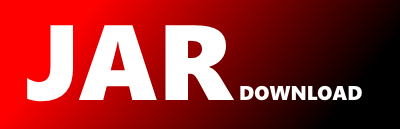
software.amazon.awssdk.services.alexaforbusiness.AlexaForBusinessAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.alexaforbusiness;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.alexaforbusiness.model.ApproveSkillRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ApproveSkillResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateContactWithAddressBookRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateContactWithAddressBookResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateDeviceWithNetworkProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateDeviceWithNetworkProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateDeviceWithRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateDeviceWithRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateSkillGroupWithRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateSkillGroupWithRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateSkillWithSkillGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateSkillWithSkillGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateSkillWithUsersRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.AssociateSkillWithUsersResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateAddressBookRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateAddressBookResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateBusinessReportScheduleRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateBusinessReportScheduleResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateConferenceProviderRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateConferenceProviderResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateContactRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateContactResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateGatewayGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateGatewayGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateNetworkProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateNetworkProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateSkillGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateSkillGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateUserRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.CreateUserResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteAddressBookRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteAddressBookResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteBusinessReportScheduleRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteBusinessReportScheduleResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteConferenceProviderRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteConferenceProviderResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteContactRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteContactResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteDeviceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteDeviceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteDeviceUsageDataRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteDeviceUsageDataResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteGatewayGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteGatewayGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteNetworkProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteNetworkProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteRoomSkillParameterRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteRoomSkillParameterResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteSkillAuthorizationRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteSkillAuthorizationResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteSkillGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteSkillGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteUserRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DeleteUserResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateContactFromAddressBookRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateContactFromAddressBookResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateDeviceFromRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateDeviceFromRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateSkillFromSkillGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateSkillFromSkillGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateSkillFromUsersRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateSkillFromUsersResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateSkillGroupFromRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.DisassociateSkillGroupFromRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ForgetSmartHomeAppliancesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ForgetSmartHomeAppliancesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetAddressBookRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetAddressBookResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetConferencePreferenceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetConferencePreferenceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetConferenceProviderRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetConferenceProviderResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetContactRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetContactResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetDeviceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetDeviceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetGatewayGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetGatewayGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetGatewayRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetGatewayResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetInvitationConfigurationRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetInvitationConfigurationResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetNetworkProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetNetworkProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetRoomSkillParameterRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetRoomSkillParameterResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.GetSkillGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.GetSkillGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ListTagsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ListTagsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.PutConferencePreferenceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.PutConferencePreferenceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.PutInvitationConfigurationRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.PutInvitationConfigurationResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.PutRoomSkillParameterRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.PutRoomSkillParameterResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.PutSkillAuthorizationRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.PutSkillAuthorizationResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.RegisterAvsDeviceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.RegisterAvsDeviceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.RejectSkillRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.RejectSkillResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.ResolveRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.ResolveRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.RevokeInvitationRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.RevokeInvitationResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SendAnnouncementRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SendAnnouncementResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.SendInvitationRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.SendInvitationResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.StartDeviceSyncRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.StartDeviceSyncResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.StartSmartHomeApplianceDiscoveryRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.StartSmartHomeApplianceDiscoveryResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.TagResourceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.TagResourceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UntagResourceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UntagResourceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateAddressBookRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateAddressBookResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateBusinessReportScheduleRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateBusinessReportScheduleResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateConferenceProviderRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateConferenceProviderResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateContactRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateContactResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateDeviceRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateDeviceResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateGatewayGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateGatewayGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateGatewayRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateGatewayResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateNetworkProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateNetworkProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateProfileRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateProfileResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateRoomRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateRoomResponse;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateSkillGroupRequest;
import software.amazon.awssdk.services.alexaforbusiness.model.UpdateSkillGroupResponse;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListBusinessReportSchedulesPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListConferenceProvidersPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListDeviceEventsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewayGroupsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewaysPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreCategoriesPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreSkillsByCategoryPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListSmartHomeAppliancesPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.ListTagsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchAddressBooksPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchContactsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchDevicesPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchNetworkProfilesPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchProfilesPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchRoomsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchSkillGroupsPublisher;
import software.amazon.awssdk.services.alexaforbusiness.paginators.SearchUsersPublisher;
/**
* Service client for accessing Alexa For Business asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* Alexa for Business helps you use Alexa in your organization. Alexa for Business provides you with the tools to manage
* Alexa devices, enroll your users, and assign skills, at scale. You can build your own context-aware voice skills
* using the Alexa Skills Kit and the Alexa for Business API operations. You can also make these available as private
* skills for your organization. Alexa for Business makes it efficient to voice-enable your products and services, thus
* providing context-aware voice experiences for your customers. Device makers building with the Alexa Voice Service
* (AVS) can create fully integrated solutions, register their products with Alexa for Business, and manage them as
* shared devices in their organization.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface AlexaForBusinessAsyncClient extends SdkClient {
String SERVICE_NAME = "a4b";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "a4b";
/**
* Create a {@link AlexaForBusinessAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static AlexaForBusinessAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link AlexaForBusinessAsyncClient}.
*/
static AlexaForBusinessAsyncClientBuilder builder() {
return new DefaultAlexaForBusinessAsyncClientBuilder();
}
/**
*
* Associates a skill with the organization under the customer's AWS account. If a skill is private, the user
* implicitly accepts access to this skill during enablement.
*
*
* @param approveSkillRequest
* @return A Java Future containing the result of the ApproveSkill operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ApproveSkill
* @see AWS
* API Documentation
*/
default CompletableFuture approveSkill(ApproveSkillRequest approveSkillRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a skill with the organization under the customer's AWS account. If a skill is private, the user
* implicitly accepts access to this skill during enablement.
*
*
*
* This is a convenience which creates an instance of the {@link ApproveSkillRequest.Builder} avoiding the need to
* create one manually via {@link ApproveSkillRequest#builder()}
*
*
* @param approveSkillRequest
* A {@link Consumer} that will call methods on {@link ApproveSkillRequest.Builder} to create a request.
* @return A Java Future containing the result of the ApproveSkill operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ApproveSkill
* @see AWS
* API Documentation
*/
default CompletableFuture approveSkill(Consumer approveSkillRequest) {
return approveSkill(ApproveSkillRequest.builder().applyMutation(approveSkillRequest).build());
}
/**
*
* Associates a contact with a given address book.
*
*
* @param associateContactWithAddressBookRequest
* @return A Java Future containing the result of the AssociateContactWithAddressBook operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateContactWithAddressBook
* @see AWS API Documentation
*/
default CompletableFuture associateContactWithAddressBook(
AssociateContactWithAddressBookRequest associateContactWithAddressBookRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a contact with a given address book.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateContactWithAddressBookRequest.Builder}
* avoiding the need to create one manually via {@link AssociateContactWithAddressBookRequest#builder()}
*
*
* @param associateContactWithAddressBookRequest
* A {@link Consumer} that will call methods on {@link AssociateContactWithAddressBookRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateContactWithAddressBook operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateContactWithAddressBook
* @see AWS API Documentation
*/
default CompletableFuture associateContactWithAddressBook(
Consumer associateContactWithAddressBookRequest) {
return associateContactWithAddressBook(AssociateContactWithAddressBookRequest.builder()
.applyMutation(associateContactWithAddressBookRequest).build());
}
/**
*
* Associates a device with the specified network profile.
*
*
* @param associateDeviceWithNetworkProfileRequest
* @return A Java Future containing the result of the AssociateDeviceWithNetworkProfile operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateDeviceWithNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture associateDeviceWithNetworkProfile(
AssociateDeviceWithNetworkProfileRequest associateDeviceWithNetworkProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a device with the specified network profile.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDeviceWithNetworkProfileRequest.Builder}
* avoiding the need to create one manually via {@link AssociateDeviceWithNetworkProfileRequest#builder()}
*
*
* @param associateDeviceWithNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link AssociateDeviceWithNetworkProfileRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateDeviceWithNetworkProfile operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateDeviceWithNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture associateDeviceWithNetworkProfile(
Consumer associateDeviceWithNetworkProfileRequest) {
return associateDeviceWithNetworkProfile(AssociateDeviceWithNetworkProfileRequest.builder()
.applyMutation(associateDeviceWithNetworkProfileRequest).build());
}
/**
*
* Associates a device with a given room. This applies all the settings from the room profile to the device, and all
* the skills in any skill groups added to that room. This operation requires the device to be online, or else a
* manual sync is required.
*
*
* @param associateDeviceWithRoomRequest
* @return A Java Future containing the result of the AssociateDeviceWithRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateDeviceWithRoom
* @see AWS API Documentation
*/
default CompletableFuture associateDeviceWithRoom(
AssociateDeviceWithRoomRequest associateDeviceWithRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a device with a given room. This applies all the settings from the room profile to the device, and all
* the skills in any skill groups added to that room. This operation requires the device to be online, or else a
* manual sync is required.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDeviceWithRoomRequest.Builder} avoiding
* the need to create one manually via {@link AssociateDeviceWithRoomRequest#builder()}
*
*
* @param associateDeviceWithRoomRequest
* A {@link Consumer} that will call methods on {@link AssociateDeviceWithRoomRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateDeviceWithRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateDeviceWithRoom
* @see AWS API Documentation
*/
default CompletableFuture associateDeviceWithRoom(
Consumer associateDeviceWithRoomRequest) {
return associateDeviceWithRoom(AssociateDeviceWithRoomRequest.builder().applyMutation(associateDeviceWithRoomRequest)
.build());
}
/**
*
* Associates a skill group with a given room. This enables all skills in the associated skill group on all devices
* in the room.
*
*
* @param associateSkillGroupWithRoomRequest
* @return A Java Future containing the result of the AssociateSkillGroupWithRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateSkillGroupWithRoom
* @see AWS API Documentation
*/
default CompletableFuture associateSkillGroupWithRoom(
AssociateSkillGroupWithRoomRequest associateSkillGroupWithRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a skill group with a given room. This enables all skills in the associated skill group on all devices
* in the room.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateSkillGroupWithRoomRequest.Builder}
* avoiding the need to create one manually via {@link AssociateSkillGroupWithRoomRequest#builder()}
*
*
* @param associateSkillGroupWithRoomRequest
* A {@link Consumer} that will call methods on {@link AssociateSkillGroupWithRoomRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the AssociateSkillGroupWithRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateSkillGroupWithRoom
* @see AWS API Documentation
*/
default CompletableFuture associateSkillGroupWithRoom(
Consumer associateSkillGroupWithRoomRequest) {
return associateSkillGroupWithRoom(AssociateSkillGroupWithRoomRequest.builder()
.applyMutation(associateSkillGroupWithRoomRequest).build());
}
/**
*
* Associates a skill with a skill group.
*
*
* @param associateSkillWithSkillGroupRequest
* @return A Java Future containing the result of the AssociateSkillWithSkillGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SkillNotLinkedException The skill must be linked to a third-party account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateSkillWithSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture associateSkillWithSkillGroup(
AssociateSkillWithSkillGroupRequest associateSkillWithSkillGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a skill with a skill group.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateSkillWithSkillGroupRequest.Builder}
* avoiding the need to create one manually via {@link AssociateSkillWithSkillGroupRequest#builder()}
*
*
* @param associateSkillWithSkillGroupRequest
* A {@link Consumer} that will call methods on {@link AssociateSkillWithSkillGroupRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the AssociateSkillWithSkillGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SkillNotLinkedException The skill must be linked to a third-party account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateSkillWithSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture associateSkillWithSkillGroup(
Consumer associateSkillWithSkillGroupRequest) {
return associateSkillWithSkillGroup(AssociateSkillWithSkillGroupRequest.builder()
.applyMutation(associateSkillWithSkillGroupRequest).build());
}
/**
*
* Makes a private skill available for enrolled users to enable on their devices.
*
*
* @param associateSkillWithUsersRequest
* @return A Java Future containing the result of the AssociateSkillWithUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateSkillWithUsers
* @see AWS API Documentation
*/
default CompletableFuture associateSkillWithUsers(
AssociateSkillWithUsersRequest associateSkillWithUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Makes a private skill available for enrolled users to enable on their devices.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateSkillWithUsersRequest.Builder} avoiding
* the need to create one manually via {@link AssociateSkillWithUsersRequest#builder()}
*
*
* @param associateSkillWithUsersRequest
* A {@link Consumer} that will call methods on {@link AssociateSkillWithUsersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateSkillWithUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.AssociateSkillWithUsers
* @see AWS API Documentation
*/
default CompletableFuture associateSkillWithUsers(
Consumer associateSkillWithUsersRequest) {
return associateSkillWithUsers(AssociateSkillWithUsersRequest.builder().applyMutation(associateSkillWithUsersRequest)
.build());
}
/**
*
* Creates an address book with the specified details.
