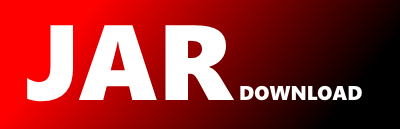
software.amazon.awsconstructs.services.dynamodbstreamslambda.DynamoDBStreamsToLambdaProps Maven / Gradle / Ivy
Show all versions of dynamodbstreamslambda Show documentation
package software.amazon.awsconstructs.services.dynamodbstreamslambda;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.103.1 (build bef2dea)", date = "2024-09-18T13:39:27.747Z")
@software.amazon.jsii.Jsii(module = software.amazon.awsconstructs.services.dynamodbstreamslambda.$Module.class, fqn = "@aws-solutions-constructs/aws-dynamodbstreams-lambda.DynamoDBStreamsToLambdaProps")
@software.amazon.jsii.Jsii.Proxy(DynamoDBStreamsToLambdaProps.Jsii$Proxy.class)
public interface DynamoDBStreamsToLambdaProps extends software.amazon.jsii.JsiiSerializable {
/**
* Whether to deploy a SQS dead letter queue when a data record reaches the Maximum Retry Attempts or Maximum Record Age, its metadata like shard ID and stream ARN will be sent to an SQS queue.
*
* Default: - true.
*/
default @org.jetbrains.annotations.Nullable java.lang.Boolean getDeploySqsDlqQueue() {
return null;
}
/**
* Optional user provided props to override the default props.
*
* Default: - Default props are used
*/
default @org.jetbrains.annotations.Nullable java.lang.Object getDynamoEventSourceProps() {
return null;
}
/**
* Optional user provided props to override the default props.
*
* Default: - Default props are used
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.dynamodb.TableProps getDynamoTableProps() {
return null;
}
/**
* Existing instance of Lambda Function object, providing both this and lambdaFunctionProps
will cause an error.
*
* Default: - None
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.lambda.Function getExistingLambdaObj() {
return null;
}
/**
* Existing instance of DynamoDB table object, providing both this and dynamoTableProps
will cause an error.
*
* Default: - None
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.dynamodb.ITable getExistingTableInterface() {
return null;
}
/**
* User provided props to override the default props for the Lambda function.
*
* Default: - Default props are used
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.lambda.FunctionProps getLambdaFunctionProps() {
return null;
}
/**
* Optional user provided properties for the SQS dead letter queue.
*
* Default: - Default props are used
*/
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.sqs.QueueProps getSqsDlqQueueProps() {
return null;
}
/**
* @return a {@link Builder} of {@link DynamoDBStreamsToLambdaProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DynamoDBStreamsToLambdaProps}
*/
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Boolean deploySqsDlqQueue;
java.lang.Object dynamoEventSourceProps;
software.amazon.awscdk.services.dynamodb.TableProps dynamoTableProps;
software.amazon.awscdk.services.lambda.Function existingLambdaObj;
software.amazon.awscdk.services.dynamodb.ITable existingTableInterface;
software.amazon.awscdk.services.lambda.FunctionProps lambdaFunctionProps;
software.amazon.awscdk.services.sqs.QueueProps sqsDlqQueueProps;
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getDeploySqsDlqQueue}
* @param deploySqsDlqQueue Whether to deploy a SQS dead letter queue when a data record reaches the Maximum Retry Attempts or Maximum Record Age, its metadata like shard ID and stream ARN will be sent to an SQS queue.
* @return {@code this}
*/
public Builder deploySqsDlqQueue(java.lang.Boolean deploySqsDlqQueue) {
this.deploySqsDlqQueue = deploySqsDlqQueue;
return this;
}
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getDynamoEventSourceProps}
* @param dynamoEventSourceProps Optional user provided props to override the default props.
* @return {@code this}
*/
public Builder dynamoEventSourceProps(java.lang.Object dynamoEventSourceProps) {
this.dynamoEventSourceProps = dynamoEventSourceProps;
return this;
}
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getDynamoTableProps}
* @param dynamoTableProps Optional user provided props to override the default props.
* @return {@code this}
*/
public Builder dynamoTableProps(software.amazon.awscdk.services.dynamodb.TableProps dynamoTableProps) {
this.dynamoTableProps = dynamoTableProps;
return this;
}
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getExistingLambdaObj}
* @param existingLambdaObj Existing instance of Lambda Function object, providing both this and lambdaFunctionProps
will cause an error.
* @return {@code this}
*/
public Builder existingLambdaObj(software.amazon.awscdk.services.lambda.Function existingLambdaObj) {
this.existingLambdaObj = existingLambdaObj;
return this;
}
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getExistingTableInterface}
* @param existingTableInterface Existing instance of DynamoDB table object, providing both this and dynamoTableProps
will cause an error.
* @return {@code this}
*/
public Builder existingTableInterface(software.amazon.awscdk.services.dynamodb.ITable existingTableInterface) {
this.existingTableInterface = existingTableInterface;
return this;
}
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getLambdaFunctionProps}
* @param lambdaFunctionProps User provided props to override the default props for the Lambda function.
* @return {@code this}
*/
public Builder lambdaFunctionProps(software.amazon.awscdk.services.lambda.FunctionProps lambdaFunctionProps) {
this.lambdaFunctionProps = lambdaFunctionProps;
return this;
}
/**
* Sets the value of {@link DynamoDBStreamsToLambdaProps#getSqsDlqQueueProps}
* @param sqsDlqQueueProps Optional user provided properties for the SQS dead letter queue.
* @return {@code this}
*/
public Builder sqsDlqQueueProps(software.amazon.awscdk.services.sqs.QueueProps sqsDlqQueueProps) {
this.sqsDlqQueueProps = sqsDlqQueueProps;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DynamoDBStreamsToLambdaProps}
* @throws NullPointerException if any required attribute was not provided
*/
@Override
public DynamoDBStreamsToLambdaProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DynamoDBStreamsToLambdaProps}
*/
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DynamoDBStreamsToLambdaProps {
private final java.lang.Boolean deploySqsDlqQueue;
private final java.lang.Object dynamoEventSourceProps;
private final software.amazon.awscdk.services.dynamodb.TableProps dynamoTableProps;
private final software.amazon.awscdk.services.lambda.Function existingLambdaObj;
private final software.amazon.awscdk.services.dynamodb.ITable existingTableInterface;
private final software.amazon.awscdk.services.lambda.FunctionProps lambdaFunctionProps;
private final software.amazon.awscdk.services.sqs.QueueProps sqsDlqQueueProps;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.deploySqsDlqQueue = software.amazon.jsii.Kernel.get(this, "deploySqsDlqQueue", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.dynamoEventSourceProps = software.amazon.jsii.Kernel.get(this, "dynamoEventSourceProps", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.dynamoTableProps = software.amazon.jsii.Kernel.get(this, "dynamoTableProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.dynamodb.TableProps.class));
this.existingLambdaObj = software.amazon.jsii.Kernel.get(this, "existingLambdaObj", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.Function.class));
this.existingTableInterface = software.amazon.jsii.Kernel.get(this, "existingTableInterface", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.dynamodb.ITable.class));
this.lambdaFunctionProps = software.amazon.jsii.Kernel.get(this, "lambdaFunctionProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.FunctionProps.class));
this.sqsDlqQueueProps = software.amazon.jsii.Kernel.get(this, "sqsDlqQueueProps", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.sqs.QueueProps.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.deploySqsDlqQueue = builder.deploySqsDlqQueue;
this.dynamoEventSourceProps = builder.dynamoEventSourceProps;
this.dynamoTableProps = builder.dynamoTableProps;
this.existingLambdaObj = builder.existingLambdaObj;
