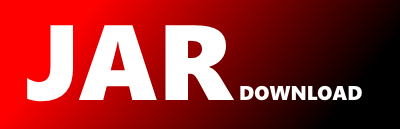
software.amazon.awssdk.services.applicationdiscovery.ApplicationDiscoveryAsyncClient Maven / Gradle / Ivy
Show all versions of applicationdiscovery Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationdiscovery;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteAgentsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteAgentsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteImportDataRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteImportDataResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeBatchDeleteConfigurationTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeBatchDeleteConfigurationTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartBatchDeleteConfigurationTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartBatchDeleteConfigurationTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartContinuousExportRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartContinuousExportResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartImportTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartImportTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StopContinuousExportRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StopContinuousExportResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher;
import software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeContinuousExportsPublisher;
import software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher;
import software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher;
import software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher;
import software.amazon.awssdk.services.applicationdiscovery.paginators.ListConfigurationsPublisher;
/**
* Service client for accessing AWS Application Discovery Service asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
* Amazon Web Services Application Discovery Service
*
* Amazon Web Services Application Discovery Service (Application Discovery Service) helps you plan application
* migration projects. It automatically identifies servers, virtual machines (VMs), and network dependencies in your
* on-premises data centers. For more information, see the Amazon Web Services Application Discovery Service FAQ.
*
*
* Application Discovery Service offers three ways of performing discovery and collecting data about your on-premises
* servers:
*
*
* -
*
* Agentless discovery using Amazon Web Services Application Discovery Service Agentless Collector (Agentless
* Collector), which doesn't require you to install an agent on each host.
*
*
* -
*
* Agentless Collector gathers server information regardless of the operating systems, which minimizes the time required
* for initial on-premises infrastructure assessment.
*
*
* -
*
* Agentless Collector doesn't collect information about network dependencies, only agent-based discovery collects that
* information.
*
*
*
*
*
*
* -
*
* Agent-based discovery using the Amazon Web Services Application Discovery Agent (Application Discovery Agent)
* collects a richer set of data than agentless discovery, which you install on one or more hosts in your data center.
*
*
* -
*
* The agent captures infrastructure and application information, including an inventory of running processes, system
* performance information, resource utilization, and network dependencies.
*
*
* -
*
* The information collected by agents is secured at rest and in transit to the Application Discovery Service database
* in the Amazon Web Services cloud. For more information, see Amazon Web Services
* Application Discovery Agent.
*
*
*
*
*
*
* -
*
* Amazon Web Services Partner Network (APN) solutions integrate with Application Discovery Service, enabling you
* to import details of your on-premises environment directly into Amazon Web Services Migration Hub (Migration Hub)
* without using Agentless Collector or Application Discovery Agent.
*
*
* -
*
* Third-party application discovery tools can query Amazon Web Services Application Discovery Service, and they can
* write to the Application Discovery Service database using the public API.
*
*
* -
*
* In this way, you can import data into Migration Hub and view it, so that you can associate applications with servers
* and track migrations.
*
*
*
*
*
*
* Working With This Guide
*
*
* This API reference provides descriptions, syntax, and usage examples for each of the actions and data types for
* Application Discovery Service. The topic for each action shows the API request parameters and the response.
* Alternatively, you can use one of the Amazon Web Services SDKs to access an API that is tailored to the programming
* language or platform that you're using. For more information, see Amazon
* Web Services SDKs.
*
*
*
* -
*
* Remember that you must set your Migration Hub home Region before you call any of these APIs.
*
*
* -
*
* You must make API calls for write actions (create, notify, associate, disassociate, import, or put) while in your
* home Region, or a HomeRegionNotSetException
error is returned.
*
*
* -
*
* API calls for read actions (list, describe, stop, and delete) are permitted outside of your home Region.
*
*
* -
*
* Although it is unlikely, the Migration Hub home Region could change. If you call APIs outside the home Region, an
* InvalidInputException
is returned.
*
*
* -
*
* You must call GetHomeRegion
to obtain the latest Migration Hub home Region.
*
*
*
*
*
* This guide is intended for use with the Amazon Web Services Application Discovery
* Service User Guide.
*
*
*
* All data is handled according to the Amazon Web Services Privacy
* Policy. You can operate Application Discovery Service offline to inspect collected data before it is shared with
* the service.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface ApplicationDiscoveryAsyncClient extends AwsClient {
String SERVICE_NAME = "discovery";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "discovery";
/**
*
* Associates one or more configuration items with an application.
*
*
* @param associateConfigurationItemsToApplicationRequest
* @return A Java Future containing the result of the AssociateConfigurationItemsToApplication operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.AssociateConfigurationItemsToApplication
*/
default CompletableFuture associateConfigurationItemsToApplication(
AssociateConfigurationItemsToApplicationRequest associateConfigurationItemsToApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates one or more configuration items with an application.
*
*
*
* This is a convenience which creates an instance of the
* {@link AssociateConfigurationItemsToApplicationRequest.Builder} avoiding the need to create one manually via
* {@link AssociateConfigurationItemsToApplicationRequest#builder()}
*
*
* @param associateConfigurationItemsToApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the AssociateConfigurationItemsToApplication operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.AssociateConfigurationItemsToApplication
*/
default CompletableFuture associateConfigurationItemsToApplication(
Consumer associateConfigurationItemsToApplicationRequest) {
return associateConfigurationItemsToApplication(AssociateConfigurationItemsToApplicationRequest.builder()
.applyMutation(associateConfigurationItemsToApplicationRequest).build());
}
/**
*
* Deletes one or more agents or collectors as specified by ID. Deleting an agent or collector does not delete the
* previously discovered data. To delete the data collected, use StartBatchDeleteConfigurationTask
.
*
*
* @param batchDeleteAgentsRequest
* @return A Java Future containing the result of the BatchDeleteAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.BatchDeleteAgents
*/
default CompletableFuture batchDeleteAgents(BatchDeleteAgentsRequest batchDeleteAgentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes one or more agents or collectors as specified by ID. Deleting an agent or collector does not delete the
* previously discovered data. To delete the data collected, use StartBatchDeleteConfigurationTask
.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDeleteAgentsRequest.Builder} avoiding the need
* to create one manually via {@link BatchDeleteAgentsRequest#builder()}
*
*
* @param batchDeleteAgentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteAgentsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the BatchDeleteAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.BatchDeleteAgents
*/
default CompletableFuture batchDeleteAgents(
Consumer batchDeleteAgentsRequest) {
return batchDeleteAgents(BatchDeleteAgentsRequest.builder().applyMutation(batchDeleteAgentsRequest).build());
}
/**
*
* Deletes one or more import tasks, each identified by their import ID. Each import task has a number of records
* that can identify servers or applications.
*
*
* Amazon Web Services Application Discovery Service has built-in matching logic that will identify when discovered
* servers match existing entries that you've previously discovered, the information for the already-existing
* discovered server is updated. When you delete an import task that contains records that were used to match, the
* information in those matched records that comes from the deleted records will also be deleted.
*
*
* @param batchDeleteImportDataRequest
* @return A Java Future containing the result of the BatchDeleteImportData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.BatchDeleteImportData
*/
default CompletableFuture batchDeleteImportData(
BatchDeleteImportDataRequest batchDeleteImportDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes one or more import tasks, each identified by their import ID. Each import task has a number of records
* that can identify servers or applications.
*
*
* Amazon Web Services Application Discovery Service has built-in matching logic that will identify when discovered
* servers match existing entries that you've previously discovered, the information for the already-existing
* discovered server is updated. When you delete an import task that contains records that were used to match, the
* information in those matched records that comes from the deleted records will also be deleted.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDeleteImportDataRequest.Builder} avoiding the
* need to create one manually via {@link BatchDeleteImportDataRequest#builder()}
*
*
* @param batchDeleteImportDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteImportDataRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the BatchDeleteImportData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.BatchDeleteImportData
*/
default CompletableFuture batchDeleteImportData(
Consumer batchDeleteImportDataRequest) {
return batchDeleteImportData(BatchDeleteImportDataRequest.builder().applyMutation(batchDeleteImportDataRequest).build());
}
/**
*
* Creates an application with the given name and description.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateApplication
*/
default CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an application with the given name and description.
*
*
*
* This is a convenience which creates an instance of the {@link CreateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link CreateApplicationRequest#builder()}
*
*
* @param createApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateApplication
*/
default CompletableFuture createApplication(
Consumer createApplicationRequest) {
return createApplication(CreateApplicationRequest.builder().applyMutation(createApplicationRequest).build());
}
/**
*
* Creates one or more tags for configuration items. Tags are metadata that help you categorize IT assets. This API
* accepts a list of multiple configuration items.
*
*
*
* Do not store sensitive information (like personal data) in tags.
*
*
*
* @param createTagsRequest
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateTags
*/
default CompletableFuture createTags(CreateTagsRequest createTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates one or more tags for configuration items. Tags are metadata that help you categorize IT assets. This API
* accepts a list of multiple configuration items.
*
*
*
* Do not store sensitive information (like personal data) in tags.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTagsRequest.Builder} avoiding the need to
* create one manually via {@link CreateTagsRequest#builder()}
*
*
* @param createTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateTags
*/
default CompletableFuture createTags(Consumer createTagsRequest) {
return createTags(CreateTagsRequest.builder().applyMutation(createTagsRequest).build());
}
/**
*
* Deletes a list of applications and their associations with configuration items.
*
*
* @param deleteApplicationsRequest
* @return A Java Future containing the result of the DeleteApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteApplications
*/
default CompletableFuture deleteApplications(DeleteApplicationsRequest deleteApplicationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a list of applications and their associations with configuration items.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteApplicationsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteApplicationsRequest#builder()}
*
*
* @param deleteApplicationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteApplications
*/
default CompletableFuture deleteApplications(
Consumer deleteApplicationsRequest) {
return deleteApplications(DeleteApplicationsRequest.builder().applyMutation(deleteApplicationsRequest).build());
}
/**
*
* Deletes the association between configuration items and one or more tags. This API accepts a list of multiple
* configuration items.
*
*
* @param deleteTagsRequest
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteTags
*/
default CompletableFuture deleteTags(DeleteTagsRequest deleteTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the association between configuration items and one or more tags. This API accepts a list of multiple
* configuration items.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTagsRequest.Builder} avoiding the need to
* create one manually via {@link DeleteTagsRequest#builder()}
*
*
* @param deleteTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteTags
*/
default CompletableFuture deleteTags(Consumer deleteTagsRequest) {
return deleteTags(DeleteTagsRequest.builder().applyMutation(deleteTagsRequest).build());
}
/**
*
* Lists agents or collectors as specified by ID or other filters. All agents/collectors associated with your user
* can be listed if you call DescribeAgents
as is without passing any parameters.
*
*
* @param describeAgentsRequest
* @return A Java Future containing the result of the DescribeAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
default CompletableFuture describeAgents(DescribeAgentsRequest describeAgentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists agents or collectors as specified by ID or other filters. All agents/collectors associated with your user
* can be listed if you call DescribeAgents
as is without passing any parameters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAgentsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAgentsRequest#builder()}
*
*
* @param describeAgentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
default CompletableFuture describeAgents(Consumer describeAgentsRequest) {
return describeAgents(DescribeAgentsRequest.builder().applyMutation(describeAgentsRequest).build());
}
/**
*
* Lists agents or collectors as specified by ID or other filters. All agents/collectors associated with your user
* can be listed if you call DescribeAgents
as is without passing any parameters.
*
*
* @return A Java Future containing the result of the DescribeAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
default CompletableFuture describeAgents() {
return describeAgents(DescribeAgentsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeAgents(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher publisher = client.describeAgentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher publisher = client.describeAgentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAgents(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
default DescribeAgentsPublisher describeAgentsPaginator() {
return describeAgentsPaginator(DescribeAgentsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeAgents(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher publisher = client.describeAgentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher publisher = client.describeAgentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAgents(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest)}
* operation.
*
*
* @param describeAgentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
default DescribeAgentsPublisher describeAgentsPaginator(DescribeAgentsRequest describeAgentsRequest) {
return new DescribeAgentsPublisher(this, describeAgentsRequest);
}
/**
*
* This is a variant of
* {@link #describeAgents(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher publisher = client.describeAgentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeAgentsPublisher publisher = client.describeAgentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAgents(software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAgentsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAgentsRequest#builder()}
*
*
* @param describeAgentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
default DescribeAgentsPublisher describeAgentsPaginator(Consumer describeAgentsRequest) {
return describeAgentsPaginator(DescribeAgentsRequest.builder().applyMutation(describeAgentsRequest).build());
}
/**
*
* Takes a unique deletion task identifier as input and returns metadata about a configuration deletion task.
*
*
* @param describeBatchDeleteConfigurationTaskRequest
* @return A Java Future containing the result of the DescribeBatchDeleteConfigurationTask operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeBatchDeleteConfigurationTask
*/
default CompletableFuture describeBatchDeleteConfigurationTask(
DescribeBatchDeleteConfigurationTaskRequest describeBatchDeleteConfigurationTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Takes a unique deletion task identifier as input and returns metadata about a configuration deletion task.
*
*
*
* This is a convenience which creates an instance of the
* {@link DescribeBatchDeleteConfigurationTaskRequest.Builder} avoiding the need to create one manually via
* {@link DescribeBatchDeleteConfigurationTaskRequest#builder()}
*
*
* @param describeBatchDeleteConfigurationTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeBatchDeleteConfigurationTaskRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeBatchDeleteConfigurationTask operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeBatchDeleteConfigurationTask
*/
default CompletableFuture describeBatchDeleteConfigurationTask(
Consumer describeBatchDeleteConfigurationTaskRequest) {
return describeBatchDeleteConfigurationTask(DescribeBatchDeleteConfigurationTaskRequest.builder()
.applyMutation(describeBatchDeleteConfigurationTaskRequest).build());
}
/**
*
* Retrieves attributes for a list of configuration item IDs.
*
*
*
* All of the supplied IDs must be for the same asset type from one of the following:
*
*
* -
*
* server
*
*
* -
*
* application
*
*
* -
*
* process
*
*
* -
*
* connection
*
*
*
*
* Output fields are specific to the asset type specified. For example, the output for a server configuration
* item includes a list of attributes about the server, such as host name, operating system, number of network
* cards, etc.
*
*
* For a complete list of outputs for each asset type, see Using the DescribeConfigurations Action in the Amazon Web Services Application Discovery Service User
* Guide.
*
*
*
* @param describeConfigurationsRequest
* @return A Java Future containing the result of the DescribeConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeConfigurations
*/
default CompletableFuture describeConfigurations(
DescribeConfigurationsRequest describeConfigurationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves attributes for a list of configuration item IDs.
*
*
*
* All of the supplied IDs must be for the same asset type from one of the following:
*
*
* -
*
* server
*
*
* -
*
* application
*
*
* -
*
* process
*
*
* -
*
* connection
*
*
*
*
* Output fields are specific to the asset type specified. For example, the output for a server configuration
* item includes a list of attributes about the server, such as host name, operating system, number of network
* cards, etc.
*
*
* For a complete list of outputs for each asset type, see Using the DescribeConfigurations Action in the Amazon Web Services Application Discovery Service User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeConfigurationsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeConfigurationsRequest#builder()}
*
*
* @param describeConfigurationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeConfigurations
*/
default CompletableFuture describeConfigurations(
Consumer describeConfigurationsRequest) {
return describeConfigurations(DescribeConfigurationsRequest.builder().applyMutation(describeConfigurationsRequest)
.build());
}
/**
*
* Lists exports as specified by ID. All continuous exports associated with your user can be listed if you call
* DescribeContinuousExports
as is without passing any parameters.
*
*
* @param describeContinuousExportsRequest
* @return A Java Future containing the result of the DescribeContinuousExports operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeContinuousExports
*/
default CompletableFuture describeContinuousExports(
DescribeContinuousExportsRequest describeContinuousExportsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists exports as specified by ID. All continuous exports associated with your user can be listed if you call
* DescribeContinuousExports
as is without passing any parameters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContinuousExportsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeContinuousExportsRequest#builder()}
*
*
* @param describeContinuousExportsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeContinuousExports operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeContinuousExports
*/
default CompletableFuture describeContinuousExports(
Consumer describeContinuousExportsRequest) {
return describeContinuousExports(DescribeContinuousExportsRequest.builder()
.applyMutation(describeContinuousExportsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeContinuousExports(software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeContinuousExportsPublisher publisher = client.describeContinuousExportsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeContinuousExportsPublisher publisher = client.describeContinuousExportsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeContinuousExports(software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest)}
* operation.
*
*
* @param describeContinuousExportsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeContinuousExports
*/
default DescribeContinuousExportsPublisher describeContinuousExportsPaginator(
DescribeContinuousExportsRequest describeContinuousExportsRequest) {
return new DescribeContinuousExportsPublisher(this, describeContinuousExportsRequest);
}
/**
*
* This is a variant of
* {@link #describeContinuousExports(software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeContinuousExportsPublisher publisher = client.describeContinuousExportsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeContinuousExportsPublisher publisher = client.describeContinuousExportsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeContinuousExports(software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContinuousExportsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeContinuousExportsRequest#builder()}
*
*
* @param describeContinuousExportsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeContinuousExports
*/
default DescribeContinuousExportsPublisher describeContinuousExportsPaginator(
Consumer describeContinuousExportsRequest) {
return describeContinuousExportsPaginator(DescribeContinuousExportsRequest.builder()
.applyMutation(describeContinuousExportsRequest).build());
}
/**
*
* Retrieve status of one or more export tasks. You can retrieve the status of up to 100 export tasks.
*
*
* @param describeExportTasksRequest
* @return A Java Future containing the result of the DescribeExportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
default CompletableFuture describeExportTasks(
DescribeExportTasksRequest describeExportTasksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve status of one or more export tasks. You can retrieve the status of up to 100 export tasks.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeExportTasksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeExportTasksRequest#builder()}
*
*
* @param describeExportTasksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeExportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
default CompletableFuture describeExportTasks(
Consumer describeExportTasksRequest) {
return describeExportTasks(DescribeExportTasksRequest.builder().applyMutation(describeExportTasksRequest).build());
}
/**
*
* Retrieve status of one or more export tasks. You can retrieve the status of up to 100 export tasks.
*
*
* @return A Java Future containing the result of the DescribeExportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
default CompletableFuture describeExportTasks() {
return describeExportTasks(DescribeExportTasksRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeExportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher publisher = client.describeExportTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher publisher = client.describeExportTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeExportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
default DescribeExportTasksPublisher describeExportTasksPaginator() {
return describeExportTasksPaginator(DescribeExportTasksRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeExportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher publisher = client.describeExportTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher publisher = client.describeExportTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeExportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest)}
* operation.
*
*
* @param describeExportTasksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
default DescribeExportTasksPublisher describeExportTasksPaginator(DescribeExportTasksRequest describeExportTasksRequest) {
return new DescribeExportTasksPublisher(this, describeExportTasksRequest);
}
/**
*
* This is a variant of
* {@link #describeExportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher publisher = client.describeExportTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeExportTasksPublisher publisher = client.describeExportTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeExportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeExportTasksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeExportTasksRequest#builder()}
*
*
* @param describeExportTasksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
default DescribeExportTasksPublisher describeExportTasksPaginator(
Consumer describeExportTasksRequest) {
return describeExportTasksPaginator(DescribeExportTasksRequest.builder().applyMutation(describeExportTasksRequest)
.build());
}
/**
*
* Returns an array of import tasks for your account, including status information, times, IDs, the Amazon S3 Object
* URL for the import file, and more.
*
*
* @param describeImportTasksRequest
* @return A Java Future containing the result of the DescribeImportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
default CompletableFuture describeImportTasks(
DescribeImportTasksRequest describeImportTasksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of import tasks for your account, including status information, times, IDs, the Amazon S3 Object
* URL for the import file, and more.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImportTasksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeImportTasksRequest#builder()}
*
*
* @param describeImportTasksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeImportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
default CompletableFuture describeImportTasks(
Consumer describeImportTasksRequest) {
return describeImportTasks(DescribeImportTasksRequest.builder().applyMutation(describeImportTasksRequest).build());
}
/**
*
* Returns an array of import tasks for your account, including status information, times, IDs, the Amazon S3 Object
* URL for the import file, and more.
*
*
* @return A Java Future containing the result of the DescribeImportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
default CompletableFuture describeImportTasks() {
return describeImportTasks(DescribeImportTasksRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeImportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher publisher = client.describeImportTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher publisher = client.describeImportTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
default DescribeImportTasksPublisher describeImportTasksPaginator() {
return describeImportTasksPaginator(DescribeImportTasksRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeImportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher publisher = client.describeImportTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher publisher = client.describeImportTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest)}
* operation.
*
*
* @param describeImportTasksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
default DescribeImportTasksPublisher describeImportTasksPaginator(DescribeImportTasksRequest describeImportTasksRequest) {
return new DescribeImportTasksPublisher(this, describeImportTasksRequest);
}
/**
*
* This is a variant of
* {@link #describeImportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher publisher = client.describeImportTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeImportTasksPublisher publisher = client.describeImportTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImportTasks(software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImportTasksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeImportTasksRequest#builder()}
*
*
* @param describeImportTasksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
default DescribeImportTasksPublisher describeImportTasksPaginator(
Consumer describeImportTasksRequest) {
return describeImportTasksPaginator(DescribeImportTasksRequest.builder().applyMutation(describeImportTasksRequest)
.build());
}
/**
*
* Retrieves a list of configuration items that have tags as specified by the key-value pairs, name and value,
* passed to the optional parameter filters
.
*
*
* There are three valid tag filter names:
*
*
* -
*
* tagKey
*
*
* -
*
* tagValue
*
*
* -
*
* configurationId
*
*
*
*
* Also, all configuration items associated with your user that have tags can be listed if you call
* DescribeTags
as is without passing any parameters.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
default CompletableFuture describeTags(DescribeTagsRequest describeTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of configuration items that have tags as specified by the key-value pairs, name and value,
* passed to the optional parameter filters
.
*
*
* There are three valid tag filter names:
*
*
* -
*
* tagKey
*
*
* -
*
* tagValue
*
*
* -
*
* configurationId
*
*
*
*
* Also, all configuration items associated with your user that have tags can be listed if you call
* DescribeTags
as is without passing any parameters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTagsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTagsRequest#builder()}
*
*
* @param describeTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
default CompletableFuture describeTags(Consumer describeTagsRequest) {
return describeTags(DescribeTagsRequest.builder().applyMutation(describeTagsRequest).build());
}
/**
*
* Retrieves a list of configuration items that have tags as specified by the key-value pairs, name and value,
* passed to the optional parameter filters
.
*
*
* There are three valid tag filter names:
*
*
* -
*
* tagKey
*
*
* -
*
* tagValue
*
*
* -
*
* configurationId
*
*
*
*
* Also, all configuration items associated with your user that have tags can be listed if you call
* DescribeTags
as is without passing any parameters.
*
*
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
default CompletableFuture describeTags() {
return describeTags(DescribeTagsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeTags(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher publisher = client.describeTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher publisher = client.describeTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTags(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
default DescribeTagsPublisher describeTagsPaginator() {
return describeTagsPaginator(DescribeTagsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeTags(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher publisher = client.describeTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher publisher = client.describeTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTags(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest)}
* operation.
*
*
* @param describeTagsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
default DescribeTagsPublisher describeTagsPaginator(DescribeTagsRequest describeTagsRequest) {
return new DescribeTagsPublisher(this, describeTagsRequest);
}
/**
*
* This is a variant of
* {@link #describeTags(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher publisher = client.describeTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.DescribeTagsPublisher publisher = client.describeTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTags(software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTagsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTagsRequest#builder()}
*
*
* @param describeTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
default DescribeTagsPublisher describeTagsPaginator(Consumer describeTagsRequest) {
return describeTagsPaginator(DescribeTagsRequest.builder().applyMutation(describeTagsRequest).build());
}
/**
*
* Disassociates one or more configuration items from an application.
*
*
* @param disassociateConfigurationItemsFromApplicationRequest
* @return A Java Future containing the result of the DisassociateConfigurationItemsFromApplication operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DisassociateConfigurationItemsFromApplication
*/
default CompletableFuture disassociateConfigurationItemsFromApplication(
DisassociateConfigurationItemsFromApplicationRequest disassociateConfigurationItemsFromApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates one or more configuration items from an application.
*
*
*
* This is a convenience which creates an instance of the
* {@link DisassociateConfigurationItemsFromApplicationRequest.Builder} avoiding the need to create one manually via
* {@link DisassociateConfigurationItemsFromApplicationRequest#builder()}
*
*
* @param disassociateConfigurationItemsFromApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DisassociateConfigurationItemsFromApplication operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DisassociateConfigurationItemsFromApplication
*/
default CompletableFuture disassociateConfigurationItemsFromApplication(
Consumer disassociateConfigurationItemsFromApplicationRequest) {
return disassociateConfigurationItemsFromApplication(DisassociateConfigurationItemsFromApplicationRequest.builder()
.applyMutation(disassociateConfigurationItemsFromApplicationRequest).build());
}
/**
*
* Retrieves a short summary of discovered assets.
*
*
* This API operation takes no request parameters and is called as is at the command prompt as shown in the example.
*
*
* @param getDiscoverySummaryRequest
* @return A Java Future containing the result of the GetDiscoverySummary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.GetDiscoverySummary
*/
default CompletableFuture getDiscoverySummary(
GetDiscoverySummaryRequest getDiscoverySummaryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a short summary of discovered assets.
*
*
* This API operation takes no request parameters and is called as is at the command prompt as shown in the example.
*
*
*
* This is a convenience which creates an instance of the {@link GetDiscoverySummaryRequest.Builder} avoiding the
* need to create one manually via {@link GetDiscoverySummaryRequest#builder()}
*
*
* @param getDiscoverySummaryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetDiscoverySummary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.GetDiscoverySummary
*/
default CompletableFuture getDiscoverySummary(
Consumer getDiscoverySummaryRequest) {
return getDiscoverySummary(GetDiscoverySummaryRequest.builder().applyMutation(getDiscoverySummaryRequest).build());
}
/**
*
* Retrieves a short summary of discovered assets.
*
*
* This API operation takes no request parameters and is called as is at the command prompt as shown in the example.
*
*
* @return A Java Future containing the result of the GetDiscoverySummary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.GetDiscoverySummary
*/
default CompletableFuture getDiscoverySummary() {
return getDiscoverySummary(GetDiscoverySummaryRequest.builder().build());
}
/**
*
* Retrieves a list of configuration items as specified by the value passed to the required parameter
* configurationType
. Optional filtering may be applied to refine search results.
*
*
* @param listConfigurationsRequest
* @return A Java Future containing the result of the ListConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListConfigurations
*/
default CompletableFuture listConfigurations(ListConfigurationsRequest listConfigurationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of configuration items as specified by the value passed to the required parameter
* configurationType
. Optional filtering may be applied to refine search results.
