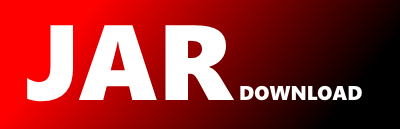
software.amazon.awssdk.services.apprunner.model.ServiceSummary Maven / Gradle / Ivy
Show all versions of apprunner Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.apprunner.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides summary information for an App Runner service.
*
*
* This type contains limited information about a service. It doesn't include configuration details. It's returned by
* the ListServices action.
* Complete service information is returned by the CreateService, DescribeService, and DeleteService actions using the Service type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceName").getter(getter(ServiceSummary::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceName").build()).build();
private static final SdkField SERVICE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceId").getter(getter(ServiceSummary::serviceId)).setter(setter(Builder::serviceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceId").build()).build();
private static final SdkField SERVICE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceArn").getter(getter(ServiceSummary::serviceArn)).setter(setter(Builder::serviceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceArn").build()).build();
private static final SdkField SERVICE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceUrl").getter(getter(ServiceSummary::serviceUrl)).setter(setter(Builder::serviceUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUrl").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedAt").getter(getter(ServiceSummary::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("UpdatedAt").getter(getter(ServiceSummary::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedAt").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ServiceSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_NAME_FIELD,
SERVICE_ID_FIELD, SERVICE_ARN_FIELD, SERVICE_URL_FIELD, CREATED_AT_FIELD, UPDATED_AT_FIELD, STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ServiceName", SERVICE_NAME_FIELD);
put("ServiceId", SERVICE_ID_FIELD);
put("ServiceArn", SERVICE_ARN_FIELD);
put("ServiceUrl", SERVICE_URL_FIELD);
put("CreatedAt", CREATED_AT_FIELD);
put("UpdatedAt", UPDATED_AT_FIELD);
put("Status", STATUS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String serviceName;
private final String serviceId;
private final String serviceArn;
private final String serviceUrl;
private final Instant createdAt;
private final Instant updatedAt;
private final String status;
private ServiceSummary(BuilderImpl builder) {
this.serviceName = builder.serviceName;
this.serviceId = builder.serviceId;
this.serviceArn = builder.serviceArn;
this.serviceUrl = builder.serviceUrl;
this.createdAt = builder.createdAt;
this.updatedAt = builder.updatedAt;
this.status = builder.status;
}
/**
*
* The customer-provided service name.
*
*
* @return The customer-provided service name.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* An ID that App Runner generated for this service. It's unique within the Amazon Web Services Region.
*
*
* @return An ID that App Runner generated for this service. It's unique within the Amazon Web Services Region.
*/
public final String serviceId() {
return serviceId;
}
/**
*
* The Amazon Resource Name (ARN) of this service.
*
*
* @return The Amazon Resource Name (ARN) of this service.
*/
public final String serviceArn() {
return serviceArn;
}
/**
*
* A subdomain URL that App Runner generated for this service. You can use this URL to access your service web
* application.
*
*
* @return A subdomain URL that App Runner generated for this service. You can use this URL to access your service
* web application.
*/
public final String serviceUrl() {
return serviceUrl;
}
/**
*
* The time when the App Runner service was created. It's in the Unix time stamp format.
*
*
* @return The time when the App Runner service was created. It's in the Unix time stamp format.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The time when the App Runner service was last updated. It's in theUnix time stamp format.
*
*
* @return The time when the App Runner service was last updated. It's in theUnix time stamp format.
*/
public final Instant updatedAt() {
return updatedAt;
}
/**
*
* The current state of the App Runner service. These particular values mean the following.
*
*
* -
*
* CREATE_FAILED
– The service failed to create. The failed service isn't usable, and still counts
* towards your service quota. To troubleshoot this failure, read the failure events and logs, change any parameters
* that need to be fixed, and rebuild your service using UpdateService
.
*
*
* -
*
* DELETE_FAILED
– The service failed to delete and can't be successfully recovered. Retry the service
* deletion call to ensure that all related resources are removed.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ServiceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current state of the App Runner service. These particular values mean the following.
*
* -
*
* CREATE_FAILED
– The service failed to create. The failed service isn't usable, and still
* counts towards your service quota. To troubleshoot this failure, read the failure events and logs, change
* any parameters that need to be fixed, and rebuild your service using UpdateService
.
*
*
* -
*
* DELETE_FAILED
– The service failed to delete and can't be successfully recovered. Retry the
* service deletion call to ensure that all related resources are removed.
*
*
* @see ServiceStatus
*/
public final ServiceStatus status() {
return ServiceStatus.fromValue(status);
}
/**
*
* The current state of the App Runner service. These particular values mean the following.
*
*
* -
*
* CREATE_FAILED
– The service failed to create. The failed service isn't usable, and still counts
* towards your service quota. To troubleshoot this failure, read the failure events and logs, change any parameters
* that need to be fixed, and rebuild your service using UpdateService
.
*
*
* -
*
* DELETE_FAILED
– The service failed to delete and can't be successfully recovered. Retry the service
* deletion call to ensure that all related resources are removed.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ServiceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current state of the App Runner service. These particular values mean the following.
*
* -
*
* CREATE_FAILED
– The service failed to create. The failed service isn't usable, and still
* counts towards your service quota. To troubleshoot this failure, read the failure events and logs, change
* any parameters that need to be fixed, and rebuild your service using UpdateService
.
*
*
* -
*
* DELETE_FAILED
– The service failed to delete and can't be successfully recovered. Retry the
* service deletion call to ensure that all related resources are removed.
*
*
* @see ServiceStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(serviceId());
hashCode = 31 * hashCode + Objects.hashCode(serviceArn());
hashCode = 31 * hashCode + Objects.hashCode(serviceUrl());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceSummary)) {
return false;
}
ServiceSummary other = (ServiceSummary) obj;
return Objects.equals(serviceName(), other.serviceName()) && Objects.equals(serviceId(), other.serviceId())
&& Objects.equals(serviceArn(), other.serviceArn()) && Objects.equals(serviceUrl(), other.serviceUrl())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(updatedAt(), other.updatedAt())
&& Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceSummary").add("ServiceName", serviceName()).add("ServiceId", serviceId())
.add("ServiceArn", serviceArn()).add("ServiceUrl", serviceUrl()).add("CreatedAt", createdAt())
.add("UpdatedAt", updatedAt()).add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ServiceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "ServiceId":
return Optional.ofNullable(clazz.cast(serviceId()));
case "ServiceArn":
return Optional.ofNullable(clazz.cast(serviceArn()));
case "ServiceUrl":
return Optional.ofNullable(clazz.cast(serviceUrl()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "UpdatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function