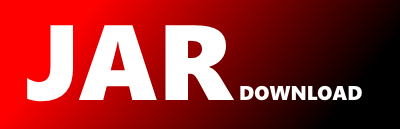
software.amazon.awssdk.services.apprunner.model.ImageRepository Maven / Gradle / Ivy
Show all versions of apprunner Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.apprunner.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a source image repository.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ImageRepository implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField IMAGE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageIdentifier").getter(getter(ImageRepository::imageIdentifier))
.setter(setter(Builder::imageIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageIdentifier").build()).build();
private static final SdkField IMAGE_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ImageConfiguration")
.getter(getter(ImageRepository::imageConfiguration)).setter(setter(Builder::imageConfiguration))
.constructor(ImageConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageConfiguration").build())
.build();
private static final SdkField IMAGE_REPOSITORY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageRepositoryType").getter(getter(ImageRepository::imageRepositoryTypeAsString))
.setter(setter(Builder::imageRepositoryType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageRepositoryType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IMAGE_IDENTIFIER_FIELD,
IMAGE_CONFIGURATION_FIELD, IMAGE_REPOSITORY_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String imageIdentifier;
private final ImageConfiguration imageConfiguration;
private final String imageRepositoryType;
private ImageRepository(BuilderImpl builder) {
this.imageIdentifier = builder.imageIdentifier;
this.imageConfiguration = builder.imageConfiguration;
this.imageRepositoryType = builder.imageRepositoryType;
}
/**
*
* The identifier of an image.
*
*
* For an image in Amazon Elastic Container Registry (Amazon ECR), this is an image name. For the image name format,
* see Pulling an
* image in the Amazon ECR User Guide.
*
*
* @return The identifier of an image.
*
* For an image in Amazon Elastic Container Registry (Amazon ECR), this is an image name. For the image name
* format, see Pulling an
* image in the Amazon ECR User Guide.
*/
public final String imageIdentifier() {
return imageIdentifier;
}
/**
*
* Configuration for running the identified image.
*
*
* @return Configuration for running the identified image.
*/
public final ImageConfiguration imageConfiguration() {
return imageConfiguration;
}
/**
*
* The type of the image repository. This reflects the repository provider and whether the repository is private or
* public.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageRepositoryType} will return {@link ImageRepositoryType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #imageRepositoryTypeAsString}.
*
*
* @return The type of the image repository. This reflects the repository provider and whether the repository is
* private or public.
* @see ImageRepositoryType
*/
public final ImageRepositoryType imageRepositoryType() {
return ImageRepositoryType.fromValue(imageRepositoryType);
}
/**
*
* The type of the image repository. This reflects the repository provider and whether the repository is private or
* public.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageRepositoryType} will return {@link ImageRepositoryType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #imageRepositoryTypeAsString}.
*
*
* @return The type of the image repository. This reflects the repository provider and whether the repository is
* private or public.
* @see ImageRepositoryType
*/
public final String imageRepositoryTypeAsString() {
return imageRepositoryType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(imageIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(imageConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(imageRepositoryTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ImageRepository)) {
return false;
}
ImageRepository other = (ImageRepository) obj;
return Objects.equals(imageIdentifier(), other.imageIdentifier())
&& Objects.equals(imageConfiguration(), other.imageConfiguration())
&& Objects.equals(imageRepositoryTypeAsString(), other.imageRepositoryTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ImageRepository").add("ImageIdentifier", imageIdentifier())
.add("ImageConfiguration", imageConfiguration()).add("ImageRepositoryType", imageRepositoryTypeAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ImageIdentifier":
return Optional.ofNullable(clazz.cast(imageIdentifier()));
case "ImageConfiguration":
return Optional.ofNullable(clazz.cast(imageConfiguration()));
case "ImageRepositoryType":
return Optional.ofNullable(clazz.cast(imageRepositoryTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function