*
*
*
* This is a convenience which creates an instance of the {@link ListConfigurationsRequest.Builder} avoiding the
* need to create one manually via {@link ListConfigurationsRequest#builder()}
*
*
* @param listConfigurationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListConfigurations
*/
default CompletableFuture listConfigurations(
Consumer listConfigurationsRequest) {
return listConfigurations(ListConfigurationsRequest.builder().applyMutation(listConfigurationsRequest).build());
}
/**
*
* This is a variant of
* {@link #listConfigurations(software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.ListConfigurationsPublisher publisher = client.listConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.ListConfigurationsPublisher publisher = client.listConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConfigurations(software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest)}
* operation.
*
*
* @param listConfigurationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListConfigurations
*/
default ListConfigurationsPublisher listConfigurationsPaginator(ListConfigurationsRequest listConfigurationsRequest) {
return new ListConfigurationsPublisher(this, listConfigurationsRequest);
}
/**
*
* This is a variant of
* {@link #listConfigurations(software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.ListConfigurationsPublisher publisher = client.listConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.applicationdiscovery.paginators.ListConfigurationsPublisher publisher = client.listConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConfigurations(software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListConfigurationsRequest.Builder} avoiding the
* need to create one manually via {@link ListConfigurationsRequest#builder()}
*
*
* @param listConfigurationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListConfigurations
*/
default ListConfigurationsPublisher listConfigurationsPaginator(
Consumer listConfigurationsRequest) {
return listConfigurationsPaginator(ListConfigurationsRequest.builder().applyMutation(listConfigurationsRequest).build());
}
/**
*
* Retrieves a list of servers that are one network hop away from a specified server.
*
*
* @param listServerNeighborsRequest
* @return A Java Future containing the result of the ListServerNeighbors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListServerNeighbors
*/
default CompletableFuture listServerNeighbors(
ListServerNeighborsRequest listServerNeighborsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of servers that are one network hop away from a specified server.
*
*
*
* This is a convenience which creates an instance of the {@link ListServerNeighborsRequest.Builder} avoiding the
* need to create one manually via {@link ListServerNeighborsRequest#builder()}
*
*
* @param listServerNeighborsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListServerNeighbors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListServerNeighbors
*/
default CompletableFuture listServerNeighbors(
Consumer listServerNeighborsRequest) {
return listServerNeighbors(ListServerNeighborsRequest.builder().applyMutation(listServerNeighborsRequest).build());
}
/**
*
* Takes a list of configurationId as input and starts an asynchronous deletion task to remove the
* configurationItems. Returns a unique deletion task identifier.
*
*
* @param startBatchDeleteConfigurationTaskRequest
* @return A Java Future containing the result of the StartBatchDeleteConfigurationTask operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The limit of 200 configuration IDs per request has been exceeded.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - OperationNotPermittedException This operation is not permitted.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartBatchDeleteConfigurationTask
*/
default CompletableFuture startBatchDeleteConfigurationTask(
StartBatchDeleteConfigurationTaskRequest startBatchDeleteConfigurationTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Takes a list of configurationId as input and starts an asynchronous deletion task to remove the
* configurationItems. Returns a unique deletion task identifier.
*
*
*
* This is a convenience which creates an instance of the {@link StartBatchDeleteConfigurationTaskRequest.Builder}
* avoiding the need to create one manually via {@link StartBatchDeleteConfigurationTaskRequest#builder()}
*
*
* @param startBatchDeleteConfigurationTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StartBatchDeleteConfigurationTaskRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StartBatchDeleteConfigurationTask operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The limit of 200 configuration IDs per request has been exceeded.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - OperationNotPermittedException This operation is not permitted.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartBatchDeleteConfigurationTask
*/
default CompletableFuture startBatchDeleteConfigurationTask(
Consumer startBatchDeleteConfigurationTaskRequest) {
return startBatchDeleteConfigurationTask(StartBatchDeleteConfigurationTaskRequest.builder()
.applyMutation(startBatchDeleteConfigurationTaskRequest).build());
}
/**
*
* Start the continuous flow of agent's discovered data into Amazon Athena.
*
*
* @param startContinuousExportRequest
* @return A Java Future containing the result of the StartContinuousExport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictErrorException Conflict error.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartContinuousExport
*/
default CompletableFuture startContinuousExport(
StartContinuousExportRequest startContinuousExportRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Start the continuous flow of agent's discovered data into Amazon Athena.
*
*
*
* This is a convenience which creates an instance of the {@link StartContinuousExportRequest.Builder} avoiding the
* need to create one manually via {@link StartContinuousExportRequest#builder()}
*
*
* @param startContinuousExportRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StartContinuousExportRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the StartContinuousExport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictErrorException Conflict error.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartContinuousExport
*/
default CompletableFuture startContinuousExport(
Consumer startContinuousExportRequest) {
return startContinuousExport(StartContinuousExportRequest.builder().applyMutation(startContinuousExportRequest).build());
}
/**
*
* Instructs the specified agents to start collecting data.
*
*
* @param startDataCollectionByAgentIdsRequest
* @return A Java Future containing the result of the StartDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartDataCollectionByAgentIds
*/
default CompletableFuture startDataCollectionByAgentIds(
StartDataCollectionByAgentIdsRequest startDataCollectionByAgentIdsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Instructs the specified agents to start collecting data.
*
*
*
* This is a convenience which creates an instance of the {@link StartDataCollectionByAgentIdsRequest.Builder}
* avoiding the need to create one manually via {@link StartDataCollectionByAgentIdsRequest#builder()}
*
*
* @param startDataCollectionByAgentIdsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StartDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartDataCollectionByAgentIds
*/
default CompletableFuture startDataCollectionByAgentIds(
Consumer startDataCollectionByAgentIdsRequest) {
return startDataCollectionByAgentIds(StartDataCollectionByAgentIdsRequest.builder()
.applyMutation(startDataCollectionByAgentIdsRequest).build());
}
/**
*
* Begins the export of a discovered data report to an Amazon S3 bucket managed by Amazon Web Services.
*
*
*
* Exports might provide an estimate of fees and savings based on certain information that you provide. Fee
* estimates do not include any taxes that might apply. Your actual fees and savings depend on a variety of factors,
* including your actual usage of Amazon Web Services services, which might vary from the estimates provided in this
* report.
*
*
*
* If you do not specify preferences
or agentIds
in the filter, a summary of all servers,
* applications, tags, and performance is generated. This data is an aggregation of all server data collected
* through on-premises tooling, file import, application grouping and applying tags.
*
*
* If you specify agentIds
in a filter, the task exports up to 72 hours of detailed data collected by
* the identified Application Discovery Agent, including network, process, and performance details. A time range for
* exported agent data may be set by using startTime
and endTime
. Export of detailed agent
* data is limited to five concurrently running exports. Export of detailed agent data is limited to two exports per
* day.
*
*
* If you enable ec2RecommendationsPreferences
in preferences
, an Amazon EC2 instance
* matching the characteristics of each server in Application Discovery Service is generated. Changing the
* attributes of the ec2RecommendationsPreferences
changes the criteria of the recommendation.
*
*
* @param startExportTaskRequest
* @return A Java Future containing the result of the StartExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartExportTask
*/
default CompletableFuture startExportTask(StartExportTaskRequest startExportTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Begins the export of a discovered data report to an Amazon S3 bucket managed by Amazon Web Services.
*
*
*
* Exports might provide an estimate of fees and savings based on certain information that you provide. Fee
* estimates do not include any taxes that might apply. Your actual fees and savings depend on a variety of factors,
* including your actual usage of Amazon Web Services services, which might vary from the estimates provided in this
* report.
*
*
*
* If you do not specify preferences
or agentIds
in the filter, a summary of all servers,
* applications, tags, and performance is generated. This data is an aggregation of all server data collected
* through on-premises tooling, file import, application grouping and applying tags.
*
*
* If you specify agentIds
in a filter, the task exports up to 72 hours of detailed data collected by
* the identified Application Discovery Agent, including network, process, and performance details. A time range for
* exported agent data may be set by using startTime
and endTime
. Export of detailed agent
* data is limited to five concurrently running exports. Export of detailed agent data is limited to two exports per
* day.
*
*
* If you enable ec2RecommendationsPreferences
in preferences
, an Amazon EC2 instance
* matching the characteristics of each server in Application Discovery Service is generated. Changing the
* attributes of the ec2RecommendationsPreferences
changes the criteria of the recommendation.
*
*
*
* This is a convenience which creates an instance of the {@link StartExportTaskRequest.Builder} avoiding the need
* to create one manually via {@link StartExportTaskRequest#builder()}
*
*
* @param startExportTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the StartExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartExportTask
*/
default CompletableFuture startExportTask(
Consumer startExportTaskRequest) {
return startExportTask(StartExportTaskRequest.builder().applyMutation(startExportTaskRequest).build());
}
/**
*
* Begins the export of a discovered data report to an Amazon S3 bucket managed by Amazon Web Services.
*
*
*
* Exports might provide an estimate of fees and savings based on certain information that you provide. Fee
* estimates do not include any taxes that might apply. Your actual fees and savings depend on a variety of factors,
* including your actual usage of Amazon Web Services services, which might vary from the estimates provided in this
* report.
*
*
*
* If you do not specify preferences
or agentIds
in the filter, a summary of all servers,
* applications, tags, and performance is generated. This data is an aggregation of all server data collected
* through on-premises tooling, file import, application grouping and applying tags.
*
*
* If you specify agentIds
in a filter, the task exports up to 72 hours of detailed data collected by
* the identified Application Discovery Agent, including network, process, and performance details. A time range for
* exported agent data may be set by using startTime
and endTime
. Export of detailed agent
* data is limited to five concurrently running exports. Export of detailed agent data is limited to two exports per
* day.
*
*
* If you enable ec2RecommendationsPreferences
in preferences
, an Amazon EC2 instance
* matching the characteristics of each server in Application Discovery Service is generated. Changing the
* attributes of the ec2RecommendationsPreferences
changes the criteria of the recommendation.
*
*
* @return A Java Future containing the result of the StartExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartExportTask
*/
default CompletableFuture startExportTask() {
return startExportTask(StartExportTaskRequest.builder().build());
}
/**
*
* Starts an import task, which allows you to import details of your on-premises environment directly into Amazon
* Web Services Migration Hub without having to use the Amazon Web Services Application Discovery Service
* (Application Discovery Service) tools such as the Amazon Web Services Application Discovery Service Agentless
* Collector or Application Discovery Agent. This gives you the option to perform migration assessment and planning
* directly from your imported data, including the ability to group your devices as applications and track their
* migration status.
*
*
* To start an import request, do this:
*
*
* -
*
* Download the specially formatted comma separated value (CSV) import template, which you can find here: https://s3.us-west-2.amazonaws.com/templates-7cffcf56-bd96-4b1c-b45b-a5b42f282e46/import_template.csv.
*
*
* -
*
* Fill out the template with your server and application data.
*
*
* -
*
* Upload your import file to an Amazon S3 bucket, and make a note of it's Object URL. Your import file must be in
* the CSV format.
*
*
* -
*
* Use the console or the StartImportTask
command with the Amazon Web Services CLI or one of the Amazon
* Web Services SDKs to import the records from your file.
*
*
*
*
* For more information, including step-by-step procedures, see Migration Hub
* Import in the Amazon Web Services Application Discovery Service User Guide.
*
*
*
* There are limits to the number of import tasks you can create (and delete) in an Amazon Web Services account. For
* more information, see Amazon Web
* Services Application Discovery Service Limits in the Amazon Web Services Application Discovery Service
* User Guide.
*
*
*
* @param startImportTaskRequest
* @return A Java Future containing the result of the StartImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartImportTask
*/
default CompletableFuture startImportTask(StartImportTaskRequest startImportTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts an import task, which allows you to import details of your on-premises environment directly into Amazon
* Web Services Migration Hub without having to use the Amazon Web Services Application Discovery Service
* (Application Discovery Service) tools such as the Amazon Web Services Application Discovery Service Agentless
* Collector or Application Discovery Agent. This gives you the option to perform migration assessment and planning
* directly from your imported data, including the ability to group your devices as applications and track their
* migration status.
*
*
* To start an import request, do this:
*
*
* -
*
* Download the specially formatted comma separated value (CSV) import template, which you can find here: https://s3.us-west-2.amazonaws.com/templates-7cffcf56-bd96-4b1c-b45b-a5b42f282e46/import_template.csv.
*
*
* -
*
* Fill out the template with your server and application data.
*
*
* -
*
* Upload your import file to an Amazon S3 bucket, and make a note of it's Object URL. Your import file must be in
* the CSV format.
*
*
* -
*
* Use the console or the StartImportTask
command with the Amazon Web Services CLI or one of the Amazon
* Web Services SDKs to import the records from your file.
*
*
*
*
* For more information, including step-by-step procedures, see Migration Hub
* Import in the Amazon Web Services Application Discovery Service User Guide.
*
*
*
* There are limits to the number of import tasks you can create (and delete) in an Amazon Web Services account. For
* more information, see Amazon Web
* Services Application Discovery Service Limits in the Amazon Web Services Application Discovery Service
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link StartImportTaskRequest.Builder} avoiding the need
* to create one manually via {@link StartImportTaskRequest#builder()}
*
*
* @param startImportTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StartImportTaskRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the StartImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartImportTask
*/
default CompletableFuture startImportTask(
Consumer startImportTaskRequest) {
return startImportTask(StartImportTaskRequest.builder().applyMutation(startImportTaskRequest).build());
}
/**
*
* Stop the continuous flow of agent's discovered data into Amazon Athena.
*
*
* @param stopContinuousExportRequest
* @return A Java Future containing the result of the StopContinuousExport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopContinuousExport
*/
default CompletableFuture stopContinuousExport(
StopContinuousExportRequest stopContinuousExportRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stop the continuous flow of agent's discovered data into Amazon Athena.
*
*
*
* This is a convenience which creates an instance of the {@link StopContinuousExportRequest.Builder} avoiding the
* need to create one manually via {@link StopContinuousExportRequest#builder()}
*
*
* @param stopContinuousExportRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StopContinuousExportRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the StopContinuousExport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopContinuousExport
*/
default CompletableFuture stopContinuousExport(
Consumer stopContinuousExportRequest) {
return stopContinuousExport(StopContinuousExportRequest.builder().applyMutation(stopContinuousExportRequest).build());
}
/**
*
* Instructs the specified agents to stop collecting data.
*
*
* @param stopDataCollectionByAgentIdsRequest
* @return A Java Future containing the result of the StopDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopDataCollectionByAgentIds
*/
default CompletableFuture stopDataCollectionByAgentIds(
StopDataCollectionByAgentIdsRequest stopDataCollectionByAgentIdsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Instructs the specified agents to stop collecting data.
*
*
*
* This is a convenience which creates an instance of the {@link StopDataCollectionByAgentIdsRequest.Builder}
* avoiding the need to create one manually via {@link StopDataCollectionByAgentIdsRequest#builder()}
*
*
* @param stopDataCollectionByAgentIdsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StopDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopDataCollectionByAgentIds
*/
default CompletableFuture stopDataCollectionByAgentIds(
Consumer stopDataCollectionByAgentIdsRequest) {
return stopDataCollectionByAgentIds(StopDataCollectionByAgentIdsRequest.builder()
.applyMutation(stopDataCollectionByAgentIdsRequest).build());
}
/**
*
* Updates metadata about an application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.UpdateApplication
*/
default CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates metadata about an application.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link UpdateApplicationRequest#builder()}
*
*
* @param updateApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.UpdateApplication
*/
default CompletableFuture updateApplication(
Consumer updateApplicationRequest) {
return updateApplication(UpdateApplicationRequest.builder().applyMutation(updateApplicationRequest).build());
}
@Override
default ApplicationDiscoveryServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link ApplicationDiscoveryAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ApplicationDiscoveryAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ApplicationDiscoveryAsyncClient}.
*/
static ApplicationDiscoveryAsyncClientBuilder builder() {
return new DefaultApplicationDiscoveryAsyncClientBuilder();
}
}