* @param description The name or description that's used to identity the certificate.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getInactiveDate}
* @param inactiveDate An optional date that specifies when the certificate becomes inactive.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inactiveDate(java.lang.String inactiveDate) {
this.inactiveDate = inactiveDate;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getPrivateKey}
* @param privateKey The file that contains the private key for the certificate that's being imported.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder privateKey(java.lang.String privateKey) {
this.privateKey = privateKey;
return this;
}
/**
* Sets the value of {@link CfnCertificateProps#getTags}
* @param tags Key-value pairs that can be used to group and search for certificates.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnCertificateProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnCertificateProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnCertificateProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnCertificateProps {
private final java.lang.String certificate;
private final java.lang.String usage;
private final java.lang.String activeDate;
private final java.lang.String certificateChain;
private final java.lang.String description;
private final java.lang.String inactiveDate;
private final java.lang.String privateKey;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.certificate = software.amazon.jsii.Kernel.get(this, "certificate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.usage = software.amazon.jsii.Kernel.get(this, "usage", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.activeDate = software.amazon.jsii.Kernel.get(this, "activeDate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.certificateChain = software.amazon.jsii.Kernel.get(this, "certificateChain", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.inactiveDate = software.amazon.jsii.Kernel.get(this, "inactiveDate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.privateKey = software.amazon.jsii.Kernel.get(this, "privateKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.certificate = java.util.Objects.requireNonNull(builder.certificate, "certificate is required");
this.usage = java.util.Objects.requireNonNull(builder.usage, "usage is required");
this.activeDate = builder.activeDate;
this.certificateChain = builder.certificateChain;
this.description = builder.description;
this.inactiveDate = builder.inactiveDate;
this.privateKey = builder.privateKey;
this.tags = (java.util.List)builder.tags;
}
@Override
public final java.lang.String getCertificate() {
return this.certificate;
}
@Override
public final java.lang.String getUsage() {
return this.usage;
}
@Override
public final java.lang.String getActiveDate() {
return this.activeDate;
}
@Override
public final java.lang.String getCertificateChain() {
return this.certificateChain;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.String getInactiveDate() {
return this.inactiveDate;
}
@Override
public final java.lang.String getPrivateKey() {
return this.privateKey;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("certificate", om.valueToTree(this.getCertificate()));
data.set("usage", om.valueToTree(this.getUsage()));
if (this.getActiveDate() != null) {
data.set("activeDate", om.valueToTree(this.getActiveDate()));
}
if (this.getCertificateChain() != null) {
data.set("certificateChain", om.valueToTree(this.getCertificateChain()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getInactiveDate() != null) {
data.set("inactiveDate", om.valueToTree(this.getInactiveDate()));
}
if (this.getPrivateKey() != null) {
data.set("privateKey", om.valueToTree(this.getPrivateKey()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-transfer.CfnCertificateProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnCertificateProps.Jsii$Proxy that = (CfnCertificateProps.Jsii$Proxy) o;
if (!certificate.equals(that.certificate)) return false;
if (!usage.equals(that.usage)) return false;
if (this.activeDate != null ? !this.activeDate.equals(that.activeDate) : that.activeDate != null) return false;
if (this.certificateChain != null ? !this.certificateChain.equals(that.certificateChain) : that.certificateChain != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.inactiveDate != null ? !this.inactiveDate.equals(that.inactiveDate) : that.inactiveDate != null) return false;
if (this.privateKey != null ? !this.privateKey.equals(that.privateKey) : that.privateKey != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public final int hashCode() {
int result = this.certificate.hashCode();
result = 31 * result + (this.usage.hashCode());
result = 31 * result + (this.activeDate != null ? this.activeDate.hashCode() : 0);
result = 31 * result + (this.certificateChain != null ? this.certificateChain.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.inactiveDate != null ? this.inactiveDate.hashCode() : 0);
result = 31 * result + (this.privateKey != null ? this.privateKey.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy