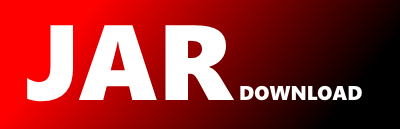
software.amazon.awssdk.services.amp.AmpAsyncClient Maven / Gradle / Ivy
Show all versions of amp Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.amp;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.amp.model.CreateAlertManagerDefinitionRequest;
import software.amazon.awssdk.services.amp.model.CreateAlertManagerDefinitionResponse;
import software.amazon.awssdk.services.amp.model.CreateLoggingConfigurationRequest;
import software.amazon.awssdk.services.amp.model.CreateLoggingConfigurationResponse;
import software.amazon.awssdk.services.amp.model.CreateRuleGroupsNamespaceRequest;
import software.amazon.awssdk.services.amp.model.CreateRuleGroupsNamespaceResponse;
import software.amazon.awssdk.services.amp.model.CreateScraperRequest;
import software.amazon.awssdk.services.amp.model.CreateScraperResponse;
import software.amazon.awssdk.services.amp.model.CreateWorkspaceRequest;
import software.amazon.awssdk.services.amp.model.CreateWorkspaceResponse;
import software.amazon.awssdk.services.amp.model.DeleteAlertManagerDefinitionRequest;
import software.amazon.awssdk.services.amp.model.DeleteAlertManagerDefinitionResponse;
import software.amazon.awssdk.services.amp.model.DeleteLoggingConfigurationRequest;
import software.amazon.awssdk.services.amp.model.DeleteLoggingConfigurationResponse;
import software.amazon.awssdk.services.amp.model.DeleteRuleGroupsNamespaceRequest;
import software.amazon.awssdk.services.amp.model.DeleteRuleGroupsNamespaceResponse;
import software.amazon.awssdk.services.amp.model.DeleteScraperRequest;
import software.amazon.awssdk.services.amp.model.DeleteScraperResponse;
import software.amazon.awssdk.services.amp.model.DeleteWorkspaceRequest;
import software.amazon.awssdk.services.amp.model.DeleteWorkspaceResponse;
import software.amazon.awssdk.services.amp.model.DescribeAlertManagerDefinitionRequest;
import software.amazon.awssdk.services.amp.model.DescribeAlertManagerDefinitionResponse;
import software.amazon.awssdk.services.amp.model.DescribeLoggingConfigurationRequest;
import software.amazon.awssdk.services.amp.model.DescribeLoggingConfigurationResponse;
import software.amazon.awssdk.services.amp.model.DescribeRuleGroupsNamespaceRequest;
import software.amazon.awssdk.services.amp.model.DescribeRuleGroupsNamespaceResponse;
import software.amazon.awssdk.services.amp.model.DescribeScraperRequest;
import software.amazon.awssdk.services.amp.model.DescribeScraperResponse;
import software.amazon.awssdk.services.amp.model.DescribeWorkspaceRequest;
import software.amazon.awssdk.services.amp.model.DescribeWorkspaceResponse;
import software.amazon.awssdk.services.amp.model.GetDefaultScraperConfigurationRequest;
import software.amazon.awssdk.services.amp.model.GetDefaultScraperConfigurationResponse;
import software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest;
import software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesResponse;
import software.amazon.awssdk.services.amp.model.ListScrapersRequest;
import software.amazon.awssdk.services.amp.model.ListScrapersResponse;
import software.amazon.awssdk.services.amp.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.amp.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.amp.model.ListWorkspacesRequest;
import software.amazon.awssdk.services.amp.model.ListWorkspacesResponse;
import software.amazon.awssdk.services.amp.model.PutAlertManagerDefinitionRequest;
import software.amazon.awssdk.services.amp.model.PutAlertManagerDefinitionResponse;
import software.amazon.awssdk.services.amp.model.PutRuleGroupsNamespaceRequest;
import software.amazon.awssdk.services.amp.model.PutRuleGroupsNamespaceResponse;
import software.amazon.awssdk.services.amp.model.TagResourceRequest;
import software.amazon.awssdk.services.amp.model.TagResourceResponse;
import software.amazon.awssdk.services.amp.model.UntagResourceRequest;
import software.amazon.awssdk.services.amp.model.UntagResourceResponse;
import software.amazon.awssdk.services.amp.model.UpdateLoggingConfigurationRequest;
import software.amazon.awssdk.services.amp.model.UpdateLoggingConfigurationResponse;
import software.amazon.awssdk.services.amp.model.UpdateScraperRequest;
import software.amazon.awssdk.services.amp.model.UpdateScraperResponse;
import software.amazon.awssdk.services.amp.model.UpdateWorkspaceAliasRequest;
import software.amazon.awssdk.services.amp.model.UpdateWorkspaceAliasResponse;
import software.amazon.awssdk.services.amp.paginators.ListRuleGroupsNamespacesPublisher;
import software.amazon.awssdk.services.amp.paginators.ListScrapersPublisher;
import software.amazon.awssdk.services.amp.paginators.ListWorkspacesPublisher;
import software.amazon.awssdk.services.amp.waiters.AmpAsyncWaiter;
/**
* Service client for accessing Amazon Prometheus Service asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* Amazon Managed Service for Prometheus is a serverless, Prometheus-compatible monitoring service for container metrics
* that makes it easier to securely monitor container environments at scale. With Amazon Managed Service for Prometheus,
* you can use the same open-source Prometheus data model and query language that you use today to monitor the
* performance of your containerized workloads, and also enjoy improved scalability, availability, and security without
* having to manage the underlying infrastructure.