*
*
* @param createAddressBookRequest
* @return A Java Future containing the result of the CreateAddressBook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateAddressBook
* @see AWS API Documentation
*/
default CompletableFuture createAddressBook(CreateAddressBookRequest createAddressBookRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an address book with the specified details.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAddressBookRequest.Builder} avoiding the need
* to create one manually via {@link CreateAddressBookRequest#builder()}
*
*
* @param createAddressBookRequest
* A {@link Consumer} that will call methods on {@link CreateAddressBookRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateAddressBook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateAddressBook
* @see AWS API Documentation
*/
default CompletableFuture createAddressBook(
Consumer createAddressBookRequest) {
return createAddressBook(CreateAddressBookRequest.builder().applyMutation(createAddressBookRequest).build());
}
/**
*
* Creates a recurring schedule for usage reports to deliver to the specified S3 location with a specified daily or
* weekly interval.
*
*
* @param createBusinessReportScheduleRequest
* @return A Java Future containing the result of the CreateBusinessReportSchedule operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateBusinessReportSchedule
* @see AWS API Documentation
*/
default CompletableFuture createBusinessReportSchedule(
CreateBusinessReportScheduleRequest createBusinessReportScheduleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a recurring schedule for usage reports to deliver to the specified S3 location with a specified daily or
* weekly interval.
*
*
*
* This is a convenience which creates an instance of the {@link CreateBusinessReportScheduleRequest.Builder}
* avoiding the need to create one manually via {@link CreateBusinessReportScheduleRequest#builder()}
*
*
* @param createBusinessReportScheduleRequest
* A {@link Consumer} that will call methods on {@link CreateBusinessReportScheduleRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateBusinessReportSchedule operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateBusinessReportSchedule
* @see AWS API Documentation
*/
default CompletableFuture createBusinessReportSchedule(
Consumer createBusinessReportScheduleRequest) {
return createBusinessReportSchedule(CreateBusinessReportScheduleRequest.builder()
.applyMutation(createBusinessReportScheduleRequest).build());
}
/**
*
* Adds a new conference provider under the user's AWS account.
*
*
* @param createConferenceProviderRequest
* @return A Java Future containing the result of the CreateConferenceProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateConferenceProvider
* @see AWS API Documentation
*/
default CompletableFuture createConferenceProvider(
CreateConferenceProviderRequest createConferenceProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a new conference provider under the user's AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link CreateConferenceProviderRequest.Builder} avoiding
* the need to create one manually via {@link CreateConferenceProviderRequest#builder()}
*
*
* @param createConferenceProviderRequest
* A {@link Consumer} that will call methods on {@link CreateConferenceProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateConferenceProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateConferenceProvider
* @see AWS API Documentation
*/
default CompletableFuture createConferenceProvider(
Consumer createConferenceProviderRequest) {
return createConferenceProvider(CreateConferenceProviderRequest.builder().applyMutation(createConferenceProviderRequest)
.build());
}
/**
*
* Creates a contact with the specified details.
*
*
* @param createContactRequest
* @return A Java Future containing the result of the CreateContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateContact
* @see AWS API Documentation
*/
default CompletableFuture createContact(CreateContactRequest createContactRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a contact with the specified details.
*
*
*
* This is a convenience which creates an instance of the {@link CreateContactRequest.Builder} avoiding the need to
* create one manually via {@link CreateContactRequest#builder()}
*
*
* @param createContactRequest
* A {@link Consumer} that will call methods on {@link CreateContactRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateContact
* @see AWS API Documentation
*/
default CompletableFuture createContact(Consumer createContactRequest) {
return createContact(CreateContactRequest.builder().applyMutation(createContactRequest).build());
}
/**
*
* Creates a gateway group with the specified details.
*
*
* @param createGatewayGroupRequest
* @return A Java Future containing the result of the CreateGatewayGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateGatewayGroup
* @see AWS API Documentation
*/
default CompletableFuture createGatewayGroup(CreateGatewayGroupRequest createGatewayGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a gateway group with the specified details.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGatewayGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateGatewayGroupRequest#builder()}
*
*
* @param createGatewayGroupRequest
* A {@link Consumer} that will call methods on {@link CreateGatewayGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateGatewayGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateGatewayGroup
* @see AWS API Documentation
*/
default CompletableFuture createGatewayGroup(
Consumer createGatewayGroupRequest) {
return createGatewayGroup(CreateGatewayGroupRequest.builder().applyMutation(createGatewayGroupRequest).build());
}
/**
*
* Creates a network profile with the specified details.
*
*
* @param createNetworkProfileRequest
* @return A Java Future containing the result of the CreateNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - InvalidCertificateAuthorityException The Certificate Authority can't issue or revoke a certificate.
* - InvalidServiceLinkedRoleStateException The service linked role is locked for deletion.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture createNetworkProfile(
CreateNetworkProfileRequest createNetworkProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a network profile with the specified details.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNetworkProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateNetworkProfileRequest#builder()}
*
*
* @param createNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link CreateNetworkProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - InvalidCertificateAuthorityException The Certificate Authority can't issue or revoke a certificate.
* - InvalidServiceLinkedRoleStateException The service linked role is locked for deletion.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture createNetworkProfile(
Consumer createNetworkProfileRequest) {
return createNetworkProfile(CreateNetworkProfileRequest.builder().applyMutation(createNetworkProfileRequest).build());
}
/**
*
* Creates a new room profile with the specified details.
*
*
* @param createProfileRequest
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - AlreadyExistsException The resource being created already exists.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateProfile
* @see AWS API Documentation
*/
default CompletableFuture createProfile(CreateProfileRequest createProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new room profile with the specified details.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProfileRequest.Builder} avoiding the need to
* create one manually via {@link CreateProfileRequest#builder()}
*
*
* @param createProfileRequest
* A {@link Consumer} that will call methods on {@link CreateProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - AlreadyExistsException The resource being created already exists.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateProfile
* @see AWS API Documentation
*/
default CompletableFuture createProfile(Consumer createProfileRequest) {
return createProfile(CreateProfileRequest.builder().applyMutation(createProfileRequest).build());
}
/**
*
* Creates a room with the specified details.
*
*
* @param createRoomRequest
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateRoom
* @see AWS
* API Documentation
*/
default CompletableFuture createRoom(CreateRoomRequest createRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a room with the specified details.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRoomRequest.Builder} avoiding the need to
* create one manually via {@link CreateRoomRequest#builder()}
*
*
* @param createRoomRequest
* A {@link Consumer} that will call methods on {@link CreateRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateRoom
* @see AWS
* API Documentation
*/
default CompletableFuture createRoom(Consumer createRoomRequest) {
return createRoom(CreateRoomRequest.builder().applyMutation(createRoomRequest).build());
}
/**
*
* Creates a skill group with a specified name and description.
*
*
* @param createSkillGroupRequest
* @return A Java Future containing the result of the CreateSkillGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture createSkillGroup(CreateSkillGroupRequest createSkillGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a skill group with a specified name and description.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSkillGroupRequest.Builder} avoiding the need
* to create one manually via {@link CreateSkillGroupRequest#builder()}
*
*
* @param createSkillGroupRequest
* A {@link Consumer} that will call methods on {@link CreateSkillGroupRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateSkillGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AlreadyExistsException The resource being created already exists.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture createSkillGroup(
Consumer createSkillGroupRequest) {
return createSkillGroup(CreateSkillGroupRequest.builder().applyMutation(createSkillGroupRequest).build());
}
/**
*
* Creates a user.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The resource in the request is already in use.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateUser
* @see AWS
* API Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The resource in the request is already in use.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.CreateUser
* @see AWS
* API Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deletes an address book by the address book ARN.
*
*
* @param deleteAddressBookRequest
* @return A Java Future containing the result of the DeleteAddressBook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteAddressBook
* @see AWS API Documentation
*/
default CompletableFuture deleteAddressBook(DeleteAddressBookRequest deleteAddressBookRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an address book by the address book ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAddressBookRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAddressBookRequest#builder()}
*
*
* @param deleteAddressBookRequest
* A {@link Consumer} that will call methods on {@link DeleteAddressBookRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteAddressBook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteAddressBook
* @see AWS API Documentation
*/
default CompletableFuture deleteAddressBook(
Consumer deleteAddressBookRequest) {
return deleteAddressBook(DeleteAddressBookRequest.builder().applyMutation(deleteAddressBookRequest).build());
}
/**
*
* Deletes the recurring report delivery schedule with the specified schedule ARN.
*
*
* @param deleteBusinessReportScheduleRequest
* @return A Java Future containing the result of the DeleteBusinessReportSchedule operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteBusinessReportSchedule
* @see AWS API Documentation
*/
default CompletableFuture deleteBusinessReportSchedule(
DeleteBusinessReportScheduleRequest deleteBusinessReportScheduleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the recurring report delivery schedule with the specified schedule ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBusinessReportScheduleRequest.Builder}
* avoiding the need to create one manually via {@link DeleteBusinessReportScheduleRequest#builder()}
*
*
* @param deleteBusinessReportScheduleRequest
* A {@link Consumer} that will call methods on {@link DeleteBusinessReportScheduleRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteBusinessReportSchedule operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteBusinessReportSchedule
* @see AWS API Documentation
*/
default CompletableFuture deleteBusinessReportSchedule(
Consumer deleteBusinessReportScheduleRequest) {
return deleteBusinessReportSchedule(DeleteBusinessReportScheduleRequest.builder()
.applyMutation(deleteBusinessReportScheduleRequest).build());
}
/**
*
* Deletes a conference provider.
*
*
* @param deleteConferenceProviderRequest
* @return A Java Future containing the result of the DeleteConferenceProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteConferenceProvider
* @see AWS API Documentation
*/
default CompletableFuture deleteConferenceProvider(
DeleteConferenceProviderRequest deleteConferenceProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a conference provider.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteConferenceProviderRequest.Builder} avoiding
* the need to create one manually via {@link DeleteConferenceProviderRequest#builder()}
*
*
* @param deleteConferenceProviderRequest
* A {@link Consumer} that will call methods on {@link DeleteConferenceProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteConferenceProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteConferenceProvider
* @see AWS API Documentation
*/
default CompletableFuture deleteConferenceProvider(
Consumer deleteConferenceProviderRequest) {
return deleteConferenceProvider(DeleteConferenceProviderRequest.builder().applyMutation(deleteConferenceProviderRequest)
.build());
}
/**
*
* Deletes a contact by the contact ARN.
*
*
* @param deleteContactRequest
* @return A Java Future containing the result of the DeleteContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteContact
* @see AWS API Documentation
*/
default CompletableFuture deleteContact(DeleteContactRequest deleteContactRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a contact by the contact ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteContactRequest.Builder} avoiding the need to
* create one manually via {@link DeleteContactRequest#builder()}
*
*
* @param deleteContactRequest
* A {@link Consumer} that will call methods on {@link DeleteContactRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteContact
* @see AWS API Documentation
*/
default CompletableFuture deleteContact(Consumer deleteContactRequest) {
return deleteContact(DeleteContactRequest.builder().applyMutation(deleteContactRequest).build());
}
/**
*
* Removes a device from Alexa For Business.
*
*
* @param deleteDeviceRequest
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - InvalidCertificateAuthorityException The Certificate Authority can't issue or revoke a certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteDevice
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDevice(DeleteDeviceRequest deleteDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes a device from Alexa For Business.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDeviceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDeviceRequest#builder()}
*
*
* @param deleteDeviceRequest
* A {@link Consumer} that will call methods on {@link DeleteDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - InvalidCertificateAuthorityException The Certificate Authority can't issue or revoke a certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteDevice
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDevice(Consumer deleteDeviceRequest) {
return deleteDevice(DeleteDeviceRequest.builder().applyMutation(deleteDeviceRequest).build());
}
/**
*
* When this action is called for a specified shared device, it allows authorized users to delete the device's
* entire previous history of voice input data and associated response data. This action can be called once every 24
* hours for a specific shared device.
