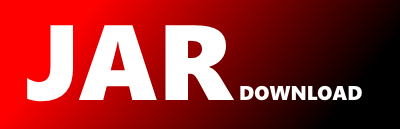
software.amazon.awssdk.services.appconfig.model.StartDeploymentResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appconfig.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartDeploymentResponse extends AppConfigResponse implements
ToCopyableBuilder {
private static final SdkField APPLICATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationId").getter(getter(StartDeploymentResponse::applicationId))
.setter(setter(Builder::applicationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationId").build()).build();
private static final SdkField ENVIRONMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentId").getter(getter(StartDeploymentResponse::environmentId))
.setter(setter(Builder::environmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentId").build()).build();
private static final SdkField DEPLOYMENT_STRATEGY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DeploymentStrategyId").getter(getter(StartDeploymentResponse::deploymentStrategyId))
.setter(setter(Builder::deploymentStrategyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentStrategyId").build())
.build();
private static final SdkField CONFIGURATION_PROFILE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationProfileId").getter(getter(StartDeploymentResponse::configurationProfileId))
.setter(setter(Builder::configurationProfileId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationProfileId").build())
.build();
private static final SdkField DEPLOYMENT_NUMBER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("DeploymentNumber").getter(getter(StartDeploymentResponse::deploymentNumber))
.setter(setter(Builder::deploymentNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentNumber").build()).build();
private static final SdkField CONFIGURATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationName").getter(getter(StartDeploymentResponse::configurationName))
.setter(setter(Builder::configurationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationName").build()).build();
private static final SdkField CONFIGURATION_LOCATION_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationLocationUri").getter(getter(StartDeploymentResponse::configurationLocationUri))
.setter(setter(Builder::configurationLocationUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationLocationUri").build())
.build();
private static final SdkField CONFIGURATION_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationVersion").getter(getter(StartDeploymentResponse::configurationVersion))
.setter(setter(Builder::configurationVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationVersion").build())
.build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(StartDeploymentResponse::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField DEPLOYMENT_DURATION_IN_MINUTES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("DeploymentDurationInMinutes")
.getter(getter(StartDeploymentResponse::deploymentDurationInMinutes))
.setter(setter(Builder::deploymentDurationInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentDurationInMinutes")
.build()).build();
private static final SdkField GROWTH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GrowthType").getter(getter(StartDeploymentResponse::growthTypeAsString))
.setter(setter(Builder::growthType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrowthType").build()).build();
private static final SdkField GROWTH_FACTOR_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("GrowthFactor").getter(getter(StartDeploymentResponse::growthFactor))
.setter(setter(Builder::growthFactor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrowthFactor").build()).build();
private static final SdkField FINAL_BAKE_TIME_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("FinalBakeTimeInMinutes").getter(getter(StartDeploymentResponse::finalBakeTimeInMinutes))
.setter(setter(Builder::finalBakeTimeInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FinalBakeTimeInMinutes").build())
.build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(StartDeploymentResponse::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField> EVENT_LOG_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EventLog")
.getter(getter(StartDeploymentResponse::eventLog))
.setter(setter(Builder::eventLog))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventLog").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DeploymentEvent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PERCENTAGE_COMPLETE_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("PercentageComplete").getter(getter(StartDeploymentResponse::percentageComplete))
.setter(setter(Builder::percentageComplete))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentageComplete").build())
.build();
private static final SdkField STARTED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("StartedAt")
.getter(getter(StartDeploymentResponse::startedAt))
.setter(setter(Builder::startedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField COMPLETED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("CompletedAt")
.getter(getter(StartDeploymentResponse::completedAt))
.setter(setter(Builder::completedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField> APPLIED_EXTENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AppliedExtensions")
.getter(getter(StartDeploymentResponse::appliedExtensions))
.setter(setter(Builder::appliedExtensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppliedExtensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AppliedExtension::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField KMS_KEY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyArn").getter(getter(StartDeploymentResponse::kmsKeyArn)).setter(setter(Builder::kmsKeyArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyArn").build()).build();
private static final SdkField KMS_KEY_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyIdentifier").getter(getter(StartDeploymentResponse::kmsKeyIdentifier))
.setter(setter(Builder::kmsKeyIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyIdentifier").build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(StartDeploymentResponse::versionLabel))
.setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionLabel").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_ID_FIELD,
ENVIRONMENT_ID_FIELD, DEPLOYMENT_STRATEGY_ID_FIELD, CONFIGURATION_PROFILE_ID_FIELD, DEPLOYMENT_NUMBER_FIELD,
CONFIGURATION_NAME_FIELD, CONFIGURATION_LOCATION_URI_FIELD, CONFIGURATION_VERSION_FIELD, DESCRIPTION_FIELD,
DEPLOYMENT_DURATION_IN_MINUTES_FIELD, GROWTH_TYPE_FIELD, GROWTH_FACTOR_FIELD, FINAL_BAKE_TIME_IN_MINUTES_FIELD,
STATE_FIELD, EVENT_LOG_FIELD, PERCENTAGE_COMPLETE_FIELD, STARTED_AT_FIELD, COMPLETED_AT_FIELD,
APPLIED_EXTENSIONS_FIELD, KMS_KEY_ARN_FIELD, KMS_KEY_IDENTIFIER_FIELD, VERSION_LABEL_FIELD));
private final String applicationId;
private final String environmentId;
private final String deploymentStrategyId;
private final String configurationProfileId;
private final Integer deploymentNumber;
private final String configurationName;
private final String configurationLocationUri;
private final String configurationVersion;
private final String description;
private final Integer deploymentDurationInMinutes;
private final String growthType;
private final Float growthFactor;
private final Integer finalBakeTimeInMinutes;
private final String state;
private final List eventLog;
private final Float percentageComplete;
private final Instant startedAt;
private final Instant completedAt;
private final List appliedExtensions;
private final String kmsKeyArn;
private final String kmsKeyIdentifier;
private final String versionLabel;
private StartDeploymentResponse(BuilderImpl builder) {
super(builder);
this.applicationId = builder.applicationId;
this.environmentId = builder.environmentId;
this.deploymentStrategyId = builder.deploymentStrategyId;
this.configurationProfileId = builder.configurationProfileId;
this.deploymentNumber = builder.deploymentNumber;
this.configurationName = builder.configurationName;
this.configurationLocationUri = builder.configurationLocationUri;
this.configurationVersion = builder.configurationVersion;
this.description = builder.description;
this.deploymentDurationInMinutes = builder.deploymentDurationInMinutes;
this.growthType = builder.growthType;
this.growthFactor = builder.growthFactor;
this.finalBakeTimeInMinutes = builder.finalBakeTimeInMinutes;
this.state = builder.state;
this.eventLog = builder.eventLog;
this.percentageComplete = builder.percentageComplete;
this.startedAt = builder.startedAt;
this.completedAt = builder.completedAt;
this.appliedExtensions = builder.appliedExtensions;
this.kmsKeyArn = builder.kmsKeyArn;
this.kmsKeyIdentifier = builder.kmsKeyIdentifier;
this.versionLabel = builder.versionLabel;
}
/**
*
* The ID of the application that was deployed.
*
*
* @return The ID of the application that was deployed.
*/
public final String applicationId() {
return applicationId;
}
/**
*
* The ID of the environment that was deployed.
*
*
* @return The ID of the environment that was deployed.
*/
public final String environmentId() {
return environmentId;
}
/**
*
* The ID of the deployment strategy that was deployed.
*
*
* @return The ID of the deployment strategy that was deployed.
*/
public final String deploymentStrategyId() {
return deploymentStrategyId;
}
/**
*
* The ID of the configuration profile that was deployed.
*
*
* @return The ID of the configuration profile that was deployed.
*/
public final String configurationProfileId() {
return configurationProfileId;
}
/**
*
* The sequence number of the deployment.
*
*
* @return The sequence number of the deployment.
*/
public final Integer deploymentNumber() {
return deploymentNumber;
}
/**
*
* The name of the configuration.
*
*
* @return The name of the configuration.
*/
public final String configurationName() {
return configurationName;
}
/**
*
* Information about the source location of the configuration.
*
*
* @return Information about the source location of the configuration.
*/
public final String configurationLocationUri() {
return configurationLocationUri;
}
/**
*
* The configuration version that was deployed.
*
*
* @return The configuration version that was deployed.
*/
public final String configurationVersion() {
return configurationVersion;
}
/**
*
* The description of the deployment.
*
*
* @return The description of the deployment.
*/
public final String description() {
return description;
}
/**
*
* Total amount of time the deployment lasted.
*
*
* @return Total amount of time the deployment lasted.
*/
public final Integer deploymentDurationInMinutes() {
return deploymentDurationInMinutes;
}
/**
*
* The algorithm used to define how percentage grew over time.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #growthType} will
* return {@link GrowthType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #growthTypeAsString}.
*
*
* @return The algorithm used to define how percentage grew over time.
* @see GrowthType
*/
public final GrowthType growthType() {
return GrowthType.fromValue(growthType);
}
/**
*
* The algorithm used to define how percentage grew over time.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #growthType} will
* return {@link GrowthType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #growthTypeAsString}.
*
*
* @return The algorithm used to define how percentage grew over time.