*
*
* For more information about Amazon Managed Service for Prometheus, see the Amazon
* Managed Service for Prometheus User Guide.
*
*
* Amazon Managed Service for Prometheus includes two APIs.
*
*
* -
*
* Use the Amazon Web Services API described in this guide to manage Amazon Managed Service for Prometheus resources,
* such as workspaces, rule groups, and alert managers.
*
*
* -
*
* Use the Prometheus-compatible API to work within your Prometheus workspace.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface AmpAsyncClient extends AwsClient {
String SERVICE_NAME = "aps";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "aps";
/**
*
* The CreateAlertManagerDefinition
operation creates the alert manager definition in a workspace. If a
* workspace already has an alert manager definition, don't use this operation to update it. Instead, use
* PutAlertManagerDefinition
.
*
*
* @param createAlertManagerDefinitionRequest
* Represents the input of a CreateAlertManagerDefinition
operation.
* @return A Java Future containing the result of the CreateAlertManagerDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateAlertManagerDefinition
* @see AWS API Documentation
*/
default CompletableFuture createAlertManagerDefinition(
CreateAlertManagerDefinitionRequest createAlertManagerDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The CreateAlertManagerDefinition
operation creates the alert manager definition in a workspace. If a
* workspace already has an alert manager definition, don't use this operation to update it. Instead, use
* PutAlertManagerDefinition
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAlertManagerDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link CreateAlertManagerDefinitionRequest#builder()}
*
*
* @param createAlertManagerDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.CreateAlertManagerDefinitionRequest.Builder} to create a
* request. Represents the input of a CreateAlertManagerDefinition
operation.
* @return A Java Future containing the result of the CreateAlertManagerDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateAlertManagerDefinition
* @see AWS API Documentation
*/
default CompletableFuture createAlertManagerDefinition(
Consumer createAlertManagerDefinitionRequest) {
return createAlertManagerDefinition(CreateAlertManagerDefinitionRequest.builder()
.applyMutation(createAlertManagerDefinitionRequest).build());
}
/**
*
* The CreateLoggingConfiguration
operation creates a logging configuration for the workspace. Use this
* operation to set the CloudWatch log group to which the logs will be published to.
*
*
* @param createLoggingConfigurationRequest
* Represents the input of a CreateLoggingConfiguration
operation.
* @return A Java Future containing the result of the CreateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture createLoggingConfiguration(
CreateLoggingConfigurationRequest createLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The CreateLoggingConfiguration
operation creates a logging configuration for the workspace. Use this
* operation to set the CloudWatch log group to which the logs will be published to.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link CreateLoggingConfigurationRequest#builder()}
*
*
* @param createLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.CreateLoggingConfigurationRequest.Builder} to create a
* request. Represents the input of a CreateLoggingConfiguration
operation.
* @return A Java Future containing the result of the CreateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture createLoggingConfiguration(
Consumer createLoggingConfigurationRequest) {
return createLoggingConfiguration(CreateLoggingConfigurationRequest.builder()
.applyMutation(createLoggingConfigurationRequest).build());
}
/**
*
* The CreateRuleGroupsNamespace
operation creates a rule groups namespace within a workspace. A rule
* groups namespace is associated with exactly one rules file. A workspace can have multiple rule groups namespaces.
*
*
* Use this operation only to create new rule groups namespaces. To update an existing rule groups namespace, use
* PutRuleGroupsNamespace
.
*
*
* @param createRuleGroupsNamespaceRequest
* Represents the input of a CreateRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the CreateRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateRuleGroupsNamespace
* @see AWS
* API Documentation
*/
default CompletableFuture createRuleGroupsNamespace(
CreateRuleGroupsNamespaceRequest createRuleGroupsNamespaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The CreateRuleGroupsNamespace
operation creates a rule groups namespace within a workspace. A rule
* groups namespace is associated with exactly one rules file. A workspace can have multiple rule groups namespaces.
*
*
* Use this operation only to create new rule groups namespaces. To update an existing rule groups namespace, use
* PutRuleGroupsNamespace
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRuleGroupsNamespaceRequest.Builder} avoiding
* the need to create one manually via {@link CreateRuleGroupsNamespaceRequest#builder()}
*
*
* @param createRuleGroupsNamespaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.CreateRuleGroupsNamespaceRequest.Builder} to create a
* request. Represents the input of a CreateRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the CreateRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateRuleGroupsNamespace
* @see AWS
* API Documentation
*/
default CompletableFuture createRuleGroupsNamespace(
Consumer createRuleGroupsNamespaceRequest) {
return createRuleGroupsNamespace(CreateRuleGroupsNamespaceRequest.builder()
.applyMutation(createRuleGroupsNamespaceRequest).build());
}
/**
*
* The CreateScraper
operation creates a scraper to collect metrics. A scraper pulls metrics from
* Prometheus-compatible sources within an Amazon EKS cluster, and sends them to your Amazon Managed Service for
* Prometheus workspace. Scrapers are flexible, and can be configured to control what metrics are collected, the
* frequency of collection, what transformations are applied to the metrics, and more.
