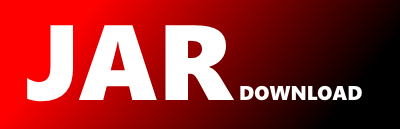
software.amazon.awssdk.services.applicationautoscaling.model.ScalingPolicy Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationautoscaling.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a scaling policy to use with Application Auto Scaling.
*
*
* For more information about configuring scaling policies for a specific service, see Amazon Web
* Services services that you can use with Application Auto Scaling in the Application Auto Scaling User
* Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ScalingPolicy implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField POLICY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyARN").getter(getter(ScalingPolicy::policyARN)).setter(setter(Builder::policyARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyARN").build()).build();
private static final SdkField POLICY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyName").getter(getter(ScalingPolicy::policyName)).setter(setter(Builder::policyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyName").build()).build();
private static final SdkField SERVICE_NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceNamespace").getter(getter(ScalingPolicy::serviceNamespaceAsString))
.setter(setter(Builder::serviceNamespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceNamespace").build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceId").getter(getter(ScalingPolicy::resourceId)).setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceId").build()).build();
private static final SdkField SCALABLE_DIMENSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ScalableDimension").getter(getter(ScalingPolicy::scalableDimensionAsString))
.setter(setter(Builder::scalableDimension))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScalableDimension").build()).build();
private static final SdkField POLICY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyType").getter(getter(ScalingPolicy::policyTypeAsString)).setter(setter(Builder::policyType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyType").build()).build();
private static final SdkField STEP_SCALING_POLICY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("StepScalingPolicyConfiguration")
.getter(getter(ScalingPolicy::stepScalingPolicyConfiguration))
.setter(setter(Builder::stepScalingPolicyConfiguration))
.constructor(StepScalingPolicyConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StepScalingPolicyConfiguration")
.build()).build();
private static final SdkField TARGET_TRACKING_SCALING_POLICY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("TargetTrackingScalingPolicyConfiguration")
.getter(getter(ScalingPolicy::targetTrackingScalingPolicyConfiguration))
.setter(setter(Builder::targetTrackingScalingPolicyConfiguration))
.constructor(TargetTrackingScalingPolicyConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("TargetTrackingScalingPolicyConfiguration").build()).build();
private static final SdkField PREDICTIVE_SCALING_POLICY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("PredictiveScalingPolicyConfiguration")
.getter(getter(ScalingPolicy::predictiveScalingPolicyConfiguration))
.setter(setter(Builder::predictiveScalingPolicyConfiguration))
.constructor(PredictiveScalingPolicyConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("PredictiveScalingPolicyConfiguration").build()).build();
private static final SdkField> ALARMS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Alarms")
.getter(getter(ScalingPolicy::alarms))
.setter(setter(Builder::alarms))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Alarms").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Alarm::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(ScalingPolicy::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POLICY_ARN_FIELD,
POLICY_NAME_FIELD, SERVICE_NAMESPACE_FIELD, RESOURCE_ID_FIELD, SCALABLE_DIMENSION_FIELD, POLICY_TYPE_FIELD,
STEP_SCALING_POLICY_CONFIGURATION_FIELD, TARGET_TRACKING_SCALING_POLICY_CONFIGURATION_FIELD,
PREDICTIVE_SCALING_POLICY_CONFIGURATION_FIELD, ALARMS_FIELD, CREATION_TIME_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("PolicyARN", POLICY_ARN_FIELD);
put("PolicyName", POLICY_NAME_FIELD);
put("ServiceNamespace", SERVICE_NAMESPACE_FIELD);
put("ResourceId", RESOURCE_ID_FIELD);
put("ScalableDimension", SCALABLE_DIMENSION_FIELD);
put("PolicyType", POLICY_TYPE_FIELD);
put("StepScalingPolicyConfiguration", STEP_SCALING_POLICY_CONFIGURATION_FIELD);
put("TargetTrackingScalingPolicyConfiguration", TARGET_TRACKING_SCALING_POLICY_CONFIGURATION_FIELD);
put("PredictiveScalingPolicyConfiguration", PREDICTIVE_SCALING_POLICY_CONFIGURATION_FIELD);
put("Alarms", ALARMS_FIELD);
put("CreationTime", CREATION_TIME_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String policyARN;
private final String policyName;
private final String serviceNamespace;
private final String resourceId;
private final String scalableDimension;
private final String policyType;
private final StepScalingPolicyConfiguration stepScalingPolicyConfiguration;
private final TargetTrackingScalingPolicyConfiguration targetTrackingScalingPolicyConfiguration;
private final PredictiveScalingPolicyConfiguration predictiveScalingPolicyConfiguration;
private final List alarms;
private final Instant creationTime;
private ScalingPolicy(BuilderImpl builder) {
this.policyARN = builder.policyARN;
this.policyName = builder.policyName;
this.serviceNamespace = builder.serviceNamespace;
this.resourceId = builder.resourceId;
this.scalableDimension = builder.scalableDimension;
this.policyType = builder.policyType;
this.stepScalingPolicyConfiguration = builder.stepScalingPolicyConfiguration;
this.targetTrackingScalingPolicyConfiguration = builder.targetTrackingScalingPolicyConfiguration;
this.predictiveScalingPolicyConfiguration = builder.predictiveScalingPolicyConfiguration;
this.alarms = builder.alarms;
this.creationTime = builder.creationTime;
}
/**
*
* The Amazon Resource Name (ARN) of the scaling policy.
