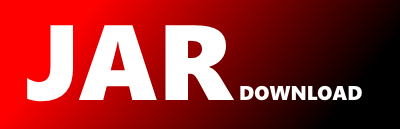
software.amazon.awsconstructs.services.apigatewaykinesisstreams.ApiGatewayToKinesisStreamsProps Maven / Gradle / Ivy
Show all versions of apigatewaykinesisstreams Show documentation
package software.amazon.awsconstructs.services.apigatewaykinesisstreams;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.106.0 (build e852934)", date = "2024-12-24T18:32:46.879Z")
@software.amazon.jsii.Jsii(module = software.amazon.awsconstructs.services.apigatewaykinesisstreams.$Module.class, fqn = "@aws-solutions-constructs/aws-apigateway-kinesisstreams.ApiGatewayToKinesisStreamsProps")
@software.amazon.jsii.Jsii.Proxy(ApiGatewayToKinesisStreamsProps.Jsii$Proxy.class)
public interface ApiGatewayToKinesisStreamsProps extends software.amazon.jsii.JsiiSerializable {
/**
* Optional PutRecord Request Templates for content-types other than application/json
.
*
* Use the putRecordRequestTemplate
property to set the request template for the application/json
content-type.
*
* Default: - None
*/
default @org.jetbrains.annotations.Nullable java.util.Map getAdditionalPutRecordRequestTemplates() {
return null;
}
/**
* Optional PutRecords Request Templates for content-types other than application/json
.
*
* Use the putRecordsRequestTemplate
property to set the request template for the application/json
content-type.
*
* Default: - None
*/
default @org.jetbrains.annotations.Nullable java.util.Map getAdditionalPutRecordsRequestTemplates() {
return null;
}
/**
* Optional user-provided props to override the default props for the API Gateway.
*
* Default: - Default properties are used.
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.apigateway.RestApiProps getApiGatewayProps() {
return null;
}
/**
* Whether to create recommended CloudWatch alarms.
*
* Default: - Alarms are created
*/
default @org.jetbrains.annotations.Nullable java.lang.Boolean getCreateCloudWatchAlarms() {
return null;
}
/**
* Existing instance of Kinesis Stream, providing both this and kinesisStreamProps
will cause an error.
*
* Default: - None
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.kinesis.Stream getExistingStreamObj() {
return null;
}
/**
* Optional user-provided props to override the default props for the Kinesis Data Stream.
*
* Default: - Default properties are used.
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.kinesis.StreamProps getKinesisStreamProps() {
return null;
}
/**
* User provided props to override the default props for the CloudWatchLogs LogGroup.
*
* Default: - Default props are used
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.logs.LogGroupProps getLogGroupProps() {
return null;
}
/**
* Optional, custom API Gateway Integration Response for the PutRecord action.
*
* Default: - [{statusCode:"200"},{statusCode:"500",responseTemplates:{"text/html":"Error"},selectionPattern:"500"}]
*/
default @org.jetbrains.annotations.Nullable java.util.List getPutRecordIntegrationResponses() {
return null;
}
/**
* Optional, custom API Gateway Method Responses for the PutRecord action.
*
* Default: - [
* {
* statusCode: "200",
* responseParameters: {
* "method.response.header.Content-Type": true
* }
* },
* {
* statusCode: "500",
* responseParameters: {
* "method.response.header.Content-Type": true
* },
* }
* ]
*/
default @org.jetbrains.annotations.Nullable java.util.List getPutRecordMethodResponses() {
return null;
}
/**
* API Gateway request model for the PutRecord action.
*
* If not provided, a default one will be created.
*
* Default: - {"$schema":"http://json-schema.org/draft-04/schema#","title":"PutRecord proxy single-record payload","type":"object",
* "required":["data","partitionKey"],"properties":{"data":{"type":"string"},"partitionKey":{"type":"string"}}}
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.apigateway.ModelOptions getPutRecordRequestModel() {
return null;
}
/**
* API Gateway request template for the PutRecord action.
*
* If not provided, a default one will be used.
*
* Default: - { "StreamName": "${this.kinesisStream.streamName}", "Data": "$util.base64Encode($input.json('$.data'))",
* "PartitionKey": "$input.path('$.partitionKey')" }
*/
default @org.jetbrains.annotations.Nullable java.lang.String getPutRecordRequestTemplate() {
return null;
}
/**
* Optional, custom API Gateway Integration Response for the PutRecords action.
*
* Default: - [{statusCode:"200"},{statusCode:"500",responseTemplates:{"text/html":"Error"},selectionPattern:"500"}]
*/
default @org.jetbrains.annotations.Nullable java.util.List getPutRecordsIntegrationResponses() {
return null;
}
/**
* Optional, custom API Gateway Method Responses for the PutRecord action.
*
* Default: - [
* {
* statusCode: "200",
* responseParameters: {
* "method.response.header.Content-Type": true
* }
* },
* {
* statusCode: "500",
* responseParameters: {
* "method.response.header.Content-Type": true
* },
* }
* ]
*/
default @org.jetbrains.annotations.Nullable java.util.List getPutRecordsMethodResponses() {
return null;
}
/**
* API Gateway request model for the PutRecords action.
*
* If not provided, a default one will be created.
*
* Default: - {"$schema":"http://json-schema.org/draft-04/schema#","title":"PutRecords proxy payload data","type":"object","required":["records"],
* "properties":{"records":{"type":"array","items":{"type":"object",
* "required":["data","partitionKey"],"properties":{"data":{"type":"string"},"partitionKey":{"type":"string"}}}}}}
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.apigateway.ModelOptions getPutRecordsRequestModel() {
return null;
}
/**
* API Gateway request template for the PutRecords action for the default application/json
content-type.
*
* If not provided, a default one will be used.
*
* Default: - { "StreamName": "${this.kinesisStream.streamName}", "Records": [ #foreach($elem in $input.path('$.records'))
* { "Data": "$util.base64Encode($elem.data)", "PartitionKey": "$elem.partitionKey"}#if($foreach.hasNext),#end #end ] }
*/
default @org.jetbrains.annotations.Nullable java.lang.String getPutRecordsRequestTemplate() {
return null;
}
/**
* @return a {@link Builder} of {@link ApiGatewayToKinesisStreamsProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ApiGatewayToKinesisStreamsProps}
*/
public static final class Builder implements software.amazon.jsii.Builder {
java.util.Map additionalPutRecordRequestTemplates;
java.util.Map additionalPutRecordsRequestTemplates;
software.amazon.awscdk.services.apigateway.RestApiProps apiGatewayProps;
java.lang.Boolean createCloudWatchAlarms;
software.amazon.awscdk.services.kinesis.Stream existingStreamObj;
software.amazon.awscdk.services.kinesis.StreamProps kinesisStreamProps;
software.amazon.awscdk.services.logs.LogGroupProps logGroupProps;
java.util.List putRecordIntegrationResponses;
java.util.List putRecordMethodResponses;
software.amazon.awscdk.services.apigateway.ModelOptions putRecordRequestModel;
java.lang.String putRecordRequestTemplate;
java.util.List putRecordsIntegrationResponses;
java.util.List putRecordsMethodResponses;
software.amazon.awscdk.services.apigateway.ModelOptions putRecordsRequestModel;
java.lang.String putRecordsRequestTemplate;
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getAdditionalPutRecordRequestTemplates}
* @param additionalPutRecordRequestTemplates Optional PutRecord Request Templates for content-types other than application/json
.
* Use the putRecordRequestTemplate
property to set the request template for the application/json
content-type.
* @return {@code this}
*/
public Builder additionalPutRecordRequestTemplates(java.util.Map additionalPutRecordRequestTemplates) {
this.additionalPutRecordRequestTemplates = additionalPutRecordRequestTemplates;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getAdditionalPutRecordsRequestTemplates}
* @param additionalPutRecordsRequestTemplates Optional PutRecords Request Templates for content-types other than application/json
.
* Use the putRecordsRequestTemplate
property to set the request template for the application/json
content-type.
* @return {@code this}
*/
public Builder additionalPutRecordsRequestTemplates(java.util.Map additionalPutRecordsRequestTemplates) {
this.additionalPutRecordsRequestTemplates = additionalPutRecordsRequestTemplates;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getApiGatewayProps}
* @param apiGatewayProps Optional user-provided props to override the default props for the API Gateway.
* @return {@code this}
*/
public Builder apiGatewayProps(software.amazon.awscdk.services.apigateway.RestApiProps apiGatewayProps) {
this.apiGatewayProps = apiGatewayProps;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getCreateCloudWatchAlarms}
* @param createCloudWatchAlarms Whether to create recommended CloudWatch alarms.
* @return {@code this}
*/
public Builder createCloudWatchAlarms(java.lang.Boolean createCloudWatchAlarms) {
this.createCloudWatchAlarms = createCloudWatchAlarms;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getExistingStreamObj}
* @param existingStreamObj Existing instance of Kinesis Stream, providing both this and kinesisStreamProps
will cause an error.
* @return {@code this}
*/
public Builder existingStreamObj(software.amazon.awscdk.services.kinesis.Stream existingStreamObj) {
this.existingStreamObj = existingStreamObj;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getKinesisStreamProps}
* @param kinesisStreamProps Optional user-provided props to override the default props for the Kinesis Data Stream.
* @return {@code this}
*/
public Builder kinesisStreamProps(software.amazon.awscdk.services.kinesis.StreamProps kinesisStreamProps) {
this.kinesisStreamProps = kinesisStreamProps;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getLogGroupProps}
* @param logGroupProps User provided props to override the default props for the CloudWatchLogs LogGroup.
* @return {@code this}
*/
public Builder logGroupProps(software.amazon.awscdk.services.logs.LogGroupProps logGroupProps) {
this.logGroupProps = logGroupProps;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordIntegrationResponses}
* @param putRecordIntegrationResponses Optional, custom API Gateway Integration Response for the PutRecord action.
* @return {@code this}
*/
@SuppressWarnings("unchecked")
public Builder putRecordIntegrationResponses(java.util.List extends software.amazon.awscdk.services.apigateway.IntegrationResponse> putRecordIntegrationResponses) {
this.putRecordIntegrationResponses = (java.util.List)putRecordIntegrationResponses;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordMethodResponses}
* @param putRecordMethodResponses Optional, custom API Gateway Method Responses for the PutRecord action.
* @return {@code this}
*/
@SuppressWarnings("unchecked")
public Builder putRecordMethodResponses(java.util.List extends software.amazon.awscdk.services.apigateway.MethodResponse> putRecordMethodResponses) {
this.putRecordMethodResponses = (java.util.List)putRecordMethodResponses;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordRequestModel}
* @param putRecordRequestModel API Gateway request model for the PutRecord action.
* If not provided, a default one will be created.
* @return {@code this}
*/
public Builder putRecordRequestModel(software.amazon.awscdk.services.apigateway.ModelOptions putRecordRequestModel) {
this.putRecordRequestModel = putRecordRequestModel;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordRequestTemplate}
* @param putRecordRequestTemplate API Gateway request template for the PutRecord action.
* If not provided, a default one will be used.
* @return {@code this}
*/
public Builder putRecordRequestTemplate(java.lang.String putRecordRequestTemplate) {
this.putRecordRequestTemplate = putRecordRequestTemplate;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordsIntegrationResponses}
* @param putRecordsIntegrationResponses Optional, custom API Gateway Integration Response for the PutRecords action.
* @return {@code this}
*/
@SuppressWarnings("unchecked")
public Builder putRecordsIntegrationResponses(java.util.List extends software.amazon.awscdk.services.apigateway.IntegrationResponse> putRecordsIntegrationResponses) {
this.putRecordsIntegrationResponses = (java.util.List)putRecordsIntegrationResponses;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordsMethodResponses}
* @param putRecordsMethodResponses Optional, custom API Gateway Method Responses for the PutRecord action.
* @return {@code this}
*/
@SuppressWarnings("unchecked")
public Builder putRecordsMethodResponses(java.util.List extends software.amazon.awscdk.services.apigateway.MethodResponse> putRecordsMethodResponses) {
this.putRecordsMethodResponses = (java.util.List)putRecordsMethodResponses;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordsRequestModel}
* @param putRecordsRequestModel API Gateway request model for the PutRecords action.
* If not provided, a default one will be created.
* @return {@code this}
*/
public Builder putRecordsRequestModel(software.amazon.awscdk.services.apigateway.ModelOptions putRecordsRequestModel) {
this.putRecordsRequestModel = putRecordsRequestModel;
return this;
}
/**
* Sets the value of {@link ApiGatewayToKinesisStreamsProps#getPutRecordsRequestTemplate}
* @param putRecordsRequestTemplate API Gateway request template for the PutRecords action for the default application/json
content-type.
* If not provided, a default one will be used.
* @return {@code this}
*/
public Builder putRecordsRequestTemplate(java.lang.String putRecordsRequestTemplate) {
this.putRecordsRequestTemplate = putRecordsRequestTemplate;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ApiGatewayToKinesisStreamsProps}
* @throws NullPointerException if any required attribute was not provided
*/
@Override
public ApiGatewayToKinesisStreamsProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ApiGatewayToKinesisStreamsProps}
*/
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ApiGatewayToKinesisStreamsProps {
private final java.util.Map additionalPutRecordRequestTemplates;
private final java.util.Map additionalPutRecordsRequestTemplates;
private final software.amazon.awscdk.services.apigateway.RestApiProps apiGatewayProps;
private final java.lang.Boolean createCloudWatchAlarms;
private final software.amazon.awscdk.services.kinesis.Stream existingStreamObj;
private final software.amazon.awscdk.services.kinesis.StreamProps kinesisStreamProps;
private final software.amazon.awscdk.services.logs.LogGroupProps logGroupProps;
private final java.util.List putRecordIntegrationResponses;
private final java.util.List putRecordMethodResponses;
private final software.amazon.awscdk.services.apigateway.ModelOptions putRecordRequestModel;
private final java.lang.String putRecordRequestTemplate;
private final java.util.List putRecordsIntegrationResponses;
private final java.util.List putRecordsMethodResponses;
private final software.amazon.awscdk.services.apigateway.ModelOptions putRecordsRequestModel;
private final java.lang.String putRecordsRequestTemplate;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.additionalPutRecordRequestTemplates = software.amazon.jsii.Kernel.get(this, "additionalPutRecordRequestTemplates", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.additionalPutRecordsRequestTemplates = software.amazon.jsii.Kernel.get(this, "additionalPutRecordsRequestTemplates", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.apiGatewayProps = software.amazon.jsii.Kernel.get(this, "apiGatewayProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.RestApiProps.class));
this.createCloudWatchAlarms = software.amazon.jsii.Kernel.get(this, "createCloudWatchAlarms", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.existingStreamObj = software.amazon.jsii.Kernel.get(this, "existingStreamObj", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.kinesis.Stream.class));
this.kinesisStreamProps = software.amazon.jsii.Kernel.get(this, "kinesisStreamProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.kinesis.StreamProps.class));
this.logGroupProps = software.amazon.jsii.Kernel.get(this, "logGroupProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.logs.LogGroupProps.class));
this.putRecordIntegrationResponses = software.amazon.jsii.Kernel.get(this, "putRecordIntegrationResponses", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.IntegrationResponse.class)));
this.putRecordMethodResponses = software.amazon.jsii.Kernel.get(this, "putRecordMethodResponses", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.MethodResponse.class)));
this.putRecordRequestModel = software.amazon.jsii.Kernel.get(this, "putRecordRequestModel", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.ModelOptions.class));
this.putRecordRequestTemplate = software.amazon.jsii.Kernel.get(this, "putRecordRequestTemplate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.putRecordsIntegrationResponses = software.amazon.jsii.Kernel.get(this, "putRecordsIntegrationResponses", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.IntegrationResponse.class)));
this.putRecordsMethodResponses = software.amazon.jsii.Kernel.get(this, "putRecordsMethodResponses", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.MethodResponse.class)));
this.putRecordsRequestModel = software.amazon.jsii.Kernel.get(this, "putRecordsRequestModel", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apigateway.ModelOptions.class));
this.putRecordsRequestTemplate = software.amazon.jsii.Kernel.get(this, "putRecordsRequestTemplate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.additionalPutRecordRequestTemplates = builder.additionalPutRecordRequestTemplates;
this.additionalPutRecordsRequestTemplates = builder.additionalPutRecordsRequestTemplates;
this.apiGatewayProps = builder.apiGatewayProps;
this.createCloudWatchAlarms = builder.createCloudWatchAlarms;
this.existingStreamObj = builder.existingStreamObj;
this.kinesisStreamProps = builder.kinesisStreamProps;
this.logGroupProps = builder.logGroupProps;