*
*
* An IAM role will be created for you that Amazon Managed Service for Prometheus uses to access the metrics in your
* cluster. You must configure this role with a policy that allows it to scrape metrics from your cluster. For more
* information, see Configuring your Amazon EKS cluster in the Amazon Managed Service for Prometheus User Guide.
*
*
* The scrapeConfiguration
parameter contains the base-64 encoded YAML configuration for the scraper.
*
*
*
* For more information about collectors, including what metrics are collected, and how to configure the scraper,
* see Using an Amazon
* Web Services managed collector in the Amazon Managed Service for Prometheus User Guide.
*
*
*
* @param createScraperRequest
* Represents the input of a CreateScraper
operation.
* @return A Java Future containing the result of the CreateScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateScraper
* @see AWS API
* Documentation
*/
default CompletableFuture createScraper(CreateScraperRequest createScraperRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The CreateScraper
operation creates a scraper to collect metrics. A scraper pulls metrics from
* Prometheus-compatible sources within an Amazon EKS cluster, and sends them to your Amazon Managed Service for
* Prometheus workspace. Scrapers are flexible, and can be configured to control what metrics are collected, the
* frequency of collection, what transformations are applied to the metrics, and more.
*
*
* An IAM role will be created for you that Amazon Managed Service for Prometheus uses to access the metrics in your
* cluster. You must configure this role with a policy that allows it to scrape metrics from your cluster. For more
* information, see Configuring your Amazon EKS cluster in the Amazon Managed Service for Prometheus User Guide.
*
*
* The scrapeConfiguration
parameter contains the base-64 encoded YAML configuration for the scraper.
*
*
*
* For more information about collectors, including what metrics are collected, and how to configure the scraper,
* see Using an Amazon
* Web Services managed collector in the Amazon Managed Service for Prometheus User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateScraperRequest.Builder} avoiding the need to
* create one manually via {@link CreateScraperRequest#builder()}
*
*
* @param createScraperRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.CreateScraperRequest.Builder} to create a request.
* Represents the input of a CreateScraper
operation.
* @return A Java Future containing the result of the CreateScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateScraper
* @see AWS API
* Documentation
*/
default CompletableFuture createScraper(Consumer createScraperRequest) {
return createScraper(CreateScraperRequest.builder().applyMutation(createScraperRequest).build());
}
/**
*
* Creates a Prometheus workspace. A workspace is a logical space dedicated to the storage and querying of
* Prometheus metrics. You can have one or more workspaces in each Region in your account.
*
*
* @param createWorkspaceRequest
* Represents the input of a CreateWorkspace
operation.
* @return A Java Future containing the result of the CreateWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture createWorkspace(CreateWorkspaceRequest createWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Prometheus workspace. A workspace is a logical space dedicated to the storage and querying of
* Prometheus metrics. You can have one or more workspaces in each Region in your account.
*
*
*
* This is a convenience which creates an instance of the {@link CreateWorkspaceRequest.Builder} avoiding the need
* to create one manually via {@link CreateWorkspaceRequest#builder()}
*
*
* @param createWorkspaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.CreateWorkspaceRequest.Builder} to create a request.
* Represents the input of a CreateWorkspace
operation.
* @return A Java Future containing the result of the CreateWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.CreateWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture createWorkspace(
Consumer createWorkspaceRequest) {
return createWorkspace(CreateWorkspaceRequest.builder().applyMutation(createWorkspaceRequest).build());
}
/**
*
* Deletes the alert manager definition from a workspace.
*
*
* @param deleteAlertManagerDefinitionRequest
* Represents the input of a DeleteAlertManagerDefinition
operation.
* @return A Java Future containing the result of the DeleteAlertManagerDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteAlertManagerDefinition
* @see AWS API Documentation
*/
default CompletableFuture deleteAlertManagerDefinition(
DeleteAlertManagerDefinitionRequest deleteAlertManagerDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the alert manager definition from a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAlertManagerDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteAlertManagerDefinitionRequest#builder()}
*
*
* @param deleteAlertManagerDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DeleteAlertManagerDefinitionRequest.Builder} to create a
* request. Represents the input of a DeleteAlertManagerDefinition
operation.
* @return A Java Future containing the result of the DeleteAlertManagerDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteAlertManagerDefinition
* @see AWS API Documentation
*/
default CompletableFuture deleteAlertManagerDefinition(
Consumer deleteAlertManagerDefinitionRequest) {
return deleteAlertManagerDefinition(DeleteAlertManagerDefinitionRequest.builder()
.applyMutation(deleteAlertManagerDefinitionRequest).build());
}
/**
*
* Deletes the logging configuration for a workspace.
*
*
* @param deleteLoggingConfigurationRequest
* Represents the input of a DeleteLoggingConfiguration
operation.
* @return A Java Future containing the result of the DeleteLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteLoggingConfiguration(
DeleteLoggingConfigurationRequest deleteLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the logging configuration for a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link DeleteLoggingConfigurationRequest#builder()}
*
*
* @param deleteLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DeleteLoggingConfigurationRequest.Builder} to create a
* request. Represents the input of a DeleteLoggingConfiguration
operation.