*
*
* @return The Amazon Resource Name (ARN) of the scaling policy.
*/
public final String policyARN() {
return policyARN;
}
/**
*
* The name of the scaling policy.
*
*
* @return The name of the scaling policy.
*/
public final String policyName() {
return policyName;
}
/**
*
* The namespace of the Amazon Web Services service that provides the resource, or a custom-resource
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceNamespace}
* will return {@link ServiceNamespace#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceNamespaceAsString}.
*
*
* @return The namespace of the Amazon Web Services service that provides the resource, or a
* custom-resource
.
* @see ServiceNamespace
*/
public final ServiceNamespace serviceNamespace() {
return ServiceNamespace.fromValue(serviceNamespace);
}
/**
*
* The namespace of the Amazon Web Services service that provides the resource, or a custom-resource
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceNamespace}
* will return {@link ServiceNamespace#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceNamespaceAsString}.
*
*
* @return The namespace of the Amazon Web Services service that provides the resource, or a
* custom-resource
.
* @see ServiceNamespace
*/
public final String serviceNamespaceAsString() {
return serviceNamespace;
}
/**
*
* The identifier of the resource associated with the scaling policy. This string consists of the resource type and
* unique identifier.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/my-cluster/my-service
.
*
*
* -
*
* Spot Fleet - The resource type is spot-fleet-request
and the unique identifier is the Spot Fleet
* request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the table name. Example:
* table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the index
* name. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster name.
* Example: cluster:my-db-cluster
.
*
*
* -
*
* SageMaker endpoint variant - The resource type is variant
and the unique identifier is the resource
* ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the OutputValue
* from the CloudFormation template stack used to access the resources. The unique identifier is defined by the
* service provider. More information is available in our GitHub repository.
*
*
* -
*
* Amazon Comprehend document classification endpoint - The resource type and unique identifier are specified using
* the endpoint ARN. Example:
* arn:aws:comprehend:us-west-2:123456789012:document-classifier-endpoint/EXAMPLE
.
*
*
* -
*
* Amazon Comprehend entity recognizer endpoint - The resource type and unique identifier are specified using the
* endpoint ARN. Example: arn:aws:comprehend:us-west-2:123456789012:entity-recognizer-endpoint/EXAMPLE
.
*
*
* -
*
* Lambda provisioned concurrency - The resource type is function
and the unique identifier is the
* function name with a function version or alias name suffix that is not $LATEST
. Example:
* function:my-function:prod
or function:my-function:1
.
*
*
* -
*
* Amazon Keyspaces table - The resource type is table
and the unique identifier is the table name.
* Example: keyspace/mykeyspace/table/mytable
.
*
*
* -
*
* Amazon MSK cluster - The resource type and unique identifier are specified using the cluster ARN. Example:
* arn:aws:kafka:us-east-1:123456789012:cluster/demo-cluster-1/6357e0b2-0e6a-4b86-a0b4-70df934c2e31-5
.
*
*
* -
*
* Amazon ElastiCache replication group - The resource type is replication-group
and the unique
* identifier is the replication group name. Example: replication-group/mycluster
.
*
*
* -
*
* Neptune cluster - The resource type is cluster
and the unique identifier is the cluster name.
* Example: cluster:mycluster
.
*
*
* -
*
* SageMaker serverless endpoint - The resource type is variant
and the unique identifier is the
* resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* SageMaker inference component - The resource type is inference-component
and the unique identifier
* is the resource ID. Example: inference-component/my-inference-component
.
*
*
* -
*
* Pool of WorkSpaces - The resource type is workspacespool
and the unique identifier is the pool ID.
* Example: workspacespool/wspool-123456
.
*
*
*
*
* @return The identifier of the resource associated with the scaling policy. This string consists of the resource
* type and unique identifier.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/my-cluster/my-service
.
*
*
* -
*
* Spot Fleet - The resource type is spot-fleet-request
and the unique identifier is the Spot
* Fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet
* name. Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the table name.
* Example: table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is
* the index name. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster
* name. Example: cluster:my-db-cluster
.
*
*
* -
*
* SageMaker endpoint variant - The resource type is variant
and the unique identifier is the
* resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the
* OutputValue
from the CloudFormation template stack used to access the resources. The unique
* identifier is defined by the service provider. More information is available in our GitHub repository.
*
*
* -
*
* Amazon Comprehend document classification endpoint - The resource type and unique identifier are
* specified using the endpoint ARN. Example:
* arn:aws:comprehend:us-west-2:123456789012:document-classifier-endpoint/EXAMPLE
.
*
*
* -
*
* Amazon Comprehend entity recognizer endpoint - The resource type and unique identifier are specified
* using the endpoint ARN. Example:
* arn:aws:comprehend:us-west-2:123456789012:entity-recognizer-endpoint/EXAMPLE
.
*
*
* -
*
* Lambda provisioned concurrency - The resource type is function
and the unique identifier is
* the function name with a function version or alias name suffix that is not $LATEST
. Example:
* function:my-function:prod
or function:my-function:1
.
*
*
* -
*
* Amazon Keyspaces table - The resource type is table
and the unique identifier is the table
* name. Example: keyspace/mykeyspace/table/mytable
.
*
*
* -
*
* Amazon MSK cluster - The resource type and unique identifier are specified using the cluster ARN.
* Example:
* arn:aws:kafka:us-east-1:123456789012:cluster/demo-cluster-1/6357e0b2-0e6a-4b86-a0b4-70df934c2e31-5
* .
*
*
* -
*
* Amazon ElastiCache replication group - The resource type is replication-group
and the unique
* identifier is the replication group name. Example: replication-group/mycluster
.
*
*
* -
*
* Neptune cluster - The resource type is cluster
and the unique identifier is the cluster
* name. Example: cluster:mycluster
.
*
*
* -
*
* SageMaker serverless endpoint - The resource type is variant
and the unique identifier is
* the resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* SageMaker inference component - The resource type is inference-component
and the unique
* identifier is the resource ID. Example: inference-component/my-inference-component
.
*
*
* -
*
* Pool of WorkSpaces - The resource type is workspacespool
and the unique identifier is the
* pool ID. Example: workspacespool/wspool-123456
.
*
*
*/
public final String resourceId() {
return resourceId;
}
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The task count of an ECS service.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot Fleet.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition and Aurora PostgreSQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for a SageMaker model endpoint
* variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
* -
*
* comprehend:document-classifier-endpoint:DesiredInferenceUnits
- The number of inference units for an
* Amazon Comprehend document classification endpoint.
*
*
* -
*
* comprehend:entity-recognizer-endpoint:DesiredInferenceUnits
- The number of inference units for an
* Amazon Comprehend entity recognizer endpoint.
*
*
* -
*
* lambda:function:ProvisionedConcurrency
- The provisioned concurrency for a Lambda function.
*
*
* -
*
* cassandra:table:ReadCapacityUnits
- The provisioned read capacity for an Amazon Keyspaces table.
*
*
* -
*
* cassandra:table:WriteCapacityUnits
- The provisioned write capacity for an Amazon Keyspaces table.
*
*
* -
*
* kafka:broker-storage:VolumeSize
- The provisioned volume size (in GiB) for brokers in an Amazon MSK
* cluster.
*
*
* -
*
* elasticache:replication-group:NodeGroups
- The number of node groups for an Amazon ElastiCache
* replication group.
*
*
* -
*
* elasticache:replication-group:Replicas
- The number of replicas per node group for an Amazon
* ElastiCache replication group.
