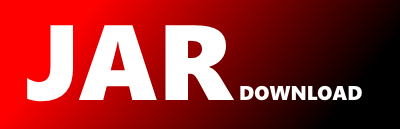
software.amazon.awssdk.services.appconfig.model.DeploymentStrategy Maven / Gradle / Ivy
Show all versions of appconfig Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appconfig.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeploymentStrategy implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(DeploymentStrategy::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(DeploymentStrategy::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(DeploymentStrategy::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField DEPLOYMENT_DURATION_IN_MINUTES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("DeploymentDurationInMinutes")
.getter(getter(DeploymentStrategy::deploymentDurationInMinutes))
.setter(setter(Builder::deploymentDurationInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentDurationInMinutes")
.build()).build();
private static final SdkField GROWTH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GrowthType").getter(getter(DeploymentStrategy::growthTypeAsString)).setter(setter(Builder::growthType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrowthType").build()).build();
private static final SdkField GROWTH_FACTOR_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("GrowthFactor").getter(getter(DeploymentStrategy::growthFactor)).setter(setter(Builder::growthFactor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrowthFactor").build()).build();
private static final SdkField FINAL_BAKE_TIME_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("FinalBakeTimeInMinutes").getter(getter(DeploymentStrategy::finalBakeTimeInMinutes))
.setter(setter(Builder::finalBakeTimeInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FinalBakeTimeInMinutes").build())
.build();
private static final SdkField REPLICATE_TO_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicateTo").getter(getter(DeploymentStrategy::replicateToAsString))
.setter(setter(Builder::replicateTo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicateTo").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
DESCRIPTION_FIELD, DEPLOYMENT_DURATION_IN_MINUTES_FIELD, GROWTH_TYPE_FIELD, GROWTH_FACTOR_FIELD,
FINAL_BAKE_TIME_IN_MINUTES_FIELD, REPLICATE_TO_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String name;
private final String description;
private final Integer deploymentDurationInMinutes;
private final String growthType;
private final Float growthFactor;
private final Integer finalBakeTimeInMinutes;
private final String replicateTo;
private DeploymentStrategy(BuilderImpl builder) {
this.id = builder.id;
this.name = builder.name;
this.description = builder.description;
this.deploymentDurationInMinutes = builder.deploymentDurationInMinutes;
this.growthType = builder.growthType;
this.growthFactor = builder.growthFactor;
this.finalBakeTimeInMinutes = builder.finalBakeTimeInMinutes;
this.replicateTo = builder.replicateTo;
}
/**
*
* The deployment strategy ID.
*
*
* @return The deployment strategy ID.
*/
public final String id() {
return id;
}
/**
*
* The name of the deployment strategy.
*
*
* @return The name of the deployment strategy.
*/
public final String name() {
return name;
}
/**
*
* The description of the deployment strategy.
*
*
* @return The description of the deployment strategy.
*/
public final String description() {
return description;
}
/**
*
* Total amount of time the deployment lasted.
*
*
* @return Total amount of time the deployment lasted.
*/
public final Integer deploymentDurationInMinutes() {
return deploymentDurationInMinutes;
}
/**
*
* The algorithm used to define how percentage grew over time.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #growthType} will
* return {@link GrowthType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #growthTypeAsString}.
*
*
* @return The algorithm used to define how percentage grew over time.
* @see GrowthType
*/
public final GrowthType growthType() {
return GrowthType.fromValue(growthType);
}
/**
*
* The algorithm used to define how percentage grew over time.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #growthType} will
* return {@link GrowthType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #growthTypeAsString}.
*
*
* @return The algorithm used to define how percentage grew over time.
* @see GrowthType
*/
public final String growthTypeAsString() {
return growthType;
}
/**
*
* The percentage of targets that received a deployed configuration during each interval.
*
*
* @return The percentage of targets that received a deployed configuration during each interval.
*/
public final Float growthFactor() {
return growthFactor;
}
/**
*
* The amount of time AppConfig monitored for alarms before considering the deployment to be complete and no longer
* eligible for automatic roll back.
*
*
* @return The amount of time AppConfig monitored for alarms before considering the deployment to be complete and no
* longer eligible for automatic roll back.
*/
public final Integer finalBakeTimeInMinutes() {
return finalBakeTimeInMinutes;
}
/**
*
* Save the deployment strategy to a Systems Manager (SSM) document.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replicateTo} will
* return {@link ReplicateTo#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #replicateToAsString}.
*
*
* @return Save the deployment strategy to a Systems Manager (SSM) document.
* @see ReplicateTo
*/
public final ReplicateTo replicateTo() {
return ReplicateTo.fromValue(replicateTo);
}
/**
*
* Save the deployment strategy to a Systems Manager (SSM) document.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replicateTo} will
* return {@link ReplicateTo#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #replicateToAsString}.
*
*
* @return Save the deployment strategy to a Systems Manager (SSM) document.
* @see ReplicateTo
*/
public final String replicateToAsString() {
return replicateTo;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(deploymentDurationInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(growthTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(growthFactor());
hashCode = 31 * hashCode + Objects.hashCode(finalBakeTimeInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(replicateToAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeploymentStrategy)) {
return false;
}
DeploymentStrategy other = (DeploymentStrategy) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name())
&& Objects.equals(description(), other.description())
&& Objects.equals(deploymentDurationInMinutes(), other.deploymentDurationInMinutes())
&& Objects.equals(growthTypeAsString(), other.growthTypeAsString())
&& Objects.equals(growthFactor(), other.growthFactor())
&& Objects.equals(finalBakeTimeInMinutes(), other.finalBakeTimeInMinutes())
&& Objects.equals(replicateToAsString(), other.replicateToAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeploymentStrategy").add("Id", id()).add("Name", name()).add("Description", description())
.add("DeploymentDurationInMinutes", deploymentDurationInMinutes()).add("GrowthType", growthTypeAsString())
.add("GrowthFactor", growthFactor()).add("FinalBakeTimeInMinutes", finalBakeTimeInMinutes())
.add("ReplicateTo", replicateToAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "DeploymentDurationInMinutes":
return Optional.ofNullable(clazz.cast(deploymentDurationInMinutes()));
case "GrowthType":
return Optional.ofNullable(clazz.cast(growthTypeAsString()));
case "GrowthFactor":
return Optional.ofNullable(clazz.cast(growthFactor()));
case "FinalBakeTimeInMinutes":
return Optional.ofNullable(clazz.cast(finalBakeTimeInMinutes()));
case "ReplicateTo":
return Optional.ofNullable(clazz.cast(replicateToAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function