* @return A Java Future containing the result of the DeleteLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteLoggingConfiguration(
Consumer deleteLoggingConfigurationRequest) {
return deleteLoggingConfiguration(DeleteLoggingConfigurationRequest.builder()
.applyMutation(deleteLoggingConfigurationRequest).build());
}
/**
*
* Deletes one rule groups namespace and its associated rule groups definition.
*
*
* @param deleteRuleGroupsNamespaceRequest
* Represents the input of a DeleteRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the DeleteRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteRuleGroupsNamespace
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRuleGroupsNamespace(
DeleteRuleGroupsNamespaceRequest deleteRuleGroupsNamespaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes one rule groups namespace and its associated rule groups definition.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRuleGroupsNamespaceRequest.Builder} avoiding
* the need to create one manually via {@link DeleteRuleGroupsNamespaceRequest#builder()}
*
*
* @param deleteRuleGroupsNamespaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DeleteRuleGroupsNamespaceRequest.Builder} to create a
* request. Represents the input of a DeleteRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the DeleteRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteRuleGroupsNamespace
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRuleGroupsNamespace(
Consumer deleteRuleGroupsNamespaceRequest) {
return deleteRuleGroupsNamespace(DeleteRuleGroupsNamespaceRequest.builder()
.applyMutation(deleteRuleGroupsNamespaceRequest).build());
}
/**
*
* The DeleteScraper
operation deletes one scraper, and stops any metrics collection that the scraper
* performs.
*
*
* @param deleteScraperRequest
* Represents the input of a DeleteScraper
operation.
* @return A Java Future containing the result of the DeleteScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteScraper
* @see AWS API
* Documentation
*/
default CompletableFuture deleteScraper(DeleteScraperRequest deleteScraperRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The DeleteScraper
operation deletes one scraper, and stops any metrics collection that the scraper
* performs.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteScraperRequest.Builder} avoiding the need to
* create one manually via {@link DeleteScraperRequest#builder()}
*
*
* @param deleteScraperRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DeleteScraperRequest.Builder} to create a request.
* Represents the input of a DeleteScraper
operation.
* @return A Java Future containing the result of the DeleteScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteScraper
* @see AWS API
* Documentation
*/
default CompletableFuture deleteScraper(Consumer deleteScraperRequest) {
return deleteScraper(DeleteScraperRequest.builder().applyMutation(deleteScraperRequest).build());
}
/**
*
* Deletes an existing workspace.
*
*
*
* When you delete a workspace, the data that has been ingested into it is not immediately deleted. It will be
* permanently deleted within one month.
*
*
*
* @param deleteWorkspaceRequest
* Represents the input of a DeleteWorkspace
operation.
* @return A Java Future containing the result of the DeleteWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture deleteWorkspace(DeleteWorkspaceRequest deleteWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing workspace.
*
*
*
* When you delete a workspace, the data that has been ingested into it is not immediately deleted. It will be
* permanently deleted within one month.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteWorkspaceRequest.Builder} avoiding the need
* to create one manually via {@link DeleteWorkspaceRequest#builder()}
*
*
* @param deleteWorkspaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DeleteWorkspaceRequest.Builder} to create a request.
* Represents the input of a DeleteWorkspace
operation.
* @return A Java Future containing the result of the DeleteWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DeleteWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture deleteWorkspace(
Consumer deleteWorkspaceRequest) {
return deleteWorkspace(DeleteWorkspaceRequest.builder().applyMutation(deleteWorkspaceRequest).build());
}
/**
*
* Retrieves the full information about the alert manager definition for a workspace.
*
*
* @param describeAlertManagerDefinitionRequest
* Represents the input of a DescribeAlertManagerDefinition
operation.
* @return A Java Future containing the result of the DescribeAlertManagerDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeAlertManagerDefinition
* @see AWS API Documentation
*/
default CompletableFuture describeAlertManagerDefinition(
DescribeAlertManagerDefinitionRequest describeAlertManagerDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the full information about the alert manager definition for a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAlertManagerDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link DescribeAlertManagerDefinitionRequest#builder()}
*
*
* @param describeAlertManagerDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DescribeAlertManagerDefinitionRequest.Builder} to create
* a request. Represents the input of a DescribeAlertManagerDefinition
operation.
* @return A Java Future containing the result of the DescribeAlertManagerDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeAlertManagerDefinition
* @see AWS API Documentation
*/
default CompletableFuture describeAlertManagerDefinition(
Consumer describeAlertManagerDefinitionRequest) {
return describeAlertManagerDefinition(DescribeAlertManagerDefinitionRequest.builder()
.applyMutation(describeAlertManagerDefinitionRequest).build());
}
/**
*
* Returns complete information about the current logging configuration of the workspace.
*
*
* @param describeLoggingConfigurationRequest
* Represents the input of a DescribeLoggingConfiguration
operation.
* @return A Java Future containing the result of the DescribeLoggingConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture describeLoggingConfiguration(
DescribeLoggingConfigurationRequest describeLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns complete information about the current logging configuration of the workspace.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLoggingConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DescribeLoggingConfigurationRequest#builder()}
*
*
* @param describeLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DescribeLoggingConfigurationRequest.Builder} to create a
* request. Represents the input of a DescribeLoggingConfiguration
operation.
* @return A Java Future containing the result of the DescribeLoggingConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture describeLoggingConfiguration(
Consumer describeLoggingConfigurationRequest) {
return describeLoggingConfiguration(DescribeLoggingConfigurationRequest.builder()
.applyMutation(describeLoggingConfigurationRequest).build());
}
/**
*
* Returns complete information about one rule groups namespace. To retrieve a list of rule groups namespaces, use
* ListRuleGroupsNamespaces
.
*
*
* @param describeRuleGroupsNamespaceRequest
* Represents the input of a DescribeRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the DescribeRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeRuleGroupsNamespace
* @see AWS API Documentation
*/
default CompletableFuture describeRuleGroupsNamespace(
DescribeRuleGroupsNamespaceRequest describeRuleGroupsNamespaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns complete information about one rule groups namespace. To retrieve a list of rule groups namespaces, use
* ListRuleGroupsNamespaces
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRuleGroupsNamespaceRequest.Builder}
* avoiding the need to create one manually via {@link DescribeRuleGroupsNamespaceRequest#builder()}
*
*
* @param describeRuleGroupsNamespaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DescribeRuleGroupsNamespaceRequest.Builder} to create a
* request. Represents the input of a DescribeRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the DescribeRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeRuleGroupsNamespace
* @see AWS API Documentation
*/
default CompletableFuture describeRuleGroupsNamespace(
Consumer describeRuleGroupsNamespaceRequest) {
return describeRuleGroupsNamespace(DescribeRuleGroupsNamespaceRequest.builder()
.applyMutation(describeRuleGroupsNamespaceRequest).build());
}
/**
*
* The DescribeScraper
operation displays information about an existing scraper.
*
*
* @param describeScraperRequest
* Represents the input of a DescribeScraper
operation.
* @return A Java Future containing the result of the DescribeScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeScraper
* @see AWS API
* Documentation
*/
default CompletableFuture describeScraper(DescribeScraperRequest describeScraperRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The DescribeScraper
operation displays information about an existing scraper.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeScraperRequest.Builder} avoiding the need
* to create one manually via {@link DescribeScraperRequest#builder()}
*
*
* @param describeScraperRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DescribeScraperRequest.Builder} to create a request.
* Represents the input of a DescribeScraper
operation.
* @return A Java Future containing the result of the DescribeScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeScraper
* @see AWS API
* Documentation
*/
default CompletableFuture describeScraper(
Consumer describeScraperRequest) {
return describeScraper(DescribeScraperRequest.builder().applyMutation(describeScraperRequest).build());
}
/**
*
* Returns information about an existing workspace.
*
*
* @param describeWorkspaceRequest
* Represents the input of a DescribeWorkspace
operation.
* @return A Java Future containing the result of the DescribeWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture describeWorkspace(DescribeWorkspaceRequest describeWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about an existing workspace.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeWorkspaceRequest.Builder} avoiding the need
* to create one manually via {@link DescribeWorkspaceRequest#builder()}
*
*
* @param describeWorkspaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.DescribeWorkspaceRequest.Builder} to create a request.
* Represents the input of a DescribeWorkspace
operation.
* @return A Java Future containing the result of the DescribeWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.DescribeWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture describeWorkspace(
Consumer describeWorkspaceRequest) {
return describeWorkspace(DescribeWorkspaceRequest.builder().applyMutation(describeWorkspaceRequest).build());
}
/**
*
* The GetDefaultScraperConfiguration
operation returns the default scraper configuration used when
* Amazon EKS creates a scraper for you.
*
*
* @param getDefaultScraperConfigurationRequest
* Represents the input of a GetDefaultScraperConfiguration
operation.
* @return A Java Future containing the result of the GetDefaultScraperConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.GetDefaultScraperConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getDefaultScraperConfiguration(
GetDefaultScraperConfigurationRequest getDefaultScraperConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The GetDefaultScraperConfiguration
operation returns the default scraper configuration used when
* Amazon EKS creates a scraper for you.
*
*
*
* This is a convenience which creates an instance of the {@link GetDefaultScraperConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetDefaultScraperConfigurationRequest#builder()}
*
*
* @param getDefaultScraperConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.GetDefaultScraperConfigurationRequest.Builder} to create
* a request. Represents the input of a GetDefaultScraperConfiguration
operation.
* @return A Java Future containing the result of the GetDefaultScraperConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.GetDefaultScraperConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getDefaultScraperConfiguration(
Consumer getDefaultScraperConfigurationRequest) {
return getDefaultScraperConfiguration(GetDefaultScraperConfigurationRequest.builder()
.applyMutation(getDefaultScraperConfigurationRequest).build());
}
/**
*
* Returns a list of rule groups namespaces in a workspace.
*
*
* @param listRuleGroupsNamespacesRequest
* Represents the input of a ListRuleGroupsNamespaces
operation.
* @return A Java Future containing the result of the ListRuleGroupsNamespaces operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListRuleGroupsNamespaces
* @see AWS
* API Documentation
*/
default CompletableFuture listRuleGroupsNamespaces(
ListRuleGroupsNamespacesRequest listRuleGroupsNamespacesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of rule groups namespaces in a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link ListRuleGroupsNamespacesRequest.Builder} avoiding
* the need to create one manually via {@link ListRuleGroupsNamespacesRequest#builder()}
*
*
* @param listRuleGroupsNamespacesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest.Builder} to create a
* request. Represents the input of a ListRuleGroupsNamespaces
operation.