* @see GrowthType
*/
public final String growthTypeAsString() {
return growthType;
}
/**
*
* The percentage of targets to receive a deployed configuration during each interval.
*
*
* @return The percentage of targets to receive a deployed configuration during each interval.
*/
public final Float growthFactor() {
return growthFactor;
}
/**
*
* The amount of time that AppConfig monitored for alarms before considering the deployment to be complete and no
* longer eligible for automatic rollback.
*
*
* @return The amount of time that AppConfig monitored for alarms before considering the deployment to be complete
* and no longer eligible for automatic rollback.
*/
public final Integer finalBakeTimeInMinutes() {
return finalBakeTimeInMinutes;
}
/**
*
* The state of the deployment.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link DeploymentState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the deployment.
* @see DeploymentState
*/
public final DeploymentState state() {
return DeploymentState.fromValue(state);
}
/**
*
* The state of the deployment.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link DeploymentState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the deployment.
* @see DeploymentState
*/
public final String stateAsString() {
return state;
}
/**
* For responses, this returns true if the service returned a value for the EventLog property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasEventLog() {
return eventLog != null && !(eventLog instanceof SdkAutoConstructList);
}
/**
*
* A list containing all events related to a deployment. The most recent events are displayed first.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEventLog} method.
*
*
* @return A list containing all events related to a deployment. The most recent events are displayed first.
*/
public final List eventLog() {
return eventLog;
}
/**
*
* The percentage of targets for which the deployment is available.
*
*
* @return The percentage of targets for which the deployment is available.
*/
public final Float percentageComplete() {
return percentageComplete;
}
/**
*
* The time the deployment started.
*
*
* @return The time the deployment started.
*/
public final Instant startedAt() {
return startedAt;
}
/**
*
* The time the deployment completed.
*
*
* @return The time the deployment completed.
*/
public final Instant completedAt() {
return completedAt;
}
/**
* For responses, this returns true if the service returned a value for the AppliedExtensions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAppliedExtensions() {
return appliedExtensions != null && !(appliedExtensions instanceof SdkAutoConstructList);
}
/**
*
* A list of extensions that were processed as part of the deployment. The extensions that were previously
* associated to the configuration profile, environment, or the application when StartDeployment
was
* called.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAppliedExtensions} method.
*
*
* @return A list of extensions that were processed as part of the deployment. The extensions that were previously
* associated to the configuration profile, environment, or the application when
* StartDeployment
was called.
*/
public final List appliedExtensions() {
return appliedExtensions;
}
/**
*
* The Amazon Resource Name of the Key Management Service key used to encrypt configuration data. You can encrypt
* secrets stored in Secrets Manager, Amazon Simple Storage Service (Amazon S3) objects encrypted with SSE-KMS, or
* secure string parameters stored in Amazon Web Services Systems Manager Parameter Store.
*
*
* @return The Amazon Resource Name of the Key Management Service key used to encrypt configuration data. You can
* encrypt secrets stored in Secrets Manager, Amazon Simple Storage Service (Amazon S3) objects encrypted
* with SSE-KMS, or secure string parameters stored in Amazon Web Services Systems Manager Parameter Store.
*/
public final String kmsKeyArn() {
return kmsKeyArn;
}
/**
*
* The Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was created
* or updated.
*
*
* @return The Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was
* created or updated.
*/
public final String kmsKeyIdentifier() {
return kmsKeyIdentifier;
}
/**
*
* A user-defined label for an AppConfig hosted configuration version.
*
*
* @return A user-defined label for an AppConfig hosted configuration version.