this.putRecordIntegrationResponses = (java.util.List)builder.putRecordIntegrationResponses;
this.putRecordMethodResponses = (java.util.List)builder.putRecordMethodResponses;
this.putRecordRequestModel = builder.putRecordRequestModel;
this.putRecordRequestTemplate = builder.putRecordRequestTemplate;
this.putRecordsIntegrationResponses = (java.util.List)builder.putRecordsIntegrationResponses;
this.putRecordsMethodResponses = (java.util.List)builder.putRecordsMethodResponses;
this.putRecordsRequestModel = builder.putRecordsRequestModel;
this.putRecordsRequestTemplate = builder.putRecordsRequestTemplate;
}
@Override
public final java.util.Map getAdditionalPutRecordRequestTemplates() {
return this.additionalPutRecordRequestTemplates;
}
@Override
public final java.util.Map getAdditionalPutRecordsRequestTemplates() {
return this.additionalPutRecordsRequestTemplates;
}
@Override
public final software.amazon.awscdk.services.apigateway.RestApiProps getApiGatewayProps() {
return this.apiGatewayProps;
}
@Override
public final java.lang.Boolean getCreateCloudWatchAlarms() {
return this.createCloudWatchAlarms;
}
@Override
public final software.amazon.awscdk.services.kinesis.Stream getExistingStreamObj() {
return this.existingStreamObj;
}
@Override
public final software.amazon.awscdk.services.kinesis.StreamProps getKinesisStreamProps() {
return this.kinesisStreamProps;
}
@Override
public final software.amazon.awscdk.services.logs.LogGroupProps getLogGroupProps() {
return this.logGroupProps;
}
@Override
public final java.util.List getPutRecordIntegrationResponses() {
return this.putRecordIntegrationResponses;
}
@Override
public final java.util.List getPutRecordMethodResponses() {
return this.putRecordMethodResponses;
}
@Override
public final software.amazon.awscdk.services.apigateway.ModelOptions getPutRecordRequestModel() {
return this.putRecordRequestModel;
}
@Override
public final java.lang.String getPutRecordRequestTemplate() {
return this.putRecordRequestTemplate;
}
@Override
public final java.util.List getPutRecordsIntegrationResponses() {
return this.putRecordsIntegrationResponses;
}
@Override
public final java.util.List getPutRecordsMethodResponses() {
return this.putRecordsMethodResponses;
}
@Override
public final software.amazon.awscdk.services.apigateway.ModelOptions getPutRecordsRequestModel() {
return this.putRecordsRequestModel;
}
@Override
public final java.lang.String getPutRecordsRequestTemplate() {
return this.putRecordsRequestTemplate;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAdditionalPutRecordRequestTemplates() != null) {
data.set("additionalPutRecordRequestTemplates", om.valueToTree(this.getAdditionalPutRecordRequestTemplates()));
}
if (this.getAdditionalPutRecordsRequestTemplates() != null) {
data.set("additionalPutRecordsRequestTemplates", om.valueToTree(this.getAdditionalPutRecordsRequestTemplates()));
}
if (this.getApiGatewayProps() != null) {
data.set("apiGatewayProps", om.valueToTree(this.getApiGatewayProps()));
}
if (this.getCreateCloudWatchAlarms() != null) {
data.set("createCloudWatchAlarms", om.valueToTree(this.getCreateCloudWatchAlarms()));
}
if (this.getExistingStreamObj() != null) {
data.set("existingStreamObj", om.valueToTree(this.getExistingStreamObj()));
}
if (this.getKinesisStreamProps() != null) {
data.set("kinesisStreamProps", om.valueToTree(this.getKinesisStreamProps()));
}
if (this.getLogGroupProps() != null) {
data.set("logGroupProps", om.valueToTree(this.getLogGroupProps()));
}
if (this.getPutRecordIntegrationResponses() != null) {
data.set("putRecordIntegrationResponses", om.valueToTree(this.getPutRecordIntegrationResponses()));
}
if (this.getPutRecordMethodResponses() != null) {
data.set("putRecordMethodResponses", om.valueToTree(this.getPutRecordMethodResponses()));
}
if (this.getPutRecordRequestModel() != null) {
data.set("putRecordRequestModel", om.valueToTree(this.getPutRecordRequestModel()));
}
if (this.getPutRecordRequestTemplate() != null) {
data.set("putRecordRequestTemplate", om.valueToTree(this.getPutRecordRequestTemplate()));
}
if (this.getPutRecordsIntegrationResponses() != null) {
data.set("putRecordsIntegrationResponses", om.valueToTree(this.getPutRecordsIntegrationResponses()));
}
if (this.getPutRecordsMethodResponses() != null) {
data.set("putRecordsMethodResponses", om.valueToTree(this.getPutRecordsMethodResponses()));
}
if (this.getPutRecordsRequestModel() != null) {
data.set("putRecordsRequestModel", om.valueToTree(this.getPutRecordsRequestModel()));
}
if (this.getPutRecordsRequestTemplate() != null) {