* @return A Java Future containing the result of the ListRuleGroupsNamespaces operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListRuleGroupsNamespaces
* @see AWS
* API Documentation
*/
default CompletableFuture listRuleGroupsNamespaces(
Consumer listRuleGroupsNamespacesRequest) {
return listRuleGroupsNamespaces(ListRuleGroupsNamespacesRequest.builder().applyMutation(listRuleGroupsNamespacesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listRuleGroupsNamespaces(software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListRuleGroupsNamespacesPublisher publisher = client.listRuleGroupsNamespacesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListRuleGroupsNamespacesPublisher publisher = client.listRuleGroupsNamespacesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRuleGroupsNamespaces(software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest)}
* operation.
*
*
* @param listRuleGroupsNamespacesRequest
* Represents the input of a ListRuleGroupsNamespaces
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListRuleGroupsNamespaces
* @see AWS
* API Documentation
*/
default ListRuleGroupsNamespacesPublisher listRuleGroupsNamespacesPaginator(
ListRuleGroupsNamespacesRequest listRuleGroupsNamespacesRequest) {
return new ListRuleGroupsNamespacesPublisher(this, listRuleGroupsNamespacesRequest);
}
/**
*
* This is a variant of
* {@link #listRuleGroupsNamespaces(software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListRuleGroupsNamespacesPublisher publisher = client.listRuleGroupsNamespacesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListRuleGroupsNamespacesPublisher publisher = client.listRuleGroupsNamespacesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRuleGroupsNamespaces(software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListRuleGroupsNamespacesRequest.Builder} avoiding
* the need to create one manually via {@link ListRuleGroupsNamespacesRequest#builder()}
*
*
* @param listRuleGroupsNamespacesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListRuleGroupsNamespacesRequest.Builder} to create a
* request. Represents the input of a ListRuleGroupsNamespaces
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListRuleGroupsNamespaces
* @see AWS
* API Documentation
*/
default ListRuleGroupsNamespacesPublisher listRuleGroupsNamespacesPaginator(
Consumer listRuleGroupsNamespacesRequest) {
return listRuleGroupsNamespacesPaginator(ListRuleGroupsNamespacesRequest.builder()
.applyMutation(listRuleGroupsNamespacesRequest).build());
}
/**
*
* The ListScrapers
operation lists all of the scrapers in your account. This includes scrapers being
* created or deleted. You can optionally filter the returned list.
*
*
* @param listScrapersRequest
* Represents the input of a ListScrapers
operation.
* @return A Java Future containing the result of the ListScrapers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListScrapers
* @see AWS API
* Documentation
*/
default CompletableFuture listScrapers(ListScrapersRequest listScrapersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The ListScrapers
operation lists all of the scrapers in your account. This includes scrapers being
* created or deleted. You can optionally filter the returned list.
*
*
*
* This is a convenience which creates an instance of the {@link ListScrapersRequest.Builder} avoiding the need to
* create one manually via {@link ListScrapersRequest#builder()}
*
*
* @param listScrapersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListScrapersRequest.Builder} to create a request.
* Represents the input of a ListScrapers
operation.
* @return A Java Future containing the result of the ListScrapers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListScrapers
* @see AWS API
* Documentation
*/
default CompletableFuture listScrapers(Consumer listScrapersRequest) {
return listScrapers(ListScrapersRequest.builder().applyMutation(listScrapersRequest).build());
}
/**
*
* This is a variant of {@link #listScrapers(software.amazon.awssdk.services.amp.model.ListScrapersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListScrapersPublisher publisher = client.listScrapersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListScrapersPublisher publisher = client.listScrapersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.amp.model.ListScrapersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listScrapers(software.amazon.awssdk.services.amp.model.ListScrapersRequest)} operation.
*
*
* @param listScrapersRequest
* Represents the input of a ListScrapers
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListScrapers
* @see AWS API
* Documentation
*/
default ListScrapersPublisher listScrapersPaginator(ListScrapersRequest listScrapersRequest) {
return new ListScrapersPublisher(this, listScrapersRequest);
}
/**
*
* This is a variant of {@link #listScrapers(software.amazon.awssdk.services.amp.model.ListScrapersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListScrapersPublisher publisher = client.listScrapersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListScrapersPublisher publisher = client.listScrapersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.amp.model.ListScrapersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listScrapers(software.amazon.awssdk.services.amp.model.ListScrapersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListScrapersRequest.Builder} avoiding the need to
* create one manually via {@link ListScrapersRequest#builder()}
*
*
* @param listScrapersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListScrapersRequest.Builder} to create a request.
* Represents the input of a ListScrapers
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListScrapers
* @see AWS API
* Documentation
*/
default ListScrapersPublisher listScrapersPaginator(Consumer listScrapersRequest) {
return listScrapersPaginator(ListScrapersRequest.builder().applyMutation(listScrapersRequest).build());
}
/**
*
* The ListTagsForResource
operation returns the tags that are associated with an Amazon Managed
* Service for Prometheus resource. Currently, the only resources that can be tagged are scrapers, workspaces, and
* rule groups namespaces.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The ListTagsForResource
operation returns the tags that are associated with an Amazon Managed
* Service for Prometheus resource. Currently, the only resources that can be tagged are scrapers, workspaces, and
* rule groups namespaces.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListTagsForResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Lists all of the Amazon Managed Service for Prometheus workspaces in your account. This includes workspaces being
* created or deleted.
*
*
* @param listWorkspacesRequest
* Represents the input of a ListWorkspaces
operation.
* @return A Java Future containing the result of the ListWorkspaces operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListWorkspaces
* @see AWS API
* Documentation
*/
default CompletableFuture listWorkspaces(ListWorkspacesRequest listWorkspacesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the Amazon Managed Service for Prometheus workspaces in your account. This includes workspaces being
* created or deleted.
*
*
*
* This is a convenience which creates an instance of the {@link ListWorkspacesRequest.Builder} avoiding the need to
* create one manually via {@link ListWorkspacesRequest#builder()}
*
*
* @param listWorkspacesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListWorkspacesRequest.Builder} to create a request.
* Represents the input of a ListWorkspaces
operation.
* @return A Java Future containing the result of the ListWorkspaces operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListWorkspaces
* @see AWS API
* Documentation
*/
default CompletableFuture listWorkspaces(Consumer listWorkspacesRequest) {
return listWorkspaces(ListWorkspacesRequest.builder().applyMutation(listWorkspacesRequest).build());
}
/**
*
* This is a variant of {@link #listWorkspaces(software.amazon.awssdk.services.amp.model.ListWorkspacesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.amp.model.ListWorkspacesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listWorkspaces(software.amazon.awssdk.services.amp.model.ListWorkspacesRequest)} operation.
*
*
* @param listWorkspacesRequest
* Represents the input of a ListWorkspaces
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListWorkspaces
* @see AWS API
* Documentation
*/
default ListWorkspacesPublisher listWorkspacesPaginator(ListWorkspacesRequest listWorkspacesRequest) {
return new ListWorkspacesPublisher(this, listWorkspacesRequest);
}
/**
*
* This is a variant of {@link #listWorkspaces(software.amazon.awssdk.services.amp.model.ListWorkspacesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.amp.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.amp.model.ListWorkspacesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listWorkspaces(software.amazon.awssdk.services.amp.model.ListWorkspacesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListWorkspacesRequest.Builder} avoiding the need to
* create one manually via {@link ListWorkspacesRequest#builder()}
*
*
* @param listWorkspacesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.ListWorkspacesRequest.Builder} to create a request.
* Represents the input of a ListWorkspaces
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.ListWorkspaces
* @see AWS API
* Documentation
*/
default ListWorkspacesPublisher listWorkspacesPaginator(Consumer listWorkspacesRequest) {
return listWorkspacesPaginator(ListWorkspacesRequest.builder().applyMutation(listWorkspacesRequest).build());
}
/**
*
* Updates an existing alert manager definition in a workspace. If the workspace does not already have an alert
* manager definition, don't use this operation to create it. Instead, use CreateAlertManagerDefinition
* .
*
*
* @param putAlertManagerDefinitionRequest
* Represents the input of a PutAlertManagerDefinition
operation.
* @return A Java Future containing the result of the PutAlertManagerDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.PutAlertManagerDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture putAlertManagerDefinition(
PutAlertManagerDefinitionRequest putAlertManagerDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing alert manager definition in a workspace. If the workspace does not already have an alert
* manager definition, don't use this operation to create it. Instead, use CreateAlertManagerDefinition
* .
*
*
*
* This is a convenience which creates an instance of the {@link PutAlertManagerDefinitionRequest.Builder} avoiding
* the need to create one manually via {@link PutAlertManagerDefinitionRequest#builder()}
*
*
* @param putAlertManagerDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.PutAlertManagerDefinitionRequest.Builder} to create a
* request. Represents the input of a PutAlertManagerDefinition
operation.
* @return A Java Future containing the result of the PutAlertManagerDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.PutAlertManagerDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture putAlertManagerDefinition(
Consumer putAlertManagerDefinitionRequest) {
return putAlertManagerDefinition(PutAlertManagerDefinitionRequest.builder()
.applyMutation(putAlertManagerDefinitionRequest).build());
}
/**
*
* Updates an existing rule groups namespace within a workspace. A rule groups namespace is associated with exactly
* one rules file. A workspace can have multiple rule groups namespaces.
*
*
* Use this operation only to update existing rule groups namespaces. To create a new rule groups namespace, use
* CreateRuleGroupsNamespace
.
*
*
* You can't use this operation to add tags to an existing rule groups namespace. Instead, use
* TagResource
.
*
*
* @param putRuleGroupsNamespaceRequest
* Represents the input of a PutRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the PutRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.PutRuleGroupsNamespace
* @see AWS
* API Documentation
*/
default CompletableFuture putRuleGroupsNamespace(
PutRuleGroupsNamespaceRequest putRuleGroupsNamespaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing rule groups namespace within a workspace. A rule groups namespace is associated with exactly
* one rules file. A workspace can have multiple rule groups namespaces.
*
*
* Use this operation only to update existing rule groups namespaces. To create a new rule groups namespace, use
* CreateRuleGroupsNamespace
.
*
*
* You can't use this operation to add tags to an existing rule groups namespace. Instead, use
* TagResource
.
