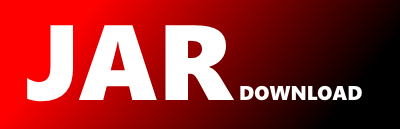
software.amazon.awssdk.services.apprunner.DefaultAppRunnerClient Maven / Gradle / Ivy
Show all versions of apprunner Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.apprunner;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.apprunner.internal.AppRunnerServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.apprunner.model.AppRunnerException;
import software.amazon.awssdk.services.apprunner.model.AssociateCustomDomainRequest;
import software.amazon.awssdk.services.apprunner.model.AssociateCustomDomainResponse;
import software.amazon.awssdk.services.apprunner.model.CreateAutoScalingConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.CreateAutoScalingConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.CreateConnectionRequest;
import software.amazon.awssdk.services.apprunner.model.CreateConnectionResponse;
import software.amazon.awssdk.services.apprunner.model.CreateObservabilityConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.CreateObservabilityConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.CreateServiceRequest;
import software.amazon.awssdk.services.apprunner.model.CreateServiceResponse;
import software.amazon.awssdk.services.apprunner.model.CreateVpcConnectorRequest;
import software.amazon.awssdk.services.apprunner.model.CreateVpcConnectorResponse;
import software.amazon.awssdk.services.apprunner.model.CreateVpcIngressConnectionRequest;
import software.amazon.awssdk.services.apprunner.model.CreateVpcIngressConnectionResponse;
import software.amazon.awssdk.services.apprunner.model.DeleteAutoScalingConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.DeleteAutoScalingConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.DeleteConnectionRequest;
import software.amazon.awssdk.services.apprunner.model.DeleteConnectionResponse;
import software.amazon.awssdk.services.apprunner.model.DeleteObservabilityConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.DeleteObservabilityConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.DeleteServiceRequest;
import software.amazon.awssdk.services.apprunner.model.DeleteServiceResponse;
import software.amazon.awssdk.services.apprunner.model.DeleteVpcConnectorRequest;
import software.amazon.awssdk.services.apprunner.model.DeleteVpcConnectorResponse;
import software.amazon.awssdk.services.apprunner.model.DeleteVpcIngressConnectionRequest;
import software.amazon.awssdk.services.apprunner.model.DeleteVpcIngressConnectionResponse;
import software.amazon.awssdk.services.apprunner.model.DescribeAutoScalingConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.DescribeAutoScalingConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.DescribeCustomDomainsRequest;
import software.amazon.awssdk.services.apprunner.model.DescribeCustomDomainsResponse;
import software.amazon.awssdk.services.apprunner.model.DescribeObservabilityConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.DescribeObservabilityConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.DescribeServiceRequest;
import software.amazon.awssdk.services.apprunner.model.DescribeServiceResponse;
import software.amazon.awssdk.services.apprunner.model.DescribeVpcConnectorRequest;
import software.amazon.awssdk.services.apprunner.model.DescribeVpcConnectorResponse;
import software.amazon.awssdk.services.apprunner.model.DescribeVpcIngressConnectionRequest;
import software.amazon.awssdk.services.apprunner.model.DescribeVpcIngressConnectionResponse;
import software.amazon.awssdk.services.apprunner.model.DisassociateCustomDomainRequest;
import software.amazon.awssdk.services.apprunner.model.DisassociateCustomDomainResponse;
import software.amazon.awssdk.services.apprunner.model.InternalServiceErrorException;
import software.amazon.awssdk.services.apprunner.model.InvalidRequestException;
import software.amazon.awssdk.services.apprunner.model.InvalidStateException;
import software.amazon.awssdk.services.apprunner.model.ListAutoScalingConfigurationsRequest;
import software.amazon.awssdk.services.apprunner.model.ListAutoScalingConfigurationsResponse;
import software.amazon.awssdk.services.apprunner.model.ListConnectionsRequest;
import software.amazon.awssdk.services.apprunner.model.ListConnectionsResponse;
import software.amazon.awssdk.services.apprunner.model.ListObservabilityConfigurationsRequest;
import software.amazon.awssdk.services.apprunner.model.ListObservabilityConfigurationsResponse;
import software.amazon.awssdk.services.apprunner.model.ListOperationsRequest;
import software.amazon.awssdk.services.apprunner.model.ListOperationsResponse;
import software.amazon.awssdk.services.apprunner.model.ListServicesForAutoScalingConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.ListServicesForAutoScalingConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.ListServicesRequest;
import software.amazon.awssdk.services.apprunner.model.ListServicesResponse;
import software.amazon.awssdk.services.apprunner.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.apprunner.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.apprunner.model.ListVpcConnectorsRequest;
import software.amazon.awssdk.services.apprunner.model.ListVpcConnectorsResponse;
import software.amazon.awssdk.services.apprunner.model.ListVpcIngressConnectionsRequest;
import software.amazon.awssdk.services.apprunner.model.ListVpcIngressConnectionsResponse;
import software.amazon.awssdk.services.apprunner.model.PauseServiceRequest;
import software.amazon.awssdk.services.apprunner.model.PauseServiceResponse;
import software.amazon.awssdk.services.apprunner.model.ResourceNotFoundException;
import software.amazon.awssdk.services.apprunner.model.ResumeServiceRequest;
import software.amazon.awssdk.services.apprunner.model.ResumeServiceResponse;
import software.amazon.awssdk.services.apprunner.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.apprunner.model.StartDeploymentRequest;
import software.amazon.awssdk.services.apprunner.model.StartDeploymentResponse;
import software.amazon.awssdk.services.apprunner.model.TagResourceRequest;
import software.amazon.awssdk.services.apprunner.model.TagResourceResponse;
import software.amazon.awssdk.services.apprunner.model.UntagResourceRequest;
import software.amazon.awssdk.services.apprunner.model.UntagResourceResponse;
import software.amazon.awssdk.services.apprunner.model.UpdateDefaultAutoScalingConfigurationRequest;
import software.amazon.awssdk.services.apprunner.model.UpdateDefaultAutoScalingConfigurationResponse;
import software.amazon.awssdk.services.apprunner.model.UpdateServiceRequest;
import software.amazon.awssdk.services.apprunner.model.UpdateServiceResponse;
import software.amazon.awssdk.services.apprunner.model.UpdateVpcIngressConnectionRequest;
import software.amazon.awssdk.services.apprunner.model.UpdateVpcIngressConnectionResponse;
import software.amazon.awssdk.services.apprunner.transform.AssociateCustomDomainRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.CreateAutoScalingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.CreateConnectionRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.CreateObservabilityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.CreateServiceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.CreateVpcConnectorRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.CreateVpcIngressConnectionRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DeleteAutoScalingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DeleteConnectionRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DeleteObservabilityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DeleteServiceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DeleteVpcConnectorRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DeleteVpcIngressConnectionRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DescribeAutoScalingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DescribeCustomDomainsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DescribeObservabilityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DescribeServiceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DescribeVpcConnectorRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DescribeVpcIngressConnectionRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.DisassociateCustomDomainRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListAutoScalingConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListConnectionsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListObservabilityConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListOperationsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListServicesForAutoScalingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListServicesRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListVpcConnectorsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ListVpcIngressConnectionsRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.PauseServiceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.ResumeServiceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.StartDeploymentRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.UpdateDefaultAutoScalingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.UpdateServiceRequestMarshaller;
import software.amazon.awssdk.services.apprunner.transform.UpdateVpcIngressConnectionRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link AppRunnerClient}.
*
* @see AppRunnerClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultAppRunnerClient implements AppRunnerClient {
private static final Logger log = Logger.loggerFor(DefaultAppRunnerClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultAppRunnerClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Associate your own domain name with the App Runner subdomain URL of your App Runner service.
*
*
* After you call AssociateCustomDomain
and receive a successful response, use the information in the
* CustomDomain record that's returned to add CNAME records to your Domain Name System (DNS). For each mapped
* domain name, add a mapping to the target App Runner subdomain and one or more certificate validation records. App
* Runner then performs DNS validation to verify that you own or control the domain name that you associated. App
* Runner tracks domain validity in a certificate stored in AWS Certificate Manager (ACM).
*
*
* @param associateCustomDomainRequest
* @return Result of the AssociateCustomDomain operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.AssociateCustomDomain
* @see AWS API Documentation
*/
@Override
public AssociateCustomDomainResponse associateCustomDomain(AssociateCustomDomainRequest associateCustomDomainRequest)
throws InvalidRequestException, InternalServiceErrorException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateCustomDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateCustomDomainRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateCustomDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateCustomDomain");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateCustomDomain").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateCustomDomainRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateCustomDomainRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Create an App Runner automatic scaling configuration resource. App Runner requires this resource when you create
* or update App Runner services and you require non-default auto scaling settings. You can share an auto scaling
* configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* AutoScalingConfigurationName
. The call returns incremental
* AutoScalingConfigurationRevision
values. When you create a service and configure an auto scaling
* configuration resource, the service uses the latest active revision of the auto scaling configuration by default.
* You can optionally configure the service to use a specific revision.
*
*
* Configure a higher MinSize
to increase the spread of your App Runner service over more Availability
* Zones in the Amazon Web Services Region. The tradeoff is a higher minimal cost.
*
*
* Configure a lower MaxSize
to control your cost. The tradeoff is lower responsiveness during peak
* demand.
*
*
* @param createAutoScalingConfigurationRequest
* @return Result of the CreateAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.CreateAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public CreateAutoScalingConfigurationResponse createAutoScalingConfiguration(
CreateAutoScalingConfigurationRequest createAutoScalingConfigurationRequest) throws InvalidRequestException,
InternalServiceErrorException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAutoScalingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAutoScalingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createAutoScalingConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAutoScalingConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAutoScalingConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createAutoScalingConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAutoScalingConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Create an App Runner connection resource. App Runner requires a connection resource when you create App Runner
* services that access private repositories from certain third-party providers. You can share a connection across
* multiple services.
*
*
* A connection resource is needed to access GitHub and Bitbucket repositories. Both require a user interface
* approval process through the App Runner console before you can use the connection.
*
*
* @param createConnectionRequest
* @return Result of the CreateConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.CreateConnection
* @see AWS
* API Documentation
*/
@Override
public CreateConnectionResponse createConnection(CreateConnectionRequest createConnectionRequest)
throws InvalidRequestException, InternalServiceErrorException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConnection");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateConnection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Create an App Runner observability configuration resource. App Runner requires this resource when you create or
* update App Runner services and you want to enable non-default observability features. You can share an
* observability configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* ObservabilityConfigurationName
. The call returns incremental
* ObservabilityConfigurationRevision
values. When you create a service and configure an observability
* configuration resource, the service uses the latest active revision of the observability configuration by
* default. You can optionally configure the service to use a specific revision.
*
*
* The observability configuration resource is designed to configure multiple features (currently one feature,
* tracing). This action takes optional parameters that describe the configuration of these features (currently one
* parameter, TraceConfiguration
). If you don't specify a feature parameter, App Runner doesn't enable
* the feature.
*
*
* @param createObservabilityConfigurationRequest
* @return Result of the CreateObservabilityConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.CreateObservabilityConfiguration
* @see AWS API Documentation
*/
@Override
public CreateObservabilityConfigurationResponse createObservabilityConfiguration(
CreateObservabilityConfigurationRequest createObservabilityConfigurationRequest) throws InvalidRequestException,
InternalServiceErrorException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateObservabilityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createObservabilityConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createObservabilityConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateObservabilityConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateObservabilityConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createObservabilityConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateObservabilityConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Create an App Runner service. After the service is created, the action also automatically starts a deployment.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to
* track the operation's progress.
*
*
* @param createServiceRequest
* @return Result of the CreateService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.CreateService
* @see AWS API
* Documentation
*/
@Override
public CreateServiceResponse createService(CreateServiceRequest createServiceRequest) throws InvalidRequestException,
InternalServiceErrorException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Create an App Runner VPC connector resource. App Runner requires this resource when you want to associate your
* App Runner service to a custom Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createVpcConnectorRequest
* @return Result of the CreateVpcConnector operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.CreateVpcConnector
* @see AWS
* API Documentation
*/
@Override
public CreateVpcConnectorResponse createVpcConnector(CreateVpcConnectorRequest createVpcConnectorRequest)
throws InvalidRequestException, InternalServiceErrorException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVpcConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createVpcConnectorRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVpcConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVpcConnector");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateVpcConnector").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createVpcConnectorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateVpcConnectorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Create an App Runner VPC Ingress Connection resource. App Runner requires this resource when you want to
* associate your App Runner service with an Amazon VPC endpoint.
*
*
* @param createVpcIngressConnectionRequest
* @return Result of the CreateVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.CreateVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public CreateVpcIngressConnectionResponse createVpcIngressConnection(
CreateVpcIngressConnectionRequest createVpcIngressConnectionRequest) throws InvalidRequestException,
InvalidStateException, InternalServiceErrorException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVpcIngressConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createVpcIngressConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVpcIngressConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVpcIngressConnection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVpcIngressConnection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createVpcIngressConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateVpcIngressConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Delete an App Runner automatic scaling configuration resource. You can delete a top level auto scaling
* configuration, a specific revision of one, or all revisions associated with the top level configuration. You
* can't delete the default auto scaling configuration or a configuration that's used by one or more App Runner
* services.
*
*
* @param deleteAutoScalingConfigurationRequest
* @return Result of the DeleteAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DeleteAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteAutoScalingConfigurationResponse deleteAutoScalingConfiguration(
DeleteAutoScalingConfigurationRequest deleteAutoScalingConfigurationRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAutoScalingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAutoScalingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteAutoScalingConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAutoScalingConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAutoScalingConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteAutoScalingConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAutoScalingConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Delete an App Runner connection. You must first ensure that there are no running App Runner services that use
* this connection. If there are any, the DeleteConnection
action fails.
*
*
* @param deleteConnectionRequest
* @return Result of the DeleteConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DeleteConnection
* @see AWS
* API Documentation
*/
@Override
public DeleteConnectionResponse deleteConnection(DeleteConnectionRequest deleteConnectionRequest)
throws InvalidRequestException, ResourceNotFoundException, InternalServiceErrorException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConnection");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteConnection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Delete an App Runner observability configuration resource. You can delete a specific revision or the latest
* active revision. You can't delete a configuration that's used by one or more App Runner services.
*
*
* @param deleteObservabilityConfigurationRequest
* @return Result of the DeleteObservabilityConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DeleteObservabilityConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteObservabilityConfigurationResponse deleteObservabilityConfiguration(
DeleteObservabilityConfigurationRequest deleteObservabilityConfigurationRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteObservabilityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteObservabilityConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteObservabilityConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteObservabilityConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteObservabilityConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteObservabilityConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteObservabilityConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Delete an App Runner service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
*
* Make sure that you don't have any active VPCIngressConnections associated with the service you want to delete.
*
*
*
* @param deleteServiceRequest
* @return Result of the DeleteService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DeleteService
* @see AWS API
* Documentation
*/
@Override
public DeleteServiceResponse deleteService(DeleteServiceRequest deleteServiceRequest) throws InvalidRequestException,
ResourceNotFoundException, InvalidStateException, InternalServiceErrorException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Delete an App Runner VPC connector resource. You can't delete a connector that's used by one or more App Runner
* services.
*
*
* @param deleteVpcConnectorRequest
* @return Result of the DeleteVpcConnector operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DeleteVpcConnector
* @see AWS
* API Documentation
*/
@Override
public DeleteVpcConnectorResponse deleteVpcConnector(DeleteVpcConnectorRequest deleteVpcConnectorRequest)
throws InvalidRequestException, InternalServiceErrorException, ResourceNotFoundException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVpcConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteVpcConnectorRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVpcConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVpcConnector");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteVpcConnector").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteVpcConnectorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteVpcConnectorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Delete an App Runner VPC Ingress Connection resource that's associated with an App Runner service. The VPC
* Ingress Connection must be in one of the following states to be deleted:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
* -
*
* FAILED_DELETION
*
*
*
*
* @param deleteVpcIngressConnectionRequest
* @return Result of the DeleteVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DeleteVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public DeleteVpcIngressConnectionResponse deleteVpcIngressConnection(
DeleteVpcIngressConnectionRequest deleteVpcIngressConnectionRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVpcIngressConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteVpcIngressConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVpcIngressConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVpcIngressConnection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVpcIngressConnection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteVpcIngressConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteVpcIngressConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a full description of an App Runner automatic scaling configuration resource.
*
*
* @param describeAutoScalingConfigurationRequest
* @return Result of the DescribeAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DescribeAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeAutoScalingConfigurationResponse describeAutoScalingConfiguration(
DescribeAutoScalingConfigurationRequest describeAutoScalingConfigurationRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAutoScalingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAutoScalingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeAutoScalingConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAutoScalingConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAutoScalingConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeAutoScalingConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAutoScalingConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a description of custom domain names that are associated with an App Runner service.
*
*
* @param describeCustomDomainsRequest
* @return Result of the DescribeCustomDomains operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DescribeCustomDomains
* @see AWS API Documentation
*/
@Override
public DescribeCustomDomainsResponse describeCustomDomains(DescribeCustomDomainsRequest describeCustomDomainsRequest)
throws InvalidRequestException, InternalServiceErrorException, ResourceNotFoundException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCustomDomainsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCustomDomainsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCustomDomainsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCustomDomains");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeCustomDomains").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCustomDomainsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCustomDomainsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a full description of an App Runner observability configuration resource.
*
*
* @param describeObservabilityConfigurationRequest
* @return Result of the DescribeObservabilityConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DescribeObservabilityConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeObservabilityConfigurationResponse describeObservabilityConfiguration(
DescribeObservabilityConfigurationRequest describeObservabilityConfigurationRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeObservabilityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeObservabilityConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeObservabilityConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeObservabilityConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeObservabilityConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeObservabilityConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeObservabilityConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a full description of an App Runner service.
*
*
* @param describeServiceRequest
* @return Result of the DescribeService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DescribeService
* @see AWS API
* Documentation
*/
@Override
public DescribeServiceResponse describeService(DescribeServiceRequest describeServiceRequest) throws InvalidRequestException,
ResourceNotFoundException, InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeServiceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a description of an App Runner VPC connector resource.
*
*
* @param describeVpcConnectorRequest
* @return Result of the DescribeVpcConnector operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DescribeVpcConnector
* @see AWS API Documentation
*/
@Override
public DescribeVpcConnectorResponse describeVpcConnector(DescribeVpcConnectorRequest describeVpcConnectorRequest)
throws InvalidRequestException, InternalServiceErrorException, ResourceNotFoundException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeVpcConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeVpcConnectorRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeVpcConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeVpcConnector");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeVpcConnector").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeVpcConnectorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeVpcConnectorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a full description of an App Runner VPC Ingress Connection resource.
*
*
* @param describeVpcIngressConnectionRequest
* @return Result of the DescribeVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DescribeVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public DescribeVpcIngressConnectionResponse describeVpcIngressConnection(
DescribeVpcIngressConnectionRequest describeVpcIngressConnectionRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeVpcIngressConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeVpcIngressConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeVpcIngressConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeVpcIngressConnection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeVpcIngressConnection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeVpcIngressConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeVpcIngressConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociate a custom domain name from an App Runner service.
*
*
* Certificates tracking domain validity are associated with a custom domain and are stored in AWS Certificate Manager (ACM). These certificates
* aren't deleted as part of this action. App Runner delays certificate deletion for 30 days after a domain is
* disassociated from your service.
*
*
* @param disassociateCustomDomainRequest
* @return Result of the DisassociateCustomDomain operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.DisassociateCustomDomain
* @see AWS API Documentation
*/
@Override
public DisassociateCustomDomainResponse disassociateCustomDomain(
DisassociateCustomDomainRequest disassociateCustomDomainRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateCustomDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateCustomDomainRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateCustomDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateCustomDomain");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateCustomDomain").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateCustomDomainRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateCustomDomainRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of active App Runner automatic scaling configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListAutoScalingConfigurations
.
*
*
* @param listAutoScalingConfigurationsRequest
* @return Result of the ListAutoScalingConfigurations operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListAutoScalingConfigurations
* @see AWS API Documentation
*/
@Override
public ListAutoScalingConfigurationsResponse listAutoScalingConfigurations(
ListAutoScalingConfigurationsRequest listAutoScalingConfigurationsRequest) throws InvalidRequestException,
InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAutoScalingConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAutoScalingConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listAutoScalingConfigurationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAutoScalingConfigurations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAutoScalingConfigurations").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listAutoScalingConfigurationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListAutoScalingConfigurationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of App Runner connections that are associated with your Amazon Web Services account.
*
*
* @param listConnectionsRequest
* @return Result of the ListConnections operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListConnections
* @see AWS API
* Documentation
*/
@Override
public ListConnectionsResponse listConnections(ListConnectionsRequest listConnectionsRequest) throws InvalidRequestException,
InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListConnectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listConnectionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConnectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConnections");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListConnections").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listConnectionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListConnectionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of active App Runner observability configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListObservabilityConfigurations
.