*
*
* @param deleteDeviceUsageDataRequest
* @return A Java Future containing the result of the DeleteDeviceUsageData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteDeviceUsageData
* @see AWS API Documentation
*/
default CompletableFuture deleteDeviceUsageData(
DeleteDeviceUsageDataRequest deleteDeviceUsageDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* When this action is called for a specified shared device, it allows authorized users to delete the device's
* entire previous history of voice input data and associated response data. This action can be called once every 24
* hours for a specific shared device.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDeviceUsageDataRequest.Builder} avoiding the
* need to create one manually via {@link DeleteDeviceUsageDataRequest#builder()}
*
*
* @param deleteDeviceUsageDataRequest
* A {@link Consumer} that will call methods on {@link DeleteDeviceUsageDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDeviceUsageData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteDeviceUsageData
* @see AWS API Documentation
*/
default CompletableFuture deleteDeviceUsageData(
Consumer deleteDeviceUsageDataRequest) {
return deleteDeviceUsageData(DeleteDeviceUsageDataRequest.builder().applyMutation(deleteDeviceUsageDataRequest).build());
}
/**
*
* Deletes a gateway group.
*
*
* @param deleteGatewayGroupRequest
* @return A Java Future containing the result of the DeleteGatewayGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAssociatedException Another resource is associated with the resource in the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteGatewayGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteGatewayGroup(DeleteGatewayGroupRequest deleteGatewayGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a gateway group.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGatewayGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteGatewayGroupRequest#builder()}
*
*
* @param deleteGatewayGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteGatewayGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteGatewayGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAssociatedException Another resource is associated with the resource in the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteGatewayGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteGatewayGroup(
Consumer deleteGatewayGroupRequest) {
return deleteGatewayGroup(DeleteGatewayGroupRequest.builder().applyMutation(deleteGatewayGroupRequest).build());
}
/**
*
* Deletes a network profile by the network profile ARN.
*
*
* @param deleteNetworkProfileRequest
* @return A Java Future containing the result of the DeleteNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The resource in the request is already in use.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture deleteNetworkProfile(
DeleteNetworkProfileRequest deleteNetworkProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a network profile by the network profile ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNetworkProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteNetworkProfileRequest#builder()}
*
*
* @param deleteNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteNetworkProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The resource in the request is already in use.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture deleteNetworkProfile(
Consumer deleteNetworkProfileRequest) {
return deleteNetworkProfile(DeleteNetworkProfileRequest.builder().applyMutation(deleteNetworkProfileRequest).build());
}
/**
*
* Deletes a room profile by the profile ARN.
*
*
* @param deleteProfileRequest
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteProfile
* @see AWS API Documentation
*/
default CompletableFuture deleteProfile(DeleteProfileRequest deleteProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a room profile by the profile ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProfileRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProfileRequest#builder()}
*
*
* @param deleteProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteProfile
* @see AWS API Documentation
*/
default CompletableFuture deleteProfile(Consumer deleteProfileRequest) {
return deleteProfile(DeleteProfileRequest.builder().applyMutation(deleteProfileRequest).build());
}
/**
*
* Deletes a room by the room ARN.
*
*
* @param deleteRoomRequest
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteRoom
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRoom(DeleteRoomRequest deleteRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a room by the room ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRoomRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRoomRequest#builder()}
*
*
* @param deleteRoomRequest
* A {@link Consumer} that will call methods on {@link DeleteRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteRoom
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRoom(Consumer deleteRoomRequest) {
return deleteRoom(DeleteRoomRequest.builder().applyMutation(deleteRoomRequest).build());
}
/**
*
* Deletes room skill parameter details by room, skill, and parameter key ID.
*
*
* @param deleteRoomSkillParameterRequest
* @return A Java Future containing the result of the DeleteRoomSkillParameter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteRoomSkillParameter
* @see AWS API Documentation
*/
default CompletableFuture deleteRoomSkillParameter(
DeleteRoomSkillParameterRequest deleteRoomSkillParameterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes room skill parameter details by room, skill, and parameter key ID.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRoomSkillParameterRequest.Builder} avoiding
* the need to create one manually via {@link DeleteRoomSkillParameterRequest#builder()}
*
*
* @param deleteRoomSkillParameterRequest
* A {@link Consumer} that will call methods on {@link DeleteRoomSkillParameterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteRoomSkillParameter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteRoomSkillParameter
* @see AWS API Documentation
*/
default CompletableFuture deleteRoomSkillParameter(
Consumer deleteRoomSkillParameterRequest) {
return deleteRoomSkillParameter(DeleteRoomSkillParameterRequest.builder().applyMutation(deleteRoomSkillParameterRequest)
.build());
}
/**
*
* Unlinks a third-party account from a skill.
*
*
* @param deleteSkillAuthorizationRequest
* @return A Java Future containing the result of the DeleteSkillAuthorization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteSkillAuthorization
* @see AWS API Documentation
*/
default CompletableFuture deleteSkillAuthorization(
DeleteSkillAuthorizationRequest deleteSkillAuthorizationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Unlinks a third-party account from a skill.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSkillAuthorizationRequest.Builder} avoiding
* the need to create one manually via {@link DeleteSkillAuthorizationRequest#builder()}
*
*
* @param deleteSkillAuthorizationRequest
* A {@link Consumer} that will call methods on {@link DeleteSkillAuthorizationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteSkillAuthorization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteSkillAuthorization
* @see AWS API Documentation
*/
default CompletableFuture deleteSkillAuthorization(
Consumer deleteSkillAuthorizationRequest) {
return deleteSkillAuthorization(DeleteSkillAuthorizationRequest.builder().applyMutation(deleteSkillAuthorizationRequest)
.build());
}
/**
*
* Deletes a skill group by skill group ARN.
*
*
* @param deleteSkillGroupRequest
* @return A Java Future containing the result of the DeleteSkillGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteSkillGroup(DeleteSkillGroupRequest deleteSkillGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a skill group by skill group ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSkillGroupRequest.Builder} avoiding the need
* to create one manually via {@link DeleteSkillGroupRequest#builder()}
*
*
* @param deleteSkillGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteSkillGroupRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteSkillGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteSkillGroup(
Consumer deleteSkillGroupRequest) {
return deleteSkillGroup(DeleteSkillGroupRequest.builder().applyMutation(deleteSkillGroupRequest).build());
}
/**
*
* Deletes a specified user by user ARN and enrollment ARN.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteUser
* @see AWS
* API Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified user by user ARN and enrollment ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DeleteUser
* @see AWS
* API Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Disassociates a contact from a given address book.
*
*
* @param disassociateContactFromAddressBookRequest
* @return A Java Future containing the result of the DisassociateContactFromAddressBook operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateContactFromAddressBook
* @see AWS API Documentation
*/
default CompletableFuture disassociateContactFromAddressBook(
DisassociateContactFromAddressBookRequest disassociateContactFromAddressBookRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a contact from a given address book.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateContactFromAddressBookRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateContactFromAddressBookRequest#builder()}
*
*
* @param disassociateContactFromAddressBookRequest
* A {@link Consumer} that will call methods on {@link DisassociateContactFromAddressBookRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateContactFromAddressBook operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateContactFromAddressBook
* @see AWS API Documentation
*/
default CompletableFuture disassociateContactFromAddressBook(
Consumer disassociateContactFromAddressBookRequest) {
return disassociateContactFromAddressBook(DisassociateContactFromAddressBookRequest.builder()
.applyMutation(disassociateContactFromAddressBookRequest).build());
}
/**
*
* Disassociates a device from its current room. The device continues to be connected to the Wi-Fi network and is
* still registered to the account. The device settings and skills are removed from the room.
*
*
* @param disassociateDeviceFromRoomRequest
* @return A Java Future containing the result of the DisassociateDeviceFromRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateDeviceFromRoom
* @see AWS API Documentation
*/
default CompletableFuture disassociateDeviceFromRoom(
DisassociateDeviceFromRoomRequest disassociateDeviceFromRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a device from its current room. The device continues to be connected to the Wi-Fi network and is
* still registered to the account. The device settings and skills are removed from the room.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateDeviceFromRoomRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateDeviceFromRoomRequest#builder()}
*
*
* @param disassociateDeviceFromRoomRequest
* A {@link Consumer} that will call methods on {@link DisassociateDeviceFromRoomRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateDeviceFromRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - DeviceNotRegisteredException The request failed because this device is no longer registered and
* therefore no longer managed by this account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateDeviceFromRoom
* @see AWS API Documentation
*/
default CompletableFuture disassociateDeviceFromRoom(
Consumer disassociateDeviceFromRoomRequest) {
return disassociateDeviceFromRoom(DisassociateDeviceFromRoomRequest.builder()
.applyMutation(disassociateDeviceFromRoomRequest).build());
}
/**
*
* Disassociates a skill from a skill group.
*
*
* @param disassociateSkillFromSkillGroupRequest
* @return A Java Future containing the result of the DisassociateSkillFromSkillGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateSkillFromSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture disassociateSkillFromSkillGroup(
DisassociateSkillFromSkillGroupRequest disassociateSkillFromSkillGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a skill from a skill group.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateSkillFromSkillGroupRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateSkillFromSkillGroupRequest#builder()}
*
*
* @param disassociateSkillFromSkillGroupRequest
* A {@link Consumer} that will call methods on {@link DisassociateSkillFromSkillGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateSkillFromSkillGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateSkillFromSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture disassociateSkillFromSkillGroup(
Consumer disassociateSkillFromSkillGroupRequest) {
return disassociateSkillFromSkillGroup(DisassociateSkillFromSkillGroupRequest.builder()
.applyMutation(disassociateSkillFromSkillGroupRequest).build());
}
/**
*
* Makes a private skill unavailable for enrolled users and prevents them from enabling it on their devices.
*
*
* @param disassociateSkillFromUsersRequest
* @return A Java Future containing the result of the DisassociateSkillFromUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateSkillFromUsers
* @see AWS API Documentation
*/
default CompletableFuture disassociateSkillFromUsers(
DisassociateSkillFromUsersRequest disassociateSkillFromUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Makes a private skill unavailable for enrolled users and prevents them from enabling it on their devices.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateSkillFromUsersRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateSkillFromUsersRequest#builder()}
*
*
* @param disassociateSkillFromUsersRequest
* A {@link Consumer} that will call methods on {@link DisassociateSkillFromUsersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateSkillFromUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateSkillFromUsers
* @see AWS API Documentation
*/
default CompletableFuture disassociateSkillFromUsers(
Consumer disassociateSkillFromUsersRequest) {
return disassociateSkillFromUsers(DisassociateSkillFromUsersRequest.builder()
.applyMutation(disassociateSkillFromUsersRequest).build());
}
/**
*
* Disassociates a skill group from a specified room. This disables all skills in the skill group on all devices in
* the room.
*
*
* @param disassociateSkillGroupFromRoomRequest
* @return A Java Future containing the result of the DisassociateSkillGroupFromRoom operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateSkillGroupFromRoom
* @see AWS API Documentation
*/
default CompletableFuture disassociateSkillGroupFromRoom(
DisassociateSkillGroupFromRoomRequest disassociateSkillGroupFromRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a skill group from a specified room. This disables all skills in the skill group on all devices in
* the room.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateSkillGroupFromRoomRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateSkillGroupFromRoomRequest#builder()}
*
*
* @param disassociateSkillGroupFromRoomRequest
* A {@link Consumer} that will call methods on {@link DisassociateSkillGroupFromRoomRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateSkillGroupFromRoom operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.DisassociateSkillGroupFromRoom
* @see AWS API Documentation
*/
default CompletableFuture disassociateSkillGroupFromRoom(
Consumer disassociateSkillGroupFromRoomRequest) {
return disassociateSkillGroupFromRoom(DisassociateSkillGroupFromRoomRequest.builder()
.applyMutation(disassociateSkillGroupFromRoomRequest).build());
}
/**
*
* Forgets smart home appliances associated to a room.
*
*
* @param forgetSmartHomeAppliancesRequest
* @return A Java Future containing the result of the ForgetSmartHomeAppliances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ForgetSmartHomeAppliances
* @see AWS API Documentation
*/
default CompletableFuture forgetSmartHomeAppliances(
ForgetSmartHomeAppliancesRequest forgetSmartHomeAppliancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Forgets smart home appliances associated to a room.
*
*
*
* This is a convenience which creates an instance of the {@link ForgetSmartHomeAppliancesRequest.Builder} avoiding
* the need to create one manually via {@link ForgetSmartHomeAppliancesRequest#builder()}
*
*
* @param forgetSmartHomeAppliancesRequest
* A {@link Consumer} that will call methods on {@link ForgetSmartHomeAppliancesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ForgetSmartHomeAppliances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ForgetSmartHomeAppliances
* @see AWS API Documentation
*/
default CompletableFuture forgetSmartHomeAppliances(
Consumer forgetSmartHomeAppliancesRequest) {
return forgetSmartHomeAppliances(ForgetSmartHomeAppliancesRequest.builder()
.applyMutation(forgetSmartHomeAppliancesRequest).build());
}
/**
*
* Gets address the book details by the address book ARN.