*
*
*
* This is a convenience which creates an instance of the {@link PutRuleGroupsNamespaceRequest.Builder} avoiding the
* need to create one manually via {@link PutRuleGroupsNamespaceRequest#builder()}
*
*
* @param putRuleGroupsNamespaceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.PutRuleGroupsNamespaceRequest.Builder} to create a
* request. Represents the input of a PutRuleGroupsNamespace
operation.
* @return A Java Future containing the result of the PutRuleGroupsNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.PutRuleGroupsNamespace
* @see AWS
* API Documentation
*/
default CompletableFuture putRuleGroupsNamespace(
Consumer putRuleGroupsNamespaceRequest) {
return putRuleGroupsNamespace(PutRuleGroupsNamespaceRequest.builder().applyMutation(putRuleGroupsNamespaceRequest)
.build());
}
/**
*
* The TagResource
operation associates tags with an Amazon Managed Service for Prometheus resource.
* The only resources that can be tagged are rule groups namespaces, scrapers, and workspaces.
*
*
* If you specify a new tag key for the resource, this tag is appended to the list of tags associated with the
* resource. If you specify a tag key that is already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag. To remove a tag, use UntagResource
.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The TagResource
operation associates tags with an Amazon Managed Service for Prometheus resource.
* The only resources that can be tagged are rule groups namespaces, scrapers, and workspaces.
*
*
* If you specify a new tag key for the resource, this tag is appended to the list of tags associated with the
* resource. If you specify a tag key that is already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag. To remove a tag, use UntagResource
.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes the specified tags from an Amazon Managed Service for Prometheus resource. The only resources that can be
* tagged are rule groups namespaces, scrapers, and workspaces.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from an Amazon Managed Service for Prometheus resource. The only resources that can be
* tagged are rule groups namespaces, scrapers, and workspaces.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the log group ARN or the workspace ID of the current logging configuration.
*
*
* @param updateLoggingConfigurationRequest
* Represents the input of an UpdateLoggingConfiguration
operation.
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture updateLoggingConfiguration(
UpdateLoggingConfigurationRequest updateLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the log group ARN or the workspace ID of the current logging configuration.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link UpdateLoggingConfigurationRequest#builder()}
*
*
* @param updateLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.UpdateLoggingConfigurationRequest.Builder} to create a
* request. Represents the input of an UpdateLoggingConfiguration
operation.
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture updateLoggingConfiguration(
Consumer updateLoggingConfigurationRequest) {
return updateLoggingConfiguration(UpdateLoggingConfigurationRequest.builder()
.applyMutation(updateLoggingConfigurationRequest).build());
}
/**
*
* Updates an existing scraper.
*
*
* You can't use this function to update the source from which the scraper is collecting metrics. To change the
* source, delete the scraper and create a new one.
*
*
* @param updateScraperRequest
* @return A Java Future containing the result of the UpdateScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UpdateScraper
* @see AWS API
* Documentation
*/
default CompletableFuture updateScraper(UpdateScraperRequest updateScraperRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing scraper.
*
*
* You can't use this function to update the source from which the scraper is collecting metrics. To change the
* source, delete the scraper and create a new one.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateScraperRequest.Builder} avoiding the need to
* create one manually via {@link UpdateScraperRequest#builder()}
*
*
* @param updateScraperRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.UpdateScraperRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateScraper operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UpdateScraper
* @see AWS API
* Documentation
*/
default CompletableFuture updateScraper(Consumer updateScraperRequest) {
return updateScraper(UpdateScraperRequest.builder().applyMutation(updateScraperRequest).build());
}
/**
*
* Updates the alias of an existing workspace.
*
*
* @param updateWorkspaceAliasRequest
* Represents the input of an UpdateWorkspaceAlias
operation.
* @return A Java Future containing the result of the UpdateWorkspaceAlias operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UpdateWorkspaceAlias
* @see AWS API
* Documentation
*/
default CompletableFuture updateWorkspaceAlias(
UpdateWorkspaceAliasRequest updateWorkspaceAliasRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the alias of an existing workspace.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateWorkspaceAliasRequest.Builder} avoiding the
* need to create one manually via {@link UpdateWorkspaceAliasRequest#builder()}
*
*
* @param updateWorkspaceAliasRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.amp.model.UpdateWorkspaceAliasRequest.Builder} to create a request.
* Represents the input of an UpdateWorkspaceAlias
operation.
* @return A Java Future containing the result of the UpdateWorkspaceAlias operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The request would cause an inconsistent state.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The request references a resources that doesn't exist.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - ServiceQuotaExceededException Completing the request would cause a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AmpException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample AmpAsyncClient.UpdateWorkspaceAlias
* @see AWS API
* Documentation
*/
default CompletableFuture updateWorkspaceAlias(
Consumer updateWorkspaceAliasRequest) {
return updateWorkspaceAlias(UpdateWorkspaceAliasRequest.builder().applyMutation(updateWorkspaceAliasRequest).build());
}
/**
* Create an instance of {@link AmpAsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link AmpAsyncWaiter}
*/
default AmpAsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
@Override
default AmpServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link AmpAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static AmpAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link AmpAsyncClient}.
*/
static AmpAsyncClientBuilder builder() {
return new DefaultAmpAsyncClientBuilder();
}
}