*
*
* @param listObservabilityConfigurationsRequest
* @return Result of the ListObservabilityConfigurations operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListObservabilityConfigurations
* @see AWS API Documentation
*/
@Override
public ListObservabilityConfigurationsResponse listObservabilityConfigurations(
ListObservabilityConfigurationsRequest listObservabilityConfigurationsRequest) throws InvalidRequestException,
InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListObservabilityConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listObservabilityConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listObservabilityConfigurationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListObservabilityConfigurations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListObservabilityConfigurations").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listObservabilityConfigurationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListObservabilityConfigurationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a list of operations that occurred on an App Runner service.
*
*
* The resulting list of OperationSummary objects is sorted in reverse chronological order. The first object
* on the list represents the last started operation.
*
*
* @param listOperationsRequest
* @return Result of the ListOperations operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListOperations
* @see AWS API
* Documentation
*/
@Override
public ListOperationsResponse listOperations(ListOperationsRequest listOperationsRequest) throws InvalidRequestException,
InternalServiceErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListOperationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listOperationsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listOperationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListOperations");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListOperations").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listOperationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListOperationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of running App Runner services in your Amazon Web Services account.
*
*
* @param listServicesRequest
* @return Result of the ListServices operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListServices
* @see AWS API
* Documentation
*/
@Override
public ListServicesResponse listServices(ListServicesRequest listServicesRequest) throws InvalidRequestException,
InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListServicesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listServicesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listServicesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListServices");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListServices").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listServicesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListServicesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of the associated App Runner services using an auto scaling configuration.
*
*
* @param listServicesForAutoScalingConfigurationRequest
* @return Result of the ListServicesForAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListServicesForAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public ListServicesForAutoScalingConfigurationResponse listServicesForAutoScalingConfiguration(
ListServicesForAutoScalingConfigurationRequest listServicesForAutoScalingConfigurationRequest)
throws InvalidRequestException, InternalServiceErrorException, ResourceNotFoundException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListServicesForAutoScalingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listServicesForAutoScalingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listServicesForAutoScalingConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListServicesForAutoScalingConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListServicesForAutoScalingConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(listServicesForAutoScalingConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListServicesForAutoScalingConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* List tags that are associated with for an App Runner resource. The response contains a list of tag key-value
* pairs.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ResourceNotFoundException, InternalServiceErrorException, InvalidRequestException, InvalidStateException,
AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of App Runner VPC connectors in your Amazon Web Services account.
*
*
* @param listVpcConnectorsRequest
* @return Result of the ListVpcConnectors operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListVpcConnectors
* @see AWS
* API Documentation
*/
@Override
public ListVpcConnectorsResponse listVpcConnectors(ListVpcConnectorsRequest listVpcConnectorsRequest)
throws InvalidRequestException, InternalServiceErrorException, AwsServiceException, SdkClientException,
AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListVpcConnectorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listVpcConnectorsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listVpcConnectorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListVpcConnectors");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListVpcConnectors").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listVpcConnectorsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListVpcConnectorsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Return a list of App Runner VPC Ingress Connections in your Amazon Web Services account.
*
*
* @param listVpcIngressConnectionsRequest
* @return Result of the ListVpcIngressConnections operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ListVpcIngressConnections
* @see AWS API Documentation
*/
@Override
public ListVpcIngressConnectionsResponse listVpcIngressConnections(
ListVpcIngressConnectionsRequest listVpcIngressConnectionsRequest) throws InvalidRequestException,
InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListVpcIngressConnectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listVpcIngressConnectionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listVpcIngressConnectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListVpcIngressConnections");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListVpcIngressConnections").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listVpcIngressConnectionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListVpcIngressConnectionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Pause an active App Runner service. App Runner reduces compute capacity for the service to zero and loses state
* (for example, ephemeral storage is removed).