*
*
* -
*
* neptune:cluster:ReadReplicaCount
- The count of read replicas in an Amazon Neptune DB cluster.
*
*
* -
*
* sagemaker:variant:DesiredProvisionedConcurrency
- The provisioned concurrency for a SageMaker
* serverless endpoint.
*
*
* -
*
* sagemaker:inference-component:DesiredCopyCount
- The number of copies across an endpoint for a
* SageMaker inference component.
*
*
* -
*
* workspaces:workspacespool:DesiredUserSessions
- The number of user sessions for the WorkSpaces in
* the pool.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scalableDimension}
* will return {@link ScalableDimension#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #scalableDimensionAsString}.
*
*
* @return The scalable dimension. This string consists of the service namespace, resource type, and scaling
* property.
*
* -
*
* ecs:service:DesiredCount
- The task count of an ECS service.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot Fleet.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster.
* Available for Aurora MySQL-compatible edition and Aurora PostgreSQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for a SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource
* provided by your own application or service.
*
*
* -
*
* comprehend:document-classifier-endpoint:DesiredInferenceUnits
- The number of inference
* units for an Amazon Comprehend document classification endpoint.
*
*
* -
*
* comprehend:entity-recognizer-endpoint:DesiredInferenceUnits
- The number of inference units
* for an Amazon Comprehend entity recognizer endpoint.
*
*
* -
*
* lambda:function:ProvisionedConcurrency
- The provisioned concurrency for a Lambda function.
*
*
* -
*
* cassandra:table:ReadCapacityUnits
- The provisioned read capacity for an Amazon Keyspaces
* table.
*
*
* -
*
* cassandra:table:WriteCapacityUnits
- The provisioned write capacity for an Amazon Keyspaces
* table.
*
*
* -
*
* kafka:broker-storage:VolumeSize
- The provisioned volume size (in GiB) for brokers in an
* Amazon MSK cluster.
*
*
* -
*
* elasticache:replication-group:NodeGroups
- The number of node groups for an Amazon
* ElastiCache replication group.
*
*
* -
*
* elasticache:replication-group:Replicas
- The number of replicas per node group for an Amazon
* ElastiCache replication group.
*
*
* -
*
* neptune:cluster:ReadReplicaCount
- The count of read replicas in an Amazon Neptune DB
* cluster.
*
*
* -
*
* sagemaker:variant:DesiredProvisionedConcurrency
- The provisioned concurrency for a
* SageMaker serverless endpoint.
*
*
* -
*
* sagemaker:inference-component:DesiredCopyCount
- The number of copies across an endpoint for
* a SageMaker inference component.
*
*
* -
*
* workspaces:workspacespool:DesiredUserSessions
- The number of user sessions for the
* WorkSpaces in the pool.
*
*
* @see ScalableDimension
*/
public final ScalableDimension scalableDimension() {
return ScalableDimension.fromValue(scalableDimension);
}
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The task count of an ECS service.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot Fleet.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition and Aurora PostgreSQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for a SageMaker model endpoint
* variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
* -
*
* comprehend:document-classifier-endpoint:DesiredInferenceUnits
- The number of inference units for an
* Amazon Comprehend document classification endpoint.
*
*
* -
*
* comprehend:entity-recognizer-endpoint:DesiredInferenceUnits
- The number of inference units for an
* Amazon Comprehend entity recognizer endpoint.
*
*
* -
*
* lambda:function:ProvisionedConcurrency
- The provisioned concurrency for a Lambda function.
*
*
* -
*
* cassandra:table:ReadCapacityUnits
- The provisioned read capacity for an Amazon Keyspaces table.
*
*
* -
*
* cassandra:table:WriteCapacityUnits
- The provisioned write capacity for an Amazon Keyspaces table.
*
*
* -
*
* kafka:broker-storage:VolumeSize
- The provisioned volume size (in GiB) for brokers in an Amazon MSK
* cluster.
*
*
* -
*
* elasticache:replication-group:NodeGroups
- The number of node groups for an Amazon ElastiCache
* replication group.
*
*
* -
*
* elasticache:replication-group:Replicas
- The number of replicas per node group for an Amazon
* ElastiCache replication group.
*
*
* -
*
* neptune:cluster:ReadReplicaCount
- The count of read replicas in an Amazon Neptune DB cluster.
*
*
* -
*
* sagemaker:variant:DesiredProvisionedConcurrency
- The provisioned concurrency for a SageMaker
* serverless endpoint.