*/
public final String versionLabel() {
return versionLabel;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(environmentId());
hashCode = 31 * hashCode + Objects.hashCode(deploymentStrategyId());
hashCode = 31 * hashCode + Objects.hashCode(configurationProfileId());
hashCode = 31 * hashCode + Objects.hashCode(deploymentNumber());
hashCode = 31 * hashCode + Objects.hashCode(configurationName());
hashCode = 31 * hashCode + Objects.hashCode(configurationLocationUri());
hashCode = 31 * hashCode + Objects.hashCode(configurationVersion());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(deploymentDurationInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(growthTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(growthFactor());
hashCode = 31 * hashCode + Objects.hashCode(finalBakeTimeInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasEventLog() ? eventLog() : null);
hashCode = 31 * hashCode + Objects.hashCode(percentageComplete());
hashCode = 31 * hashCode + Objects.hashCode(startedAt());
hashCode = 31 * hashCode + Objects.hashCode(completedAt());
hashCode = 31 * hashCode + Objects.hashCode(hasAppliedExtensions() ? appliedExtensions() : null);
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyArn());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartDeploymentResponse)) {
return false;
}
StartDeploymentResponse other = (StartDeploymentResponse) obj;
return Objects.equals(applicationId(), other.applicationId()) && Objects.equals(environmentId(), other.environmentId())
&& Objects.equals(deploymentStrategyId(), other.deploymentStrategyId())
&& Objects.equals(configurationProfileId(), other.configurationProfileId())
&& Objects.equals(deploymentNumber(), other.deploymentNumber())
&& Objects.equals(configurationName(), other.configurationName())
&& Objects.equals(configurationLocationUri(), other.configurationLocationUri())
&& Objects.equals(configurationVersion(), other.configurationVersion())
&& Objects.equals(description(), other.description())
&& Objects.equals(deploymentDurationInMinutes(), other.deploymentDurationInMinutes())
&& Objects.equals(growthTypeAsString(), other.growthTypeAsString())
&& Objects.equals(growthFactor(), other.growthFactor())
&& Objects.equals(finalBakeTimeInMinutes(), other.finalBakeTimeInMinutes())
&& Objects.equals(stateAsString(), other.stateAsString()) && hasEventLog() == other.hasEventLog()
&& Objects.equals(eventLog(), other.eventLog())
&& Objects.equals(percentageComplete(), other.percentageComplete())
&& Objects.equals(startedAt(), other.startedAt()) && Objects.equals(completedAt(), other.completedAt())
&& hasAppliedExtensions() == other.hasAppliedExtensions()
&& Objects.equals(appliedExtensions(), other.appliedExtensions())
&& Objects.equals(kmsKeyArn(), other.kmsKeyArn()) && Objects.equals(kmsKeyIdentifier(), other.kmsKeyIdentifier())
&& Objects.equals(versionLabel(), other.versionLabel());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartDeploymentResponse").add("ApplicationId", applicationId())
.add("EnvironmentId", environmentId()).add("DeploymentStrategyId", deploymentStrategyId())
.add("ConfigurationProfileId", configurationProfileId()).add("DeploymentNumber", deploymentNumber())
.add("ConfigurationName", configurationName()).add("ConfigurationLocationUri", configurationLocationUri())
.add("ConfigurationVersion", configurationVersion()).add("Description", description())
.add("DeploymentDurationInMinutes", deploymentDurationInMinutes()).add("GrowthType", growthTypeAsString())
.add("GrowthFactor", growthFactor()).add("FinalBakeTimeInMinutes", finalBakeTimeInMinutes())
.add("State", stateAsString()).add("EventLog", hasEventLog() ? eventLog() : null)
.add("PercentageComplete", percentageComplete()).add("StartedAt", startedAt()).add("CompletedAt", completedAt())
.add("AppliedExtensions", hasAppliedExtensions() ? appliedExtensions() : null).add("KmsKeyArn", kmsKeyArn())
.add("KmsKeyIdentifier", kmsKeyIdentifier()).add("VersionLabel", versionLabel()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationId":
return Optional.ofNullable(clazz.cast(applicationId()));
case "EnvironmentId":
return Optional.ofNullable(clazz.cast(environmentId()));
case "DeploymentStrategyId":
return Optional.ofNullable(clazz.cast(deploymentStrategyId()));
case "ConfigurationProfileId":
return Optional.ofNullable(clazz.cast(configurationProfileId()));
case "DeploymentNumber":
return Optional.ofNullable(clazz.cast(deploymentNumber()));
case "ConfigurationName":
return Optional.ofNullable(clazz.cast(configurationName()));
case "ConfigurationLocationUri":
return Optional.ofNullable(clazz.cast(configurationLocationUri()));
case "ConfigurationVersion":
return Optional.ofNullable(clazz.cast(configurationVersion()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "DeploymentDurationInMinutes":
return Optional.ofNullable(clazz.cast(deploymentDurationInMinutes()));
case "GrowthType":
return Optional.ofNullable(clazz.cast(growthTypeAsString()));
case "GrowthFactor":
return Optional.ofNullable(clazz.cast(growthFactor()));
case "FinalBakeTimeInMinutes":
return Optional.ofNullable(clazz.cast(finalBakeTimeInMinutes()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "EventLog":
return Optional.ofNullable(clazz.cast(eventLog()));
case "PercentageComplete":
return Optional.ofNullable(clazz.cast(percentageComplete()));
case "StartedAt":
return Optional.ofNullable(clazz.cast(startedAt()));
case "CompletedAt":
return Optional.ofNullable(clazz.cast(completedAt()));
case "AppliedExtensions":
return Optional.ofNullable(clazz.cast(appliedExtensions()));
case "KmsKeyArn":
return Optional.ofNullable(clazz.cast(kmsKeyArn()));
case "KmsKeyIdentifier":
return Optional.ofNullable(clazz.cast(kmsKeyIdentifier()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function