*
*
* @param getAddressBookRequest
* @return A Java Future containing the result of the GetAddressBook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetAddressBook
* @see AWS API Documentation
*/
default CompletableFuture getAddressBook(GetAddressBookRequest getAddressBookRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets address the book details by the address book ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetAddressBookRequest.Builder} avoiding the need to
* create one manually via {@link GetAddressBookRequest#builder()}
*
*
* @param getAddressBookRequest
* A {@link Consumer} that will call methods on {@link GetAddressBookRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetAddressBook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetAddressBook
* @see AWS API Documentation
*/
default CompletableFuture getAddressBook(Consumer getAddressBookRequest) {
return getAddressBook(GetAddressBookRequest.builder().applyMutation(getAddressBookRequest).build());
}
/**
*
* Retrieves the existing conference preferences.
*
*
* @param getConferencePreferenceRequest
* @return A Java Future containing the result of the GetConferencePreference operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetConferencePreference
* @see AWS API Documentation
*/
default CompletableFuture getConferencePreference(
GetConferencePreferenceRequest getConferencePreferenceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the existing conference preferences.
*
*
*
* This is a convenience which creates an instance of the {@link GetConferencePreferenceRequest.Builder} avoiding
* the need to create one manually via {@link GetConferencePreferenceRequest#builder()}
*
*
* @param getConferencePreferenceRequest
* A {@link Consumer} that will call methods on {@link GetConferencePreferenceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetConferencePreference operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetConferencePreference
* @see AWS API Documentation
*/
default CompletableFuture getConferencePreference(
Consumer getConferencePreferenceRequest) {
return getConferencePreference(GetConferencePreferenceRequest.builder().applyMutation(getConferencePreferenceRequest)
.build());
}
/**
*
* Gets details about a specific conference provider.
*
*
* @param getConferenceProviderRequest
* @return A Java Future containing the result of the GetConferenceProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetConferenceProvider
* @see AWS API Documentation
*/
default CompletableFuture getConferenceProvider(
GetConferenceProviderRequest getConferenceProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets details about a specific conference provider.
*
*
*
* This is a convenience which creates an instance of the {@link GetConferenceProviderRequest.Builder} avoiding the
* need to create one manually via {@link GetConferenceProviderRequest#builder()}
*
*
* @param getConferenceProviderRequest
* A {@link Consumer} that will call methods on {@link GetConferenceProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetConferenceProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetConferenceProvider
* @see AWS API Documentation
*/
default CompletableFuture getConferenceProvider(
Consumer getConferenceProviderRequest) {
return getConferenceProvider(GetConferenceProviderRequest.builder().applyMutation(getConferenceProviderRequest).build());
}
/**
*
* Gets the contact details by the contact ARN.
*
*
* @param getContactRequest
* @return A Java Future containing the result of the GetContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetContact
* @see AWS
* API Documentation
*/
default CompletableFuture getContact(GetContactRequest getContactRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the contact details by the contact ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetContactRequest.Builder} avoiding the need to
* create one manually via {@link GetContactRequest#builder()}
*
*
* @param getContactRequest
* A {@link Consumer} that will call methods on {@link GetContactRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetContact
* @see AWS
* API Documentation
*/
default CompletableFuture getContact(Consumer getContactRequest) {
return getContact(GetContactRequest.builder().applyMutation(getContactRequest).build());
}
/**
*
* Gets the details of a device by device ARN.
*
*
* @param getDeviceRequest
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetDevice
* @see AWS
* API Documentation
*/
default CompletableFuture getDevice(GetDeviceRequest getDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a device by device ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeviceRequest.Builder} avoiding the need to
* create one manually via {@link GetDeviceRequest#builder()}
*
*
* @param getDeviceRequest
* A {@link Consumer} that will call methods on {@link GetDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetDevice
* @see AWS
* API Documentation
*/
default CompletableFuture getDevice(Consumer getDeviceRequest) {
return getDevice(GetDeviceRequest.builder().applyMutation(getDeviceRequest).build());
}
/**
*
* Retrieves the details of a gateway.
*
*
* @param getGatewayRequest
* @return A Java Future containing the result of the GetGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetGateway
* @see AWS
* API Documentation
*/
default CompletableFuture getGateway(GetGatewayRequest getGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the details of a gateway.
*
*
*
* This is a convenience which creates an instance of the {@link GetGatewayRequest.Builder} avoiding the need to
* create one manually via {@link GetGatewayRequest#builder()}
*
*
* @param getGatewayRequest
* A {@link Consumer} that will call methods on {@link GetGatewayRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetGateway
* @see AWS
* API Documentation
*/
default CompletableFuture getGateway(Consumer getGatewayRequest) {
return getGateway(GetGatewayRequest.builder().applyMutation(getGatewayRequest).build());
}
/**
*
* Retrieves the details of a gateway group.
*
*
* @param getGatewayGroupRequest
* @return A Java Future containing the result of the GetGatewayGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetGatewayGroup
* @see AWS API Documentation
*/
default CompletableFuture getGatewayGroup(GetGatewayGroupRequest getGatewayGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the details of a gateway group.
*
*
*
* This is a convenience which creates an instance of the {@link GetGatewayGroupRequest.Builder} avoiding the need
* to create one manually via {@link GetGatewayGroupRequest#builder()}
*
*
* @param getGatewayGroupRequest
* A {@link Consumer} that will call methods on {@link GetGatewayGroupRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetGatewayGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetGatewayGroup
* @see AWS API Documentation
*/
default CompletableFuture getGatewayGroup(
Consumer getGatewayGroupRequest) {
return getGatewayGroup(GetGatewayGroupRequest.builder().applyMutation(getGatewayGroupRequest).build());
}
/**
*
* Retrieves the configured values for the user enrollment invitation email template.
*
*
* @param getInvitationConfigurationRequest
* @return A Java Future containing the result of the GetInvitationConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetInvitationConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getInvitationConfiguration(
GetInvitationConfigurationRequest getInvitationConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the configured values for the user enrollment invitation email template.
*
*
*
* This is a convenience which creates an instance of the {@link GetInvitationConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link GetInvitationConfigurationRequest#builder()}
*
*
* @param getInvitationConfigurationRequest
* A {@link Consumer} that will call methods on {@link GetInvitationConfigurationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetInvitationConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetInvitationConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getInvitationConfiguration(
Consumer getInvitationConfigurationRequest) {
return getInvitationConfiguration(GetInvitationConfigurationRequest.builder()
.applyMutation(getInvitationConfigurationRequest).build());
}
/**
*
* Gets the network profile details by the network profile ARN.
*
*
* @param getNetworkProfileRequest
* @return A Java Future containing the result of the GetNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - InvalidSecretsManagerResourceException A password in SecretsManager is in an invalid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture getNetworkProfile(GetNetworkProfileRequest getNetworkProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network profile details by the network profile ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkProfileRequest.Builder} avoiding the need
* to create one manually via {@link GetNetworkProfileRequest#builder()}
*
*
* @param getNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link GetNetworkProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - InvalidSecretsManagerResourceException A password in SecretsManager is in an invalid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetNetworkProfile
* @see AWS API Documentation
*/
default CompletableFuture getNetworkProfile(
Consumer getNetworkProfileRequest) {
return getNetworkProfile(GetNetworkProfileRequest.builder().applyMutation(getNetworkProfileRequest).build());
}
/**
*
* Gets the details of a room profile by profile ARN.
*
*
* @param getProfileRequest
* @return A Java Future containing the result of the GetProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetProfile
* @see AWS
* API Documentation
*/
default CompletableFuture getProfile(GetProfileRequest getProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a room profile by profile ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetProfileRequest.Builder} avoiding the need to
* create one manually via {@link GetProfileRequest#builder()}
*
*
* @param getProfileRequest
* A {@link Consumer} that will call methods on {@link GetProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetProfile
* @see AWS
* API Documentation
*/
default CompletableFuture getProfile(Consumer getProfileRequest) {
return getProfile(GetProfileRequest.builder().applyMutation(getProfileRequest).build());
}
/**
*
* Gets room details by room ARN.
*
*
* @param getRoomRequest
* @return A Java Future containing the result of the GetRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetRoom
* @see AWS API
* Documentation
*/
default CompletableFuture getRoom(GetRoomRequest getRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets room details by room ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetRoomRequest.Builder} avoiding the need to create
* one manually via {@link GetRoomRequest#builder()}
*
*
* @param getRoomRequest
* A {@link Consumer} that will call methods on {@link GetRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetRoom
* @see AWS API
* Documentation
*/
default CompletableFuture getRoom(Consumer getRoomRequest) {
return getRoom(GetRoomRequest.builder().applyMutation(getRoomRequest).build());
}
/**
*
* Gets room skill parameter details by room, skill, and parameter key ARN.
*
*
* @param getRoomSkillParameterRequest
* @return A Java Future containing the result of the GetRoomSkillParameter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetRoomSkillParameter
* @see AWS API Documentation
*/
default CompletableFuture getRoomSkillParameter(
GetRoomSkillParameterRequest getRoomSkillParameterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets room skill parameter details by room, skill, and parameter key ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetRoomSkillParameterRequest.Builder} avoiding the
* need to create one manually via {@link GetRoomSkillParameterRequest#builder()}
*
*
* @param getRoomSkillParameterRequest
* A {@link Consumer} that will call methods on {@link GetRoomSkillParameterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetRoomSkillParameter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetRoomSkillParameter
* @see AWS API Documentation
*/
default CompletableFuture getRoomSkillParameter(
Consumer getRoomSkillParameterRequest) {
return getRoomSkillParameter(GetRoomSkillParameterRequest.builder().applyMutation(getRoomSkillParameterRequest).build());
}
/**
*
* Gets skill group details by skill group ARN.
*
*
* @param getSkillGroupRequest
* @return A Java Future containing the result of the GetSkillGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture getSkillGroup(GetSkillGroupRequest getSkillGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets skill group details by skill group ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetSkillGroupRequest.Builder} avoiding the need to
* create one manually via {@link GetSkillGroupRequest#builder()}
*
*
* @param getSkillGroupRequest
* A {@link Consumer} that will call methods on {@link GetSkillGroupRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetSkillGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.GetSkillGroup
* @see AWS API Documentation
*/
default CompletableFuture getSkillGroup(Consumer getSkillGroupRequest) {
return getSkillGroup(GetSkillGroupRequest.builder().applyMutation(getSkillGroupRequest).build());
}
/**
*
* Lists the details of the schedules that a user configured. A download URL of the report associated with each
* schedule is returned every time this action is called. A new download URL is returned each time, and is valid for
* 24 hours.
*
*
* @param listBusinessReportSchedulesRequest
* @return A Java Future containing the result of the ListBusinessReportSchedules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListBusinessReportSchedules
* @see AWS API Documentation
*/
default CompletableFuture listBusinessReportSchedules(
ListBusinessReportSchedulesRequest listBusinessReportSchedulesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the details of the schedules that a user configured. A download URL of the report associated with each
* schedule is returned every time this action is called. A new download URL is returned each time, and is valid for
* 24 hours.
*
*
*
* This is a convenience which creates an instance of the {@link ListBusinessReportSchedulesRequest.Builder}
* avoiding the need to create one manually via {@link ListBusinessReportSchedulesRequest#builder()}
*
*
* @param listBusinessReportSchedulesRequest
* A {@link Consumer} that will call methods on {@link ListBusinessReportSchedulesRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListBusinessReportSchedules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListBusinessReportSchedules
* @see AWS API Documentation
*/
default CompletableFuture listBusinessReportSchedules(
Consumer listBusinessReportSchedulesRequest) {
return listBusinessReportSchedules(ListBusinessReportSchedulesRequest.builder()
.applyMutation(listBusinessReportSchedulesRequest).build());
}
/**
*
* Lists the details of the schedules that a user configured. A download URL of the report associated with each
* schedule is returned every time this action is called. A new download URL is returned each time, and is valid for
* 24 hours.
*
*
*
* This is a variant of
* {@link #listBusinessReportSchedules(software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListBusinessReportSchedulesPublisher publisher = client.listBusinessReportSchedulesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListBusinessReportSchedulesPublisher publisher = client.listBusinessReportSchedulesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBusinessReportSchedules(software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesRequest)}
* operation.
*
*
* @param listBusinessReportSchedulesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListBusinessReportSchedules
* @see AWS API Documentation
*/
default ListBusinessReportSchedulesPublisher listBusinessReportSchedulesPaginator(
ListBusinessReportSchedulesRequest listBusinessReportSchedulesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the details of the schedules that a user configured. A download URL of the report associated with each
* schedule is returned every time this action is called. A new download URL is returned each time, and is valid for
* 24 hours.
*
*
*
* This is a variant of
* {@link #listBusinessReportSchedules(software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListBusinessReportSchedulesPublisher publisher = client.listBusinessReportSchedulesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListBusinessReportSchedulesPublisher publisher = client.listBusinessReportSchedulesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBusinessReportSchedules(software.amazon.awssdk.services.alexaforbusiness.model.ListBusinessReportSchedulesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListBusinessReportSchedulesRequest.Builder}
* avoiding the need to create one manually via {@link ListBusinessReportSchedulesRequest#builder()}
*
*
* @param listBusinessReportSchedulesRequest
* A {@link Consumer} that will call methods on {@link ListBusinessReportSchedulesRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListBusinessReportSchedules
* @see AWS API Documentation
*/
default ListBusinessReportSchedulesPublisher listBusinessReportSchedulesPaginator(
Consumer listBusinessReportSchedulesRequest) {
return listBusinessReportSchedulesPaginator(ListBusinessReportSchedulesRequest.builder()
.applyMutation(listBusinessReportSchedulesRequest).build());
}
/**
*
* Lists conference providers under a specific AWS account.
*
*
* @param listConferenceProvidersRequest
* @return A Java Future containing the result of the ListConferenceProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListConferenceProviders
* @see AWS API Documentation
*/
default CompletableFuture listConferenceProviders(
ListConferenceProvidersRequest listConferenceProvidersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists conference providers under a specific AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListConferenceProvidersRequest.Builder} avoiding
* the need to create one manually via {@link ListConferenceProvidersRequest#builder()}
*
*
* @param listConferenceProvidersRequest
* A {@link Consumer} that will call methods on {@link ListConferenceProvidersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListConferenceProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListConferenceProviders
* @see AWS API Documentation
*/
default CompletableFuture listConferenceProviders(
Consumer listConferenceProvidersRequest) {
return listConferenceProviders(ListConferenceProvidersRequest.builder().applyMutation(listConferenceProvidersRequest)
.build());
}
/**
*
* Lists conference providers under a specific AWS account.
*
*
*
* This is a variant of
* {@link #listConferenceProviders(software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListConferenceProvidersPublisher publisher = client.listConferenceProvidersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListConferenceProvidersPublisher publisher = client.listConferenceProvidersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConferenceProviders(software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersRequest)}
* operation.
*
*
* @param listConferenceProvidersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListConferenceProviders
* @see AWS API Documentation
*/
default ListConferenceProvidersPublisher listConferenceProvidersPaginator(
ListConferenceProvidersRequest listConferenceProvidersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists conference providers under a specific AWS account.
*
*
*
* This is a variant of
* {@link #listConferenceProviders(software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListConferenceProvidersPublisher publisher = client.listConferenceProvidersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListConferenceProvidersPublisher publisher = client.listConferenceProvidersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConferenceProviders(software.amazon.awssdk.services.alexaforbusiness.model.ListConferenceProvidersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListConferenceProvidersRequest.Builder} avoiding
* the need to create one manually via {@link ListConferenceProvidersRequest#builder()}
*
*
* @param listConferenceProvidersRequest
* A {@link Consumer} that will call methods on {@link ListConferenceProvidersRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListConferenceProviders
* @see AWS API Documentation
*/
default ListConferenceProvidersPublisher listConferenceProvidersPaginator(
Consumer listConferenceProvidersRequest) {
return listConferenceProvidersPaginator(ListConferenceProvidersRequest.builder()
.applyMutation(listConferenceProvidersRequest).build());
}
/**
*
* Lists the device event history, including device connection status, for up to 30 days.
*
*
* @param listDeviceEventsRequest
* @return A Java Future containing the result of the ListDeviceEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListDeviceEvents
* @see AWS API Documentation
*/
default CompletableFuture listDeviceEvents(ListDeviceEventsRequest listDeviceEventsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the device event history, including device connection status, for up to 30 days.
*
*
*
* This is a convenience which creates an instance of the {@link ListDeviceEventsRequest.Builder} avoiding the need
* to create one manually via {@link ListDeviceEventsRequest#builder()}
*
*
* @param listDeviceEventsRequest
* A {@link Consumer} that will call methods on {@link ListDeviceEventsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListDeviceEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListDeviceEvents
* @see AWS API Documentation
*/
default CompletableFuture listDeviceEvents(
Consumer listDeviceEventsRequest) {
return listDeviceEvents(ListDeviceEventsRequest.builder().applyMutation(listDeviceEventsRequest).build());
}
/**
*
* Lists the device event history, including device connection status, for up to 30 days.
*
*
*
* This is a variant of
* {@link #listDeviceEvents(software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListDeviceEventsPublisher publisher = client.listDeviceEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListDeviceEventsPublisher publisher = client.listDeviceEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDeviceEvents(software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsRequest)}
* operation.
*
*
* @param listDeviceEventsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListDeviceEvents
* @see AWS API Documentation
*/
default ListDeviceEventsPublisher listDeviceEventsPaginator(ListDeviceEventsRequest listDeviceEventsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the device event history, including device connection status, for up to 30 days.
*
*
*
* This is a variant of
* {@link #listDeviceEvents(software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListDeviceEventsPublisher publisher = client.listDeviceEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListDeviceEventsPublisher publisher = client.listDeviceEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDeviceEvents(software.amazon.awssdk.services.alexaforbusiness.model.ListDeviceEventsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDeviceEventsRequest.Builder} avoiding the need
* to create one manually via {@link ListDeviceEventsRequest#builder()}
*
*
* @param listDeviceEventsRequest
* A {@link Consumer} that will call methods on {@link ListDeviceEventsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListDeviceEvents
* @see AWS API Documentation
*/
default ListDeviceEventsPublisher listDeviceEventsPaginator(Consumer listDeviceEventsRequest) {
return listDeviceEventsPaginator(ListDeviceEventsRequest.builder().applyMutation(listDeviceEventsRequest).build());
}
/**
*
* Retrieves a list of gateway group summaries. Use GetGatewayGroup to retrieve details of a specific gateway group.
*
*
* @param listGatewayGroupsRequest
* @return A Java Future containing the result of the ListGatewayGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGatewayGroups
* @see AWS API Documentation
*/
default CompletableFuture listGatewayGroups(ListGatewayGroupsRequest listGatewayGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of gateway group summaries. Use GetGatewayGroup to retrieve details of a specific gateway group.
*
*
*
* This is a convenience which creates an instance of the {@link ListGatewayGroupsRequest.Builder} avoiding the need
* to create one manually via {@link ListGatewayGroupsRequest#builder()}
*
*
* @param listGatewayGroupsRequest
* A {@link Consumer} that will call methods on {@link ListGatewayGroupsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListGatewayGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGatewayGroups
* @see AWS API Documentation
*/
default CompletableFuture listGatewayGroups(
Consumer listGatewayGroupsRequest) {
return listGatewayGroups(ListGatewayGroupsRequest.builder().applyMutation(listGatewayGroupsRequest).build());
}
/**
*
* Retrieves a list of gateway group summaries. Use GetGatewayGroup to retrieve details of a specific gateway group.
*
*
*
* This is a variant of
* {@link #listGatewayGroups(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewayGroupsPublisher publisher = client.listGatewayGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewayGroupsPublisher publisher = client.listGatewayGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGatewayGroups(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsRequest)}
* operation.
*
*
* @param listGatewayGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGatewayGroups
* @see AWS API Documentation
*/
default ListGatewayGroupsPublisher listGatewayGroupsPaginator(ListGatewayGroupsRequest listGatewayGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of gateway group summaries. Use GetGatewayGroup to retrieve details of a specific gateway group.
*
*
*
* This is a variant of
* {@link #listGatewayGroups(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewayGroupsPublisher publisher = client.listGatewayGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewayGroupsPublisher publisher = client.listGatewayGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGatewayGroups(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewayGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListGatewayGroupsRequest.Builder} avoiding the need
* to create one manually via {@link ListGatewayGroupsRequest#builder()}
*
*
* @param listGatewayGroupsRequest
* A {@link Consumer} that will call methods on {@link ListGatewayGroupsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGatewayGroups
* @see AWS API Documentation
*/
default ListGatewayGroupsPublisher listGatewayGroupsPaginator(
Consumer listGatewayGroupsRequest) {
return listGatewayGroupsPaginator(ListGatewayGroupsRequest.builder().applyMutation(listGatewayGroupsRequest).build());
}
/**
*
* Retrieves a list of gateway summaries. Use GetGateway to retrieve details of a specific gateway. An optional
* gateway group ARN can be provided to only retrieve gateway summaries of gateways that are associated with that
* gateway group ARN.
*
*
* @param listGatewaysRequest
* @return A Java Future containing the result of the ListGateways operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGateways
* @see AWS
* API Documentation
*/
default CompletableFuture listGateways(ListGatewaysRequest listGatewaysRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of gateway summaries. Use GetGateway to retrieve details of a specific gateway. An optional
* gateway group ARN can be provided to only retrieve gateway summaries of gateways that are associated with that
* gateway group ARN.
*
*
*
* This is a convenience which creates an instance of the {@link ListGatewaysRequest.Builder} avoiding the need to
* create one manually via {@link ListGatewaysRequest#builder()}
*
*
* @param listGatewaysRequest
* A {@link Consumer} that will call methods on {@link ListGatewaysRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListGateways operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGateways
* @see AWS
* API Documentation
*/
default CompletableFuture listGateways(Consumer listGatewaysRequest) {
return listGateways(ListGatewaysRequest.builder().applyMutation(listGatewaysRequest).build());
}
/**
*
* Retrieves a list of gateway summaries. Use GetGateway to retrieve details of a specific gateway. An optional
* gateway group ARN can be provided to only retrieve gateway summaries of gateways that are associated with that
* gateway group ARN.
*
*
*
* This is a variant of
* {@link #listGateways(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewaysPublisher publisher = client.listGatewaysPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewaysPublisher publisher = client.listGatewaysPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGateways(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysRequest)} operation.
*
*
* @param listGatewaysRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGateways
* @see AWS
* API Documentation
*/
default ListGatewaysPublisher listGatewaysPaginator(ListGatewaysRequest listGatewaysRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of gateway summaries. Use GetGateway to retrieve details of a specific gateway. An optional
* gateway group ARN can be provided to only retrieve gateway summaries of gateways that are associated with that
* gateway group ARN.
*
*
*
* This is a variant of
* {@link #listGateways(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewaysPublisher publisher = client.listGatewaysPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListGatewaysPublisher publisher = client.listGatewaysPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGateways(software.amazon.awssdk.services.alexaforbusiness.model.ListGatewaysRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListGatewaysRequest.Builder} avoiding the need to
* create one manually via {@link ListGatewaysRequest#builder()}
*
*
* @param listGatewaysRequest
* A {@link Consumer} that will call methods on {@link ListGatewaysRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListGateways
* @see AWS
* API Documentation
*/
default ListGatewaysPublisher listGatewaysPaginator(Consumer listGatewaysRequest) {
return listGatewaysPaginator(ListGatewaysRequest.builder().applyMutation(listGatewaysRequest).build());
}
/**
*
* Lists all enabled skills in a specific skill group.
*
*
* @param listSkillsRequest
* @return A Java Future containing the result of the ListSkills operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkills
* @see AWS
* API Documentation
*/
default CompletableFuture listSkills(ListSkillsRequest listSkillsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all enabled skills in a specific skill group.
*
*
*
* This is a convenience which creates an instance of the {@link ListSkillsRequest.Builder} avoiding the need to
* create one manually via {@link ListSkillsRequest#builder()}
*
*
* @param listSkillsRequest
* A {@link Consumer} that will call methods on {@link ListSkillsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListSkills operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkills
* @see AWS
* API Documentation
*/
default CompletableFuture listSkills(Consumer listSkillsRequest) {
return listSkills(ListSkillsRequest.builder().applyMutation(listSkillsRequest).build());
}
/**
*
* Lists all enabled skills in a specific skill group.
*
*
*
* This is a variant of
* {@link #listSkills(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsPublisher publisher = client.listSkillsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsPublisher publisher = client.listSkillsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSkills(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsRequest)} operation.
*
*
* @param listSkillsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkills
* @see AWS
* API Documentation
*/
default ListSkillsPublisher listSkillsPaginator(ListSkillsRequest listSkillsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all enabled skills in a specific skill group.
*
*
*
* This is a variant of
* {@link #listSkills(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsPublisher publisher = client.listSkillsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsPublisher publisher = client.listSkillsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSkills(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSkillsRequest.Builder} avoiding the need to
* create one manually via {@link ListSkillsRequest#builder()}
*
*
* @param listSkillsRequest
* A {@link Consumer} that will call methods on {@link ListSkillsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkills
* @see AWS
* API Documentation
*/
default ListSkillsPublisher listSkillsPaginator(Consumer listSkillsRequest) {
return listSkillsPaginator(ListSkillsRequest.builder().applyMutation(listSkillsRequest).build());
}
/**
*
* Lists all categories in the Alexa skill store.