*
*
* -
*
* sagemaker:inference-component:DesiredCopyCount
- The number of copies across an endpoint for a
* SageMaker inference component.
*
*
* -
*
* workspaces:workspacespool:DesiredUserSessions
- The number of user sessions for the WorkSpaces in
* the pool.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scalableDimension}
* will return {@link ScalableDimension#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #scalableDimensionAsString}.
*
*
* @return The scalable dimension. This string consists of the service namespace, resource type, and scaling
* property.
*
* -
*
* ecs:service:DesiredCount
- The task count of an ECS service.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot Fleet.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster.
* Available for Aurora MySQL-compatible edition and Aurora PostgreSQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for a SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource
* provided by your own application or service.
*
*
* -
*
* comprehend:document-classifier-endpoint:DesiredInferenceUnits
- The number of inference
* units for an Amazon Comprehend document classification endpoint.
*
*
* -
*
* comprehend:entity-recognizer-endpoint:DesiredInferenceUnits
- The number of inference units
* for an Amazon Comprehend entity recognizer endpoint.
*
*
* -
*
* lambda:function:ProvisionedConcurrency
- The provisioned concurrency for a Lambda function.
*
*
* -
*
* cassandra:table:ReadCapacityUnits
- The provisioned read capacity for an Amazon Keyspaces
* table.
*
*
* -
*
* cassandra:table:WriteCapacityUnits
- The provisioned write capacity for an Amazon Keyspaces
* table.
*
*
* -
*
* kafka:broker-storage:VolumeSize
- The provisioned volume size (in GiB) for brokers in an
* Amazon MSK cluster.
*
*
* -
*
* elasticache:replication-group:NodeGroups
- The number of node groups for an Amazon
* ElastiCache replication group.
*
*
* -
*
* elasticache:replication-group:Replicas
- The number of replicas per node group for an Amazon
* ElastiCache replication group.
*
*
* -
*
* neptune:cluster:ReadReplicaCount
- The count of read replicas in an Amazon Neptune DB
* cluster.
*
*
* -
*
* sagemaker:variant:DesiredProvisionedConcurrency
- The provisioned concurrency for a
* SageMaker serverless endpoint.
*
*
* -
*
* sagemaker:inference-component:DesiredCopyCount
- The number of copies across an endpoint for
* a SageMaker inference component.
*
*
* -
*
* workspaces:workspacespool:DesiredUserSessions
- The number of user sessions for the
* WorkSpaces in the pool.
*
*
* @see ScalableDimension
*/
public final String scalableDimensionAsString() {
return scalableDimension;
}
/**
*
* The scaling policy type.
*
*
* The following policy types are supported:
*
*
* TargetTrackingScaling
—Not supported for Amazon EMR
*
*
* StepScaling
—Not supported for DynamoDB, Amazon Comprehend, Lambda, Amazon Keyspaces, Amazon MSK,
* Amazon ElastiCache, or Neptune.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #policyType} will
* return {@link PolicyType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #policyTypeAsString}.
*
*
* @return The scaling policy type.
*
* The following policy types are supported:
*
*
* TargetTrackingScaling
—Not supported for Amazon EMR
*
*
* StepScaling
—Not supported for DynamoDB, Amazon Comprehend, Lambda, Amazon Keyspaces, Amazon
* MSK, Amazon ElastiCache, or Neptune.
* @see PolicyType
*/
public final PolicyType policyType() {
return PolicyType.fromValue(policyType);
}
/**
*
* The scaling policy type.
*
*
* The following policy types are supported:
*
*
* TargetTrackingScaling
—Not supported for Amazon EMR
*
*
* StepScaling
—Not supported for DynamoDB, Amazon Comprehend, Lambda, Amazon Keyspaces, Amazon MSK,
* Amazon ElastiCache, or Neptune.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #policyType} will
* return {@link PolicyType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #policyTypeAsString}.
*
*
* @return The scaling policy type.
*
* The following policy types are supported:
*
*
* TargetTrackingScaling
—Not supported for Amazon EMR
*
*
* StepScaling
—Not supported for DynamoDB, Amazon Comprehend, Lambda, Amazon Keyspaces, Amazon
* MSK, Amazon ElastiCache, or Neptune.
* @see PolicyType
*/
public final String policyTypeAsString() {
return policyType;
}
/**
*
* A step scaling policy.