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param pauseServiceRequest
* @return Result of the PauseService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.PauseService
* @see AWS API
* Documentation
*/
@Override
public PauseServiceResponse pauseService(PauseServiceRequest pauseServiceRequest) throws InvalidRequestException,
ResourceNotFoundException, InternalServiceErrorException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PauseServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(pauseServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, pauseServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PauseService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PauseService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(pauseServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PauseServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Resume an active App Runner service. App Runner provisions compute capacity for the service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param resumeServiceRequest
* @return Result of the ResumeService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.ResumeService
* @see AWS API
* Documentation
*/
@Override
public ResumeServiceResponse resumeService(ResumeServiceRequest resumeServiceRequest) throws InvalidRequestException,
ResourceNotFoundException, InternalServiceErrorException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ResumeServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(resumeServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, resumeServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ResumeService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ResumeService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(resumeServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ResumeServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Initiate a manual deployment of the latest commit in a source code repository or the latest image in a source
* image repository to an App Runner service.
*
*
* For a source code repository, App Runner retrieves the commit and builds a Docker image. For a source image
* repository, App Runner retrieves the latest Docker image. In both cases, App Runner then deploys the new image to
* your service and starts a new container instance.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param startDeploymentRequest
* @return Result of the StartDeployment operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.StartDeployment
* @see AWS API
* Documentation
*/
@Override
public StartDeploymentResponse startDeployment(StartDeploymentRequest startDeploymentRequest) throws InvalidRequestException,
ResourceNotFoundException, InternalServiceErrorException, AwsServiceException, SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startDeploymentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartDeployment");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartDeployment").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(startDeploymentRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartDeploymentRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Add tags to, or update the tag values of, an App Runner resource. A tag is a key-value pair.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ResourceNotFoundException,
InternalServiceErrorException, InvalidRequestException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(tagResourceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Remove tags from an App Runner resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ResourceNotFoundException,
InternalServiceErrorException, InvalidRequestException, InvalidStateException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Update an auto scaling configuration to be the default. The existing default auto scaling configuration will be
* set to non-default automatically.
*
*
* @param updateDefaultAutoScalingConfigurationRequest
* @return Result of the UpdateDefaultAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.UpdateDefaultAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateDefaultAutoScalingConfigurationResponse updateDefaultAutoScalingConfiguration(
UpdateDefaultAutoScalingConfigurationRequest updateDefaultAutoScalingConfigurationRequest)
throws InvalidRequestException, InternalServiceErrorException, ResourceNotFoundException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, UpdateDefaultAutoScalingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateDefaultAutoScalingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
updateDefaultAutoScalingConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateDefaultAutoScalingConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateDefaultAutoScalingConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(updateDefaultAutoScalingConfigurationRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateDefaultAutoScalingConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Update an App Runner service. You can update the source configuration and instance configuration of the service.
* You can also update the ARN of the auto scaling configuration resource that's associated with the service.
* However, you can't change the name or the encryption configuration of the service. These can be set only when you
* create the service.
*
*
* To update the tags applied to your service, use the separate actions TagResource and UntagResource.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param updateServiceRequest
* @return Result of the UpdateService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.UpdateService
* @see AWS API
* Documentation
*/
@Override
public UpdateServiceResponse updateService(UpdateServiceRequest updateServiceRequest) throws InvalidRequestException,
ResourceNotFoundException, InvalidStateException, InternalServiceErrorException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Update an existing App Runner VPC Ingress Connection resource. The VPC Ingress Connection must be in one of the
* following states to be updated:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
*
*
* @param updateVpcIngressConnectionRequest
* @return Result of the UpdateVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppRunnerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppRunnerClient.UpdateVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public UpdateVpcIngressConnectionResponse updateVpcIngressConnection(
UpdateVpcIngressConnectionRequest updateVpcIngressConnectionRequest) throws InvalidRequestException,
ResourceNotFoundException, InvalidStateException, InternalServiceErrorException, AwsServiceException,
SdkClientException, AppRunnerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateVpcIngressConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateVpcIngressConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateVpcIngressConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppRunner");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateVpcIngressConnection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateVpcIngressConnection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateVpcIngressConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateVpcIngressConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
AppRunnerServiceClientConfigurationBuilder serviceConfigBuilder = new AppRunnerServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(AppRunnerException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.0")
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRequestException")
.exceptionBuilderSupplier(InvalidRequestException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidStateException")
.exceptionBuilderSupplier(InvalidStateException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServiceErrorException")
.exceptionBuilderSupplier(InternalServiceErrorException::builder).httpStatusCode(500).build());
}
@Override
public final AppRunnerServiceClientConfiguration serviceClientConfiguration() {
return new AppRunnerServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}