*
*
* @param listSkillsStoreCategoriesRequest
* @return A Java Future containing the result of the ListSkillsStoreCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreCategories
* @see AWS API Documentation
*/
default CompletableFuture listSkillsStoreCategories(
ListSkillsStoreCategoriesRequest listSkillsStoreCategoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all categories in the Alexa skill store.
*
*
*
* This is a convenience which creates an instance of the {@link ListSkillsStoreCategoriesRequest.Builder} avoiding
* the need to create one manually via {@link ListSkillsStoreCategoriesRequest#builder()}
*
*
* @param listSkillsStoreCategoriesRequest
* A {@link Consumer} that will call methods on {@link ListSkillsStoreCategoriesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListSkillsStoreCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreCategories
* @see AWS API Documentation
*/
default CompletableFuture listSkillsStoreCategories(
Consumer listSkillsStoreCategoriesRequest) {
return listSkillsStoreCategories(ListSkillsStoreCategoriesRequest.builder()
.applyMutation(listSkillsStoreCategoriesRequest).build());
}
/**
*
* Lists all categories in the Alexa skill store.
*
*
*
* This is a variant of
* {@link #listSkillsStoreCategories(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreCategoriesPublisher publisher = client.listSkillsStoreCategoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreCategoriesPublisher publisher = client.listSkillsStoreCategoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSkillsStoreCategories(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesRequest)}
* operation.
*
*
* @param listSkillsStoreCategoriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreCategories
* @see AWS API Documentation
*/
default ListSkillsStoreCategoriesPublisher listSkillsStoreCategoriesPaginator(
ListSkillsStoreCategoriesRequest listSkillsStoreCategoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all categories in the Alexa skill store.
*
*
*
* This is a variant of
* {@link #listSkillsStoreCategories(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreCategoriesPublisher publisher = client.listSkillsStoreCategoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreCategoriesPublisher publisher = client.listSkillsStoreCategoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSkillsStoreCategories(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreCategoriesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListSkillsStoreCategoriesRequest.Builder} avoiding
* the need to create one manually via {@link ListSkillsStoreCategoriesRequest#builder()}
*
*
* @param listSkillsStoreCategoriesRequest
* A {@link Consumer} that will call methods on {@link ListSkillsStoreCategoriesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreCategories
* @see AWS API Documentation
*/
default ListSkillsStoreCategoriesPublisher listSkillsStoreCategoriesPaginator(
Consumer listSkillsStoreCategoriesRequest) {
return listSkillsStoreCategoriesPaginator(ListSkillsStoreCategoriesRequest.builder()
.applyMutation(listSkillsStoreCategoriesRequest).build());
}
/**
*
* Lists all skills in the Alexa skill store by category.
*
*
* @param listSkillsStoreSkillsByCategoryRequest
* @return A Java Future containing the result of the ListSkillsStoreSkillsByCategory operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreSkillsByCategory
* @see AWS API Documentation
*/
default CompletableFuture listSkillsStoreSkillsByCategory(
ListSkillsStoreSkillsByCategoryRequest listSkillsStoreSkillsByCategoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all skills in the Alexa skill store by category.
*
*
*
* This is a convenience which creates an instance of the {@link ListSkillsStoreSkillsByCategoryRequest.Builder}
* avoiding the need to create one manually via {@link ListSkillsStoreSkillsByCategoryRequest#builder()}
*
*
* @param listSkillsStoreSkillsByCategoryRequest
* A {@link Consumer} that will call methods on {@link ListSkillsStoreSkillsByCategoryRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListSkillsStoreSkillsByCategory operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreSkillsByCategory
* @see AWS API Documentation
*/
default CompletableFuture listSkillsStoreSkillsByCategory(
Consumer listSkillsStoreSkillsByCategoryRequest) {
return listSkillsStoreSkillsByCategory(ListSkillsStoreSkillsByCategoryRequest.builder()
.applyMutation(listSkillsStoreSkillsByCategoryRequest).build());
}
/**
*
* Lists all skills in the Alexa skill store by category.
*
*
*
* This is a variant of
* {@link #listSkillsStoreSkillsByCategory(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreSkillsByCategoryPublisher publisher = client.listSkillsStoreSkillsByCategoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreSkillsByCategoryPublisher publisher = client.listSkillsStoreSkillsByCategoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSkillsStoreSkillsByCategory(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryRequest)}
* operation.
*
*
* @param listSkillsStoreSkillsByCategoryRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreSkillsByCategory
* @see AWS API Documentation
*/
default ListSkillsStoreSkillsByCategoryPublisher listSkillsStoreSkillsByCategoryPaginator(
ListSkillsStoreSkillsByCategoryRequest listSkillsStoreSkillsByCategoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all skills in the Alexa skill store by category.
*
*
*
* This is a variant of
* {@link #listSkillsStoreSkillsByCategory(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreSkillsByCategoryPublisher publisher = client.listSkillsStoreSkillsByCategoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSkillsStoreSkillsByCategoryPublisher publisher = client.listSkillsStoreSkillsByCategoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSkillsStoreSkillsByCategory(software.amazon.awssdk.services.alexaforbusiness.model.ListSkillsStoreSkillsByCategoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListSkillsStoreSkillsByCategoryRequest.Builder}
* avoiding the need to create one manually via {@link ListSkillsStoreSkillsByCategoryRequest#builder()}
*
*
* @param listSkillsStoreSkillsByCategoryRequest
* A {@link Consumer} that will call methods on {@link ListSkillsStoreSkillsByCategoryRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSkillsStoreSkillsByCategory
* @see AWS API Documentation
*/
default ListSkillsStoreSkillsByCategoryPublisher listSkillsStoreSkillsByCategoryPaginator(
Consumer listSkillsStoreSkillsByCategoryRequest) {
return listSkillsStoreSkillsByCategoryPaginator(ListSkillsStoreSkillsByCategoryRequest.builder()
.applyMutation(listSkillsStoreSkillsByCategoryRequest).build());
}
/**
*
* Lists all of the smart home appliances associated with a room.
*
*
* @param listSmartHomeAppliancesRequest
* @return A Java Future containing the result of the ListSmartHomeAppliances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSmartHomeAppliances
* @see AWS API Documentation
*/
default CompletableFuture listSmartHomeAppliances(
ListSmartHomeAppliancesRequest listSmartHomeAppliancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the smart home appliances associated with a room.
*
*
*
* This is a convenience which creates an instance of the {@link ListSmartHomeAppliancesRequest.Builder} avoiding
* the need to create one manually via {@link ListSmartHomeAppliancesRequest#builder()}
*
*
* @param listSmartHomeAppliancesRequest
* A {@link Consumer} that will call methods on {@link ListSmartHomeAppliancesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListSmartHomeAppliances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSmartHomeAppliances
* @see AWS API Documentation
*/
default CompletableFuture listSmartHomeAppliances(
Consumer listSmartHomeAppliancesRequest) {
return listSmartHomeAppliances(ListSmartHomeAppliancesRequest.builder().applyMutation(listSmartHomeAppliancesRequest)
.build());
}
/**
*
* Lists all of the smart home appliances associated with a room.
*
*
*
* This is a variant of
* {@link #listSmartHomeAppliances(software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSmartHomeAppliancesPublisher publisher = client.listSmartHomeAppliancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSmartHomeAppliancesPublisher publisher = client.listSmartHomeAppliancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSmartHomeAppliances(software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesRequest)}
* operation.
*
*
* @param listSmartHomeAppliancesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSmartHomeAppliances
* @see AWS API Documentation
*/
default ListSmartHomeAppliancesPublisher listSmartHomeAppliancesPaginator(
ListSmartHomeAppliancesRequest listSmartHomeAppliancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the smart home appliances associated with a room.
*
*
*
* This is a variant of
* {@link #listSmartHomeAppliances(software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSmartHomeAppliancesPublisher publisher = client.listSmartHomeAppliancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListSmartHomeAppliancesPublisher publisher = client.listSmartHomeAppliancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSmartHomeAppliances(software.amazon.awssdk.services.alexaforbusiness.model.ListSmartHomeAppliancesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListSmartHomeAppliancesRequest.Builder} avoiding
* the need to create one manually via {@link ListSmartHomeAppliancesRequest#builder()}
*
*
* @param listSmartHomeAppliancesRequest
* A {@link Consumer} that will call methods on {@link ListSmartHomeAppliancesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListSmartHomeAppliances
* @see AWS API Documentation
*/
default ListSmartHomeAppliancesPublisher listSmartHomeAppliancesPaginator(
Consumer listSmartHomeAppliancesRequest) {
return listSmartHomeAppliancesPaginator(ListSmartHomeAppliancesRequest.builder()
.applyMutation(listSmartHomeAppliancesRequest).build());
}
/**
*
* Lists all tags for the specified resource.
*
*
* @param listTagsRequest
* @return A Java Future containing the result of the ListTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListTags
* @see AWS API
* Documentation
*/
default CompletableFuture listTags(ListTagsRequest listTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags for the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListTagsRequest#builder()}
*
*
* @param listTagsRequest
* A {@link Consumer} that will call methods on {@link ListTagsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListTags
* @see AWS API
* Documentation
*/
default CompletableFuture listTags(Consumer listTagsRequest) {
return listTags(ListTagsRequest.builder().applyMutation(listTagsRequest).build());
}
/**
*
* Lists all tags for the specified resource.
*
*
*
* This is a variant of {@link #listTags(software.amazon.awssdk.services.alexaforbusiness.model.ListTagsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTags(software.amazon.awssdk.services.alexaforbusiness.model.ListTagsRequest)} operation.
*
*
* @param listTagsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListTags
* @see AWS API
* Documentation
*/
default ListTagsPublisher listTagsPaginator(ListTagsRequest listTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags for the specified resource.
*
*
*
* This is a variant of {@link #listTags(software.amazon.awssdk.services.alexaforbusiness.model.ListTagsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.ListTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTags(software.amazon.awssdk.services.alexaforbusiness.model.ListTagsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListTagsRequest#builder()}
*
*
* @param listTagsRequest
* A {@link Consumer} that will call methods on {@link ListTagsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ListTags
* @see AWS API
* Documentation
*/
default ListTagsPublisher listTagsPaginator(Consumer listTagsRequest) {
return listTagsPaginator(ListTagsRequest.builder().applyMutation(listTagsRequest).build());
}
/**
*
* Sets the conference preferences on a specific conference provider at the account level.
*
*
* @param putConferencePreferenceRequest
* @return A Java Future containing the result of the PutConferencePreference operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutConferencePreference
* @see AWS API Documentation
*/
default CompletableFuture putConferencePreference(
PutConferencePreferenceRequest putConferencePreferenceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets the conference preferences on a specific conference provider at the account level.
*
*
*
* This is a convenience which creates an instance of the {@link PutConferencePreferenceRequest.Builder} avoiding
* the need to create one manually via {@link PutConferencePreferenceRequest#builder()}
*
*
* @param putConferencePreferenceRequest
* A {@link Consumer} that will call methods on {@link PutConferencePreferenceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutConferencePreference operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutConferencePreference
* @see AWS API Documentation
*/
default CompletableFuture putConferencePreference(
Consumer putConferencePreferenceRequest) {
return putConferencePreference(PutConferencePreferenceRequest.builder().applyMutation(putConferencePreferenceRequest)
.build());
}
/**
*
* Configures the email template for the user enrollment invitation with the specified attributes.
*
*
* @param putInvitationConfigurationRequest
* @return A Java Future containing the result of the PutInvitationConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutInvitationConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putInvitationConfiguration(
PutInvitationConfigurationRequest putInvitationConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Configures the email template for the user enrollment invitation with the specified attributes.
*
*
*
* This is a convenience which creates an instance of the {@link PutInvitationConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link PutInvitationConfigurationRequest#builder()}
*
*
* @param putInvitationConfigurationRequest
* A {@link Consumer} that will call methods on {@link PutInvitationConfigurationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutInvitationConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutInvitationConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putInvitationConfiguration(
Consumer putInvitationConfigurationRequest) {
return putInvitationConfiguration(PutInvitationConfigurationRequest.builder()
.applyMutation(putInvitationConfigurationRequest).build());
}
/**
*
* Updates room skill parameter details by room, skill, and parameter key ID. Not all skills have a room skill
* parameter.
*
*
* @param putRoomSkillParameterRequest
* @return A Java Future containing the result of the PutRoomSkillParameter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutRoomSkillParameter
* @see AWS API Documentation
*/
default CompletableFuture putRoomSkillParameter(
PutRoomSkillParameterRequest putRoomSkillParameterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates room skill parameter details by room, skill, and parameter key ID. Not all skills have a room skill
* parameter.