this.existingTableInterface = builder.existingTableInterface;
this.lambdaFunctionProps = builder.lambdaFunctionProps;
this.sqsDlqQueueProps = builder.sqsDlqQueueProps;
}
@Override
public final java.lang.Boolean getDeploySqsDlqQueue() {
return this.deploySqsDlqQueue;
}
@Override
public final java.lang.Object getDynamoEventSourceProps() {
return this.dynamoEventSourceProps;
}
@Override
public final software.amazon.awscdk.services.dynamodb.TableProps getDynamoTableProps() {
return this.dynamoTableProps;
}
@Override
public final software.amazon.awscdk.services.lambda.Function getExistingLambdaObj() {
return this.existingLambdaObj;
}
@Override
public final software.amazon.awscdk.services.dynamodb.ITable getExistingTableInterface() {
return this.existingTableInterface;
}
@Override
public final software.amazon.awscdk.services.lambda.FunctionProps getLambdaFunctionProps() {
return this.lambdaFunctionProps;
}
@Override
public final software.amazon.awscdk.services.sqs.QueueProps getSqsDlqQueueProps() {
return this.sqsDlqQueueProps;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDeploySqsDlqQueue() != null) {
data.set("deploySqsDlqQueue", om.valueToTree(this.getDeploySqsDlqQueue()));
}
if (this.getDynamoEventSourceProps() != null) {
data.set("dynamoEventSourceProps", om.valueToTree(this.getDynamoEventSourceProps()));
}
if (this.getDynamoTableProps() != null) {
data.set("dynamoTableProps", om.valueToTree(this.getDynamoTableProps()));
}
if (this.getExistingLambdaObj() != null) {
data.set("existingLambdaObj", om.valueToTree(this.getExistingLambdaObj()));
}
if (this.getExistingTableInterface() != null) {
data.set("existingTableInterface", om.valueToTree(this.getExistingTableInterface()));
}
if (this.getLambdaFunctionProps() != null) {
data.set("lambdaFunctionProps", om.valueToTree(this.getLambdaFunctionProps()));
}
if (this.getSqsDlqQueueProps() != null) {
data.set("sqsDlqQueueProps", om.valueToTree(this.getSqsDlqQueueProps()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-solutions-constructs/aws-dynamodbstreams-lambda.DynamoDBStreamsToLambdaProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DynamoDBStreamsToLambdaProps.Jsii$Proxy that = (DynamoDBStreamsToLambdaProps.Jsii$Proxy) o;
if (this.deploySqsDlqQueue != null ? !this.deploySqsDlqQueue.equals(that.deploySqsDlqQueue) : that.deploySqsDlqQueue != null) return false;
if (this.dynamoEventSourceProps != null ? !this.dynamoEventSourceProps.equals(that.dynamoEventSourceProps) : that.dynamoEventSourceProps != null) return false;
if (this.dynamoTableProps != null ? !this.dynamoTableProps.equals(that.dynamoTableProps) : that.dynamoTableProps != null) return false;
if (this.existingLambdaObj != null ? !this.existingLambdaObj.equals(that.existingLambdaObj) : that.existingLambdaObj != null) return false;
if (this.existingTableInterface != null ? !this.existingTableInterface.equals(that.existingTableInterface) : that.existingTableInterface != null) return false;
if (this.lambdaFunctionProps != null ? !this.lambdaFunctionProps.equals(that.lambdaFunctionProps) : that.lambdaFunctionProps != null) return false;
return this.sqsDlqQueueProps != null ? this.sqsDlqQueueProps.equals(that.sqsDlqQueueProps) : that.sqsDlqQueueProps == null;
}
@Override
public final int hashCode() {
int result = this.deploySqsDlqQueue != null ? this.deploySqsDlqQueue.hashCode() : 0;
result = 31 * result + (this.dynamoEventSourceProps != null ? this.dynamoEventSourceProps.hashCode() : 0);
result = 31 * result + (this.dynamoTableProps != null ? this.dynamoTableProps.hashCode() : 0);
result = 31 * result + (this.existingLambdaObj != null ? this.existingLambdaObj.hashCode() : 0);
result = 31 * result + (this.existingTableInterface != null ? this.existingTableInterface.hashCode() : 0);
result = 31 * result + (this.lambdaFunctionProps != null ? this.lambdaFunctionProps.hashCode() : 0);
result = 31 * result + (this.sqsDlqQueueProps != null ? this.sqsDlqQueueProps.hashCode() : 0);
return result;
}
}
}