*
*
* @return A step scaling policy.
*/
public final StepScalingPolicyConfiguration stepScalingPolicyConfiguration() {
return stepScalingPolicyConfiguration;
}
/**
*
* A target tracking scaling policy.
*
*
* @return A target tracking scaling policy.
*/
public final TargetTrackingScalingPolicyConfiguration targetTrackingScalingPolicyConfiguration() {
return targetTrackingScalingPolicyConfiguration;
}
/**
*
* The predictive scaling policy configuration.
*
*
* @return The predictive scaling policy configuration.
*/
public final PredictiveScalingPolicyConfiguration predictiveScalingPolicyConfiguration() {
return predictiveScalingPolicyConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the Alarms property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAlarms() {
return alarms != null && !(alarms instanceof SdkAutoConstructList);
}
/**
*
* The CloudWatch alarms associated with the scaling policy.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAlarms} method.
*
*
* @return The CloudWatch alarms associated with the scaling policy.
*/
public final List alarms() {
return alarms;
}
/**
*
* The Unix timestamp for when the scaling policy was created.
*
*
* @return The Unix timestamp for when the scaling policy was created.
*/
public final Instant creationTime() {
return creationTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(policyARN());
hashCode = 31 * hashCode + Objects.hashCode(policyName());
hashCode = 31 * hashCode + Objects.hashCode(serviceNamespaceAsString());
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(scalableDimensionAsString());
hashCode = 31 * hashCode + Objects.hashCode(policyTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(stepScalingPolicyConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(targetTrackingScalingPolicyConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(predictiveScalingPolicyConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasAlarms() ? alarms() : null);
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ScalingPolicy)) {
return false;
}
ScalingPolicy other = (ScalingPolicy) obj;
return Objects.equals(policyARN(), other.policyARN()) && Objects.equals(policyName(), other.policyName())
&& Objects.equals(serviceNamespaceAsString(), other.serviceNamespaceAsString())
&& Objects.equals(resourceId(), other.resourceId())
&& Objects.equals(scalableDimensionAsString(), other.scalableDimensionAsString())
&& Objects.equals(policyTypeAsString(), other.policyTypeAsString())
&& Objects.equals(stepScalingPolicyConfiguration(), other.stepScalingPolicyConfiguration())
&& Objects.equals(targetTrackingScalingPolicyConfiguration(), other.targetTrackingScalingPolicyConfiguration())
&& Objects.equals(predictiveScalingPolicyConfiguration(), other.predictiveScalingPolicyConfiguration())
&& hasAlarms() == other.hasAlarms() && Objects.equals(alarms(), other.alarms())
&& Objects.equals(creationTime(), other.creationTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ScalingPolicy").add("PolicyARN", policyARN()).add("PolicyName", policyName())
.add("ServiceNamespace", serviceNamespaceAsString()).add("ResourceId", resourceId())
.add("ScalableDimension", scalableDimensionAsString()).add("PolicyType", policyTypeAsString())
.add("StepScalingPolicyConfiguration", stepScalingPolicyConfiguration())
.add("TargetTrackingScalingPolicyConfiguration", targetTrackingScalingPolicyConfiguration())
.add("PredictiveScalingPolicyConfiguration", predictiveScalingPolicyConfiguration())
.add("Alarms", hasAlarms() ? alarms() : null).add("CreationTime", creationTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PolicyARN":
return Optional.ofNullable(clazz.cast(policyARN()));
case "PolicyName":
return Optional.ofNullable(clazz.cast(policyName()));
case "ServiceNamespace":
return Optional.ofNullable(clazz.cast(serviceNamespaceAsString()));
case "ResourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "ScalableDimension":
return Optional.ofNullable(clazz.cast(scalableDimensionAsString()));
case "PolicyType":
return Optional.ofNullable(clazz.cast(policyTypeAsString()));
case "StepScalingPolicyConfiguration":
return Optional.ofNullable(clazz.cast(stepScalingPolicyConfiguration()));
case "TargetTrackingScalingPolicyConfiguration":
return Optional.ofNullable(clazz.cast(targetTrackingScalingPolicyConfiguration()));
case "PredictiveScalingPolicyConfiguration":
return Optional.ofNullable(clazz.cast(predictiveScalingPolicyConfiguration()));
case "Alarms":
return Optional.ofNullable(clazz.cast(alarms()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function