*
*
*
* This is a convenience which creates an instance of the {@link PutRoomSkillParameterRequest.Builder} avoiding the
* need to create one manually via {@link PutRoomSkillParameterRequest#builder()}
*
*
* @param putRoomSkillParameterRequest
* A {@link Consumer} that will call methods on {@link PutRoomSkillParameterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutRoomSkillParameter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutRoomSkillParameter
* @see AWS API Documentation
*/
default CompletableFuture putRoomSkillParameter(
Consumer putRoomSkillParameterRequest) {
return putRoomSkillParameter(PutRoomSkillParameterRequest.builder().applyMutation(putRoomSkillParameterRequest).build());
}
/**
*
* Links a user's account to a third-party skill provider. If this API operation is called by an assumed IAM role,
* the skill being linked must be a private skill. Also, the skill must be owned by the AWS account that assumed the
* IAM role.
*
*
* @param putSkillAuthorizationRequest
* @return A Java Future containing the result of the PutSkillAuthorization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedException The caller has no permissions to operate on the resource involved in the API
* call.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutSkillAuthorization
* @see AWS API Documentation
*/
default CompletableFuture putSkillAuthorization(
PutSkillAuthorizationRequest putSkillAuthorizationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Links a user's account to a third-party skill provider. If this API operation is called by an assumed IAM role,
* the skill being linked must be a private skill. Also, the skill must be owned by the AWS account that assumed the
* IAM role.
*
*
*
* This is a convenience which creates an instance of the {@link PutSkillAuthorizationRequest.Builder} avoiding the
* need to create one manually via {@link PutSkillAuthorizationRequest#builder()}
*
*
* @param putSkillAuthorizationRequest
* A {@link Consumer} that will call methods on {@link PutSkillAuthorizationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutSkillAuthorization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedException The caller has no permissions to operate on the resource involved in the API
* call.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.PutSkillAuthorization
* @see AWS API Documentation
*/
default CompletableFuture putSkillAuthorization(
Consumer putSkillAuthorizationRequest) {
return putSkillAuthorization(PutSkillAuthorizationRequest.builder().applyMutation(putSkillAuthorizationRequest).build());
}
/**
*
* Registers an Alexa-enabled device built by an Original Equipment Manufacturer (OEM) using Alexa Voice Service
* (AVS).
*
*
* @param registerAvsDeviceRequest
* @return A Java Future containing the result of the RegisterAVSDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - InvalidDeviceException The device is in an invalid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.RegisterAVSDevice
* @see AWS API Documentation
*/
default CompletableFuture registerAVSDevice(RegisterAvsDeviceRequest registerAvsDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Registers an Alexa-enabled device built by an Original Equipment Manufacturer (OEM) using Alexa Voice Service
* (AVS).
*
*
*
* This is a convenience which creates an instance of the {@link RegisterAvsDeviceRequest.Builder} avoiding the need
* to create one manually via {@link RegisterAvsDeviceRequest#builder()}
*
*
* @param registerAvsDeviceRequest
* A {@link Consumer} that will call methods on {@link RegisterAVSDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the RegisterAVSDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - InvalidDeviceException The device is in an invalid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.RegisterAVSDevice
* @see AWS API Documentation
*/
default CompletableFuture registerAVSDevice(
Consumer registerAvsDeviceRequest) {
return registerAVSDevice(RegisterAvsDeviceRequest.builder().applyMutation(registerAvsDeviceRequest).build());
}
/**
*
* Disassociates a skill from the organization under a user's AWS account. If the skill is a private skill, it moves
* to an AcceptStatus of PENDING. Any private or public skill that is rejected can be added later by calling the
* ApproveSkill API.
*
*
* @param rejectSkillRequest
* @return A Java Future containing the result of the RejectSkill operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.RejectSkill
* @see AWS
* API Documentation
*/
default CompletableFuture rejectSkill(RejectSkillRequest rejectSkillRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a skill from the organization under a user's AWS account. If the skill is a private skill, it moves
* to an AcceptStatus of PENDING. Any private or public skill that is rejected can be added later by calling the
* ApproveSkill API.
*
*
*
* This is a convenience which creates an instance of the {@link RejectSkillRequest.Builder} avoiding the need to
* create one manually via {@link RejectSkillRequest#builder()}
*
*
* @param rejectSkillRequest
* A {@link Consumer} that will call methods on {@link RejectSkillRequest.Builder} to create a request.
* @return A Java Future containing the result of the RejectSkill operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is a concurrent modification of resources.
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.RejectSkill
* @see AWS
* API Documentation
*/
default CompletableFuture rejectSkill(Consumer rejectSkillRequest) {
return rejectSkill(RejectSkillRequest.builder().applyMutation(rejectSkillRequest).build());
}
/**
*
* Determines the details for the room from which a skill request was invoked. This operation is used by skill
* developers.
*
*
* To query ResolveRoom from an Alexa skill, the skill ID needs to be authorized. When the skill is using an AWS
* Lambda function, the skill is automatically authorized when you publish your skill as a private skill to your AWS
* account. Skills that are hosted using a custom web service must be manually authorized. To get your skill
* authorized, contact AWS Support with your AWS account ID that queries the ResolveRoom API and skill ID.
*
*
* @param resolveRoomRequest
* @return A Java Future containing the result of the ResolveRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ResolveRoom
* @see AWS
* API Documentation
*/
default CompletableFuture resolveRoom(ResolveRoomRequest resolveRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Determines the details for the room from which a skill request was invoked. This operation is used by skill
* developers.
*
*
* To query ResolveRoom from an Alexa skill, the skill ID needs to be authorized. When the skill is using an AWS
* Lambda function, the skill is automatically authorized when you publish your skill as a private skill to your AWS
* account. Skills that are hosted using a custom web service must be manually authorized. To get your skill
* authorized, contact AWS Support with your AWS account ID that queries the ResolveRoom API and skill ID.
*
*
*
* This is a convenience which creates an instance of the {@link ResolveRoomRequest.Builder} avoiding the need to
* create one manually via {@link ResolveRoomRequest#builder()}
*
*
* @param resolveRoomRequest
* A {@link Consumer} that will call methods on {@link ResolveRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the ResolveRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.ResolveRoom
* @see AWS
* API Documentation
*/
default CompletableFuture resolveRoom(Consumer resolveRoomRequest) {
return resolveRoom(ResolveRoomRequest.builder().applyMutation(resolveRoomRequest).build());
}
/**
*
* Revokes an invitation and invalidates the enrollment URL.
*
*
* @param revokeInvitationRequest
* @return A Java Future containing the result of the RevokeInvitation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.RevokeInvitation
* @see AWS API Documentation
*/
default CompletableFuture revokeInvitation(RevokeInvitationRequest revokeInvitationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Revokes an invitation and invalidates the enrollment URL.
*
*
*
* This is a convenience which creates an instance of the {@link RevokeInvitationRequest.Builder} avoiding the need
* to create one manually via {@link RevokeInvitationRequest#builder()}
*
*
* @param revokeInvitationRequest
* A {@link Consumer} that will call methods on {@link RevokeInvitationRequest.Builder} to create a request.
* @return A Java Future containing the result of the RevokeInvitation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The resource is not found.
* - ConcurrentModificationException There is a concurrent modification of resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.RevokeInvitation
* @see AWS API Documentation
*/
default CompletableFuture revokeInvitation(
Consumer revokeInvitationRequest) {
return revokeInvitation(RevokeInvitationRequest.builder().applyMutation(revokeInvitationRequest).build());
}
/**
*
* Searches address books and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchAddressBooksRequest
* @return A Java Future containing the result of the SearchAddressBooks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchAddressBooks
* @see AWS API Documentation
*/
default CompletableFuture searchAddressBooks(SearchAddressBooksRequest searchAddressBooksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches address books and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchAddressBooksRequest.Builder} avoiding the
* need to create one manually via {@link SearchAddressBooksRequest#builder()}
*
*
* @param searchAddressBooksRequest
* A {@link Consumer} that will call methods on {@link SearchAddressBooksRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SearchAddressBooks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchAddressBooks
* @see AWS API Documentation
*/
default CompletableFuture searchAddressBooks(
Consumer searchAddressBooksRequest) {
return searchAddressBooks(SearchAddressBooksRequest.builder().applyMutation(searchAddressBooksRequest).build());
}
/**
*
* Searches address books and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchAddressBooks(software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchAddressBooksPublisher publisher = client.searchAddressBooksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchAddressBooksPublisher publisher = client.searchAddressBooksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchAddressBooks(software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksRequest)}
* operation.
*
*
* @param searchAddressBooksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchAddressBooks
* @see AWS API Documentation
*/
default SearchAddressBooksPublisher searchAddressBooksPaginator(SearchAddressBooksRequest searchAddressBooksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches address books and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchAddressBooks(software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchAddressBooksPublisher publisher = client.searchAddressBooksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchAddressBooksPublisher publisher = client.searchAddressBooksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchAddressBooks(software.amazon.awssdk.services.alexaforbusiness.model.SearchAddressBooksRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchAddressBooksRequest.Builder} avoiding the
* need to create one manually via {@link SearchAddressBooksRequest#builder()}
*
*
* @param searchAddressBooksRequest
* A {@link Consumer} that will call methods on {@link SearchAddressBooksRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchAddressBooks
* @see AWS API Documentation
*/
default SearchAddressBooksPublisher searchAddressBooksPaginator(
Consumer searchAddressBooksRequest) {
return searchAddressBooksPaginator(SearchAddressBooksRequest.builder().applyMutation(searchAddressBooksRequest).build());
}
/**
*
* Searches contacts and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchContactsRequest
* @return A Java Future containing the result of the SearchContacts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchContacts
* @see AWS API Documentation
*/
default CompletableFuture searchContacts(SearchContactsRequest searchContactsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches contacts and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchContactsRequest.Builder} avoiding the need to
* create one manually via {@link SearchContactsRequest#builder()}
*
*
* @param searchContactsRequest
* A {@link Consumer} that will call methods on {@link SearchContactsRequest.Builder} to create a request.
* @return A Java Future containing the result of the SearchContacts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchContacts
* @see AWS API Documentation
*/
default CompletableFuture searchContacts(Consumer searchContactsRequest) {
return searchContacts(SearchContactsRequest.builder().applyMutation(searchContactsRequest).build());
}
/**
*
* Searches contacts and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchContacts(software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchContactsPublisher publisher = client.searchContactsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchContactsPublisher publisher = client.searchContactsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchContacts(software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsRequest)}
* operation.
*
*
* @param searchContactsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchContacts
* @see AWS API Documentation
*/
default SearchContactsPublisher searchContactsPaginator(SearchContactsRequest searchContactsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches contacts and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchContacts(software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchContactsPublisher publisher = client.searchContactsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchContactsPublisher publisher = client.searchContactsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchContacts(software.amazon.awssdk.services.alexaforbusiness.model.SearchContactsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchContactsRequest.Builder} avoiding the need to
* create one manually via {@link SearchContactsRequest#builder()}
*
*
* @param searchContactsRequest
* A {@link Consumer} that will call methods on {@link SearchContactsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchContacts
* @see AWS API Documentation
*/
default SearchContactsPublisher searchContactsPaginator(Consumer searchContactsRequest) {
return searchContactsPaginator(SearchContactsRequest.builder().applyMutation(searchContactsRequest).build());
}
/**
*
* Searches devices and lists the ones that meet a set of filter criteria.
*
*
* @param searchDevicesRequest
* @return A Java Future containing the result of the SearchDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchDevices
* @see AWS API Documentation
*/
default CompletableFuture searchDevices(SearchDevicesRequest searchDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches devices and lists the ones that meet a set of filter criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchDevicesRequest.Builder} avoiding the need to
* create one manually via {@link SearchDevicesRequest#builder()}
*
*
* @param searchDevicesRequest
* A {@link Consumer} that will call methods on {@link SearchDevicesRequest.Builder} to create a request.
* @return A Java Future containing the result of the SearchDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchDevices
* @see AWS API Documentation
*/
default CompletableFuture searchDevices(Consumer searchDevicesRequest) {
return searchDevices(SearchDevicesRequest.builder().applyMutation(searchDevicesRequest).build());
}
/**
*
* Searches devices and lists the ones that meet a set of filter criteria.
*
*
*
* This is a variant of
* {@link #searchDevices(software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchDevicesPublisher publisher = client.searchDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchDevicesPublisher publisher = client.searchDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchDevices(software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesRequest)}
* operation.
*
*
* @param searchDevicesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchDevices
* @see AWS API Documentation
*/
default SearchDevicesPublisher searchDevicesPaginator(SearchDevicesRequest searchDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches devices and lists the ones that meet a set of filter criteria.
