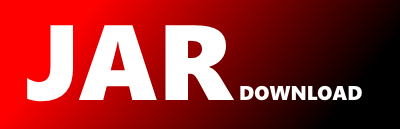
software.amazon.awssdk.services.appconfig.model.GetHostedConfigurationVersionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig Show documentation
Show all versions of appconfig Show documentation
The AWS Java SDK for AppConfig module holds the client classes that are used for
communicating with AppConfig.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appconfig.model;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetHostedConfigurationVersionResponse extends AppConfigResponse implements
ToCopyableBuilder {
private static final SdkField APPLICATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationId").getter(getter(GetHostedConfigurationVersionResponse::applicationId))
.setter(setter(Builder::applicationId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Application-Id").build()).build();
private static final SdkField CONFIGURATION_PROFILE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationProfileId").getter(getter(GetHostedConfigurationVersionResponse::configurationProfileId))
.setter(setter(Builder::configurationProfileId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Configuration-Profile-Id").build())
.build();
private static final SdkField VERSION_NUMBER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("VersionNumber").getter(getter(GetHostedConfigurationVersionResponse::versionNumber))
.setter(setter(Builder::versionNumber))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Version-Number").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(GetHostedConfigurationVersionResponse::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Description").build()).build();
private static final SdkField CONTENT_FIELD = SdkField
. builder(MarshallingType.SDK_BYTES)
.memberName("Content")
.getter(getter(GetHostedConfigurationVersionResponse::content))
.setter(setter(Builder::content))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Content").build(),
PayloadTrait.create()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ContentType").getter(getter(GetHostedConfigurationVersionResponse::contentType))
.setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Type").build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(GetHostedConfigurationVersionResponse::versionLabel))
.setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("VersionLabel").build()).build();
private static final SdkField KMS_KEY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyArn").getter(getter(GetHostedConfigurationVersionResponse::kmsKeyArn))
.setter(setter(Builder::kmsKeyArn))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("KmsKeyArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_ID_FIELD,
CONFIGURATION_PROFILE_ID_FIELD, VERSION_NUMBER_FIELD, DESCRIPTION_FIELD, CONTENT_FIELD, CONTENT_TYPE_FIELD,
VERSION_LABEL_FIELD, KMS_KEY_ARN_FIELD));
private final String applicationId;
private final String configurationProfileId;
private final Integer versionNumber;
private final String description;
private final SdkBytes content;
private final String contentType;
private final String versionLabel;
private final String kmsKeyArn;
private GetHostedConfigurationVersionResponse(BuilderImpl builder) {
super(builder);
this.applicationId = builder.applicationId;
this.configurationProfileId = builder.configurationProfileId;
this.versionNumber = builder.versionNumber;
this.description = builder.description;
this.content = builder.content;
this.contentType = builder.contentType;
this.versionLabel = builder.versionLabel;
this.kmsKeyArn = builder.kmsKeyArn;
}
/**
*
* The application ID.
*
*
* @return The application ID.
*/
public final String applicationId() {
return applicationId;
}
/**
*
* The configuration profile ID.
*
*
* @return The configuration profile ID.
*/
public final String configurationProfileId() {
return configurationProfileId;
}
/**
*
* The configuration version.
*
*
* @return The configuration version.
*/
public final Integer versionNumber() {
return versionNumber;
}
/**
*
* A description of the configuration.
*
*
* @return A description of the configuration.
*/
public final String description() {
return description;
}
/**
*
* The content of the configuration or the configuration data.
*
*
* @return The content of the configuration or the configuration data.
*/
public final SdkBytes content() {
return content;
}
/**
*
* A standard MIME type describing the format of the configuration content. For more information, see Content-Type.
*
*
* @return A standard MIME type describing the format of the configuration content. For more information, see Content-Type.
*/
public final String contentType() {
return contentType;
}
/**
*
* A user-defined label for an AppConfig hosted configuration version.
*
*
* @return A user-defined label for an AppConfig hosted configuration version.
*/
public final String versionLabel() {
return versionLabel;
}
/**
*
* The Amazon Resource Name of the Key Management Service key that was used to encrypt this specific version of the
* configuration data in the AppConfig hosted configuration store.
*
*
* @return The Amazon Resource Name of the Key Management Service key that was used to encrypt this specific version
* of the configuration data in the AppConfig hosted configuration store.
*/
public final String kmsKeyArn() {
return kmsKeyArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(configurationProfileId());
hashCode = 31 * hashCode + Objects.hashCode(versionNumber());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(content());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetHostedConfigurationVersionResponse)) {
return false;
}
GetHostedConfigurationVersionResponse other = (GetHostedConfigurationVersionResponse) obj;
return Objects.equals(applicationId(), other.applicationId())
&& Objects.equals(configurationProfileId(), other.configurationProfileId())
&& Objects.equals(versionNumber(), other.versionNumber()) && Objects.equals(description(), other.description())
&& Objects.equals(content(), other.content()) && Objects.equals(contentType(), other.contentType())
&& Objects.equals(versionLabel(), other.versionLabel()) && Objects.equals(kmsKeyArn(), other.kmsKeyArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetHostedConfigurationVersionResponse").add("ApplicationId", applicationId())
.add("ConfigurationProfileId", configurationProfileId()).add("VersionNumber", versionNumber())
.add("Description", description()).add("Content", content() == null ? null : "*** Sensitive Data Redacted ***")
.add("ContentType", contentType()).add("VersionLabel", versionLabel()).add("KmsKeyArn", kmsKeyArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationId":
return Optional.ofNullable(clazz.cast(applicationId()));
case "ConfigurationProfileId":
return Optional.ofNullable(clazz.cast(configurationProfileId()));
case "VersionNumber":
return Optional.ofNullable(clazz.cast(versionNumber()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Content":
return Optional.ofNullable(clazz.cast(content()));
case "ContentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
case "KmsKeyArn":
return Optional.ofNullable(clazz.cast(kmsKeyArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy