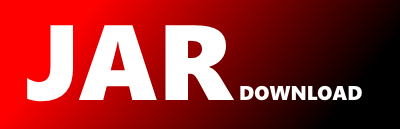
software.amazon.awssdk.services.applicationdiscovery.DefaultApplicationDiscoveryAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationdiscovery;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.applicationdiscovery.internal.ApplicationDiscoveryServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.applicationdiscovery.model.ApplicationDiscoveryException;
import software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.AuthorizationErrorException;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteAgentsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteAgentsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteImportDataRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.BatchDeleteImportDataResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.ConflictErrorException;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeBatchDeleteConfigurationTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeBatchDeleteConfigurationTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeContinuousExportsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeImportTasksResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.HomeRegionNotSetException;
import software.amazon.awssdk.services.applicationdiscovery.model.InvalidParameterException;
import software.amazon.awssdk.services.applicationdiscovery.model.InvalidParameterValueException;
import software.amazon.awssdk.services.applicationdiscovery.model.LimitExceededException;
import software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.OperationNotPermittedException;
import software.amazon.awssdk.services.applicationdiscovery.model.ResourceInUseException;
import software.amazon.awssdk.services.applicationdiscovery.model.ResourceNotFoundException;
import software.amazon.awssdk.services.applicationdiscovery.model.ServerInternalErrorException;
import software.amazon.awssdk.services.applicationdiscovery.model.StartBatchDeleteConfigurationTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartBatchDeleteConfigurationTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartContinuousExportRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartContinuousExportResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartImportTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartImportTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StopContinuousExportRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StopContinuousExportResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.transform.AssociateConfigurationItemsToApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.BatchDeleteAgentsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.BatchDeleteImportDataRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.CreateTagsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DeleteApplicationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DeleteTagsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeAgentsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeBatchDeleteConfigurationTaskRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeContinuousExportsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeExportTasksRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeImportTasksRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeTagsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DisassociateConfigurationItemsFromApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.GetDiscoverySummaryRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ListConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ListServerNeighborsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartBatchDeleteConfigurationTaskRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartContinuousExportRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartDataCollectionByAgentIdsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartExportTaskRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartImportTaskRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StopContinuousExportRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StopDataCollectionByAgentIdsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ApplicationDiscoveryAsyncClient}.
*
* @see ApplicationDiscoveryAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultApplicationDiscoveryAsyncClient implements ApplicationDiscoveryAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultApplicationDiscoveryAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultApplicationDiscoveryAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Associates one or more configuration items with an application.
*
*
* @param associateConfigurationItemsToApplicationRequest
* @return A Java Future containing the result of the AssociateConfigurationItemsToApplication operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.AssociateConfigurationItemsToApplication
*/
@Override
public CompletableFuture associateConfigurationItemsToApplication(
AssociateConfigurationItemsToApplicationRequest associateConfigurationItemsToApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
associateConfigurationItemsToApplicationRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateConfigurationItemsToApplicationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateConfigurationItemsToApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, AssociateConfigurationItemsToApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateConfigurationItemsToApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateConfigurationItemsToApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateConfigurationItemsToApplicationRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes one or more agents or collectors as specified by ID. Deleting an agent or collector does not delete the
* previously discovered data. To delete the data collected, use StartBatchDeleteConfigurationTask
.
*
*
* @param batchDeleteAgentsRequest
* @return A Java Future containing the result of the BatchDeleteAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.BatchDeleteAgents
*/
@Override
public CompletableFuture batchDeleteAgents(BatchDeleteAgentsRequest batchDeleteAgentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteAgentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteAgentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteAgents");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteAgentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteAgents").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeleteAgentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeleteAgentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes one or more import tasks, each identified by their import ID. Each import task has a number of records
* that can identify servers or applications.
*
*
* Amazon Web Services Application Discovery Service has built-in matching logic that will identify when discovered
* servers match existing entries that you've previously discovered, the information for the already-existing
* discovered server is updated. When you delete an import task that contains records that were used to match, the
* information in those matched records that comes from the deleted records will also be deleted.
*
*
* @param batchDeleteImportDataRequest
* @return A Java Future containing the result of the BatchDeleteImportData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.BatchDeleteImportData
*/
@Override
public CompletableFuture batchDeleteImportData(
BatchDeleteImportDataRequest batchDeleteImportDataRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteImportDataRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteImportDataRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteImportData");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteImportDataResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteImportData").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeleteImportDataRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeleteImportDataRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an application with the given name and description.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateApplication
*/
@Override
public CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates one or more tags for configuration items. Tags are metadata that help you categorize IT assets. This API
* accepts a list of multiple configuration items.
*
*
*
* Do not store sensitive information (like personal data) in tags.
*
*
*
* @param createTagsRequest
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateTags
*/
@Override
public CompletableFuture createTags(CreateTagsRequest createTagsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTags");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateTags")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createTagsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a list of applications and their associations with configuration items.
*
*
* @param deleteApplicationsRequest
* @return A Java Future containing the result of the DeleteApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteApplications
*/
@Override
public CompletableFuture deleteApplications(DeleteApplicationsRequest deleteApplicationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteApplicationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApplicationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApplications");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApplicationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApplications").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteApplicationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteApplicationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the association between configuration items and one or more tags. This API accepts a list of multiple
* configuration items.
*
*
* @param deleteTagsRequest
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteTags
*/
@Override
public CompletableFuture deleteTags(DeleteTagsRequest deleteTagsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTags");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteTags")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteTagsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists agents or collectors as specified by ID or other filters. All agents/collectors associated with your user
* can be listed if you call DescribeAgents
as is without passing any parameters.
*
*
* @param describeAgentsRequest
* @return A Java Future containing the result of the DescribeAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
@Override
public CompletableFuture describeAgents(DescribeAgentsRequest describeAgentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAgentsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAgentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAgents");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAgentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAgents").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAgentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAgentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Takes a unique deletion task identifier as input and returns metadata about a configuration deletion task.
*
*
* @param describeBatchDeleteConfigurationTaskRequest
* @return A Java Future containing the result of the DescribeBatchDeleteConfigurationTask operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeBatchDeleteConfigurationTask
*/
@Override
public CompletableFuture describeBatchDeleteConfigurationTask(
DescribeBatchDeleteConfigurationTaskRequest describeBatchDeleteConfigurationTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeBatchDeleteConfigurationTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeBatchDeleteConfigurationTaskRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeBatchDeleteConfigurationTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DescribeBatchDeleteConfigurationTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeBatchDeleteConfigurationTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeBatchDeleteConfigurationTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeBatchDeleteConfigurationTaskRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves attributes for a list of configuration item IDs.
*
*
*
* All of the supplied IDs must be for the same asset type from one of the following:
*
*
* -
*
* server
*
*
* -
*
* application
*
*
* -
*
* process
*
*
* -
*
* connection
*
*
*
*
* Output fields are specific to the asset type specified. For example, the output for a server configuration
* item includes a list of attributes about the server, such as host name, operating system, number of network
* cards, etc.
*
*
* For a complete list of outputs for each asset type, see Using the DescribeConfigurations Action in the Amazon Web Services Application Discovery Service User
* Guide.
*
*
*
* @param describeConfigurationsRequest
* @return A Java Future containing the result of the DescribeConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeConfigurations
*/
@Override
public CompletableFuture describeConfigurations(
DescribeConfigurationsRequest describeConfigurationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeConfigurationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeConfigurations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeConfigurations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeConfigurationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists exports as specified by ID. All continuous exports associated with your user can be listed if you call
* DescribeContinuousExports
as is without passing any parameters.
*
*
* @param describeContinuousExportsRequest
* @return A Java Future containing the result of the DescribeContinuousExports operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeContinuousExports
*/
@Override
public CompletableFuture describeContinuousExports(
DescribeContinuousExportsRequest describeContinuousExportsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeContinuousExportsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeContinuousExportsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeContinuousExports");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeContinuousExportsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeContinuousExports").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeContinuousExportsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeContinuousExportsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve status of one or more export tasks. You can retrieve the status of up to 100 export tasks.
*
*
* @param describeExportTasksRequest
* @return A Java Future containing the result of the DescribeExportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
@Override
public CompletableFuture describeExportTasks(
DescribeExportTasksRequest describeExportTasksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeExportTasksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeExportTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeExportTasks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeExportTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeExportTasks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeExportTasksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeExportTasksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of import tasks for your account, including status information, times, IDs, the Amazon S3 Object
* URL for the import file, and more.
*
*
* @param describeImportTasksRequest
* @return A Java Future containing the result of the DescribeImportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeImportTasks
*/
@Override
public CompletableFuture describeImportTasks(
DescribeImportTasksRequest describeImportTasksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeImportTasksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeImportTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeImportTasks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeImportTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeImportTasks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeImportTasksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeImportTasksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of configuration items that have tags as specified by the key-value pairs, name and value,
* passed to the optional parameter filters
.
*
*
* There are three valid tag filter names:
*
*
* -
*
* tagKey
*
*
* -
*
* tagValue
*
*
* -
*
* configurationId
*
*
*
*
* Also, all configuration items associated with your user that have tags can be listed if you call
* DescribeTags
as is without passing any parameters.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
@Override
public CompletableFuture describeTags(DescribeTagsRequest describeTagsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTags");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTags").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeTagsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disassociates one or more configuration items from an application.
*
*
* @param disassociateConfigurationItemsFromApplicationRequest
* @return A Java Future containing the result of the DisassociateConfigurationItemsFromApplication operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DisassociateConfigurationItemsFromApplication
*/
@Override
public CompletableFuture disassociateConfigurationItemsFromApplication(
DisassociateConfigurationItemsFromApplicationRequest disassociateConfigurationItemsFromApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
disassociateConfigurationItemsFromApplicationRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateConfigurationItemsFromApplicationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateConfigurationItemsFromApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DisassociateConfigurationItemsFromApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateConfigurationItemsFromApplication")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateConfigurationItemsFromApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateConfigurationItemsFromApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((
r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a short summary of discovered assets.
*
*
* This API operation takes no request parameters and is called as is at the command prompt as shown in the example.
*
*
* @param getDiscoverySummaryRequest
* @return A Java Future containing the result of the GetDiscoverySummary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.GetDiscoverySummary
*/
@Override
public CompletableFuture getDiscoverySummary(
GetDiscoverySummaryRequest getDiscoverySummaryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDiscoverySummaryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDiscoverySummaryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDiscoverySummary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDiscoverySummaryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDiscoverySummary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDiscoverySummaryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDiscoverySummaryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of configuration items as specified by the value passed to the required parameter
* configurationType
. Optional filtering may be applied to refine search results.
*
*
* @param listConfigurationsRequest
* @return A Java Future containing the result of the ListConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListConfigurations
*/
@Override
public CompletableFuture listConfigurations(ListConfigurationsRequest listConfigurationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConfigurationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConfigurations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListConfigurations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listConfigurationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of servers that are one network hop away from a specified server.
*
*
* @param listServerNeighborsRequest
* @return A Java Future containing the result of the ListServerNeighbors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListServerNeighbors
*/
@Override
public CompletableFuture listServerNeighbors(
ListServerNeighborsRequest listServerNeighborsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listServerNeighborsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listServerNeighborsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListServerNeighbors");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListServerNeighborsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListServerNeighbors").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListServerNeighborsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listServerNeighborsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Takes a list of configurationId as input and starts an asynchronous deletion task to remove the
* configurationItems. Returns a unique deletion task identifier.
*
*
* @param startBatchDeleteConfigurationTaskRequest
* @return A Java Future containing the result of the StartBatchDeleteConfigurationTask operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The limit of 200 configuration IDs per request has been exceeded.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - OperationNotPermittedException This operation is not permitted.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartBatchDeleteConfigurationTask
*/
@Override
public CompletableFuture startBatchDeleteConfigurationTask(
StartBatchDeleteConfigurationTaskRequest startBatchDeleteConfigurationTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startBatchDeleteConfigurationTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
startBatchDeleteConfigurationTaskRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartBatchDeleteConfigurationTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, StartBatchDeleteConfigurationTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartBatchDeleteConfigurationTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartBatchDeleteConfigurationTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startBatchDeleteConfigurationTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Start the continuous flow of agent's discovered data into Amazon Athena.
*
*
* @param startContinuousExportRequest
* @return A Java Future containing the result of the StartContinuousExport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictErrorException Conflict error.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartContinuousExport
*/
@Override
public CompletableFuture startContinuousExport(
StartContinuousExportRequest startContinuousExportRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startContinuousExportRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startContinuousExportRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartContinuousExport");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartContinuousExportResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartContinuousExport").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartContinuousExportRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startContinuousExportRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Instructs the specified agents to start collecting data.
*
*
* @param startDataCollectionByAgentIdsRequest
* @return A Java Future containing the result of the StartDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartDataCollectionByAgentIds
*/
@Override
public CompletableFuture startDataCollectionByAgentIds(
StartDataCollectionByAgentIdsRequest startDataCollectionByAgentIdsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startDataCollectionByAgentIdsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
startDataCollectionByAgentIdsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartDataCollectionByAgentIds");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartDataCollectionByAgentIdsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartDataCollectionByAgentIds").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartDataCollectionByAgentIdsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startDataCollectionByAgentIdsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Begins the export of a discovered data report to an Amazon S3 bucket managed by Amazon Web Services.
*
*
*
* Exports might provide an estimate of fees and savings based on certain information that you provide. Fee
* estimates do not include any taxes that might apply. Your actual fees and savings depend on a variety of factors,
* including your actual usage of Amazon Web Services services, which might vary from the estimates provided in this
* report.
*
*
*
* If you do not specify preferences
or agentIds
in the filter, a summary of all servers,
* applications, tags, and performance is generated. This data is an aggregation of all server data collected
* through on-premises tooling, file import, application grouping and applying tags.
*
*
* If you specify agentIds
in a filter, the task exports up to 72 hours of detailed data collected by
* the identified Application Discovery Agent, including network, process, and performance details. A time range for
* exported agent data may be set by using startTime
and endTime
. Export of detailed agent
* data is limited to five concurrently running exports. Export of detailed agent data is limited to two exports per
* day.
*
*
* If you enable ec2RecommendationsPreferences
in preferences
, an Amazon EC2 instance
* matching the characteristics of each server in Application Discovery Service is generated. Changing the
* attributes of the ec2RecommendationsPreferences
changes the criteria of the recommendation.
*
*
* @param startExportTaskRequest
* @return A Java Future containing the result of the StartExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartExportTask
*/
@Override
public CompletableFuture startExportTask(StartExportTaskRequest startExportTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startExportTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startExportTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartExportTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartExportTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartExportTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartExportTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startExportTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts an import task, which allows you to import details of your on-premises environment directly into Amazon
* Web Services Migration Hub without having to use the Amazon Web Services Application Discovery Service
* (Application Discovery Service) tools such as the Amazon Web Services Application Discovery Service Agentless
* Collector or Application Discovery Agent. This gives you the option to perform migration assessment and planning
* directly from your imported data, including the ability to group your devices as applications and track their
* migration status.
*
*
* To start an import request, do this:
*
*
* -
*
* Download the specially formatted comma separated value (CSV) import template, which you can find here: https://s3.us-west-2.amazonaws.com/templates-7cffcf56-bd96-4b1c-b45b-a5b42f282e46/import_template.csv.
*
*
* -
*
* Fill out the template with your server and application data.
*
*
* -
*
* Upload your import file to an Amazon S3 bucket, and make a note of it's Object URL. Your import file must be in
* the CSV format.
*
*
* -
*
* Use the console or the StartImportTask
command with the Amazon Web Services CLI or one of the Amazon
* Web Services SDKs to import the records from your file.
*
*
*
*
* For more information, including step-by-step procedures, see Migration Hub
* Import in the Amazon Web Services Application Discovery Service User Guide.
*
*
*
* There are limits to the number of import tasks you can create (and delete) in an Amazon Web Services account. For
* more information, see Amazon Web
* Services Application Discovery Service Limits in the Amazon Web Services Application Discovery Service
* User Guide.
*
*
*
* @param startImportTaskRequest
* @return A Java Future containing the result of the StartImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartImportTask
*/
@Override
public CompletableFuture startImportTask(StartImportTaskRequest startImportTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startImportTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startImportTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartImportTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartImportTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartImportTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartImportTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startImportTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Stop the continuous flow of agent's discovered data into Amazon Athena.
*
*
* @param stopContinuousExportRequest
* @return A Java Future containing the result of the StopContinuousExport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - ResourceInUseException This issue occurs when the same
clientRequestToken
is used with
* the StartImportTask
action, but with different parameters. For example, you use the same
* request token but have two different import URLs, you can encounter this issue. If the import tasks are
* meant to be different, use a different clientRequestToken
, and try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopContinuousExport
*/
@Override
public CompletableFuture stopContinuousExport(
StopContinuousExportRequest stopContinuousExportRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(stopContinuousExportRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopContinuousExportRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopContinuousExport");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StopContinuousExportResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StopContinuousExport").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StopContinuousExportRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(stopContinuousExportRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Instructs the specified agents to stop collecting data.
*
*
* @param stopDataCollectionByAgentIdsRequest
* @return A Java Future containing the result of the StopDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopDataCollectionByAgentIds
*/
@Override
public CompletableFuture stopDataCollectionByAgentIds(
StopDataCollectionByAgentIdsRequest stopDataCollectionByAgentIdsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(stopDataCollectionByAgentIdsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopDataCollectionByAgentIdsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopDataCollectionByAgentIds");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StopDataCollectionByAgentIdsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StopDataCollectionByAgentIds").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StopDataCollectionByAgentIdsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(stopDataCollectionByAgentIdsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates metadata about an application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthorizationErrorException The user does not have permission to perform the action. Check the IAM
* policy associated with this user.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - HomeRegionNotSetException The home Region is not set. Set the home Region to continue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.UpdateApplication
*/
@Override
public CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Application Discovery Service");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final ApplicationDiscoveryServiceClientConfiguration serviceClientConfiguration() {
return new ApplicationDiscoveryServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(ApplicationDiscoveryException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServerInternalErrorException")
.exceptionBuilderSupplier(ServerInternalErrorException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterException")
.exceptionBuilderSupplier(InvalidParameterException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterValueException")
.exceptionBuilderSupplier(InvalidParameterValueException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceInUseException")
.exceptionBuilderSupplier(ResourceInUseException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictErrorException")
.exceptionBuilderSupplier(ConflictErrorException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OperationNotPermittedException")
.exceptionBuilderSupplier(OperationNotPermittedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("HomeRegionNotSetException")
.exceptionBuilderSupplier(HomeRegionNotSetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AuthorizationErrorException")
.exceptionBuilderSupplier(AuthorizationErrorException::builder).httpStatusCode(400).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
ApplicationDiscoveryServiceClientConfigurationBuilder serviceConfigBuilder = new ApplicationDiscoveryServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata, Function> exceptionMetadataMapper) {
return protocolFactory.createErrorResponseHandler(operationMetadata, exceptionMetadataMapper);
}
@Override
public void close() {
clientHandler.close();
}
}