*
*
*
* This is a variant of
* {@link #searchDevices(software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchDevicesPublisher publisher = client.searchDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchDevicesPublisher publisher = client.searchDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchDevices(software.amazon.awssdk.services.alexaforbusiness.model.SearchDevicesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchDevicesRequest.Builder} avoiding the need to
* create one manually via {@link SearchDevicesRequest#builder()}
*
*
* @param searchDevicesRequest
* A {@link Consumer} that will call methods on {@link SearchDevicesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchDevices
* @see AWS API Documentation
*/
default SearchDevicesPublisher searchDevicesPaginator(Consumer searchDevicesRequest) {
return searchDevicesPaginator(SearchDevicesRequest.builder().applyMutation(searchDevicesRequest).build());
}
/**
*
* Searches network profiles and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchNetworkProfilesRequest
* @return A Java Future containing the result of the SearchNetworkProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchNetworkProfiles
* @see AWS API Documentation
*/
default CompletableFuture searchNetworkProfiles(
SearchNetworkProfilesRequest searchNetworkProfilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches network profiles and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchNetworkProfilesRequest.Builder} avoiding the
* need to create one manually via {@link SearchNetworkProfilesRequest#builder()}
*
*
* @param searchNetworkProfilesRequest
* A {@link Consumer} that will call methods on {@link SearchNetworkProfilesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SearchNetworkProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchNetworkProfiles
* @see AWS API Documentation
*/
default CompletableFuture searchNetworkProfiles(
Consumer searchNetworkProfilesRequest) {
return searchNetworkProfiles(SearchNetworkProfilesRequest.builder().applyMutation(searchNetworkProfilesRequest).build());
}
/**
*
* Searches network profiles and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchNetworkProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchNetworkProfilesPublisher publisher = client.searchNetworkProfilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchNetworkProfilesPublisher publisher = client.searchNetworkProfilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchNetworkProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesRequest)}
* operation.
*
*
* @param searchNetworkProfilesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchNetworkProfiles
* @see AWS API Documentation
*/
default SearchNetworkProfilesPublisher searchNetworkProfilesPaginator(
SearchNetworkProfilesRequest searchNetworkProfilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches network profiles and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchNetworkProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchNetworkProfilesPublisher publisher = client.searchNetworkProfilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchNetworkProfilesPublisher publisher = client.searchNetworkProfilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchNetworkProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchNetworkProfilesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchNetworkProfilesRequest.Builder} avoiding the
* need to create one manually via {@link SearchNetworkProfilesRequest#builder()}
*
*
* @param searchNetworkProfilesRequest
* A {@link Consumer} that will call methods on {@link SearchNetworkProfilesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchNetworkProfiles
* @see AWS API Documentation
*/
default SearchNetworkProfilesPublisher searchNetworkProfilesPaginator(
Consumer searchNetworkProfilesRequest) {
return searchNetworkProfilesPaginator(SearchNetworkProfilesRequest.builder().applyMutation(searchNetworkProfilesRequest)
.build());
}
/**
*
* Searches room profiles and lists the ones that meet a set of filter criteria.
*
*
* @param searchProfilesRequest
* @return A Java Future containing the result of the SearchProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchProfiles
* @see AWS API Documentation
*/
default CompletableFuture searchProfiles(SearchProfilesRequest searchProfilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches room profiles and lists the ones that meet a set of filter criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchProfilesRequest.Builder} avoiding the need to
* create one manually via {@link SearchProfilesRequest#builder()}
*
*
* @param searchProfilesRequest
* A {@link Consumer} that will call methods on {@link SearchProfilesRequest.Builder} to create a request.
* @return A Java Future containing the result of the SearchProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchProfiles
* @see AWS API Documentation
*/
default CompletableFuture searchProfiles(Consumer searchProfilesRequest) {
return searchProfiles(SearchProfilesRequest.builder().applyMutation(searchProfilesRequest).build());
}
/**
*
* Searches room profiles and lists the ones that meet a set of filter criteria.
*
*
*
* This is a variant of
* {@link #searchProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchProfilesPublisher publisher = client.searchProfilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchProfilesPublisher publisher = client.searchProfilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesRequest)}
* operation.
*
*
* @param searchProfilesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchProfiles
* @see AWS API Documentation
*/
default SearchProfilesPublisher searchProfilesPaginator(SearchProfilesRequest searchProfilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches room profiles and lists the ones that meet a set of filter criteria.
*
*
*
* This is a variant of
* {@link #searchProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchProfilesPublisher publisher = client.searchProfilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchProfilesPublisher publisher = client.searchProfilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchProfiles(software.amazon.awssdk.services.alexaforbusiness.model.SearchProfilesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchProfilesRequest.Builder} avoiding the need to
* create one manually via {@link SearchProfilesRequest#builder()}
*
*
* @param searchProfilesRequest
* A {@link Consumer} that will call methods on {@link SearchProfilesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchProfiles
* @see AWS API Documentation
*/
default SearchProfilesPublisher searchProfilesPaginator(Consumer searchProfilesRequest) {
return searchProfilesPaginator(SearchProfilesRequest.builder().applyMutation(searchProfilesRequest).build());
}
/**
*
* Searches rooms and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchRoomsRequest
* @return A Java Future containing the result of the SearchRooms operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchRooms
* @see AWS
* API Documentation
*/
default CompletableFuture searchRooms(SearchRoomsRequest searchRoomsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches rooms and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchRoomsRequest.Builder} avoiding the need to
* create one manually via {@link SearchRoomsRequest#builder()}
*
*
* @param searchRoomsRequest
* A {@link Consumer} that will call methods on {@link SearchRoomsRequest.Builder} to create a request.
* @return A Java Future containing the result of the SearchRooms operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchRooms
* @see AWS
* API Documentation
*/
default CompletableFuture searchRooms(Consumer searchRoomsRequest) {
return searchRooms(SearchRoomsRequest.builder().applyMutation(searchRoomsRequest).build());
}
/**
*
* Searches rooms and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchRooms(software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchRoomsPublisher publisher = client.searchRoomsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchRoomsPublisher publisher = client.searchRoomsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchRooms(software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsRequest)} operation.
*
*
* @param searchRoomsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchRooms
* @see AWS
* API Documentation
*/
default SearchRoomsPublisher searchRoomsPaginator(SearchRoomsRequest searchRoomsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches rooms and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchRooms(software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchRoomsPublisher publisher = client.searchRoomsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchRoomsPublisher publisher = client.searchRoomsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchRooms(software.amazon.awssdk.services.alexaforbusiness.model.SearchRoomsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link SearchRoomsRequest.Builder} avoiding the need to
* create one manually via {@link SearchRoomsRequest#builder()}
*
*
* @param searchRoomsRequest
* A {@link Consumer} that will call methods on {@link SearchRoomsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchRooms
* @see AWS
* API Documentation
*/
default SearchRoomsPublisher searchRoomsPaginator(Consumer searchRoomsRequest) {
return searchRoomsPaginator(SearchRoomsRequest.builder().applyMutation(searchRoomsRequest).build());
}
/**
*
* Searches skill groups and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchSkillGroupsRequest
* @return A Java Future containing the result of the SearchSkillGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchSkillGroups
* @see AWS API Documentation
*/
default CompletableFuture searchSkillGroups(SearchSkillGroupsRequest searchSkillGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches skill groups and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchSkillGroupsRequest.Builder} avoiding the need
* to create one manually via {@link SearchSkillGroupsRequest#builder()}
*
*
* @param searchSkillGroupsRequest
* A {@link Consumer} that will call methods on {@link SearchSkillGroupsRequest.Builder} to create a request.
* @return A Java Future containing the result of the SearchSkillGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchSkillGroups
* @see AWS API Documentation
*/
default CompletableFuture searchSkillGroups(
Consumer searchSkillGroupsRequest) {
return searchSkillGroups(SearchSkillGroupsRequest.builder().applyMutation(searchSkillGroupsRequest).build());
}
/**
*
* Searches skill groups and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchSkillGroups(software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchSkillGroupsPublisher publisher = client.searchSkillGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchSkillGroupsPublisher publisher = client.searchSkillGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchSkillGroups(software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsRequest)}
* operation.
*
*
* @param searchSkillGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchSkillGroups
* @see AWS API Documentation
*/
default SearchSkillGroupsPublisher searchSkillGroupsPaginator(SearchSkillGroupsRequest searchSkillGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches skill groups and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchSkillGroups(software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchSkillGroupsPublisher publisher = client.searchSkillGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchSkillGroupsPublisher publisher = client.searchSkillGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchSkillGroups(software.amazon.awssdk.services.alexaforbusiness.model.SearchSkillGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchSkillGroupsRequest.Builder} avoiding the need
* to create one manually via {@link SearchSkillGroupsRequest#builder()}
*
*
* @param searchSkillGroupsRequest
* A {@link Consumer} that will call methods on {@link SearchSkillGroupsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchSkillGroups
* @see AWS API Documentation
*/
default SearchSkillGroupsPublisher searchSkillGroupsPaginator(
Consumer searchSkillGroupsRequest) {
return searchSkillGroupsPaginator(SearchSkillGroupsRequest.builder().applyMutation(searchSkillGroupsRequest).build());
}
/**
*
* Searches users and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchUsersRequest
* @return A Java Future containing the result of the SearchUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchUsers
* @see AWS
* API Documentation
*/
default CompletableFuture searchUsers(SearchUsersRequest searchUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches users and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a convenience which creates an instance of the {@link SearchUsersRequest.Builder} avoiding the need to
* create one manually via {@link SearchUsersRequest#builder()}
*
*
* @param searchUsersRequest
* A {@link Consumer} that will call methods on {@link SearchUsersRequest.Builder} to create a request.
* @return A Java Future containing the result of the SearchUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchUsers
* @see AWS
* API Documentation
*/
default CompletableFuture searchUsers(Consumer searchUsersRequest) {
return searchUsers(SearchUsersRequest.builder().applyMutation(searchUsersRequest).build());
}
/**
*
* Searches users and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchUsers(software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchUsersPublisher publisher = client.searchUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchUsersPublisher publisher = client.searchUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchUsers(software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersRequest)} operation.
*
*
* @param searchUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchUsers
* @see AWS
* API Documentation
*/
default SearchUsersPublisher searchUsersPaginator(SearchUsersRequest searchUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Searches users and lists the ones that meet a set of filter and sort criteria.
*
*
*
* This is a variant of
* {@link #searchUsers(software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchUsersPublisher publisher = client.searchUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.alexaforbusiness.paginators.SearchUsersPublisher publisher = client.searchUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchUsers(software.amazon.awssdk.services.alexaforbusiness.model.SearchUsersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link SearchUsersRequest.Builder} avoiding the need to
* create one manually via {@link SearchUsersRequest#builder()}
*
*
* @param searchUsersRequest
* A {@link Consumer} that will call methods on {@link SearchUsersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SearchUsers
* @see AWS
* API Documentation
*/
default SearchUsersPublisher searchUsersPaginator(Consumer searchUsersRequest) {
return searchUsersPaginator(SearchUsersRequest.builder().applyMutation(searchUsersRequest).build());
}
/**
*
* Triggers an asynchronous flow to send text, SSML, or audio announcements to rooms that are identified by a search
* or filter.
*
*
* @param sendAnnouncementRequest
* @return A Java Future containing the result of the SendAnnouncement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - AlreadyExistsException The resource being created already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SendAnnouncement
* @see AWS API Documentation
*/
default CompletableFuture sendAnnouncement(SendAnnouncementRequest sendAnnouncementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Triggers an asynchronous flow to send text, SSML, or audio announcements to rooms that are identified by a search
* or filter.
*
*
*
* This is a convenience which creates an instance of the {@link SendAnnouncementRequest.Builder} avoiding the need
* to create one manually via {@link SendAnnouncementRequest#builder()}
*
*
* @param sendAnnouncementRequest
* A {@link Consumer} that will call methods on {@link SendAnnouncementRequest.Builder} to create a request.
* @return A Java Future containing the result of the SendAnnouncement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You are performing an action that would put you beyond your account's limits.
* - AlreadyExistsException The resource being created already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AlexaForBusinessException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample AlexaForBusinessAsyncClient.SendAnnouncement
* @see AWS API Documentation
*/
default CompletableFuture sendAnnouncement(
Consumer sendAnnouncementRequest) {
return sendAnnouncement(SendAnnouncementRequest.builder().applyMutation(sendAnnouncementRequest).build());
}
/**
*
* Sends an enrollment invitation email with a URL to a user. The URL is valid for 30 days or until you call this
* operation again, whichever comes first.
*
*
* @param sendInvitationRequest
* @return A Java Future containing the result of the SendInvitation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
*