data.set("putRecordsRequestTemplate", om.valueToTree(this.getPutRecordsRequestTemplate()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-solutions-constructs/aws-apigateway-kinesisstreams.ApiGatewayToKinesisStreamsProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ApiGatewayToKinesisStreamsProps.Jsii$Proxy that = (ApiGatewayToKinesisStreamsProps.Jsii$Proxy) o;
if (this.additionalPutRecordRequestTemplates != null ? !this.additionalPutRecordRequestTemplates.equals(that.additionalPutRecordRequestTemplates) : that.additionalPutRecordRequestTemplates != null) return false;
if (this.additionalPutRecordsRequestTemplates != null ? !this.additionalPutRecordsRequestTemplates.equals(that.additionalPutRecordsRequestTemplates) : that.additionalPutRecordsRequestTemplates != null) return false;
if (this.apiGatewayProps != null ? !this.apiGatewayProps.equals(that.apiGatewayProps) : that.apiGatewayProps != null) return false;
if (this.createCloudWatchAlarms != null ? !this.createCloudWatchAlarms.equals(that.createCloudWatchAlarms) : that.createCloudWatchAlarms != null) return false;
if (this.existingStreamObj != null ? !this.existingStreamObj.equals(that.existingStreamObj) : that.existingStreamObj != null) return false;
if (this.kinesisStreamProps != null ? !this.kinesisStreamProps.equals(that.kinesisStreamProps) : that.kinesisStreamProps != null) return false;
if (this.logGroupProps != null ? !this.logGroupProps.equals(that.logGroupProps) : that.logGroupProps != null) return false;
if (this.putRecordIntegrationResponses != null ? !this.putRecordIntegrationResponses.equals(that.putRecordIntegrationResponses) : that.putRecordIntegrationResponses != null) return false;
if (this.putRecordMethodResponses != null ? !this.putRecordMethodResponses.equals(that.putRecordMethodResponses) : that.putRecordMethodResponses != null) return false;
if (this.putRecordRequestModel != null ? !this.putRecordRequestModel.equals(that.putRecordRequestModel) : that.putRecordRequestModel != null) return false;
if (this.putRecordRequestTemplate != null ? !this.putRecordRequestTemplate.equals(that.putRecordRequestTemplate) : that.putRecordRequestTemplate != null) return false;
if (this.putRecordsIntegrationResponses != null ? !this.putRecordsIntegrationResponses.equals(that.putRecordsIntegrationResponses) : that.putRecordsIntegrationResponses != null) return false;
if (this.putRecordsMethodResponses != null ? !this.putRecordsMethodResponses.equals(that.putRecordsMethodResponses) : that.putRecordsMethodResponses != null) return false;
if (this.putRecordsRequestModel != null ? !this.putRecordsRequestModel.equals(that.putRecordsRequestModel) : that.putRecordsRequestModel != null) return false;
return this.putRecordsRequestTemplate != null ? this.putRecordsRequestTemplate.equals(that.putRecordsRequestTemplate) : that.putRecordsRequestTemplate == null;
}
@Override
public final int hashCode() {
int result = this.additionalPutRecordRequestTemplates != null ? this.additionalPutRecordRequestTemplates.hashCode() : 0;
result = 31 * result + (this.additionalPutRecordsRequestTemplates != null ? this.additionalPutRecordsRequestTemplates.hashCode() : 0);
result = 31 * result + (this.apiGatewayProps != null ? this.apiGatewayProps.hashCode() : 0);
result = 31 * result + (this.createCloudWatchAlarms != null ? this.createCloudWatchAlarms.hashCode() : 0);
result = 31 * result + (this.existingStreamObj != null ? this.existingStreamObj.hashCode() : 0);
result = 31 * result + (this.kinesisStreamProps != null ? this.kinesisStreamProps.hashCode() : 0);
result = 31 * result + (this.logGroupProps != null ? this.logGroupProps.hashCode() : 0);
result = 31 * result + (this.putRecordIntegrationResponses != null ? this.putRecordIntegrationResponses.hashCode() : 0);
result = 31 * result + (this.putRecordMethodResponses != null ? this.putRecordMethodResponses.hashCode() : 0);
result = 31 * result + (this.putRecordRequestModel != null ? this.putRecordRequestModel.hashCode() : 0);
result = 31 * result + (this.putRecordRequestTemplate != null ? this.putRecordRequestTemplate.hashCode() : 0);
result = 31 * result + (this.putRecordsIntegrationResponses != null ? this.putRecordsIntegrationResponses.hashCode() : 0);
result = 31 * result + (this.putRecordsMethodResponses != null ? this.putRecordsMethodResponses.hashCode() : 0);
result = 31 * result + (this.putRecordsRequestModel != null ? this.putRecordsRequestModel.hashCode() : 0);
result = 31 * result + (this.putRecordsRequestTemplate != null ? this.putRecordsRequestTemplate.hashCode() : 0);
return result;
}
}
}